diff --git a/.gitignore b/.gitignore
index 4acda55..6b5b65e 100644
--- a/.gitignore
+++ b/.gitignore
@@ -3,4 +3,5 @@ storage/*
*.log
deploy/docker-compose/conf
deploy/docker-compose/data
+builds
diff --git a/build.bat b/build.bat
new file mode 100644
index 0000000..8dd1d0f
--- /dev/null
+++ b/build.bat
@@ -0,0 +1,45 @@
+@echo off
+setlocal
+
+:: 编译 Linux ARM32
+set GOOS=linux
+set GOARCH=arm
+set GOARM=7
+set OUTPUT_DIR=.\builds\PandoraHelper-%1-%GOOS%-%GOARCH%
+mkdir %OUTPUT_DIR%
+go build -ldflags="-s -w" -o %OUTPUT_DIR%\PandoraHelper .\cmd\server\main.go
+upx %OUTPUT_DIR%\PandoraHelper
+copy .\config.json "%OUTPUT_DIR%"\
+
+:: 编译 Linux ARM64
+set GOOS=linux
+set GOARCH=arm64
+set OUTPUT_DIR=.\builds\PandoraHelper-%1-%GOOS%-%GOARCH%
+go build -ldflags="-s -w" -o %OUTPUT_DIR%\PandoraHelper .\cmd\server\main.go
+upx %OUTPUT_DIR%\PandoraHelper
+COPY .\config.json "%OUTPUT_DIR%"\
+
+:: 编译为Linux 64位
+set GOOS=linux
+set GOARCH=amd64
+set OUTPUT_DIR=.\builds\PandoraHelper-%1-%GOOS%-%GOARCH%
+go build -ldflags="-s -w" -o %OUTPUT_DIR%\PandoraHelper .\cmd\server\main.go
+upx %OUTPUT_DIR%\PandoraHelper
+COPY .\config.json "%OUTPUT_DIR%"\
+
+:: 编译 Windows 64 位
+set GOOS=windows
+set GOARCH=amd64
+set OUTPUT_DIR=.\builds\PandoraHelper-%1-%GOOS%-%GOARCH%
+go build -ldflags="-s -w" -o %OUTPUT_DIR%\PandoraHelper.exe .\cmd\server\main.go
+upx %OUTPUT_DIR%\PandoraHelper.exe
+COPY .\config.json "%OUTPUT_DIR%"\
+
+:: 编译为macOS 64位
+set GOOS=darwin
+set GOARCH=amd64
+set OUTPUT_DIR=.\builds\PandoraHelper-%1-%GOOS%-%GOARCH%
+go build -ldflags="-s -w" -o %OUTPUT_DIR%\PandoraHelper .\cmd\server\main.go
+COPY .\config.json "%OUTPUT_DIR%"\
+
+echo Compilation and compression complete.
diff --git a/cmd/migration/main.go b/cmd/migration/main.go
deleted file mode 100644
index e2e8fff..0000000
--- a/cmd/migration/main.go
+++ /dev/null
@@ -1,26 +0,0 @@
-package main
-
-import (
- "PandoraHelper/cmd/migration/wire"
- "PandoraHelper/pkg/config"
- "PandoraHelper/pkg/log"
- "context"
- "flag"
-)
-
-func main() {
- var envConf = flag.String("conf", "config/local.yml", "config path, eg: -conf ./config/local.yml")
- flag.Parse()
- conf := config.NewConfig(*envConf)
-
- logger := log.NewLog(conf)
-
- app, cleanup, err := wire.NewWire(conf, logger)
- defer cleanup()
- if err != nil {
- panic(err)
- }
- if err = app.Run(context.Background()); err != nil {
- panic(err)
- }
-}
diff --git a/cmd/migration/wire/wire.go b/cmd/migration/wire/wire.go
deleted file mode 100644
index 45a4af7..0000000
--- a/cmd/migration/wire/wire.go
+++ /dev/null
@@ -1,38 +0,0 @@
-//go:build wireinject
-// +build wireinject
-
-package wire
-
-import (
- "PandoraHelper/internal/repository"
- "PandoraHelper/internal/server"
- "PandoraHelper/pkg/app"
- "PandoraHelper/pkg/log"
- "github.com/google/wire"
- "github.com/spf13/viper"
-)
-
-var repositorySet = wire.NewSet(
- repository.NewDB,
- //repository.NewRedis,
- repository.NewRepository,
-)
-var serverSet = wire.NewSet(
- server.NewMigrate,
-)
-
-// build App
-func newApp(migrate *server.Migrate) *app.App {
- return app.NewApp(
- app.WithServer(migrate),
- app.WithName("demo-migrate"),
- )
-}
-
-func NewWire(*viper.Viper, *log.Logger) (*app.App, func(), error) {
- panic(wire.Build(
- repositorySet,
- serverSet,
- newApp,
- ))
-}
diff --git a/cmd/migration/wire/wire_gen.go b/cmd/migration/wire/wire_gen.go
deleted file mode 100644
index 7dd0fec..0000000
--- a/cmd/migration/wire/wire_gen.go
+++ /dev/null
@@ -1,37 +0,0 @@
-// Code generated by Wire. DO NOT EDIT.
-
-//go:generate go run -mod=mod github.com/google/wire/cmd/wire
-//go:build !wireinject
-// +build !wireinject
-
-package wire
-
-import (
- "PandoraHelper/internal/repository"
- "PandoraHelper/internal/server"
- "PandoraHelper/pkg/app"
- "PandoraHelper/pkg/log"
- "github.com/google/wire"
- "github.com/spf13/viper"
-)
-
-// Injectors from wire.go:
-
-func NewWire(viperViper *viper.Viper, logger *log.Logger) (*app.App, func(), error) {
- db := repository.NewDB(viperViper, logger)
- migrate := server.NewMigrate(db, logger)
- appApp := newApp(migrate)
- return appApp, func() {
- }, nil
-}
-
-// wire.go:
-
-var repositorySet = wire.NewSet(repository.NewDB, repository.NewRepository)
-
-var serverSet = wire.NewSet(server.NewMigrate)
-
-// build App
-func newApp(migrate *server.Migrate) *app.App {
- return app.NewApp(app.WithServer(migrate), app.WithName("demo-migrate"))
-}
diff --git a/cmd/server/main.go b/cmd/server/main.go
index 5ef0d5d..0835cf2 100644
--- a/cmd/server/main.go
+++ b/cmd/server/main.go
@@ -2,6 +2,7 @@ package main
import (
"context"
+ "errors"
"flag"
"fmt"
@@ -27,13 +28,18 @@ import (
// @externalDocs.description OpenAPI
// @externalDocs.url https://swagger.io/resources/open-api/
func main() {
- var envConf = flag.String("conf", "config/local.yml", "config path, eg: -conf ./config/local.yml")
+ var envConf = flag.String("conf", "./config/", "config path, eg: -conf ./config/local.yml")
flag.Parse()
conf := config.NewConfig(*envConf)
logger := log.NewLog(conf)
app, cleanup, err := wire.NewWire(conf, logger)
+ pwd := conf.GetString("security.admin_password")
+ if pwd == "" || len(pwd) < 8 {
+ panic(errors.New("未设置密码或密码长度小于8"))
+ }
+
defer cleanup()
if err != nil {
panic(err)
diff --git a/cmd/server/wire/wire.go b/cmd/server/wire/wire.go
index 5e924b0..87152a9 100644
--- a/cmd/server/wire/wire.go
+++ b/cmd/server/wire/wire.go
@@ -39,6 +39,10 @@ var serviceSet = wire.NewSet(
server.NewTask,
)
+var migrateSet = wire.NewSet(
+ server.NewMigrate,
+)
+
var handlerSet = wire.NewSet(
handler.NewHandler,
handler.NewUserHandler,
@@ -52,9 +56,9 @@ var serverSet = wire.NewSet(
)
// build App
-func newApp(httpServer *http.Server, job *server.Job, task *server.Task) *app.App {
+func newApp(httpServer *http.Server, job *server.Job, task *server.Task, migrate *server.Migrate) *app.App {
return app.NewApp(
- app.WithServer(httpServer, job, task),
+ app.WithServer(httpServer, job, task, migrate),
app.WithName("demo-server"),
)
}
@@ -65,6 +69,7 @@ func NewWire(*viper.Viper, *log.Logger) (*app.App, func(), error) {
serviceSet,
handlerSet,
serverSet,
+ migrateSet,
sid.NewSid,
jwt.NewJwt,
newApp,
diff --git a/cmd/server/wire/wire_gen.go b/cmd/server/wire/wire_gen.go
index b815b5b..eb4d018 100644
--- a/cmd/server/wire/wire_gen.go
+++ b/cmd/server/wire/wire_gen.go
@@ -42,7 +42,8 @@ func NewWire(viperViper *viper.Viper, logger *log.Logger) (*app.App, func(), err
httpServer := server.NewHTTPServer(logger, viperViper, jwtJWT, userHandler, shareHandler, accountHandler)
job := server.NewJob(logger)
task := server.NewTask(logger, accountService, shareService)
- appApp := newApp(httpServer, job, task)
+ migrate := server.NewMigrate(db, logger)
+ appApp := newApp(httpServer, job, task, migrate)
return appApp, func() {
}, nil
}
@@ -55,11 +56,13 @@ var serviceCoordinatorSet = wire.NewSet(service.NewServiceCoordinator)
var serviceSet = wire.NewSet(service.NewService, service.NewUserService, serviceCoordinatorSet, service.NewAccountService, service.NewShareService, server.NewTask)
+var migrateSet = wire.NewSet(server.NewMigrate)
+
var handlerSet = wire.NewSet(handler.NewHandler, handler.NewUserHandler, handler.NewShareHandler, handler.NewAccountHandler)
var serverSet = wire.NewSet(server.NewHTTPServer, server.NewJob)
// build App
-func newApp(httpServer *http.Server, job *server.Job, task *server.Task) *app.App {
- return app.NewApp(app.WithServer(httpServer, job, task), app.WithName("demo-server"))
+func newApp(httpServer *http.Server, job *server.Job, task *server.Task, migrate *server.Migrate) *app.App {
+ return app.NewApp(app.WithServer(httpServer, job, task, migrate), app.WithName("demo-server"))
}
diff --git a/config.json b/config.json
new file mode 100644
index 0000000..06f24ad
--- /dev/null
+++ b/config.json
@@ -0,0 +1,29 @@
+{
+ "security": {
+ "admin_password": ""
+ },
+ "http": {
+ "host": "127.0.0.1",
+ "port": 8080
+ },
+ "database": {
+ "driver": "sqlite",
+ "dsn": "./data.db"
+ },
+ "pandora": {
+ "domain": {
+ "chat": "https://chat.oaifree.com",
+ "token": "https://token.oaifree.com",
+ "new": "https://new.oaifree.com"
+ }
+ },
+ "log": {
+ "level": "info",
+ "output": "console",
+ "log_file_name": "./logs/server.log",
+ "max_backups": 30,
+ "max_age": 7,
+ "max_size": 1024,
+ "compress": true
+ }
+}
\ No newline at end of file
diff --git a/config/local.yml b/config/local.yml
deleted file mode 100644
index 61c3124..0000000
--- a/config/local.yml
+++ /dev/null
@@ -1,29 +0,0 @@
-env: local
-http:
- # host: 0.0.0.0
- host: 127.0.0.1
- port: 8000
-security:
- admin_password: 123456
- # will be random
- jwt:
- key: QQYnRFerJTSEcrfB89fw8prOaObmrch8
-data:
- db:
- user:
- driver: sqlite
- dsn: storage/nunu-test.db?_busy_timeout=5000
-
-pandora:
- domain:
- chat: https://chat.oaifree.com
- token: https://token.oaifree.com
-
-log:
- log_level: info
- encoding: console # json or console
- log_file_name: "./storage/logs/server.log"
- max_backups: 30
- max_age: 7
- max_size: 1024
- compress: true
\ No newline at end of file
diff --git a/config/prod.yml b/config/prod.yml
deleted file mode 100644
index 27027cb..0000000
--- a/config/prod.yml
+++ /dev/null
@@ -1,37 +0,0 @@
-env: prod
-http:
- host: 0.0.0.0
- # host: 127.0.0.1
- port: 8000
-security:
- api_sign:
- app_key: 123456
- app_security: 123456
- jwt:
- key: QQYnRFerJTSEcrfB89fw8prOaObmrch8
-data:
- db:
- user:
- driver: sqlite
- dsn: storage/nunu-test.db?_busy_timeout=5000
- # user:
- # driver: mysql
- # dsn: root:123456@tcp(127.0.0.1:3380)/user?charset=utf8mb4&parseTime=True&loc=Local
- # user:
- # driver: postgres
- # dsn: host=localhost user=gorm password=gorm dbname=gorm port=9920 sslmode=disable TimeZone=Asia/Shanghai
- redis:
- addr: 127.0.0.1:6350
- password: ""
- db: 0
- read_timeout: 0.2s
- write_timeout: 0.2s
-
-log:
- log_level: info
- encoding: json # json or console
- log_file_name: "./storage/logs/server.log"
- max_backups: 30
- max_age: 7
- max_size: 1024
- compress: true
\ No newline at end of file
diff --git a/frontend/.dockerignore b/frontend/.dockerignore
new file mode 100644
index 0000000..8b7acb3
--- /dev/null
+++ b/frontend/.dockerignore
@@ -0,0 +1,5 @@
+.github
+.husky
+.vscode/
+dist/
+node_modules/
diff --git a/frontend/.editorconfig b/frontend/.editorconfig
new file mode 100644
index 0000000..dccf841
--- /dev/null
+++ b/frontend/.editorconfig
@@ -0,0 +1,19 @@
+root = true
+
+[*]
+charset=utf-8
+end_of_line=lf
+insert_final_newline=true
+indent_style=space
+indent_size=2
+max_line_length = 100
+
+[*.{yml,yaml,json}]
+indent_style = space
+indent_size = 2
+
+[*.md]
+trim_trailing_whitespace = false
+
+[Makefile]
+indent_style = tab
diff --git a/frontend/.env b/frontend/.env
new file mode 100644
index 0000000..25d78e7
--- /dev/null
+++ b/frontend/.env
@@ -0,0 +1,2 @@
+VITE_GLOB_APP_TITLE = Vite React TS Template
+REACT_EDITOR=webstorm
diff --git a/frontend/.env.development b/frontend/.env.development
new file mode 100644
index 0000000..835c5d2
--- /dev/null
+++ b/frontend/.env.development
@@ -0,0 +1,2 @@
+VITE_APP_BASE_API=/api
+VITE_APP_HOMEPAGE=/home
diff --git a/frontend/.env.production b/frontend/.env.production
new file mode 100644
index 0000000..835c5d2
--- /dev/null
+++ b/frontend/.env.production
@@ -0,0 +1,2 @@
+VITE_APP_BASE_API=/api
+VITE_APP_HOMEPAGE=/home
diff --git a/frontend/.eslintignore b/frontend/.eslintignore
new file mode 100644
index 0000000..8647ad9
--- /dev/null
+++ b/frontend/.eslintignore
@@ -0,0 +1,22 @@
+*.sh
+node_modules
+*.lock
+**/*.svg
+**/*.md
+**/*.svg
+**/*.ejs
+**/*.html
+**/*.png
+**/*.toml
+**/*.md
+.vscode
+.idea
+dist
+/public
+/docs
+.husky
+.local
+/bin
+Dockerfile
+pnpm-lock.yaml
+tsconfig.node.json
diff --git a/frontend/.eslintrc.cjs b/frontend/.eslintrc.cjs
new file mode 100644
index 0000000..d5ef074
--- /dev/null
+++ b/frontend/.eslintrc.cjs
@@ -0,0 +1,144 @@
+module.exports = {
+ root: true, // 表示当前目录即为根目录,ESLint 规则将被限制到该目录下
+ env: { browser: true, es2020: true, node: true },
+ /* 解析器 */
+ parser: '@typescript-eslint/parser', // 指定ESLint解析器
+ parserOptions: {
+ project: './tsconfig.json', // tsconfig.json的路径
+ ecmaVersion: 'latest',
+ sourceType: 'module',
+ ecmaFeatures: {
+ jsx: true, // 启用JSX
+ },
+ extraFileExtensions: ['.json'],
+ },
+ settings: {
+ // 识别 @ # alias
+ 'import/resolver': {
+ alias: {
+ map: [
+ ['@', './src'],
+ ['#', './types'],
+ ],
+ extensions: ['.ts', '.tsx', '.js', '.jsx', '.json'],
+ },
+ },
+ },
+ /* ESLint 中基础配置需要继承的配置 */
+ extends: [
+ 'airbnb',
+ 'airbnb-typescript',
+ 'airbnb/hooks',
+ 'plugin:@typescript-eslint/recommended', // 使用@typescript-eslint/eslint-plugin推荐的规则
+ 'plugin:jsx-a11y/recommended',
+ 'plugin:import/errors',
+ 'plugin:import/warnings',
+ 'prettier', // 增加 prettier 相关的校验规则
+ 'plugin:prettier/recommended', // 开启 Prettier 插件推荐的规则
+ ],
+ /* ESLint文件所依赖的插件 */
+ plugins: [
+ '@typescript-eslint',
+ 'prettier',
+ 'react',
+ 'react-hooks',
+ 'jsx-a11y',
+ 'import',
+ 'unused-imports',
+ ],
+ /**
+ * 定义规则
+ * "off" 或 0 - 关闭规则
+ * "warn" 或 1 - 开启规则,使用警告级别的错误:warn (不会导致程序退出)
+ * "error" 或 2 - 开启规则,使用错误级别的错误:error (当被触发的时候,程序会退出)
+ */
+ rules: {
+ 'no-console': 'off',
+ 'no-unused-vars': 'off',
+ 'no-case-declarations': 'off',
+ 'no-use-before-define': 'off',
+ 'no-param-reassign': 'off',
+ 'space-before-function-paren': 'off',
+ 'class-methods-use-this': 'off',
+
+ 'jsx-a11y/click-events-have-key-events': 'off',
+ 'jsx-a11y/interactive-supports-focus': 'off',
+ 'jsx-a11y/no-noninteractive-element-interactions': 'off',
+ 'jsx-a11y/no-static-element-interactions': 'off',
+
+ // 不用手动引入react
+ 'react/react-in-jsx-scope': 'off',
+ 'react/button-has-type': 'off',
+ 'react/require-default-props': 'off',
+ 'react/no-array-index-key': 'off',
+ 'react/jsx-props-no-spreading': 'off',
+
+ 'import/first': 'warn',
+ 'import/newline-after-import': 'warn',
+ 'import/no-duplicates': 'warn',
+ 'import/no-extraneous-dependencies': 'off',
+ 'import/prefer-default-export': 'off',
+ 'import/order': [
+ 'warn',
+ {
+ groups: [
+ 'builtin', // Node.js内置模块
+ 'external', // 第三方模块
+ 'internal', // 应用程序内部的模块
+ 'parent', // 父级目录中导入的模块
+ ['sibling', 'index'], // 具有相同或更高目录的兄弟模块
+ 'object',
+ 'type',
+ ],
+ pathGroups: [
+ {
+ pattern: '@/**',
+ group: 'internal',
+ },
+ {
+ pattern: '#/**',
+ group: 'type',
+ },
+ {
+ pattern: '*.{scss,css,less,styl,stylus}',
+ group: 'parent',
+ },
+ {
+ pattern: '*.{js,jsx,ts,tsx}',
+ group: 'sibling',
+ },
+ ],
+ 'newlines-between': 'always', // 在组之间插入空行
+ pathGroupsExcludedImportTypes: ['sibling', 'index'],
+ warnOnUnassignedImports: true,
+ alphabetize: { order: 'asc', caseInsensitive: true }, // 对于每个组,按字母表顺序排序。
+ },
+ ],
+
+ 'unused-imports/no-unused-imports-ts': 'warn',
+ 'unused-imports/no-unused-vars-ts': [
+ 'warn',
+ { vars: 'all', varsIgnorePattern: '^_', args: 'after-used', argsIgnorePattern: '^_' },
+ ],
+
+ '@typescript-eslint/no-unused-vars': [
+ 'warn',
+ {
+ argsIgnorePattern: '^_',
+ varsIgnorePattern: '^_',
+ },
+ ],
+ '@typescript-eslint/no-unused-expressions': 'off',
+ '@typescript-eslint/ban-ts-ignore': 'off',
+ '@typescript-eslint/ban-ts-comment': 'off',
+ '@typescript-eslint/ban-types': 'off',
+ '@typescript-eslint/explicit-function-return-type': 'off',
+ '@typescript-eslint/no-explicit-any': 'off',
+ '@typescript-eslint/no-var-requires': 'off',
+ '@typescript-eslint/no-empty-function': 'off',
+ '@typescript-eslint/no-use-before-define': 'off',
+ '@typescript-eslint/no-non-null-assertion': 'off',
+ '@typescript-eslint/no-shadow': 'off',
+ '@typescript-eslint/explicit-module-boundary-types': 'off',
+ },
+};
diff --git a/frontend/.github/workflows/ci.yml b/frontend/.github/workflows/ci.yml
new file mode 100644
index 0000000..b9ed669
--- /dev/null
+++ b/frontend/.github/workflows/ci.yml
@@ -0,0 +1,46 @@
+name: CI
+
+on:
+ push:
+ branches: [ "main" ]
+ pull_request:
+ branches: [ "main" ]
+
+jobs:
+ build-and-deploy:
+
+ runs-on: ubuntu-latest
+
+ strategy:
+ matrix:
+ node-version: [16.x]
+ # See supported Node.js release schedule at https://nodejs.org/en/about/releases/
+
+ steps:
+ - name: Checkout 🛎️
+ uses: actions/checkout@v3
+
+ - name: Setup pnpm
+ uses: pnpm/action-setup@v2
+ with:
+ version: 8
+
+ - name: Setup Node.js ${{ matrix.node-version }}
+ uses: actions/setup-node@v3
+ with:
+ node-version: ${{ matrix.node-version }}
+ cache: 'pnpm'
+
+ - name: Install and Build 🔧
+ run: |
+ pnpm install
+ pnpm build
+
+ - name: Deploy 🚀
+ uses: JamesIves/github-pages-deploy-action@v4
+ with:
+ TOKEN: ${{ secrets.ACCESS_TOKEN }}
+ FOLDER: dist
+ CLEAN: true
+
+
diff --git a/frontend/.gitignore b/frontend/.gitignore
new file mode 100644
index 0000000..86144f8
--- /dev/null
+++ b/frontend/.gitignore
@@ -0,0 +1,29 @@
+# Logs
+logs
+*.log
+npm-debug.log*
+yarn-debug.log*
+yarn-error.log*
+pnpm-debug.log*
+lerna-debug.log*
+
+node_modules
+dist-ssr
+*.local
+
+# Editor directories and files
+.idea
+.DS_Store
+*.suo
+*.ntvs*
+*.njsproj
+*.sln
+*.sw?
+.vscode
+.husky
+
+# vite 打包分析产物
+stats.html
+dist
+
+
diff --git a/frontend/.lintstagedrc b/frontend/.lintstagedrc
new file mode 100644
index 0000000..784055b
--- /dev/null
+++ b/frontend/.lintstagedrc
@@ -0,0 +1,5 @@
+{
+ "src/**/*.{js,jsx,ts,tsx}": ["eslint --fix"],
+ "src/**/*.{css,scss}": ["stylelint --fix"],
+ "*.{json,md}": ["prettier --write"]
+}
diff --git a/frontend/.prettierignore b/frontend/.prettierignore
new file mode 100644
index 0000000..b172d95
--- /dev/null
+++ b/frontend/.prettierignore
@@ -0,0 +1,21 @@
+*.sh
+node_modules
+*.lock
+**/*.svg
+**/*.md
+**/*.svg
+**/*.ejs
+**/*.html
+**/*.png
+**/*.toml
+**/*.md
+.vscode
+.idea
+dist
+/public
+/docs
+.husky
+.local
+/bin
+Dockerfile
+pnpm-lock.yaml
diff --git a/frontend/.prettierrc b/frontend/.prettierrc
new file mode 100644
index 0000000..6df60f9
--- /dev/null
+++ b/frontend/.prettierrc
@@ -0,0 +1,21 @@
+{
+ "printWidth": 100,
+ "semi": true,
+ "tabWidth": 2,
+ "singleQuote": true,
+ "trailingComma": "all",
+ "proseWrap": "never",
+ "htmlWhitespaceSensitivity": "strict",
+ "endOfLine": "auto",
+ "overrides": [
+ {
+ "files": "*rc",
+ "options": {
+ "parser": "json"
+ }
+ }
+ ],
+ "plugins": [
+ "prettier-plugin-tailwindcss"
+ ]
+}
diff --git a/frontend/.stylelintignore b/frontend/.stylelintignore
new file mode 100644
index 0000000..b715f87
--- /dev/null
+++ b/frontend/.stylelintignore
@@ -0,0 +1,8 @@
+node_modules/
+dist/
+build/
+*.min.css
+*.js
+*.tsx
+*.ts
+*.json
diff --git a/frontend/.stylelintrc b/frontend/.stylelintrc
new file mode 100644
index 0000000..a751afb
--- /dev/null
+++ b/frontend/.stylelintrc
@@ -0,0 +1,71 @@
+{
+ "extends": [
+ "stylelint-config-standard",
+ "stylelint-config-rational-order"
+ ],
+ "plugins": ["stylelint-order"],
+ "rules": {
+ "indentation": 2,
+ "declaration-colon-space-after": "always",
+ "declaration-colon-space-before": "never",
+ "declaration-block-semicolon-newline-after": "always-multi-line",
+ "selector-not-notation": null,
+ "import-notation": null,
+ "function-no-unknown": null,
+ "selector-class-pattern": null,
+ "selector-pseudo-class-no-unknown": [
+ true,
+ {
+ "ignorePseudoClasses": ["global", "local"]
+ }
+ ],
+ "at-rule-no-unknown": [
+ true,
+ {
+ "ignoreAtRules": [
+ "tailwind",
+ "apply",
+ "variants",
+ "responsive",
+ "screen",
+ "function",
+ "if",
+ "each",
+ "include",
+ "mixin",
+ "extend"
+ ]
+ }
+ ],
+ "no-empty-source": null,
+ "string-quotes": null,
+ "named-grid-areas-no-invalid": null,
+ "no-descending-specificity": null,
+ "font-family-no-missing-generic-family-keyword": null,
+ "rule-empty-line-before": [
+ "always",
+ {
+ "ignore": ["after-comment", "first-nested"]
+ }
+ ],
+ "unit-no-unknown": [true, { "ignoreUnits": ["rpx"] }],
+ "order/order": [
+ [
+ "dollar-variables",
+ "custom-properties",
+ "at-rules",
+ "declarations",
+ {
+ "type": "at-rule",
+ "name": "supports"
+ },
+ {
+ "type": "at-rule",
+ "name": "media"
+ },
+ "rules"
+ ],
+ { "severity": "error" }
+ ]
+ }
+}
diff --git a/frontend/Dockerfile b/frontend/Dockerfile
new file mode 100644
index 0000000..00f5ab7
--- /dev/null
+++ b/frontend/Dockerfile
@@ -0,0 +1,22 @@
+# Stage 1: build stage
+FROM node:16-alpine as build-stage
+# make the 'app' folder the current working directory
+WORKDIR /app
+# copy project files and folders to the current working directory (i.e. 'app' folder)
+COPY . ./
+# config node options
+ENV NODE_OPTIONS=--max_old_space_size=8192
+# config pnpm, install dependencies and build
+RUN npm install pnpm -g && \
+ pnpm install && \
+ pnpm build
+RUN echo "build successful 🎉 🎉 🎉"
+
+
+# Stage 2: production stage
+FROM nginx:latest as production-stage
+COPY --from=build-stage /app/dist /usr/share/nginx/html
+EXPOSE 80
+CMD ["nginx", "-g", "daemon off;"]
+RUN echo "deploy to nginx successful 🎉 🎉 🎉"
+
diff --git a/frontend/LICENSE b/frontend/LICENSE
new file mode 100644
index 0000000..ceb73cc
--- /dev/null
+++ b/frontend/LICENSE
@@ -0,0 +1,21 @@
+MIT License
+
+Copyright (c) 2023 d3george
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/frontend/README.md b/frontend/README.md
new file mode 100644
index 0000000..e28ca5e
--- /dev/null
+++ b/frontend/README.md
@@ -0,0 +1,116 @@
+
+
+
+
Slash Admin
+
+
+
+**English** | [中文](./README.zh-CN.md)
+
+## Introduction
+Slash Admin is a modern admin dashboard template built with React 18, Vite, Ant Design, and TypeScript. It is designed to help developers quickly create powerful admin management systems.
