diff --git a/.github/FUNDING.yml b/.github/FUNDING.yml
index 5d3d358..e95d6e9 100644
--- a/.github/FUNDING.yml
+++ b/.github/FUNDING.yml
@@ -1 +1 @@
-github: [mrousavy]
+github: [mrousavy, chrispader]
diff --git a/.github/workflows/build-android-release.yml b/.github/workflows/build-android-release.yml
index 75dc9e1..327f659 100644
--- a/.github/workflows/build-android-release.yml
+++ b/.github/workflows/build-android-release.yml
@@ -41,7 +41,7 @@ jobs:
with:
upload_url: ${{ github.event.release.upload_url }}
asset_path: example/android/app/build/outputs/apk/release/app-release.apk
- asset_name: "QuickSQLiteExample-${{ github.event.release.tag_name }}.apk"
+ asset_name: "NitroSQLiteExample-${{ github.event.release.tag_name }}.apk"
asset_content_type: application/vnd.android.package-archive
# Gradle cache doesn't like daemons
diff --git a/.github/workflows/build-ios.yml b/.github/workflows/build-ios.yml
index 2fcdf65..32ab449 100644
--- a/.github/workflows/build-ios.yml
+++ b/.github/workflows/build-ios.yml
@@ -67,8 +67,8 @@ jobs:
run: "set -o pipefail && xcodebuild \
CC=clang CPLUSPLUS=clang++ LD=clang LDPLUSPLUS=clang++ \
-derivedDataPath build -UseModernBuildSystem=YES \
- -workspace QuickSQLiteExample.xcworkspace \
- -scheme QuickSQLiteExample \
+ -workspace NitroSQLiteExample.xcworkspace \
+ -scheme NitroSQLiteExample \
-sdk iphonesimulator \
-configuration Debug \
-destination 'platform=iOS Simulator,name=iPhone 16' \
@@ -114,8 +114,8 @@ jobs:
run: "set -o pipefail && xcodebuild \
CC=clang CPLUSPLUS=clang++ LD=clang LDPLUSPLUS=clang++ \
-derivedDataPath build -UseModernBuildSystem=YES \
- -workspace QuickSQLiteExample.xcworkspace \
- -scheme QuickSQLiteExample \
+ -workspace NitroSQLiteExample.xcworkspace \
+ -scheme NitroSQLiteExample \
-sdk iphonesimulator \
-configuration Debug \
-destination 'platform=iOS Simulator,name=iPhone 16' \
diff --git a/.github/workflows/lint-typescript.yml b/.github/workflows/lint-typescript.yml
index 8f9efa6..20f5ea3 100644
--- a/.github/workflows/lint-typescript.yml
+++ b/.github/workflows/lint-typescript.yml
@@ -63,14 +63,14 @@ jobs:
- name: Run ESLint CI in example/
working-directory: example
run: bun lint-ci
- - name: Run ESLint CI in react-native-quick-sqlite
+ - name: Run ESLint CI in react-native-nitro-sqlite
working-directory: package
run: bun lint-ci
- name: Run ESLint with auto-fix in example/
working-directory: example
run: bun lint
- - name: Run ESLint with auto-fix in react-native-quick-sqlite
+ - name: Run ESLint with auto-fix in react-native-nitro-sqlite
working-directory: package
run: bun lint
diff --git a/CONTRIBUTING.md b/CONTRIBUTING.md
index e302aa1..af64350 100644
--- a/CONTRIBUTING.md
+++ b/CONTRIBUTING.md
@@ -49,9 +49,9 @@ Remember to add tests for your change if possible. Run the unit tests by:
bun test
```
-To edit the Objective-C files, open `example/ios/SequelExample.xcworkspace` in XCode and find the source files at `Pods > Development Pods > react-native-quick-sqlite`.
+To edit the Objective-C files, open `example/ios/SequelExample.xcworkspace` in XCode and find the source files at `Pods > Development Pods > react-native-nitro-sqlite`.
-To edit the Kotlin files, open `example/android` in Android studio and find the source files at `rnquicksqlite` under `Android`.
+To edit the Kotlin files, open `example/android` in Android studio and find the source files at `rnnitrosqlite` under `Android`.
### Commit message convention
diff --git a/README.md b/README.md
index b60f911..887febe 100644
--- a/README.md
+++ b/README.md
@@ -1,8 +1,8 @@
-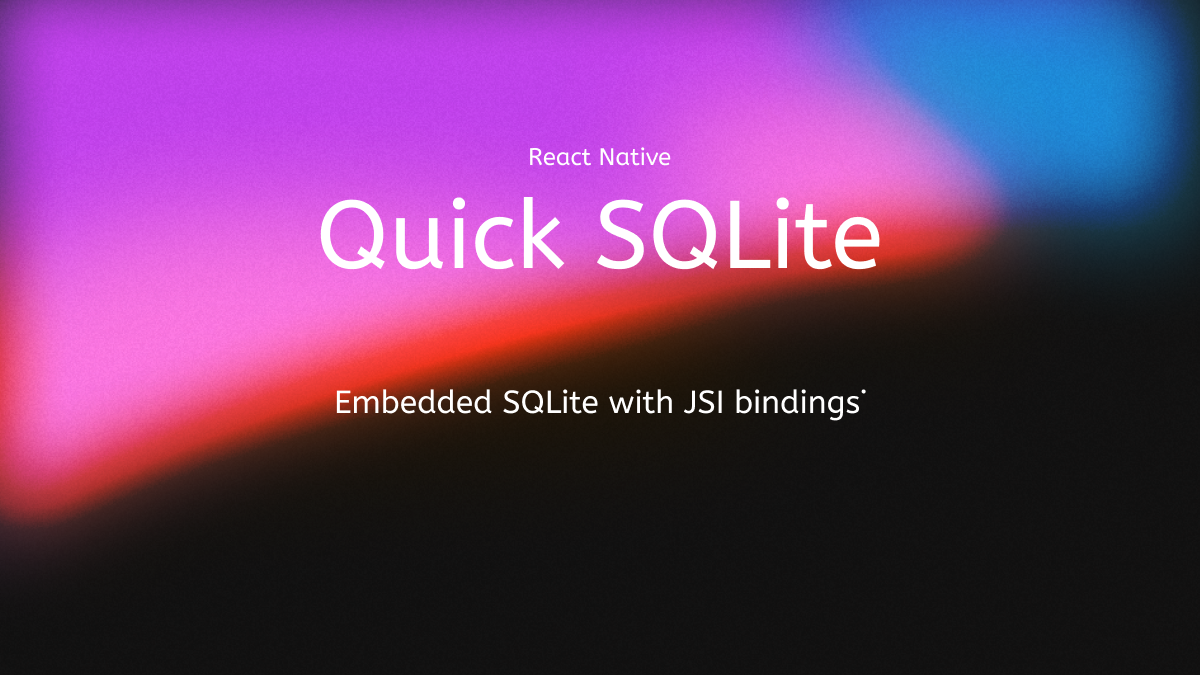
+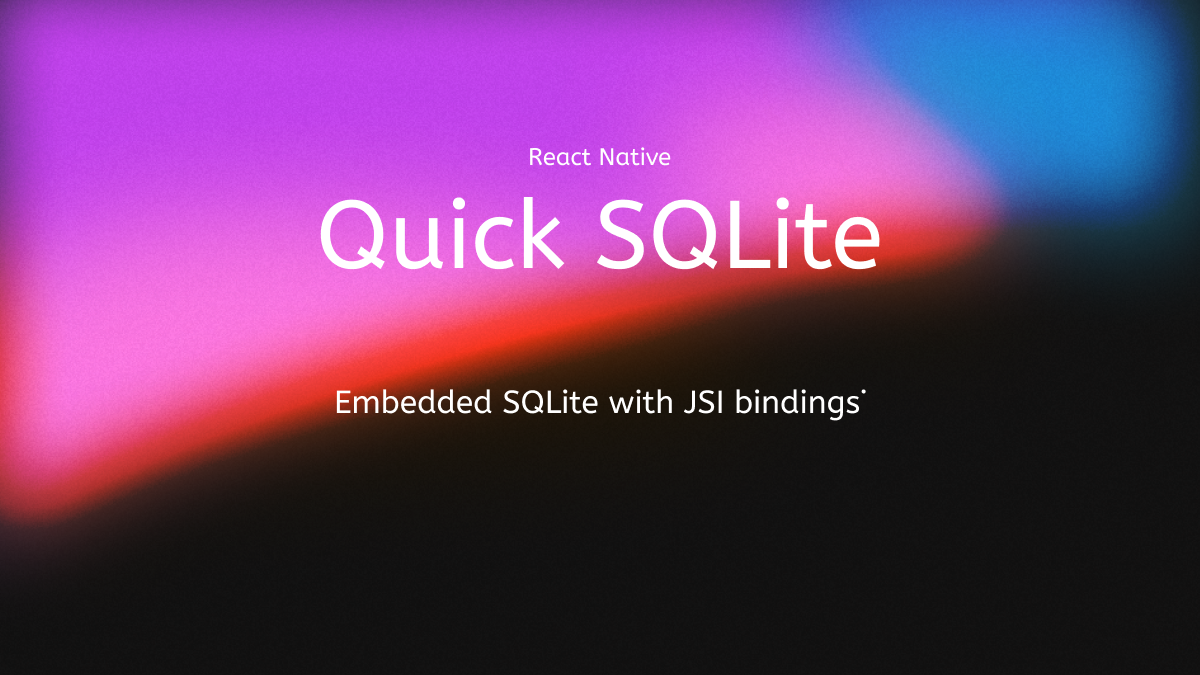
- bun add react-native-quick-sqlite
+ bun add react-native-nitro-sqlite
npx pod-install
@@ -14,7 +14,7 @@
-Quick SQLite embeds the latest version of SQLite and provides a low-level JSI-backed API to execute SQL queries.
+Nitro SQLite embeds the latest version of SQLite and provides a low-level JSI-backed API to execute SQL queries.
Performance metrics are intentionally not presented, [anecdotic testimonies](https://dev.to/craftzdog/a-performant-way-to-use-pouchdb7-on-react-native-in-2022-24ej) suggest anywhere between 2x and 5x speed improvement. On small queries you might not notice a difference with the old bridge but as you send large data to JS the speed increase is considerable.
@@ -25,7 +25,7 @@ TypeORM is officially supported, however, there is currently a parsing issue wit
## API
```typescript
-import {open} from 'react-native-quick-sqlite'
+import {open} from 'react-native-nitro-sqlite'
const db = open('myDb.sqlite')
@@ -54,7 +54,7 @@ db = {
The basic query is **synchronous**, it will block rendering on large operations, further below you will find async versions.
```typescript
-import { open } from 'react-native-quick-sqlite';
+import { open } from 'react-native-nitro-sqlite';
try {
const db = open('myDb.sqlite');
@@ -83,7 +83,7 @@ Throwing an error inside the callback will ROLLBACK the transaction.
If you want to execute a large set of commands as fast as possible you should use the `executeBatch` method, it wraps all the commands in a transaction and has less overhead.
```typescript
-await QuickSQLite.transaction('myDatabase', (tx) => {
+await NitroSQLite.transaction('myDatabase', (tx) => {
const { status } = tx.execute(
'UPDATE sometable SET somecolumn = ? where somekey = ?',
[0, 1]
@@ -117,7 +117,7 @@ const commands = [
[('INSERT INTO TEST (id) VALUES (?)', [[3], [4], [5], [6]])],
];
-const res = QuickSQLite.executeSqlBatch('myDatabase', commands);
+const res = NitroSQLite.executeSqlBatch('myDatabase', commands);
console.log(`Batch affected ${result.rowsAffected} rows`);
```
@@ -130,7 +130,7 @@ This can be done by testing the returned data directly, but in some cases may no
SQLite datatypes. When fetching data directly from tables or views linked to table columns, SQLite can identify the table declared types:
```typescript
-let { metadata } = QuickSQLite.executeSql(
+let { metadata } = NitroSQLite.executeSql(
'myDatabase',
'SELECT int_column_1, bol_column_2 FROM sometable'
);
@@ -148,7 +148,7 @@ metadata.forEach((column) => {
You might have too much SQL to process and it will cause your application to freeze. There are async versions for some of the operations. This will offload the SQLite processing to a different thread.
```ts
-QuickSQLite.executeAsync(
+NitroSQLite.executeAsync(
'myDatabase',
'SELECT * FROM "User";',
[]).then(({rows}) => {
@@ -170,15 +170,15 @@ SQLite has a limit for attached databases: A default of 10, and a global max of
References: [Attach](https://www.sqlite.org/lang_attach.html) - [Detach](https://www.sqlite.org/lang_detach.html)
```ts
-QuickSQLite.attach('mainDatabase', 'statistics', 'stats', '../databases');
+NitroSQLite.attach('mainDatabase', 'statistics', 'stats', '../databases');
-const res = QuickSQLite.executeSql(
+const res = NitroSQLite.executeSql(
'mainDatabase',
'SELECT * FROM some_table_from_mainschema a INNER JOIN stats.some_table b on a.id_column = b.id_column'
);
// You can detach databases at any moment
-QuickSQLite.detach('mainDatabase', 'stats');
+NitroSQLite.detach('mainDatabase', 'stats');
if (!detachResult.status) {
// Database de-attached
}
@@ -189,7 +189,7 @@ if (!detachResult.status) {
If you have a plain SQL file, you can load it directly, with low memory consumption.
```typescript
-const { rowsAffected, commands } = QuickSQLite.loadFile(
+const { rowsAffected, commands } = NitroSQLite.loadFile(
'myDatabase',
'/absolute/path/to/file.sql'
);
@@ -198,7 +198,7 @@ const { rowsAffected, commands } = QuickSQLite.loadFile(
Or use the async version which will load the file in another native thread
```typescript
-QuickSQLite.loadFileAsync('myDatabase', '/absolute/path/to/file.sql').then(
+NitroSQLite.loadFileAsync('myDatabase', '/absolute/path/to/file.sql').then(
(res) => {
const { rowsAffected, commands } = res;
}
@@ -210,7 +210,7 @@ QuickSQLite.loadFileAsync('myDatabase', '/absolute/path/to/file.sql').then(
On iOS you can use the embedded SQLite, when running `pod-install` add an environment flag:
```
-QUICK_SQLITE_USE_PHONE_VERSION=1 npx pod-install
+Nitro_SQLITE_USE_PHONE_VERSION=1 npx pod-install
```
On Android, it is not possible to link (using C++) the embedded SQLite. It is also a bad idea due to vendor changes, old android bugs, etc. Unfortunately, this means this library will add some megabytes to your app size.
@@ -240,7 +240,7 @@ bun patch-package --exclude 'nothing' typeorm
Now every time you install your node_modules that line will be added.
