diff --git a/README.md b/README.md
index cb345cf..45462cf 100644
--- a/README.md
+++ b/README.md
@@ -1,5 +1,5 @@
-# Terminal2 - v1.0.3.2
-## !Quite usable now!
+# Terminal2 - v1.0.4.0
+## !With better accuracy on timestamps
Terminal emulator for Embedded Engineers with Serial and TCP socket capabilities
@@ -19,7 +19,7 @@ With Terminal2, you can define colorful profiles and quickly switch between sess
Terminal Emulator, Baud rate, Parity, Stop bits, Handshaking, TCP Client, TCP Server, ASCII, HEX, Line feed, Carriage return, Log files, Profiles, Fonts, Macros
## Building Terminal2
-1. Windows 10, 11 with .NET 4.6.1 or later
+1. Windows 10, 11 with .NET 4.7.2 or later
2. Visual Studio (2019 or later) with C#.
3. Load the solution (.sln file).
4. Build (Debug or Release).
@@ -33,8 +33,8 @@ Here is an example from Scotty's emulator on Enterprise (NCC-1701-B)
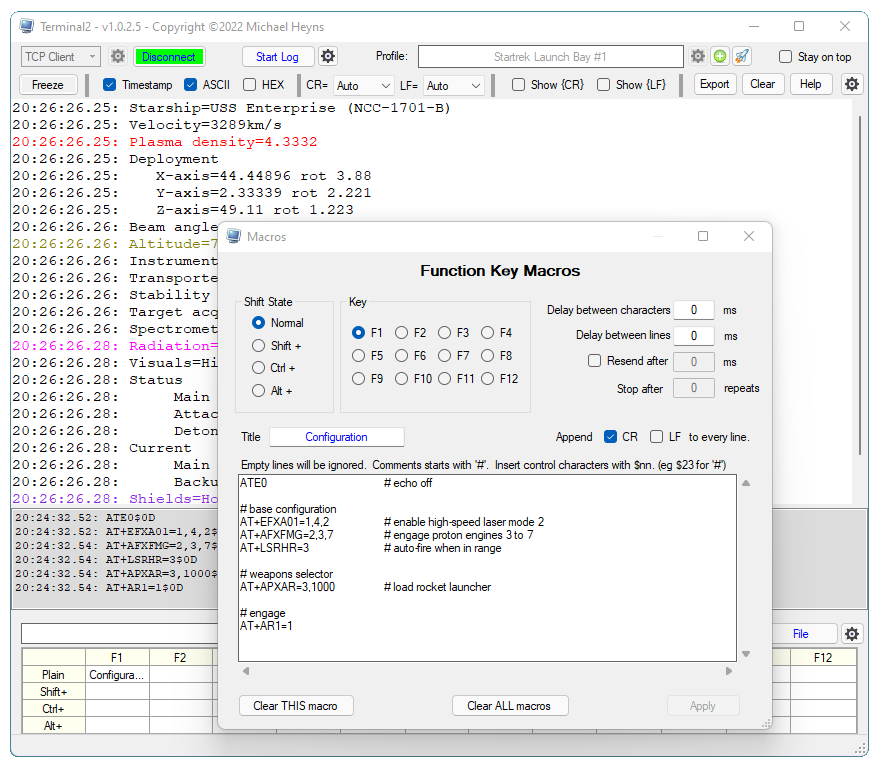
### The name
-The name "Terminal2" was chosen to allow existing users of the very useful (but unmaintained) "Terminal" program (by Br@dy++) to adapt quickly.
+The name "Terminal2" was chosen to allow existing users of the very useful (now unmaintained) "Terminal" program to adapt quickly.
It will definitely change some time in the future.
## Feedback
-Constructive feedback welcome.
+Constructive feedback very welcome.
diff --git a/Terminal2/Comms.cs b/Terminal2/Comms.cs
index 3aa4eb1..1a34029 100644
--- a/Terminal2/Comms.cs
+++ b/Terminal2/Comms.cs
@@ -26,11 +26,146 @@ internal class Comms
private TcpClient _client = null;
private Socket _socket = null;
private Profile _profile;
+ private Embelishments _embelishments;
private Thread _tcpThread = null;
private Thread _serialThread = null;
private static int _localThreadCount = 0;
+ private bool _ignoreNextLF = false;
+ private bool _showTimestamp = false;
+ private int _hexColumn = 0;
+
+ private void Enqueue(string str)
+ {
+ string embelished = string.Empty;
+
+ foreach (char c in str)
+ {
+ if (c == '\r')
+ {
+ if (_embelishments.ShowASCII)
+ {
+ if (_showTimestamp)
+ {
+ embelished += Utils.Timestamp();
+ _showTimestamp = false;
+ }
+
+ if (_embelishments.ShowCR)
+ embelished += "{CR}";
+
+ embelished += "\n";
+ }
+
+ if (_embelishments.ShowHEX)
+ {
+ if (!_embelishments.ShowASCII && _hexColumn == 0)
+ embelished += Utils.Timestamp();
+
+ byte b = Convert.ToByte(c);
+ embelished += $"{b:X2} ";
+
+ if (!_embelishments.ShowASCII)
+ {
+ _hexColumn++;
+ if (_hexColumn == 8 || _hexColumn == 16 || _hexColumn == 24)
+ {
+ embelished += " - ";
+ }
+ else if (_hexColumn == 32)
+ {
+ embelished += "\n";
+ _hexColumn = 0;
+ }
+ }
+ }
+
+ _ignoreNextLF = true;
+
+ if (_embelishments.ShowTimestamp)
+ _showTimestamp = true;
+ }
+ else if (c == '\n')
+ {
+ if (_embelishments.ShowASCII && _embelishments.ShowLF)
+ embelished += "{LF}";
+
+ if (!_ignoreNextLF)
+ {
+ if (_embelishments.ShowASCII)
+ {
+ if (_showTimestamp)
+ {
+ embelished += Utils.Timestamp();
+ _showTimestamp = false;
+ }
+ embelished += "\n";
+ }
+ }
+ if (_embelishments.ShowHEX)
+ {
+ if (!_embelishments.ShowASCII && _hexColumn == 0)
+ embelished += Utils.Timestamp();
+
+ byte b = Convert.ToByte(c);
+ embelished += $"{b:X2} ";
+
+ if (!_embelishments.ShowASCII)
+ {
+ _hexColumn++;
+ if (_hexColumn == 8 || _hexColumn == 16 || _hexColumn == 24)
+ {
+ embelished += " - ";
+ }
+ else if (_hexColumn == 32)
+ {
+ embelished += "\n";
+ _hexColumn = 0;
+ }
+ }
+ }
+ _ignoreNextLF = false;
+ }
+ else
+ {
+ if (_embelishments.ShowASCII && _showTimestamp)
+ {
+ embelished += Utils.Timestamp();
+ _showTimestamp = false;
+ }
+
+ if (_embelishments.ShowASCII)
+ embelished += c;
+
+ if (_embelishments.ShowHEX)
+ {
+ if (!_embelishments.ShowASCII && _hexColumn == 0)
+ embelished += Utils.Timestamp();
+
+ byte b = Convert.ToByte(c);
+ embelished += $"{b:X2} ";
+
+ if (!_embelishments.ShowASCII)
+ {
+ _hexColumn++;
+ if (_hexColumn == 8 || _hexColumn == 16 || _hexColumn == 24)
+ {
+ embelished += " - ";
+ }
+ else if (_hexColumn == 32)
+ {
+ embelished += "\n";
+ _hexColumn = 0;
+ }
+ }
+ }
+ _ignoreNextLF = false;
+ }
+ }
+ _inputQueue.Enqueue(embelished);
+ }
+
private void SerialReaderThread()
{
_localThreadCount++;
@@ -42,7 +177,7 @@ private void SerialReaderThread()
if (count > 0)
{
string str = _com.ReadExisting();
- _inputQueue.Enqueue(str);
+ Enqueue(str);
}
else
{
@@ -74,7 +209,7 @@ private void TCPReaderThread()
_socket.Receive(tcp_data);
str = System.Text.Encoding.Default.GetString(tcp_data);
}
- _inputQueue.Enqueue(str);
+ Enqueue(str);
}
else
{
@@ -97,6 +232,11 @@ public Comms(Label[] leds, Label[] controls, EventQueue inputQueue)
control.Visible = false;
}
+ public void SetEmbelishments(Embelishments embelishments)
+ {
+ _embelishments = embelishments;
+ }
+
private enum CommType
{ cNONE, ctSERIAL, ctSERVER, ctCLIENT };
diff --git a/Terminal2/Database.cs b/Terminal2/Database.cs
index b45578e..5f0407a 100644
--- a/Terminal2/Database.cs
+++ b/Terminal2/Database.cs
@@ -165,20 +165,20 @@ public static bool SaveProfile(Profile profile, string filename)
data += "[Profile]\n";
data += $"Name={profile.name}\n";
- data += $"ShowCR={profile.showCurlyCR}\n";
- data += $"ShowLF={profile.showCurlyLF}\n";
- data += $"ShowASCII={profile.ascii}\n";
- data += $"TranslateCR={profile.translateCR}\n";
- data += $"TranslateLF={profile.translateLF}\n";
data += $"SendCR={profile.sendCR}\n";
data += $"SendLF={profile.sendLF}\n";
data += $"ClearCMD={profile.clearCMD}\n";
data += $"OnTop={profile.stayontop}\n";
- data += $"TimeInput={profile.timestampInput}\n";
data += $"TimeOutput={profile.displayOptions.timestampOutputLines}\n";
data += $"MaxLines={profile.displayOptions.maxLines}\n";
data += $"CutLines={profile.displayOptions.cutXtraLines}\n";
+ data += $"ShowCR={profile.embelishments.ShowCR}\n";
+ data += $"ShowLF={profile.embelishments.ShowLF}\n";
+ data += $"ShowASCII={profile.embelishments.ShowASCII}\n";
+ data += $"ShowHEX={profile.embelishments.ShowHEX}\n";
+ data += $"TimeInput={profile.embelishments.ShowTimestamp}\n";
+
if (!Directory.Exists(profile.logOptions.Directory))
{
Directory.CreateDirectory(profile.logOptions.Directory);
@@ -325,26 +325,6 @@ public static Profile ReadProfile(string name)
{
profile.name = line.Substring(5);
}
- else if (line.StartsWith("ShowCR="))
- {
- profile.showCurlyCR = line.Contains("True");
- }
- else if (line.StartsWith("ShowLF="))
- {
- profile.showCurlyLF = line.Contains("True");
- }
- else if (line.StartsWith("ShowASCII="))
- {
- profile.ascii = line.Contains("True");
- }
- else if (line.StartsWith("TranslateCR="))
- {
- profile.translateCR = Utils.Int(line.Substring(12));
- }