+
+## Preview
++ https://admin.slashspaces.com/
+
+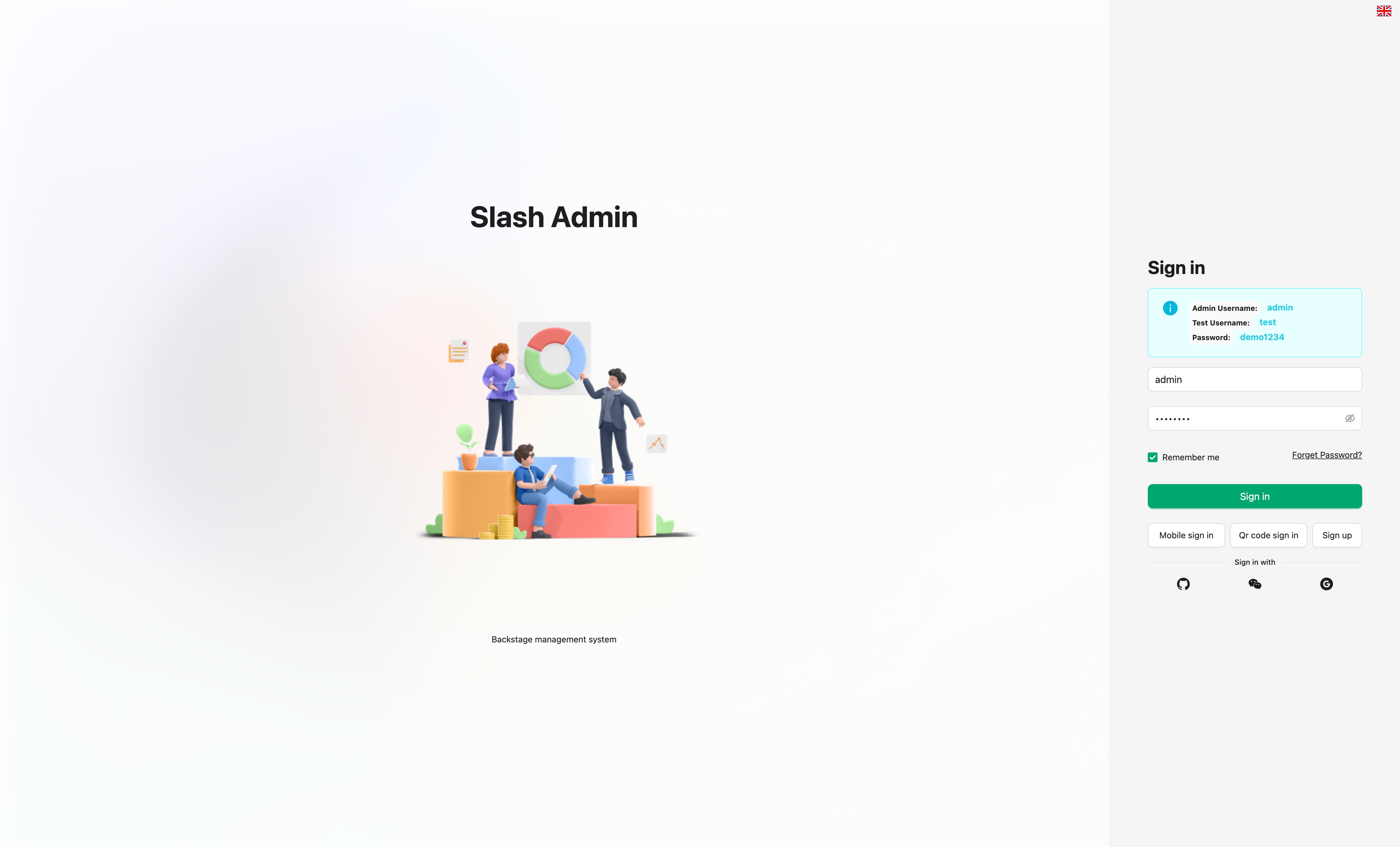
+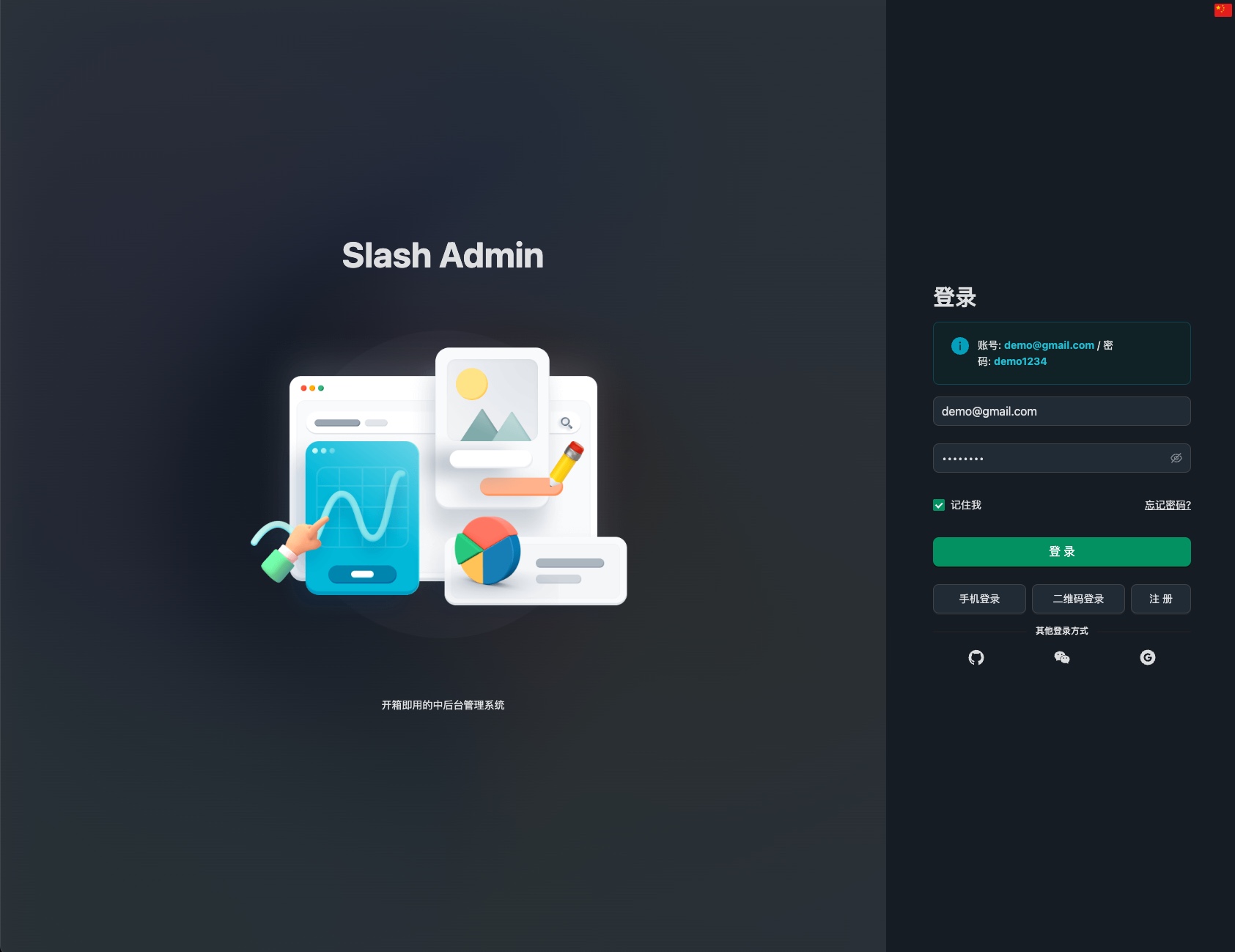
+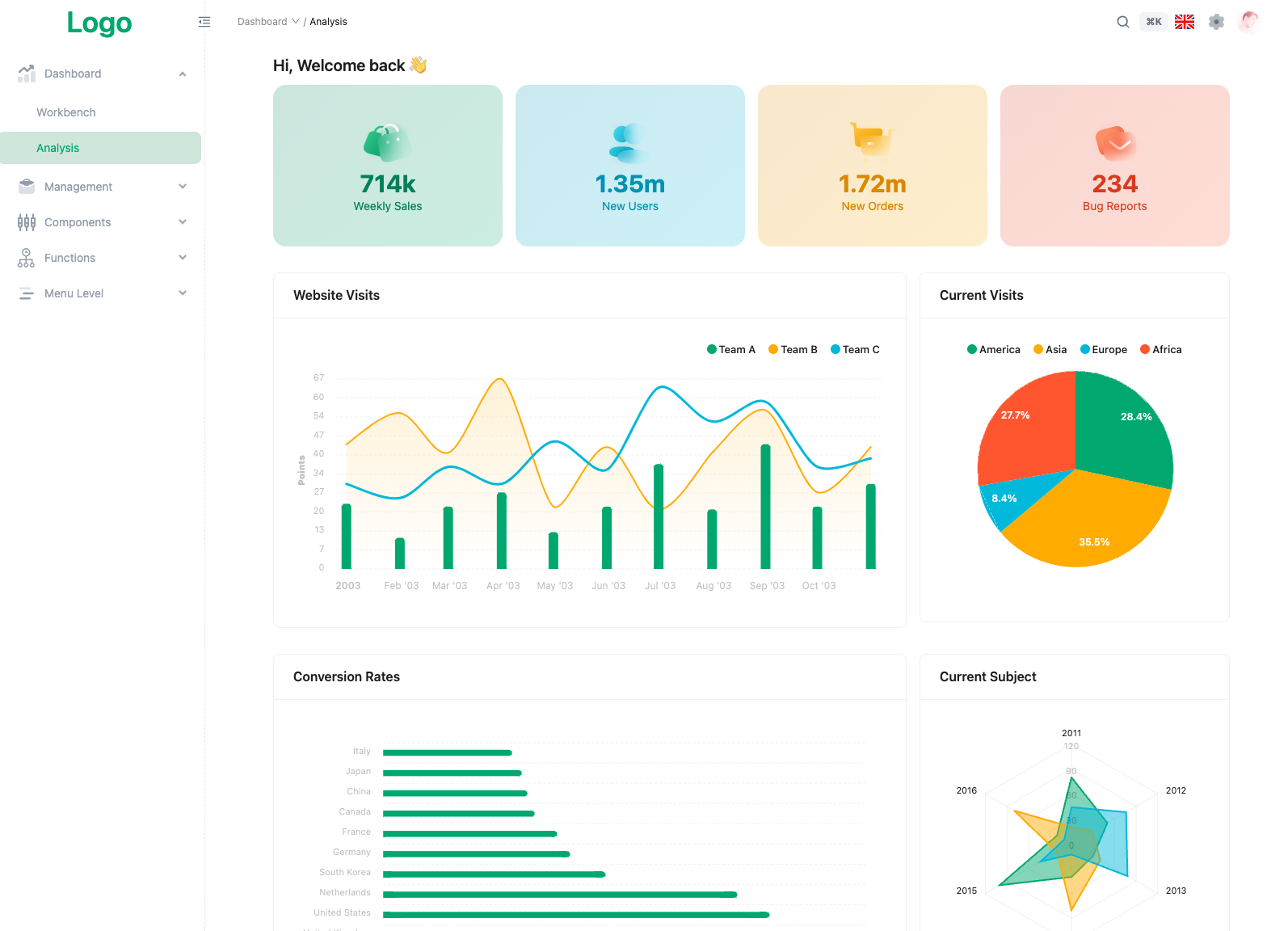
+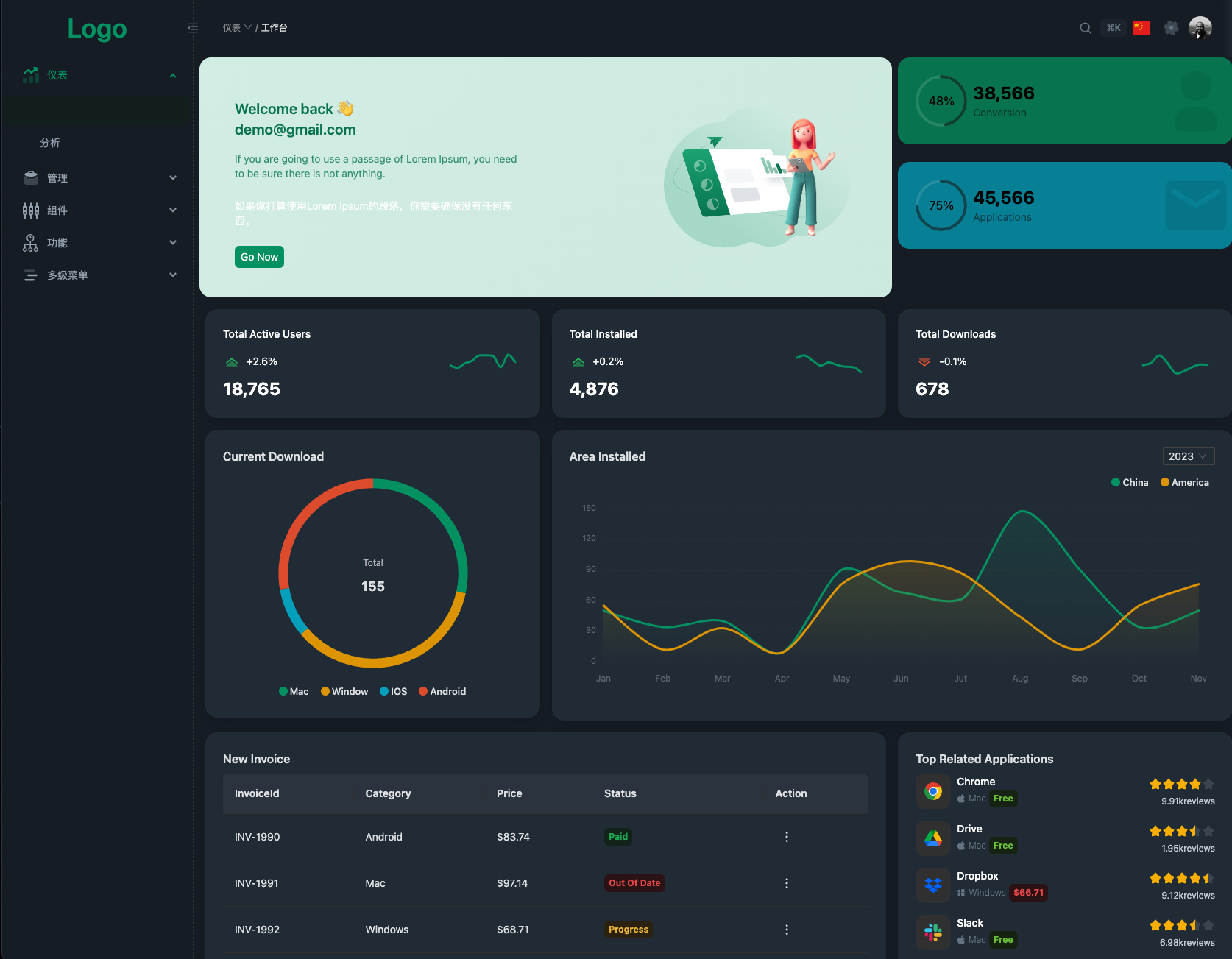
+
+## Features
+
+- Built using React 18 hooks.
+- Powered by Vite for rapid development and hot module replacement.
+- Integrates Ant Design, providing a rich set of UI components and design patterns.
+- Written in TypeScript, offering type safety and an improved development experience.
+- Responsive design, adapting to various screen sizes and devices.
+- Flexible routing configuration, supporting nested routes.
+- Integrated access control based on user roles.
+- Supports internationalization for easy language switching.
+- Includes common admin features like user management, role management, and permission management.
+- Customizable themes and styles to meet your branding needs.
+- Mocking solution based on MSW and Faker.js.
+- State management using Zustand.
+- Data fetching using React-Query.
+
+## Quick Start
+
+### Get the Project Code
+
+```bash
+git clone https://github.com/d3george/slash-admin.git
+```
+
+### Install Dependencies
+
+In the project's root directory, run the following command to install project dependencies:
+
+```bash
+pnpm install
+```
+
+### Start the Development Server
+
+Run the following command to start the development server:
+
+```bash
+pnpm dev
+```
+
+Visit [http://localhost:3001](http://localhost:3001) to view your application.
+
+### Build for Production
+
+Run the following command to build the production version:
+
+```bash
+pnpm build
+```
+
+## Docker deployment
+
+
+### Build image and Run container
+#### build image
+Enter the project root directory in the terminal and execute the following command to build the Docker image:
+```
+docker build -t your-image-name .
+```
+Make sure to replace `your-image-name` with your own image name
+
+#### run container
+Run your application in the Docker container using the following command:
+```
+docker run -p 3001:80 your-image-name
+```
+This will run your application on port `80`(exposed in `Dockerfile`) of the container and map it to port `3001` on your host.
+
+Now you can access http://localhost:3001 to view the deployed applications.
+
+### use docker-compose.yaml
+Enter the project root directory in the terminal and execute the following command to start Docker Compose:
+```
+docker-compose up -d
+```
+Docker Compose will build an image based on the configuration defined by 'docker-compose. yaml' and run the container in the background.
+
+After the container runs successfully, it can also be accessed through http://localhost:3001 To view the deployed applications.
+
+
+## Git Contribution submission specification
+
+reference[.commitlint.config.js](./commitlint.config.js)
+
+- `feat` new features
+- `fix` fix the
+- `docs` documentation or comments
+- `style` code format (changes that do not affect code execution)
+- `refactor` refactor
+- `perf` performance optimization
+- `revert` revert commit
+- `test` test related
+- `chore` changes in the construction process or auxiliary tools
+- `ci` modify CI configuration and scripts
+- `types` type definition file changes
+- `wip` in development
diff --git a/frontend/README.zh-CN.md b/frontend/README.zh-CN.md
new file mode 100644
index 0000000..97d1461
--- /dev/null
+++ b/frontend/README.zh-CN.md
@@ -0,0 +1,115 @@
+
+
+
+
Slash Admin
+
+
+
+**中文** | [English](./README.md)
+
+## 简介
+
+Slash Admin 是一个现代化的后台管理模板,基于 React 18、Vite、Ant Design 和 TypeScript 构建。它旨在帮助开发人员快速搭建功能强大的后台管理系统。
+
+## 预览
++ https://admin.slashspaces.com/
+
+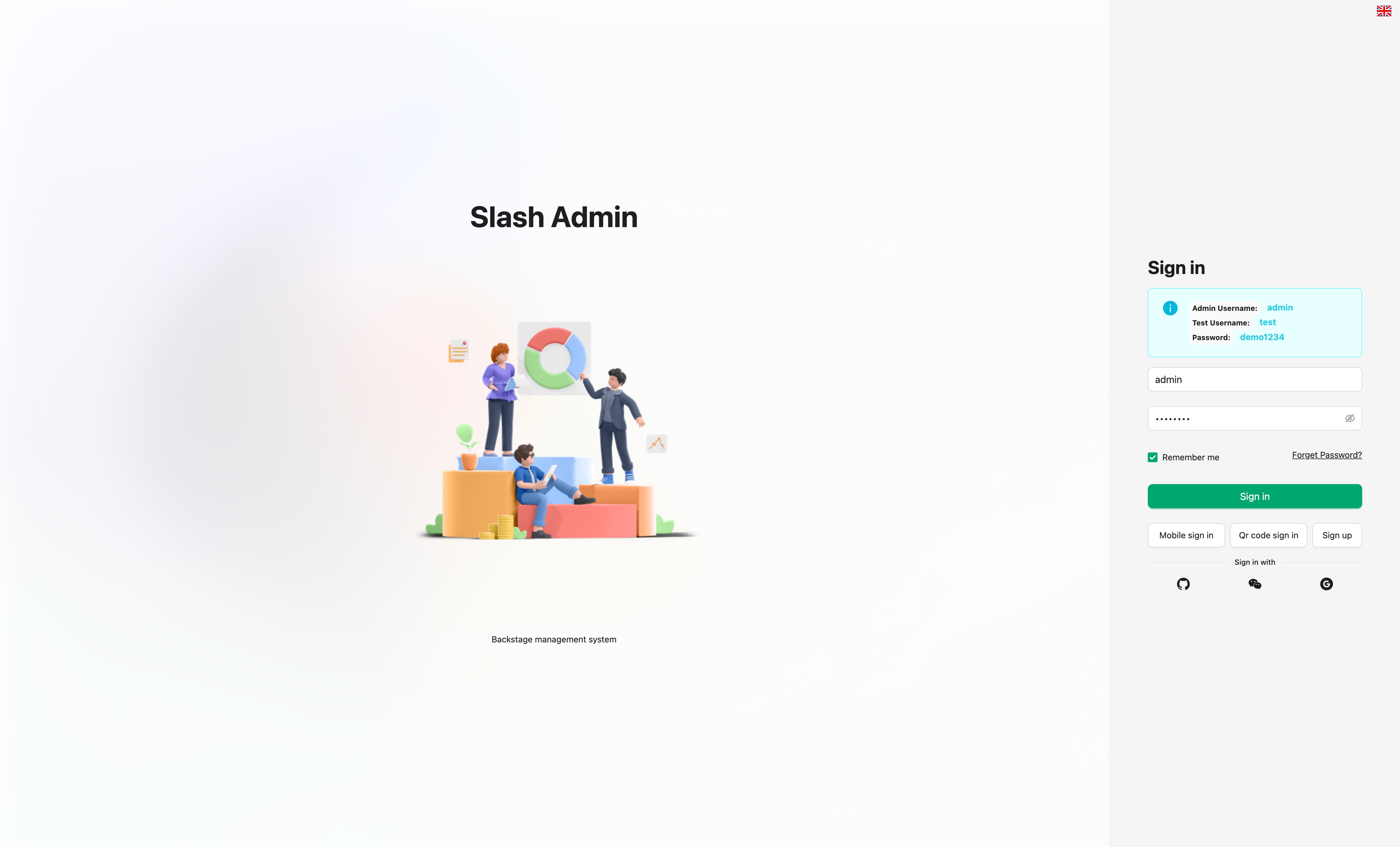
+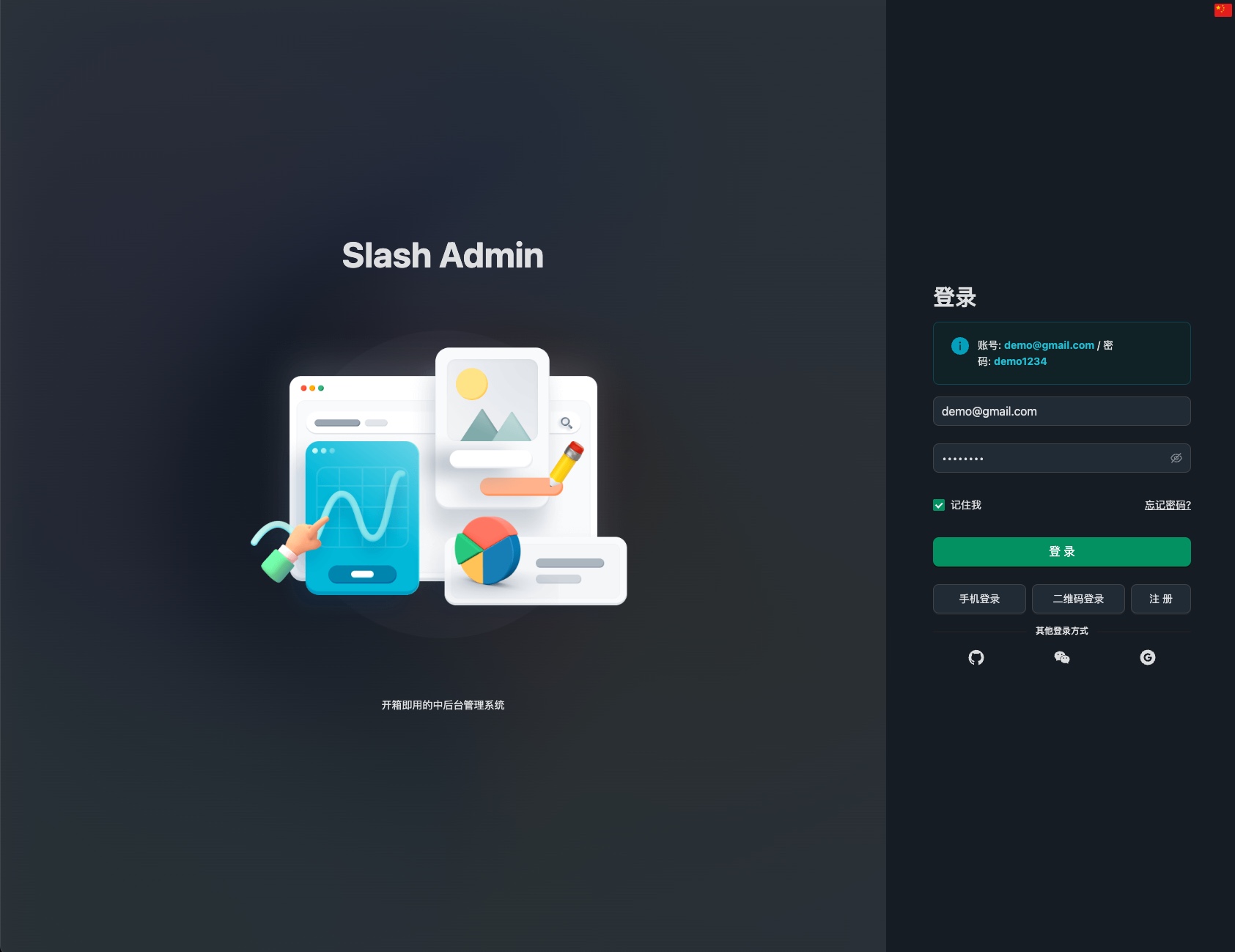
+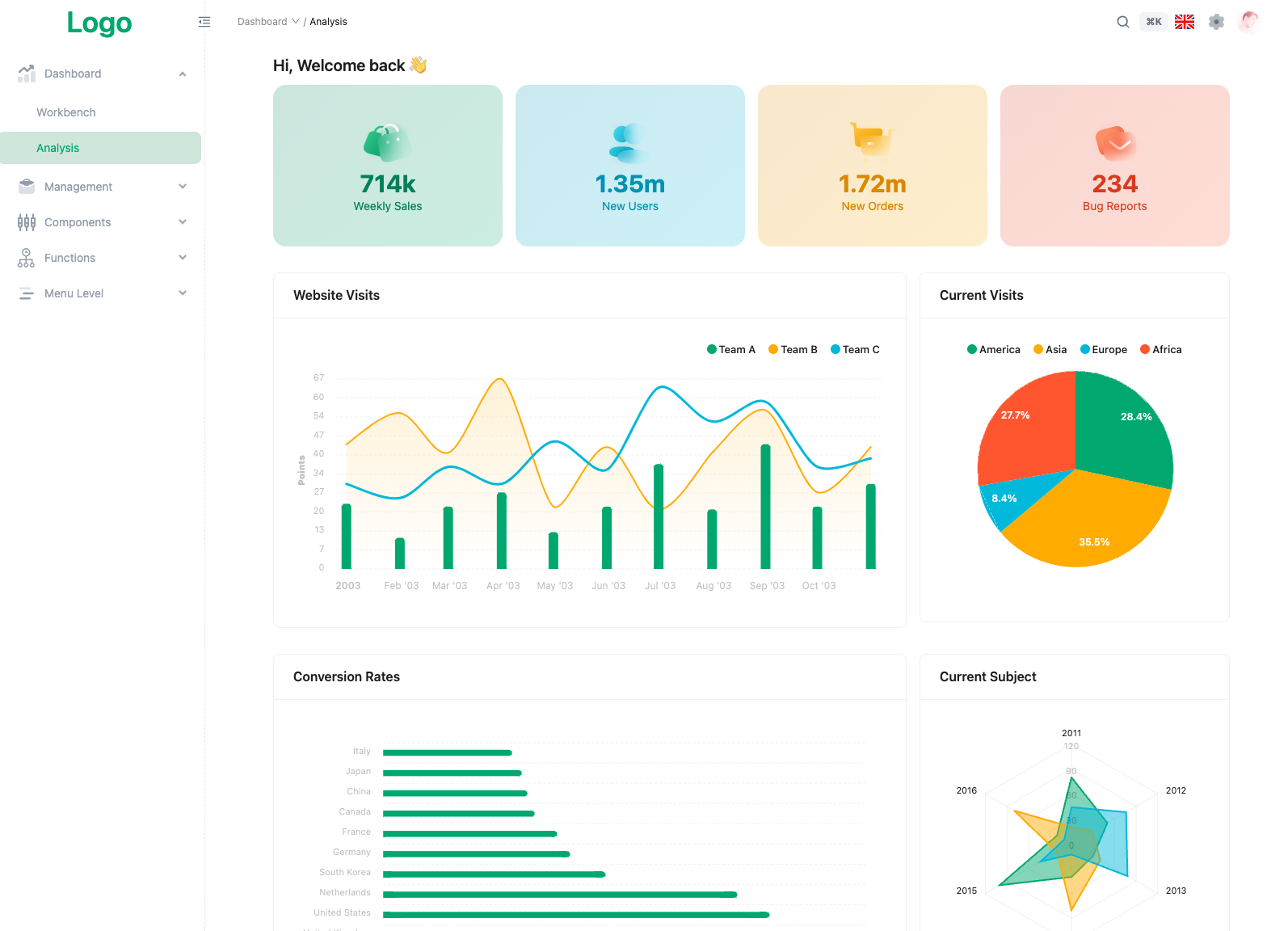
+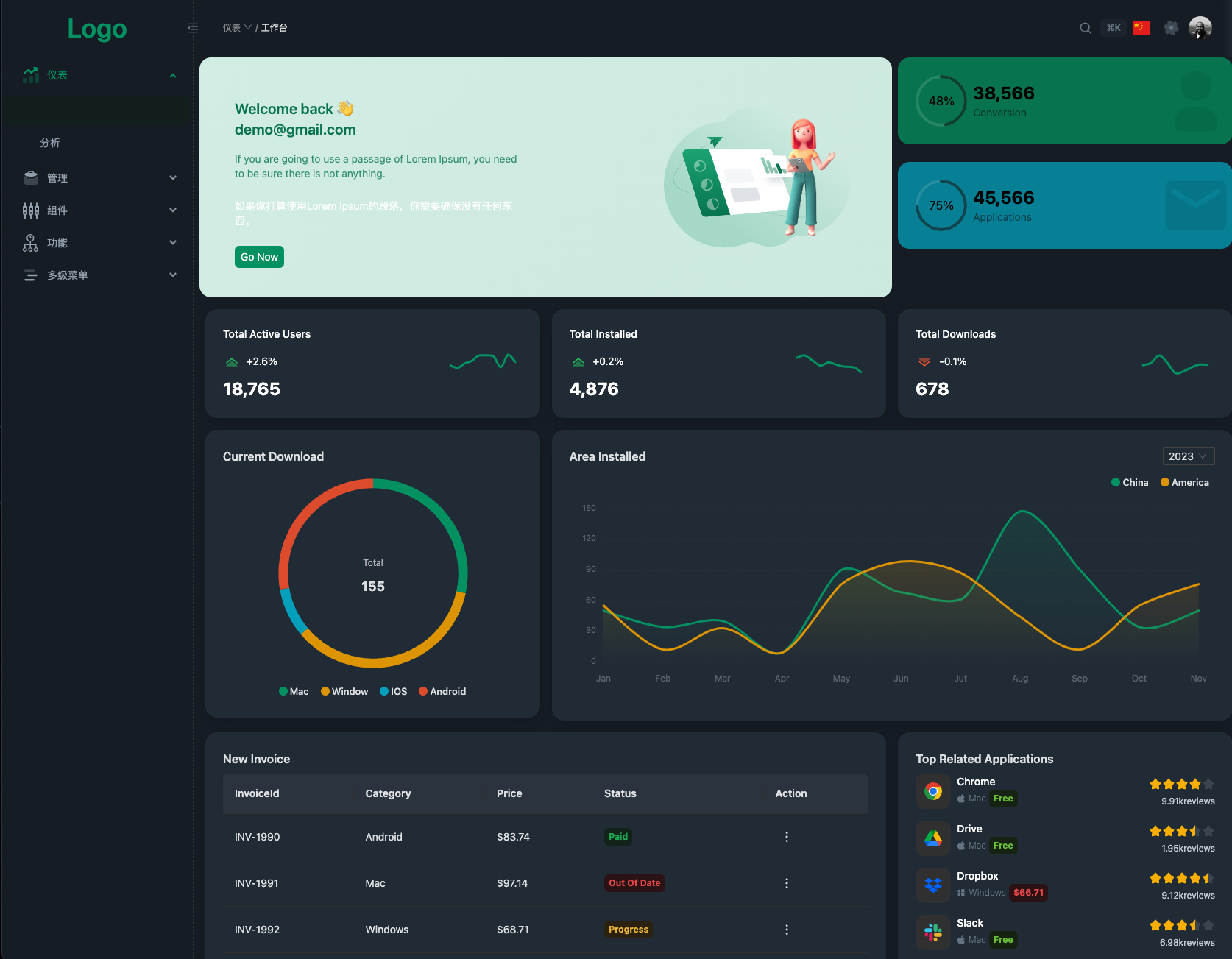
+## 特性
+
+- 使用 React 18 hooks 进行构建。
+- 基于 Vite 进行快速开发和热模块替换。
+- 集成 Ant Design,提供丰富的 UI 组件和设计模式。
+- 使用 TypeScript 编写,提供类型安全性和更好的开发体验。
+- 响应式设计,适应各种屏幕尺寸和设备。
+- 灵活的路由配置,支持多级嵌套路由。
+- 集成权限管理,根据用户角色控制页面访问权限。
+- 集成国际化支持,轻松切换多语言。
+- 集成常见的后台管理功能,如用户管理、角色管理、权限管理等。
+- 可定制的主题和样式,以满足您的品牌需求。
+- 基于 MSW 和 Faker.js 的Mock方案
+- 使用 Zustand 进行状态管理
+- 使用 React-Query 进行数据获取
+
+## 快速开始
+
+### 获取项目代码
+
+```bash
+git clone https://github.com/d3george/slash-admin.git
+```
+
+### 安装依赖
+
+在项目根目录下运行以下命令安装项目依赖:
+
+```bash
+pnpm install
+```
+
+### 启动开发服务器
+
+运行以下命令以启动开发服务器:
+
+```bash
+pnpm dev
+```
+
+访问 [http://localhost:3001](http://localhost:3001) 查看您的应用程序。
+
+### 构建生产版本
+
+运行以下命令以构建生产版本:
+
+```bash
+pnpm build
+```
+
+构建后的文件将位于 `dist` 目录中。
+
+## 容器化部署
+
+### 构建镜像并运行容器
+#### 构建镜像
+在终端中进入项目根目录,并执行以下命令来构建 Docker 镜像:
+```
+docker build -t your-image-name .
+```
+确保将 `your-image-name` 替换为你自己的镜像名称
+
+#### 运行容器
+使用以下命令在 Docker 容器中运行你的应用:
+```
+docker run -p 3001:80 your-image-name
+```
+这将在容器的端口 `80` (暴露在`Dockerfile`中) 上运行你的应用,并将其映射到你主机的端口 `3001` 上。
+
+现在,你可以通过访问 http://localhost:3001 来查看部署的应用。
+
+
+### 使用docker-compose.yaml
+在终端中进入项目根目录,并执行以下命令来启动 Docker Compose:
+```
+docker-compose up -d
+```
+Docker Compose 根据`docker-compose.yaml`定义的配置构建镜像并在后台运行容器.
+
+容器运行成功后,同样可以通过访问 http://localhost:3001来查看部署的应用。
+
+参考[.commitlint.config.js](./commitlint.config.js)
+
+- `feat` 新功能
+- `fix` 修复bug
+- `docs` 文档注释
+- `style` 代码格式(不影响代码运行的变动)
+- `refactor` 重构
+- `perf` 性能优化
+- `revert` 回滚commit
+- `test` 测试相关
+- `chore` 构建过程或辅助工具的变动
+- `ci` 修改CI配置、脚本
+- `types` 类型定义文件修改
+- `wip` 开发中
diff --git a/frontend/commitlint.config.js b/frontend/commitlint.config.js
new file mode 100644
index 0000000..66686e4
--- /dev/null
+++ b/frontend/commitlint.config.js
@@ -0,0 +1,30 @@
+export default {
+ // 继承的规则
+ extends: ['@commitlint/config-conventional'],
+ // 定义规则类型
+ rules: {
+ 'body-leading-blank': [2, 'always'], // 确保提交消息正文之前有一行空白行
+ 'type-empty': [2, 'never'], // 不允许提交消息的 type 类型为空
+ 'subject-case': [0], // subject 大小写不做校验
+ // type 类型定义,表示 git 提交的 type 必须在以下类型范围内
+ 'type-enum': [
+ 2,
+ 'always',
+ [
+ 'feat', // 新功能 feature
+ 'fix', // 修复 bug
+ 'docs', // 文档注释
+ 'style', // 代码格式(不影响代码运行的变动)
+ 'refactor', // 重构(既不增加新功能,也不是修复bug)
+ 'perf', // 性能优化
+ 'test', // 添加疏漏测试或已有测试改动
+ 'chore', // 构建过程或辅助工具的变动
+ 'revert', // 回滚commit
+ 'build', // 构建流程、外部依赖变更 (如升级 npm 包、修改打包配置等)',
+ 'ci', // 修改CI配置、脚本
+ 'types', // 类型定义文件修改
+ 'wip', // 开发中
+ ],
+ ],
+ },
+};
diff --git a/frontend/docker-compose.yaml b/frontend/docker-compose.yaml
new file mode 100644
index 0000000..c34a48a
--- /dev/null
+++ b/frontend/docker-compose.yaml
@@ -0,0 +1,7 @@
+services:
+ slash:
+ build:
+ context: .
+ ports:
+ - "3001:80"
+ restart: always
diff --git a/frontend/index.html b/frontend/index.html
new file mode 100644
index 0000000..f69755f
--- /dev/null
+++ b/frontend/index.html
@@ -0,0 +1,14 @@
+
+
+
+
+
+
+ Slash Admin
+
+
+ You need to enable JavaScript to run this app.