-Next, we need to trick TypeORM to resolve the dependency of `react-native-sqlite-storage` to `react-native-quick-sqlite`, on your `babel.config.js` add the following:
+Next, we need to trick TypeORM to resolve the dependency of `react-native-sqlite-storage` to `react-native-nitro-sqlite`, on your `babel.config.js` add the following:
```js
plugins: [
@@ -250,7 +250,7 @@ plugins: [
'module-resolver',
{
alias: {
- "react-native-sqlite-storage": "react-native-quick-sqlite"
+ "react-native-sqlite-storage": "react-native-nitro-sqlite"
},
},
],
@@ -266,7 +266,7 @@ bun add babel-plugin-module-resolver
Finally, you will now be able to start the app without any metro/babel errors (you will also need to follow the instructions on how to setup TypeORM), now we can feed the driver into TypeORM:
```ts
-import { typeORMDriver } from 'react-native-quick-sqlite'
+import { typeORMDriver } from 'react-native-nitro-sqlite'
datasource = new DataSource({
type: 'react-native',
@@ -280,7 +280,7 @@ datasource = new DataSource({
# Loading existing DBs
-The library creates/opens databases by appending the passed name plus, the [documents directory on iOS](https://github.com/margelo/react-native-quick-sqlite/blob/733e876d98896f5efc80f989ae38120f16533a66/ios/QuickSQLite.mm#L34-L35) and the [files directory on Android](https://github.com/margelo/react-native-quick-sqlite/blob/main/android/src/main/java/com/margelo/rnquicksqlite/QuickSQLiteBridge.java#L16), this differs from other SQL libraries (some place it in a `www` folder, some in androids `databases` folder, etc.).
+The library creates/opens databases by appending the passed name plus, the [documents directory on iOS](https://github.com/margelo/react-native-nitro-sqlite/blob/733e876d98896f5efc80f989ae38120f16533a66/ios/NitroSQLite.mm#L34-L35) and the [files directory on Android](https://github.com/margelo/react-native-nitro-sqlite/blob/main/android/src/main/java/com/margelo/rnnitrosqlite/NitroSQLiteBridge.java#L16), this differs from other SQL libraries (some place it in a `www` folder, some in androids `databases` folder, etc.).
If you have an existing database file you want to load you can navigate from these directories using dot notation. e.g. `../www/myDb.sqlite`. Note that on iOS the file system is sand-boxed, so you cannot access files/directories outside your app bundle directories.
@@ -297,7 +297,7 @@ Add a `post_install` block to your `/ios/Podfile` like so:
```ruby
post_install do |installer|
installer.pods_project.targets.each do |target|
- if target.name == "react-native-quick-sqlite" then
+ if target.name == "react-native-nitro-sqlite" then
target.build_configurations.each do |config|
config.build_settings['GCC_PREPROCESSOR_DEFINITIONS'] << 'SQLITE_ENABLE_FTS5=1'
end
@@ -314,7 +314,7 @@ For example, you could add `SQLITE_ENABLE_FTS5=1` to `GCC_PREPROCESSOR_DEFINITIO
You can specify flags via `/android/gradle.properties` like so:
```
-quickSqliteFlags=""
+nitroSqliteFlags=""
```
## Additional configuration
@@ -323,15 +323,15 @@ quickSqliteFlags=""
On iOS, the SQLite database can be placed in an app group, in order to make it accessible from other apps in that app group. E.g. for sharing capabilities.
-To use an app group, add the app group ID as the value for the `RNQuickSQLite_AppGroup` key in your project's `Info.plist` file. You'll also need to configure the app group in your project settings. (Xcode -> Project Settings -> Signing & Capabilities -> Add Capability -> App Groups)
+To use an app group, add the app group ID as the value for the `RNNitroSQLite_AppGroup` key in your project's `Info.plist` file. You'll also need to configure the app group in your project settings. (Xcode -> Project Settings -> Signing & Capabilities -> Add Capability -> App Groups)
## Community Discord
-[Join the Margelo Community Discord](https://discord.gg/6CSHz2qAvA) to chat about react-native-quick-sqlite or other Margelo libraries.
+[Join the Margelo Community Discord](https://discord.gg/6CSHz2qAvA) to chat about react-native-nitro-sqlite or other Margelo libraries.
## Oscar
-react-native-quick-sqlite was originally created by [Oscar Franco](https://github.com/ospfranco). Thanks Oscar!
+react-native-nitro-sqlite was originally created by [Oscar Franco](https://github.com/ospfranco). Thanks Oscar!
## License
diff --git a/bun.lockb b/bun.lockb
index a30a08c..a4ec91f 100755
Binary files a/bun.lockb and b/bun.lockb differ
diff --git a/example/android/app/build.gradle b/example/android/app/build.gradle
index 4cd46cc..0bf9003 100644
--- a/example/android/app/build.gradle
+++ b/example/android/app/build.gradle
@@ -77,9 +77,9 @@ android {
buildToolsVersion rootProject.ext.buildToolsVersion
compileSdk rootProject.ext.compileSdkVersion
- namespace "com.margelo.rnquicksqlite.example"
+ namespace "com.margelo.rnnitrosqlite.example"
defaultConfig {
- applicationId "com.margelo.rnquicksqlite.example"
+ applicationId "com.margelo.rnnitrosqlite.example"
minSdkVersion rootProject.ext.minSdkVersion
targetSdkVersion rootProject.ext.targetSdkVersion
versionCode 1
diff --git a/example/android/app/src/main/java/com/margelo/rnquicksqlite/example/MainActivity.kt b/example/android/app/src/main/java/com/margelo/rnnitrosqlite/example/MainActivity.kt
similarity index 87%
rename from example/android/app/src/main/java/com/margelo/rnquicksqlite/example/MainActivity.kt
rename to example/android/app/src/main/java/com/margelo/rnnitrosqlite/example/MainActivity.kt
index 91e4e7a..9fbce32 100644
--- a/example/android/app/src/main/java/com/margelo/rnquicksqlite/example/MainActivity.kt
+++ b/example/android/app/src/main/java/com/margelo/rnnitrosqlite/example/MainActivity.kt
@@ -1,4 +1,4 @@
-package com.margelo.rnquicksqlite.example
+package com.margelo.rnnitrosqlite.example
import com.facebook.react.ReactActivity
import com.facebook.react.ReactActivityDelegate
@@ -11,7 +11,7 @@ class MainActivity : ReactActivity() {
* Returns the name of the main component registered from JavaScript. This is used to schedule
* rendering of the component.
*/
- override fun getMainComponentName(): String = "QuickSQLiteExample"
+ override fun getMainComponentName(): String = "NitroSQLiteExample"
/**
* Returns the instance of the [ReactActivityDelegate]. We use [DefaultReactActivityDelegate]
diff --git a/example/android/app/src/main/java/com/margelo/rnquicksqlite/example/MainApplication.kt b/example/android/app/src/main/java/com/margelo/rnnitrosqlite/example/MainApplication.kt
similarity index 97%
rename from example/android/app/src/main/java/com/margelo/rnquicksqlite/example/MainApplication.kt
rename to example/android/app/src/main/java/com/margelo/rnnitrosqlite/example/MainApplication.kt
index 47f0c2c..07309b0 100644
--- a/example/android/app/src/main/java/com/margelo/rnquicksqlite/example/MainApplication.kt
+++ b/example/android/app/src/main/java/com/margelo/rnnitrosqlite/example/MainApplication.kt
@@ -1,4 +1,4 @@
-package com.margelo.rnquicksqlite.example;
+package com.margelo.rnnitrosqlite.example;
import android.app.Application
import com.facebook.react.PackageList
diff --git a/example/android/app/src/main/res/values/strings.xml b/example/android/app/src/main/res/values/strings.xml
index 5ed0fc9..97b98ed 100644
--- a/example/android/app/src/main/res/values/strings.xml
+++ b/example/android/app/src/main/res/values/strings.xml
@@ -1,3 +1,3 @@
- QuickSQLiteExample
+ NitroSQLiteExample
diff --git a/example/android/settings.gradle b/example/android/settings.gradle
index e566e76..f5671d5 100644
--- a/example/android/settings.gradle
+++ b/example/android/settings.gradle
@@ -1,6 +1,6 @@
pluginManagement { includeBuild("../../node_modules/@react-native/gradle-plugin") }
plugins { id("com.facebook.react.settings") }
extensions.configure(com.facebook.react.ReactSettingsExtension){ ex -> ex.autolinkLibrariesFromCommand() }
-rootProject.name = 'QuickSQLiteExample'
+rootProject.name = 'NitroSQLiteExample'
include ':app'
includeBuild('../../node_modules/@react-native/gradle-plugin')
diff --git a/example/app.json b/example/app.json
index 4bcf88c..39aa826 100644
--- a/example/app.json
+++ b/example/app.json
@@ -1,10 +1,10 @@
{
- "name": "QuickSQLiteExample",
- "displayName": "QuickSQLite Example",
+ "name": "NitroSQLiteExample",
+ "displayName": "NitroSQLite Example",
"components": [
{
- "appKey": "QuickSQLiteExample",
- "displayName": "QuickSQLite Example"
+ "appKey": "NitroSQLiteExample",
+ "displayName": "NitroSQLite Example"
}
],
"resources": {
@@ -15,9 +15,9 @@
"windows": ["dist/assets", "dist/main.windows.bundle"]
},
"android": {
- "package": "com.margelo.rnquicksqlite.example"
+ "package": "com.margelo.rnnitrosqlite.example"
},
"ios": {
- "bundleIdentifier": "com.margelo.rnquicksqlite.example"
+ "bundleIdentifier": "com.margelo.rnnitrosqlite.example"
}
}
diff --git a/example/babel.config.js b/example/babel.config.js
index 4bad783..3ca7eba 100644
--- a/example/babel.config.js
+++ b/example/babel.config.js
@@ -11,7 +11,7 @@ module.exports = {
alias: {
[pak.name]: path.join(__dirname, '../package', pak.source),
stream: 'stream-browserify',
- 'react-native-sqlite-storage': 'react-native-quick-sqlite',
+ 'react-native-sqlite-storage': 'react-native-nitro-sqlite',
},
},
],
diff --git a/example/ios/QuickSQLiteExample.xcodeproj/project.pbxproj b/example/ios/NitroSQLiteExample.xcodeproj/project.pbxproj
similarity index 78%
rename from example/ios/QuickSQLiteExample.xcodeproj/project.pbxproj
rename to example/ios/NitroSQLiteExample.xcodeproj/project.pbxproj
index 37eb6ee..d145531 100644
--- a/example/ios/QuickSQLiteExample.xcodeproj/project.pbxproj
+++ b/example/ios/NitroSQLiteExample.xcodeproj/project.pbxproj
@@ -7,14 +7,14 @@
objects = {
/* Begin PBXBuildFile section */
- 00E356F31AD99517003FC87E /* QuickSQLiteExampleTests.m in Sources */ = {isa = PBXBuildFile; fileRef = 00E356F21AD99517003FC87E /* QuickSQLiteExampleTests.m */; };
+ 00E356F31AD99517003FC87E /* NitroSQLiteExampleTests.m in Sources */ = {isa = PBXBuildFile; fileRef = 00E356F21AD99517003FC87E /* NitroSQLiteExampleTests.m */; };
13B07FBC1A68108700A75B9A /* AppDelegate.mm in Sources */ = {isa = PBXBuildFile; fileRef = 13B07FB01A68108700A75B9A /* AppDelegate.mm */; };
13B07FBF1A68108700A75B9A /* Images.xcassets in Resources */ = {isa = PBXBuildFile; fileRef = 13B07FB51A68108700A75B9A /* Images.xcassets */; };
13B07FC11A68108700A75B9A /* main.m in Sources */ = {isa = PBXBuildFile; fileRef = 13B07FB71A68108700A75B9A /* main.m */; };
17EA225CB5C6FBE4FF95B3B8 /* PrivacyInfo.xcprivacy in Resources */ = {isa = PBXBuildFile; fileRef = B62F4C3A8445C44CF7F54399 /* PrivacyInfo.xcprivacy */; };
81AB9BB82411601600AC10FF /* LaunchScreen.storyboard in Resources */ = {isa = PBXBuildFile; fileRef = 81AB9BB72411601600AC10FF /* LaunchScreen.storyboard */; };
- D7537A007033719516C57748 /* libPods-QuickSQLiteExample-QuickSQLiteExampleTests.a in Frameworks */ = {isa = PBXBuildFile; fileRef = F61ADDCA341B787B4A3AFAFC /* libPods-QuickSQLiteExample-QuickSQLiteExampleTests.a */; };
- F6FA2C48029804D08B79E96F /* libPods-QuickSQLiteExample.a in Frameworks */ = {isa = PBXBuildFile; fileRef = BCC1E776B313FBFED7863A3D /* libPods-QuickSQLiteExample.a */; };
+ D7537A007033719516C57748 /* libPods-NitroSQLiteExample-NitroSQLiteExampleTests.a in Frameworks */ = {isa = PBXBuildFile; fileRef = F61ADDCA341B787B4A3AFAFC /* libPods-NitroSQLiteExample-NitroSQLiteExampleTests.a */; };
+ F6FA2C48029804D08B79E96F /* libPods-NitroSQLiteExample.a in Frameworks */ = {isa = PBXBuildFile; fileRef = BCC1E776B313FBFED7863A3D /* libPods-NitroSQLiteExample.a */; };
/* End PBXBuildFile section */
/* Begin PBXContainerItemProxy section */
@@ -23,29 +23,29 @@
containerPortal = 83CBB9F71A601CBA00E9B192 /* Project object */;