- else if (line.StartsWith("TranslateLF="))
- {
- profile.translateLF = Utils.Int(line.Substring(12));
- }
else if (line.StartsWith("SendCR="))
{
profile.sendCR = line.Contains("True");
@@ -361,9 +341,25 @@ public static Profile ReadProfile(string name)
{
profile.stayontop = line.Contains("True");
}
+ else if (line.StartsWith("ShowCR="))
+ {
+ profile.embelishments.ShowCR = line.Contains("True");
+ }
+ else if (line.StartsWith("ShowLF="))
+ {
+ profile.embelishments.ShowLF = line.Contains("True");
+ }
+ else if (line.StartsWith("ShowASCII="))
+ {
+ profile.embelishments.ShowASCII = line.Contains("True");
+ }
+ else if (line.StartsWith("ShowHEX="))
+ {
+ profile.embelishments.ShowHEX = line.Contains("True");
+ }
else if (line.StartsWith("TimeInput="))
{
- profile.timestampInput = line.Contains("True");
+ profile.embelishments.ShowTimestamp = line.Contains("True");
}
else if (line.StartsWith("OutFont="))
{
diff --git a/Terminal2/FrmDisplayOptions.Designer.cs b/Terminal2/FrmDisplayOptions.Designer.cs
index 8916376..42c8a4e 100644
--- a/Terminal2/FrmDisplayOptions.Designer.cs
+++ b/Terminal2/FrmDisplayOptions.Designer.cs
@@ -36,6 +36,19 @@ private void InitializeComponent()
this.btnOk = new System.Windows.Forms.Button();
this.btnSelectFontInput = new System.Windows.Forms.Button();
this.grpColourFilters = new System.Windows.Forms.GroupBox();
+ this.label13 = new System.Windows.Forms.Label();
+ this.e2 = new System.Windows.Forms.CheckBox();
+ this.e6 = new System.Windows.Forms.CheckBox();
+ this.e12 = new System.Windows.Forms.CheckBox();
+ this.e8 = new System.Windows.Forms.CheckBox();
+ this.e9 = new System.Windows.Forms.CheckBox();
+ this.e1 = new System.Windows.Forms.CheckBox();
+ this.e7 = new System.Windows.Forms.CheckBox();
+ this.e3 = new System.Windows.Forms.CheckBox();
+ this.e11 = new System.Windows.Forms.CheckBox();
+ this.e10 = new System.Windows.Forms.CheckBox();
+ this.e4 = new System.Windows.Forms.CheckBox();
+ this.e5 = new System.Windows.Forms.CheckBox();
this.pictureBox1 = new System.Windows.Forms.PictureBox();
this.label18 = new System.Windows.Forms.Label();
this.label23 = new System.Windows.Forms.Label();
@@ -116,19 +129,6 @@ private void InitializeComponent()
this.label11 = new System.Windows.Forms.Label();
this.label10 = new System.Windows.Forms.Label();
this.textBox1 = new System.Windows.Forms.TextBox();
- this.label13 = new System.Windows.Forms.Label();
- this.e2 = new System.Windows.Forms.CheckBox();
- this.e6 = new System.Windows.Forms.CheckBox();
- this.e12 = new System.Windows.Forms.CheckBox();
- this.e8 = new System.Windows.Forms.CheckBox();
- this.e9 = new System.Windows.Forms.CheckBox();
- this.e1 = new System.Windows.Forms.CheckBox();
- this.e7 = new System.Windows.Forms.CheckBox();
- this.e3 = new System.Windows.Forms.CheckBox();
- this.e11 = new System.Windows.Forms.CheckBox();
- this.e10 = new System.Windows.Forms.CheckBox();
- this.e4 = new System.Windows.Forms.CheckBox();
- this.e5 = new System.Windows.Forms.CheckBox();
this.grpColourFilters.SuspendLayout();
((System.ComponentModel.ISupportInitialize)(this.pictureBox1)).BeginInit();
this.groupBox2.SuspendLayout();
@@ -147,7 +147,7 @@ private void InitializeComponent()
this.label1.Font = new System.Drawing.Font("Microsoft Sans Serif", 9.75F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label1.Location = new System.Drawing.Point(250, 9);
this.label1.Name = "label1";
- this.label1.Size = new System.Drawing.Size(118, 16);
+ this.label1.Size = new System.Drawing.Size(117, 16);
this.label1.TabIndex = 9;
this.label1.Text = "Display Options";
//
@@ -250,6 +250,147 @@ private void InitializeComponent()
this.grpColourFilters.TabStop = false;
this.grpColourFilters.Text = "Colored Text Filters";
//
+ // label13
+ //
+ this.label13.AutoSize = true;
+ this.label13.Location = new System.Drawing.Point(288, 38);
+ this.label13.Name = "label13";
+ this.label13.Size = new System.Drawing.Size(39, 13);
+ this.label13.TabIndex = 97;
+ this.label13.Text = "Freeze";
+ //
+ // e2
+ //
+ this.e2.AutoSize = true;
+ this.e2.Location = new System.Drawing.Point(298, 82);
+ this.e2.Name = "e2";
+ this.e2.Size = new System.Drawing.Size(15, 14);
+ this.e2.TabIndex = 87;
+ this.e2.Tag = "1";
+ this.e2.UseVisualStyleBackColor = true;
+ this.e2.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
+ // e6
+ //
+ this.e6.AutoSize = true;
+ this.e6.Location = new System.Drawing.Point(298, 186);
+ this.e6.Name = "e6";
+ this.e6.Size = new System.Drawing.Size(15, 14);
+ this.e6.TabIndex = 90;
+ this.e6.Tag = "5";
+ this.e6.UseVisualStyleBackColor = true;
+ this.e6.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
+ // e12
+ //
+ this.e12.AutoSize = true;
+ this.e12.Location = new System.Drawing.Point(298, 342);
+ this.e12.Name = "e12";
+ this.e12.Size = new System.Drawing.Size(15, 14);
+ this.e12.TabIndex = 96;
+ this.e12.Tag = "11";
+ this.e12.UseVisualStyleBackColor = true;
+ this.e12.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
+ // e8
+ //
+ this.e8.AutoSize = true;
+ this.e8.Location = new System.Drawing.Point(298, 238);
+ this.e8.Name = "e8";
+ this.e8.Size = new System.Drawing.Size(15, 14);
+ this.e8.TabIndex = 92;
+ this.e8.Tag = "7";
+ this.e8.UseVisualStyleBackColor = true;
+ this.e8.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
+ // e9
+ //
+ this.e9.AutoSize = true;
+ this.e9.Location = new System.Drawing.Point(298, 264);
+ this.e9.Name = "e9";
+ this.e9.Size = new System.Drawing.Size(15, 14);
+ this.e9.TabIndex = 93;
+ this.e9.Tag = "8";
+ this.e9.UseVisualStyleBackColor = true;
+ this.e9.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
+ // e1
+ //
+ this.e1.AutoSize = true;
+ this.e1.Location = new System.Drawing.Point(298, 57);
+ this.e1.Name = "e1";
+ this.e1.Size = new System.Drawing.Size(15, 14);
+ this.e1.TabIndex = 85;
+ this.e1.Tag = "0";
+ this.e1.UseVisualStyleBackColor = true;
+ this.e1.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
+ // e7
+ //
+ this.e7.AutoSize = true;
+ this.e7.Location = new System.Drawing.Point(298, 212);
+ this.e7.Name = "e7";
+ this.e7.Size = new System.Drawing.Size(15, 14);
+ this.e7.TabIndex = 91;
+ this.e7.Tag = "6";
+ this.e7.UseVisualStyleBackColor = true;
+ this.e7.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
+ // e3
+ //
+ this.e3.AutoSize = true;
+ this.e3.Location = new System.Drawing.Point(298, 107);
+ this.e3.Name = "e3";
+ this.e3.Size = new System.Drawing.Size(15, 14);
+ this.e3.TabIndex = 86;
+ this.e3.Tag = "2";
+ this.e3.UseVisualStyleBackColor = true;
+ this.e3.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
+ // e11
+ //
+ this.e11.AutoSize = true;