+
+
+
+
diff --git a/frontend/package.json b/frontend/package.json
new file mode 100644
index 0000000..eca4cad
--- /dev/null
+++ b/frontend/package.json
@@ -0,0 +1,118 @@
+{
+ "name": "slash-admin",
+ "private": true,
+ "version": "0.0.0",
+ "type": "module",
+ "homepage": "https://d3george.github.io/react-admin-template",
+ "scripts": {
+ "dev": "vite",
+ "build": "tsc && vite build",
+ "lint": "eslint src --ext ts,tsx --report-unused-disable-directives --max-warnings 0",
+ "preview": "vite preview",
+ "prepare": "cd .. && husky install frontend/.husky"
+ },
+ "dependencies": {
+ "@ant-design/cssinjs": "^1.17.2",
+ "@ant-design/icons": "^5.2.6",
+ "@fullcalendar/common": "^5.11.5",
+ "@fullcalendar/core": "^6.1.9",
+ "@fullcalendar/daygrid": "^6.1.9",
+ "@fullcalendar/interaction": "^6.1.9",
+ "@fullcalendar/list": "^6.1.9",
+ "@fullcalendar/react": "^6.1.9",
+ "@fullcalendar/timegrid": "^6.1.9",
+ "@fullcalendar/timeline": "^6.1.9",
+ "@hcaptcha/react-hcaptcha": "^1.9.3",
+ "@iconify/react": "^4.1.1",
+ "@tanstack/react-query": "^4.36.1",
+ "@tanstack/react-query-devtools": "^4.36.1",
+ "@vitejs/plugin-react": "^4.1.0",
+ "antd": "^5.9.3",
+ "apexcharts": "^3.43.0",
+ "autosuggest-highlight": "^3.3.4",
+ "axios": "^1.5.1",
+ "classnames": "^2.3.2",
+ "color": "^4.2.3",
+ "dayjs": "^1.11.10",
+ "framer-motion": "^10.16.4",
+ "highlight.js": "^11.9.0",
+ "humps": "^2.0.1",
+ "i18next": "^23.5.1",
+ "i18next-browser-languagedetector": "^7.1.0",
+ "nprogress": "^0.2.0",
+ "numeral": "^2.0.6",
+ "ramda": "^0.29.1",
+ "react": "^18.2.0",
+ "react-apexcharts": "^1.4.1",
+ "react-beautiful-dnd": "^13.1.1",
+ "react-dom": "^18.2.0",
+ "react-helmet-async": "^1.3.0",
+ "react-i18next": "^13.2.2",
+ "react-icons": "^4.11.0",
+ "react-markdown": "^8.0.7",
+ "react-organizational-chart": "^2.2.1",
+ "react-quill": "^2.0.0",
+ "react-router-dom": "^6.16.0",
+ "react-use": "^17.4.0",
+ "rehype-highlight": "^6.0.0",
+ "rehype-raw": "^6.1.1",
+ "remark-gfm": "^3.0.1",
+ "reset-css": "^5.0.2",
+ "screenfull": "^6.0.2",
+ "simplebar-react": "^3.2.4",
+ "styled-components": "^6.0.9",
+ "vite": "^4.4.11",
+ "zustand": "^4.4.3"
+ },
+ "devDependencies": {
+ "@commitlint/cli": "^17.7.2",
+ "@commitlint/config-conventional": "^17.7.0",
+ "@react-dev-inspector/vite-plugin": "^2.0.0",
+ "@types/autosuggest-highlight": "^3.2.0",
+ "@types/color": "^3.0.4",
+ "@types/humps": "^2.0.6",
+ "@types/nprogress": "^0.2.1",
+ "@types/numeral": "^2.0.3",
+ "@types/ramda": "^0.29.6",
+ "@types/react": "^18.2.28",
+ "@types/react-beautiful-dnd": "^13.1.6",
+ "@types/react-dom": "^18.2.13",
+ "@types/react-router-dom": "^5.3.3",
+ "@types/styled-components": "^5.1.28",
+ "@typescript-eslint/eslint-plugin": "^5.62.0",
+ "@typescript-eslint/parser": "^5.62.0",
+ "autoprefixer": "^10.4.16",
+ "eslint": "^8.51.0",
+ "eslint-config-airbnb": "^19.0.4",
+ "eslint-config-airbnb-typescript": "^17.1.0",
+ "eslint-config-prettier": "^8.10.0",
+ "eslint-import-resolver-alias": "^1.1.2",
+ "eslint-plugin-import": "^2.28.1",
+ "eslint-plugin-jsx-a11y": "^6.7.1",
+ "eslint-plugin-prettier": "^4.2.1",
+ "eslint-plugin-react": "^7.33.2",
+ "eslint-plugin-react-hooks": "^4.6.0",
+ "eslint-plugin-unused-imports": "^2.0.0",
+ "husky": "^8.0.3",
+ "lint-staged": "^13.3.0",
+ "postcss": "^8.4.31",
+ "postcss-import": "^15.1.0",
+ "postcss-nesting": "^11.3.0",
+ "prettier": "^2.8.8",
+ "prettier-plugin-tailwindcss": "^0.3.0",
+ "react-dev-inspector": "^2.0.0",
+ "rollup-plugin-visualizer": "^5.9.2",
+ "sass": "^1.69.3",
+ "stylelint": "^15.10.3",
+ "stylelint-config-rational-order": "^0.1.2",
+ "stylelint-config-standard": "^33.0.0",
+ "stylelint-declaration-block-no-ignored-properties": "^2.7.0",
+ "stylelint-order": "^6.0.3",
+ "tailwindcss": "^3.3.3",
+ "terser": "^5.26.0",
+ "ts-node": "^10.9.1",
+ "typescript": "^5.2.2",
+ "vite-plugin-svg-icons": "^2.0.1",
+ "vite-tsconfig-paths": "^4.2.1"
+ }
+}
diff --git a/frontend/pnpm-lock.yaml b/frontend/pnpm-lock.yaml
new file mode 100644
index 0000000..cf3623e
--- /dev/null
+++ b/frontend/pnpm-lock.yaml
@@ -0,0 +1,10849 @@
+lockfileVersion: '6.0'
+
+settings:
+ autoInstallPeers: true
+ excludeLinksFromLockfile: false
+
+dependencies:
+ '@ant-design/cssinjs':
+ specifier: ^1.17.2
+ version: 1.20.0(react-dom@18.3.0)(react@18.3.0)
+ '@ant-design/icons':
+ specifier: ^5.2.6
+ version: 5.3.6(react-dom@18.3.0)(react@18.3.0)
+ '@fullcalendar/common':
+ specifier: ^5.11.5
+ version: 5.11.5
+ '@fullcalendar/core':
+ specifier: ^6.1.9
+ version: 6.1.11
+ '@fullcalendar/daygrid':
+ specifier: ^6.1.9
+ version: 6.1.11(@fullcalendar/core@6.1.11)
+ '@fullcalendar/interaction':
+ specifier: ^6.1.9
+ version: 6.1.11(@fullcalendar/core@6.1.11)
+ '@fullcalendar/list':
+ specifier: ^6.1.9
+ version: 6.1.11(@fullcalendar/core@6.1.11)
+ '@fullcalendar/react':
+ specifier: ^6.1.9
+ version: 6.1.11(@fullcalendar/core@6.1.11)(react-dom@18.3.0)(react@18.3.0)
+ '@fullcalendar/timegrid':
+ specifier: ^6.1.9
+ version: 6.1.11(@fullcalendar/core@6.1.11)
+ '@fullcalendar/timeline':
+ specifier: ^6.1.9
+ version: 6.1.11(@fullcalendar/core@6.1.11)
+ '@hcaptcha/react-hcaptcha':
+ specifier: ^1.9.3
+ version: 1.10.1(react-dom@18.3.0)(react@18.3.0)
+ '@iconify/react':
+ specifier: ^4.1.1
+ version: 4.1.1(react@18.3.0)
+ '@tanstack/react-query':
+ specifier: ^4.36.1
+ version: 4.36.1(react-dom@18.3.0)(react@18.3.0)
+ '@tanstack/react-query-devtools':
+ specifier: ^4.36.1
+ version: 4.36.1(@tanstack/react-query@4.36.1)(react-dom@18.3.0)(react@18.3.0)
+ '@vitejs/plugin-react':
+ specifier: ^4.1.0
+ version: 4.2.1(vite@4.5.3)
+ antd:
+ specifier: ^5.9.3
+ version: 5.16.4(react-dom@18.3.0)(react@18.3.0)
+ apexcharts:
+ specifier: ^3.43.0
+ version: 3.49.0
+ autosuggest-highlight:
+ specifier: ^3.3.4
+ version: 3.3.4
+ axios:
+ specifier: ^1.5.1
+ version: 1.6.8
+ classnames:
+ specifier: ^2.3.2
+ version: 2.5.1
+ color:
+ specifier: ^4.2.3
+ version: 4.2.3
+ dayjs:
+ specifier: ^1.11.10
+ version: 1.11.10
+ framer-motion:
+ specifier: ^10.16.4
+ version: 10.18.0(react-dom@18.3.0)(react@18.3.0)
+ highlight.js:
+ specifier: ^11.9.0
+ version: 11.9.0
+ humps:
+ specifier: ^2.0.1
+ version: 2.0.1
+ i18next:
+ specifier: ^23.5.1
+ version: 23.11.2
+ i18next-browser-languagedetector:
+ specifier: ^7.1.0
+ version: 7.2.1
+ nprogress:
+ specifier: ^0.2.0
+ version: 0.2.0
+ numeral:
+ specifier: ^2.0.6
+ version: 2.0.6
+ ramda:
+ specifier: ^0.29.1
+ version: 0.29.1
+ react:
+ specifier: ^18.2.0
+ version: 18.3.0
+ react-apexcharts:
+ specifier: ^1.4.1
+ version: 1.4.1(apexcharts@3.49.0)(react@18.3.0)
+ react-beautiful-dnd:
+ specifier: ^13.1.1
+ version: 13.1.1(react-dom@18.3.0)(react@18.3.0)
+ react-dom:
+ specifier: ^18.2.0
+ version: 18.3.0(react@18.3.0)
+ react-helmet-async:
+ specifier: ^1.3.0
+ version: 1.3.0(react-dom@18.3.0)(react@18.3.0)
+ react-i18next:
+ specifier: ^13.2.2
+ version: 13.5.0(i18next@23.11.2)(react-dom@18.3.0)(react@18.3.0)
+ react-icons:
+ specifier: ^4.11.0
+ version: 4.12.0(react@18.3.0)
+ react-markdown:
+ specifier: ^8.0.7
+ version: 8.0.7(@types/react@18.3.0)(react@18.3.0)
+ react-organizational-chart:
+ specifier: ^2.2.1
+ version: 2.2.1(react-dom@18.3.0)(react@18.3.0)
+ react-quill:
+ specifier: ^2.0.0
+ version: 2.0.0(react-dom@18.3.0)(react@18.3.0)
+ react-router-dom:
+ specifier: ^6.16.0
+ version: 6.23.0(react-dom@18.3.0)(react@18.3.0)
+ react-use:
+ specifier: ^17.4.0
+ version: 17.5.0(react-dom@18.3.0)(react@18.3.0)
+ rehype-highlight:
+ specifier: ^6.0.0
+ version: 6.0.0
+ rehype-raw:
+ specifier: ^6.1.1
+ version: 6.1.1
+ remark-gfm:
+ specifier: ^3.0.1
+ version: 3.0.1
+ reset-css:
+ specifier: ^5.0.2
+ version: 5.0.2
+ screenfull:
+ specifier: ^6.0.2
+ version: 6.0.2
+ simplebar-react:
+ specifier: ^3.2.4
+ version: 3.2.4(react@18.3.0)
+ styled-components:
+ specifier: ^6.0.9
+ version: 6.1.8(react-dom@18.3.0)(react@18.3.0)
+ vite:
+ specifier: ^4.4.11
+ version: 4.5.3(@types/node@20.12.7)(sass@1.75.0)(terser@5.30.4)
+ zustand:
+ specifier: ^4.4.3
+ version: 4.5.2(@types/react@18.3.0)(react@18.3.0)
+
+devDependencies:
+ '@commitlint/cli':
+ specifier: ^17.7.2
+ version: 17.8.1
+ '@commitlint/config-conventional':
+ specifier: ^17.7.0
+ version: 17.8.1
+ '@react-dev-inspector/vite-plugin':
+ specifier: ^2.0.0
+ version: 2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0)
+ '@types/autosuggest-highlight':
+ specifier: ^3.2.0
+ version: 3.2.3
+ '@types/color':
+ specifier: ^3.0.4
+ version: 3.0.6
+ '@types/humps':
+ specifier: ^2.0.6
+ version: 2.0.6
+ '@types/nprogress':
+ specifier: ^0.2.1
+ version: 0.2.3
+ '@types/numeral':
+ specifier: ^2.0.3
+ version: 2.0.5
+ '@types/ramda':
+ specifier: ^0.29.6
+ version: 0.29.12
+ '@types/react':
+ specifier: ^18.2.28
+ version: 18.3.0
+ '@types/react-beautiful-dnd':
+ specifier: ^13.1.6
+ version: 13.1.8
+ '@types/react-dom':
+ specifier: ^18.2.13
+ version: 18.3.0
+ '@types/react-router-dom':
+ specifier: ^5.3.3
+ version: 5.3.3
+ '@types/styled-components':
+ specifier: ^5.1.28
+ version: 5.1.34
+ '@typescript-eslint/eslint-plugin':
+ specifier: ^5.62.0
+ version: 5.62.0(@typescript-eslint/parser@5.62.0)(eslint@8.57.0)(typescript@5.4.5)
+ '@typescript-eslint/parser':
+ specifier: ^5.62.0
+ version: 5.62.0(eslint@8.57.0)(typescript@5.4.5)
+ autoprefixer:
+ specifier: ^10.4.16
+ version: 10.4.19(postcss@8.4.38)
+ eslint:
+ specifier: ^8.51.0
+ version: 8.57.0
+ eslint-config-airbnb:
+ specifier: ^19.0.4
+ version: 19.0.4(eslint-plugin-import@2.29.1)(eslint-plugin-jsx-a11y@6.8.0)(eslint-plugin-react-hooks@4.6.1)(eslint-plugin-react@7.34.1)(eslint@8.57.0)
+ eslint-config-airbnb-typescript:
+ specifier: ^17.1.0
+ version: 17.1.0(@typescript-eslint/eslint-plugin@5.62.0)(@typescript-eslint/parser@5.62.0)(eslint-plugin-import@2.29.1)(eslint@8.57.0)
+ eslint-config-prettier:
+ specifier: ^8.10.0
+ version: 8.10.0(eslint@8.57.0)
+ eslint-import-resolver-alias:
+ specifier: ^1.1.2
+ version: 1.1.2(eslint-plugin-import@2.29.1)
+ eslint-plugin-import:
+ specifier: ^2.28.1
+ version: 2.29.1(@typescript-eslint/parser@5.62.0)(eslint@8.57.0)
+ eslint-plugin-jsx-a11y:
+ specifier: ^6.7.1
+ version: 6.8.0(eslint@8.57.0)
+ eslint-plugin-prettier:
+ specifier: ^4.2.1
+ version: 4.2.1(eslint-config-prettier@8.10.0)(eslint@8.57.0)(prettier@2.8.8)
+ eslint-plugin-react:
+ specifier: ^7.33.2
+ version: 7.34.1(eslint@8.57.0)
+ eslint-plugin-react-hooks:
+ specifier: ^4.6.0
+ version: 4.6.1(eslint@8.57.0)
+ eslint-plugin-unused-imports:
+ specifier: ^2.0.0
+ version: 2.0.0(@typescript-eslint/eslint-plugin@5.62.0)(eslint@8.57.0)
+ husky:
+ specifier: ^8.0.3
+ version: 8.0.3
+ lint-staged:
+ specifier: ^13.3.0
+ version: 13.3.0
+ postcss:
+ specifier: ^8.4.31
+ version: 8.4.38
+ postcss-import:
+ specifier: ^15.1.0
+ version: 15.1.0(postcss@8.4.38)
+ postcss-nesting:
+ specifier: ^11.3.0
+ version: 11.3.0(postcss@8.4.38)
+ prettier:
+ specifier: ^2.8.8
+ version: 2.8.8
+ prettier-plugin-tailwindcss:
+ specifier: ^0.3.0
+ version: 0.3.0(prettier@2.8.8)
+ react-dev-inspector:
+ specifier: ^2.0.0
+ version: 2.0.1(eslint@8.57.0)(react@18.3.0)(typescript@5.4.5)(webpack@5.91.0)
+ rollup-plugin-visualizer:
+ specifier: ^5.9.2
+ version: 5.12.0
+ sass:
+ specifier: ^1.69.3
+ version: 1.75.0
+ stylelint:
+ specifier: ^15.10.3
+ version: 15.11.0(typescript@5.4.5)
+ stylelint-config-rational-order:
+ specifier: ^0.1.2
+ version: 0.1.2
+ stylelint-config-standard:
+ specifier: ^33.0.0
+ version: 33.0.0(stylelint@15.11.0)
+ stylelint-declaration-block-no-ignored-properties:
+ specifier: ^2.7.0
+ version: 2.8.0(stylelint@15.11.0)
+ stylelint-order:
+ specifier: ^6.0.3
+ version: 6.0.4(stylelint@15.11.0)
+ tailwindcss:
+ specifier: ^3.3.3
+ version: 3.4.3(ts-node@10.9.2)
+ terser:
+ specifier: ^5.26.0
+ version: 5.30.4
+ ts-node:
+ specifier: ^10.9.1
+ version: 10.9.2(@types/node@20.12.7)(typescript@5.4.5)
+ typescript:
+ specifier: ^5.2.2
+ version: 5.4.5
+ vite-plugin-svg-icons:
+ specifier: ^2.0.1
+ version: 2.0.1(vite@4.5.3)
+ vite-tsconfig-paths:
+ specifier: ^4.2.1
+ version: 4.3.2(typescript@5.4.5)(vite@4.5.3)
+
+packages:
+
+ /@aashutoshrathi/word-wrap@1.2.6:
+ resolution: {integrity: sha512-1Yjs2SvM8TflER/OD3cOjhWWOZb58A2t7wpE2S9XfBYTiIl+XFhQG2bjy4Pu1I+EAlCNUzRDYDdFwFYUKvXcIA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /@alloc/quick-lru@5.2.0:
+ resolution: {integrity: sha512-UrcABB+4bUrFABwbluTIBErXwvbsU/V7TZWfmbgJfbkwiBuziS9gxdODUyuiecfdGQ85jglMW6juS3+z5TsKLw==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /@ampproject/remapping@2.3.0:
+ resolution: {integrity: sha512-30iZtAPgz+LTIYoeivqYo853f02jBYSd5uGnGpkFV0M3xOt9aN73erkgYAmZU43x4VfqcnLxW9Kpg3R5LC4YYw==}
+ engines: {node: '>=6.0.0'}
+ dependencies:
+ '@jridgewell/gen-mapping': 0.3.5
+ '@jridgewell/trace-mapping': 0.3.25
+
+ /@ant-design/colors@7.0.2:
+ resolution: {integrity: sha512-7KJkhTiPiLHSu+LmMJnehfJ6242OCxSlR3xHVBecYxnMW8MS/878NXct1GqYARyL59fyeFdKRxXTfvR9SnDgJg==}
+ dependencies:
+ '@ctrl/tinycolor': 3.6.1
+ dev: false
+
+ /@ant-design/cssinjs@1.20.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-uG3iWzJxgNkADdZmc6W0Ci3iQAUOvLMcM8SnnmWq3r6JeocACft4ChnY/YWvI2Y+rG/68QBla/O+udke1yH3vg==}
+ peerDependencies:
+ react: '>=16.0.0'
+ react-dom: '>=16.0.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@emotion/hash': 0.8.0
+ '@emotion/unitless': 0.7.5
+ classnames: 2.5.1
+ csstype: 3.1.3
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ stylis: 4.3.2
+ dev: false
+
+ /@ant-design/icons-svg@4.4.2:
+ resolution: {integrity: sha512-vHbT+zJEVzllwP+CM+ul7reTEfBR0vgxFe7+lREAsAA7YGsYpboiq2sQNeQeRvh09GfQgs/GyFEvZpJ9cLXpXA==}
+ dev: false
+
+ /@ant-design/icons@5.3.6(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-JeWsgNjvkTTC73YDPgWOgdScRku/iHN9JU0qk39OSEmJSCiRghQMLlxGTCY5ovbRRoXjxHXnUKgQEgBDnQfKmA==}
+ engines: {node: '>=8'}
+ peerDependencies:
+ react: '>=16.0.0'
+ react-dom: '>=16.0.0'
+ dependencies:
+ '@ant-design/colors': 7.0.2
+ '@ant-design/icons-svg': 4.4.2
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /@ant-design/react-slick@1.1.2(react@18.3.0):
+ resolution: {integrity: sha512-EzlvzE6xQUBrZuuhSAFTdsr4P2bBBHGZwKFemEfq8gIGyIQCxalYfZW/T2ORbtQx5rU69o+WycP3exY/7T1hGA==}
+ peerDependencies:
+ react: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ json2mq: 0.2.0
+ react: 18.3.0
+ resize-observer-polyfill: 1.5.1
+ throttle-debounce: 5.0.0
+ dev: false
+
+ /@babel/code-frame@7.24.2:
+ resolution: {integrity: sha512-y5+tLQyV8pg3fsiln67BVLD1P13Eg4lh5RW9mF0zUuvLrv9uIQ4MCL+CRT+FTsBlBjcIan6PGsLcBN0m3ClUyQ==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/highlight': 7.24.2
+ picocolors: 1.0.0
+
+ /@babel/compat-data@7.24.4:
+ resolution: {integrity: sha512-vg8Gih2MLK+kOkHJp4gBEIkyaIi00jgWot2D9QOmmfLC8jINSOzmCLta6Bvz/JSBCqnegV0L80jhxkol5GWNfQ==}
+ engines: {node: '>=6.9.0'}
+
+ /@babel/core@7.24.4:
+ resolution: {integrity: sha512-MBVlMXP+kkl5394RBLSxxk/iLTeVGuXTV3cIDXavPpMMqnSnt6apKgan/U8O3USWZCWZT/TbgfEpKa4uMgN4Dg==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@ampproject/remapping': 2.3.0
+ '@babel/code-frame': 7.24.2
+ '@babel/generator': 7.24.4
+ '@babel/helper-compilation-targets': 7.23.6
+ '@babel/helper-module-transforms': 7.23.3(@babel/core@7.24.4)
+ '@babel/helpers': 7.24.4
+ '@babel/parser': 7.24.4
+ '@babel/template': 7.24.0
+ '@babel/traverse': 7.24.1
+ '@babel/types': 7.24.0
+ convert-source-map: 2.0.0
+ debug: 4.3.4
+ gensync: 1.0.0-beta.2
+ json5: 2.2.3
+ semver: 6.3.1
+ transitivePeerDependencies:
+ - supports-color
+
+ /@babel/generator@7.24.4:
+ resolution: {integrity: sha512-Xd6+v6SnjWVx/nus+y0l1sxMOTOMBkyL4+BIdbALyatQnAe/SRVjANeDPSCYaX+i1iJmuGSKf3Z+E+V/va1Hvw==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/types': 7.24.0
+ '@jridgewell/gen-mapping': 0.3.5
+ '@jridgewell/trace-mapping': 0.3.25
+ jsesc: 2.5.2
+
+ /@babel/helper-compilation-targets@7.23.6:
+ resolution: {integrity: sha512-9JB548GZoQVmzrFgp8o7KxdgkTGm6xs9DW0o/Pim72UDjzr5ObUQ6ZzYPqA+g9OTS2bBQoctLJrky0RDCAWRgQ==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/compat-data': 7.24.4
+ '@babel/helper-validator-option': 7.23.5
+ browserslist: 4.23.0
+ lru-cache: 5.1.1
+ semver: 6.3.1
+
+ /@babel/helper-environment-visitor@7.22.20:
+ resolution: {integrity: sha512-zfedSIzFhat/gFhWfHtgWvlec0nqB9YEIVrpuwjruLlXfUSnA8cJB0miHKwqDnQ7d32aKo2xt88/xZptwxbfhA==}
+ engines: {node: '>=6.9.0'}
+
+ /@babel/helper-function-name@7.23.0:
+ resolution: {integrity: sha512-OErEqsrxjZTJciZ4Oo+eoZqeW9UIiOcuYKRJA4ZAgV9myA+pOXhhmpfNCKjEH/auVfEYVFJ6y1Tc4r0eIApqiw==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/template': 7.24.0
+ '@babel/types': 7.24.0
+
+ /@babel/helper-hoist-variables@7.22.5:
+ resolution: {integrity: sha512-wGjk9QZVzvknA6yKIUURb8zY3grXCcOZt+/7Wcy8O2uctxhplmUPkOdlgoNhmdVee2c92JXbf1xpMtVNbfoxRw==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/types': 7.24.0
+
+ /@babel/helper-module-imports@7.24.3:
+ resolution: {integrity: sha512-viKb0F9f2s0BCS22QSF308z/+1YWKV/76mwt61NBzS5izMzDPwdq1pTrzf+Li3npBWX9KdQbkeCt1jSAM7lZqg==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/types': 7.24.0
+
+ /@babel/helper-module-transforms@7.23.3(@babel/core@7.24.4):
+ resolution: {integrity: sha512-7bBs4ED9OmswdfDzpz4MpWgSrV7FXlc3zIagvLFjS5H+Mk7Snr21vQ6QwrsoCGMfNC4e4LQPdoULEt4ykz0SRQ==}
+ engines: {node: '>=6.9.0'}
+ peerDependencies:
+ '@babel/core': ^7.0.0
+ dependencies:
+ '@babel/core': 7.24.4
+ '@babel/helper-environment-visitor': 7.22.20
+ '@babel/helper-module-imports': 7.24.3
+ '@babel/helper-simple-access': 7.22.5
+ '@babel/helper-split-export-declaration': 7.22.6
+ '@babel/helper-validator-identifier': 7.22.20
+
+ /@babel/helper-plugin-utils@7.24.0:
+ resolution: {integrity: sha512-9cUznXMG0+FxRuJfvL82QlTqIzhVW9sL0KjMPHhAOOvpQGL8QtdxnBKILjBqxlHyliz0yCa1G903ZXI/FuHy2w==}
+ engines: {node: '>=6.9.0'}
+ dev: false
+
+ /@babel/helper-simple-access@7.22.5:
+ resolution: {integrity: sha512-n0H99E/K+Bika3++WNL17POvo4rKWZ7lZEp1Q+fStVbUi8nxPQEBOlTmCOxW/0JsS56SKKQ+ojAe2pHKJHN35w==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/types': 7.24.0
+
+ /@babel/helper-split-export-declaration@7.22.6:
+ resolution: {integrity: sha512-AsUnxuLhRYsisFiaJwvp1QF+I3KjD5FOxut14q/GzovUe6orHLesW2C7d754kRm53h5gqrz6sFl6sxc4BVtE/g==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/types': 7.24.0
+
+ /@babel/helper-string-parser@7.24.1:
+ resolution: {integrity: sha512-2ofRCjnnA9y+wk8b9IAREroeUP02KHp431N2mhKniy2yKIDKpbrHv9eXwm8cBeWQYcJmzv5qKCu65P47eCF7CQ==}
+ engines: {node: '>=6.9.0'}
+
+ /@babel/helper-validator-identifier@7.22.20:
+ resolution: {integrity: sha512-Y4OZ+ytlatR8AI+8KZfKuL5urKp7qey08ha31L8b3BwewJAoJamTzyvxPR/5D+KkdJCGPq/+8TukHBlY10FX9A==}
+ engines: {node: '>=6.9.0'}
+
+ /@babel/helper-validator-option@7.23.5:
+ resolution: {integrity: sha512-85ttAOMLsr53VgXkTbkx8oA6YTfT4q7/HzXSLEYmjcSTJPMPQtvq1BD79Byep5xMUYbGRzEpDsjUf3dyp54IKw==}
+ engines: {node: '>=6.9.0'}
+
+ /@babel/helpers@7.24.4:
+ resolution: {integrity: sha512-FewdlZbSiwaVGlgT1DPANDuCHaDMiOo+D/IDYRFYjHOuv66xMSJ7fQwwODwRNAPkADIO/z1EoF/l2BCWlWABDw==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/template': 7.24.0
+ '@babel/traverse': 7.24.1
+ '@babel/types': 7.24.0
+ transitivePeerDependencies:
+ - supports-color
+
+ /@babel/highlight@7.24.2:
+ resolution: {integrity: sha512-Yac1ao4flkTxTteCDZLEvdxg2fZfz1v8M4QpaGypq/WPDqg3ijHYbDfs+LG5hvzSoqaSZ9/Z9lKSP3CjZjv+pA==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/helper-validator-identifier': 7.22.20
+ chalk: 2.4.2
+ js-tokens: 4.0.0
+ picocolors: 1.0.0
+
+ /@babel/parser@7.24.4:
+ resolution: {integrity: sha512-zTvEBcghmeBma9QIGunWevvBAp4/Qu9Bdq+2k0Ot4fVMD6v3dsC9WOcRSKk7tRRyBM/53yKMJko9xOatGQAwSg==}
+ engines: {node: '>=6.0.0'}
+ hasBin: true
+ dependencies:
+ '@babel/types': 7.20.5
+
+ /@babel/plugin-transform-react-jsx-self@7.24.1(@babel/core@7.24.4):
+ resolution: {integrity: sha512-kDJgnPujTmAZ/9q2CN4m2/lRsUUPDvsG3+tSHWUJIzMGTt5U/b/fwWd3RO3n+5mjLrsBrVa5eKFRVSQbi3dF1w==}
+ engines: {node: '>=6.9.0'}
+ peerDependencies:
+ '@babel/core': ^7.0.0-0
+ dependencies:
+ '@babel/core': 7.24.4
+ '@babel/helper-plugin-utils': 7.24.0
+ dev: false
+
+ /@babel/plugin-transform-react-jsx-source@7.24.1(@babel/core@7.24.4):
+ resolution: {integrity: sha512-1v202n7aUq4uXAieRTKcwPzNyphlCuqHHDcdSNc+vdhoTEZcFMh+L5yZuCmGaIO7bs1nJUNfHB89TZyoL48xNA==}
+ engines: {node: '>=6.9.0'}
+ peerDependencies:
+ '@babel/core': ^7.0.0-0
+ dependencies:
+ '@babel/core': 7.24.4
+ '@babel/helper-plugin-utils': 7.24.0
+ dev: false
+
+ /@babel/runtime@7.24.4:
+ resolution: {integrity: sha512-dkxf7+hn8mFBwKjs9bvBlArzLVxVbS8usaPUDd5p2a9JCL9tB8OaOVN1isD4+Xyk4ns89/xeOmbQvgdK7IIVdA==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ regenerator-runtime: 0.14.1
+
+ /@babel/template@7.24.0:
+ resolution: {integrity: sha512-Bkf2q8lMB0AFpX0NFEqSbx1OkTHf0f+0j82mkw+ZpzBnkk7e9Ql0891vlfgi+kHwOk8tQjiQHpqh4LaSa0fKEA==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/code-frame': 7.24.2
+ '@babel/parser': 7.24.4
+ '@babel/types': 7.24.0
+
+ /@babel/traverse@7.24.1:
+ resolution: {integrity: sha512-xuU6o9m68KeqZbQuDt2TcKSxUw/mrsvavlEqQ1leZ/B+C9tk6E4sRWy97WaXgvq5E+nU3cXMxv3WKOCanVMCmQ==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/code-frame': 7.24.2
+ '@babel/generator': 7.24.4
+ '@babel/helper-environment-visitor': 7.22.20
+ '@babel/helper-function-name': 7.23.0
+ '@babel/helper-hoist-variables': 7.22.5
+ '@babel/helper-split-export-declaration': 7.22.6
+ '@babel/parser': 7.24.4
+ '@babel/types': 7.24.0
+ debug: 4.3.4
+ globals: 11.12.0
+ transitivePeerDependencies:
+ - supports-color
+
+ /@babel/types@7.20.5:
+ resolution: {integrity: sha512-c9fst/h2/dcF7H+MJKZ2T0KjEQ8hY/BNnDk/H3XY8C4Aw/eWQXWn/lWntHF9ooUBnGmEvbfGrTgLWc+um0YDUg==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/helper-string-parser': 7.24.1
+ '@babel/helper-validator-identifier': 7.22.20