proxyType = 1;
remoteGlobalIDString = 13B07F861A680F5B00A75B9A;
- remoteInfo = QuickSQLiteExample;
+ remoteInfo = NitroSQLiteExample;
};
/* End PBXContainerItemProxy section */
/* Begin PBXFileReference section */
- 00E356EE1AD99517003FC87E /* QuickSQLiteExampleTests.xctest */ = {isa = PBXFileReference; explicitFileType = wrapper.cfbundle; includeInIndex = 0; path = QuickSQLiteExampleTests.xctest; sourceTree = BUILT_PRODUCTS_DIR; };
+ 00E356EE1AD99517003FC87E /* NitroSQLiteExampleTests.xctest */ = {isa = PBXFileReference; explicitFileType = wrapper.cfbundle; includeInIndex = 0; path = NitroSQLiteExampleTests.xctest; sourceTree = BUILT_PRODUCTS_DIR; };
00E356F11AD99517003FC87E /* Info.plist */ = {isa = PBXFileReference; lastKnownFileType = text.plist.xml; path = Info.plist; sourceTree = ""; };
- 00E356F21AD99517003FC87E /* QuickSQLiteExampleTests.m */ = {isa = PBXFileReference; lastKnownFileType = sourcecode.c.objc; path = QuickSQLiteExampleTests.m; sourceTree = ""; };
- 03D39CB013C879B46EC1C979 /* Pods-QuickSQLiteExample.release.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; name = "Pods-QuickSQLiteExample.release.xcconfig"; path = "Target Support Files/Pods-QuickSQLiteExample/Pods-QuickSQLiteExample.release.xcconfig"; sourceTree = ""; };
- 1251E54A0A604DFB2905E20E /* Pods-QuickSQLiteExample-QuickSQLiteExampleTests.debug.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; name = "Pods-QuickSQLiteExample-QuickSQLiteExampleTests.debug.xcconfig"; path = "Target Support Files/Pods-QuickSQLiteExample-QuickSQLiteExampleTests/Pods-QuickSQLiteExample-QuickSQLiteExampleTests.debug.xcconfig"; sourceTree = ""; };
- 13B07F961A680F5B00A75B9A /* QuickSQLiteExample.app */ = {isa = PBXFileReference; explicitFileType = wrapper.application; includeInIndex = 0; path = QuickSQLiteExample.app; sourceTree = BUILT_PRODUCTS_DIR; };
- 13B07FAF1A68108700A75B9A /* AppDelegate.h */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.c.h; name = AppDelegate.h; path = QuickSQLiteExample/AppDelegate.h; sourceTree = ""; };
- 13B07FB01A68108700A75B9A /* AppDelegate.mm */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.cpp.objcpp; name = AppDelegate.mm; path = QuickSQLiteExample/AppDelegate.mm; sourceTree = ""; };
- 13B07FB51A68108700A75B9A /* Images.xcassets */ = {isa = PBXFileReference; lastKnownFileType = folder.assetcatalog; name = Images.xcassets; path = QuickSQLiteExample/Images.xcassets; sourceTree = ""; };
- 13B07FB61A68108700A75B9A /* Info.plist */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = text.plist.xml; name = Info.plist; path = QuickSQLiteExample/Info.plist; sourceTree = ""; };
- 13B07FB71A68108700A75B9A /* main.m */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.c.objc; name = main.m; path = QuickSQLiteExample/main.m; sourceTree = ""; };
- 81AB9BB72411601600AC10FF /* LaunchScreen.storyboard */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = file.storyboard; name = LaunchScreen.storyboard; path = QuickSQLiteExample/LaunchScreen.storyboard; sourceTree = ""; };
- B62F4C3A8445C44CF7F54399 /* PrivacyInfo.xcprivacy */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xml; name = PrivacyInfo.xcprivacy; path = QuickSQLiteExample/PrivacyInfo.xcprivacy; sourceTree = ""; };
- BCC1E776B313FBFED7863A3D /* libPods-QuickSQLiteExample.a */ = {isa = PBXFileReference; explicitFileType = archive.ar; includeInIndex = 0; path = "libPods-QuickSQLiteExample.a"; sourceTree = BUILT_PRODUCTS_DIR; };
- CA20110A9117BB91D9E63081 /* Pods-QuickSQLiteExample.debug.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; name = "Pods-QuickSQLiteExample.debug.xcconfig"; path = "Target Support Files/Pods-QuickSQLiteExample/Pods-QuickSQLiteExample.debug.xcconfig"; sourceTree = ""; };
- CE317F99C7B7933DEB58C2E6 /* Pods-QuickSQLiteExample-QuickSQLiteExampleTests.release.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; name = "Pods-QuickSQLiteExample-QuickSQLiteExampleTests.release.xcconfig"; path = "Target Support Files/Pods-QuickSQLiteExample-QuickSQLiteExampleTests/Pods-QuickSQLiteExample-QuickSQLiteExampleTests.release.xcconfig"; sourceTree = ""; };
+ 00E356F21AD99517003FC87E /* NitroSQLiteExampleTests.m */ = {isa = PBXFileReference; lastKnownFileType = sourcecode.c.objc; path = NitroSQLiteExampleTests.m; sourceTree = ""; };
+ 03D39CB013C879B46EC1C979 /* Pods-NitroSQLiteExample.release.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; name = "Pods-NitroSQLiteExample.release.xcconfig"; path = "Target Support Files/Pods-NitroSQLiteExample/Pods-NitroSQLiteExample.release.xcconfig"; sourceTree = ""; };
+ 1251E54A0A604DFB2905E20E /* Pods-NitroSQLiteExample-NitroSQLiteExampleTests.debug.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; name = "Pods-NitroSQLiteExample-NitroSQLiteExampleTests.debug.xcconfig"; path = "Target Support Files/Pods-NitroSQLiteExample-NitroSQLiteExampleTests/Pods-NitroSQLiteExample-NitroSQLiteExampleTests.debug.xcconfig"; sourceTree = ""; };
+ 13B07F961A680F5B00A75B9A /* NitroSQLiteExample.app */ = {isa = PBXFileReference; explicitFileType = wrapper.application; includeInIndex = 0; path = NitroSQLiteExample.app; sourceTree = BUILT_PRODUCTS_DIR; };
+ 13B07FAF1A68108700A75B9A /* AppDelegate.h */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.c.h; name = AppDelegate.h; path = NitroSQLiteExample/AppDelegate.h; sourceTree = ""; };
+ 13B07FB01A68108700A75B9A /* AppDelegate.mm */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.cpp.objcpp; name = AppDelegate.mm; path = NitroSQLiteExample/AppDelegate.mm; sourceTree = ""; };
+ 13B07FB51A68108700A75B9A /* Images.xcassets */ = {isa = PBXFileReference; lastKnownFileType = folder.assetcatalog; name = Images.xcassets; path = NitroSQLiteExample/Images.xcassets; sourceTree = ""; };
+ 13B07FB61A68108700A75B9A /* Info.plist */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = text.plist.xml; name = Info.plist; path = NitroSQLiteExample/Info.plist; sourceTree = ""; };
+ 13B07FB71A68108700A75B9A /* main.m */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = sourcecode.c.objc; name = main.m; path = NitroSQLiteExample/main.m; sourceTree = ""; };
+ 81AB9BB72411601600AC10FF /* LaunchScreen.storyboard */ = {isa = PBXFileReference; fileEncoding = 4; lastKnownFileType = file.storyboard; name = LaunchScreen.storyboard; path = NitroSQLiteExample/LaunchScreen.storyboard; sourceTree = ""; };
+ B62F4C3A8445C44CF7F54399 /* PrivacyInfo.xcprivacy */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xml; name = PrivacyInfo.xcprivacy; path = NitroSQLiteExample/PrivacyInfo.xcprivacy; sourceTree = ""; };
+ BCC1E776B313FBFED7863A3D /* libPods-NitroSQLiteExample.a */ = {isa = PBXFileReference; explicitFileType = archive.ar; includeInIndex = 0; path = "libPods-NitroSQLiteExample.a"; sourceTree = BUILT_PRODUCTS_DIR; };
+ CA20110A9117BB91D9E63081 /* Pods-NitroSQLiteExample.debug.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; name = "Pods-NitroSQLiteExample.debug.xcconfig"; path = "Target Support Files/Pods-NitroSQLiteExample/Pods-NitroSQLiteExample.debug.xcconfig"; sourceTree = ""; };
+ CE317F99C7B7933DEB58C2E6 /* Pods-NitroSQLiteExample-NitroSQLiteExampleTests.release.xcconfig */ = {isa = PBXFileReference; includeInIndex = 1; lastKnownFileType = text.xcconfig; name = "Pods-NitroSQLiteExample-NitroSQLiteExampleTests.release.xcconfig"; path = "Target Support Files/Pods-NitroSQLiteExample-NitroSQLiteExampleTests/Pods-NitroSQLiteExample-NitroSQLiteExampleTests.release.xcconfig"; sourceTree = ""; };
ED297162215061F000B7C4FE /* JavaScriptCore.framework */ = {isa = PBXFileReference; lastKnownFileType = wrapper.framework; name = JavaScriptCore.framework; path = System/Library/Frameworks/JavaScriptCore.framework; sourceTree = SDKROOT; };
- F61ADDCA341B787B4A3AFAFC /* libPods-QuickSQLiteExample-QuickSQLiteExampleTests.a */ = {isa = PBXFileReference; explicitFileType = archive.ar; includeInIndex = 0; path = "libPods-QuickSQLiteExample-QuickSQLiteExampleTests.a"; sourceTree = BUILT_PRODUCTS_DIR; };
+ F61ADDCA341B787B4A3AFAFC /* libPods-NitroSQLiteExample-NitroSQLiteExampleTests.a */ = {isa = PBXFileReference; explicitFileType = archive.ar; includeInIndex = 0; path = "libPods-NitroSQLiteExample-NitroSQLiteExampleTests.a"; sourceTree = BUILT_PRODUCTS_DIR; };
/* End PBXFileReference section */
/* Begin PBXFrameworksBuildPhase section */
@@ -53,7 +53,7 @@
isa = PBXFrameworksBuildPhase;
buildActionMask = 2147483647;
files = (
- D7537A007033719516C57748 /* libPods-QuickSQLiteExample-QuickSQLiteExampleTests.a in Frameworks */,
+ D7537A007033719516C57748 /* libPods-NitroSQLiteExample-NitroSQLiteExampleTests.a in Frameworks */,
);
runOnlyForDeploymentPostprocessing = 0;
};
@@ -61,20 +61,20 @@
isa = PBXFrameworksBuildPhase;
buildActionMask = 2147483647;
files = (
- F6FA2C48029804D08B79E96F /* libPods-QuickSQLiteExample.a in Frameworks */,
+ F6FA2C48029804D08B79E96F /* libPods-NitroSQLiteExample.a in Frameworks */,
);
runOnlyForDeploymentPostprocessing = 0;
};
/* End PBXFrameworksBuildPhase section */
/* Begin PBXGroup section */
- 00E356EF1AD99517003FC87E /* QuickSQLiteExampleTests */ = {
+ 00E356EF1AD99517003FC87E /* NitroSQLiteExampleTests */ = {
isa = PBXGroup;
children = (
- 00E356F21AD99517003FC87E /* QuickSQLiteExampleTests.m */,
+ 00E356F21AD99517003FC87E /* NitroSQLiteExampleTests.m */,
00E356F01AD99517003FC87E /* Supporting Files */,
);
- path = QuickSQLiteExampleTests;
+ path = NitroSQLiteExampleTests;
sourceTree = "";
};
00E356F01AD99517003FC87E /* Supporting Files */ = {
@@ -85,7 +85,7 @@
name = "Supporting Files";
sourceTree = "";
};
- 13B07FAE1A68108700A75B9A /* QuickSQLiteExample */ = {
+ 13B07FAE1A68108700A75B9A /* NitroSQLiteExample */ = {
isa = PBXGroup;
children = (
13B07FAF1A68108700A75B9A /* AppDelegate.h */,
@@ -96,15 +96,15 @@
13B07FB71A68108700A75B9A /* main.m */,
B62F4C3A8445C44CF7F54399 /* PrivacyInfo.xcprivacy */,
);
- name = QuickSQLiteExample;
+ name = NitroSQLiteExample;
sourceTree = "";
};
2D16E6871FA4F8E400B85C8A /* Frameworks */ = {
isa = PBXGroup;
children = (
ED297162215061F000B7C4FE /* JavaScriptCore.framework */,
- BCC1E776B313FBFED7863A3D /* libPods-QuickSQLiteExample.a */,
- F61ADDCA341B787B4A3AFAFC /* libPods-QuickSQLiteExample-QuickSQLiteExampleTests.a */,
+ BCC1E776B313FBFED7863A3D /* libPods-NitroSQLiteExample.a */,
+ F61ADDCA341B787B4A3AFAFC /* libPods-NitroSQLiteExample-NitroSQLiteExampleTests.a */,
);
name = Frameworks;
sourceTree = "";
@@ -119,9 +119,9 @@
83CBB9F61A601CBA00E9B192 = {
isa = PBXGroup;
children = (
- 13B07FAE1A68108700A75B9A /* QuickSQLiteExample */,
+ 13B07FAE1A68108700A75B9A /* NitroSQLiteExample */,
832341AE1AAA6A7D00B99B32 /* Libraries */,
- 00E356EF1AD99517003FC87E /* QuickSQLiteExampleTests */,
+ 00E356EF1AD99517003FC87E /* NitroSQLiteExampleTests */,
83CBBA001A601CBA00E9B192 /* Products */,
2D16E6871FA4F8E400B85C8A /* Frameworks */,
BBD78D7AC51CEA395F1C20DB /* Pods */,
@@ -134,8 +134,8 @@
83CBBA001A601CBA00E9B192 /* Products */ = {
isa = PBXGroup;
children = (
- 13B07F961A680F5B00A75B9A /* QuickSQLiteExample.app */,
- 00E356EE1AD99517003FC87E /* QuickSQLiteExampleTests.xctest */,
+ 13B07F961A680F5B00A75B9A /* NitroSQLiteExample.app */,
+ 00E356EE1AD99517003FC87E /* NitroSQLiteExampleTests.xctest */,
);
name = Products;
sourceTree = "";
@@ -143,10 +143,10 @@
BBD78D7AC51CEA395F1C20DB /* Pods */ = {
isa = PBXGroup;
children = (
- CA20110A9117BB91D9E63081 /* Pods-QuickSQLiteExample.debug.xcconfig */,
- 03D39CB013C879B46EC1C979 /* Pods-QuickSQLiteExample.release.xcconfig */,
- 1251E54A0A604DFB2905E20E /* Pods-QuickSQLiteExample-QuickSQLiteExampleTests.debug.xcconfig */,
- CE317F99C7B7933DEB58C2E6 /* Pods-QuickSQLiteExample-QuickSQLiteExampleTests.release.xcconfig */,