+ this.e11.Location = new System.Drawing.Point(298, 316);
+ this.e11.Name = "e11";
+ this.e11.Size = new System.Drawing.Size(15, 14);
+ this.e11.TabIndex = 95;
+ this.e11.Tag = "10";
+ this.e11.UseVisualStyleBackColor = true;
+ this.e11.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
+ // e10
+ //
+ this.e10.AutoSize = true;
+ this.e10.Location = new System.Drawing.Point(298, 290);
+ this.e10.Name = "e10";
+ this.e10.Size = new System.Drawing.Size(15, 14);
+ this.e10.TabIndex = 94;
+ this.e10.Tag = "9";
+ this.e10.UseVisualStyleBackColor = true;
+ this.e10.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
+ // e4
+ //
+ this.e4.AutoSize = true;
+ this.e4.Location = new System.Drawing.Point(298, 133);
+ this.e4.Name = "e4";
+ this.e4.Size = new System.Drawing.Size(15, 14);
+ this.e4.TabIndex = 88;
+ this.e4.Tag = "3";
+ this.e4.UseVisualStyleBackColor = true;
+ this.e4.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
+ // e5
+ //
+ this.e5.AutoSize = true;
+ this.e5.Location = new System.Drawing.Point(298, 159);
+ this.e5.Name = "e5";
+ this.e5.Size = new System.Drawing.Size(15, 14);
+ this.e5.TabIndex = 89;
+ this.e5.Tag = "4";
+ this.e5.UseVisualStyleBackColor = true;
+ this.e5.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
+ //
// pictureBox1
//
this.pictureBox1.Image = ((System.Drawing.Image)(resources.GetObject("pictureBox1.Image")));
@@ -1123,147 +1264,6 @@ private void InitializeComponent()
this.textBox1.TabIndex = 0;
this.textBox1.Text = resources.GetString("textBox1.Text");
//
- // label13
- //
- this.label13.AutoSize = true;
- this.label13.Location = new System.Drawing.Point(288, 38);
- this.label13.Name = "label13";
- this.label13.Size = new System.Drawing.Size(39, 13);
- this.label13.TabIndex = 97;
- this.label13.Text = "Freeze";
- //
- // e2
- //
- this.e2.AutoSize = true;
- this.e2.Location = new System.Drawing.Point(298, 82);
- this.e2.Name = "e2";
- this.e2.Size = new System.Drawing.Size(15, 14);
- this.e2.TabIndex = 87;
- this.e2.Tag = "1";
- this.e2.UseVisualStyleBackColor = true;
- this.e2.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
- // e6
- //
- this.e6.AutoSize = true;
- this.e6.Location = new System.Drawing.Point(298, 186);
- this.e6.Name = "e6";
- this.e6.Size = new System.Drawing.Size(15, 14);
- this.e6.TabIndex = 90;
- this.e6.Tag = "5";
- this.e6.UseVisualStyleBackColor = true;
- this.e6.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
- // e12
- //
- this.e12.AutoSize = true;
- this.e12.Location = new System.Drawing.Point(298, 342);
- this.e12.Name = "e12";
- this.e12.Size = new System.Drawing.Size(15, 14);
- this.e12.TabIndex = 96;
- this.e12.Tag = "11";
- this.e12.UseVisualStyleBackColor = true;
- this.e12.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
- // e8
- //
- this.e8.AutoSize = true;
- this.e8.Location = new System.Drawing.Point(298, 238);
- this.e8.Name = "e8";
- this.e8.Size = new System.Drawing.Size(15, 14);
- this.e8.TabIndex = 92;
- this.e8.Tag = "7";
- this.e8.UseVisualStyleBackColor = true;
- this.e8.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
- // e9
- //
- this.e9.AutoSize = true;
- this.e9.Location = new System.Drawing.Point(298, 264);
- this.e9.Name = "e9";
- this.e9.Size = new System.Drawing.Size(15, 14);
- this.e9.TabIndex = 93;
- this.e9.Tag = "8";
- this.e9.UseVisualStyleBackColor = true;
- this.e9.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
- // e1
- //
- this.e1.AutoSize = true;
- this.e1.Location = new System.Drawing.Point(298, 57);
- this.e1.Name = "e1";
- this.e1.Size = new System.Drawing.Size(15, 14);
- this.e1.TabIndex = 85;
- this.e1.Tag = "0";
- this.e1.UseVisualStyleBackColor = true;
- this.e1.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
- // e7
- //
- this.e7.AutoSize = true;
- this.e7.Location = new System.Drawing.Point(298, 212);
- this.e7.Name = "e7";
- this.e7.Size = new System.Drawing.Size(15, 14);
- this.e7.TabIndex = 91;
- this.e7.Tag = "6";
- this.e7.UseVisualStyleBackColor = true;
- this.e7.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
- // e3
- //
- this.e3.AutoSize = true;
- this.e3.Location = new System.Drawing.Point(298, 107);
- this.e3.Name = "e3";
- this.e3.Size = new System.Drawing.Size(15, 14);
- this.e3.TabIndex = 86;
- this.e3.Tag = "2";
- this.e3.UseVisualStyleBackColor = true;
- this.e3.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
- // e11
- //
- this.e11.AutoSize = true;
- this.e11.Location = new System.Drawing.Point(298, 316);
- this.e11.Name = "e11";
- this.e11.Size = new System.Drawing.Size(15, 14);
- this.e11.TabIndex = 95;
- this.e11.Tag = "10";
- this.e11.UseVisualStyleBackColor = true;
- this.e11.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
- // e10
- //
- this.e10.AutoSize = true;
- this.e10.Location = new System.Drawing.Point(298, 290);
- this.e10.Name = "e10";
- this.e10.Size = new System.Drawing.Size(15, 14);
- this.e10.TabIndex = 94;
- this.e10.Tag = "9";
- this.e10.UseVisualStyleBackColor = true;
- this.e10.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
- // e4
- //
- this.e4.AutoSize = true;
- this.e4.Location = new System.Drawing.Point(298, 133);
- this.e4.Name = "e4";
- this.e4.Size = new System.Drawing.Size(15, 14);
- this.e4.TabIndex = 88;
- this.e4.Tag = "3";
- this.e4.UseVisualStyleBackColor = true;
- this.e4.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
- // e5
- //
- this.e5.AutoSize = true;
- this.e5.Location = new System.Drawing.Point(298, 159);
- this.e5.Name = "e5";
- this.e5.Size = new System.Drawing.Size(15, 14);
- this.e5.TabIndex = 89;
- this.e5.Tag = "4";
- this.e5.UseVisualStyleBackColor = true;
- this.e5.CheckedChanged += new System.EventHandler(this.E1_CheckedChanged);
- //
// FrmDisplayOptions
//
this.AcceptButton = this.btnOk;
diff --git a/Terminal2/FrmDisplayOptions.resx b/Terminal2/FrmDisplayOptions.resx
index e218d46..54dd1f8 100644
--- a/Terminal2/FrmDisplayOptions.resx
+++ b/Terminal2/FrmDisplayOptions.resx
@@ -136,7 +136,11 @@
- v1.0.3.0
+ v1.0.4.0
+ * Moved timestamping to the point of entry of serial and TCP data. Much more accurate now.
+ * Other embelishments done at the point of entry as well. Faster and cleaner solution.
+
+v1.0.3.0
* Major re-write of the display and comms processes
v1.0.2.9
@@ -189,16 +193,6 @@ v1.0.1.0
* Added 'Export' to *.rtf/*.txt option. (.RTF files retain colors information and are opened by Word).
* Pressing 'Escape' will close a dialog box
* Ability to freeze the display when a specified key word is detected
-
-Beta-13
- * Added more baud rate constants
-
-Beta-12
- * Increased the Rx and Tx buffer sizes. (It was missing data).