+ to-fast-properties: 2.0.0
+
+ /@babel/types@7.24.0:
+ resolution: {integrity: sha512-+j7a5c253RfKh8iABBhywc8NSfP5LURe7Uh4qpsh6jc+aLJguvmIUBdjSdEMQv2bENrCR5MfRdjGo7vzS/ob7w==}
+ engines: {node: '>=6.9.0'}
+ dependencies:
+ '@babel/helper-string-parser': 7.24.1
+ '@babel/helper-validator-identifier': 7.22.20
+ to-fast-properties: 2.0.0
+
+ /@commitlint/cli@17.8.1:
+ resolution: {integrity: sha512-ay+WbzQesE0Rv4EQKfNbSMiJJ12KdKTDzIt0tcK4k11FdsWmtwP0Kp1NWMOUswfIWo6Eb7p7Ln721Nx9FLNBjg==}
+ engines: {node: '>=v14'}
+ hasBin: true
+ dependencies:
+ '@commitlint/format': 17.8.1
+ '@commitlint/lint': 17.8.1
+ '@commitlint/load': 17.8.1
+ '@commitlint/read': 17.8.1
+ '@commitlint/types': 17.8.1
+ execa: 5.1.1
+ lodash.isfunction: 3.0.9
+ resolve-from: 5.0.0
+ resolve-global: 1.0.0
+ yargs: 17.7.2
+ transitivePeerDependencies:
+ - '@swc/core'
+ - '@swc/wasm'
+ dev: true
+
+ /@commitlint/config-conventional@17.8.1:
+ resolution: {integrity: sha512-NxCOHx1kgneig3VLauWJcDWS40DVjg7nKOpBEEK9E5fjJpQqLCilcnKkIIjdBH98kEO1q3NpE5NSrZ2kl/QGJg==}
+ engines: {node: '>=v14'}
+ dependencies:
+ conventional-changelog-conventionalcommits: 6.1.0
+ dev: true
+
+ /@commitlint/config-validator@17.8.1:
+ resolution: {integrity: sha512-UUgUC+sNiiMwkyiuIFR7JG2cfd9t/7MV8VB4TZ+q02ZFkHoduUS4tJGsCBWvBOGD9Btev6IecPMvlWUfJorkEA==}
+ engines: {node: '>=v14'}
+ dependencies:
+ '@commitlint/types': 17.8.1
+ ajv: 8.12.0
+ dev: true
+
+ /@commitlint/ensure@17.8.1:
+ resolution: {integrity: sha512-xjafwKxid8s1K23NFpL8JNo6JnY/ysetKo8kegVM7c8vs+kWLP8VrQq+NbhgVlmCojhEDbzQKp4eRXSjVOGsow==}
+ engines: {node: '>=v14'}
+ dependencies:
+ '@commitlint/types': 17.8.1
+ lodash.camelcase: 4.3.0
+ lodash.kebabcase: 4.1.1
+ lodash.snakecase: 4.1.1
+ lodash.startcase: 4.4.0
+ lodash.upperfirst: 4.3.1
+ dev: true
+
+ /@commitlint/execute-rule@17.8.1:
+ resolution: {integrity: sha512-JHVupQeSdNI6xzA9SqMF+p/JjrHTcrJdI02PwesQIDCIGUrv04hicJgCcws5nzaoZbROapPs0s6zeVHoxpMwFQ==}
+ engines: {node: '>=v14'}
+ dev: true
+
+ /@commitlint/format@17.8.1:
+ resolution: {integrity: sha512-f3oMTyZ84M9ht7fb93wbCKmWxO5/kKSbwuYvS867duVomoOsgrgljkGGIztmT/srZnaiGbaK8+Wf8Ik2tSr5eg==}
+ engines: {node: '>=v14'}
+ dependencies:
+ '@commitlint/types': 17.8.1
+ chalk: 4.1.2
+ dev: true
+
+ /@commitlint/is-ignored@17.8.1:
+ resolution: {integrity: sha512-UshMi4Ltb4ZlNn4F7WtSEugFDZmctzFpmbqvpyxD3la510J+PLcnyhf9chs7EryaRFJMdAKwsEKfNK0jL/QM4g==}
+ engines: {node: '>=v14'}
+ dependencies:
+ '@commitlint/types': 17.8.1
+ semver: 7.5.4
+ dev: true
+
+ /@commitlint/lint@17.8.1:
+ resolution: {integrity: sha512-aQUlwIR1/VMv2D4GXSk7PfL5hIaFSfy6hSHV94O8Y27T5q+DlDEgd/cZ4KmVI+MWKzFfCTiTuWqjfRSfdRllCA==}
+ engines: {node: '>=v14'}
+ dependencies:
+ '@commitlint/is-ignored': 17.8.1
+ '@commitlint/parse': 17.8.1
+ '@commitlint/rules': 17.8.1
+ '@commitlint/types': 17.8.1
+ dev: true
+
+ /@commitlint/load@17.8.1:
+ resolution: {integrity: sha512-iF4CL7KDFstP1kpVUkT8K2Wl17h2yx9VaR1ztTc8vzByWWcbO/WaKwxsnCOqow9tVAlzPfo1ywk9m2oJ9ucMqA==}
+ engines: {node: '>=v14'}
+ dependencies:
+ '@commitlint/config-validator': 17.8.1
+ '@commitlint/execute-rule': 17.8.1
+ '@commitlint/resolve-extends': 17.8.1
+ '@commitlint/types': 17.8.1
+ '@types/node': 20.5.1
+ chalk: 4.1.2
+ cosmiconfig: 8.3.6(typescript@5.4.5)
+ cosmiconfig-typescript-loader: 4.4.0(@types/node@20.5.1)(cosmiconfig@8.3.6)(ts-node@10.9.2)(typescript@5.4.5)
+ lodash.isplainobject: 4.0.6
+ lodash.merge: 4.6.2
+ lodash.uniq: 4.5.0
+ resolve-from: 5.0.0
+ ts-node: 10.9.2(@types/node@20.5.1)(typescript@5.4.5)
+ typescript: 5.4.5
+ transitivePeerDependencies:
+ - '@swc/core'
+ - '@swc/wasm'
+ dev: true
+
+ /@commitlint/message@17.8.1:
+ resolution: {integrity: sha512-6bYL1GUQsD6bLhTH3QQty8pVFoETfFQlMn2Nzmz3AOLqRVfNNtXBaSY0dhZ0dM6A2MEq4+2d7L/2LP8TjqGRkA==}
+ engines: {node: '>=v14'}
+ dev: true
+
+ /@commitlint/parse@17.8.1:
+ resolution: {integrity: sha512-/wLUickTo0rNpQgWwLPavTm7WbwkZoBy3X8PpkUmlSmQJyWQTj0m6bDjiykMaDt41qcUbfeFfaCvXfiR4EGnfw==}
+ engines: {node: '>=v14'}
+ dependencies:
+ '@commitlint/types': 17.8.1
+ conventional-changelog-angular: 6.0.0
+ conventional-commits-parser: 4.0.0
+ dev: true
+
+ /@commitlint/read@17.8.1:
+ resolution: {integrity: sha512-Fd55Oaz9irzBESPCdMd8vWWgxsW3OWR99wOntBDHgf9h7Y6OOHjWEdS9Xzen1GFndqgyoaFplQS5y7KZe0kO2w==}
+ engines: {node: '>=v14'}
+ dependencies:
+ '@commitlint/top-level': 17.8.1
+ '@commitlint/types': 17.8.1
+ fs-extra: 11.2.0
+ git-raw-commits: 2.0.11
+ minimist: 1.2.8
+ dev: true
+
+ /@commitlint/resolve-extends@17.8.1:
+ resolution: {integrity: sha512-W/ryRoQ0TSVXqJrx5SGkaYuAaE/BUontL1j1HsKckvM6e5ZaG0M9126zcwL6peKSuIetJi7E87PRQF8O86EW0Q==}
+ engines: {node: '>=v14'}
+ dependencies:
+ '@commitlint/config-validator': 17.8.1
+ '@commitlint/types': 17.8.1
+ import-fresh: 3.3.0
+ lodash.mergewith: 4.6.2
+ resolve-from: 5.0.0
+ resolve-global: 1.0.0
+ dev: true
+
+ /@commitlint/rules@17.8.1:
+ resolution: {integrity: sha512-2b7OdVbN7MTAt9U0vKOYKCDsOvESVXxQmrvuVUZ0rGFMCrCPJWWP1GJ7f0lAypbDAhaGb8zqtdOr47192LBrIA==}
+ engines: {node: '>=v14'}
+ dependencies:
+ '@commitlint/ensure': 17.8.1
+ '@commitlint/message': 17.8.1
+ '@commitlint/to-lines': 17.8.1
+ '@commitlint/types': 17.8.1
+ execa: 5.1.1
+ dev: true
+
+ /@commitlint/to-lines@17.8.1:
+ resolution: {integrity: sha512-LE0jb8CuR/mj6xJyrIk8VLz03OEzXFgLdivBytoooKO5xLt5yalc8Ma5guTWobw998sbR3ogDd+2jed03CFmJA==}
+ engines: {node: '>=v14'}
+ dev: true
+
+ /@commitlint/top-level@17.8.1:
+ resolution: {integrity: sha512-l6+Z6rrNf5p333SHfEte6r+WkOxGlWK4bLuZKbtf/2TXRN+qhrvn1XE63VhD8Oe9oIHQ7F7W1nG2k/TJFhx2yA==}
+ engines: {node: '>=v14'}
+ dependencies:
+ find-up: 5.0.0
+ dev: true
+
+ /@commitlint/types@17.8.1:
+ resolution: {integrity: sha512-PXDQXkAmiMEG162Bqdh9ChML/GJZo6vU+7F03ALKDK8zYc6SuAr47LjG7hGYRqUOz+WK0dU7bQ0xzuqFMdxzeQ==}
+ engines: {node: '>=v14'}
+ dependencies:
+ chalk: 4.1.2
+ dev: true
+
+ /@cspotcode/source-map-support@0.8.1:
+ resolution: {integrity: sha512-IchNf6dN4tHoMFIn/7OE8LWZ19Y6q/67Bmf6vnGREv8RSbBVb9LPJxEcnwrcwX6ixSvaiGoomAUvu4YSxXrVgw==}
+ engines: {node: '>=12'}
+ dependencies:
+ '@jridgewell/trace-mapping': 0.3.9
+ dev: true
+
+ /@csstools/css-parser-algorithms@2.6.1(@csstools/css-tokenizer@2.2.4):
+ resolution: {integrity: sha512-ubEkAaTfVZa+WwGhs5jbo5Xfqpeaybr/RvWzvFxRs4jfq16wH8l8Ty/QEEpINxll4xhuGfdMbipRyz5QZh9+FA==}
+ engines: {node: ^14 || ^16 || >=18}
+ peerDependencies:
+ '@csstools/css-tokenizer': ^2.2.4
+ dependencies:
+ '@csstools/css-tokenizer': 2.2.4
+ dev: true
+
+ /@csstools/css-tokenizer@2.2.4:
+ resolution: {integrity: sha512-PuWRAewQLbDhGeTvFuq2oClaSCKPIBmHyIobCV39JHRYN0byDcUWJl5baPeNUcqrjtdMNqFooE0FGl31I3JOqw==}
+ engines: {node: ^14 || ^16 || >=18}
+ dev: true
+
+ /@csstools/media-query-list-parser@2.1.9(@csstools/css-parser-algorithms@2.6.1)(@csstools/css-tokenizer@2.2.4):
+ resolution: {integrity: sha512-qqGuFfbn4rUmyOB0u8CVISIp5FfJ5GAR3mBrZ9/TKndHakdnm6pY0L/fbLcpPnrzwCyyTEZl1nUcXAYHEWneTA==}
+ engines: {node: ^14 || ^16 || >=18}
+ peerDependencies:
+ '@csstools/css-parser-algorithms': ^2.6.1
+ '@csstools/css-tokenizer': ^2.2.4
+ dependencies:
+ '@csstools/css-parser-algorithms': 2.6.1(@csstools/css-tokenizer@2.2.4)
+ '@csstools/css-tokenizer': 2.2.4
+ dev: true
+
+ /@csstools/selector-specificity@2.2.0(postcss-selector-parser@6.0.16):
+ resolution: {integrity: sha512-+OJ9konv95ClSTOJCmMZqpd5+YGsB2S+x6w3E1oaM8UuR5j8nTNHYSz8c9BEPGDOCMQYIEEGlVPj/VY64iTbGw==}
+ engines: {node: ^14 || ^16 || >=18}
+ peerDependencies:
+ postcss-selector-parser: ^6.0.10
+ dependencies:
+ postcss-selector-parser: 6.0.16
+ dev: true
+
+ /@csstools/selector-specificity@3.0.3(postcss-selector-parser@6.0.16):
+ resolution: {integrity: sha512-KEPNw4+WW5AVEIyzC80rTbWEUatTW2lXpN8+8ILC8PiPeWPjwUzrPZDIOZ2wwqDmeqOYTdSGyL3+vE5GC3FB3Q==}
+ engines: {node: ^14 || ^16 || >=18}
+ peerDependencies:
+ postcss-selector-parser: ^6.0.13
+ dependencies:
+ postcss-selector-parser: 6.0.16
+ dev: true
+
+ /@ctrl/tinycolor@3.6.1:
+ resolution: {integrity: sha512-SITSV6aIXsuVNV3f3O0f2n/cgyEDWoSqtZMYiAmcsYHydcKrOz3gUxB/iXd/Qf08+IZX4KpgNbvUdMBmWz+kcA==}
+ engines: {node: '>=10'}
+ dev: false
+
+ /@emotion/babel-plugin@11.11.0:
+ resolution: {integrity: sha512-m4HEDZleaaCH+XgDDsPF15Ht6wTLsgDTeR3WYj9Q/k76JtWhrJjcP4+/XlG8LGT/Rol9qUfOIztXeA84ATpqPQ==}
+ dependencies:
+ '@babel/helper-module-imports': 7.24.3
+ '@babel/runtime': 7.24.4
+ '@emotion/hash': 0.9.1
+ '@emotion/memoize': 0.8.1
+ '@emotion/serialize': 1.1.4
+ babel-plugin-macros: 3.1.0
+ convert-source-map: 1.9.0
+ escape-string-regexp: 4.0.0
+ find-root: 1.1.0
+ source-map: 0.5.7
+ stylis: 4.2.0
+ dev: false
+
+ /@emotion/cache@11.11.0:
+ resolution: {integrity: sha512-P34z9ssTCBi3e9EI1ZsWpNHcfY1r09ZO0rZbRO2ob3ZQMnFI35jB536qoXbkdesr5EUhYi22anuEJuyxifaqAQ==}
+ dependencies:
+ '@emotion/memoize': 0.8.1
+ '@emotion/sheet': 1.2.2
+ '@emotion/utils': 1.2.1
+ '@emotion/weak-memoize': 0.3.1
+ stylis: 4.2.0
+ dev: false
+
+ /@emotion/css@11.11.2:
+ resolution: {integrity: sha512-VJxe1ucoMYMS7DkiMdC2T7PWNbrEI0a39YRiyDvK2qq4lXwjRbVP/z4lpG+odCsRzadlR+1ywwrTzhdm5HNdew==}
+ dependencies:
+ '@emotion/babel-plugin': 11.11.0
+ '@emotion/cache': 11.11.0
+ '@emotion/serialize': 1.1.4
+ '@emotion/sheet': 1.2.2
+ '@emotion/utils': 1.2.1
+ dev: false
+
+ /@emotion/hash@0.8.0:
+ resolution: {integrity: sha512-kBJtf7PH6aWwZ6fka3zQ0p6SBYzx4fl1LoZXE2RrnYST9Xljm7WfKJrU4g/Xr3Beg72MLrp1AWNUmuYJTL7Cow==}
+ dev: false
+
+ /@emotion/hash@0.9.1:
+ resolution: {integrity: sha512-gJB6HLm5rYwSLI6PQa+X1t5CFGrv1J1TWG+sOyMCeKz2ojaj6Fnl/rZEspogG+cvqbt4AE/2eIyD2QfLKTBNlQ==}
+ dev: false
+
+ /@emotion/is-prop-valid@0.8.8:
+ resolution: {integrity: sha512-u5WtneEAr5IDG2Wv65yhunPSMLIpuKsbuOktRojfrEiEvRyC85LgPMZI63cr7NUqT8ZIGdSVg8ZKGxIug4lXcA==}
+ requiresBuild: true
+ dependencies:
+ '@emotion/memoize': 0.7.4
+ dev: false
+ optional: true
+
+ /@emotion/is-prop-valid@1.2.1:
+ resolution: {integrity: sha512-61Mf7Ufx4aDxx1xlDeOm8aFFigGHE4z+0sKCa+IHCeZKiyP9RLD0Mmx7m8b9/Cf37f7NAvQOOJAbQQGVr5uERw==}
+ dependencies:
+ '@emotion/memoize': 0.8.1
+ dev: false
+
+ /@emotion/memoize@0.7.4:
+ resolution: {integrity: sha512-Ja/Vfqe3HpuzRsG1oBtWTHk2PGZ7GR+2Vz5iYGelAw8dx32K0y7PjVuxK6z1nMpZOqAFsRUPCkK1YjJ56qJlgw==}
+ requiresBuild: true
+ dev: false
+ optional: true
+
+ /@emotion/memoize@0.8.1:
+ resolution: {integrity: sha512-W2P2c/VRW1/1tLox0mVUalvnWXxavmv/Oum2aPsRcoDJuob75FC3Y8FbpfLwUegRcxINtGUMPq0tFCvYNTBXNA==}
+ dev: false
+
+ /@emotion/serialize@1.1.4:
+ resolution: {integrity: sha512-RIN04MBT8g+FnDwgvIUi8czvr1LU1alUMI05LekWB5DGyTm8cCBMCRpq3GqaiyEDRptEXOyXnvZ58GZYu4kBxQ==}
+ dependencies:
+ '@emotion/hash': 0.9.1
+ '@emotion/memoize': 0.8.1
+ '@emotion/unitless': 0.8.1
+ '@emotion/utils': 1.2.1
+ csstype: 3.1.3
+ dev: false
+
+ /@emotion/sheet@1.2.2:
+ resolution: {integrity: sha512-0QBtGvaqtWi+nx6doRwDdBIzhNdZrXUppvTM4dtZZWEGTXL/XE/yJxLMGlDT1Gt+UHH5IX1n+jkXyytE/av7OA==}
+ dev: false
+
+ /@emotion/unitless@0.7.5:
+ resolution: {integrity: sha512-OWORNpfjMsSSUBVrRBVGECkhWcULOAJz9ZW8uK9qgxD+87M7jHRcvh/A96XXNhXTLmKcoYSQtBEX7lHMO7YRwg==}
+ dev: false
+
+ /@emotion/unitless@0.8.0:
+ resolution: {integrity: sha512-VINS5vEYAscRl2ZUDiT3uMPlrFQupiKgHz5AA4bCH1miKBg4qtwkim1qPmJj/4WG6TreYMY111rEFsjupcOKHw==}
+ dev: false
+
+ /@emotion/unitless@0.8.1:
+ resolution: {integrity: sha512-KOEGMu6dmJZtpadb476IsZBclKvILjopjUii3V+7MnXIQCYh8W3NgNcgwo21n9LXZX6EDIKvqfjYxXebDwxKmQ==}
+ dev: false
+
+ /@emotion/utils@1.2.1:
+ resolution: {integrity: sha512-Y2tGf3I+XVnajdItskUCn6LX+VUDmP6lTL4fcqsXAv43dnlbZiuW4MWQW38rW/BVWSE7Q/7+XQocmpnRYILUmg==}
+ dev: false
+
+ /@emotion/weak-memoize@0.3.1:
+ resolution: {integrity: sha512-EsBwpc7hBUJWAsNPBmJy4hxWx12v6bshQsldrVmjxJoc3isbxhOrF2IcCpaXxfvq03NwkI7sbsOLXbYuqF/8Ww==}
+ dev: false
+
+ /@esbuild/android-arm64@0.18.20:
+ resolution: {integrity: sha512-Nz4rJcchGDtENV0eMKUNa6L12zz2zBDXuhj/Vjh18zGqB44Bi7MBMSXjgunJgjRhCmKOjnPuZp4Mb6OKqtMHLQ==}
+ engines: {node: '>=12'}
+ cpu: [arm64]
+ os: [android]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/android-arm@0.18.20:
+ resolution: {integrity: sha512-fyi7TDI/ijKKNZTUJAQqiG5T7YjJXgnzkURqmGj13C6dCqckZBLdl4h7bkhHt/t0WP+zO9/zwroDvANaOqO5Sw==}
+ engines: {node: '>=12'}
+ cpu: [arm]
+ os: [android]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/android-x64@0.18.20:
+ resolution: {integrity: sha512-8GDdlePJA8D6zlZYJV/jnrRAi6rOiNaCC/JclcXpB+KIuvfBN4owLtgzY2bsxnx666XjJx2kDPUmnTtR8qKQUg==}
+ engines: {node: '>=12'}
+ cpu: [x64]
+ os: [android]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/darwin-arm64@0.18.20:
+ resolution: {integrity: sha512-bxRHW5kHU38zS2lPTPOyuyTm+S+eobPUnTNkdJEfAddYgEcll4xkT8DB9d2008DtTbl7uJag2HuE5NZAZgnNEA==}
+ engines: {node: '>=12'}
+ cpu: [arm64]
+ os: [darwin]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/darwin-x64@0.18.20:
+ resolution: {integrity: sha512-pc5gxlMDxzm513qPGbCbDukOdsGtKhfxD1zJKXjCCcU7ju50O7MeAZ8c4krSJcOIJGFR+qx21yMMVYwiQvyTyQ==}
+ engines: {node: '>=12'}
+ cpu: [x64]
+ os: [darwin]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/freebsd-arm64@0.18.20:
+ resolution: {integrity: sha512-yqDQHy4QHevpMAaxhhIwYPMv1NECwOvIpGCZkECn8w2WFHXjEwrBn3CeNIYsibZ/iZEUemj++M26W3cNR5h+Tw==}
+ engines: {node: '>=12'}
+ cpu: [arm64]
+ os: [freebsd]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/freebsd-x64@0.18.20:
+ resolution: {integrity: sha512-tgWRPPuQsd3RmBZwarGVHZQvtzfEBOreNuxEMKFcd5DaDn2PbBxfwLcj4+aenoh7ctXcbXmOQIn8HI6mCSw5MQ==}
+ engines: {node: '>=12'}
+ cpu: [x64]
+ os: [freebsd]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/linux-arm64@0.18.20:
+ resolution: {integrity: sha512-2YbscF+UL7SQAVIpnWvYwM+3LskyDmPhe31pE7/aoTMFKKzIc9lLbyGUpmmb8a8AixOL61sQ/mFh3jEjHYFvdA==}
+ engines: {node: '>=12'}
+ cpu: [arm64]
+ os: [linux]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/linux-arm@0.18.20:
+ resolution: {integrity: sha512-/5bHkMWnq1EgKr1V+Ybz3s1hWXok7mDFUMQ4cG10AfW3wL02PSZi5kFpYKrptDsgb2WAJIvRcDm+qIvXf/apvg==}
+ engines: {node: '>=12'}
+ cpu: [arm]
+ os: [linux]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/linux-ia32@0.18.20:
+ resolution: {integrity: sha512-P4etWwq6IsReT0E1KHU40bOnzMHoH73aXp96Fs8TIT6z9Hu8G6+0SHSw9i2isWrD2nbx2qo5yUqACgdfVGx7TA==}
+ engines: {node: '>=12'}
+ cpu: [ia32]
+ os: [linux]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/linux-loong64@0.18.20:
+ resolution: {integrity: sha512-nXW8nqBTrOpDLPgPY9uV+/1DjxoQ7DoB2N8eocyq8I9XuqJ7BiAMDMf9n1xZM9TgW0J8zrquIb/A7s3BJv7rjg==}
+ engines: {node: '>=12'}
+ cpu: [loong64]
+ os: [linux]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/linux-mips64el@0.18.20:
+ resolution: {integrity: sha512-d5NeaXZcHp8PzYy5VnXV3VSd2D328Zb+9dEq5HE6bw6+N86JVPExrA6O68OPwobntbNJ0pzCpUFZTo3w0GyetQ==}
+ engines: {node: '>=12'}
+ cpu: [mips64el]
+ os: [linux]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/linux-ppc64@0.18.20:
+ resolution: {integrity: sha512-WHPyeScRNcmANnLQkq6AfyXRFr5D6N2sKgkFo2FqguP44Nw2eyDlbTdZwd9GYk98DZG9QItIiTlFLHJHjxP3FA==}
+ engines: {node: '>=12'}
+ cpu: [ppc64]
+ os: [linux]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/linux-riscv64@0.18.20:
+ resolution: {integrity: sha512-WSxo6h5ecI5XH34KC7w5veNnKkju3zBRLEQNY7mv5mtBmrP/MjNBCAlsM2u5hDBlS3NGcTQpoBvRzqBcRtpq1A==}
+ engines: {node: '>=12'}
+ cpu: [riscv64]
+ os: [linux]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/linux-s390x@0.18.20:
+ resolution: {integrity: sha512-+8231GMs3mAEth6Ja1iK0a1sQ3ohfcpzpRLH8uuc5/KVDFneH6jtAJLFGafpzpMRO6DzJ6AvXKze9LfFMrIHVQ==}
+ engines: {node: '>=12'}
+ cpu: [s390x]
+ os: [linux]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/linux-x64@0.18.20:
+ resolution: {integrity: sha512-UYqiqemphJcNsFEskc73jQ7B9jgwjWrSayxawS6UVFZGWrAAtkzjxSqnoclCXxWtfwLdzU+vTpcNYhpn43uP1w==}
+ engines: {node: '>=12'}
+ cpu: [x64]
+ os: [linux]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/netbsd-x64@0.18.20:
+ resolution: {integrity: sha512-iO1c++VP6xUBUmltHZoMtCUdPlnPGdBom6IrO4gyKPFFVBKioIImVooR5I83nTew5UOYrk3gIJhbZh8X44y06A==}
+ engines: {node: '>=12'}
+ cpu: [x64]
+ os: [netbsd]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/openbsd-x64@0.18.20:
+ resolution: {integrity: sha512-e5e4YSsuQfX4cxcygw/UCPIEP6wbIL+se3sxPdCiMbFLBWu0eiZOJ7WoD+ptCLrmjZBK1Wk7I6D/I3NglUGOxg==}
+ engines: {node: '>=12'}
+ cpu: [x64]
+ os: [openbsd]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/sunos-x64@0.18.20:
+ resolution: {integrity: sha512-kDbFRFp0YpTQVVrqUd5FTYmWo45zGaXe0X8E1G/LKFC0v8x0vWrhOWSLITcCn63lmZIxfOMXtCfti/RxN/0wnQ==}
+ engines: {node: '>=12'}
+ cpu: [x64]
+ os: [sunos]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/win32-arm64@0.18.20:
+ resolution: {integrity: sha512-ddYFR6ItYgoaq4v4JmQQaAI5s7npztfV4Ag6NrhiaW0RrnOXqBkgwZLofVTlq1daVTQNhtI5oieTvkRPfZrePg==}
+ engines: {node: '>=12'}
+ cpu: [arm64]
+ os: [win32]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/win32-ia32@0.18.20:
+ resolution: {integrity: sha512-Wv7QBi3ID/rROT08SABTS7eV4hX26sVduqDOTe1MvGMjNd3EjOz4b7zeexIR62GTIEKrfJXKL9LFxTYgkyeu7g==}
+ engines: {node: '>=12'}
+ cpu: [ia32]
+ os: [win32]
+ requiresBuild: true
+ optional: true
+
+ /@esbuild/win32-x64@0.18.20:
+ resolution: {integrity: sha512-kTdfRcSiDfQca/y9QIkng02avJ+NCaQvrMejlsB3RRv5sE9rRoeBPISaZpKxHELzRxZyLvNts1P27W3wV+8geQ==}
+ engines: {node: '>=12'}
+ cpu: [x64]
+ os: [win32]
+ requiresBuild: true
+ optional: true
+
+ /@eslint-community/eslint-utils@4.4.0(eslint@8.57.0):
+ resolution: {integrity: sha512-1/sA4dwrzBAyeUoQ6oxahHKmrZvsnLCg4RfxW3ZFGGmQkSNQPFNLV9CUEFQP1x9EYXHTo5p6xdhZM1Ne9p/AfA==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ peerDependencies:
+ eslint: ^6.0.0 || ^7.0.0 || >=8.0.0
+ dependencies:
+ eslint: 8.57.0
+ eslint-visitor-keys: 3.4.3
+ dev: true
+
+ /@eslint-community/regexpp@4.10.0:
+ resolution: {integrity: sha512-Cu96Sd2By9mCNTx2iyKOmq10v22jUVQv0lQnlGNy16oE9589yE+QADPbrMGCkA51cKZSg3Pu/aTJVTGfL/qjUA==}
+ engines: {node: ^12.0.0 || ^14.0.0 || >=16.0.0}
+ dev: true
+
+ /@eslint/eslintrc@2.1.4:
+ resolution: {integrity: sha512-269Z39MS6wVJtsoUl10L60WdkhJVdPG24Q4eZTH3nnF6lpvSShEK3wQjDX9JRWAUPvPh7COouPpU9IrqaZFvtQ==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ dependencies:
+ ajv: 6.12.6
+ debug: 4.3.4
+ espree: 9.6.1
+ globals: 13.24.0
+ ignore: 5.3.1
+ import-fresh: 3.3.0
+ js-yaml: 4.1.0
+ minimatch: 3.1.2
+ strip-json-comments: 3.1.1
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /@eslint/js@8.57.0:
+ resolution: {integrity: sha512-Ys+3g2TaW7gADOJzPt83SJtCDhMjndcDMFVQ/Tj9iA1BfJzFKD9mAUXT3OenpuPHbI6P/myECxRJrofUsDx/5g==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ dev: true
+
+ /@fullcalendar/common@5.11.5:
+ resolution: {integrity: sha512-3iAYiUbHXhjSVXnYWz27Od2cslztUPsOwiwKlfGvQxBixv2Kl6a8IPwaijKFYJHXdwYmfPoEgK7rvqAGVoIYwA==}
+ dependencies:
+ tslib: 2.6.2
+ dev: false
+
+ /@fullcalendar/core@6.1.11:
+ resolution: {integrity: sha512-TjG7c8sUz+Vkui2FyCNJ+xqyu0nq653Ibe99A66LoW95oBo6tVhhKIaG1Wh0GVKymYiqAQN/OEdYTuj4ay27kA==}
+ dependencies:
+ preact: 10.12.1
+ dev: false
+
+ /@fullcalendar/daygrid@6.1.11(@fullcalendar/core@6.1.11):
+ resolution: {integrity: sha512-hF5jJB7cgUIxWD5MVjj8IU407HISyLu7BWXcEIuTytkfr8oolOXeCazqnnjmRbnFOncoJQVstTtq6SIhaT32Xg==}
+ peerDependencies:
+ '@fullcalendar/core': ~6.1.11
+ dependencies:
+ '@fullcalendar/core': 6.1.11
+ dev: false
+
+ /@fullcalendar/interaction@6.1.11(@fullcalendar/core@6.1.11):
+ resolution: {integrity: sha512-ynOKjzuPwEAMgTQ6R/Z2zvzIIqG4p8/Qmnhi1q0vzPZZxSIYx3rlZuvpEK2WGBZZ1XEafDOP/LGfbWoNZe+qdg==}
+ peerDependencies:
+ '@fullcalendar/core': ~6.1.11
+ dependencies:
+ '@fullcalendar/core': 6.1.11
+ dev: false
+
+ /@fullcalendar/list@6.1.11(@fullcalendar/core@6.1.11):
+ resolution: {integrity: sha512-9Qx8uvik9pXD12u50FiHwNzlHv4wkhfsr+r03ycahW7vEeIAKCsIZGTkUfFP+96I5wHihrfLazu1cFQG4MPiuw==}
+ peerDependencies:
+ '@fullcalendar/core': ~6.1.11
+ dependencies:
+ '@fullcalendar/core': 6.1.11
+ dev: false
+
+ /@fullcalendar/premium-common@6.1.11(@fullcalendar/core@6.1.11):
+ resolution: {integrity: sha512-3WsSizkGDJEu+AlgQlFXKI+/DYCYTRp0PQRz0vS63qKBEUsVzfSD0oi/7jCylZJlCUteIjbi4Hu1I2I5o6UHrQ==}
+ peerDependencies:
+ '@fullcalendar/core': ~6.1.11
+ dependencies:
+ '@fullcalendar/core': 6.1.11
+ dev: false
+
+ /@fullcalendar/react@6.1.11(@fullcalendar/core@6.1.11)(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-Og0Tv0OiglTFp+b++yRyEhAeWnAmKkMLQ3iS0eJE1KDEov6QqGkoO+dUG4x8zp2w55IJqzik/a9iHi0s3oQDbA==}
+ peerDependencies:
+ '@fullcalendar/core': ~6.1.11
+ react: ^16.7.0 || ^17 || ^18
+ react-dom: ^16.7.0 || ^17 || ^18
+ dependencies:
+ '@fullcalendar/core': 6.1.11
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /@fullcalendar/scrollgrid@6.1.11(@fullcalendar/core@6.1.11):
+ resolution: {integrity: sha512-GRhhU8ACHqgfxdMnP59VPThfNG3Stkp9zq1sLnI992g1fZQ+V2UhYvl5qhu3eEVSp2KTDPx4NaoR35cQGrC1Mw==}
+ peerDependencies:
+ '@fullcalendar/core': ~6.1.11
+ dependencies:
+ '@fullcalendar/core': 6.1.11
+ '@fullcalendar/premium-common': 6.1.11(@fullcalendar/core@6.1.11)
+ dev: false
+
+ /@fullcalendar/timegrid@6.1.11(@fullcalendar/core@6.1.11):
+ resolution: {integrity: sha512-0seUHK/ferH89IeuCvV4Bib0zWjgK0nsptNdmAc9wDBxD/d9hm5Mdti0URJX6bDoRtsSfRDu5XsRcrzwoc+AUQ==}
+ peerDependencies:
+ '@fullcalendar/core': ~6.1.11
+ dependencies:
+ '@fullcalendar/core': 6.1.11
+ '@fullcalendar/daygrid': 6.1.11(@fullcalendar/core@6.1.11)
+ dev: false
+
+ /@fullcalendar/timeline@6.1.11(@fullcalendar/core@6.1.11):
+ resolution: {integrity: sha512-MGUEAHlFBWxpuhSWhZbHOtD9i+b8GZjqNV82MH+vwPHq1Jjqe8qj9Wby00vKikdWH+RuAB+IuiKjQaROEU0Ecw==}
+ peerDependencies:
+ '@fullcalendar/core': ~6.1.11
+ dependencies:
+ '@fullcalendar/core': 6.1.11
+ '@fullcalendar/premium-common': 6.1.11(@fullcalendar/core@6.1.11)
+ '@fullcalendar/scrollgrid': 6.1.11(@fullcalendar/core@6.1.11)
+ dev: false
+
+ /@hcaptcha/loader@1.2.4:
+ resolution: {integrity: sha512-3MNrIy/nWBfyVVvMPBKdKrX7BeadgiimW0AL/a/8TohNtJqxoySKgTJEXOQvYwlHemQpUzFrIsK74ody7JiMYw==}
+ dev: false