+ CA20110A9117BB91D9E63081 /* Pods-NitroSQLiteExample.debug.xcconfig */,
+ 03D39CB013C879B46EC1C979 /* Pods-NitroSQLiteExample.release.xcconfig */,
+ 1251E54A0A604DFB2905E20E /* Pods-NitroSQLiteExample-NitroSQLiteExampleTests.debug.xcconfig */,
+ CE317F99C7B7933DEB58C2E6 /* Pods-NitroSQLiteExample-NitroSQLiteExampleTests.release.xcconfig */,
);
path = Pods;
sourceTree = "";
@@ -154,9 +154,9 @@
/* End PBXGroup section */
/* Begin PBXNativeTarget section */
- 00E356ED1AD99517003FC87E /* QuickSQLiteExampleTests */ = {
+ 00E356ED1AD99517003FC87E /* NitroSQLiteExampleTests */ = {
isa = PBXNativeTarget;
- buildConfigurationList = 00E357021AD99517003FC87E /* Build configuration list for PBXNativeTarget "QuickSQLiteExampleTests" */;
+ buildConfigurationList = 00E357021AD99517003FC87E /* Build configuration list for PBXNativeTarget "NitroSQLiteExampleTests" */;
buildPhases = (
2CDC9C54CCFE5D9F92A50675 /* [CP] Check Pods Manifest.lock */,
00E356EA1AD99517003FC87E /* Sources */,
@@ -170,14 +170,14 @@
dependencies = (
00E356F51AD99517003FC87E /* PBXTargetDependency */,
);
- name = QuickSQLiteExampleTests;
- productName = QuickSQLiteExampleTests;
- productReference = 00E356EE1AD99517003FC87E /* QuickSQLiteExampleTests.xctest */;
+ name = NitroSQLiteExampleTests;
+ productName = NitroSQLiteExampleTests;
+ productReference = 00E356EE1AD99517003FC87E /* NitroSQLiteExampleTests.xctest */;
productType = "com.apple.product-type.bundle.unit-test";
};
- 13B07F861A680F5B00A75B9A /* QuickSQLiteExample */ = {
+ 13B07F861A680F5B00A75B9A /* NitroSQLiteExample */ = {
isa = PBXNativeTarget;
- buildConfigurationList = 13B07F931A680F5B00A75B9A /* Build configuration list for PBXNativeTarget "QuickSQLiteExample" */;
+ buildConfigurationList = 13B07F931A680F5B00A75B9A /* Build configuration list for PBXNativeTarget "NitroSQLiteExample" */;
buildPhases = (
8851BB48F109827B5AB11D8D /* [CP] Check Pods Manifest.lock */,
FD10A7F022414F080027D42C /* Start Packager */,
@@ -192,9 +192,9 @@
);
dependencies = (
);
- name = QuickSQLiteExample;
- productName = QuickSQLiteExample;
- productReference = 13B07F961A680F5B00A75B9A /* QuickSQLiteExample.app */;
+ name = NitroSQLiteExample;
+ productName = NitroSQLiteExample;
+ productReference = 13B07F961A680F5B00A75B9A /* NitroSQLiteExample.app */;
productType = "com.apple.product-type.application";
};
/* End PBXNativeTarget section */
@@ -214,7 +214,7 @@
};
};
};
- buildConfigurationList = 83CBB9FA1A601CBA00E9B192 /* Build configuration list for PBXProject "QuickSQLiteExample" */;
+ buildConfigurationList = 83CBB9FA1A601CBA00E9B192 /* Build configuration list for PBXProject "NitroSQLiteExample" */;
compatibilityVersion = "Xcode 12.0";
developmentRegion = en;
hasScannedForEncodings = 0;
@@ -227,8 +227,8 @@
projectDirPath = "";
projectRoot = "";
targets = (
- 13B07F861A680F5B00A75B9A /* QuickSQLiteExample */,
- 00E356ED1AD99517003FC87E /* QuickSQLiteExampleTests */,
+ 13B07F861A680F5B00A75B9A /* NitroSQLiteExample */,
+ 00E356ED1AD99517003FC87E /* NitroSQLiteExampleTests */,
);
};
/* End PBXProject section */
@@ -285,7 +285,7 @@
outputFileListPaths = (
);
outputPaths = (
- "$(DERIVED_FILE_DIR)/Pods-QuickSQLiteExample-QuickSQLiteExampleTests-checkManifestLockResult.txt",
+ "$(DERIVED_FILE_DIR)/Pods-NitroSQLiteExample-NitroSQLiteExampleTests-checkManifestLockResult.txt",
);
runOnlyForDeploymentPostprocessing = 0;
shellPath = /bin/sh;
@@ -298,15 +298,15 @@
files = (
);
inputFileListPaths = (
- "${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample/Pods-QuickSQLiteExample-resources-${CONFIGURATION}-input-files.xcfilelist",
+ "${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample/Pods-NitroSQLiteExample-resources-${CONFIGURATION}-input-files.xcfilelist",
);
name = "[CP] Copy Pods Resources";
outputFileListPaths = (
- "${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample/Pods-QuickSQLiteExample-resources-${CONFIGURATION}-output-files.xcfilelist",
+ "${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample/Pods-NitroSQLiteExample-resources-${CONFIGURATION}-output-files.xcfilelist",
);
runOnlyForDeploymentPostprocessing = 0;
shellPath = /bin/sh;
- shellScript = "\"${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample/Pods-QuickSQLiteExample-resources.sh\"\n";
+ shellScript = "\"${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample/Pods-NitroSQLiteExample-resources.sh\"\n";
showEnvVarsInLog = 0;
};
6930F3E39E7F1174A4E95A27 /* [CP] Embed Pods Frameworks */ = {
@@ -315,15 +315,15 @@
files = (
);
inputFileListPaths = (
- "${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample-QuickSQLiteExampleTests/Pods-QuickSQLiteExample-QuickSQLiteExampleTests-frameworks-${CONFIGURATION}-input-files.xcfilelist",
+ "${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample-NitroSQLiteExampleTests/Pods-NitroSQLiteExample-NitroSQLiteExampleTests-frameworks-${CONFIGURATION}-input-files.xcfilelist",
);
name = "[CP] Embed Pods Frameworks";
outputFileListPaths = (
- "${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample-QuickSQLiteExampleTests/Pods-QuickSQLiteExample-QuickSQLiteExampleTests-frameworks-${CONFIGURATION}-output-files.xcfilelist",
+ "${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample-NitroSQLiteExampleTests/Pods-NitroSQLiteExample-NitroSQLiteExampleTests-frameworks-${CONFIGURATION}-output-files.xcfilelist",
);
runOnlyForDeploymentPostprocessing = 0;
shellPath = /bin/sh;
- shellScript = "\"${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample-QuickSQLiteExampleTests/Pods-QuickSQLiteExample-QuickSQLiteExampleTests-frameworks.sh\"\n";
+ shellScript = "\"${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample-NitroSQLiteExampleTests/Pods-NitroSQLiteExample-NitroSQLiteExampleTests-frameworks.sh\"\n";
showEnvVarsInLog = 0;
};
8851BB48F109827B5AB11D8D /* [CP] Check Pods Manifest.lock */ = {
@@ -341,7 +341,7 @@
outputFileListPaths = (
);
outputPaths = (
- "$(DERIVED_FILE_DIR)/Pods-QuickSQLiteExample-checkManifestLockResult.txt",
+ "$(DERIVED_FILE_DIR)/Pods-NitroSQLiteExample-checkManifestLockResult.txt",
);
runOnlyForDeploymentPostprocessing = 0;
shellPath = /bin/sh;
@@ -354,15 +354,15 @@
files = (
);
inputFileListPaths = (
- "${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample/Pods-QuickSQLiteExample-frameworks-${CONFIGURATION}-input-files.xcfilelist",
+ "${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample/Pods-NitroSQLiteExample-frameworks-${CONFIGURATION}-input-files.xcfilelist",
);
name = "[CP] Embed Pods Frameworks";
outputFileListPaths = (
- "${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample/Pods-QuickSQLiteExample-frameworks-${CONFIGURATION}-output-files.xcfilelist",
+ "${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample/Pods-NitroSQLiteExample-frameworks-${CONFIGURATION}-output-files.xcfilelist",
);
runOnlyForDeploymentPostprocessing = 0;
shellPath = /bin/sh;
- shellScript = "\"${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample/Pods-QuickSQLiteExample-frameworks.sh\"\n";
+ shellScript = "\"${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample/Pods-NitroSQLiteExample-frameworks.sh\"\n";
showEnvVarsInLog = 0;
};
9DED98E0C31C147A92DCCCAA /* [CP] Copy Pods Resources */ = {
@@ -371,15 +371,15 @@
files = (
);
inputFileListPaths = (
- "${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample-QuickSQLiteExampleTests/Pods-QuickSQLiteExample-QuickSQLiteExampleTests-resources-${CONFIGURATION}-input-files.xcfilelist",
+ "${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample-NitroSQLiteExampleTests/Pods-NitroSQLiteExample-NitroSQLiteExampleTests-resources-${CONFIGURATION}-input-files.xcfilelist",
);
name = "[CP] Copy Pods Resources";
outputFileListPaths = (
- "${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample-QuickSQLiteExampleTests/Pods-QuickSQLiteExample-QuickSQLiteExampleTests-resources-${CONFIGURATION}-output-files.xcfilelist",
+ "${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample-NitroSQLiteExampleTests/Pods-NitroSQLiteExample-NitroSQLiteExampleTests-resources-${CONFIGURATION}-output-files.xcfilelist",
);
runOnlyForDeploymentPostprocessing = 0;
shellPath = /bin/sh;
- shellScript = "\"${PODS_ROOT}/Target Support Files/Pods-QuickSQLiteExample-QuickSQLiteExampleTests/Pods-QuickSQLiteExample-QuickSQLiteExampleTests-resources.sh\"\n";
+ shellScript = "\"${PODS_ROOT}/Target Support Files/Pods-NitroSQLiteExample-NitroSQLiteExampleTests/Pods-NitroSQLiteExample-NitroSQLiteExampleTests-resources.sh\"\n";
showEnvVarsInLog = 0;
};
FD10A7F022414F080027D42C /* Start Packager */ = {
@@ -408,7 +408,7 @@
isa = PBXSourcesBuildPhase;
buildActionMask = 2147483647;
files = (
- 00E356F31AD99517003FC87E /* QuickSQLiteExampleTests.m in Sources */,
+ 00E356F31AD99517003FC87E /* NitroSQLiteExampleTests.m in Sources */,
);
runOnlyForDeploymentPostprocessing = 0;
};
@@ -426,7 +426,7 @@
/* Begin PBXTargetDependency section */
00E356F51AD99517003FC87E /* PBXTargetDependency */ = {
isa = PBXTargetDependency;
- target = 13B07F861A680F5B00A75B9A /* QuickSQLiteExample */;
+ target = 13B07F861A680F5B00A75B9A /* NitroSQLiteExample */;
targetProxy = 00E356F41AD99517003FC87E /* PBXContainerItemProxy */;
};
/* End PBXTargetDependency section */
@@ -434,14 +434,14 @@
/* Begin XCBuildConfiguration section */
00E356F61AD99517003FC87E /* Debug */ = {
isa = XCBuildConfiguration;
- baseConfigurationReference = 1251E54A0A604DFB2905E20E /* Pods-QuickSQLiteExample-QuickSQLiteExampleTests.debug.xcconfig */;
+ baseConfigurationReference = 1251E54A0A604DFB2905E20E /* Pods-NitroSQLiteExample-NitroSQLiteExampleTests.debug.xcconfig */;
buildSettings = {
BUNDLE_LOADER = "$(TEST_HOST)";
GCC_PREPROCESSOR_DEFINITIONS = (
"DEBUG=1",
"$(inherited)",
);
- INFOPLIST_FILE = QuickSQLiteExampleTests/Info.plist;
+ INFOPLIST_FILE = NitroSQLiteExampleTests/Info.plist;
IPHONEOS_DEPLOYMENT_TARGET = 15.1;
LD_RUNPATH_SEARCH_PATHS = (
"$(inherited)",
@@ -455,17 +455,17 @@
);
PRODUCT_BUNDLE_IDENTIFIER = "org.reactjs.native.example.$(PRODUCT_NAME:rfc1034identifier)";
PRODUCT_NAME = "$(TARGET_NAME)";
- TEST_HOST = "$(BUILT_PRODUCTS_DIR)/QuickSQLiteExample.app/QuickSQLiteExample";
+ TEST_HOST = "$(BUILT_PRODUCTS_DIR)/NitroSQLiteExample.app/NitroSQLiteExample";
};
name = Debug;
};
00E356F71AD99517003FC87E /* Release */ = {
isa = XCBuildConfiguration;
- baseConfigurationReference = CE317F99C7B7933DEB58C2E6 /* Pods-QuickSQLiteExample-QuickSQLiteExampleTests.release.xcconfig */;
+ baseConfigurationReference = CE317F99C7B7933DEB58C2E6 /* Pods-NitroSQLiteExample-NitroSQLiteExampleTests.release.xcconfig */;
buildSettings = {
BUNDLE_LOADER = "$(TEST_HOST)";
COPY_PHASE_STRIP = NO;
- INFOPLIST_FILE = QuickSQLiteExampleTests/Info.plist;
+ INFOPLIST_FILE = NitroSQLiteExampleTests/Info.plist;
IPHONEOS_DEPLOYMENT_TARGET = 15.1;
LD_RUNPATH_SEARCH_PATHS = (
"$(inherited)",
@@ -479,20 +479,20 @@
);
PRODUCT_BUNDLE_IDENTIFIER = "org.reactjs.native.example.$(PRODUCT_NAME:rfc1034identifier)";
PRODUCT_NAME = "$(TARGET_NAME)";
- TEST_HOST = "$(BUILT_PRODUCTS_DIR)/QuickSQLiteExample.app/QuickSQLiteExample";
+ TEST_HOST = "$(BUILT_PRODUCTS_DIR)/NitroSQLiteExample.app/NitroSQLiteExample";
};
name = Release;
};
13B07F941A680F5B00A75B9A /* Debug */ = {
isa = XCBuildConfiguration;
- baseConfigurationReference = CA20110A9117BB91D9E63081 /* Pods-QuickSQLiteExample.debug.xcconfig */;
+ baseConfigurationReference = CA20110A9117BB91D9E63081 /* Pods-NitroSQLiteExample.debug.xcconfig */;
buildSettings = {
ASSETCATALOG_COMPILER_APPICON_NAME = AppIcon;
CLANG_ENABLE_MODULES = YES;
CURRENT_PROJECT_VERSION = 1;
DEVELOPMENT_TEAM = WYL5V2KKR3;
ENABLE_BITCODE = NO;
- INFOPLIST_FILE = QuickSQLiteExample/Info.plist;