- * Added feature to set initial RTS and DTR states
- * Fixed bug when clicking on HISTORY line
- * Serial and Tx and Rx timeouts added - else it may sit forever waiting on the port
- * Eliminated the send queue and put straight into send buffer - faster response on sending
diff --git a/Terminal2/FrmHelp.resx b/Terminal2/FrmHelp.resx
index c61a230..25efe14 100644
--- a/Terminal2/FrmHelp.resx
+++ b/Terminal2/FrmHelp.resx
@@ -118,7 +118,22 @@
System.Resources.ResXResourceWriter, System.Windows.Forms, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089
- Profiles
+ Rules for EOL (End-Of-Line)
+ 1) xxxxx[CR]xxxxx --> xxxxx[EOL] + xxxxx
+ 2) xxxxx[LF]xxxxx --> xxxxx[EOL] + xxxxx
+ 3) xxxxx[CR][LF]xxxxx --> xxxxx[EOL] + xxxxx
+
+Rules for {CR} and {LF} embelishments
+ 1) xxxxx[CR]xxxxx --> xxxxx{CR}[EOL] + xxxxx
+ 2) xxxxx[LF]xxxxx --> xxxxx{LF}[EOL] + xxxxx
+ 3) xxxxx[CR][LF]xxxxx --> xxxxx{CR}[EOL]{LF} + xxxxx
+
+Rules for Timestamping
+ 1) xxxxx[CR]xxxxx --> xxxxx[EOL] + [Timestamp]xxxxx
+ 2) xxxxx[LF]xxxxx --> xxxxx[EOL] + [Timestamp]xxxxx
+ 3) xxxxx[CR][LF]xxxxx --> xxxxx[EOL] + [Timestamp]xxxxx
+
+Profiles
1) Profiles remember all the settings and colour options
2) The profile database is automatically updated when the program exits
3) All changes made will be saved under the current profile
@@ -126,13 +141,6 @@
Options
1) Click the 'gear' icon to edit the options for a particular section
-CR-LF:
- 1) "Auto" should satisfy most needs
- ttttt CR tttt
- ttttt LF tttt
- ttttt CR LF tttt
- are treated the same for display purposes
-
Input Panel:
1) You may select a section of text with the mouse
2) Drag over an area to select text. Copy with Ctrl-C or right-click
diff --git a/Terminal2/FrmMain.Designer.cs b/Terminal2/FrmMain.Designer.cs
index b546e94..25e5e55 100644
--- a/Terminal2/FrmMain.Designer.cs
+++ b/Terminal2/FrmMain.Designer.cs
@@ -30,19 +30,19 @@ private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
System.ComponentModel.ComponentResourceManager resources = new System.ComponentModel.ComponentResourceManager(typeof(FrmMain));
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle64 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle65 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle53 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle54 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle55 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle56 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle57 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle58 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle59 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle60 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle61 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle62 = new System.Windows.Forms.DataGridViewCellStyle();
- System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle63 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle25 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle26 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle14 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle15 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle16 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle17 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle18 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle19 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle20 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle21 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle22 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle23 = new System.Windows.Forms.DataGridViewCellStyle();
+ System.Windows.Forms.DataGridViewCellStyle dataGridViewCellStyle24 = new System.Windows.Forms.DataGridViewCellStyle();
this.statusStrip1 = new System.Windows.Forms.StatusStrip();
this.blank2 = new System.Windows.Forms.ToolStripStatusLabel();
this.stsConnectionDetail = new System.Windows.Forms.ToolStripStatusLabel();
@@ -92,14 +92,9 @@ private void InitializeComponent()
this.ctrlTwo = new System.Windows.Forms.Label();
this.lbOutput = new System.Windows.Forms.ListBox();
this.PanelTwo = new System.Windows.Forms.Panel();
- this.lbTranslateLF = new System.Windows.Forms.ComboBox();
- this.lbTranslateCR = new System.Windows.Forms.ComboBox();
- this.label3 = new System.Windows.Forms.Label();
this.cbFreeze = new System.Windows.Forms.CheckBox();
this.sep1 = new System.Windows.Forms.Panel();
- this.label2 = new System.Windows.Forms.Label();
this.panel3 = new System.Windows.Forms.Panel();
- this.panel2 = new System.Windows.Forms.Panel();
this.panel1 = new System.Windows.Forms.Panel();
this.btnExport = new System.Windows.Forms.Button();
this.btnHelp = new System.Windows.Forms.Button();
@@ -265,7 +260,7 @@ private void InitializeComponent()
this.lblSaving.ForeColor = System.Drawing.Color.Red;
this.lblSaving.Location = new System.Drawing.Point(819, 10);
this.lblSaving.Name = "lblSaving";
- this.lblSaving.Size = new System.Drawing.Size(14, 16);
+ this.lblSaving.Size = new System.Drawing.Size(13, 16);
this.lblSaving.TabIndex = 24;
this.lblSaving.Text = "*";
this.lblSaving.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
@@ -418,14 +413,14 @@ private void InitializeComponent()
this.scF11,
this.scF12});
this.dgMacroTable.Cursor = System.Windows.Forms.Cursors.Default;
- dataGridViewCellStyle64.Alignment = System.Windows.Forms.DataGridViewContentAlignment.MiddleCenter;
- dataGridViewCellStyle64.BackColor = System.Drawing.SystemColors.Window;
- dataGridViewCellStyle64.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
- dataGridViewCellStyle64.ForeColor = System.Drawing.SystemColors.ControlText;
- dataGridViewCellStyle64.SelectionBackColor = System.Drawing.SystemColors.Highlight;
- dataGridViewCellStyle64.SelectionForeColor = System.Drawing.SystemColors.HighlightText;
- dataGridViewCellStyle64.WrapMode = System.Windows.Forms.DataGridViewTriState.False;
- this.dgMacroTable.DefaultCellStyle = dataGridViewCellStyle64;
+ dataGridViewCellStyle25.Alignment = System.Windows.Forms.DataGridViewContentAlignment.MiddleCenter;
+ dataGridViewCellStyle25.BackColor = System.Drawing.SystemColors.Window;
+ dataGridViewCellStyle25.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
+ dataGridViewCellStyle25.ForeColor = System.Drawing.SystemColors.ControlText;
+ dataGridViewCellStyle25.SelectionBackColor = System.Drawing.SystemColors.Highlight;
+ dataGridViewCellStyle25.SelectionForeColor = System.Drawing.SystemColors.HighlightText;
+ dataGridViewCellStyle25.WrapMode = System.Windows.Forms.DataGridViewTriState.False;
+ this.dgMacroTable.DefaultCellStyle = dataGridViewCellStyle25;
this.dgMacroTable.Dock = System.Windows.Forms.DockStyle.Top;
this.dgMacroTable.EditMode = System.Windows.Forms.DataGridViewEditMode.EditProgrammatically;
this.dgMacroTable.Location = new System.Drawing.Point(10, 0);
@@ -434,8 +429,8 @@ private void InitializeComponent()
this.dgMacroTable.ReadOnly = true;
this.dgMacroTable.RowHeadersVisible = false;
this.dgMacroTable.RowHeadersWidthSizeMode = System.Windows.Forms.DataGridViewRowHeadersWidthSizeMode.DisableResizing;