+
+ /@hcaptcha/react-hcaptcha@1.10.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-P0en4gEZAecah7Pt3WIaJO2gFlaLZKkI0+Tfdg8fNqsDxqT9VytZWSkH4WAkiPRULK1QcGgUZK+J56MXYmPifw==}
+ peerDependencies:
+ react: '>= 16.3.0'
+ react-dom: '>= 16.3.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@hcaptcha/loader': 1.2.4
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /@humanwhocodes/config-array@0.11.14:
+ resolution: {integrity: sha512-3T8LkOmg45BV5FICb15QQMsyUSWrQ8AygVfC7ZG32zOalnqrilm018ZVCw0eapXux8FtA33q8PSRSstjee3jSg==}
+ engines: {node: '>=10.10.0'}
+ dependencies:
+ '@humanwhocodes/object-schema': 2.0.3
+ debug: 4.3.4
+ minimatch: 3.1.2
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /@humanwhocodes/module-importer@1.0.1:
+ resolution: {integrity: sha512-bxveV4V8v5Yb4ncFTT3rPSgZBOpCkjfK0y4oVVVJwIuDVBRMDXrPyXRL988i5ap9m9bnyEEjWfm5WkBmtffLfA==}
+ engines: {node: '>=12.22'}
+ dev: true
+
+ /@humanwhocodes/object-schema@2.0.3:
+ resolution: {integrity: sha512-93zYdMES/c1D69yZiKDBj0V24vqNzB/koF26KPaagAfd3P/4gUlh3Dys5ogAK+Exi9QyzlD8x/08Zt7wIKcDcA==}
+ dev: true
+
+ /@iconify/react@4.1.1(react@18.3.0):
+ resolution: {integrity: sha512-jed14EjvKjee8mc0eoscGxlg7mSQRkwQG3iX3cPBCO7UlOjz0DtlvTqxqEcHUJGh+z1VJ31Yhu5B9PxfO0zbdg==}
+ peerDependencies:
+ react: '>=16'
+ dependencies:
+ '@iconify/types': 2.0.0
+ react: 18.3.0
+ dev: false
+
+ /@iconify/types@2.0.0:
+ resolution: {integrity: sha512-+wluvCrRhXrhyOmRDJ3q8mux9JkKy5SJ/v8ol2tu4FVjyYvtEzkc/3pK15ET6RKg4b4w4BmTk1+gsCUhf21Ykg==}
+ dev: false
+
+ /@isaacs/cliui@8.0.2:
+ resolution: {integrity: sha512-O8jcjabXaleOG9DQ0+ARXWZBTfnP4WNAqzuiJK7ll44AmxGKv/J2M4TPjxjY3znBCfvBXFzucm1twdyFybFqEA==}
+ engines: {node: '>=12'}
+ dependencies:
+ string-width: 5.1.2
+ string-width-cjs: /string-width@4.2.3
+ strip-ansi: 7.1.0
+ strip-ansi-cjs: /strip-ansi@6.0.1
+ wrap-ansi: 8.1.0
+ wrap-ansi-cjs: /wrap-ansi@7.0.0
+ dev: true
+
+ /@jridgewell/gen-mapping@0.3.5:
+ resolution: {integrity: sha512-IzL8ZoEDIBRWEzlCcRhOaCupYyN5gdIK+Q6fbFdPDg6HqX6jpkItn7DFIpW9LQzXG6Df9sA7+OKnq0qlz/GaQg==}
+ engines: {node: '>=6.0.0'}
+ dependencies:
+ '@jridgewell/set-array': 1.2.1
+ '@jridgewell/sourcemap-codec': 1.4.15
+ '@jridgewell/trace-mapping': 0.3.25
+
+ /@jridgewell/resolve-uri@3.1.2:
+ resolution: {integrity: sha512-bRISgCIjP20/tbWSPWMEi54QVPRZExkuD9lJL+UIxUKtwVJA8wW1Trb1jMs1RFXo1CBTNZ/5hpC9QvmKWdopKw==}
+ engines: {node: '>=6.0.0'}
+
+ /@jridgewell/set-array@1.2.1:
+ resolution: {integrity: sha512-R8gLRTZeyp03ymzP/6Lil/28tGeGEzhx1q2k703KGWRAI1VdvPIXdG70VJc2pAMw3NA6JKL5hhFu1sJX0Mnn/A==}
+ engines: {node: '>=6.0.0'}
+
+ /@jridgewell/source-map@0.3.6:
+ resolution: {integrity: sha512-1ZJTZebgqllO79ue2bm3rIGud/bOe0pP5BjSRCRxxYkEZS8STV7zN84UBbiYu7jy+eCKSnVIUgoWWE/tt+shMQ==}
+ dependencies:
+ '@jridgewell/gen-mapping': 0.3.5
+ '@jridgewell/trace-mapping': 0.3.25
+
+ /@jridgewell/sourcemap-codec@1.4.15:
+ resolution: {integrity: sha512-eF2rxCRulEKXHTRiDrDy6erMYWqNw4LPdQ8UQA4huuxaQsVeRPFl2oM8oDGxMFhJUWZf9McpLtJasDDZb/Bpeg==}
+
+ /@jridgewell/trace-mapping@0.3.25:
+ resolution: {integrity: sha512-vNk6aEwybGtawWmy/PzwnGDOjCkLWSD2wqvjGGAgOAwCGWySYXfYoxt00IJkTF+8Lb57DwOb3Aa0o9CApepiYQ==}
+ dependencies:
+ '@jridgewell/resolve-uri': 3.1.2
+ '@jridgewell/sourcemap-codec': 1.4.15
+
+ /@jridgewell/trace-mapping@0.3.9:
+ resolution: {integrity: sha512-3Belt6tdc8bPgAtbcmdtNJlirVoTmEb5e2gC94PnkwEW9jI6CAHUeoG85tjWP5WquqfavoMtMwiG4P926ZKKuQ==}
+ dependencies:
+ '@jridgewell/resolve-uri': 3.1.2
+ '@jridgewell/sourcemap-codec': 1.4.15
+ dev: true
+
+ /@mrmlnc/readdir-enhanced@2.2.1:
+ resolution: {integrity: sha512-bPHp6Ji8b41szTOcaP63VlnbbO5Ny6dwAATtY6JTjh5N2OLrb5Qk/Th5cRkRQhkWCt+EJsYrNB0MiL+Gpn6e3g==}
+ engines: {node: '>=4'}
+ dependencies:
+ call-me-maybe: 1.0.2
+ glob-to-regexp: 0.3.0
+ dev: true
+
+ /@nodelib/fs.scandir@2.1.5:
+ resolution: {integrity: sha512-vq24Bq3ym5HEQm2NKCr3yXDwjc7vTsEThRDnkp2DK9p1uqLR+DHurm/NOTo0KG7HYHU7eppKZj3MyqYuMBf62g==}
+ engines: {node: '>= 8'}
+ dependencies:
+ '@nodelib/fs.stat': 2.0.5
+ run-parallel: 1.2.0
+ dev: true
+
+ /@nodelib/fs.stat@1.1.3:
+ resolution: {integrity: sha512-shAmDyaQC4H92APFoIaVDHCx5bStIocgvbwQyxPRrbUY20V1EYTbSDchWbuwlMG3V17cprZhA6+78JfB+3DTPw==}
+ engines: {node: '>= 6'}
+ dev: true
+
+ /@nodelib/fs.stat@2.0.5:
+ resolution: {integrity: sha512-RkhPPp2zrqDAQA/2jNhnztcPAlv64XdhIp7a7454A5ovI7Bukxgt7MX7udwAu3zg1DcpPU0rz3VV1SeaqvY4+A==}
+ engines: {node: '>= 8'}
+ dev: true
+
+ /@nodelib/fs.walk@1.2.8:
+ resolution: {integrity: sha512-oGB+UxlgWcgQkgwo8GcEGwemoTFt3FIO9ababBmaGwXIoBKZ+GTy0pP185beGg7Llih/NSHSV2XAs1lnznocSg==}
+ engines: {node: '>= 8'}
+ dependencies:
+ '@nodelib/fs.scandir': 2.1.5
+ fastq: 1.17.1
+ dev: true
+
+ /@pkgjs/parseargs@0.11.0:
+ resolution: {integrity: sha512-+1VkjdD0QBLPodGrJUeqarH8VAIvQODIbwh9XpP5Syisf7YoQgsJKPNFoqqLQlu+VQ/tVSshMR6loPMn8U+dPg==}
+ engines: {node: '>=14'}
+ requiresBuild: true
+ dev: true
+ optional: true
+
+ /@rc-component/color-picker@1.5.3(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-+tGGH3nLmYXTalVe0L8hSZNs73VTP5ueSHwUlDC77KKRaN7G4DS4wcpG5DTDzdcV/Yas+rzA6UGgIyzd8fS4cw==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@ctrl/tinycolor': 3.6.1
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /@rc-component/context@1.4.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-kFcNxg9oLRMoL3qki0OMxK+7g5mypjgaaJp/pkOis/6rVxma9nJBF/8kCIuTYHUQNr0ii7MxqE33wirPZLJQ2w==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /@rc-component/mini-decimal@1.1.0:
+ resolution: {integrity: sha512-jS4E7T9Li2GuYwI6PyiVXmxTiM6b07rlD9Ge8uGZSCz3WlzcG5ZK7g5bbuKNeZ9pgUuPK/5guV781ujdVpm4HQ==}
+ engines: {node: '>=8.x'}
+ dependencies:
+ '@babel/runtime': 7.24.4
+ dev: false
+
+ /@rc-component/mutate-observer@1.1.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-QjrOsDXQusNwGZPf4/qRQasg7UFEj06XiCJ8iuiq/Io7CrHrgVi6Uuetw60WAMG1799v+aM8kyc+1L/GBbHSlw==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /@rc-component/portal@1.1.2(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-6f813C0IsasTZms08kfA8kPAGxbbkYToa8ALaiDIGGECU4i9hj8Plgbx0sNJDrey3EtHO30hmdaxtT0138xZcg==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /@rc-component/tour@1.14.2(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-A75DZ8LVvahBIvxooj3Gvf2sxe+CGOkmzPNX7ek0i0AJHyKZ1HXe5ieIGo3m0FMdZfVOlbCJ952Duq8VKAHk6g==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/portal': 1.1.2(react-dom@18.3.0)(react@18.3.0)
+ '@rc-component/trigger': 2.1.1(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /@rc-component/trigger@2.1.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-UjHkedkgtEcgQu87w1VuWug1idoDJV7VUt0swxHXRcmei2uu1AuUzGBPEUlmOmXGJ+YtTgZfVLi7kuAUKoZTMA==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/portal': 1.1.2(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-resize-observer: 1.4.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /@react-dev-inspector/babel-plugin@2.0.1:
+ resolution: {integrity: sha512-V2MzN9dj3uZu6NvAjSxXwa3+FOciVIuwAUwPLpO6ji5xpUyx8E6UiEng1QqzttdpacKHFKtkNYjtQAE+Lsqa5A==}
+ engines: {node: '>=12.0.0'}
+ dependencies:
+ '@babel/core': 7.24.4
+ '@babel/generator': 7.24.4
+ '@babel/parser': 7.24.4
+ '@babel/traverse': 7.24.1
+ '@babel/types': 7.20.5
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /@react-dev-inspector/middleware@2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0):
+ resolution: {integrity: sha512-qDMtBzAxNNAX01jjU1THZVuNiVB7J1Hjk42k8iLSSwfinc3hk667iqgdzeq1Za1a0V2bF5Ev6D4+nkZ+E1YUrQ==}
+ engines: {node: '>=12.0.0'}
+ dependencies:
+ react-dev-utils: 12.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0)
+ transitivePeerDependencies:
+ - eslint
+ - supports-color
+ - typescript
+ - vue-template-compiler
+ - webpack
+ dev: true
+
+ /@react-dev-inspector/umi3-plugin@2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0):
+ resolution: {integrity: sha512-lRw65yKQdI/1BwrRXWJEHDJel4DWboOartGmR3S5xiTF+EiOLjmndxdA5LoVSdqbcggdtq5SWcsoZqI0TkhH7Q==}
+ engines: {node: '>=12.0.0'}
+ dependencies:
+ '@react-dev-inspector/babel-plugin': 2.0.1
+ '@react-dev-inspector/middleware': 2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0)
+ transitivePeerDependencies:
+ - eslint
+ - supports-color
+ - typescript
+ - vue-template-compiler
+ - webpack
+ dev: true
+
+ /@react-dev-inspector/umi4-plugin@2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0):
+ resolution: {integrity: sha512-vTefsJVAZsgpuO9IZ1ZFIoyryVUU+hjV8OPD8DfDU+po5LjVXc5Uncn+MkFOsT24AMpNdDvCnTRYiuSkFn8EsA==}
+ engines: {node: '>=12.0.0'}
+ dependencies:
+ '@react-dev-inspector/babel-plugin': 2.0.1
+ '@react-dev-inspector/middleware': 2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0)
+ transitivePeerDependencies:
+ - eslint
+ - supports-color
+ - typescript
+ - vue-template-compiler
+ - webpack
+ dev: true
+
+ /@react-dev-inspector/vite-plugin@2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0):
+ resolution: {integrity: sha512-J1eI7cIm2IXE6EwhHR1OyoefvobUJEn/vJWEBwOM5uW4JkkLwuVoV9vk++XJyAmKUNQ87gdWZvSWrI2LjfrSug==}
+ engines: {node: '>=12.0.0'}
+ dependencies:
+ '@react-dev-inspector/middleware': 2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0)
+ transitivePeerDependencies:
+ - eslint
+ - supports-color
+ - typescript
+ - vue-template-compiler
+ - webpack
+ dev: true
+
+ /@remix-run/router@1.16.0:
+ resolution: {integrity: sha512-Quz1KOffeEf/zwkCBM3kBtH4ZoZ+pT3xIXBG4PPW/XFtDP7EGhtTiC2+gpL9GnR7+Qdet5Oa6cYSvwKYg6kN9Q==}
+ engines: {node: '>=14.0.0'}
+ dev: false
+
+ /@tanstack/match-sorter-utils@8.15.1:
+ resolution: {integrity: sha512-PnVV3d2poenUM31ZbZi/yXkBu3J7kd5k2u51CGwwNojag451AjTH9N6n41yjXz2fpLeewleyLBmNS6+HcGDlXw==}
+ engines: {node: '>=12'}
+ dependencies:
+ remove-accents: 0.5.0
+ dev: false
+
+ /@tanstack/query-core@4.36.1:
+ resolution: {integrity: sha512-DJSilV5+ytBP1FbFcEJovv4rnnm/CokuVvrBEtW/Va9DvuJ3HksbXUJEpI0aV1KtuL4ZoO9AVE6PyNLzF7tLeA==}
+ dev: false
+
+ /@tanstack/react-query-devtools@4.36.1(@tanstack/react-query@4.36.1)(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-WYku83CKP3OevnYSG8Y/QO9g0rT75v1om5IvcWUwiUZJ4LanYGLVCZ8TdFG5jfsq4Ej/lu2wwDAULEUnRIMBSw==}
+ peerDependencies:
+ '@tanstack/react-query': ^4.36.1
+ react: ^16.8.0 || ^17.0.0 || ^18.0.0
+ react-dom: ^16.8.0 || ^17.0.0 || ^18.0.0
+ dependencies:
+ '@tanstack/match-sorter-utils': 8.15.1
+ '@tanstack/react-query': 4.36.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ superjson: 1.13.3
+ use-sync-external-store: 1.2.1(react@18.3.0)
+ dev: false
+
+ /@tanstack/react-query@4.36.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-y7ySVHFyyQblPl3J3eQBWpXZkliroki3ARnBKsdJchlgt7yJLRDUcf4B8soufgiYt3pEQIkBWBx1N9/ZPIeUWw==}
+ peerDependencies:
+ react: ^16.8.0 || ^17.0.0 || ^18.0.0
+ react-dom: ^16.8.0 || ^17.0.0 || ^18.0.0
+ react-native: '*'
+ peerDependenciesMeta:
+ react-dom:
+ optional: true
+ react-native:
+ optional: true
+ dependencies:
+ '@tanstack/query-core': 4.36.1
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ use-sync-external-store: 1.2.1(react@18.3.0)
+ dev: false
+
+ /@trysound/sax@0.2.0:
+ resolution: {integrity: sha512-L7z9BgrNEcYyUYtF+HaEfiS5ebkh9jXqbszz7pC0hRBPaatV0XjSD3+eHrpqFemQfgwiFF0QPIarnIihIDn7OA==}
+ engines: {node: '>=10.13.0'}
+ dev: true
+
+ /@tsconfig/node10@1.0.11:
+ resolution: {integrity: sha512-DcRjDCujK/kCk/cUe8Xz8ZSpm8mS3mNNpta+jGCA6USEDfktlNvm1+IuZ9eTcDbNk41BHwpHHeW+N1lKCz4zOw==}
+ dev: true
+
+ /@tsconfig/node12@1.0.11:
+ resolution: {integrity: sha512-cqefuRsh12pWyGsIoBKJA9luFu3mRxCA+ORZvA4ktLSzIuCUtWVxGIuXigEwO5/ywWFMZ2QEGKWvkZG1zDMTag==}
+ dev: true
+
+ /@tsconfig/node14@1.0.3:
+ resolution: {integrity: sha512-ysT8mhdixWK6Hw3i1V2AeRqZ5WfXg1G43mqoYlM2nc6388Fq5jcXyr5mRsqViLx/GJYdoL0bfXD8nmF+Zn/Iow==}
+ dev: true
+
+ /@tsconfig/node16@1.0.4:
+ resolution: {integrity: sha512-vxhUy4J8lyeyinH7Azl1pdd43GJhZH/tP2weN8TntQblOY+A0XbT8DJk1/oCPuOOyg/Ja757rG0CgHcWC8OfMA==}
+ dev: true
+
+ /@types/autosuggest-highlight@3.2.3:
+ resolution: {integrity: sha512-8Mb21KWtpn6PvRQXjsKhrXIcxbSloGqNH50RntwGeJsGPW4xvNhfml+3kKulaKpO/7pgZfOmzsJz7VbepArlGQ==}
+ dev: true
+
+ /@types/babel__core@7.20.5:
+ resolution: {integrity: sha512-qoQprZvz5wQFJwMDqeseRXWv3rqMvhgpbXFfVyWhbx9X47POIA6i/+dXefEmZKoAgOaTdaIgNSMqMIU61yRyzA==}
+ dependencies:
+ '@babel/parser': 7.24.4
+ '@babel/types': 7.24.0
+ '@types/babel__generator': 7.6.8
+ '@types/babel__template': 7.4.4
+ '@types/babel__traverse': 7.20.5
+ dev: false
+
+ /@types/babel__generator@7.6.8:
+ resolution: {integrity: sha512-ASsj+tpEDsEiFr1arWrlN6V3mdfjRMZt6LtK/Vp/kreFLnr5QH5+DhvD5nINYZXzwJvXeGq+05iUXcAzVrqWtw==}
+ dependencies:
+ '@babel/types': 7.24.0
+ dev: false
+
+ /@types/babel__template@7.4.4:
+ resolution: {integrity: sha512-h/NUaSyG5EyxBIp8YRxo4RMe2/qQgvyowRwVMzhYhBCONbW8PUsg4lkFMrhgZhUe5z3L3MiLDuvyJ/CaPa2A8A==}
+ dependencies:
+ '@babel/parser': 7.24.4
+ '@babel/types': 7.24.0
+ dev: false
+
+ /@types/babel__traverse@7.20.5:
+ resolution: {integrity: sha512-WXCyOcRtH37HAUkpXhUduaxdm82b4GSlyTqajXviN4EfiuPgNYR109xMCKvpl6zPIpua0DGlMEDCq+g8EdoheQ==}
+ dependencies:
+ '@babel/types': 7.24.0
+ dev: false
+
+ /@types/color-convert@2.0.3:
+ resolution: {integrity: sha512-2Q6wzrNiuEvYxVQqhh7sXM2mhIhvZR/Paq4FdsQkOMgWsCIkKvSGj8Le1/XalulrmgOzPMqNa0ix+ePY4hTrfg==}
+ dependencies:
+ '@types/color-name': 1.1.4
+ dev: true
+
+ /@types/color-name@1.1.4:
+ resolution: {integrity: sha512-hulKeREDdLFesGQjl96+4aoJSHY5b2GRjagzzcqCfIrWhe5vkCqIvrLbqzBaI1q94Vg8DNJZZqTR5ocdWmWclg==}
+ dev: true
+
+ /@types/color@3.0.6:
+ resolution: {integrity: sha512-NMiNcZFRUAiUUCCf7zkAelY8eV3aKqfbzyFQlXpPIEeoNDbsEHGpb854V3gzTsGKYj830I5zPuOwU/TP5/cW6A==}
+ dependencies:
+ '@types/color-convert': 2.0.3
+ dev: true
+
+ /@types/debug@4.1.12:
+ resolution: {integrity: sha512-vIChWdVG3LG1SMxEvI/AK+FWJthlrqlTu7fbrlywTkkaONwk/UAGaULXRlf8vkzFBLVm0zkMdCquhL5aOjhXPQ==}
+ dependencies:
+ '@types/ms': 0.7.34
+ dev: false
+
+ /@types/eslint-scope@3.7.7:
+ resolution: {integrity: sha512-MzMFlSLBqNF2gcHWO0G1vP/YQyfvrxZ0bF+u7mzUdZ1/xK4A4sru+nraZz5i3iEIk1l1uyicaDVTB4QbbEkAYg==}
+ dependencies:
+ '@types/eslint': 8.56.10
+ '@types/estree': 1.0.5
+ dev: true
+
+ /@types/eslint@8.56.10:
+ resolution: {integrity: sha512-Shavhk87gCtY2fhXDctcfS3e6FdxWkCx1iUZ9eEUbh7rTqlZT0/IzOkCOVt0fCjcFuZ9FPYfuezTBImfHCDBGQ==}
+ dependencies:
+ '@types/estree': 1.0.5
+ '@types/json-schema': 7.0.15
+ dev: true
+
+ /@types/estree@1.0.5:
+ resolution: {integrity: sha512-/kYRxGDLWzHOB7q+wtSUQlFrtcdUccpfy+X+9iMBpHK8QLLhx2wIPYuS5DYtR9Wa/YlZAbIovy7qVdB1Aq6Lyw==}
+ dev: true
+
+ /@types/glob@7.2.0:
+ resolution: {integrity: sha512-ZUxbzKl0IfJILTS6t7ip5fQQM/J3TJYubDm3nMbgubNNYS62eXeUpoLUC8/7fJNiFYHTrGPQn7hspDUzIHX3UA==}
+ dependencies:
+ '@types/minimatch': 5.1.2
+ '@types/node': 20.12.7
+ dev: true
+
+ /@types/hast@2.3.10:
+ resolution: {integrity: sha512-McWspRw8xx8J9HurkVBfYj0xKoE25tOFlHGdx4MJ5xORQrMGZNqJhVQWaIbm6Oyla5kYOXtDiopzKRJzEOkwJw==}
+ dependencies:
+ '@types/unist': 2.0.10
+ dev: false
+
+ /@types/history@4.7.11:
+ resolution: {integrity: sha512-qjDJRrmvBMiTx+jyLxvLfJU7UznFuokDv4f3WRuriHKERccVpFU+8XMQUAbDzoiJCsmexxRExQeMwwCdamSKDA==}
+ dev: true
+
+ /@types/hoist-non-react-statics@3.3.5:
+ resolution: {integrity: sha512-SbcrWzkKBw2cdwRTwQAswfpB9g9LJWfjtUeW/jvNwbhC8cpmmNYVePa+ncbUe0rGTQ7G3Ff6mYUN2VMfLVr+Sg==}
+ dependencies:
+ '@types/react': 18.3.0
+ hoist-non-react-statics: 3.3.2
+
+ /@types/humps@2.0.6:
+ resolution: {integrity: sha512-Fagm1/a/1J9gDKzGdtlPmmTN5eSw/aaTzHtj740oSfo+MODsSY2WglxMmhTdOglC8nxqUhGGQ+5HfVtBvxo3Kg==}
+ dev: true
+
+ /@types/js-cookie@2.2.7:
+ resolution: {integrity: sha512-aLkWa0C0vO5b4Sr798E26QgOkss68Un0bLjs7u9qxzPT5CG+8DuNTffWES58YzJs3hrVAOs1wonycqEBqNJubA==}
+ dev: false
+
+ /@types/json-schema@7.0.15:
+ resolution: {integrity: sha512-5+fP8P8MFNC+AyZCDxrB2pkZFPGzqQWUzpSeuuVLvm8VMcorNYavBqoFcxK8bQz4Qsbn4oUEEem4wDLfcysGHA==}
+ dev: true
+
+ /@types/json5@0.0.29:
+ resolution: {integrity: sha512-dRLjCWHYg4oaA77cxO64oO+7JwCwnIzkZPdrrC71jQmQtlhM556pwKo5bUzqvZndkVbeFLIIi+9TC40JNF5hNQ==}
+ dev: true
+
+ /@types/lodash-es@4.17.12:
+ resolution: {integrity: sha512-0NgftHUcV4v34VhXm8QBSftKVXtbkBG3ViCjs6+eJ5a6y6Mi/jiFGPc1sC7QK+9BFhWrURE3EOggmWaSxL9OzQ==}
+ dependencies:
+ '@types/lodash': 4.17.0
+ dev: false
+
+ /@types/lodash@4.17.0:
+ resolution: {integrity: sha512-t7dhREVv6dbNj0q17X12j7yDG4bD/DHYX7o5/DbDxobP0HnGPgpRz2Ej77aL7TZT3DSw13fqUTj8J4mMnqa7WA==}
+ dev: false
+
+ /@types/mdast@3.0.15:
+ resolution: {integrity: sha512-LnwD+mUEfxWMa1QpDraczIn6k0Ee3SMicuYSSzS6ZYl2gKS09EClnJYGd8Du6rfc5r/GZEk5o1mRb8TaTj03sQ==}
+ dependencies:
+ '@types/unist': 2.0.10
+ dev: false
+
+ /@types/minimatch@5.1.2:
+ resolution: {integrity: sha512-K0VQKziLUWkVKiRVrx4a40iPaxTUefQmjtkQofBkYRcoaaL/8rhwDWww9qWbrgicNOgnpIsMxyNIUM4+n6dUIA==}
+ dev: true
+
+ /@types/minimist@1.2.5:
+ resolution: {integrity: sha512-hov8bUuiLiyFPGyFPE1lwWhmzYbirOXQNNo40+y3zow8aFVTeyn3VWL0VFFfdNddA8S4Vf0Tc062rzyNr7Paag==}
+ dev: true
+
+ /@types/ms@0.7.34:
+ resolution: {integrity: sha512-nG96G3Wp6acyAgJqGasjODb+acrI7KltPiRxzHPXnP3NgI28bpQDRv53olbqGXbfcgF5aiiHmO3xpwEpS5Ld9g==}
+ dev: false
+
+ /@types/node@20.12.7:
+ resolution: {integrity: sha512-wq0cICSkRLVaf3UGLMGItu/PtdY7oaXaI/RVU+xliKVOtRna3PRY57ZDfztpDL0n11vfymMUnXv8QwYCO7L1wg==}
+ dependencies:
+ undici-types: 5.26.5
+
+ /@types/node@20.5.1:
+ resolution: {integrity: sha512-4tT2UrL5LBqDwoed9wZ6N3umC4Yhz3W3FloMmiiG4JwmUJWpie0c7lcnUNd4gtMKuDEO4wRVS8B6Xa0uMRsMKg==}
+ dev: true
+
+ /@types/normalize-package-data@2.4.4:
+ resolution: {integrity: sha512-37i+OaWTh9qeK4LSHPsyRC7NahnGotNuZvjLSgcPzblpHB3rrCJxAOgI5gCdKm7coonsaX1Of0ILiTcnZjbfxA==}
+ dev: true
+
+ /@types/nprogress@0.2.3:
+ resolution: {integrity: sha512-k7kRA033QNtC+gLc4VPlfnue58CM1iQLgn1IMAU8VPHGOj7oIHPp9UlhedEnD/Gl8evoCjwkZjlBORtZ3JByUA==}
+ dev: true
+
+ /@types/numeral@2.0.5:
+ resolution: {integrity: sha512-kH8I7OSSwQu9DS9JYdFWbuvhVzvFRoCPCkGxNwoGgaPeDfEPJlcxNvEOypZhQ3XXHsGbfIuYcxcJxKUfJHnRfw==}
+ dev: true
+
+ /@types/parse-json@4.0.2:
+ resolution: {integrity: sha512-dISoDXWWQwUquiKsyZ4Ng+HX2KsPL7LyHKHQwgGFEA3IaKac4Obd+h2a/a6waisAoepJlBcx9paWqjA8/HVjCw==}
+
+ /@types/parse5@6.0.3:
+ resolution: {integrity: sha512-SuT16Q1K51EAVPz1K29DJ/sXjhSQ0zjvsypYJ6tlwVsRV9jwW5Adq2ch8Dq8kDBCkYnELS7N7VNCSB5nC56t/g==}
+ dev: false
+
+ /@types/prop-types@15.7.12:
+ resolution: {integrity: sha512-5zvhXYtRNRluoE/jAp4GVsSduVUzNWKkOZrCDBWYtE7biZywwdC2AcEzg+cSMLFRfVgeAFqpfNabiPjxFddV1Q==}
+
+ /@types/quill@1.3.10:
+ resolution: {integrity: sha512-IhW3fPW+bkt9MLNlycw8u8fWb7oO7W5URC9MfZYHBlA24rex9rs23D5DETChu1zvgVdc5ka64ICjJOgQMr6Shw==}
+ dependencies:
+ parchment: 1.1.4
+ dev: false
+
+ /@types/ramda@0.29.12:
+ resolution: {integrity: sha512-sgIEjpJhdQPB52gDF4aphs9nl0xe54CR22DPdWqT8gQHjZYmVApgA0R3/CpMbl0Y8az2TEZrPNL2zy0EvjbkLA==}
+ dependencies:
+ types-ramda: 0.29.10
+ dev: true
+
+ /@types/react-beautiful-dnd@13.1.8:
+ resolution: {integrity: sha512-E3TyFsro9pQuK4r8S/OL6G99eq7p8v29sX0PM7oT8Z+PJfZvSQTx4zTQbUJ+QZXioAF0e7TGBEcA1XhYhCweyQ==}
+ dependencies:
+ '@types/react': 18.3.0
+ dev: true
+
+ /@types/react-dom@18.3.0:
+ resolution: {integrity: sha512-EhwApuTmMBmXuFOikhQLIBUn6uFg81SwLMOAUgodJF14SOBOCMdU04gDoYi0WOJJHD144TL32z4yDqCW3dnkQg==}
+ dependencies:
+ '@types/react': 18.3.0
+ dev: true
+
+ /@types/react-reconciler@0.28.8:
+ resolution: {integrity: sha512-SN9c4kxXZonFhbX4hJrZy37yw9e7EIxcpHCxQv5JUS18wDE5ovkQKlqQEkufdJCCMfuI9BnjUJvhYeJ9x5Ra7g==}
+ dependencies:
+ '@types/react': 18.3.0
+ dev: true
+
+ /@types/react-redux@7.1.33:
+ resolution: {integrity: sha512-NF8m5AjWCkert+fosDsN3hAlHzpjSiXlVy9EgQEmLoBhaNXbmyeGs/aj5dQzKuF+/q+S7JQagorGDW8pJ28Hmg==}
+ dependencies:
+ '@types/hoist-non-react-statics': 3.3.5
+ '@types/react': 18.3.0
+ hoist-non-react-statics: 3.3.2
+ redux: 4.2.1
+ dev: false
+
+ /@types/react-router-dom@5.3.3:
+ resolution: {integrity: sha512-kpqnYK4wcdm5UaWI3fLcELopqLrHgLqNsdpHauzlQktfkHL3npOSwtj1Uz9oKBAzs7lFtVkV8j83voAz2D8fhw==}
+ dependencies:
+ '@types/history': 4.7.11
+ '@types/react': 18.3.0
+ '@types/react-router': 5.1.20
+ dev: true
+
+ /@types/react-router@5.1.20:
+ resolution: {integrity: sha512-jGjmu/ZqS7FjSH6owMcD5qpq19+1RS9DeVRqfl1FeBMxTDQAGwlMWOcs52NDoXaNKyG3d1cYQFMs9rCrb88o9Q==}
+ dependencies:
+ '@types/history': 4.7.11
+ '@types/react': 18.3.0
+ dev: true
+
+ /@types/react@18.3.0:
+ resolution: {integrity: sha512-DiUcKjzE6soLyln8NNZmyhcQjVv+WsUIFSqetMN0p8927OztKT4VTfFTqsbAi5oAGIcgOmOajlfBqyptDDjZRw==}
+ dependencies:
+ '@types/prop-types': 15.7.12
+ csstype: 3.1.3
+
+ /@types/semver@7.5.8:
+ resolution: {integrity: sha512-I8EUhyrgfLrcTkzV3TSsGyl1tSuPrEDzr0yd5m90UgNxQkyDXULk3b6MlQqTCpZpNtWe1K0hzclnZkTcLBe2UQ==}
+ dev: true
+
+ /@types/styled-components@5.1.34:
+ resolution: {integrity: sha512-mmiVvwpYklFIv9E8qfxuPyIt/OuyIrn6gMOAMOFUO3WJfSrSE+sGUoa4PiZj77Ut7bKZpaa6o1fBKS/4TOEvnA==}
+ dependencies:
+ '@types/hoist-non-react-statics': 3.3.5
+ '@types/react': 18.3.0
+ csstype: 3.1.3
+ dev: true
+
+ /@types/stylis@4.2.0:
+ resolution: {integrity: sha512-n4sx2bqL0mW1tvDf/loQ+aMX7GQD3lc3fkCMC55VFNDu/vBOabO+LTIeXKM14xK0ppk5TUGcWRjiSpIlUpghKw==}
+ dev: false
+
+ /@types/svgo@2.6.4:
+ resolution: {integrity: sha512-l4cmyPEckf8moNYHdJ+4wkHvFxjyW6ulm9l4YGaOxeyBWPhBOT0gvni1InpFPdzx1dKf/2s62qGITwxNWnPQng==}
+ dependencies:
+ '@types/node': 20.12.7
+ dev: true
+
+ /@types/unist@2.0.10:
+ resolution: {integrity: sha512-IfYcSBWE3hLpBg8+X2SEa8LVkJdJEkT2Ese2aaLs3ptGdVtABxndrMaxuFlQ1qdFf9Q5rDvDpxI3WwgvKFAsQA==}
+
+ /@types/unist@3.0.2:
+ resolution: {integrity: sha512-dqId9J8K/vGi5Zr7oo212BGii5m3q5Hxlkwy3WpYuKPklmBEvsbMYYyLxAQpSffdLl/gdW0XUpKWFvYmyoWCoQ==}
+ dev: true
+
+ /@types/vfile-message@2.0.0:
+ resolution: {integrity: sha512-GpTIuDpb9u4zIO165fUy9+fXcULdD8HFRNli04GehoMVbeNq7D6OBnqSmg3lxZnC+UvgUhEWKxdKiwYUkGltIw==}
+ deprecated: This is a stub types definition. vfile-message provides its own type definitions, so you do not need this installed.