+ INFOPLIST_FILE = NitroSQLiteExample/Info.plist;
IPHONEOS_DEPLOYMENT_TARGET = 15.1;
LD_RUNPATH_SEARCH_PATHS = (
"$(inherited)",
@@ -504,7 +504,7 @@
"-lc++",
);
PRODUCT_BUNDLE_IDENTIFIER = "org.reactjs.native.example.$(PRODUCT_NAME:rfc1034identifier)";
- PRODUCT_NAME = QuickSQLiteExample;
+ PRODUCT_NAME = NitroSQLiteExample;
SWIFT_OPTIMIZATION_LEVEL = "-Onone";
SWIFT_VERSION = 5.0;
VERSIONING_SYSTEM = "apple-generic";
@@ -513,13 +513,13 @@
};
13B07F951A680F5B00A75B9A /* Release */ = {
isa = XCBuildConfiguration;
- baseConfigurationReference = 03D39CB013C879B46EC1C979 /* Pods-QuickSQLiteExample.release.xcconfig */;
+ baseConfigurationReference = 03D39CB013C879B46EC1C979 /* Pods-NitroSQLiteExample.release.xcconfig */;
buildSettings = {
ASSETCATALOG_COMPILER_APPICON_NAME = AppIcon;
CLANG_ENABLE_MODULES = YES;
CURRENT_PROJECT_VERSION = 1;
DEVELOPMENT_TEAM = WYL5V2KKR3;
- INFOPLIST_FILE = QuickSQLiteExample/Info.plist;
+ INFOPLIST_FILE = NitroSQLiteExample/Info.plist;
IPHONEOS_DEPLOYMENT_TARGET = 15.1;
LD_RUNPATH_SEARCH_PATHS = (
"$(inherited)",
@@ -531,7 +531,7 @@
"-lc++",
);
PRODUCT_BUNDLE_IDENTIFIER = "org.reactjs.native.example.$(PRODUCT_NAME:rfc1034identifier)";
- PRODUCT_NAME = QuickSQLiteExample;
+ PRODUCT_NAME = NitroSQLiteExample;
SWIFT_VERSION = 5.0;
VERSIONING_SYSTEM = "apple-generic";
};
@@ -700,7 +700,7 @@
/* End XCBuildConfiguration section */
/* Begin XCConfigurationList section */
- 00E357021AD99517003FC87E /* Build configuration list for PBXNativeTarget "QuickSQLiteExampleTests" */ = {
+ 00E357021AD99517003FC87E /* Build configuration list for PBXNativeTarget "NitroSQLiteExampleTests" */ = {
isa = XCConfigurationList;
buildConfigurations = (
00E356F61AD99517003FC87E /* Debug */,
@@ -709,7 +709,7 @@
defaultConfigurationIsVisible = 0;
defaultConfigurationName = Release;
};
- 13B07F931A680F5B00A75B9A /* Build configuration list for PBXNativeTarget "QuickSQLiteExample" */ = {
+ 13B07F931A680F5B00A75B9A /* Build configuration list for PBXNativeTarget "NitroSQLiteExample" */ = {
isa = XCConfigurationList;
buildConfigurations = (
13B07F941A680F5B00A75B9A /* Debug */,
@@ -718,7 +718,7 @@
defaultConfigurationIsVisible = 0;
defaultConfigurationName = Release;
};
- 83CBB9FA1A601CBA00E9B192 /* Build configuration list for PBXProject "QuickSQLiteExample" */ = {
+ 83CBB9FA1A601CBA00E9B192 /* Build configuration list for PBXProject "NitroSQLiteExample" */ = {
isa = XCConfigurationList;
buildConfigurations = (
83CBBA201A601CBA00E9B192 /* Debug */,
diff --git a/example/ios/NitroSQLiteExample.xcodeproj/xcshareddata/xcschemes/NitroSQLiteExample.xcscheme b/example/ios/NitroSQLiteExample.xcodeproj/xcshareddata/xcschemes/NitroSQLiteExample.xcscheme
new file mode 100644
index 0000000..e220b63
--- /dev/null
+++ b/example/ios/NitroSQLiteExample.xcodeproj/xcshareddata/xcschemes/NitroSQLiteExample.xcscheme
@@ -0,0 +1,88 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/example/ios/NitroSQLiteExample.xcworkspace/contents.xcworkspacedata b/example/ios/NitroSQLiteExample.xcworkspace/contents.xcworkspacedata
new file mode 100644
index 0000000..cb9898e
--- /dev/null
+++ b/example/ios/NitroSQLiteExample.xcworkspace/contents.xcworkspacedata
@@ -0,0 +1,10 @@
+
+
+
+
+
+
+
diff --git a/example/ios/QuickSQLiteExample.xcworkspace/xcshareddata/IDEWorkspaceChecks.plist b/example/ios/NitroSQLiteExample.xcworkspace/xcshareddata/IDEWorkspaceChecks.plist
similarity index 100%
rename from example/ios/QuickSQLiteExample.xcworkspace/xcshareddata/IDEWorkspaceChecks.plist
rename to example/ios/NitroSQLiteExample.xcworkspace/xcshareddata/IDEWorkspaceChecks.plist
diff --git a/example/ios/QuickSQLiteExample/AppDelegate.h b/example/ios/NitroSQLiteExample/AppDelegate.h
similarity index 100%
rename from example/ios/QuickSQLiteExample/AppDelegate.h
rename to example/ios/NitroSQLiteExample/AppDelegate.h
diff --git a/example/ios/QuickSQLiteExample/AppDelegate.mm b/example/ios/NitroSQLiteExample/AppDelegate.mm
similarity index 93%
rename from example/ios/QuickSQLiteExample/AppDelegate.mm
rename to example/ios/NitroSQLiteExample/AppDelegate.mm
index a617319..dde628a 100644
--- a/example/ios/QuickSQLiteExample/AppDelegate.mm
+++ b/example/ios/NitroSQLiteExample/AppDelegate.mm
@@ -6,7 +6,7 @@ @implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
- self.moduleName = @"QuickSQLiteExample";
+ self.moduleName = @"NitroSQLiteExample";
return [super application:application didFinishLaunchingWithOptions:launchOptions];
}
diff --git a/example/ios/QuickSQLiteExample/Images.xcassets/AppIcon.appiconset/Contents.json b/example/ios/NitroSQLiteExample/Images.xcassets/AppIcon.appiconset/Contents.json
similarity index 100%
rename from example/ios/QuickSQLiteExample/Images.xcassets/AppIcon.appiconset/Contents.json
rename to example/ios/NitroSQLiteExample/Images.xcassets/AppIcon.appiconset/Contents.json
diff --git a/example/ios/QuickSQLiteExample/Images.xcassets/Contents.json b/example/ios/NitroSQLiteExample/Images.xcassets/Contents.json
similarity index 100%
rename from example/ios/QuickSQLiteExample/Images.xcassets/Contents.json
rename to example/ios/NitroSQLiteExample/Images.xcassets/Contents.json
diff --git a/example/ios/NitroSQLiteExample/Info.plist b/example/ios/NitroSQLiteExample/Info.plist
new file mode 100644
index 0000000..eaf978d
--- /dev/null
+++ b/example/ios/NitroSQLiteExample/Info.plist
@@ -0,0 +1,52 @@
+
+
+
+
+ CFBundleDevelopmentRegion
+ en
+ CFBundleDisplayName
+ NitroSQLiteExample
+ CFBundleExecutable
+ $(EXECUTABLE_NAME)
+ CFBundleIdentifier
+ $(PRODUCT_BUNDLE_IDENTIFIER)
+ CFBundleInfoDictionaryVersion
+ 6.0
+ CFBundleName
+ $(PRODUCT_NAME)
+ CFBundlePackageType
+ APPL
+ CFBundleShortVersionString
+ 1.0
+ CFBundleSignature
+ ????
+ CFBundleVersion
+ 1
+ LSRequiresIPhoneOS
+
+ NSAppTransportSecurity
+
+
+ NSAllowsArbitraryLoads
+
+ NSAllowsLocalNetworking
+
+
+ NSLocationWhenInUseUsageDescription
+
+ UILaunchStoryboardName
+ LaunchScreen
+ UIRequiredDeviceCapabilities
+
+ arm64
+
+ UISupportedInterfaceOrientations
+
+ UIInterfaceOrientationPortrait
+ UIInterfaceOrientationLandscapeLeft
+ UIInterfaceOrientationLandscapeRight
+
+ UIViewControllerBasedStatusBarAppearance
+
+
+
diff --git a/example/ios/NitroSQLiteExample/LaunchScreen.storyboard b/example/ios/NitroSQLiteExample/LaunchScreen.storyboard
new file mode 100644
index 0000000..4bd4247
--- /dev/null
+++ b/example/ios/NitroSQLiteExample/LaunchScreen.storyboard
@@ -0,0 +1,74 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/example/ios/QuickSQLiteExample/PrivacyInfo.xcprivacy b/example/ios/NitroSQLiteExample/PrivacyInfo.xcprivacy
similarity index 100%
rename from example/ios/QuickSQLiteExample/PrivacyInfo.xcprivacy
rename to example/ios/NitroSQLiteExample/PrivacyInfo.xcprivacy
diff --git a/example/ios/QuickSQLiteExample/main.m b/example/ios/NitroSQLiteExample/main.m
similarity index 100%
rename from example/ios/QuickSQLiteExample/main.m
rename to example/ios/NitroSQLiteExample/main.m
diff --git a/example/ios/QuickSQLiteExampleTests/Info.plist b/example/ios/NitroSQLiteExampleTests/Info.plist
similarity index 100%
rename from example/ios/QuickSQLiteExampleTests/Info.plist
rename to example/ios/NitroSQLiteExampleTests/Info.plist
diff --git a/example/ios/QuickSQLiteExampleTests/QuickSQLiteExampleTests.m b/example/ios/NitroSQLiteExampleTests/NitroSQLiteExampleTests.m
similarity index 95%
rename from example/ios/QuickSQLiteExampleTests/QuickSQLiteExampleTests.m
rename to example/ios/NitroSQLiteExampleTests/NitroSQLiteExampleTests.m
index 5a3993e..ab7306e 100644
--- a/example/ios/QuickSQLiteExampleTests/QuickSQLiteExampleTests.m
+++ b/example/ios/NitroSQLiteExampleTests/NitroSQLiteExampleTests.m
@@ -7,11 +7,11 @@
#define TIMEOUT_SECONDS 600
#define TEXT_TO_LOOK_FOR @"Welcome to React"
-@interface QuickSQLiteExampleTests : XCTestCase
+@interface NitroSQLiteExampleTests : XCTestCase
@end
-@implementation QuickSQLiteExampleTests
+@implementation NitroSQLiteExampleTests
- (BOOL)findSubviewInView:(UIView *)view matching:(BOOL (^)(UIView *view))test
{
diff --git a/example/ios/Podfile b/example/ios/Podfile
index 0862af0..d2a63e9 100644
--- a/example/ios/Podfile
+++ b/example/ios/Podfile
@@ -16,7 +16,7 @@ linkage = ENV['USE_FRAMEWORKS']
use_frameworks! :linkage => linkage.to_sym
end
-target 'QuickSQLiteExample' do
+target 'NitroSQLiteExample' do
config = use_native_modules!
use_react_native!(
@@ -25,9 +25,9 @@ target 'QuickSQLiteExample' do
:app_path => "#{Pod::Config.instance.installation_root}/.."
)
- # pod 'RNQuickSQLite', :path => '../../package'
+ # pod 'RNNitroSQLite', :path => '../../package'
- target 'QuickSQLiteExampleTests' do
+ target 'NitroSQLiteExampleTests' do
inherit! :complete
# Pods for testing
end
diff --git a/example/ios/Podfile.lock b/example/ios/Podfile.lock
index f5756e1..0296db1 100644
--- a/example/ios/Podfile.lock
+++ b/example/ios/Podfile.lock
@@ -1596,7 +1596,7 @@ PODS:
- React-logger (= 0.76.1)
- React-perflogger (= 0.76.1)
- React-utils (= 0.76.1)
- - RNQuickSQLite (8.2.5):
+ - RNNitroSQLite (8.2.2-nitro.1):
- DoubleConversion
- glog
- hermes-engine
@@ -1733,7 +1733,7 @@ DEPENDENCIES:
- React-utils (from `../../node_modules/react-native/ReactCommon/react/utils`)
- ReactCodegen (from `build/generated/ios`)
- ReactCommon/turbomodule/core (from `../../node_modules/react-native/ReactCommon`)
- - RNQuickSQLite (from `../../package`)
+ - RNNitroSQLite (from `../../package`)
- RNScreens (from `../../node_modules/react-native-screens`)
- Yoga (from `../../node_modules/react-native/ReactCommon/yoga`)
@@ -1871,7 +1871,7 @@ EXTERNAL SOURCES:
:path: build/generated/ios
ReactCommon:
:path: "../../node_modules/react-native/ReactCommon"
- RNQuickSQLite:
+ RNNitroSQLite:
:path: "../../package"
RNScreens:
:path: "../../node_modules/react-native-screens"
@@ -1943,11 +1943,11 @@ SPEC CHECKSUMS:
React-utils: 5362bd16a9563f9916e7a56c011ddc533507650f
ReactCodegen: 4e26d365313307cc7c95e693529e539acfb5c64c
ReactCommon: 422e364463f33e336fc4db196aeb50fd801d90d6
- RNQuickSQLite: 8ef02913f22a4ca65f44cf826acd0185aa5d6771
+ RNNitroSQLite: 414f19ca9a1a29aa1a1ff7ecfa6bc03c7884e9b1
RNScreens: e389d6a6a66a4f0d3662924ecae803073ccce8ec
SocketRocket: d4aabe649be1e368d1318fdf28a022d714d65748
Yoga: db69236006b8b1c6d55ab453390c882306cbf219
-PODFILE CHECKSUM: c6724dc625a6aa9f3f0577b2c2d3f9b39ece8968
+PODFILE CHECKSUM: 27ad3045377187b09e7ab4a604c851aa9838f52d
COCOAPODS: 1.15.2
diff --git a/example/ios/QuickSQLiteExample.xcodeproj/xcshareddata/xcschemes/QuickSQLiteExample.xcscheme b/example/ios/QuickSQLiteExample.xcodeproj/xcshareddata/xcschemes/QuickSQLiteExample.xcscheme
deleted file mode 100644
index 5f53de4..0000000
--- a/example/ios/QuickSQLiteExample.xcodeproj/xcshareddata/xcschemes/QuickSQLiteExample.xcscheme
+++ /dev/null
@@ -1,88 +0,0 @@
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
diff --git a/example/ios/QuickSQLiteExample.xcworkspace/contents.xcworkspacedata b/example/ios/QuickSQLiteExample.xcworkspace/contents.xcworkspacedata
deleted file mode 100644
index c1e31da..0000000
--- a/example/ios/QuickSQLiteExample.xcworkspace/contents.xcworkspacedata
+++ /dev/null
@@ -1,10 +0,0 @@
-
-
-
-
-
-
-
diff --git a/example/ios/QuickSQLiteExample/Info.plist b/example/ios/QuickSQLiteExample/Info.plist
deleted file mode 100644
index 1b9a707..0000000
--- a/example/ios/QuickSQLiteExample/Info.plist
+++ /dev/null
@@ -1,52 +0,0 @@
-
-
-
-
- CFBundleDevelopmentRegion
- en
- CFBundleDisplayName
- QuickSQLiteExample
- CFBundleExecutable
- $(EXECUTABLE_NAME)
- CFBundleIdentifier
- $(PRODUCT_BUNDLE_IDENTIFIER)
- CFBundleInfoDictionaryVersion
- 6.0
- CFBundleName
- $(PRODUCT_NAME)
- CFBundlePackageType
- APPL
- CFBundleShortVersionString
- 1.0
- CFBundleSignature
- ????