- dataGridViewCellStyle65.Alignment = System.Windows.Forms.DataGridViewContentAlignment.MiddleCenter;
- this.dgMacroTable.RowsDefaultCellStyle = dataGridViewCellStyle65;
+ dataGridViewCellStyle26.Alignment = System.Windows.Forms.DataGridViewContentAlignment.MiddleCenter;
+ this.dgMacroTable.RowsDefaultCellStyle = dataGridViewCellStyle26;
this.dgMacroTable.RowTemplate.ReadOnly = true;
this.dgMacroTable.ScrollBars = System.Windows.Forms.ScrollBars.None;
this.dgMacroTable.SelectionMode = System.Windows.Forms.DataGridViewSelectionMode.CellSelect;
@@ -459,80 +454,80 @@ private void InitializeComponent()
//
// F1
//
- dataGridViewCellStyle53.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
- this.F1.DefaultCellStyle = dataGridViewCellStyle53;
+ dataGridViewCellStyle14.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
+ this.F1.DefaultCellStyle = dataGridViewCellStyle14;
this.F1.HeaderText = "F1";
this.F1.Name = "F1";
this.F1.ReadOnly = true;
//
// scF2
//
- dataGridViewCellStyle54.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
- this.scF2.DefaultCellStyle = dataGridViewCellStyle54;
+ dataGridViewCellStyle15.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
+ this.scF2.DefaultCellStyle = dataGridViewCellStyle15;
this.scF2.HeaderText = "F2";
this.scF2.Name = "scF2";
this.scF2.ReadOnly = true;
//
// scF3
//
- dataGridViewCellStyle55.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
- this.scF3.DefaultCellStyle = dataGridViewCellStyle55;
+ dataGridViewCellStyle16.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
+ this.scF3.DefaultCellStyle = dataGridViewCellStyle16;
this.scF3.HeaderText = "F3";
this.scF3.Name = "scF3";
this.scF3.ReadOnly = true;
//
// scF4
//
- dataGridViewCellStyle56.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
- this.scF4.DefaultCellStyle = dataGridViewCellStyle56;
+ dataGridViewCellStyle17.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
+ this.scF4.DefaultCellStyle = dataGridViewCellStyle17;
this.scF4.HeaderText = "F4";
this.scF4.Name = "scF4";
this.scF4.ReadOnly = true;
//
// scF5
//
- dataGridViewCellStyle57.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
- this.scF5.DefaultCellStyle = dataGridViewCellStyle57;
+ dataGridViewCellStyle18.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
+ this.scF5.DefaultCellStyle = dataGridViewCellStyle18;
this.scF5.HeaderText = "F5";
this.scF5.Name = "scF5";
this.scF5.ReadOnly = true;
//
// scF6
//
- dataGridViewCellStyle58.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
- this.scF6.DefaultCellStyle = dataGridViewCellStyle58;
+ dataGridViewCellStyle19.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
+ this.scF6.DefaultCellStyle = dataGridViewCellStyle19;
this.scF6.HeaderText = "F6";
this.scF6.Name = "scF6";
this.scF6.ReadOnly = true;
//
// scF7
//
- dataGridViewCellStyle59.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
- this.scF7.DefaultCellStyle = dataGridViewCellStyle59;
+ dataGridViewCellStyle20.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
+ this.scF7.DefaultCellStyle = dataGridViewCellStyle20;
this.scF7.HeaderText = "F7";
this.scF7.Name = "scF7";
this.scF7.ReadOnly = true;
//
// scF8
//
- dataGridViewCellStyle60.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
- this.scF8.DefaultCellStyle = dataGridViewCellStyle60;
+ dataGridViewCellStyle21.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
+ this.scF8.DefaultCellStyle = dataGridViewCellStyle21;
this.scF8.HeaderText = "F8";
this.scF8.Name = "scF8";
this.scF8.ReadOnly = true;
//
// scF9
//
- dataGridViewCellStyle61.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
- this.scF9.DefaultCellStyle = dataGridViewCellStyle61;
+ dataGridViewCellStyle22.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
+ this.scF9.DefaultCellStyle = dataGridViewCellStyle22;
this.scF9.HeaderText = "F9";
this.scF9.Name = "scF9";
this.scF9.ReadOnly = true;
//
// scF10
//
- dataGridViewCellStyle62.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
- this.scF10.DefaultCellStyle = dataGridViewCellStyle62;
+ dataGridViewCellStyle23.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
+ this.scF10.DefaultCellStyle = dataGridViewCellStyle23;
this.scF10.HeaderText = "F10";
this.scF10.Name = "scF10";
this.scF10.ReadOnly = true;
@@ -545,8 +540,8 @@ private void InitializeComponent()
//
// scF12
//
- dataGridViewCellStyle63.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
- this.scF12.DefaultCellStyle = dataGridViewCellStyle63;
+ dataGridViewCellStyle24.Alignment = System.Windows.Forms.DataGridViewContentAlignment.BottomCenter;
+ this.scF12.DefaultCellStyle = dataGridViewCellStyle24;
this.scF12.HeaderText = "F12";
this.scF12.Name = "scF12";
this.scF12.ReadOnly = true;
@@ -724,14 +719,9 @@ private void InitializeComponent()
// PanelTwo
//
this.PanelTwo.BackColor = System.Drawing.SystemColors.Control;
- this.PanelTwo.Controls.Add(this.lbTranslateLF);
- this.PanelTwo.Controls.Add(this.lbTranslateCR);
- this.PanelTwo.Controls.Add(this.label3);
this.PanelTwo.Controls.Add(this.cbFreeze);
this.PanelTwo.Controls.Add(this.sep1);
- this.PanelTwo.Controls.Add(this.label2);
this.PanelTwo.Controls.Add(this.panel3);
- this.PanelTwo.Controls.Add(this.panel2);
this.PanelTwo.Controls.Add(this.panel1);
this.PanelTwo.Controls.Add(this.btnExport);
this.PanelTwo.Controls.Add(this.btnHelp);
@@ -748,47 +738,6 @@ private void InitializeComponent()
this.PanelTwo.Size = new System.Drawing.Size(926, 28);
this.PanelTwo.TabIndex = 8;
//
- // lbTranslateLF
- //
- this.lbTranslateLF.Cursor = System.Windows.Forms.Cursors.Default;
- this.lbTranslateLF.DropDownStyle = System.Windows.Forms.ComboBoxStyle.DropDownList;
- this.lbTranslateLF.FormattingEnabled = true;
- this.lbTranslateLF.Items.AddRange(new object[] {
- "Ignore",
- "LF",
- "CR+LF",
- "Auto"});
- this.lbTranslateLF.Location = new System.Drawing.Point(412, 4);
- this.lbTranslateLF.Name = "lbTranslateLF";
- this.lbTranslateLF.Size = new System.Drawing.Size(62, 21);
- this.lbTranslateLF.TabIndex = 21;
- this.lbTranslateLF.SelectedIndexChanged += new System.EventHandler(this.LbTranslateLF_SelectedIndexChanged);
- //
- // lbTranslateCR
- //
- this.lbTranslateCR.Cursor = System.Windows.Forms.Cursors.Default;
- this.lbTranslateCR.DropDownStyle = System.Windows.Forms.ComboBoxStyle.DropDownList;
- this.lbTranslateCR.FormattingEnabled = true;
- this.lbTranslateCR.Items.AddRange(new object[] {
- "Ignore",
- "CR",
- "CR+LF",
- "Auto"});
- this.lbTranslateCR.Location = new System.Drawing.Point(322, 4);
- this.lbTranslateCR.Name = "lbTranslateCR";
- this.lbTranslateCR.Size = new System.Drawing.Size(62, 21);
- this.lbTranslateCR.TabIndex = 20;
- this.lbTranslateCR.SelectedIndexChanged += new System.EventHandler(this.LbTranslateCR_SelectedIndexChanged);
- //
- // label3
- //
- this.label3.AutoSize = true;
- this.label3.Location = new System.Drawing.Point(293, 7);
- this.label3.Name = "label3";
- this.label3.Size = new System.Drawing.Size(28, 13);
- this.label3.TabIndex = 23;
- this.label3.Text = "CR=";
- //
// cbFreeze
//
this.cbFreeze.Appearance = System.Windows.Forms.Appearance.Button;
@@ -813,15 +762,6 @@ private void InitializeComponent()
this.sep1.Size = new System.Drawing.Size(4, 23);