- CFBundleVersion
- 1
- LSRequiresIPhoneOS
-
- NSAppTransportSecurity
-
-
- NSAllowsArbitraryLoads
-
- NSAllowsLocalNetworking
-
-
- NSLocationWhenInUseUsageDescription
-
- UILaunchStoryboardName
- LaunchScreen
- UIRequiredDeviceCapabilities
-
- arm64
-
- UISupportedInterfaceOrientations
-
- UIInterfaceOrientationPortrait
- UIInterfaceOrientationLandscapeLeft
- UIInterfaceOrientationLandscapeRight
-
- UIViewControllerBasedStatusBarAppearance
-
-
-
diff --git a/example/ios/QuickSQLiteExample/LaunchScreen.storyboard b/example/ios/QuickSQLiteExample/LaunchScreen.storyboard
deleted file mode 100644
index 851d08c..0000000
--- a/example/ios/QuickSQLiteExample/LaunchScreen.storyboard
+++ /dev/null
@@ -1,47 +0,0 @@
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
diff --git a/example/package.json b/example/package.json
index c55c395..5f1d186 100644
--- a/example/package.json
+++ b/example/package.json
@@ -1,6 +1,6 @@
{
- "name": "react-native-quick-sqlite-example",
- "version": "8.2.3",
+ "name": "react-native-nitro-sqlite-example",
+ "version": "8.2.2-nitro.1",
"private": true,
"scripts": {
"start": "react-native start",
@@ -24,7 +24,7 @@
"react": "*",
"react-native": "*",
"react-native-nitro-modules": "*",
- "react-native-quick-sqlite": "8.2.5",
+ "react-native-nitro-sqlite": "8.2.2-nitro.1",
"react-native-safe-area-context": "^4.14.0",
"react-native-screens": "^3.35.0",
"reflect-metadata": "^0.1.13",
diff --git a/example/src/Database.ts b/example/src/Database.ts
index 2a2514a..e3e1d3a 100644
--- a/example/src/Database.ts
+++ b/example/src/Database.ts
@@ -1,6 +1,6 @@
import { DataSource } from 'typeorm'
-import type { QueryResult } from 'react-native-quick-sqlite'
-import { typeORMDriver } from 'react-native-quick-sqlite'
+import type { QueryResult } from 'react-native-nitro-sqlite'
+import { typeORMDriver } from 'react-native-nitro-sqlite'
import { Book } from './model/Book'
import { User } from './model/User'
let datasource: DataSource
diff --git a/example/src/tests/db.ts b/example/src/tests/db.ts
index e94b65a..c1b75a4 100644
--- a/example/src/tests/db.ts
+++ b/example/src/tests/db.ts
@@ -1,13 +1,13 @@
import Chance from 'chance'
import type {
- QuickSQLiteConnection,
+ NitroSQLiteConnection,
BatchQueryCommand,
-} from 'react-native-quick-sqlite'
-import { open } from 'react-native-quick-sqlite'
+} from 'react-native-nitro-sqlite'
+import { open } from 'react-native-nitro-sqlite'
const chance = new Chance()
-export let testDb: QuickSQLiteConnection | undefined
+export let testDb: NitroSQLiteConnection | undefined
export function resetTestDb() {
try {
if (testDb != null) {
@@ -26,7 +26,7 @@ export function resetTestDb() {
// Taken from "op-sqlite" example project.
// Used to demonstrate the performance of NitroSQLite.
const ROWS = 300000
-export let largeDb: QuickSQLiteConnection | undefined
+export let largeDb: NitroSQLiteConnection | undefined
export function resetLargeDb() {
try {
if (largeDb != null) {
diff --git a/example/src/tests/typeorm.spec.ts b/example/src/tests/typeorm.spec.ts
index 9f262eb..5e51eec 100644
--- a/example/src/tests/typeorm.spec.ts
+++ b/example/src/tests/typeorm.spec.ts
@@ -1,7 +1,7 @@
import type { Repository } from 'typeorm'
import { DataSource } from 'typeorm'
import { beforeAll, it, describe, beforeEachAsync } from './MochaRNAdapter'
-import { typeORMDriver } from 'react-native-quick-sqlite'
+import { typeORMDriver } from 'react-native-nitro-sqlite'
import { User } from '../model/User'
import { Book } from '../model/Book'
import chai from 'chai'
diff --git a/example/src/tests/unitTests.spec.ts b/example/src/tests/unitTests.spec.ts
index 79d3fc8..5b53040 100644
--- a/example/src/tests/unitTests.spec.ts
+++ b/example/src/tests/unitTests.spec.ts
@@ -1,12 +1,11 @@
import Chance from 'chance'
import type {
- QuickSQLiteConnection,
+ NitroSQLiteConnection,
BatchQueryCommand,
-} from 'react-native-quick-sqlite'
+} from 'react-native-nitro-sqlite'
import { beforeEach, describe, it } from './MochaRNAdapter'
import chai from 'chai'
import { testDb as testDbInternal, resetTestDb } from './db'
-import { User } from '../model/User'
function isError(e: unknown): e is Error {
return e instanceof Error
@@ -16,7 +15,7 @@ const expect = chai.expect
const chance = new Chance()
export function registerUnitTests() {
- let testDb: QuickSQLiteConnection
+ let testDb: NitroSQLiteConnection
beforeEach(() => {
try {
@@ -119,7 +118,7 @@ export function registerUnitTests() {
'cannot store TEXT value in REAL column User.age'
)
} else {
- expect.fail('Should have thrown a valid QuickSQLiteException')
+ expect.fail('Should have thrown a valid NitroSQLiteException')
}
}
})
@@ -343,7 +342,7 @@ export function registerUnitTests() {
expect.fail('Should not resolve')
} catch (e) {
if (isError(e)) expect(e.message).to.equal('Error from callback')
- else expect.fail('Should have thrown a valid QuickSQLiteException')
+ else expect.fail('Should have thrown a valid NitroSQLiteException')
}
})
@@ -359,7 +358,7 @@ export function registerUnitTests() {
} catch (e) {
if (isError(e))
expect(e.message).to.include('no such table: tableThatDoesNotExist')
- else expect.fail('Should have thrown a valid QuickSQLiteException')
+ else expect.fail('Should have thrown a valid NitroSQLiteException')
}
})
@@ -431,7 +430,7 @@ export function registerUnitTests() {
const res = testDb.execute('SELECT * FROM User')
expect(res.rows?._array).to.eql([])
} else {
- expect.fail('Should have thrown a valid QuickSQLiteException')
+ expect.fail('Should have thrown a valid NitroSQLiteException')
}
}
})
@@ -545,7 +544,7 @@ export function registerUnitTests() {
expect.fail('Should not resolve')
} catch (e) {
if (isError(e)) expect(e.message).to.equal('Error from callback')
- else expect.fail('Should have thrown a valid QuickSQLiteException')
+ else expect.fail('Should have thrown a valid NitroSQLiteException')
}
})
@@ -562,7 +561,7 @@ export function registerUnitTests() {
} catch (e) {
if (isError(e))
expect(e.message).to.include('no such table: tableThatDoesNotExist')
- else expect.fail('Should have thrown a valid QuickSQLiteException')
+ else expect.fail('Should have thrown a valid NitroSQLiteException')
}
})
diff --git a/package.json b/package.json
index 5d83c96..23cc643 100644
--- a/package.json
+++ b/package.json
@@ -1,6 +1,6 @@
{
- "name": "react-native-quick-sqlite-workspace",
- "version": "8.2.3",
+ "name": "react-native-nitro-sqlite-workspace",
+ "version": "8.2.2-nitro.1",
"packageManager": "bun@1.1.21",
"private": "true",
"workspaces": [
@@ -9,14 +9,14 @@
],
"repository": {
"type": "git",
- "url": "git+https://github.com/margelo/react-native-quick-sqlite.git"
+ "url": "git+https://github.com/margelo/react-native-nitro-sqlite.git"
},
- "author": "Marc Rousavy (https://github.com/mrousavy)",
+ "author": "Margelo (https://github.com/margelo)",
"license": "MIT",
"bugs": {
- "url": "https://github.com/margelo/react-native-quick-sqlite/issues"
+ "url": "https://github.com/margelo/react-native-nitro-sqlite/issues"
},
- "homepage": "https://github.com/margelo/react-native-quick-sqlite#readme",
+ "homepage": "https://github.com/margelo/react-native-nitro-sqlite#readme",
"scripts": {
"postinstall": "patch-package",
"typescript": "bun --filter=\"**\" typescript",
@@ -84,7 +84,7 @@
},
{
"file": "example/package.json",
- "path": "dependencies.react-native-quick-sqlite"
+ "path": "dependencies.react-native-nitro-sqlite"
}
]
},
diff --git a/package/RNQuickSQLite.podspec b/package/RNNitroSQLite.podspec
similarity index 93%
rename from package/RNQuickSQLite.podspec
rename to package/RNNitroSQLite.podspec
index fab5913..689e43f 100644
--- a/package/RNQuickSQLite.podspec
+++ b/package/RNNitroSQLite.podspec
@@ -8,7 +8,7 @@ folly_compiler_flags = '-DFOLLY_NO_CONFIG -DFOLLY_MOBILE=1 -DFOLLY_USE_LIBCPP=1
performance_mode = 1
Pod::Spec.new do |s|
- s.name = "RNQuickSQLite"
+ s.name = "RNNitroSQLite"
s.version = package["version"]
s.summary = package["description"]
s.homepage = package["homepage"]
@@ -16,7 +16,7 @@ Pod::Spec.new do |s|
s.authors = package["author"]
s.platforms = { :ios => min_ios_version_supported, :visionos => "1.0" }
- s.source = { :git => "https://github.com/margelo/react-native-quick-sqlite.git", :tag => "#{s.version}" }
+ s.source = { :git => "https://github.com/margelo/react-native-nitro-sqlite.git", :tag => "#{s.version}" }
# s.header_mappings_dir = "cpp"
s.source_files = "ios/**/*.{h,hpp,m,mm}", "cpp/**/*.{h,hpp,c,cpp}"
@@ -29,7 +29,7 @@ Pod::Spec.new do |s|
'CLANG_CXX_LIBRARY' => 'libc++'
}
- load 'nitrogen/generated/ios/RNQuickSQLite+autolinking.rb'
+ load 'nitrogen/generated/ios/RNNitroSQLite+autolinking.rb'
add_nitrogen_files(s)
# Use install_modules_dependencies helper to install the dependencies if React Native version >=0.71.0.
@@ -69,7 +69,7 @@ Pod::Spec.new do |s|
xcconfig[:OTHER_CFLAGS] = optimizedCflags + ' -DSQLITE_THREADSAFE=1 '
end
- if ENV['QUICK_SQLITE_USE_PHONE_VERSION'] == '1' then
+ if ENV['NITRO_SQLITE_USE_PHONE_VERSION'] == '1' then
s.exclude_files = "cpp/sqlite3.c", "cpp/sqlite3.h"
s.library = "sqlite3"
end
diff --git a/package/android/CMakeLists.txt b/package/android/CMakeLists.txt
index 8ec763a..adff9c5 100644
--- a/package/android/CMakeLists.txt
+++ b/package/android/CMakeLists.txt
@@ -1,5 +1,5 @@
cmake_minimum_required(VERSION 3.13)
-project(RNQuickSQLite)
+project(RNNitroSQLite)
set (CMAKE_VERBOSE_MAKEFILE ON)
set (CMAKE_CXX_STANDARD 20)
@@ -14,12 +14,12 @@ file(GLOB_RECURSE cpp_files RELATIVE ${CMAKE_SOURCE_DIR}
"cpp-adapter.cpp"
)
-# Create library "RNQuickSQLite" and add all C++ files to it
+# Create library "RNNitroSQLite" and add all C++ files to it
add_library(${CMAKE_PROJECT_NAME} SHARED
${cpp_files}
)
-include(${CMAKE_SOURCE_DIR}/../nitrogen/generated/android/RNQuickSQLite+autolinking.cmake)
+include(${CMAKE_SOURCE_DIR}/../nitrogen/generated/android/RNNitroSQLite+autolinking.cmake)
# Specifies a path to native header files.
include_directories(
diff --git a/package/android/build.gradle b/package/android/build.gradle
index eaa5367..f5b8326 100644
--- a/package/android/build.gradle
+++ b/package/android/build.gradle
@@ -1,6 +1,6 @@
buildscript {
// Buildscript is evaluated before everything else so we can't use getExtOrDefault
- def kotlin_version = rootProject.ext.has("kotlinVersion") ? rootProject.ext.get("kotlinVersion") : project.properties["RNQuickSQLite_kotlinVersion"]
+ def kotlin_version = rootProject.ext.has("kotlinVersion") ? rootProject.ext.get("kotlinVersion") : project.properties["RNNitroSQLite_kotlinVersion"]
repositories {
google()
@@ -24,11 +24,11 @@ def isNewArchitectureEnabled() {
}
def getExtOrDefault(name) {
- return rootProject.ext.has(name) ? rootProject.ext.get(name) : project.properties["RNQuickSQLite_" + name]
+ return rootProject.ext.has(name) ? rootProject.ext.get(name) : project.properties["RNNitroSQLite_" + name]
}
def getExtOrIntegerDefault(name) {
- return rootProject.ext.has(name) ? rootProject.ext.get(name) : (project.properties["RNQuickSQLite_" + name]).toInteger()
+ return rootProject.ext.has(name) ? rootProject.ext.get(name) : (project.properties["RNNitroSQLite_" + name]).toInteger()
}
def safeAppExtGet(prop, fallback) {
@@ -63,7 +63,7 @@ def resolveReactNativeDirectory() {
return reactNativePackage.parentFile
}
- throw new GradleException("[react-native-quick-sqlite] Unable to resolve react-native location in node_modules. Your app should define `REACT_NATIVE_NODE_MODULES_DIR` extension property in `app/build.gradle` with a path to react-native in node_modules.")
+ throw new GradleException("[react-native-nitro-sqlite] Unable to resolve react-native location in node_modules. Your app should define `REACT_NATIVE_NODE_MODULES_DIR` extension property in `app/build.gradle` with a path to react-native in node_modules.")
}
def reactNativeRootDir = resolveReactNativeDirectory()
@@ -73,7 +73,7 @@ file("$reactNativeRootDir/ReactAndroid/gradle.properties").withInputStream { rea
def REACT_NATIVE_VERSION = reactProperties.getProperty("VERSION_NAME")
def REACT_NATIVE_MINOR_VERSION = REACT_NATIVE_VERSION.startsWith("0.0.0-") ? 1000 : REACT_NATIVE_VERSION.split("\\.")[1].toInteger()
-def SQLITE_FLAGS = rootProject.properties['quickSqliteFlags']
+def SQLITE_FLAGS = rootProject.properties['nitroSqliteFlags']
apply plugin: "com.android.library"
apply plugin: "kotlin-android"
@@ -82,11 +82,11 @@ if (isNewArchitectureEnabled()) {
apply plugin: "com.facebook.react"
}
-apply from: '../nitrogen/generated/android/RNQuickSQLite+autolinking.gradle'
+apply from: '../nitrogen/generated/android/RNNitroSQLite+autolinking.gradle'
android {
if (supportsNamespace()) {
- namespace "com.margelo.rnquicksqlite"
+ namespace "com.margelo.rnnitrosqlite"
sourceSets {
main {
@@ -193,7 +193,7 @@ dependencies {
if (isNewArchitectureEnabled()) {
react {
jsRootDir = file("../src/")
- libraryName = "RNQuickSQLite"
- codegenJavaPackageName = "com.margelo.rnquicksqlite"
+ libraryName = "RNNitroSQLite"
+ codegenJavaPackageName = "com.margelo.rnnitrosqlite"
}
}
diff --git a/package/android/cpp-adapter.cpp b/package/android/cpp-adapter.cpp
index 6ef673e..d0874bf 100644
--- a/package/android/cpp-adapter.cpp
+++ b/package/android/cpp-adapter.cpp
@@ -2,20 +2,20 @@
#include
#include
#include
-#include "HybridQuickSQLite.hpp"
-#include "RNQuickSQLiteOnLoad.hpp"
+#include "HybridNitroSQLite.hpp"
+#include "RNNitroSQLiteOnLoad.hpp"
-using namespace margelo::nitro::rnquicksqlite;
+using namespace margelo::nitro::rnnitrosqlite;
JNIEXPORT jint JNICALL JNI_OnLoad(JavaVM* vm, void*) {
- return margelo::nitro::rnquicksqlite::initialize(vm);
+ return margelo::nitro::rnnitrosqlite::initialize(vm);
}
extern "C"
JNIEXPORT void JNICALL
-Java_com_margelo_rnquicksqlite_DocPathSetter_setDocPathInJNI(JNIEnv *env, jclass clazz,
+Java_com_margelo_rnnitrosqlite_DocPathSetter_setDocPathInJNI(JNIEnv *env, jclass clazz,
jstring doc_path) {
const char *nativeString = env->GetStringUTFChars(doc_path, nullptr);
- HybridQuickSQLite::docPath = std::string(nativeString);
+ HybridNitroSQLite::docPath = std::string(nativeString);
env->ReleaseStringUTFChars(doc_path, nativeString);
}
diff --git a/package/android/gradle.properties b/package/android/gradle.properties
index d4af136..dabd683 100644
--- a/package/android/gradle.properties
+++ b/package/android/gradle.properties
@@ -1,5 +1,5 @@
-RNQuickSQLite_kotlinVersion=1.7.0
-RNQuickSQLite_minSdkVersion=21
-RNQuickSQLite_targetSdkVersion=31
-RNQuickSQLite_compileSdkVersion=31
-RNQuickSQLite_ndkversion=21.4.7075529
+RNNitroSQLite_kotlinVersion=1.7.0
+RNNitroSQLite_minSdkVersion=21
+RNNitroSQLite_targetSdkVersion=31
+RNNitroSQLite_compileSdkVersion=31
+RNNitroSQLite_ndkversion=21.4.7075529
diff --git a/package/android/src/main/AndroidManifest.xml b/package/android/src/main/AndroidManifest.xml
index 8862041..9838318 100644
--- a/package/android/src/main/AndroidManifest.xml
+++ b/package/android/src/main/AndroidManifest.xml
@@ -1,4 +1,4 @@
+ package="com.margelo.rnnitrosqlite">
diff --git a/package/android/src/main/java/com/margelo/rnquicksqlite/DocPathSetter.kt b/package/android/src/main/java/com/margelo/rnnitrosqlite/DocPathSetter.kt
similarity index 89%
rename from package/android/src/main/java/com/margelo/rnquicksqlite/DocPathSetter.kt
rename to package/android/src/main/java/com/margelo/rnnitrosqlite/DocPathSetter.kt
index c5f2c7b..a7c524a 100644
--- a/package/android/src/main/java/com/margelo/rnquicksqlite/DocPathSetter.kt
+++ b/package/android/src/main/java/com/margelo/rnnitrosqlite/DocPathSetter.kt
@@ -1,4 +1,4 @@
-package com.margelo.rnquicksqlite
+package com.margelo.rnnitrosqlite
import com.facebook.react.bridge.ReactApplicationContext
diff --git a/package/android/src/main/java/com/margelo/rnquicksqlite/RNQuickSQLitePackage.kt b/package/android/src/main/java/com/margelo/rnnitrosqlite/RNNitroSQLitePackage.kt
similarity index 71%
rename from package/android/src/main/java/com/margelo/rnquicksqlite/RNQuickSQLitePackage.kt
rename to package/android/src/main/java/com/margelo/rnnitrosqlite/RNNitroSQLitePackage.kt
index dc4ead0..2b83f70 100644
--- a/package/android/src/main/java/com/margelo/rnquicksqlite/RNQuickSQLitePackage.kt
+++ b/package/android/src/main/java/com/margelo/rnnitrosqlite/RNNitroSQLitePackage.kt
@@ -1,4 +1,4 @@
-package com.margelo.rnquicksqlite
+package com.margelo.rnnitrosqlite
import com.facebook.react.TurboReactPackage
import com.facebook.react.bridge.NativeModule
@@ -6,10 +6,10 @@ import com.facebook.react.bridge.ReactApplicationContext
import com.facebook.react.module.model.ReactModuleInfo
import com.facebook.react.module.model.ReactModuleInfoProvider
-class RNQuickSQLitePackage : TurboReactPackage() {
+class RNNitroSQLitePackage : TurboReactPackage() {
override fun getModule(name: String, reactContext: ReactApplicationContext): NativeModule? {
- return if (name == RNQuickSQLiteOnLoadModule.NAME) {
- RNQuickSQLiteOnLoadModule(reactContext)
+ return if (name == RNNitroSQLiteOnLoadModule.NAME) {
+ RNNitroSQLiteOnLoadModule(reactContext)
} else {
null
}
@@ -19,9 +19,9 @@ class RNQuickSQLitePackage : TurboReactPackage() {
return ReactModuleInfoProvider {
val moduleInfos: MutableMap = HashMap()
val isTurboModule: Boolean = BuildConfig.IS_NEW_ARCHITECTURE_ENABLED
- moduleInfos[RNQuickSQLiteOnLoadModule.NAME] = ReactModuleInfo(
- RNQuickSQLiteOnLoadModule.NAME,
- RNQuickSQLiteOnLoadModule.NAME,
+ moduleInfos[RNNitroSQLiteOnLoadModule.NAME] = ReactModuleInfo(
+ RNNitroSQLiteOnLoadModule.NAME,
+ RNNitroSQLiteOnLoadModule.NAME,
canOverrideExistingModule=false,
needsEagerInit=true,
hasConstants=true,
@@ -34,7 +34,7 @@ class RNQuickSQLitePackage : TurboReactPackage() {
companion object {
init {
- System.loadLibrary("RNQuickSQLite")
+ System.loadLibrary("RNNitroSQLite")
}
}
}
diff --git a/package/android/src/newarch/com/margelo/rnquicksqlite/RNQuickSQLiteOnLoadModule.kt b/package/android/src/newarch/com/margelo/rnnitrosqlite/RNNitroSQLiteOnLoadModule.kt
similarity index 77%
rename from package/android/src/newarch/com/margelo/rnquicksqlite/RNQuickSQLiteOnLoadModule.kt
rename to package/android/src/newarch/com/margelo/rnnitrosqlite/RNNitroSQLiteOnLoadModule.kt
index 4aade82..5b019ee 100644
--- a/package/android/src/newarch/com/margelo/rnquicksqlite/RNQuickSQLiteOnLoadModule.kt
+++ b/package/android/src/newarch/com/margelo/rnnitrosqlite/RNNitroSQLiteOnLoadModule.kt
@@ -1,11 +1,11 @@
-package com.margelo.rnquicksqlite
+package com.margelo.rnnitrosqlite
import com.facebook.react.bridge.Callback
import com.facebook.react.bridge.ReactApplicationContext
-import com.margelo.rnquicksqlite.NativeQuickSQLiteOnLoadSpec
+import com.margelo.rnnitrosqlite.NativeNitroSQLiteOnLoadSpec
-class RNQuickSQLiteOnLoadModule(reactContext: ReactApplicationContext) :
- NativeQuickSQLiteOnLoadSpec(reactContext) {
+class RNNitroSQLiteOnLoadModule(reactContext: ReactApplicationContext) :
+ NativeNitroSQLiteOnLoadSpec(reactContext) {
private var reactApplicationContextReadyCallback: Callback? = null
init {
@@ -31,7 +31,7 @@ class RNQuickSQLiteOnLoadModule(reactContext: ReactApplicationContext) :
}
companion object {
- const val NAME: String = "RNQuickSQLiteOnLoad"
+ const val NAME: String = "RNNitroSQLiteOnLoad"
var reactContext: ReactApplicationContext? = null
private set
diff --git a/package/android/src/oldarch/com/margelo/rnquicksqlite/RNQuickSQLiteOnLoadModule.kt b/package/android/src/oldarch/com/margelo/rnnitrosqlite/RNNitroSQLiteOnLoadModule.kt
similarity index 86%
rename from package/android/src/oldarch/com/margelo/rnquicksqlite/RNQuickSQLiteOnLoadModule.kt
rename to package/android/src/oldarch/com/margelo/rnnitrosqlite/RNNitroSQLiteOnLoadModule.kt
index cf1917b..0ed4019 100644
--- a/package/android/src/oldarch/com/margelo/rnquicksqlite/RNQuickSQLiteOnLoadModule.kt
+++ b/package/android/src/oldarch/com/margelo/rnnitrosqlite/RNNitroSQLiteOnLoadModule.kt
@@ -1,11 +1,11 @@
-package com.margelo.rnquicksqlite
+package com.margelo.rnnitrosqlite
import com.facebook.react.bridge.Callback
import com.facebook.react.bridge.ReactApplicationContext
import com.facebook.react.bridge.ReactContextBaseJavaModule
import com.facebook.react.bridge.ReactMethod
-class RNQuickSQLiteOnLoadModule(reactContext: ReactApplicationContext) :
+class RNNitroSQLiteOnLoadModule(reactContext: ReactApplicationContext) :
ReactContextBaseJavaModule(reactContext) {
@@ -33,7 +33,7 @@ class RNQuickSQLiteOnLoadModule(reactContext: ReactApplicationContext) :
}
companion object {
- const val NAME: String = "RNNQuickSQLiteOnLoad"
+ const val NAME: String = "RNNitroSQLiteOnLoad"
var reactContext: ReactApplicationContext? = null
private set
diff --git a/package/cpp/QuickSQLiteException.hpp b/package/cpp/NitroSQLiteException.hpp
similarity index 51%
rename from package/cpp/QuickSQLiteException.hpp
rename to package/cpp/NitroSQLiteException.hpp
index e81c7f5..e224cca 100644
--- a/package/cpp/QuickSQLiteException.hpp
+++ b/package/cpp/NitroSQLiteException.hpp
@@ -4,7 +4,7 @@
#include
#include
-enum QuickSQLiteExceptionType {
+enum NitroSQLiteExceptionType {
UnknownError,
DatabaseCannotBeOpened,
DatabaseNotOpen,
@@ -14,7 +14,7 @@ enum QuickSQLiteExceptionType {
NoBatchCommandsProvided
};
-inline std::unordered_map exceptionTypeStrings = {
+inline std::unordered_map exceptionTypeStrings = {
{UnknownError, "UnknownError"},
{DatabaseCannotBeOpened, "DatabaseCannotBeOpened"},
{DatabaseNotOpen, "DatabaseNotOpen"},
@@ -24,17 +24,17 @@ inline std::unordered_map exceptionTypeSt
{NoBatchCommandsProvided, "NoBatchCommandsProvided"}
};
-inline std::string typeToString(QuickSQLiteExceptionType type) {
+inline std::string typeToString(NitroSQLiteExceptionType type) {
return exceptionTypeStrings[type];
}
-class QuickSQLiteException : public std::exception {
+class NitroSQLiteException : public std::exception {
public:
- explicit QuickSQLiteException(const char* message): QuickSQLiteException(QuickSQLiteExceptionType::UnknownError, message) {}
- explicit QuickSQLiteException(const std::string& message): QuickSQLiteException(QuickSQLiteExceptionType::UnknownError, message) {}
- QuickSQLiteException(const QuickSQLiteExceptionType& type, const char* message) : QuickSQLiteException(type, std::string(message)) {}
- QuickSQLiteException(const QuickSQLiteExceptionType& type, const std::string& message)
- : _exceptionString("[react-native-quick-sqlite] " + typeToString(type) + ": " + message) {}
+ explicit NitroSQLiteException(const char* message): NitroSQLiteException(NitroSQLiteExceptionType::UnknownError, message) {}
+ explicit NitroSQLiteException(const std::string& message): NitroSQLiteException(NitroSQLiteExceptionType::UnknownError, message) {}
+ NitroSQLiteException(const NitroSQLiteExceptionType& type, const char* message) : NitroSQLiteException(type, std::string(message)) {}
+ NitroSQLiteException(const NitroSQLiteExceptionType& type, const std::string& message)
+ : _exceptionString("[react-native-nitro-sqlite] " + typeToString(type) + ": " + message) {}
private:
const std::string _exceptionString;
@@ -44,20 +44,20 @@ class QuickSQLiteException : public std::exception {
return this->_exceptionString.c_str();
}
- static QuickSQLiteException DatabaseNotOpen(const std::string& dbName) {
- return QuickSQLiteException(QuickSQLiteExceptionType::UnableToAttachToDatabase, dbName + " is not open");
+ static NitroSQLiteException DatabaseNotOpen(const std::string& dbName) {
+ return NitroSQLiteException(NitroSQLiteExceptionType::UnableToAttachToDatabase, dbName + " is not open");
}
- static QuickSQLiteException SqlExecution(const std::string& errorMessage) {
- return QuickSQLiteException(QuickSQLiteExceptionType::SqlExecutionError, errorMessage);
+ static NitroSQLiteException SqlExecution(const std::string& errorMessage) {
+ return NitroSQLiteException(NitroSQLiteExceptionType::SqlExecutionError, errorMessage);
}
- static QuickSQLiteException DatabaseFileNotFound(const std::string& dbFilePath) {
- return QuickSQLiteException(QuickSQLiteExceptionType::SqlExecutionError, "Database file not found: " + dbFilePath);
+ static NitroSQLiteException DatabaseFileNotFound(const std::string& dbFilePath) {
+ return NitroSQLiteException(NitroSQLiteExceptionType::SqlExecutionError, "Database file not found: " + dbFilePath);
}
- static QuickSQLiteException CouldNotLoadFile(const std::string& fileLocation, std::optional additionalLine = std::nullopt) {
+ static NitroSQLiteException CouldNotLoadFile(const std::string& fileLocation, std::optional additionalLine = std::nullopt) {
const auto message = "Could not load file: " + fileLocation + (additionalLine ? (". " + *additionalLine) : "");
- return QuickSQLiteException(QuickSQLiteExceptionType::CouldNotLoadFile, message);
+ return NitroSQLiteException(NitroSQLiteExceptionType::CouldNotLoadFile, message);
}
};
diff --git a/package/cpp/ThreadPool.cpp b/package/cpp/ThreadPool.cpp
index e9a83c8..c8da028 100644
--- a/package/cpp/ThreadPool.cpp
+++ b/package/cpp/ThreadPool.cpp
@@ -1,13 +1,13 @@
//
// ThreadPool.cpp
-// react-native-quick-sqlite
+// react-native-nitro-sqlite
//
// Created by Oscar on 13.03.22.