this.sep1.TabIndex = 18;
//
- // label2
- //
- this.label2.AutoSize = true;
- this.label2.Location = new System.Drawing.Point(386, 8);
- this.label2.Name = "label2";
- this.label2.Size = new System.Drawing.Size(25, 13);
- this.label2.TabIndex = 22;
- this.label2.Text = "LF=";
- //
// panel3
//
this.panel3.BackColor = System.Drawing.SystemColors.ButtonShadow;
@@ -830,18 +770,10 @@ private void InitializeComponent()
this.panel3.Size = new System.Drawing.Size(4, 23);
this.panel3.TabIndex = 19;
//
- // panel2
- //
- this.panel2.BackColor = System.Drawing.SystemColors.ButtonShadow;
- this.panel2.Location = new System.Drawing.Point(480, 3);
- this.panel2.Name = "panel2";
- this.panel2.Size = new System.Drawing.Size(4, 23);
- this.panel2.TabIndex = 19;
- //
// panel1
//
this.panel1.BackColor = System.Drawing.SystemColors.ButtonShadow;
- this.panel1.Location = new System.Drawing.Point(668, 3);
+ this.panel1.Location = new System.Drawing.Point(466, 3);
this.panel1.Name = "panel1";
this.panel1.Size = new System.Drawing.Size(4, 23);
this.panel1.TabIndex = 19;
@@ -850,7 +782,7 @@ private void InitializeComponent()
//
this.btnExport.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
this.btnExport.BackColor = System.Drawing.Color.Transparent;
- this.btnExport.Location = new System.Drawing.Point(751, 1);
+ this.btnExport.Location = new System.Drawing.Point(757, 2);
this.btnExport.Name = "btnExport";
this.btnExport.Size = new System.Drawing.Size(45, 24);
this.btnExport.TabIndex = 17;
@@ -862,7 +794,7 @@ private void InitializeComponent()
//
this.btnHelp.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
this.btnHelp.BackColor = System.Drawing.Color.Transparent;
- this.btnHelp.Location = new System.Drawing.Point(847, 1);
+ this.btnHelp.Location = new System.Drawing.Point(851, 2);
this.btnHelp.Name = "btnHelp";
this.btnHelp.Size = new System.Drawing.Size(45, 24);
this.btnHelp.TabIndex = 1;
@@ -885,7 +817,7 @@ private void InitializeComponent()
//
this.btnClear.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Right)));
this.btnClear.BackColor = System.Drawing.Color.Transparent;
- this.btnClear.Location = new System.Drawing.Point(799, 1);
+ this.btnClear.Location = new System.Drawing.Point(804, 2);
this.btnClear.Name = "btnClear";
this.btnClear.Size = new System.Drawing.Size(45, 24);
this.btnClear.TabIndex = 16;
@@ -897,25 +829,25 @@ private void InitializeComponent()
//
this.cbShowCR.AutoSize = true;
this.cbShowCR.Cursor = System.Windows.Forms.Cursors.Default;
- this.cbShowCR.Location = new System.Drawing.Point(501, 6);
+ this.cbShowCR.Location = new System.Drawing.Point(299, 6);
this.cbShowCR.Name = "cbShowCR";
this.cbShowCR.Size = new System.Drawing.Size(79, 17);
this.cbShowCR.TabIndex = 10;
this.cbShowCR.Text = "Show {CR}";
this.cbShowCR.UseVisualStyleBackColor = true;
- this.cbShowCR.CheckedChanged += new System.EventHandler(this.ResetFocus);
+ this.cbShowCR.CheckedChanged += new System.EventHandler(this.cbShowCR_CheckedChanged);
//
// cbShowLF
//
this.cbShowLF.AutoSize = true;
this.cbShowLF.Cursor = System.Windows.Forms.Cursors.Default;
- this.cbShowLF.Location = new System.Drawing.Point(586, 6);
+ this.cbShowLF.Location = new System.Drawing.Point(384, 6);
this.cbShowLF.Name = "cbShowLF";
this.cbShowLF.Size = new System.Drawing.Size(76, 17);
this.cbShowLF.TabIndex = 9;
this.cbShowLF.Text = "Show {LF}";
this.cbShowLF.UseVisualStyleBackColor = true;
- this.cbShowLF.CheckedChanged += new System.EventHandler(this.ResetFocus);
+ this.cbShowLF.CheckedChanged += new System.EventHandler(this.cbShowLF_CheckedChanged);
//
// cbTimestamp
//
@@ -930,7 +862,7 @@ private void InitializeComponent()
this.cbTimestamp.TabIndex = 8;
this.cbTimestamp.Text = "Timestamp";
this.cbTimestamp.UseVisualStyleBackColor = false;
- this.cbTimestamp.CheckedChanged += new System.EventHandler(this.ResetFocus);
+ this.cbTimestamp.CheckedChanged += new System.EventHandler(this.cbTimestamp_CheckedChanged);
//
// cbHEX
//
@@ -1093,14 +1025,17 @@ private void InitializeComponent()
//
// rtb
//
- this.rtb.BorderStyle = System.Windows.Forms.BorderStyle.None;
+ this.rtb.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle;
this.rtb.ContextMenuStrip = this.contextMenuStrip;
this.rtb.Cursor = System.Windows.Forms.Cursors.Default;
+ this.rtb.DetectUrls = false;
this.rtb.Dock = System.Windows.Forms.DockStyle.Fill;
this.rtb.Font = new System.Drawing.Font("Courier New", 9.75F);
this.rtb.Location = new System.Drawing.Point(0, 0);
this.rtb.Name = "rtb";
this.rtb.ReadOnly = true;
+ this.rtb.ScrollBars = System.Windows.Forms.RichTextBoxScrollBars.ForcedBoth;
+ this.rtb.ShowSelectionMargin = true;
this.rtb.Size = new System.Drawing.Size(926, 361);
this.rtb.TabIndex = 13;
this.rtb.Text = "";
@@ -1111,6 +1046,9 @@ private void InitializeComponent()
//
// contextMenuStrip
//
+ this.contextMenuStrip.AutoSize = false;
+ this.contextMenuStrip.BackColor = System.Drawing.Color.FromArgb(((int)(((byte)(255)))), ((int)(((byte)(255)))), ((int)(((byte)(192)))));
+ this.contextMenuStrip.BackgroundImageLayout = System.Windows.Forms.ImageLayout.None;
this.contextMenuStrip.Items.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.copyToolStripMenuItem});
this.contextMenuStrip.Name = "contextMenuStrip";
@@ -1241,14 +1179,9 @@ private void InitializeComponent()
private System.Windows.Forms.Button btnExport;
private System.Windows.Forms.SaveFileDialog saveFileDialog;
private System.Windows.Forms.Panel panel3;
- private System.Windows.Forms.Panel panel2;
private System.Windows.Forms.Panel panel1;
private System.Windows.Forms.Panel sep1;
private System.Windows.Forms.CheckBox cbClearCMD;
- private System.Windows.Forms.ComboBox lbTranslateCR;
- private System.Windows.Forms.Label label3;
- private System.Windows.Forms.Label label2;
- private System.Windows.Forms.ComboBox lbTranslateLF;
private System.Windows.Forms.ToolStripStatusLabel stsConnectionDetail;
private System.Windows.Forms.ToolStripStatusLabel stsSize;
private System.Windows.Forms.ToolStripStatusLabel stsMaxSize;
diff --git a/Terminal2/FrmMain.cs b/Terminal2/FrmMain.cs
index a5059ba..0c5a910 100644
--- a/Terminal2/FrmMain.cs
+++ b/Terminal2/FrmMain.cs
@@ -57,9 +57,6 @@ private enum State { Changing, Disconnected, Connected, Listening };
private bool _abortFileSend;
private bool _pauseFileSend;
- private bool _addTimestamp = true;
- private bool _skipNextLF = false;
-
private bool _firstActivation = true;
private bool _macroIsMoving = false;
@@ -104,13 +101,16 @@ private int FindApplicableFilter(string str, int offset)
private void ColoringHandler()
{
- int topIndex = rtb.GetCharIndexFromPosition(new Point(1, 1));
- int bottomIndex = rtb.GetCharIndexFromPosition(new Point(1, rtb.Height - 1));
- int topLine = rtb.GetLineFromCharIndex(topIndex);
- int bottomLine = rtb.GetLineFromCharIndex(bottomIndex);
-
lock (_rtbLock)
{
+ if (rtb.Lines.Length == 0)
+ return;
+
+ int topIndex = rtb.GetCharIndexFromPosition(new Point(1, 1));
+ int bottomIndex = rtb.GetCharIndexFromPosition(new Point(1, rtb.Height - 1));
+ int topLine = rtb.GetLineFromCharIndex(topIndex);
+ int bottomLine = rtb.GetLineFromCharIndex(bottomIndex);
+
for (int i = bottomLine; i >= topLine; i--)
{
// if something changed, quit immediately
@@ -122,7 +122,7 @@ private void ColoringHandler()
{
int offset = 0;
if (Utils.HasTimestamp(str))
- offset += 13;
+ offset += Utils.TimestampLength();
int index = FindApplicableFilter(str, offset);
if (index >= 0)
@@ -187,108 +187,15 @@ private void UpdateLineCounter()
}
}
- private void ProcessChunk(string str)
+ private void ProcessChunk(string chunk)
{
- string _displayStr = string.Empty;
- string _logStr = string.Empty;
- string TS = string.Empty;
- if (cbTimestamp.Checked)
- TS = Utils.Timestamp();
-
- int translateCR = _activeProfile.translateCR;
- int translateLF = _activeProfile.translateLF;
-
- for (int i = 0; i < str.Length; i++)
- {
- if (_addTimestamp)
- {
- _displayStr += TS;
- _logStr += ("R : " + TS);
- _addTimestamp = false;
- }
-
- char c = str[i];
- switch (c)
- {
- case (char)'\r':
- if (cbShowCR.Checked)
- {
- _displayStr += "{CR}";
- _logStr += "{CR}";
- }
- if (translateCR == 1) // = CR
- {
- _displayStr += "\n";
- _logStr += "\r";
- }
- else if (translateCR == 2) // = CRLF
- {
- _displayStr += "\n";
- _logStr += "\r\n";
- _addTimestamp = true;
- }
- else if (translateCR == 3) // = Auto
- {
- _displayStr += "\n";
- _logStr += "\r\n";
- _addTimestamp = true;
- _skipNextLF = true;
- }
- break;
-
- case (char)'\n':
- if (cbShowLF.Checked)
- {
- _displayStr += "{LF}";
- _logStr += "{LF}";
- }
- if (translateLF == 1) // = LF
- {
- _displayStr += "\n";
- _logStr += "\n";
- _addTimestamp = true;
- }
- else if (translateLF == 2) // = CRLF
- {
- _displayStr += "\n";
- _logStr += "\r\n";
- _addTimestamp = true;
- }
- else if (translateLF == 3) // = Auto
- {
- if (!_skipNextLF)
- {
- _displayStr += "\n";
- _logStr += "\r\n";
- _addTimestamp = true;
- _skipNextLF = false;
- }
- }
- break;
-
- default:
- if (cbASCII.Checked)
- {
- _displayStr += c.ToString();
- _logStr += c.ToString();
- }
- if (cbHEX.Checked)
- {
- byte b = Convert.ToByte(c);
- _displayStr += $"{b:X2} ";
- _logStr += $"{b:X2} ";
- }
- _skipNextLF = false;
- break;
- }
- }
-
+ Log.Add(chunk);
if (!_frozen)
{
lock (_rtbLock)
{
int lastLine = Math.Max(0, rtb.Lines.Length - 1);
- rtb.AppendText(_displayStr, _activeProfile.displayOptions.inputText);
+ rtb.AppendText(chunk, _activeProfile.displayOptions.inputText);
if (rtb.Lines.Length < 100)
_colorSemaphore.Set();
@@ -317,16 +224,9 @@ private void ProcessChunk(string str)
lastLine -= tocut;
_colorEnd = lastLine;
}
-
- // and put the caret back to the bottom
- rtb.SelectionStart = rtb.Text.Length;
}
}
- rtb.ScrollToCaret();
- UpdateLineCounter();
}
-
- Log.Add(_logStr);
}
private void UIInputQueueHandler()
@@ -336,8 +236,18 @@ private void UIInputQueueHandler()
string str = (string)_uiInputQueue.Dequeue();
ProcessChunk(str);
}
- }
+ // and put the caret back to the bottom
+ if (!_frozen)
+ {
+ lock (_rtbLock)
+ {
+ rtb.SelectionStart = rtb.Text.Length;
+ rtb.ScrollToCaret();
+ }
+ UpdateLineCounter();
+ }
+ }
private void UIOutputQueueHandler()
{
while (!_terminateFlag && _uiOutputQueue.Count > 0)
@@ -351,7 +261,7 @@ private void UIOutputQueueHandler()
else
lbOutput.Items.Add(str);
- Log.Add(" T: " + tm + str);
+ Log.Add("\r\n----> Tx: " + tm + str + "\r\n");
lbOutput.TopIndex = lbOutput.Items.Count - 1;
Application.DoEvents();
}
@@ -705,12 +615,43 @@ private void BtnStartLog_Click(object sender, EventArgs e)
tbCommand.Focus();
}
+ #region EMBELISHMENTS
+ private void cbTimestamp_CheckedChanged(object sender, EventArgs e)
+ {
+ _activeProfile.embelishments.ShowTimestamp = cbTimestamp.Checked;
+ _comms.SetEmbelishments(_activeProfile.embelishments);
+ tbCommand.Focus();
+ }
private void CbASCII_CheckedChanged(object sender, EventArgs e)
{
if (!cbASCII.Checked && !cbHEX.Checked)
cbHEX.Checked = true;
+ _activeProfile.embelishments.ShowASCII = cbASCII.Checked;
+ _comms.SetEmbelishments(_activeProfile.embelishments);
+ tbCommand.Focus();
+ }
+ private void CbHEX_CheckedChanged(object sender, EventArgs e)
+ {
+ if (!cbASCII.Checked && !cbHEX.Checked)
+ cbASCII.Checked = true;
+ _activeProfile.embelishments.ShowHEX = cbHEX.Checked;
+ _comms.SetEmbelishments(_activeProfile.embelishments);
+ tbCommand.Focus();
+ }
+ private void cbShowCR_CheckedChanged(object sender, EventArgs e)
+ {
+ _activeProfile.embelishments.ShowCR = cbShowCR.Checked;
+ _comms.SetEmbelishments(_activeProfile.embelishments);
+ tbCommand.Focus();
+ }
+
+ private void cbShowLF_CheckedChanged(object sender, EventArgs e)
+ {
+ _activeProfile.embelishments.ShowLF = cbShowLF.Checked;
+ _comms.SetEmbelishments(_activeProfile.embelishments);
tbCommand.Focus();
}
+ #endregion
private void CbFreeze_CheckedChanged(object sender, EventArgs e)
{
@@ -736,13 +677,6 @@ private void CbFreeze_CheckedChanged(object sender, EventArgs e)
tbCommand.Focus();
}
- private void CbHEX_CheckedChanged(object sender, EventArgs e)
- {
- if (!cbASCII.Checked && !cbHEX.Checked)
- cbASCII.Checked = true;
- tbCommand.Focus();
- }
-
private void CbStayOnTop_CheckedChanged(object sender, EventArgs e)
{
TopMost = cbStayOnTop.Checked;
@@ -983,13 +917,6 @@ private void SaveThisProfile()
lblSaving.Text = "*";
Application.DoEvents();
_activeProfile.stayontop = cbStayOnTop.Checked;
- _activeProfile.timestampInput = cbTimestamp.Checked;
- _activeProfile.ascii = cbASCII.Checked;
- _activeProfile.hex = cbHEX.Checked;
- _activeProfile.translateCR = lbTranslateCR.SelectedIndex;
- _activeProfile.translateLF = lbTranslateLF.SelectedIndex;
- _activeProfile.showCurlyCR = cbShowCR.Checked;
- _activeProfile.showCurlyLF = cbShowLF.Checked;
_activeProfile.sendCR = cbSendCR.Checked;
_activeProfile.sendLF = cbSendLF.Checked;
_activeProfile.clearCMD = cbClearCMD.Checked;
@@ -1023,17 +950,17 @@ private void SwitchToProfile(string name)
lblProfileName.Text = _activeProfile.name;
cbStayOnTop.Checked = _activeProfile.stayontop;
- cbTimestamp.Checked = _activeProfile.timestampInput;
- cbASCII.Checked = _activeProfile.ascii;
- cbHEX.Checked = _activeProfile.hex;
-
- lbTranslateCR.SelectedIndex = _activeProfile.translateCR;
- lbTranslateLF.SelectedIndex = _activeProfile.translateLF;
- cbShowCR.Checked = _activeProfile.showCurlyCR;
- cbShowLF.Checked = _activeProfile.showCurlyLF;
+
cbSendCR.Checked = _activeProfile.sendCR;
cbSendLF.Checked = _activeProfile.sendLF;
cbClearCMD.Checked = _activeProfile.clearCMD;
+
+ cbTimestamp.Checked = _activeProfile.embelishments.ShowTimestamp;
+ cbASCII.Checked = _activeProfile.embelishments.ShowASCII;
+ cbHEX.Checked = _activeProfile.embelishments.ShowHEX;
+ cbShowCR.Checked = _activeProfile.embelishments.ShowCR;
+ cbShowLF.Checked = _activeProfile.embelishments.ShowLF;
+
cbFreeze.Checked = false;
int i = 0;
@@ -1049,6 +976,7 @@ private void SwitchToProfile(string name)
}
}
RefreshPortPulldown();
+ _comms.SetEmbelishments(_activeProfile.embelishments);
stsMaxSize.Text = $" Max: {_activeProfile.displayOptions.maxLines} lines ";
}
}
@@ -1301,9 +1229,7 @@ private void FrmMain_Shown(object sender, EventArgs e)
{
if (_firstActivation)
{
- BtnHelp_Click(sender, e);
- Application.DoEvents();