//
#include "ThreadPool.hpp"
-namespace margelo::rnquicksqlite {
+namespace margelo::rnnitrosqlite {
ThreadPool::ThreadPool() : done(false) {
// This returns the number of threads supported by the system. If the
@@ -88,4 +88,4 @@ void ThreadPool::waitFinished() {
workQueueConditionVariable.wait(g, [&] { return workQueue.empty() && (busy == 0); });
}
-} // namespace margelo::rnquicksqlite
+} // namespace margelo::rnnitrosqlite
diff --git a/package/cpp/ThreadPool.hpp b/package/cpp/ThreadPool.hpp
index 77e75ac..d5e3ead 100644
--- a/package/cpp/ThreadPool.hpp
+++ b/package/cpp/ThreadPool.hpp
@@ -1,6 +1,6 @@
//
// ThreadPool.hpp
-// react-native-quick-sqlite
+// react-native-nitro-sqlite
//
// Created by Oscar on 13.03.22.
//
@@ -15,7 +15,7 @@
#include
#include
-namespace margelo::rnquicksqlite {
+namespace margelo::rnnitrosqlite {
class ThreadPool {
public:
@@ -47,4 +47,4 @@ class ThreadPool {
void doWork();
};
-} // namespace margelo::rnquicksqlite
+} // namespace margelo::rnnitrosqlite
diff --git a/package/cpp/importSqlFile.cpp b/package/cpp/importSqlFile.cpp
index 6127167..f2d37f4 100644
--- a/package/cpp/importSqlFile.cpp
+++ b/package/cpp/importSqlFile.cpp
@@ -5,10 +5,10 @@
#include "importSqlFile.hpp"
#include
#include
-#include "QuickSQLiteException.hpp"
+#include "NitroSQLiteException.hpp"
#include "operations.hpp"
-namespace margelo::rnquicksqlite {
+namespace margelo::rnnitrosqlite {
SQLiteOperationResult importSqlFile(const std::string& dbName, const std::string& fileLocation) {
std::string line;
@@ -24,10 +24,10 @@ SQLiteOperationResult importSqlFile(const std::string& dbName, const std::string
SQLiteOperationResult result = sqliteExecuteLiteral(dbName, line);
rowsAffected += result.rowsAffected;
commands++;
- } catch (QuickSQLiteException& e) {
+ } catch (NitroSQLiteException& e) {
sqliteExecuteLiteral(dbName, "ROLLBACK");
sqFile.close();
- throw QuickSQLiteException::CouldNotLoadFile(fileLocation, "Transaction was rolled back");
+ throw NitroSQLiteException::CouldNotLoadFile(fileLocation, "Transaction was rolled back");
}
}
}
@@ -38,11 +38,11 @@ SQLiteOperationResult importSqlFile(const std::string& dbName, const std::string
} catch (...) {
sqFile.close();
sqliteExecuteLiteral(dbName, "ROLLBACK");
- throw QuickSQLiteException(QuickSQLiteExceptionType::UnknownError, "Unexpected error. Transaction was rolled back");
+ throw NitroSQLiteException(NitroSQLiteExceptionType::UnknownError, "Unexpected error. Transaction was rolled back");
}
} else {
- throw QuickSQLiteException::CouldNotLoadFile(fileLocation);
+ throw NitroSQLiteException::CouldNotLoadFile(fileLocation);
}
}
-} // namespace margelo::rnquicksqlite
+} // namespace margelo::rnnitrosqlite
diff --git a/package/cpp/importSqlFile.hpp b/package/cpp/importSqlFile.hpp
index 845836c..0416a10 100644
--- a/package/cpp/importSqlFile.hpp
+++ b/package/cpp/importSqlFile.hpp
@@ -8,7 +8,7 @@
#include "types.hpp"
-namespace margelo::rnquicksqlite {
+namespace margelo::rnnitrosqlite {
SQLiteOperationResult importSqlFile(const std::string& dbName, const std::string& fileLocation);
diff --git a/package/cpp/logs.hpp b/package/cpp/logs.hpp
index aaa5d6d..c603622 100644
--- a/package/cpp/logs.hpp
+++ b/package/cpp/logs.hpp
@@ -1,7 +1,7 @@
#ifdef ANDROID
// LOGS ANDROID
#include
-#define LOG_TAG "react-native-quick-sqlite"
+#define LOG_TAG "react-native-nitro-sqlite"
#define LOGV(...) __android_log_print(ANDROID_LOG_VERBOSE, LOG_TAG, __VA_ARGS__)
#define LOGD(...) __android_log_print(ANDROID_LOG_DEBUG, LOG_TAG, __VA_ARGS__)
#define LOGI(...) __android_log_print(ANDROID_LOG_INFO, LOG_TAG, __VA_ARGS__)
@@ -11,7 +11,7 @@
#else
// LOGS NO ANDROID
#include
-#define LOG_TAG "react-native-quick-sqlite"
+#define LOG_TAG "react-native-nitro-sqlite"
#define LOGV(...) \
printf(" "); \
printf(__VA_ARGS__); \
diff --git a/package/cpp/operations.cpp b/package/cpp/operations.cpp
index a4af453..0655754 100644
--- a/package/cpp/operations.cpp
+++ b/package/cpp/operations.cpp
@@ -9,13 +9,13 @@
#include
#include
#include
-#include "QuickSQLiteException.hpp"
+#include "NitroSQLiteException.hpp"
using namespace facebook;
using namespace margelo::nitro;
-using namespace margelo::nitro::rnquicksqlite;
+using namespace margelo::nitro::rnnitrosqlite;
-namespace margelo::rnquicksqlite {
+namespace margelo::rnnitrosqlite {
std::map dbMap = std::map();
@@ -29,7 +29,7 @@ void sqliteOpenDb(const std::string& dbName, const std::string& docPath) {
exit = sqlite3_open_v2(dbPath.c_str(), &db, sqlOpenFlags, nullptr);
if (exit != SQLITE_OK) {
- throw QuickSQLiteException(QuickSQLiteExceptionType::DatabaseCannotBeOpened, sqlite3_errmsg(db));
+ throw NitroSQLiteException(NitroSQLiteExceptionType::DatabaseCannotBeOpened, sqlite3_errmsg(db));
} else {
dbMap[dbName] = db;
}
@@ -38,7 +38,7 @@ void sqliteOpenDb(const std::string& dbName, const std::string& docPath) {
void sqliteCloseDb(const std::string& dbName) {
if (dbMap.count(dbName) == 0) {
- throw QuickSQLiteException::DatabaseNotOpen(dbName);
+ throw NitroSQLiteException::DatabaseNotOpen(dbName);
}
sqlite3* db = dbMap[dbName];
@@ -64,11 +64,11 @@ void sqliteAttachDb(const std::string& mainDBName, const std::string& docPath, c
* */
std::string dbPath = get_db_path(databaseToAttach, docPath);
std::string statement = "ATTACH DATABASE '" + dbPath + "' AS " + alias;
-
+
try {
sqliteExecuteLiteral(mainDBName, statement);
- } catch (QuickSQLiteException& e) {
- throw QuickSQLiteException(QuickSQLiteExceptionType::UnableToAttachToDatabase, mainDBName + " was unable to attach another database: " + std::string(e.what()));
+ } catch (NitroSQLiteException& e) {
+ throw NitroSQLiteException(NitroSQLiteExceptionType::UnableToAttachToDatabase, mainDBName + " was unable to attach another database: " + std::string(e.what()));
}
}
@@ -77,11 +77,11 @@ void sqliteDetachDb(const std::string& mainDBName, const std::string& alias) {
* There is no need to check if mainDBName is opened because sqliteExecuteLiteral will do that.
* */
std::string statement = "DETACH DATABASE " + alias;
-
+
try {
sqliteExecuteLiteral(mainDBName, statement);
- } catch (QuickSQLiteException& e) {
- throw QuickSQLiteException(QuickSQLiteExceptionType::UnableToAttachToDatabase, mainDBName + " was unable to detach database: " + std::string(e.what()));
+ } catch (NitroSQLiteException& e) {
+ throw NitroSQLiteException(NitroSQLiteExceptionType::UnableToAttachToDatabase, mainDBName + " was unable to detach database: " + std::string(e.what()));
}
}
@@ -92,7 +92,7 @@ void sqliteRemoveDb(const std::string& dbName, const std::string& docPath) {
std::string dbFilePath = get_db_path(dbName, docPath);
if (!file_exists(dbFilePath)) {
- throw QuickSQLiteException::DatabaseFileNotFound(dbFilePath);
+ throw NitroSQLiteException::DatabaseFileNotFound(dbFilePath);
}
remove(dbFilePath.c_str());
@@ -102,7 +102,6 @@ void bindStatement(sqlite3_stmt* statement, const SQLiteQueryParams& values) {
for (int valueIndex = 0; valueIndex < values.size(); valueIndex++) {
int sqliteIndex = valueIndex+1;
SQLiteValue value = values.at(valueIndex);
-
// if (std::holds_alternative(value))
// {
// sqlite3_bind_null(statement, sqliteIndex);
@@ -125,7 +124,7 @@ void bindStatement(sqlite3_stmt* statement, const SQLiteQueryParams& values) {
SQLiteExecuteQueryResult sqliteExecute(const std::string& dbName, const std::string& query, const std::optional& params) {
if (dbMap.count(dbName) == 0) {
- throw QuickSQLiteException::DatabaseNotOpen(dbName);
+ throw NitroSQLiteException::DatabaseNotOpen(dbName);
}
auto db = dbMap[dbName];
@@ -138,7 +137,7 @@ SQLiteExecuteQueryResult sqliteExecute(const std::string& dbName, const std::str
bindStatement(statement, *params);
}
} else {
- throw QuickSQLiteException::SqlExecution(sqlite3_errmsg(db));
+ throw NitroSQLiteException::SqlExecution(sqlite3_errmsg(db));
}
auto isConsuming = true;
@@ -148,7 +147,6 @@ SQLiteExecuteQueryResult sqliteExecute(const std::string& dbName, const std::str
std::string column_name;
ColumnType column_declared_type;
SQLiteQueryResultRow row;
-
auto results = std::make_unique();
auto metadata = new SQLiteQueryTableMetadata();
@@ -222,7 +220,7 @@ SQLiteExecuteQueryResult sqliteExecute(const std::string& dbName, const std::str
sqlite3_finalize(statement);
if (isFailed) {
- throw QuickSQLiteException::SqlExecution(sqlite3_errmsg(db));
+ throw NitroSQLiteException::SqlExecution(sqlite3_errmsg(db));
}
int rowsAffected = sqlite3_changes(db);
@@ -232,7 +230,6 @@ SQLiteExecuteQueryResult sqliteExecute(const std::string& dbName, const std::str
? std::make_optional(std::move(*metadata))
: std::nullopt
);
-
return {
.rowsAffected = rowsAffected,
.insertId = static_cast(latestInsertRowId),
@@ -244,7 +241,7 @@ SQLiteExecuteQueryResult sqliteExecute(const std::string& dbName, const std::str
SQLiteOperationResult sqliteExecuteLiteral(const std::string& dbName, const std::string& query) {
// Check if db connection is opened
if (dbMap.count(dbName) == 0) {
- throw QuickSQLiteException::DatabaseNotOpen(dbName);
+ throw NitroSQLiteException::DatabaseNotOpen(dbName);
}
sqlite3* db = dbMap[dbName];
@@ -257,7 +254,7 @@ SQLiteOperationResult sqliteExecuteLiteral(const std::string& dbName, const std:
if (statementStatus != SQLITE_OK) // statemnet is correct, bind the passed parameters
{
- throw QuickSQLiteException::SqlExecution(sqlite3_errmsg(db));
+ throw NitroSQLiteException::SqlExecution(sqlite3_errmsg(db));
}
bool isConsuming = true;
@@ -287,10 +284,10 @@ SQLiteOperationResult sqliteExecuteLiteral(const std::string& dbName, const std:
sqlite3_finalize(statement);
if (isFailed) {
- throw QuickSQLiteException::SqlExecution(sqlite3_errmsg(db));
+ throw NitroSQLiteException::SqlExecution(sqlite3_errmsg(db));
}
return {.rowsAffected = sqlite3_changes(db)};
}
-} // namespace margelo::rnquicksqlite
+} // namespace margelo::rnnitrosqlite
diff --git a/package/cpp/operations.hpp b/package/cpp/operations.hpp
index 1e073e6..24a2dd0 100644
--- a/package/cpp/operations.hpp
+++ b/package/cpp/operations.hpp
@@ -2,7 +2,7 @@
#include "types.hpp"
-namespace margelo::rnquicksqlite {
+namespace margelo::rnnitrosqlite {
void sqliteOpenDb(const std::string& dbName, const std::string& docPath);
@@ -21,4 +21,4 @@ SQLiteOperationResult sqliteExecuteLiteral(const std::string& dbName, const std:
void sqliteCloseAll();
-} // namespace margelo::rnquicksqlite
+} // namespace margelo::rnnitrosqlite
diff --git a/package/cpp/specs/HybridNativeQueryResult.cpp b/package/cpp/specs/HybridNativeQueryResult.cpp
index bd447a8..65e8a5b 100644
--- a/package/cpp/specs/HybridNativeQueryResult.cpp
+++ b/package/cpp/specs/HybridNativeQueryResult.cpp
@@ -1,6 +1,6 @@
#include "HybridNativeQueryResult.hpp"
-namespace margelo::nitro::rnquicksqlite {
+namespace margelo::nitro::rnnitrosqlite {
std::optional HybridNativeQueryResult::getInsertId() {
return this->_insertId;
@@ -17,4 +17,4 @@ std::optional HybridNativeQueryResult::getMetadata() {
return this->_metadata;
}
-} // namespace margelo::nitro::rnquicksqlite
+} // namespace margelo::nitro::rnnitrosqlite
diff --git a/package/cpp/specs/HybridNativeQueryResult.hpp b/package/cpp/specs/HybridNativeQueryResult.hpp
index c1b9d9a..b792b7e 100644
--- a/package/cpp/specs/HybridNativeQueryResult.hpp
+++ b/package/cpp/specs/HybridNativeQueryResult.hpp
@@ -4,9 +4,15 @@
#include "types.hpp"
#include