- MessageBox.Show($"This is a one-time message.\nThe Help button has been pressed for you.\nPress it whenever you need reminders of the shortcuts.\n(Please read the information carefully and press Help to close)", "Welcome", MessageBoxButtons.OK, MessageBoxIcon.Information);
+ MessageBox.Show($"This is a once-only message.\nPress the HELP button for some tips.\nThe key concept is to create a profile for every kind of application you may have.\n\nStart by pressing the [(+)] button (top middle) to create your first profile", "Welcome", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
tbCommand.Focus();
Application.DoEvents();
@@ -1315,6 +1241,7 @@ private void BtnClear_Click(object sender, EventArgs e)
{
_uiInputQueue.Clear();
rtb.Clear();
+ rtb.ZoomFactor = 1;
_colorEnd = 0;
}
cbFreeze.Checked = false;
@@ -1527,18 +1454,6 @@ private void BtnExport_Click(object sender, EventArgs e)
btnExport.Enabled = true;
}
- private void LbTranslateCR_SelectedIndexChanged(object sender, EventArgs e)
- {
- if (lbTranslateCR.SelectedIndex == 3)
- lbTranslateLF.SelectedIndex = 3;
- }
-
- private void LbTranslateLF_SelectedIndexChanged(object sender, EventArgs e)
- {
- if (lbTranslateLF.SelectedIndex == 3)
- lbTranslateCR.SelectedIndex = 3;
- }
-
private void DgMacroTable_CellClick(object sender, DataGridViewCellEventArgs e)
{
dgMacroTable.ClearSelection();
diff --git a/Terminal2/FrmMain.resx b/Terminal2/FrmMain.resx
index 274d796..069ca65 100644
--- a/Terminal2/FrmMain.resx
+++ b/Terminal2/FrmMain.resx
@@ -124,7 +124,7 @@
iVBORw0KGgoAAAANSUhEUgAAABAAAAAQCAIAAACQkWg2AAAABGdBTUEAALGPC/xhBQAAAAlwSFlzAAAL
- EgAACxIB0t1+/AAAAAd0SU1FB+YCDAIaJl17Fa8AAADSSURBVDhPnZJNDoIwEIW9W7uup+BaHIBDsNDo
+ EAAACxABrSO9dQAAAAd0SU1FB+YCDAIaJl17Fa8AAADSSURBVDhPnZJNDoIwEIW9W7uup+BaHIBDsNDo
ShM8gZ5AKX9CgcanL9akFglOuph23tcOw1vlea61LoqiLMuqquq6bprm/oq2bbuuM8b0fT8MwziO1tow
IKUUQswASZIQQAI1Io5jAjjxASeKokgpxRwJtswh8F9gYSoCLfEy3JpusuvNYKXbk1JrHKLkA2iXnUAE
6f54wSIjhEQJgg/wfPIdVEOEfHc4Y8uJMf4FOKXfLQWmtOyjF4918Y8jQzs5eNIaZNCV89+M+b4BOjwA
@@ -166,7 +166,7 @@
iVBORw0KGgoAAAANSUhEUgAAABAAAAAQCAIAAACQkWg2AAAABGdBTUEAALGPC/xhBQAAAAlwSFlzAAAL
- EgAACxIB0t1+/AAAAAd0SU1FB+YCDAIaJl17Fa8AAADSSURBVDhPnZJNDoIwEIW9W7uup+BaHIBDsNDo
+ EAAACxABrSO9dQAAAAd0SU1FB+YCDAIaJl17Fa8AAADSSURBVDhPnZJNDoIwEIW9W7uup+BaHIBDsNDo
ShM8gZ5AKX9CgcanL9akFglOuph23tcOw1vlea61LoqiLMuqquq6bprm/oq2bbuuM8b0fT8MwziO1tow
IKUUQswASZIQQAI1Io5jAjjxASeKokgpxRwJtswh8F9gYSoCLfEy3JpusuvNYKXbk1JrHKLkA2iXnUAE
6f54wSIjhEQJgg/wfPIdVEOEfHc4Y8uJMf4FOKXfLQWmtOyjF4918Y8jQzs5eNIaZNCV89+M+b4BOjwA
@@ -176,7 +176,7 @@
iVBORw0KGgoAAAANSUhEUgAAABAAAAAQCAIAAACQkWg2AAAABGdBTUEAALGPC/xhBQAAAAlwSFlzAAAL
- EgAACxIB0t1+/AAAAAd0SU1FB+YCDAIaJl17Fa8AAADSSURBVDhPnZJNDoIwEIW9W7uup+BaHIBDsNDo
+ EAAACxABrSO9dQAAAAd0SU1FB+YCDAIaJl17Fa8AAADSSURBVDhPnZJNDoIwEIW9W7uup+BaHIBDsNDo
ShM8gZ5AKX9CgcanL9akFglOuph23tcOw1vlea61LoqiLMuqquq6bprm/oq2bbuuM8b0fT8MwziO1tow
IKUUQswASZIQQAI1Io5jAjjxASeKokgpxRwJtswh8F9gYSoCLfEy3JpusuvNYKXbk1JrHKLkA2iXnUAE
6f54wSIjhEQJgg/wfPIdVEOEfHc4Y8uJMf4FOKXfLQWmtOyjF4918Y8jQzs5eNIaZNCV89+M+b4BOjwA
@@ -225,7 +225,7 @@
iVBORw0KGgoAAAANSUhEUgAAABAAAAAQCAIAAACQkWg2AAAABGdBTUEAALGPC/xhBQAAAAlwSFlzAAAL
- EgAACxIB0t1+/AAAAAd0SU1FB+YCDAIaJl17Fa8AAADSSURBVDhPnZJNDoIwEIW9W7uup+BaHIBDsNDo
+ EAAACxABrSO9dQAAAAd0SU1FB+YCDAIaJl17Fa8AAADSSURBVDhPnZJNDoIwEIW9W7uup+BaHIBDsNDo
ShM8gZ5AKX9CgcanL9akFglOuph23tcOw1vlea61LoqiLMuqquq6bprm/oq2bbuuM8b0fT8MwziO1tow
IKUUQswASZIQQAI1Io5jAjjxASeKokgpxRwJtswh8F9gYSoCLfEy3JpusuvNYKXbk1JrHKLkA2iXnUAE
6f54wSIjhEQJgg/wfPIdVEOEfHc4Y8uJMf4FOKXfLQWmtOyjF4918Y8jQzs5eNIaZNCV89+M+b4BOjwA
@@ -235,7 +235,7 @@
iVBORw0KGgoAAAANSUhEUgAAABAAAAAQCAIAAACQkWg2AAAABGdBTUEAALGPC/xhBQAAAAlwSFlzAAAL
- EgAACxIB0t1+/AAAAAd0SU1FB+YCDAIaJl17Fa8AAADSSURBVDhPnZJNDoIwEIW9W7uup+BaHIBDsNDo
+ EAAACxABrSO9dQAAAAd0SU1FB+YCDAIaJl17Fa8AAADSSURBVDhPnZJNDoIwEIW9W7uup+BaHIBDsNDo
ShM8gZ5AKX9CgcanL9akFglOuph23tcOw1vlea61LoqiLMuqquq6bprm/oq2bbuuM8b0fT8MwziO1tow
IKUUQswASZIQQAI1Io5jAjjxASeKokgpxRwJtswh8F9gYSoCLfEy3JpusuvNYKXbk1JrHKLkA2iXnUAE
6f54wSIjhEQJgg/wfPIdVEOEfHc4Y8uJMf4FOKXfLQWmtOyjF4918Y8jQzs5eNIaZNCV89+M+b4BOjwA
diff --git a/Terminal2/Options.cs b/Terminal2/Options.cs
index 34b64d2..0d7d6be 100644
--- a/Terminal2/Options.cs
+++ b/Terminal2/Options.cs
@@ -122,23 +122,32 @@ public DisplayOptions Clone()
}
}
+ public class Embelishments
+ {
+ public bool ShowCR;
+ public bool ShowLF;
+ public bool ShowTimestamp;
+ public bool ShowASCII;
+ public bool ShowHEX;
+
+ public Embelishments Clone()
+ {
+ Embelishments emb = (Embelishments)this.MemberwiseClone();
+ return emb;
+ }
+ }
+
public class Profile
{
public bool active = false;
public string name = "Default";
- public bool showCurlyCR = false;
- public bool showCurlyLF = false;
- public bool ascii = true;
- public bool hex = false;
- public int translateCR = 3; // 0=ignore, 1=CR, 2=CRLF, 3=Auto
- public int translateLF = 3; // 0=ignore, 1=LF, 2=CRLF, 3=-Auto
public bool sendCR = true;
public bool sendLF = false;
public bool clearCMD = false;
public bool stayontop = false;
- public bool timestampInput = true;
+ public Embelishments embelishments = new Embelishments();
public ConOptions conOptions = new ConOptions();
public LoggingOptions logOptions = new LoggingOptions();
public DisplayOptions displayOptions = new DisplayOptions();
@@ -154,6 +163,7 @@ public Profile Clone(string name)
{
Profile s = (Profile)this.MemberwiseClone();
s.name = name;
+ s.embelishments = embelishments.Clone();
s.conOptions = conOptions.Clone();
s.logOptions = logOptions.Clone();
s.displayOptions = displayOptions.Clone();
@@ -193,7 +203,7 @@ public static string TimestampForFilename()
public static string Timestamp()
{
- return $"{DateTime.Now:HH:mm:ss.ff}: ";
+ return $"{DateTime.Now:HH:mm:ss.fff}: ";
}
public static bool IsNumeric(char ch)
{
@@ -202,16 +212,21 @@ public static bool IsNumeric(char ch)
return true;
}
- // "HH:mm:ss.ff: "
+ // "HH:mm:ss.fff: "
public static bool HasTimestamp(string str)
{
- if (str.Length < 13)
+ if (str.Length < 14)
return false;
- if (!IsNumeric(str[0]) || !IsNumeric(str[1]) || !IsNumeric(str[3]) || !IsNumeric(str[4]) || !IsNumeric(str[6]) || !IsNumeric(str[7]) || !IsNumeric(str[9]) || !IsNumeric(str[10]))
+ if (!IsNumeric(str[0]) || !IsNumeric(str[1]) || !IsNumeric(str[3]) || !IsNumeric(str[4]) || !IsNumeric(str[6]) || !IsNumeric(str[7]) || !IsNumeric(str[9]) || !IsNumeric(str[10]) || !IsNumeric(str[11]))
return false;
- if (str[2] != (char)':' || str[5] != (char)':' || str[8] != (char)'.' || str[11] != (char)':' || str[12] != (char)' ')
+ if (str[2] != (char)':' || str[5] != (char)':' || str[8] != (char)'.' || str[12] != (char)':')
return false;
return true;
}
+
+ public static int TimestampLength()
+ {
+ return 14;
+ }
}
}
\ No newline at end of file
diff --git a/Terminal2/Properties/AssemblyInfo.cs b/Terminal2/Properties/AssemblyInfo.cs
index a587996..d3444a1 100644
--- a/Terminal2/Properties/AssemblyInfo.cs
+++ b/Terminal2/Properties/AssemblyInfo.cs
@@ -37,8 +37,8 @@
// You can specify all the values or you can default the Build and Revision Numbers
// by using the '*' as shown below:
// [assembly: AssemblyVersion("1.0.*")]
-[assembly: AssemblyVersion("1.0.3.2")]
-[assembly: AssemblyFileVersion("1.0.3.2")]
+[assembly: AssemblyVersion("1.0.4.0")]
+[assembly: AssemblyFileVersion("1.0.4.0")]
namespace Terminal
{