+ dependencies:
+ vfile-message: 4.0.2
+ dev: true
+
+ /@types/vfile@3.0.2:
+ resolution: {integrity: sha512-b3nLFGaGkJ9rzOcuXRfHkZMdjsawuDD0ENL9fzTophtBg8FJHSGbH7daXkEpcwy3v7Xol3pAvsmlYyFhR4pqJw==}
+ dependencies:
+ '@types/node': 20.12.7
+ '@types/unist': 2.0.10
+ '@types/vfile-message': 2.0.0
+ dev: true
+
+ /@typescript-eslint/eslint-plugin@5.62.0(@typescript-eslint/parser@5.62.0)(eslint@8.57.0)(typescript@5.4.5):
+ resolution: {integrity: sha512-TiZzBSJja/LbhNPvk6yc0JrX9XqhQ0hdh6M2svYfsHGejaKFIAGd9MQ+ERIMzLGlN/kZoYIgdxFV0PuljTKXag==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ peerDependencies:
+ '@typescript-eslint/parser': ^5.0.0
+ eslint: ^6.0.0 || ^7.0.0 || ^8.0.0
+ typescript: '*'
+ peerDependenciesMeta:
+ typescript:
+ optional: true
+ dependencies:
+ '@eslint-community/regexpp': 4.10.0
+ '@typescript-eslint/parser': 5.62.0(eslint@8.57.0)(typescript@5.4.5)
+ '@typescript-eslint/scope-manager': 5.62.0
+ '@typescript-eslint/type-utils': 5.62.0(eslint@8.57.0)(typescript@5.4.5)
+ '@typescript-eslint/utils': 5.62.0(eslint@8.57.0)(typescript@5.4.5)
+ debug: 4.3.4
+ eslint: 8.57.0
+ graphemer: 1.4.0
+ ignore: 5.3.1
+ natural-compare-lite: 1.4.0
+ semver: 7.6.0
+ tsutils: 3.21.0(typescript@5.4.5)
+ typescript: 5.4.5
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /@typescript-eslint/parser@5.62.0(eslint@8.57.0)(typescript@5.4.5):
+ resolution: {integrity: sha512-VlJEV0fOQ7BExOsHYAGrgbEiZoi8D+Bl2+f6V2RrXerRSylnp+ZBHmPvaIa8cz0Ajx7WO7Z5RqfgYg7ED1nRhA==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ peerDependencies:
+ eslint: ^6.0.0 || ^7.0.0 || ^8.0.0
+ typescript: '*'
+ peerDependenciesMeta:
+ typescript:
+ optional: true
+ dependencies:
+ '@typescript-eslint/scope-manager': 5.62.0
+ '@typescript-eslint/types': 5.62.0
+ '@typescript-eslint/typescript-estree': 5.62.0(typescript@5.4.5)
+ debug: 4.3.4
+ eslint: 8.57.0
+ typescript: 5.4.5
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /@typescript-eslint/scope-manager@5.62.0:
+ resolution: {integrity: sha512-VXuvVvZeQCQb5Zgf4HAxc04q5j+WrNAtNh9OwCsCgpKqESMTu3tF/jhZ3xG6T4NZwWl65Bg8KuS2uEvhSfLl0w==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ dependencies:
+ '@typescript-eslint/types': 5.62.0
+ '@typescript-eslint/visitor-keys': 5.62.0
+ dev: true
+
+ /@typescript-eslint/type-utils@5.62.0(eslint@8.57.0)(typescript@5.4.5):
+ resolution: {integrity: sha512-xsSQreu+VnfbqQpW5vnCJdq1Z3Q0U31qiWmRhr98ONQmcp/yhiPJFPq8MXiJVLiksmOKSjIldZzkebzHuCGzew==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ peerDependencies:
+ eslint: '*'
+ typescript: '*'
+ peerDependenciesMeta:
+ typescript:
+ optional: true
+ dependencies:
+ '@typescript-eslint/typescript-estree': 5.62.0(typescript@5.4.5)
+ '@typescript-eslint/utils': 5.62.0(eslint@8.57.0)(typescript@5.4.5)
+ debug: 4.3.4
+ eslint: 8.57.0
+ tsutils: 3.21.0(typescript@5.4.5)
+ typescript: 5.4.5
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /@typescript-eslint/types@5.62.0:
+ resolution: {integrity: sha512-87NVngcbVXUahrRTqIK27gD2t5Cu1yuCXxbLcFtCzZGlfyVWWh8mLHkoxzjsB6DDNnvdL+fW8MiwPEJyGJQDgQ==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ dev: true
+
+ /@typescript-eslint/typescript-estree@5.62.0(typescript@5.4.5):
+ resolution: {integrity: sha512-CmcQ6uY7b9y694lKdRB8FEel7JbU/40iSAPomu++SjLMntB+2Leay2LO6i8VnJk58MtE9/nQSFIH6jpyRWyYzA==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ peerDependencies:
+ typescript: '*'
+ peerDependenciesMeta:
+ typescript:
+ optional: true
+ dependencies:
+ '@typescript-eslint/types': 5.62.0
+ '@typescript-eslint/visitor-keys': 5.62.0
+ debug: 4.3.4
+ globby: 11.1.0
+ is-glob: 4.0.3
+ semver: 7.6.0
+ tsutils: 3.21.0(typescript@5.4.5)
+ typescript: 5.4.5
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /@typescript-eslint/utils@5.62.0(eslint@8.57.0)(typescript@5.4.5):
+ resolution: {integrity: sha512-n8oxjeb5aIbPFEtmQxQYOLI0i9n5ySBEY/ZEHHZqKQSFnxio1rv6dthascc9dLuwrL0RC5mPCxB7vnAVGAYWAQ==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ peerDependencies:
+ eslint: ^6.0.0 || ^7.0.0 || ^8.0.0
+ dependencies:
+ '@eslint-community/eslint-utils': 4.4.0(eslint@8.57.0)
+ '@types/json-schema': 7.0.15
+ '@types/semver': 7.5.8
+ '@typescript-eslint/scope-manager': 5.62.0
+ '@typescript-eslint/types': 5.62.0
+ '@typescript-eslint/typescript-estree': 5.62.0(typescript@5.4.5)
+ eslint: 8.57.0
+ eslint-scope: 5.1.1
+ semver: 7.6.0
+ transitivePeerDependencies:
+ - supports-color
+ - typescript
+ dev: true
+
+ /@typescript-eslint/visitor-keys@5.62.0:
+ resolution: {integrity: sha512-07ny+LHRzQXepkGg6w0mFY41fVUNBrL2Roj/++7V1txKugfjm/Ci/qSND03r2RhlJhJYMcTn9AhhSSqQp0Ysyw==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ dependencies:
+ '@typescript-eslint/types': 5.62.0
+ eslint-visitor-keys: 3.4.3
+ dev: true
+
+ /@ungap/structured-clone@1.2.0:
+ resolution: {integrity: sha512-zuVdFrMJiuCDQUMCzQaD6KL28MjnqqN8XnAqiEq9PNm/hCPTSGfrXCOfwj1ow4LFb/tNymJPwsNbVePc1xFqrQ==}
+ dev: true
+
+ /@vitejs/plugin-react@4.2.1(vite@4.5.3):
+ resolution: {integrity: sha512-oojO9IDc4nCUUi8qIR11KoQm0XFFLIwsRBwHRR4d/88IWghn1y6ckz/bJ8GHDCsYEJee8mDzqtJxh15/cisJNQ==}
+ engines: {node: ^14.18.0 || >=16.0.0}
+ peerDependencies:
+ vite: ^4.2.0 || ^5.0.0
+ dependencies:
+ '@babel/core': 7.24.4
+ '@babel/plugin-transform-react-jsx-self': 7.24.1(@babel/core@7.24.4)
+ '@babel/plugin-transform-react-jsx-source': 7.24.1(@babel/core@7.24.4)
+ '@types/babel__core': 7.20.5
+ react-refresh: 0.14.1
+ vite: 4.5.3(@types/node@20.12.7)(sass@1.75.0)(terser@5.30.4)
+ transitivePeerDependencies:
+ - supports-color
+ dev: false
+
+ /@webassemblyjs/ast@1.12.1:
+ resolution: {integrity: sha512-EKfMUOPRRUTy5UII4qJDGPpqfwjOmZ5jeGFwid9mnoqIFK+e0vqoi1qH56JpmZSzEL53jKnNzScdmftJyG5xWg==}
+ dependencies:
+ '@webassemblyjs/helper-numbers': 1.11.6
+ '@webassemblyjs/helper-wasm-bytecode': 1.11.6
+ dev: true
+
+ /@webassemblyjs/floating-point-hex-parser@1.11.6:
+ resolution: {integrity: sha512-ejAj9hfRJ2XMsNHk/v6Fu2dGS+i4UaXBXGemOfQ/JfQ6mdQg/WXtwleQRLLS4OvfDhv8rYnVwH27YJLMyYsxhw==}
+ dev: true
+
+ /@webassemblyjs/helper-api-error@1.11.6:
+ resolution: {integrity: sha512-o0YkoP4pVu4rN8aTJgAyj9hC2Sv5UlkzCHhxqWj8butaLvnpdc2jOwh4ewE6CX0txSfLn/UYaV/pheS2Txg//Q==}
+ dev: true
+
+ /@webassemblyjs/helper-buffer@1.12.1:
+ resolution: {integrity: sha512-nzJwQw99DNDKr9BVCOZcLuJJUlqkJh+kVzVl6Fmq/tI5ZtEyWT1KZMyOXltXLZJmDtvLCDgwsyrkohEtopTXCw==}
+ dev: true
+
+ /@webassemblyjs/helper-numbers@1.11.6:
+ resolution: {integrity: sha512-vUIhZ8LZoIWHBohiEObxVm6hwP034jwmc9kuq5GdHZH0wiLVLIPcMCdpJzG4C11cHoQ25TFIQj9kaVADVX7N3g==}
+ dependencies:
+ '@webassemblyjs/floating-point-hex-parser': 1.11.6
+ '@webassemblyjs/helper-api-error': 1.11.6
+ '@xtuc/long': 4.2.2
+ dev: true
+
+ /@webassemblyjs/helper-wasm-bytecode@1.11.6:
+ resolution: {integrity: sha512-sFFHKwcmBprO9e7Icf0+gddyWYDViL8bpPjJJl0WHxCdETktXdmtWLGVzoHbqUcY4Be1LkNfwTmXOJUFZYSJdA==}
+ dev: true
+
+ /@webassemblyjs/helper-wasm-section@1.12.1:
+ resolution: {integrity: sha512-Jif4vfB6FJlUlSbgEMHUyk1j234GTNG9dBJ4XJdOySoj518Xj0oGsNi59cUQF4RRMS9ouBUxDDdyBVfPTypa5g==}
+ dependencies:
+ '@webassemblyjs/ast': 1.12.1
+ '@webassemblyjs/helper-buffer': 1.12.1
+ '@webassemblyjs/helper-wasm-bytecode': 1.11.6
+ '@webassemblyjs/wasm-gen': 1.12.1
+ dev: true
+
+ /@webassemblyjs/ieee754@1.11.6:
+ resolution: {integrity: sha512-LM4p2csPNvbij6U1f19v6WR56QZ8JcHg3QIJTlSwzFcmx6WSORicYj6I63f9yU1kEUtrpG+kjkiIAkevHpDXrg==}
+ dependencies:
+ '@xtuc/ieee754': 1.2.0
+ dev: true
+
+ /@webassemblyjs/leb128@1.11.6:
+ resolution: {integrity: sha512-m7a0FhE67DQXgouf1tbN5XQcdWoNgaAuoULHIfGFIEVKA6tu/edls6XnIlkmS6FrXAquJRPni3ZZKjw6FSPjPQ==}
+ dependencies:
+ '@xtuc/long': 4.2.2
+ dev: true
+
+ /@webassemblyjs/utf8@1.11.6:
+ resolution: {integrity: sha512-vtXf2wTQ3+up9Zsg8sa2yWiQpzSsMyXj0qViVP6xKGCUT8p8YJ6HqI7l5eCnWx1T/FYdsv07HQs2wTFbbof/RA==}
+ dev: true
+
+ /@webassemblyjs/wasm-edit@1.12.1:
+ resolution: {integrity: sha512-1DuwbVvADvS5mGnXbE+c9NfA8QRcZ6iKquqjjmR10k6o+zzsRVesil54DKexiowcFCPdr/Q0qaMgB01+SQ1u6g==}
+ dependencies:
+ '@webassemblyjs/ast': 1.12.1
+ '@webassemblyjs/helper-buffer': 1.12.1
+ '@webassemblyjs/helper-wasm-bytecode': 1.11.6
+ '@webassemblyjs/helper-wasm-section': 1.12.1
+ '@webassemblyjs/wasm-gen': 1.12.1
+ '@webassemblyjs/wasm-opt': 1.12.1
+ '@webassemblyjs/wasm-parser': 1.12.1
+ '@webassemblyjs/wast-printer': 1.12.1
+ dev: true
+
+ /@webassemblyjs/wasm-gen@1.12.1:
+ resolution: {integrity: sha512-TDq4Ojh9fcohAw6OIMXqiIcTq5KUXTGRkVxbSo1hQnSy6lAM5GSdfwWeSxpAo0YzgsgF182E/U0mDNhuA0tW7w==}
+ dependencies:
+ '@webassemblyjs/ast': 1.12.1
+ '@webassemblyjs/helper-wasm-bytecode': 1.11.6
+ '@webassemblyjs/ieee754': 1.11.6
+ '@webassemblyjs/leb128': 1.11.6
+ '@webassemblyjs/utf8': 1.11.6
+ dev: true
+
+ /@webassemblyjs/wasm-opt@1.12.1:
+ resolution: {integrity: sha512-Jg99j/2gG2iaz3hijw857AVYekZe2SAskcqlWIZXjji5WStnOpVoat3gQfT/Q5tb2djnCjBtMocY/Su1GfxPBg==}
+ dependencies:
+ '@webassemblyjs/ast': 1.12.1
+ '@webassemblyjs/helper-buffer': 1.12.1
+ '@webassemblyjs/wasm-gen': 1.12.1
+ '@webassemblyjs/wasm-parser': 1.12.1
+ dev: true
+
+ /@webassemblyjs/wasm-parser@1.12.1:
+ resolution: {integrity: sha512-xikIi7c2FHXysxXe3COrVUPSheuBtpcfhbpFj4gmu7KRLYOzANztwUU0IbsqvMqzuNK2+glRGWCEqZo1WCLyAQ==}
+ dependencies:
+ '@webassemblyjs/ast': 1.12.1
+ '@webassemblyjs/helper-api-error': 1.11.6
+ '@webassemblyjs/helper-wasm-bytecode': 1.11.6
+ '@webassemblyjs/ieee754': 1.11.6
+ '@webassemblyjs/leb128': 1.11.6
+ '@webassemblyjs/utf8': 1.11.6
+ dev: true
+
+ /@webassemblyjs/wast-printer@1.12.1:
+ resolution: {integrity: sha512-+X4WAlOisVWQMikjbcvY2e0rwPsKQ9F688lksZhBcPycBBuii3O7m8FACbDMWDojpAqvjIncrG8J0XHKyQfVeA==}
+ dependencies:
+ '@webassemblyjs/ast': 1.12.1
+ '@xtuc/long': 4.2.2
+ dev: true
+
+ /@xobotyi/scrollbar-width@1.9.5:
+ resolution: {integrity: sha512-N8tkAACJx2ww8vFMneJmaAgmjAG1tnVBZJRLRcx061tmsLRZHSEZSLuGWnwPtunsSLvSqXQ2wfp7Mgqg1I+2dQ==}
+ dev: false
+
+ /@xtuc/ieee754@1.2.0:
+ resolution: {integrity: sha512-DX8nKgqcGwsc0eJSqYt5lwP4DH5FlHnmuWWBRy7X0NcaGR0ZtuyeESgMwTYVEtxmsNGY+qit4QYT/MIYTOTPeA==}
+ dev: true
+
+ /@xtuc/long@4.2.2:
+ resolution: {integrity: sha512-NuHqBY1PB/D8xU6s/thBgOAiAP7HOYDQ32+BFZILJ8ivkUkAHQnWfn6WhL79Owj1qmUnoN/YPhktdIoucipkAQ==}
+ dev: true
+
+ /@yr/monotone-cubic-spline@1.0.3:
+ resolution: {integrity: sha512-FQXkOta0XBSUPHndIKON2Y9JeQz5ZeMqLYZVVK93FliNBFm7LNMIZmY6FrMEB9XPcDbE2bekMbZD6kzDkxwYjA==}
+ dev: false
+
+ /JSONStream@1.3.5:
+ resolution: {integrity: sha512-E+iruNOY8VV9s4JEbe1aNEm6MiszPRr/UfcHMz0TQh1BXSxHK+ASV1R6W4HpjBhSeS+54PIsAMCBmwD06LLsqQ==}
+ hasBin: true
+ dependencies:
+ jsonparse: 1.3.1
+ through: 2.3.8
+ dev: true
+
+ /acorn-import-assertions@1.9.0(acorn@8.11.3):
+ resolution: {integrity: sha512-cmMwop9x+8KFhxvKrKfPYmN6/pKTYYHBqLa0DfvVZcKMJWNyWLnaqND7dx/qn66R7ewM1UX5XMaDVP5wlVTaVA==}
+ peerDependencies:
+ acorn: ^8
+ dependencies:
+ acorn: 8.11.3
+ dev: true
+
+ /acorn-jsx@5.3.2(acorn@8.11.3):
+ resolution: {integrity: sha512-rq9s+JNhf0IChjtDXxllJ7g41oZk5SlXtp0LHwyA5cejwn7vKmKp4pPri6YEePv2PU65sAsegbXtIinmDFDXgQ==}
+ peerDependencies:
+ acorn: ^6.0.0 || ^7.0.0 || ^8.0.0
+ dependencies:
+ acorn: 8.11.3
+ dev: true
+
+ /acorn-walk@8.3.2:
+ resolution: {integrity: sha512-cjkyv4OtNCIeqhHrfS81QWXoCBPExR/J62oyEqepVw8WaQeSqpW2uhuLPh1m9eWhDuOo/jUXVTlifvesOWp/4A==}
+ engines: {node: '>=0.4.0'}
+ dev: true
+
+ /acorn@8.11.3:
+ resolution: {integrity: sha512-Y9rRfJG5jcKOE0CLisYbojUjIrIEE7AGMzA/Sm4BslANhbS+cDMpgBdcPT91oJ7OuJ9hYJBx59RjbhxVnrF8Xg==}
+ engines: {node: '>=0.4.0'}
+ hasBin: true
+
+ /address@1.2.2:
+ resolution: {integrity: sha512-4B/qKCfeE/ODUaAUpSwfzazo5x29WD4r3vXiWsB7I2mSDAihwEqKO+g8GELZUQSSAo5e1XTYh3ZVfLyxBc12nA==}
+ engines: {node: '>= 10.0.0'}
+ dev: true
+
+ /ajv-keywords@3.5.2(ajv@6.12.6):
+ resolution: {integrity: sha512-5p6WTN0DdTGVQk6VjcEju19IgaHudalcfabD7yhDGeA6bcQnmL+CpveLJq/3hvfwd1aof6L386Ougkx6RfyMIQ==}
+ peerDependencies:
+ ajv: ^6.9.1
+ dependencies:
+ ajv: 6.12.6
+ dev: true
+
+ /ajv@6.12.6:
+ resolution: {integrity: sha512-j3fVLgvTo527anyYyJOGTYJbG+vnnQYvE0m5mmkc1TK+nxAppkCLMIL0aZ4dblVCNoGShhm+kzE4ZUykBoMg4g==}
+ dependencies:
+ fast-deep-equal: 3.1.3
+ fast-json-stable-stringify: 2.1.0
+ json-schema-traverse: 0.4.1
+ uri-js: 4.4.1
+ dev: true
+
+ /ajv@8.12.0:
+ resolution: {integrity: sha512-sRu1kpcO9yLtYxBKvqfTeh9KzZEwO3STyX1HT+4CaDzC6HpTGYhIhPIzj9XuKU7KYDwnaeh5hcOwjy1QuJzBPA==}
+ dependencies:
+ fast-deep-equal: 3.1.3
+ json-schema-traverse: 1.0.0
+ require-from-string: 2.0.2
+ uri-js: 4.4.1
+ dev: true
+
+ /ansi-escapes@5.0.0:
+ resolution: {integrity: sha512-5GFMVX8HqE/TB+FuBJGuO5XG0WrsA6ptUqoODaT/n9mmUaZFkqnBueB4leqGBCmrUHnCnC4PCZTCd0E7QQ83bA==}
+ engines: {node: '>=12'}
+ dependencies:
+ type-fest: 1.4.0
+ dev: true
+
+ /ansi-regex@2.1.1:
+ resolution: {integrity: sha512-TIGnTpdo+E3+pCyAluZvtED5p5wCqLdezCyhPZzKPcxvFplEt4i+W7OONCKgeZFT3+y5NZZfOOS/Bdcanm1MYA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /ansi-regex@4.1.1:
+ resolution: {integrity: sha512-ILlv4k/3f6vfQ4OoP2AGvirOktlQ98ZEL1k9FaQjxa3L1abBgbuTDAdPOpvbGncC0BTVQrl+OM8xZGK6tWXt7g==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /ansi-regex@5.0.1:
+ resolution: {integrity: sha512-quJQXlTSUGL2LH9SUXo8VwsY4soanhgo6LNSm84E1LBcE8s3O0wpdiRzyR9z/ZZJMlMWv37qOOb9pdJlMUEKFQ==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /ansi-regex@6.0.1:
+ resolution: {integrity: sha512-n5M855fKb2SsfMIiFFoVrABHJC8QtHwVx+mHWP3QcEqBHYienj5dHSgjbxtC0WEZXYt4wcD6zrQElDPhFuZgfA==}
+ engines: {node: '>=12'}
+ dev: true
+
+ /ansi-styles@2.2.1:
+ resolution: {integrity: sha512-kmCevFghRiWM7HB5zTPULl4r9bVFSWjz62MhqizDGUrq2NWuNMQyuv4tHHoKJHs69M/MF64lEcHdYIocrdWQYA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /ansi-styles@3.2.1:
+ resolution: {integrity: sha512-VT0ZI6kZRdTh8YyJw3SMbYm/u+NqfsAxEpWO0Pf9sq8/e94WxxOpPKx9FR1FlyCtOVDNOQ+8ntlqFxiRc+r5qA==}
+ engines: {node: '>=4'}
+ dependencies:
+ color-convert: 1.9.3
+
+ /ansi-styles@4.3.0:
+ resolution: {integrity: sha512-zbB9rCJAT1rbjiVDb2hqKFHNYLxgtk8NURxZ3IZwD3F6NtxbXZQCnnSi1Lkx+IDohdPlFp222wVALIheZJQSEg==}
+ engines: {node: '>=8'}
+ dependencies:
+ color-convert: 2.0.1
+ dev: true
+
+ /ansi-styles@6.2.1:
+ resolution: {integrity: sha512-bN798gFfQX+viw3R7yrGWRqnrN2oRkEkUjjl4JNn4E8GxxbjtG3FbrEIIY3l8/hrwUwIeCZvi4QuOTP4MErVug==}
+ engines: {node: '>=12'}
+ dev: true
+
+ /antd@5.16.4(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-H3LtVz5hiNgs0lL8U6pzi11rluR6RDRw1cm2pWX6CsvgZmybWsaTBV2h+d+zmgFfuch53TWs5uztLdAldIoYYw==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@ant-design/colors': 7.0.2
+ '@ant-design/cssinjs': 1.20.0(react-dom@18.3.0)(react@18.3.0)
+ '@ant-design/icons': 5.3.6(react-dom@18.3.0)(react@18.3.0)
+ '@ant-design/react-slick': 1.1.2(react@18.3.0)
+ '@babel/runtime': 7.24.4
+ '@ctrl/tinycolor': 3.6.1
+ '@rc-component/color-picker': 1.5.3(react-dom@18.3.0)(react@18.3.0)
+ '@rc-component/mutate-observer': 1.1.0(react-dom@18.3.0)(react@18.3.0)
+ '@rc-component/tour': 1.14.2(react-dom@18.3.0)(react@18.3.0)
+ '@rc-component/trigger': 2.1.1(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ copy-to-clipboard: 3.3.3
+ dayjs: 1.11.10
+ qrcode.react: 3.1.0(react@18.3.0)
+ rc-cascader: 3.24.1(react-dom@18.3.0)(react@18.3.0)
+ rc-checkbox: 3.2.0(react-dom@18.3.0)(react@18.3.0)
+ rc-collapse: 3.7.3(react-dom@18.3.0)(react@18.3.0)
+ rc-dialog: 9.4.0(react-dom@18.3.0)(react@18.3.0)
+ rc-drawer: 7.1.0(react-dom@18.3.0)(react@18.3.0)
+ rc-dropdown: 4.2.0(react-dom@18.3.0)(react@18.3.0)
+ rc-field-form: 1.44.0(react-dom@18.3.0)(react@18.3.0)
+ rc-image: 7.6.0(react-dom@18.3.0)(react@18.3.0)
+ rc-input: 1.4.5(react-dom@18.3.0)(react@18.3.0)
+ rc-input-number: 9.0.0(react-dom@18.3.0)(react@18.3.0)
+ rc-mentions: 2.11.1(react-dom@18.3.0)(react@18.3.0)
+ rc-menu: 9.13.0(react-dom@18.3.0)(react@18.3.0)
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-notification: 5.4.0(react-dom@18.3.0)(react@18.3.0)
+ rc-pagination: 4.0.4(react-dom@18.3.0)(react@18.3.0)
+ rc-picker: 4.4.2(dayjs@1.11.10)(react-dom@18.3.0)(react@18.3.0)
+ rc-progress: 4.0.0(react-dom@18.3.0)(react@18.3.0)
+ rc-rate: 2.12.0(react-dom@18.3.0)(react@18.3.0)
+ rc-resize-observer: 1.4.0(react-dom@18.3.0)(react@18.3.0)
+ rc-segmented: 2.3.0(react-dom@18.3.0)(react@18.3.0)
+ rc-select: 14.13.1(react-dom@18.3.0)(react@18.3.0)
+ rc-slider: 10.6.2(react-dom@18.3.0)(react@18.3.0)
+ rc-steps: 6.0.1(react-dom@18.3.0)(react@18.3.0)
+ rc-switch: 4.1.0(react-dom@18.3.0)(react@18.3.0)
+ rc-table: 7.45.4(react-dom@18.3.0)(react@18.3.0)
+ rc-tabs: 14.1.1(react-dom@18.3.0)(react@18.3.0)
+ rc-textarea: 1.6.3(react-dom@18.3.0)(react@18.3.0)
+ rc-tooltip: 6.2.0(react-dom@18.3.0)(react@18.3.0)
+ rc-tree: 5.8.5(react-dom@18.3.0)(react@18.3.0)
+ rc-tree-select: 5.19.0(react-dom@18.3.0)(react@18.3.0)
+ rc-upload: 4.5.2(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ scroll-into-view-if-needed: 3.1.0
+ throttle-debounce: 5.0.0
+ transitivePeerDependencies:
+ - date-fns
+ - luxon
+ - moment
+ dev: false
+
+ /any-promise@1.3.0:
+ resolution: {integrity: sha512-7UvmKalWRt1wgjL1RrGxoSJW/0QZFIegpeGvZG9kjp8vrRu55XTHbwnqq2GpXm9uLbcuhxm3IqX9OB4MZR1b2A==}
+ dev: true
+
+ /anymatch@3.1.3:
+ resolution: {integrity: sha512-KMReFUr0B4t+D+OBkjR3KYqvocp2XaSzO55UcB6mgQMd3KbcE+mWTyvVV7D/zsdEbNnV6acZUutkiHQXvTr1Rw==}
+ engines: {node: '>= 8'}
+ dependencies:
+ normalize-path: 3.0.0
+ picomatch: 2.3.1
+
+ /apexcharts@3.49.0:
+ resolution: {integrity: sha512-2T9HnbQFLCuYRPndQLmh+bEQFoz0meUbvASaGgiSKDuYhWcLBodJtIpKql2aOtMx4B/sHrWW0dm90HsW4+h2PQ==}
+ dependencies:
+ '@yr/monotone-cubic-spline': 1.0.3
+ svg.draggable.js: 2.2.2
+ svg.easing.js: 2.0.0
+ svg.filter.js: 2.0.2
+ svg.pathmorphing.js: 0.1.3
+ svg.resize.js: 1.4.3
+ svg.select.js: 3.0.1
+ dev: false
+
+ /arg@4.1.3:
+ resolution: {integrity: sha512-58S9QDqG0Xx27YwPSt9fJxivjYl432YCwfDMfZ+71RAqUrZef7LrKQZ3LHLOwCS4FLNBplP533Zx895SeOCHvA==}
+ dev: true
+
+ /arg@5.0.2:
+ resolution: {integrity: sha512-PYjyFOLKQ9y57JvQ6QLo8dAgNqswh8M1RMJYdQduT6xbWSgK36P/Z/v+p888pM69jMMfS8Xd8F6I1kQ/I9HUGg==}
+ dev: true
+
+ /argparse@1.0.10:
+ resolution: {integrity: sha512-o5Roy6tNG4SL/FOkCAN6RzjiakZS25RLYFrcMttJqbdd8BWrnA+fGz57iN5Pb06pvBGvl5gQ0B48dJlslXvoTg==}
+ dependencies:
+ sprintf-js: 1.0.3
+ dev: true
+
+ /argparse@2.0.1:
+ resolution: {integrity: sha512-8+9WqebbFzpX9OR+Wa6O29asIogeRMzcGtAINdpMHHyAg10f05aSFVBbcEqGf/PXw1EjAZ+q2/bEBg3DvurK3Q==}
+ dev: true
+
+ /aria-query@5.3.0:
+ resolution: {integrity: sha512-b0P0sZPKtyu8HkeRAfCq0IfURZK+SuwMjY1UXGBU27wpAiTwQAIlq56IbIO+ytk/JjS1fMR14ee5WBBfKi5J6A==}
+ dependencies:
+ dequal: 2.0.3
+ dev: true
+
+ /arr-diff@4.0.0:
+ resolution: {integrity: sha512-YVIQ82gZPGBebQV/a8dar4AitzCQs0jjXwMPZllpXMaGjXPYVUawSxQrRsjhjupyVxEvbHgUmIhKVlND+j02kA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /arr-flatten@1.1.0:
+ resolution: {integrity: sha512-L3hKV5R/p5o81R7O02IGnwpDmkp6E982XhtbuwSe3O4qOtMMMtodicASA1Cny2U+aCXcNpml+m4dPsvsJ3jatg==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /arr-union@3.1.0:
+ resolution: {integrity: sha512-sKpyeERZ02v1FeCZT8lrfJq5u6goHCtpTAzPwJYe7c8SPFOboNjNg1vz2L4VTn9T4PQxEx13TbXLmYUcS6Ug7Q==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /array-buffer-byte-length@1.0.1:
+ resolution: {integrity: sha512-ahC5W1xgou+KTXix4sAO8Ki12Q+jf4i0+tmk3sC+zgcynshkHxzpXdImBehiUYKKKDwvfFiJl1tZt6ewscS1Mg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ is-array-buffer: 3.0.4
+ dev: true
+
+ /array-find-index@1.0.2:
+ resolution: {integrity: sha512-M1HQyIXcBGtVywBt8WVdim+lrNaK7VHp99Qt5pSNziXznKHViIBbXWtfRTpEFpF/c4FdfxNAsCCwPp5phBYJtw==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /array-ify@1.0.0:
+ resolution: {integrity: sha512-c5AMf34bKdvPhQ7tBGhqkgKNUzMr4WUs+WDtC2ZUGOUncbxKMTvqxYctiseW3+L4bA8ec+GcZ6/A/FW4m8ukng==}
+ dev: true
+
+ /array-includes@3.1.8:
+ resolution: {integrity: sha512-itaWrbYbqpGXkGhZPGUulwnhVf5Hpy1xiCFsGqyIGglbBxmG5vSjxQen3/WGOjPpNEv1RtBLKxbmVXm8HpJStQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-object-atoms: 1.0.0
+ get-intrinsic: 1.2.4
+ is-string: 1.0.7
+ dev: true
+
+ /array-tree-filter@2.1.0:
+ resolution: {integrity: sha512-4ROwICNlNw/Hqa9v+rk5h22KjmzB1JGTMVKP2AKJBOCgb0yL0ASf0+YvCcLNNwquOHNX48jkeZIJ3a+oOQqKcw==}
+ dev: false
+
+ /array-union@1.0.2:
+ resolution: {integrity: sha512-Dxr6QJj/RdU/hCaBjOfxW+q6lyuVE6JFWIrAUpuOOhoJJoQ99cUn3igRaHVB5P9WrgFVN0FfArM3x0cueOU8ng==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ array-uniq: 1.0.3
+ dev: true
+
+ /array-union@2.1.0:
+ resolution: {integrity: sha512-HGyxoOTYUyCM6stUe6EJgnd4EoewAI7zMdfqO+kGjnlZmBDz/cR5pf8r/cR4Wq60sL/p0IkcjUEEPwS3GFrIyw==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /array-uniq@1.0.3:
+ resolution: {integrity: sha512-MNha4BWQ6JbwhFhj03YK552f7cb3AzoE8SzeljgChvL1dl3IcvggXVz1DilzySZkCja+CXuZbdW7yATchWn8/Q==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /array-unique@0.3.2:
+ resolution: {integrity: sha512-SleRWjh9JUud2wH1hPs9rZBZ33H6T9HOiL0uwGnGx9FpE6wKGyfWugmbkEOIs6qWrZhg0LWeLziLrEwQJhs5mQ==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /array.prototype.findlast@1.2.5:
+ resolution: {integrity: sha512-CVvd6FHg1Z3POpBLxO6E6zr+rSKEQ9L6rZHAaY7lLfhKsWYUBBOuMs0e9o24oopj6H+geRCX0YJ+TJLBK2eHyQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-errors: 1.3.0
+ es-object-atoms: 1.0.0
+ es-shim-unscopables: 1.0.2
+ dev: true
+
+ /array.prototype.findlastindex@1.2.5:
+ resolution: {integrity: sha512-zfETvRFA8o7EiNn++N5f/kaCw221hrpGsDmcpndVupkPzEc1Wuf3VgC0qby1BbHs7f5DVYjgtEU2LLh5bqeGfQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-errors: 1.3.0
+ es-object-atoms: 1.0.0
+ es-shim-unscopables: 1.0.2
+ dev: true
+
+ /array.prototype.flat@1.3.2:
+ resolution: {integrity: sha512-djYB+Zx2vLewY8RWlNCUdHjDXs2XOgm602S9E7P/UpHgfeHL00cRiIF+IN/G/aUJ7kGPb6yO/ErDI5V2s8iycA==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-shim-unscopables: 1.0.2
+ dev: true
+
+ /array.prototype.flatmap@1.3.2:
+ resolution: {integrity: sha512-Ewyx0c9PmpcsByhSW4r+9zDU7sGjFc86qf/kKtuSCRdhfbk0SNLLkaT5qvcHnRGgc5NP/ly/y+qkXkqONX54CQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-shim-unscopables: 1.0.2
+ dev: true
+
+ /array.prototype.toreversed@1.1.2:
+ resolution: {integrity: sha512-wwDCoT4Ck4Cz7sLtgUmzR5UV3YF5mFHUlbChCzZBQZ+0m2cl/DH3tKgvphv1nKgFsJ48oCSg6p91q2Vm0I/ZMA==}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-shim-unscopables: 1.0.2
+ dev: true
+
+ /array.prototype.tosorted@1.1.3:
+ resolution: {integrity: sha512-/DdH4TiTmOKzyQbp/eadcCVexiCb36xJg7HshYOYJnNZFDj33GEv0P7GxsynpShhq4OLYJzbGcBDkLsDt7MnNg==}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-errors: 1.3.0
+ es-shim-unscopables: 1.0.2
+ dev: true
+
+ /arraybuffer.prototype.slice@1.0.3:
+ resolution: {integrity: sha512-bMxMKAjg13EBSVscxTaYA4mRc5t1UAXa2kXiGTNfZ079HIWXEkKmkgFrh/nJqamaLSrXO5H4WFFkPEaLJWbs3A==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ array-buffer-byte-length: 1.0.1
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-errors: 1.3.0
+ get-intrinsic: 1.2.4
+ is-array-buffer: 3.0.4
+ is-shared-array-buffer: 1.0.3
+ dev: true
+
+ /arrify@1.0.1:
+ resolution: {integrity: sha512-3CYzex9M9FGQjCGMGyi6/31c8GJbgb0qGyrx5HWxPd0aCwh4cB2YjMb2Xf9UuoogrMrlO9cTqnB5rI5GHZTcUA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /assign-symbols@1.0.0:
+ resolution: {integrity: sha512-Q+JC7Whu8HhmTdBph/Tq59IoRtoy6KAm5zzPv00WdujX82lbAL8K7WVjne7vdCsAmbF4AYaDOPyO3k0kl8qIrw==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /ast-types-flow@0.0.8:
+ resolution: {integrity: sha512-OH/2E5Fg20h2aPrbe+QL8JZQFko0YZaF+j4mnQ7BGhfavO7OpSLa8a0y9sBwomHdSbkhTS8TQNayBfnW5DwbvQ==}
+ dev: true
+
+ /astral-regex@1.0.0:
+ resolution: {integrity: sha512-+Ryf6g3BKoRc7jfp7ad8tM4TtMiaWvbF/1/sQcZPkkS7ag3D5nMBCe2UfOTONtAkaG0tO0ij3C5Lwmf1EiyjHg==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /astral-regex@2.0.0:
+ resolution: {integrity: sha512-Z7tMw1ytTXt5jqMcOP+OQteU1VuNK9Y02uuJtKQ1Sv69jXQKKg5cibLwGJow8yzZP+eAc18EmLGPal0bp36rvQ==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /async-validator@4.2.5:
+ resolution: {integrity: sha512-7HhHjtERjqlNbZtqNqy2rckN/SpOOlmDliet+lP7k+eKZEjPk3DgyeU9lIXLdeLz0uBbbVp+9Qdow9wJWgwwfg==}
+ dev: false
+
+ /asynckit@0.4.0:
+ resolution: {integrity: sha512-Oei9OH4tRh0YqU3GxhX79dM/mwVgvbZJaSNaRk+bshkj0S5cfHcgYakreBjrHwatXKbz+IoIdYLxrKim2MjW0Q==}
+ dev: false
+
+ /at-least-node@1.0.0:
+ resolution: {integrity: sha512-+q/t7Ekv1EDY2l6Gda6LLiX14rU9TV20Wa3ofeQmwPFZbOMo9DXrLbOjFaaclkXKWidIaopwAObQDqwWtGUjqg==}
+ engines: {node: '>= 4.0.0'}
+ dev: true
+
+ /atob@2.1.2:
+ resolution: {integrity: sha512-Wm6ukoaOGJi/73p/cl2GvLjTI5JM1k/O14isD73YML8StrH/7/lRFgmg8nICZgD3bZZvjwCGxtMOD3wWNAu8cg==}
+ engines: {node: '>= 4.5.0'}
+ hasBin: true
+ dev: true
+
+ /autoprefixer@10.4.19(postcss@8.4.38):
+ resolution: {integrity: sha512-BaENR2+zBZ8xXhM4pUaKUxlVdxZ0EZhjvbopwnXmxRUfqDmwSpC2lAi/QXvx7NRdPCo1WKEcEF6mV64si1z4Ew==}
+ engines: {node: ^10 || ^12 || >=14}
+ hasBin: true
+ peerDependencies:
+ postcss: ^8.1.0
+ dependencies:
+ browserslist: 4.23.0
+ caniuse-lite: 1.0.30001612
+ fraction.js: 4.3.7
+ normalize-range: 0.1.2
+ picocolors: 1.0.0
+ postcss: 8.4.38
+ postcss-value-parser: 4.2.0
+ dev: true
+
+ /autoprefixer@9.8.8:
+ resolution: {integrity: sha512-eM9d/swFopRt5gdJ7jrpCwgvEMIayITpojhkkSMRsFHYuH5bkSQ4p/9qTEHtmNudUZh22Tehu7I6CxAW0IXTKA==}
+ hasBin: true
+ dependencies:
+ browserslist: 4.23.0
+ caniuse-lite: 1.0.30001612
+ normalize-range: 0.1.2
+ num2fraction: 1.2.2
+ picocolors: 0.2.1
+ postcss: 7.0.39
+ postcss-value-parser: 4.2.0
+ dev: true
+
+ /autosuggest-highlight@3.3.4:
+ resolution: {integrity: sha512-j6RETBD2xYnrVcoV1S5R4t3WxOlWZKyDQjkwnggDPSjF5L4jV98ZltBpvPvbkM1HtoSe5o+bNrTHyjPbieGeYA==}
+ dependencies:
+ remove-accents: 0.4.4
+ dev: false
+
+ /available-typed-arrays@1.0.7:
+ resolution: {integrity: sha512-wvUjBtSGN7+7SjNpq/9M2Tg350UZD3q62IFZLbRAR1bSMlCo1ZaeW+BJ+D090e4hIIZLBcTDWe4Mh4jvUDajzQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ possible-typed-array-names: 1.0.0
+ dev: true
+
+ /axe-core@4.7.0:
+ resolution: {integrity: sha512-M0JtH+hlOL5pLQwHOLNYZaXuhqmvS8oExsqB1SBYgA4Dk7u/xx+YdGHXaK5pyUfed5mYXdlYiphWq3G8cRi5JQ==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /axios@1.6.8:
+ resolution: {integrity: sha512-v/ZHtJDU39mDpyBoFVkETcd/uNdxrWRrg3bKpOKzXFA6Bvqopts6ALSMU3y6ijYxbw2B+wPrIv46egTzJXCLGQ==}
+ dependencies:
+ follow-redirects: 1.15.6
+ form-data: 4.0.0
+ proxy-from-env: 1.1.0
+ transitivePeerDependencies:
+ - debug
+ dev: false
+
+ /axobject-query@3.2.1:
+ resolution: {integrity: sha512-jsyHu61e6N4Vbz/v18DHwWYKK0bSWLqn47eeDSKPB7m8tqMHF9YJ+mhIk2lVteyZrY8tnSj/jHOv4YiTCuCJgg==}
+ dependencies:
+ dequal: 2.0.3
+ dev: true
+
+ /babel-plugin-macros@3.1.0:
+ resolution: {integrity: sha512-Cg7TFGpIr01vOQNODXOOaGz2NpCU5gl8x1qJFbb6hbZxR7XrcE2vtbAsTAbJ7/xwJtUuJEw8K8Zr/AE0LHlesg==}
+ engines: {node: '>=10', npm: '>=6'}
+ dependencies:
+ '@babel/runtime': 7.24.4
+ cosmiconfig: 7.1.0
+ resolve: 1.22.8
+ dev: false
+
+ /bail@1.0.5:
+ resolution: {integrity: sha512-xFbRxM1tahm08yHBP16MMjVUAvDaBMD38zsM9EMAUN61omwLmKlOpB/Zku5QkjZ8TZ4vn53pj+t518cH0S03RQ==}
+ dev: true
+
+ /bail@2.0.2:
+ resolution: {integrity: sha512-0xO6mYd7JB2YesxDKplafRpsiOzPt9V02ddPCLbY1xYGPOX24NTyN50qnUxgCPcSoYMhKpAuBTjQoRZCAkUDRw==}
+ dev: false
+
+ /balanced-match@1.0.2:
+ resolution: {integrity: sha512-3oSeUO0TMV67hN1AmbXsK4yaqU7tjiHlbxRDZOpH0KW9+CeX4bRAaX0Anxt0tx2MrpRpWwQaPwIlISEJhYU5Pw==}
+ dev: true
+
+ /balanced-match@2.0.0:
+ resolution: {integrity: sha512-1ugUSr8BHXRnK23KfuYS+gVMC3LB8QGH9W1iGtDPsNWoQbgtXSExkBu2aDR4epiGWZOjZsj6lDl/N/AqqTC3UA==}
+ dev: true
+
+ /base@0.11.2:
+ resolution: {integrity: sha512-5T6P4xPgpp0YDFvSWwEZ4NoE3aM4QBQXDzmVbraCkFj8zHM+mba8SyqB5DbZWyR7mYHo6Y7BdQo3MoA4m0TeQg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ cache-base: 1.0.1
+ class-utils: 0.3.6
+ component-emitter: 1.3.1
+ define-property: 1.0.0
+ isobject: 3.0.1
+ mixin-deep: 1.3.2
+ pascalcase: 0.1.1
+ dev: true
+
+ /big.js@5.2.2:
+ resolution: {integrity: sha512-vyL2OymJxmarO8gxMr0mhChsO9QGwhynfuu4+MHTAW6czfq9humCB7rKpUjDd9YUiDPU4mzpyupFSvOClAwbmQ==}
+ dev: true
+
+ /binary-extensions@2.3.0:
+ resolution: {integrity: sha512-Ceh+7ox5qe7LJuLHoY0feh3pHuUDHAcRUeyL2VYghZwfpkNIy/+8Ocg0a3UuSoYzavmylwuLWQOf3hl0jjMMIw==}
+ engines: {node: '>=8'}
+
+ /bluebird@3.7.2:
+ resolution: {integrity: sha512-XpNj6GDQzdfW+r2Wnn7xiSAd7TM3jzkxGXBGTtWKuSXv1xUV+azxAm8jdWZN06QTQk+2N2XB9jRDkvbmQmcRtg==}
+ dev: true
+
+ /boolbase@1.0.0:
+ resolution: {integrity: sha512-JZOSA7Mo9sNGB8+UjSgzdLtokWAky1zbztM3WRLCbZ70/3cTANmQmOdR7y2g+J0e2WXywy1yS468tY+IruqEww==}
+ dev: true
+
+ /brace-expansion@1.1.11:
+ resolution: {integrity: sha512-iCuPHDFgrHX7H2vEI/5xpz07zSHB00TpugqhmYtVmMO6518mCuRMoOYFldEBl0g187ufozdaHgWKcYFb61qGiA==}
+ dependencies:
+ balanced-match: 1.0.2
+ concat-map: 0.0.1
+ dev: true
+
+ /brace-expansion@2.0.1:
+ resolution: {integrity: sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==}
+ dependencies:
+ balanced-match: 1.0.2
+ dev: true
+
+ /braces@2.3.2:
+ resolution: {integrity: sha512-aNdbnj9P8PjdXU4ybaWLK2IF3jc/EoDYbC7AazW6to3TRsfXxscC9UXOB5iDiEQrkyIbWp2SLQda4+QAa7nc3w==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ arr-flatten: 1.1.0
+ array-unique: 0.3.2
+ extend-shallow: 2.0.1
+ fill-range: 4.0.0
+ isobject: 3.0.1
+ repeat-element: 1.1.4
+ snapdragon: 0.8.2
+ snapdragon-node: 2.1.1
+ split-string: 3.1.0
+ to-regex: 3.0.2
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /braces@3.0.2:
+ resolution: {integrity: sha512-b8um+L1RzM3WDSzvhm6gIz1yfTbBt6YTlcEKAvsmqCZZFw46z626lVj9j1yEPW33H5H+lBQpZMP1k8l+78Ha0A==}
+ engines: {node: '>=8'}
+ dependencies:
+ fill-range: 7.0.1
+
+ /browserslist@4.23.0:
+ resolution: {integrity: sha512-QW8HiM1shhT2GuzkvklfjcKDiWFXHOeFCIA/huJPwHsslwcydgk7X+z2zXpEijP98UCY7HbubZt5J2Zgvf0CaQ==}
+ engines: {node: ^6 || ^7 || ^8 || ^9 || ^10 || ^11 || ^12 || >=13.7}
+ hasBin: true
+ dependencies:
+ caniuse-lite: 1.0.30001612
+ electron-to-chromium: 1.4.749
+ node-releases: 2.0.14
+ update-browserslist-db: 1.0.13(browserslist@4.23.0)
+
+ /buffer-from@1.1.2:
+ resolution: {integrity: sha512-E+XQCRwSbaaiChtv6k6Dwgc+bx+Bs6vuKJHHl5kox/BaKbhiXzqQOwK4cO22yElGp2OCmjwVhT3HmxgyPGnJfQ==}
+
+ /cache-base@1.0.1:
+ resolution: {integrity: sha512-AKcdTnFSWATd5/GCPRxr2ChwIJ85CeyrEyjRHlKxQ56d4XJMGym0uAiKn0xbLOGOl3+yRpOTi484dVCEc5AUzQ==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ collection-visit: 1.0.0
+ component-emitter: 1.3.1
+ get-value: 2.0.6
+ has-value: 1.0.0
+ isobject: 3.0.1
+ set-value: 2.0.1
+ to-object-path: 0.3.0
+ union-value: 1.0.1
+ unset-value: 1.0.0
+ dev: true
+
+ /call-bind@1.0.7:
+ resolution: {integrity: sha512-GHTSNSYICQ7scH7sZ+M2rFopRoLh8t2bLSW6BbgrtLsahOIB5iyAVJf9GjWK3cYTDaMj4XdBpM1cA6pIS0Kv2w==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ es-define-property: 1.0.0
+ es-errors: 1.3.0
+ function-bind: 1.1.2
+ get-intrinsic: 1.2.4
+ set-function-length: 1.2.2
+
+ /call-me-maybe@1.0.2:
+ resolution: {integrity: sha512-HpX65o1Hnr9HH25ojC1YGs7HCQLq0GCOibSaWER0eNpgJ/Z1MZv2mTc7+xh6WOPxbRVcmgbv4hGU+uSQ/2xFZQ==}
+ dev: true
+
+ /caller-callsite@2.0.0:
+ resolution: {integrity: sha512-JuG3qI4QOftFsZyOn1qq87fq5grLIyk1JYd5lJmdA+fG7aQ9pA/i3JIJGcO3q0MrRcHlOt1U+ZeHW8Dq9axALQ==}
+ engines: {node: '>=4'}
+ dependencies:
+ callsites: 2.0.0
+ dev: true
+
+ /caller-path@2.0.0:
+ resolution: {integrity: sha512-MCL3sf6nCSXOwCTzvPKhN18TU7AHTvdtam8DAogxcrJ8Rjfbbg7Lgng64H9Iy+vUV6VGFClN/TyxBkAebLRR4A==}
+ engines: {node: '>=4'}
+ dependencies:
+ caller-callsite: 2.0.0
+ dev: true
+
+ /callsites@2.0.0:
+ resolution: {integrity: sha512-ksWePWBloaWPxJYQ8TL0JHvtci6G5QTKwQ95RcWAa/lzoAKuAOflGdAK92hpHXjkwb8zLxoLNUoNYZgVsaJzvQ==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /callsites@3.1.0:
+ resolution: {integrity: sha512-P8BjAsXvZS+VIDUI11hHCQEv74YT67YUi5JJFNWIqL235sBmjX4+qx9Muvls5ivyNENctx46xQLQ3aTuE7ssaQ==}
+ engines: {node: '>=6'}
+
+ /camelcase-css@2.0.1:
+ resolution: {integrity: sha512-QOSvevhslijgYwRx6Rv7zKdMF8lbRmx+uQGx2+vDc+KI/eBnsy9kit5aj23AgGu3pa4t9AgwbnXWqS+iOY+2aA==}
+ engines: {node: '>= 6'}
+ dev: true
+
+ /camelcase-keys@4.2.0:
+ resolution: {integrity: sha512-Ej37YKYbFUI8QiYlvj9YHb6/Z60dZyPJW0Cs8sFilMbd2lP0bw3ylAq9yJkK4lcTA2dID5fG8LjmJYbO7kWb7Q==}
+ engines: {node: '>=4'}
+ dependencies:
+ camelcase: 4.1.0
+ map-obj: 2.0.0
+ quick-lru: 1.1.0
+ dev: true
+
+ /camelcase-keys@6.2.2:
+ resolution: {integrity: sha512-YrwaA0vEKazPBkn0ipTiMpSajYDSe+KjQfrjhcBMxJt/znbvlHd8Pw/Vamaz5EB4Wfhs3SUR3Z9mwRu/P3s3Yg==}
+ engines: {node: '>=8'}
+ dependencies:
+ camelcase: 5.3.1
+ map-obj: 4.3.0
+ quick-lru: 4.0.1
+ dev: true
+
+ /camelcase-keys@7.0.2:
+ resolution: {integrity: sha512-Rjs1H+A9R+Ig+4E/9oyB66UC5Mj9Xq3N//vcLf2WzgdTi/3gUu3Z9KoqmlrEG4VuuLK8wJHofxzdQXz/knhiYg==}
+ engines: {node: '>=12'}
+ dependencies:
+ camelcase: 6.3.0
+ map-obj: 4.3.0
+ quick-lru: 5.1.1
+ type-fest: 1.4.0
+ dev: true
+
+ /camelcase@4.1.0:
+ resolution: {integrity: sha512-FxAv7HpHrXbh3aPo4o2qxHay2lkLY3x5Mw3KeE4KQE8ysVfziWeRZDwcjauvwBSGEC/nXUPzZy8zeh4HokqOnw==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /camelcase@5.3.1:
+ resolution: {integrity: sha512-L28STB170nwWS63UjtlEOE3dldQApaJXZkOI1uMFfzf3rRuPegHaHesyee+YxQ+W6SvRDQV6UrdOdRiR153wJg==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /camelcase@6.3.0:
+ resolution: {integrity: sha512-Gmy6FhYlCY7uOElZUSbxo2UCDH8owEk996gkbrpsgGtrJLM3J7jGxl9Ic7Qwwj4ivOE5AWZWRMecDdF7hqGjFA==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /camelize@1.0.1:
+ resolution: {integrity: sha512-dU+Tx2fsypxTgtLoE36npi3UqcjSSMNYfkqgmoEhtZrraP5VWq0K7FkWVTYa8eMPtnU/G2txVsfdCJTn9uzpuQ==}
+ dev: false
+
+ /can-use-dom@0.1.0:
+ resolution: {integrity: sha512-ceOhN1DL7Y4O6M0j9ICgmTYziV89WMd96SvSl0REd8PMgrY0B/WBOPoed5S1KUmJqXgUXh8gzSe6E3ae27upsQ==}
+ dev: false
+
+ /caniuse-lite@1.0.30001612:
+ resolution: {integrity: sha512-lFgnZ07UhaCcsSZgWW0K5j4e69dK1u/ltrL9lTUiFOwNHs12S3UMIEYgBV0Z6C6hRDev7iRnMzzYmKabYdXF9g==}
+
+ /ccount@1.1.0:
+ resolution: {integrity: sha512-vlNK021QdI7PNeiUh/lKkC/mNHHfV0m/Ad5JoI0TYtlBnJAslM/JIkm/tGC88bkLIwO6OQ5uV6ztS6kVAtCDlg==}
+ dev: true
+
+ /ccount@2.0.1:
+ resolution: {integrity: sha512-eyrF0jiFpY+3drT6383f1qhkbGsLSifNAjA61IUjZjmLCWjItY6LB9ft9YhoDgwfmclB2zhu51Lc7+95b8NRAg==}
+ dev: false
+
+ /chalk@1.1.3:
+ resolution: {integrity: sha512-U3lRVLMSlsCfjqYPbLyVv11M9CPW4I728d6TCKMAOJueEeB9/8o+eSsMnxPJD+Q+K909sdESg7C+tIkoH6on1A==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ ansi-styles: 2.2.1
+ escape-string-regexp: 1.0.5
+ has-ansi: 2.0.0
+ strip-ansi: 3.0.1
+ supports-color: 2.0.0
+ dev: true
+
+ /chalk@2.4.2:
+ resolution: {integrity: sha512-Mti+f9lpJNcwF4tWV8/OrTTtF1gZi+f8FqlyAdouralcFWFQWF2+NgCHShjkCb+IFBLq9buZwE1xckQU4peSuQ==}
+ engines: {node: '>=4'}
+ dependencies:
+ ansi-styles: 3.2.1
+ escape-string-regexp: 1.0.5
+ supports-color: 5.5.0
+
+ /chalk@4.1.2:
+ resolution: {integrity: sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==}
+ engines: {node: '>=10'}
+ dependencies:
+ ansi-styles: 4.3.0
+ supports-color: 7.2.0
+ dev: true
+
+ /chalk@5.3.0:
+ resolution: {integrity: sha512-dLitG79d+GV1Nb/VYcCDFivJeK1hiukt9QjRNVOsUtTy1rR1YJsmpGGTZ3qJos+uw7WmWF4wUwBd9jxjocFC2w==}
+ engines: {node: ^12.17.0 || ^14.13 || >=16.0.0}
+ dev: true
+
+ /character-entities-html4@1.1.4:
+ resolution: {integrity: sha512-HRcDxZuZqMx3/a+qrzxdBKBPUpxWEq9xw2OPZ3a/174ihfrQKVsFhqtthBInFy1zZ9GgZyFXOatNujm8M+El3g==}
+ dev: true
+
+ /character-entities-legacy@1.1.4:
+ resolution: {integrity: sha512-3Xnr+7ZFS1uxeiUDvV02wQ+QDbc55o97tIV5zHScSPJpcLm/r0DFPcoY3tYRp+VZukxuMeKgXYmsXQHO05zQeA==}
+ dev: true
+
+ /character-entities@1.2.4:
+ resolution: {integrity: sha512-iBMyeEHxfVnIakwOuDXpVkc54HijNgCyQB2w0VfGQThle6NXn50zU6V/u+LDhxHcDUPojn6Kpga3PTAD8W1bQw==}
+ dev: true
+
+ /character-entities@2.0.2:
+ resolution: {integrity: sha512-shx7oQ0Awen/BRIdkjkvz54PnEEI/EjwXDSIZp86/KKdbafHh1Df/RYGBhn4hbe2+uKC9FnT5UCEdyPz3ai9hQ==}
+ dev: false
+
+ /character-reference-invalid@1.1.4:
+ resolution: {integrity: sha512-mKKUkUbhPpQlCOfIuZkvSEgktjPFIsZKRRbC6KWVEMvlzblj3i3asQv5ODsrwt0N3pHAEvjP8KTQPHkp0+6jOg==}
+ dev: true
+
+ /chokidar@3.6.0:
+ resolution: {integrity: sha512-7VT13fmjotKpGipCW9JEQAusEPE+Ei8nl6/g4FBAmIm0GOOLMua9NDDo/DWp0ZAxCr3cPq5ZpBqmPAQgDda2Pw==}
+ engines: {node: '>= 8.10.0'}
+ dependencies:
+ anymatch: 3.1.3
+ braces: 3.0.2
+ glob-parent: 5.1.2
+ is-binary-path: 2.1.0
+ is-glob: 4.0.3
+ normalize-path: 3.0.0
+ readdirp: 3.6.0
+ optionalDependencies:
+ fsevents: 2.3.3
+
+ /chrome-trace-event@1.0.3:
+ resolution: {integrity: sha512-p3KULyQg4S7NIHixdwbGX+nFHkoBiA4YQmyWtjb8XngSKV124nJmRysgAeujbUVb15vh+RvFUfCPqU7rXk+hZg==}
+ engines: {node: '>=6.0'}
+ dev: true
+
+ /class-utils@0.3.6:
+ resolution: {integrity: sha512-qOhPa/Fj7s6TY8H8esGu5QNpMMQxz79h+urzrNYN6mn+9BnxlDGf5QZ+XeCDsxSjPqsSR56XOZOJmpeurnLMeg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ arr-union: 3.1.0
+ define-property: 0.2.5
+ isobject: 3.0.1
+ static-extend: 0.1.2
+ dev: true
+
+ /classnames@2.5.1:
+ resolution: {integrity: sha512-saHYOzhIQs6wy2sVxTM6bUDsQO4F50V9RQ22qBpEdCW+I+/Wmke2HOl6lS6dTpdxVhb88/I6+Hs+438c3lfUow==}
+ dev: false
+
+ /cli-cursor@4.0.0:
+ resolution: {integrity: sha512-VGtlMu3x/4DOtIUwEkRezxUZ2lBacNJCHash0N0WeZDBS+7Ux1dm3XWAgWYxLJFMMdOeXMHXorshEFhbMSGelg==}
+ engines: {node: ^12.20.0 || ^14.13.1 || >=16.0.0}
+ dependencies:
+ restore-cursor: 4.0.0
+ dev: true
+
+ /cli-truncate@3.1.0:
+ resolution: {integrity: sha512-wfOBkjXteqSnI59oPcJkcPl/ZmwvMMOj340qUIY1SKZCv0B9Cf4D4fAucRkIKQmsIuYK3x1rrgU7MeGRruiuiA==}
+ engines: {node: ^12.20.0 || ^14.13.1 || >=16.0.0}
+ dependencies:
+ slice-ansi: 5.0.0
+ string-width: 5.1.2
+ dev: true
+
+ /cliui@8.0.1:
+ resolution: {integrity: sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==}
+ engines: {node: '>=12'}
+ dependencies:
+ string-width: 4.2.3
+ strip-ansi: 6.0.1
+ wrap-ansi: 7.0.0
+ dev: true
+
+ /clone-regexp@1.0.1:
+ resolution: {integrity: sha512-Fcij9IwRW27XedRIJnSOEupS7RVcXtObJXbcUOX93UCLqqOdRpkvzKywOOSizmEK/Is3S/RHX9dLdfo6R1Q1mw==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-regexp: 1.0.0
+ is-supported-regexp-flag: 1.0.1
+ dev: true
+
+ /clone@2.1.2:
+ resolution: {integrity: sha512-3Pe/CF1Nn94hyhIYpjtiLhdCoEoz0DqQ+988E9gmeEdQZlojxnOb74wctFyuwWQHzqyf9X7C7MG8juUpqBJT8w==}
+ engines: {node: '>=0.8'}
+
+ /collapse-white-space@1.0.6:
+ resolution: {integrity: sha512-jEovNnrhMuqyCcjfEJA56v0Xq8SkIoPKDyaHahwo3POf4qcSXqMYuwNcOTzp74vTsR9Tn08z4MxWqAhcekogkQ==}
+ dev: true
+
+ /collection-visit@1.0.0:
+ resolution: {integrity: sha512-lNkKvzEeMBBjUGHZ+q6z9pSJla0KWAQPvtzhEV9+iGyQYG+pBpl7xKDhxoNSOZH2hhv0v5k0y2yAM4o4SjoSkw==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ map-visit: 1.0.0
+ object-visit: 1.0.1
+ dev: true
+
+ /color-convert@1.9.3:
+ resolution: {integrity: sha512-QfAUtd+vFdAtFQcC8CCyYt1fYWxSqAiK2cSD6zDB8N3cpsEBAvRxp9zOGg6G/SHHJYAT88/az/IuDGALsNVbGg==}
+ dependencies:
+ color-name: 1.1.3
+
+ /color-convert@2.0.1:
+ resolution: {integrity: sha512-RRECPsj7iu/xb5oKYcsFHSppFNnsj/52OVTRKb4zP5onXwVF3zVmmToNcOfGC+CRDpfK/U584fMg38ZHCaElKQ==}
+ engines: {node: '>=7.0.0'}
+ dependencies:
+ color-name: 1.1.4
+
+ /color-name@1.1.3:
+ resolution: {integrity: sha512-72fSenhMw2HZMTVHeCA9KCmpEIbzWiQsjN+BHcBbS9vr1mtt+vJjPdksIBNUmKAW8TFUDPJK5SUU3QhE9NEXDw==}
+
+ /color-name@1.1.4:
+ resolution: {integrity: sha512-dOy+3AuW3a2wNbZHIuMZpTcgjGuLU/uBL/ubcZF9OXbDo8ff4O8yVp5Bf0efS8uEoYo5q4Fx7dY9OgQGXgAsQA==}
+
+ /color-string@1.9.1:
+ resolution: {integrity: sha512-shrVawQFojnZv6xM40anx4CkoDP+fZsw/ZerEMsW/pyzsRbElpsL/DBVW7q3ExxwusdNXI3lXpuhEZkzs8p5Eg==}
+ dependencies:
+ color-name: 1.1.4
+ simple-swizzle: 0.2.2
+ dev: false
+
+ /color@4.2.3:
+ resolution: {integrity: sha512-1rXeuUUiGGrykh+CeBdu5Ie7OJwinCgQY0bc7GCRxy5xVHy+moaqkpL/jqQq0MtQOeYcrqEz4abc5f0KtU7W4A==}
+ engines: {node: '>=12.5.0'}
+ dependencies:
+ color-convert: 2.0.1
+ color-string: 1.9.1
+ dev: false
+
+ /colord@2.9.3:
+ resolution: {integrity: sha512-jeC1axXpnb0/2nn/Y1LPuLdgXBLH7aDcHu4KEKfqw3CUhX7ZpfBSlPKyqXE6btIgEzfWtrX3/tyBCaCvXvMkOw==}
+ dev: true
+
+ /colorette@2.0.20:
+ resolution: {integrity: sha512-IfEDxwoWIjkeXL1eXcDiow4UbKjhLdq6/EuSVR9GMN7KVH3r9gQ83e73hsz1Nd1T3ijd5xv1wcWRYO+D6kCI2w==}
+ dev: true
+
+ /combined-stream@1.0.8:
+ resolution: {integrity: sha512-FQN4MRfuJeHf7cBbBMJFXhKSDq+2kAArBlmRBvcvFE5BB1HZKXtSFASDhdlz9zOYwxh8lDdnvmMOe/+5cdoEdg==}
+ engines: {node: '>= 0.8'}
+ dependencies:
+ delayed-stream: 1.0.0
+ dev: false
+
+ /comma-separated-tokens@2.0.3:
+ resolution: {integrity: sha512-Fu4hJdvzeylCfQPp9SGWidpzrMs7tTrlu6Vb8XGaRGck8QSNZJJp538Wrb60Lax4fPwR64ViY468OIUTbRlGZg==}
+ dev: false
+
+ /commander@11.0.0:
+ resolution: {integrity: sha512-9HMlXtt/BNoYr8ooyjjNRdIilOTkVJXB+GhxMTtOKwk0R4j4lS4NpjuqmRxroBfnfTSHQIHQB7wryHhXarNjmQ==}
+ engines: {node: '>=16'}
+ dev: true
+
+ /commander@2.20.3:
+ resolution: {integrity: sha512-GpVkmM8vF2vQUkj2LvZmD35JxeJOLCwJ9cUkugyk2nuhbv3+mJvpLYYt+0+USMxE+oj+ey/lJEnhZw75x/OMcQ==}
+
+ /commander@4.1.1:
+ resolution: {integrity: sha512-NOKm8xhkzAjzFx8B2v5OAHT+u5pRQc2UCa2Vq9jYL/31o2wi9mxBA7LIFs3sV5VSC49z6pEhfbMULvShKj26WA==}
+ engines: {node: '>= 6'}
+ dev: true
+
+ /commander@7.2.0:
+ resolution: {integrity: sha512-QrWXB+ZQSVPmIWIhtEO9H+gwHaMGYiF5ChvoJ+K9ZGHG/sVsa6yiesAD1GC/x46sET00Xlwo1u49RVVVzvcSkw==}
+ engines: {node: '>= 10'}
+ dev: true
+
+ /compare-func@2.0.0:
+ resolution: {integrity: sha512-zHig5N+tPWARooBnb0Zx1MFcdfpyJrfTJ3Y5L+IFvUm8rM74hHz66z0gw0x4tijh5CorKkKUCnW82R2vmpeCRA==}
+ dependencies:
+ array-ify: 1.0.0
+ dot-prop: 5.3.0
+ dev: true
+
+ /component-emitter@1.3.1:
+ resolution: {integrity: sha512-T0+barUSQRTUQASh8bx02dl+DhF54GtIDY13Y3m9oWTklKbb3Wv974meRpeZ3lp1JpLVECWWNHC4vaG2XHXouQ==}
+ dev: true
+
+ /compute-scroll-into-view@3.1.0:
+ resolution: {integrity: sha512-rj8l8pD4bJ1nx+dAkMhV1xB5RuZEyVysfxJqB1pRchh1KVvwOv9b7CGB8ZfjTImVv2oF+sYMUkMZq6Na5Ftmbg==}
+ dev: false
+
+ /concat-map@0.0.1:
+ resolution: {integrity: sha512-/Srv4dswyQNBfohGpz9o6Yb3Gz3SrUDqBH5rTuhGR7ahtlbYKnVxw2bCFMRljaA7EXHaXZ8wsHdodFvbkhKmqg==}
+ dev: true
+
+ /confusing-browser-globals@1.0.11:
+ resolution: {integrity: sha512-JsPKdmh8ZkmnHxDk55FZ1TqVLvEQTvoByJZRN9jzI0UjxK/QgAmsphz7PGtqgPieQZ/CQcHWXCR7ATDNhGe+YA==}
+ dev: true
+
+ /conventional-changelog-angular@6.0.0:
+ resolution: {integrity: sha512-6qLgrBF4gueoC7AFVHu51nHL9pF9FRjXrH+ceVf7WmAfH3gs+gEYOkvxhjMPjZu57I4AGUGoNTY8V7Hrgf1uqg==}
+ engines: {node: '>=14'}
+ dependencies:
+ compare-func: 2.0.0
+ dev: true
+
+ /conventional-changelog-conventionalcommits@6.1.0:
+ resolution: {integrity: sha512-3cS3GEtR78zTfMzk0AizXKKIdN4OvSh7ibNz6/DPbhWWQu7LqE/8+/GqSodV+sywUR2gpJAdP/1JFf4XtN7Zpw==}
+ engines: {node: '>=14'}
+ dependencies:
+ compare-func: 2.0.0
+ dev: true
+
+ /conventional-commits-parser@4.0.0:
+ resolution: {integrity: sha512-WRv5j1FsVM5FISJkoYMR6tPk07fkKT0UodruX4je86V4owk451yjXAKzKAPOs9l7y59E2viHUS9eQ+dfUA9NSg==}
+ engines: {node: '>=14'}
+ hasBin: true
+ dependencies:
+ JSONStream: 1.3.5
+ is-text-path: 1.0.1
+ meow: 8.1.2
+ split2: 3.2.2
+ dev: true
+
+ /convert-source-map@1.9.0:
+ resolution: {integrity: sha512-ASFBup0Mz1uyiIjANan1jzLQami9z1PoYSZCiiYW2FczPbenXc45FZdBZLzOT+r6+iciuEModtmCti+hjaAk0A==}
+ dev: false
+
+ /convert-source-map@2.0.0:
+ resolution: {integrity: sha512-Kvp459HrV2FEJ1CAsi1Ku+MY3kasH19TFykTz2xWmMeq6bk2NU3XXvfJ+Q61m0xktWwt+1HSYf3JZsTms3aRJg==}
+
+ /copy-anything@3.0.5:
+ resolution: {integrity: sha512-yCEafptTtb4bk7GLEQoM8KVJpxAfdBJYaXyzQEgQQQgYrZiDp8SJmGKlYza6CYjEDNstAdNdKA3UuoULlEbS6w==}
+ engines: {node: '>=12.13'}
+ dependencies:
+ is-what: 4.1.16
+ dev: false
+
+ /copy-descriptor@0.1.1:
+ resolution: {integrity: sha512-XgZ0pFcakEUlbwQEVNg3+QAis1FyTL3Qel9FYy8pSkQqoG3PNoT0bOCQtOXcOkur21r2Eq2kI+IE+gsmAEVlYw==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /copy-to-clipboard@3.3.3:
+ resolution: {integrity: sha512-2KV8NhB5JqC3ky0r9PMCAZKbUHSwtEo4CwCs0KXgruG43gX5PMqDEBbVU4OUzw2MuAWUfsuFmWvEKG5QRfSnJA==}
+ dependencies:
+ toggle-selection: 1.0.6
+ dev: false
+
+ /cors@2.8.5:
+ resolution: {integrity: sha512-KIHbLJqu73RGr/hnbrO9uBeixNGuvSQjul/jdFvS/KFSIH1hWVd1ng7zOHx+YrEfInLG7q4n6GHQ9cDtxv/P6g==}
+ engines: {node: '>= 0.10'}
+ dependencies:
+ object-assign: 4.1.1
+ vary: 1.1.2
+ dev: true
+
+ /cosmiconfig-typescript-loader@4.4.0(@types/node@20.5.1)(cosmiconfig@8.3.6)(ts-node@10.9.2)(typescript@5.4.5):
+ resolution: {integrity: sha512-BabizFdC3wBHhbI4kJh0VkQP9GkBfoHPydD0COMce1nJ1kJAB3F2TmJ/I7diULBKtmEWSwEbuN/KDtgnmUUVmw==}
+ engines: {node: '>=v14.21.3'}
+ peerDependencies:
+ '@types/node': '*'
+ cosmiconfig: '>=7'
+ ts-node: '>=10'
+ typescript: '>=4'
+ dependencies:
+ '@types/node': 20.5.1
+ cosmiconfig: 8.3.6(typescript@5.4.5)
+ ts-node: 10.9.2(@types/node@20.5.1)(typescript@5.4.5)
+ typescript: 5.4.5
+ dev: true
+
+ /cosmiconfig@5.2.1:
+ resolution: {integrity: sha512-H65gsXo1SKjf8zmrJ67eJk8aIRKV5ff2D4uKZIBZShbhGSpEmsQOPW/SKMKYhSTrqR7ufy6RP69rPogdaPh/kA==}
+ engines: {node: '>=4'}
+ dependencies:
+ import-fresh: 2.0.0
+ is-directory: 0.3.1
+ js-yaml: 3.14.1
+ parse-json: 4.0.0
+ dev: true
+
+ /cosmiconfig@6.0.0:
+ resolution: {integrity: sha512-xb3ZL6+L8b9JLLCx3ZdoZy4+2ECphCMo2PwqgP1tlfVq6M6YReyzBJtvWWtbDSpNr9hn96pkCiZqUcFEc+54Qg==}
+ engines: {node: '>=8'}
+ dependencies:
+ '@types/parse-json': 4.0.2
+ import-fresh: 3.3.0
+ parse-json: 5.2.0
+ path-type: 4.0.0
+ yaml: 1.10.2
+ dev: true
+
+ /cosmiconfig@7.1.0:
+ resolution: {integrity: sha512-AdmX6xUzdNASswsFtmwSt7Vj8po9IuqXm0UXz7QKPuEUmPB4XyjGfaAr2PSuELMwkRMVH1EpIkX5bTZGRB3eCA==}
+ engines: {node: '>=10'}
+ dependencies:
+ '@types/parse-json': 4.0.2
+ import-fresh: 3.3.0
+ parse-json: 5.2.0
+ path-type: 4.0.0
+ yaml: 1.10.2
+ dev: false
+
+ /cosmiconfig@8.3.6(typescript@5.4.5):
+ resolution: {integrity: sha512-kcZ6+W5QzcJ3P1Mt+83OUv/oHFqZHIx8DuxG6eZ5RGMERoLqp4BuGjhHLYGK+Kf5XVkQvqBSmAy/nGWN3qDgEA==}
+ engines: {node: '>=14'}
+ peerDependencies:
+ typescript: '>=4.9.5'
+ peerDependenciesMeta:
+ typescript:
+ optional: true
+ dependencies:
+ import-fresh: 3.3.0
+ js-yaml: 4.1.0
+ parse-json: 5.2.0
+ path-type: 4.0.0
+ typescript: 5.4.5
+ dev: true
+
+ /create-require@1.1.1:
+ resolution: {integrity: sha512-dcKFX3jn0MpIaXjisoRvexIJVEKzaq7z2rZKxf+MSr9TkdmHmsU4m2lcLojrj/FHl8mk5VxMmYA+ftRkP/3oKQ==}
+ dev: true
+
+ /cross-spawn@7.0.3:
+ resolution: {integrity: sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==}
+ engines: {node: '>= 8'}
+ dependencies:
+ path-key: 3.1.1
+ shebang-command: 2.0.0
+ which: 2.0.2
+ dev: true
+
+ /css-box-model@1.2.1:
+ resolution: {integrity: sha512-a7Vr4Q/kd/aw96bnJG332W9V9LkJO69JRcaCYDUqjp6/z0w6VcZjgAcTbgFxEPfBgdnAwlh3iwu+hLopa+flJw==}
+ dependencies:
+ tiny-invariant: 1.3.3
+ dev: false
+
+ /css-color-keywords@1.0.0:
+ resolution: {integrity: sha512-FyyrDHZKEjXDpNJYvVsV960FiqQyXc/LlYmsxl2BcdMb2WPx0OGRVgTg55rPSyLSNMqP52R9r8geSp7apN3Ofg==}
+ engines: {node: '>=4'}
+ dev: false
+
+ /css-functions-list@3.2.2:
+ resolution: {integrity: sha512-c+N0v6wbKVxTu5gOBBFkr9BEdBWaqqjQeiJ8QvSRIJOf+UxlJh930m8e6/WNeODIK0mYLFkoONrnj16i2EcvfQ==}
+ engines: {node: '>=12 || >=16'}
+ dev: true
+
+ /css-in-js-utils@3.1.0:
+ resolution: {integrity: sha512-fJAcud6B3rRu+KHYk+Bwf+WFL2MDCJJ1XG9x137tJQ0xYxor7XziQtuGFbWNdqrvF4Tk26O3H73nfVqXt/fW1A==}
+ dependencies:
+ hyphenate-style-name: 1.0.4
+ dev: false
+
+ /css-select@4.3.0:
+ resolution: {integrity: sha512-wPpOYtnsVontu2mODhA19JrqWxNsfdatRKd64kmpRbQgh1KtItko5sTnEpPdpSaJszTOhEMlF/RPz28qj4HqhQ==}
+ dependencies:
+ boolbase: 1.0.0
+ css-what: 6.1.0
+ domhandler: 4.3.1
+ domutils: 2.8.0
+ nth-check: 2.1.1
+ dev: true
+
+ /css-to-react-native@3.2.0:
+ resolution: {integrity: sha512-e8RKaLXMOFii+02mOlqwjbD00KSEKqblnpO9e++1aXS1fPQOpS1YoqdVHBqPjHNoxeF2mimzVqawm2KCbEdtHQ==}
+ dependencies:
+ camelize: 1.0.1
+ css-color-keywords: 1.0.0
+ postcss-value-parser: 4.2.0
+ dev: false
+
+ /css-tree@1.1.3:
+ resolution: {integrity: sha512-tRpdppF7TRazZrjJ6v3stzv93qxRcSsFmW6cX0Zm2NVKpxE1WV1HblnghVv9TreireHkqI/VDEsfolRF1p6y7Q==}
+ engines: {node: '>=8.0.0'}
+ dependencies:
+ mdn-data: 2.0.14
+ source-map: 0.6.1
+
+ /css-tree@2.3.1:
+ resolution: {integrity: sha512-6Fv1DV/TYw//QF5IzQdqsNDjx/wc8TrMBZsqjL9eW01tWb7R7k/mq+/VXfJCl7SoD5emsJop9cOByJZfs8hYIw==}
+ engines: {node: ^10 || ^12.20.0 || ^14.13.0 || >=15.0.0}
+ dependencies:
+ mdn-data: 2.0.30
+ source-map-js: 1.2.0
+ dev: true
+
+ /css-what@6.1.0:
+ resolution: {integrity: sha512-HTUrgRJ7r4dsZKU6GjmpfRK1O76h97Z8MfS1G0FozR+oF2kG6Vfe8JE6zwrkbxigziPHinCJ+gCPjA9EaBDtRw==}
+ engines: {node: '>= 6'}
+ dev: true
+
+ /cssesc@3.0.0:
+ resolution: {integrity: sha512-/Tb/JcjK111nNScGob5MNtsntNM1aCNUDipB/TkwZFhyDrrE47SOx/18wF2bbjgc3ZzCSKW1T5nt5EbFoAz/Vg==}
+ engines: {node: '>=4'}
+ hasBin: true
+ dev: true
+
+ /csso@4.2.0:
+ resolution: {integrity: sha512-wvlcdIbf6pwKEk7vHj8/Bkc0B4ylXZruLvOgs9doS5eOsOpuodOV2zJChSpkp+pRpYQLQMeF04nr3Z68Sta9jA==}
+ engines: {node: '>=8.0.0'}
+ dependencies:
+ css-tree: 1.1.3
+ dev: true
+
+ /csstype@3.1.2:
+ resolution: {integrity: sha512-I7K1Uu0MBPzaFKg4nI5Q7Vs2t+3gWWW648spaF+Rg7pI9ds18Ugn+lvg4SHczUdKlHI5LWBXyqfS8+DufyBsgQ==}
+ dev: false
+
+ /csstype@3.1.3:
+ resolution: {integrity: sha512-M1uQkMl8rQK/szD0LNhtqxIPLpimGm8sOBwU7lLnCpSbTyY3yeU1Vc7l4KT5zT4s/yOxHH5O7tIuuLOCnLADRw==}
+
+ /currently-unhandled@0.4.1:
+ resolution: {integrity: sha512-/fITjgjGU50vjQ4FH6eUoYu+iUoUKIXws2hL15JJpIR+BbTxaXQsMuuyjtNh2WqsSBS5nsaZHFsFecyw5CCAng==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ array-find-index: 1.0.2
+ dev: true
+
+ /damerau-levenshtein@1.0.8:
+ resolution: {integrity: sha512-sdQSFB7+llfUcQHUQO3+B8ERRj0Oa4w9POWMI/puGtuf7gFywGmkaLCElnudfTiKZV+NvHqL0ifzdrI8Ro7ESA==}
+ dev: true
+
+ /dargs@7.0.0:
+ resolution: {integrity: sha512-2iy1EkLdlBzQGvbweYRFxmFath8+K7+AKB0TlhHWkNuH+TmovaMH/Wp7V7R4u7f4SnX3OgLsU9t1NI9ioDnUpg==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /data-view-buffer@1.0.1:
+ resolution: {integrity: sha512-0lht7OugA5x3iJLOWFhWK/5ehONdprk0ISXqVFn/NFrDu+cuc8iADFrGQz5BnRK7LLU3JmkbXSxaqX+/mXYtUA==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ es-errors: 1.3.0
+ is-data-view: 1.0.1
+ dev: true
+
+ /data-view-byte-length@1.0.1:
+ resolution: {integrity: sha512-4J7wRJD3ABAzr8wP+OcIcqq2dlUKp4DVflx++hs5h5ZKydWMI6/D/fAot+yh6g2tHh8fLFTvNOaVN357NvSrOQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ es-errors: 1.3.0
+ is-data-view: 1.0.1
+ dev: true
+
+ /data-view-byte-offset@1.0.0:
+ resolution: {integrity: sha512-t/Ygsytq+R995EJ5PZlD4Cu56sWa8InXySaViRzw9apusqsOO2bQP+SbYzAhR0pFKoB+43lYy8rWban9JSuXnA==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ es-errors: 1.3.0
+ is-data-view: 1.0.1
+ dev: true
+
+ /dayjs@1.11.10:
+ resolution: {integrity: sha512-vjAczensTgRcqDERK0SR2XMwsF/tSvnvlv6VcF2GIhg6Sx4yOIt/irsr1RDJsKiIyBzJDpCoXiWWq28MqH2cnQ==}
+ dev: false
+
+ /debug@2.6.9:
+ resolution: {integrity: sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==}
+ peerDependencies:
+ supports-color: '*'
+ peerDependenciesMeta:
+ supports-color:
+ optional: true
+ dependencies:
+ ms: 2.0.0
+ dev: true
+
+ /debug@3.2.7:
+ resolution: {integrity: sha512-CFjzYYAi4ThfiQvizrFQevTTXHtnCqWfe7x1AhgEscTz6ZbLbfoLRLPugTQyBth6f8ZERVUSyWHFD/7Wu4t1XQ==}
+ peerDependencies:
+ supports-color: '*'
+ peerDependenciesMeta:
+ supports-color:
+ optional: true
+ dependencies:
+ ms: 2.1.3
+ dev: true
+
+ /debug@4.3.4:
+ resolution: {integrity: sha512-PRWFHuSU3eDtQJPvnNY7Jcket1j0t5OuOsFzPPzsekD52Zl8qUfFIPEiswXqIvHWGVHOgX+7G/vCNNhehwxfkQ==}
+ engines: {node: '>=6.0'}
+ peerDependencies:
+ supports-color: '*'
+ peerDependenciesMeta:
+ supports-color:
+ optional: true
+ dependencies:
+ ms: 2.1.2
+
+ /decamelize-keys@1.1.1:
+ resolution: {integrity: sha512-WiPxgEirIV0/eIOMcnFBA3/IJZAZqKnwAwWyvvdi4lsr1WCN22nhdf/3db3DoZcUjTV2SqfzIwNyp6y2xs3nmg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ decamelize: 1.2.0
+ map-obj: 1.0.1
+ dev: true
+
+ /decamelize@1.2.0:
+ resolution: {integrity: sha512-z2S+W9X73hAUUki+N+9Za2lBlun89zigOyGrsax+KUQ6wKW4ZoWpEYBkGhQjwAjjDCkWxhY0VKEhk8wzY7F5cA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /decamelize@5.0.1:
+ resolution: {integrity: sha512-VfxadyCECXgQlkoEAjeghAr5gY3Hf+IKjKb+X8tGVDtveCjN+USwprd2q3QXBR9T1+x2DG0XZF5/w+7HAtSaXA==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /decode-named-character-reference@1.0.2:
+ resolution: {integrity: sha512-O8x12RzrUF8xyVcY0KJowWsmaJxQbmy0/EtnNtHRpsOcT7dFk5W598coHqBVpmWo1oQQfsCqfCmkZN5DJrZVdg==}
+ dependencies:
+ character-entities: 2.0.2
+ dev: false
+
+ /decode-uri-component@0.2.2:
+ resolution: {integrity: sha512-FqUYQ+8o158GyGTrMFJms9qh3CqTKvAqgqsTnkLI8sKu0028orqBhxNMFkFen0zGyg6epACD32pjVk58ngIErQ==}
+ engines: {node: '>=0.10'}
+ dev: true
+
+ /deep-equal@1.1.2:
+ resolution: {integrity: sha512-5tdhKF6DbU7iIzrIOa1AOUt39ZRm13cmL1cGEh//aqR8x9+tNfbywRf0n5FD/18OKMdo7DNEtrX2t22ZAkI+eg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ is-arguments: 1.1.1
+ is-date-object: 1.0.5
+ is-regex: 1.1.4
+ object-is: 1.1.6
+ object-keys: 1.1.1
+ regexp.prototype.flags: 1.5.2
+ dev: false
+
+ /deep-is@0.1.4:
+ resolution: {integrity: sha512-oIPzksmTg4/MriiaYGO+okXDT7ztn/w3Eptv/+gSIdMdKsJo0u4CfYNFJPy+4SKMuCqGw2wxnA+URMg3t8a/bQ==}
+ dev: true
+
+ /deepmerge@4.3.1:
+ resolution: {integrity: sha512-3sUqbMEc77XqpdNO7FRyRog+eW3ph+GYCbj+rK+uYyRMuwsVy0rMiVtPn+QJlKFvWP/1PYpapqYn0Me2knFn+A==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /define-data-property@1.1.4:
+ resolution: {integrity: sha512-rBMvIzlpA8v6E+SJZoo++HAYqsLrkg7MSfIinMPFhmkorw7X+dOXVJQs+QT69zGkzMyfDnIMN2Wid1+NbL3T+A==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ es-define-property: 1.0.0
+ es-errors: 1.3.0
+ gopd: 1.0.1
+
+ /define-lazy-prop@2.0.0:
+ resolution: {integrity: sha512-Ds09qNh8yw3khSjiJjiUInaGX9xlqZDY7JVryGxdxV7NPeuqQfplOpQ66yJFZut3jLa5zOwkXw1g9EI2uKh4Og==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /define-properties@1.2.1:
+ resolution: {integrity: sha512-8QmQKqEASLd5nx0U1B1okLElbUuuttJ/AnYmRXbbbGDWh6uS208EjD4Xqq/I9wK7u0v6O08XhTWnt5XtEbR6Dg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ define-data-property: 1.1.4
+ has-property-descriptors: 1.0.2
+ object-keys: 1.1.1
+
+ /define-property@0.2.5:
+ resolution: {integrity: sha512-Rr7ADjQZenceVOAKop6ALkkRAmH1A4Gx9hV/7ZujPUN2rkATqFO0JZLZInbAjpZYoJ1gUx8MRMQVkYemcbMSTA==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-descriptor: 0.1.7
+ dev: true
+
+ /define-property@1.0.0:
+ resolution: {integrity: sha512-cZTYKFWspt9jZsMscWo8sc/5lbPC9Q0N5nBLgb+Yd915iL3udB1uFgS3B8YCx66UVHq018DAVFoee7x+gxggeA==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-descriptor: 1.0.3
+ dev: true
+
+ /define-property@2.0.2:
+ resolution: {integrity: sha512-jwK2UV4cnPpbcG7+VRARKTZPUWowwXA8bzH5NP6ud0oeAxyYPuGZUAC7hMugpCdz4BeSZl2Dl9k66CHJ/46ZYQ==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-descriptor: 1.0.3
+ isobject: 3.0.1
+ dev: true
+
+ /delayed-stream@1.0.0:
+ resolution: {integrity: sha512-ZySD7Nf91aLB0RxL4KGrKHBXl7Eds1DAmEdcoVawXnLD7SDhpNgtuII2aAkg7a7QS41jxPSZ17p4VdGnMHk3MQ==}
+ engines: {node: '>=0.4.0'}
+ dev: false
+
+ /dequal@2.0.3:
+ resolution: {integrity: sha512-0je+qPKHEMohvfRTCEo3CrPG6cAzAYgmzKyxRiYSSDkS6eGJdyVJm7WaYA5ECaAD9wLB2T4EEeymA5aFVcYXCA==}
+ engines: {node: '>=6'}
+
+ /detect-port-alt@1.1.6:
+ resolution: {integrity: sha512-5tQykt+LqfJFBEYaDITx7S7cR7mJ/zQmLXZ2qt5w04ainYZw6tBf9dBunMjVeVOdYVRUzUOE4HkY5J7+uttb5Q==}
+ engines: {node: '>= 4.2.1'}
+ hasBin: true
+ dependencies:
+ address: 1.2.2
+ debug: 2.6.9
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /didyoumean@1.2.2:
+ resolution: {integrity: sha512-gxtyfqMg7GKyhQmb056K7M3xszy/myH8w+B4RT+QXBQsvAOdc3XymqDDPHx1BgPgsdAA5SIifona89YtRATDzw==}
+ dev: true
+
+ /diff@4.0.2:
+ resolution: {integrity: sha512-58lmxKSA4BNyLz+HHMUzlOEpg09FV+ev6ZMe3vJihgdxzgcwZ8VoEEPmALCZG9LmqfVoNMMKpttIYTVG6uDY7A==}
+ engines: {node: '>=0.3.1'}
+ dev: true
+
+ /diff@5.2.0:
+ resolution: {integrity: sha512-uIFDxqpRZGZ6ThOk84hEfqWoHx2devRFvpTZcTHur85vImfaxUbTW9Ryh4CpCuDnToOP1CEtXKIgytHBPVff5A==}
+ engines: {node: '>=0.3.1'}
+ dev: false
+
+ /dir-glob@2.2.2:
+ resolution: {integrity: sha512-f9LBi5QWzIW3I6e//uxZoLBlUt9kcp66qo0sSCxL6YZKc75R1c4MFCoe/LaZiBGmgujvQdxc5Bn3QhfyvK5Hsw==}
+ engines: {node: '>=4'}
+ dependencies:
+ path-type: 3.0.0
+ dev: true
+
+ /dir-glob@3.0.1:
+ resolution: {integrity: sha512-WkrWp9GR4KXfKGYzOLmTuGVi1UWFfws377n9cc55/tb6DuqyF6pcQ5AbiHEshaDpY9v6oaSr2XCDidGmMwdzIA==}
+ engines: {node: '>=8'}
+ dependencies:
+ path-type: 4.0.0
+ dev: true
+
+ /dlv@1.1.3:
+ resolution: {integrity: sha512-+HlytyjlPKnIG8XuRG8WvmBP8xs8P71y+SKKS6ZXWoEgLuePxtDoUEiH7WkdePWrQ5JBpE6aoVqfZfJUQkjXwA==}
+ dev: true
+
+ /doctrine@2.1.0:
+ resolution: {integrity: sha512-35mSku4ZXK0vfCuHEDAwt55dg2jNajHZ1odvF+8SSr82EsZY4QmXfuWso8oEd8zRhVObSN18aM0CjSdoBX7zIw==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ esutils: 2.0.3
+ dev: true
+
+ /doctrine@3.0.0:
+ resolution: {integrity: sha512-yS+Q5i3hBf7GBkd4KG8a7eBNNWNGLTaEwwYWUijIYM7zrlYDM0BFXHjjPWlWZ1Rg7UaddZeIDmi9jF3HmqiQ2w==}
+ engines: {node: '>=6.0.0'}
+ dependencies:
+ esutils: 2.0.3
+ dev: true
+
+ /dom-serializer@0.2.2:
+ resolution: {integrity: sha512-2/xPb3ORsQ42nHYiSunXkDjPLBaEj/xTwUO4B7XCZQTRk7EBtTOPaygh10YAAh2OI1Qrp6NWfpAhzswj0ydt9g==}
+ dependencies:
+ domelementtype: 2.3.0
+ entities: 2.2.0
+ dev: true
+
+ /dom-serializer@1.4.1:
+ resolution: {integrity: sha512-VHwB3KfrcOOkelEG2ZOfxqLZdfkil8PtJi4P8N2MMXucZq2yLp75ClViUlOVwyoHEDjYU433Aq+5zWP61+RGag==}
+ dependencies:
+ domelementtype: 2.3.0
+ domhandler: 4.3.1
+ entities: 2.2.0
+ dev: true
+
+ /domelementtype@1.3.1:
+ resolution: {integrity: sha512-BSKB+TSpMpFI/HOxCNr1O8aMOTZ8hT3pM3GQ0w/mWRmkhEDSFJkkyzz4XQsBV44BChwGkrDfMyjVD0eA2aFV3w==}
+ dev: true
+
+ /domelementtype@2.3.0:
+ resolution: {integrity: sha512-OLETBj6w0OsagBwdXnPdN0cnMfF9opN69co+7ZrbfPGrdpPVNBUj02spi6B1N7wChLQiPn4CSH/zJvXw56gmHw==}
+ dev: true
+
+ /domhandler@2.4.2:
+ resolution: {integrity: sha512-JiK04h0Ht5u/80fdLMCEmV4zkNh2BcoMFBmZ/91WtYZ8qVXSKjiw7fXMgFPnHcSZgOo3XdinHvmnDUeMf5R4wA==}
+ dependencies:
+ domelementtype: 1.3.1
+ dev: true
+
+ /domhandler@4.3.1:
+ resolution: {integrity: sha512-GrwoxYN+uWlzO8uhUXRl0P+kHE4GtVPfYzVLcUxPL7KNdHKj66vvlhiweIHqYYXWlw+T8iLMp42Lm67ghw4WMQ==}
+ engines: {node: '>= 4'}
+ dependencies:
+ domelementtype: 2.3.0
+ dev: true
+
+ /domutils@1.7.0:
+ resolution: {integrity: sha512-Lgd2XcJ/NjEw+7tFvfKxOzCYKZsdct5lczQ2ZaQY8Djz7pfAD3Gbp8ySJWtreII/vDlMVmxwa6pHmdxIYgttDg==}
+ dependencies:
+ dom-serializer: 0.2.2
+ domelementtype: 1.3.1
+ dev: true
+
+ /domutils@2.8.0:
+ resolution: {integrity: sha512-w96Cjofp72M5IIhpjgobBimYEfoPjx1Vx0BSX9P30WBdZW2WIKU0T1Bd0kz2eNZ9ikjKgHbEyKx8BB6H1L3h3A==}
+ dependencies:
+ dom-serializer: 1.4.1
+ domelementtype: 2.3.0
+ domhandler: 4.3.1
+ dev: true
+
+ /dot-prop@5.3.0:
+ resolution: {integrity: sha512-QM8q3zDe58hqUqjraQOmzZ1LIH9SWQJTlEKCH4kJ2oQvLZk7RbQXvtDM2XEq3fwkV9CCvvH4LA0AV+ogFsBM2Q==}
+ engines: {node: '>=8'}
+ dependencies:
+ is-obj: 2.0.0
+ dev: true
+
+ /duplexer@0.1.2:
+ resolution: {integrity: sha512-jtD6YG370ZCIi/9GTaJKQxWTZD045+4R4hTk/x1UyoqadyJ9x9CgSi1RlVDQF8U2sxLLSnFkCaMihqljHIWgMg==}
+ dev: true
+
+ /eastasianwidth@0.2.0:
+ resolution: {integrity: sha512-I88TYZWc9XiYHRQ4/3c5rjjfgkjhLyW2luGIheGERbNQ6OY7yTybanSpDXZa8y7VUP9YmDcYa+eyq4ca7iLqWA==}
+ dev: true
+
+ /electron-to-chromium@1.4.749:
+ resolution: {integrity: sha512-LRMMrM9ITOvue0PoBrvNIraVmuDbJV5QC9ierz/z5VilMdPOVMjOtpICNld3PuXuTZ3CHH/UPxX9gHhAPwi+0Q==}
+
+ /emoji-regex@7.0.3:
+ resolution: {integrity: sha512-CwBLREIQ7LvYFB0WyRvwhq5N5qPhc6PMjD6bYggFlI5YyDgl+0vxq5VHbMOFqLg7hfWzmu8T5Z1QofhmTIhItA==}
+ dev: true
+
+ /emoji-regex@8.0.0:
+ resolution: {integrity: sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==}
+ dev: true
+
+ /emoji-regex@9.2.2:
+ resolution: {integrity: sha512-L18DaJsXSUk2+42pv8mLs5jJT2hqFkFE4j21wOmgbUqsZ2hL72NsUU785g9RXgo3s0ZNgVl42TiHp3ZtOv/Vyg==}
+ dev: true
+
+ /emojis-list@3.0.0:
+ resolution: {integrity: sha512-/kyM18EfinwXZbno9FyUGeFh87KC8HRQBQGildHZbEuRyWFOmv1U10o9BBp8XVZDVNNuQKyIGIu5ZYAAXJ0V2Q==}
+ engines: {node: '>= 4'}
+ dev: true
+
+ /enhanced-resolve@5.16.0:
+ resolution: {integrity: sha512-O+QWCviPNSSLAD9Ucn8Awv+poAkqn3T1XY5/N7kR7rQO9yfSGWkYZDwpJ+iKF7B8rxaQKWngSqACpgzeapSyoA==}
+ engines: {node: '>=10.13.0'}
+ dependencies:
+ graceful-fs: 4.2.11
+ tapable: 2.2.1
+ dev: true
+
+ /entities@1.1.2:
+ resolution: {integrity: sha512-f2LZMYl1Fzu7YSBKg+RoROelpOaNrcGmE9AZubeDfrCEia483oW4MI4VyFd5VNHIgQ/7qm1I0wUHK1eJnn2y2w==}
+ dev: true
+
+ /entities@2.2.0:
+ resolution: {integrity: sha512-p92if5Nz619I0w+akJrLZH0MX0Pb5DX39XOwQTtXSdQQOaYH03S1uIQp4mhOZtAXrxq4ViO67YTiLBo2638o9A==}
+ dev: true
+
+ /error-ex@1.3.2:
+ resolution: {integrity: sha512-7dFHNmqeFSEt2ZBsCriorKnn3Z2pj+fd9kmI6QoWw4//DL+icEBfc0U7qJCisqrTsKTjw4fNFy2pW9OqStD84g==}
+ dependencies:
+ is-arrayish: 0.2.1
+
+ /error-stack-parser@2.1.4:
+ resolution: {integrity: sha512-Sk5V6wVazPhq5MhpO+AUxJn5x7XSXGl1R93Vn7i+zS15KDVxQijejNCrz8340/2bgLBjR9GtEG8ZVKONDjcqGQ==}
+ dependencies:
+ stackframe: 1.3.4
+ dev: false
+
+ /es-abstract@1.23.3:
+ resolution: {integrity: sha512-e+HfNH61Bj1X9/jLc5v1owaLYuHdeHHSQlkhCBiTK8rBvKaULl/beGMxwrMXjpYrv4pz22BlY570vVePA2ho4A==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ array-buffer-byte-length: 1.0.1
+ arraybuffer.prototype.slice: 1.0.3
+ available-typed-arrays: 1.0.7
+ call-bind: 1.0.7
+ data-view-buffer: 1.0.1
+ data-view-byte-length: 1.0.1
+ data-view-byte-offset: 1.0.0
+ es-define-property: 1.0.0
+ es-errors: 1.3.0
+ es-object-atoms: 1.0.0
+ es-set-tostringtag: 2.0.3
+ es-to-primitive: 1.2.1
+ function.prototype.name: 1.1.6
+ get-intrinsic: 1.2.4
+ get-symbol-description: 1.0.2
+ globalthis: 1.0.3
+ gopd: 1.0.1
+ has-property-descriptors: 1.0.2
+ has-proto: 1.0.3
+ has-symbols: 1.0.3
+ hasown: 2.0.2
+ internal-slot: 1.0.7
+ is-array-buffer: 3.0.4
+ is-callable: 1.2.7
+ is-data-view: 1.0.1
+ is-negative-zero: 2.0.3
+ is-regex: 1.1.4
+ is-shared-array-buffer: 1.0.3
+ is-string: 1.0.7
+ is-typed-array: 1.1.13
+ is-weakref: 1.0.2
+ object-inspect: 1.13.1
+ object-keys: 1.1.1
+ object.assign: 4.1.5
+ regexp.prototype.flags: 1.5.2
+ safe-array-concat: 1.1.2
+ safe-regex-test: 1.0.3
+ string.prototype.trim: 1.2.9
+ string.prototype.trimend: 1.0.8
+ string.prototype.trimstart: 1.0.8
+ typed-array-buffer: 1.0.2
+ typed-array-byte-length: 1.0.1
+ typed-array-byte-offset: 1.0.2
+ typed-array-length: 1.0.6
+ unbox-primitive: 1.0.2
+ which-typed-array: 1.1.15
+ dev: true
+
+ /es-define-property@1.0.0:
+ resolution: {integrity: sha512-jxayLKShrEqqzJ0eumQbVhTYQM27CfT1T35+gCgDFoL82JLsXqTJ76zv6A0YLOgEnLUMvLzsDsGIrl8NFpT2gQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ get-intrinsic: 1.2.4
+
+ /es-errors@1.3.0:
+ resolution: {integrity: sha512-Zf5H2Kxt2xjTvbJvP2ZWLEICxA6j+hAmMzIlypy4xcBg1vKVnx89Wy0GbS+kf5cwCVFFzdCFh2XSCFNULS6csw==}
+ engines: {node: '>= 0.4'}
+
+ /es-iterator-helpers@1.0.19:
+ resolution: {integrity: sha512-zoMwbCcH5hwUkKJkT8kDIBZSz9I6mVG//+lDCinLCGov4+r7NIy0ld8o03M0cJxl2spVf6ESYVS6/gpIfq1FFw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-errors: 1.3.0
+ es-set-tostringtag: 2.0.3
+ function-bind: 1.1.2
+ get-intrinsic: 1.2.4
+ globalthis: 1.0.3
+ has-property-descriptors: 1.0.2
+ has-proto: 1.0.3
+ has-symbols: 1.0.3
+ internal-slot: 1.0.7
+ iterator.prototype: 1.1.2
+ safe-array-concat: 1.1.2
+ dev: true
+
+ /es-module-lexer@1.5.0:
+ resolution: {integrity: sha512-pqrTKmwEIgafsYZAGw9kszYzmagcE/n4dbgwGWLEXg7J4QFJVQRBld8j3Q3GNez79jzxZshq0bcT962QHOghjw==}
+ dev: true
+
+ /es-object-atoms@1.0.0:
+ resolution: {integrity: sha512-MZ4iQ6JwHOBQjahnjwaC1ZtIBH+2ohjamzAO3oaHcXYup7qxjF2fixyH+Q71voWHeOkI2q/TnJao/KfXYIZWbw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ es-errors: 1.3.0
+ dev: true
+
+ /es-set-tostringtag@2.0.3:
+ resolution: {integrity: sha512-3T8uNMC3OQTHkFUsFq8r/BwAXLHvU/9O9mE0fBc/MY5iq/8H7ncvO947LmYA6ldWw9Uh8Yhf25zu6n7nML5QWQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ get-intrinsic: 1.2.4
+ has-tostringtag: 1.0.2
+ hasown: 2.0.2
+ dev: true
+
+ /es-shim-unscopables@1.0.2:
+ resolution: {integrity: sha512-J3yBRXCzDu4ULnQwxyToo/OjdMx6akgVC7K6few0a7F/0wLtmKKN7I73AH5T2836UuXRqN7Qg+IIUw/+YJksRw==}
+ dependencies:
+ hasown: 2.0.2
+ dev: true
+
+ /es-to-primitive@1.2.1:
+ resolution: {integrity: sha512-QCOllgZJtaUo9miYBcLChTUaHNjJF3PYs1VidD7AwiEj1kYxKeQTctLAezAOH5ZKRH0g2IgPn6KwB4IT8iRpvA==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ is-callable: 1.2.7
+ is-date-object: 1.0.5
+ is-symbol: 1.0.4
+ dev: true
+
+ /esbuild@0.18.20:
+ resolution: {integrity: sha512-ceqxoedUrcayh7Y7ZX6NdbbDzGROiyVBgC4PriJThBKSVPWnnFHZAkfI1lJT8QFkOwH4qOS2SJkS4wvpGl8BpA==}
+ engines: {node: '>=12'}
+ hasBin: true
+ requiresBuild: true
+ optionalDependencies:
+ '@esbuild/android-arm': 0.18.20
+ '@esbuild/android-arm64': 0.18.20
+ '@esbuild/android-x64': 0.18.20
+ '@esbuild/darwin-arm64': 0.18.20
+ '@esbuild/darwin-x64': 0.18.20
+ '@esbuild/freebsd-arm64': 0.18.20
+ '@esbuild/freebsd-x64': 0.18.20
+ '@esbuild/linux-arm': 0.18.20
+ '@esbuild/linux-arm64': 0.18.20
+ '@esbuild/linux-ia32': 0.18.20
+ '@esbuild/linux-loong64': 0.18.20
+ '@esbuild/linux-mips64el': 0.18.20
+ '@esbuild/linux-ppc64': 0.18.20
+ '@esbuild/linux-riscv64': 0.18.20
+ '@esbuild/linux-s390x': 0.18.20
+ '@esbuild/linux-x64': 0.18.20
+ '@esbuild/netbsd-x64': 0.18.20
+ '@esbuild/openbsd-x64': 0.18.20
+ '@esbuild/sunos-x64': 0.18.20
+ '@esbuild/win32-arm64': 0.18.20
+ '@esbuild/win32-ia32': 0.18.20
+ '@esbuild/win32-x64': 0.18.20
+
+ /escalade@3.1.2:
+ resolution: {integrity: sha512-ErCHMCae19vR8vQGe50xIsVomy19rg6gFu3+r3jkEO46suLMWBksvVyoGgQV+jOfl84ZSOSlmv6Gxa89PmTGmA==}
+ engines: {node: '>=6'}
+
+ /escape-string-regexp@1.0.5:
+ resolution: {integrity: sha512-vbRorB5FUQWvla16U8R/qgaFIya2qGzwDrNmCZuYKrbdSUMG6I1ZCGQRefkRVhuOkIGVne7BQ35DSfo1qvJqFg==}
+ engines: {node: '>=0.8.0'}
+
+ /escape-string-regexp@4.0.0:
+ resolution: {integrity: sha512-TtpcNJ3XAzx3Gq8sWRzJaVajRs0uVxA2YAkdb1jm2YkPz4G6egUFAyA3n5vtEIZefPk5Wa4UXbKuS5fKkJWdgA==}
+ engines: {node: '>=10'}
+
+ /escape-string-regexp@5.0.0:
+ resolution: {integrity: sha512-/veY75JbMK4j1yjvuUxuVsiS/hr/4iHs9FTT6cgTexxdE0Ly/glccBAkloH/DofkjRbZU3bnoj38mOmhkZ0lHw==}
+ engines: {node: '>=12'}
+ dev: false
+
+ /eslint-config-airbnb-base@15.0.0(eslint-plugin-import@2.29.1)(eslint@8.57.0):
+ resolution: {integrity: sha512-xaX3z4ZZIcFLvh2oUNvcX5oEofXda7giYmuplVxoOg5A7EXJMrUyqRgR+mhDhPK8LZ4PttFOBvCYDbX3sUoUig==}
+ engines: {node: ^10.12.0 || >=12.0.0}
+ peerDependencies:
+ eslint: ^7.32.0 || ^8.2.0
+ eslint-plugin-import: ^2.25.2
+ dependencies:
+ confusing-browser-globals: 1.0.11
+ eslint: 8.57.0
+ eslint-plugin-import: 2.29.1(@typescript-eslint/parser@5.62.0)(eslint@8.57.0)
+ object.assign: 4.1.5
+ object.entries: 1.1.8
+ semver: 6.3.1
+ dev: true
+
+ /eslint-config-airbnb-typescript@17.1.0(@typescript-eslint/eslint-plugin@5.62.0)(@typescript-eslint/parser@5.62.0)(eslint-plugin-import@2.29.1)(eslint@8.57.0):
+ resolution: {integrity: sha512-GPxI5URre6dDpJ0CtcthSZVBAfI+Uw7un5OYNVxP2EYi3H81Jw701yFP7AU+/vCE7xBtFmjge7kfhhk4+RAiig==}
+ peerDependencies:
+ '@typescript-eslint/eslint-plugin': ^5.13.0 || ^6.0.0
+ '@typescript-eslint/parser': ^5.0.0 || ^6.0.0
+ eslint: ^7.32.0 || ^8.2.0
+ eslint-plugin-import: ^2.25.3
+ dependencies:
+ '@typescript-eslint/eslint-plugin': 5.62.0(@typescript-eslint/parser@5.62.0)(eslint@8.57.0)(typescript@5.4.5)
+ '@typescript-eslint/parser': 5.62.0(eslint@8.57.0)(typescript@5.4.5)
+ eslint: 8.57.0
+ eslint-config-airbnb-base: 15.0.0(eslint-plugin-import@2.29.1)(eslint@8.57.0)
+ eslint-plugin-import: 2.29.1(@typescript-eslint/parser@5.62.0)(eslint@8.57.0)
+ dev: true
+
+ /eslint-config-airbnb@19.0.4(eslint-plugin-import@2.29.1)(eslint-plugin-jsx-a11y@6.8.0)(eslint-plugin-react-hooks@4.6.1)(eslint-plugin-react@7.34.1)(eslint@8.57.0):
+ resolution: {integrity: sha512-T75QYQVQX57jiNgpF9r1KegMICE94VYwoFQyMGhrvc+lB8YF2E/M/PYDaQe1AJcWaEgqLE+ErXV1Og/+6Vyzew==}
+ engines: {node: ^10.12.0 || ^12.22.0 || ^14.17.0 || >=16.0.0}
+ peerDependencies:
+ eslint: ^7.32.0 || ^8.2.0
+ eslint-plugin-import: ^2.25.3
+ eslint-plugin-jsx-a11y: ^6.5.1
+ eslint-plugin-react: ^7.28.0
+ eslint-plugin-react-hooks: ^4.3.0
+ dependencies:
+ eslint: 8.57.0
+ eslint-config-airbnb-base: 15.0.0(eslint-plugin-import@2.29.1)(eslint@8.57.0)
+ eslint-plugin-import: 2.29.1(@typescript-eslint/parser@5.62.0)(eslint@8.57.0)
+ eslint-plugin-jsx-a11y: 6.8.0(eslint@8.57.0)
+ eslint-plugin-react: 7.34.1(eslint@8.57.0)
+ eslint-plugin-react-hooks: 4.6.1(eslint@8.57.0)
+ object.assign: 4.1.5
+ object.entries: 1.1.8
+ dev: true
+
+ /eslint-config-prettier@8.10.0(eslint@8.57.0):
+ resolution: {integrity: sha512-SM8AMJdeQqRYT9O9zguiruQZaN7+z+E4eAP9oiLNGKMtomwaB1E9dcgUD6ZAn/eQAb52USbvezbiljfZUhbJcg==}
+ hasBin: true
+ peerDependencies:
+ eslint: '>=7.0.0'
+ dependencies:
+ eslint: 8.57.0
+ dev: true
+
+ /eslint-import-resolver-alias@1.1.2(eslint-plugin-import@2.29.1):
+ resolution: {integrity: sha512-WdviM1Eu834zsfjHtcGHtGfcu+F30Od3V7I9Fi57uhBEwPkjDcii7/yW8jAT+gOhn4P/vOxxNAXbFAKsrrc15w==}
+ engines: {node: '>= 4'}
+ peerDependencies:
+ eslint-plugin-import: '>=1.4.0'
+ dependencies:
+ eslint-plugin-import: 2.29.1(@typescript-eslint/parser@5.62.0)(eslint@8.57.0)
+ dev: true
+
+ /eslint-import-resolver-node@0.3.9:
+ resolution: {integrity: sha512-WFj2isz22JahUv+B788TlO3N6zL3nNJGU8CcZbPZvVEkBPaJdCV4vy5wyghty5ROFbCRnm132v8BScu5/1BQ8g==}
+ dependencies:
+ debug: 3.2.7
+ is-core-module: 2.13.1
+ resolve: 1.22.8
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /eslint-module-utils@2.8.1(@typescript-eslint/parser@5.62.0)(eslint-import-resolver-node@0.3.9)(eslint@8.57.0):
+ resolution: {integrity: sha512-rXDXR3h7cs7dy9RNpUlQf80nX31XWJEyGq1tRMo+6GsO5VmTe4UTwtmonAD4ZkAsrfMVDA2wlGJ3790Ys+D49Q==}
+ engines: {node: '>=4'}
+ peerDependencies:
+ '@typescript-eslint/parser': '*'
+ eslint: '*'
+ eslint-import-resolver-node: '*'
+ eslint-import-resolver-typescript: '*'
+ eslint-import-resolver-webpack: '*'
+ peerDependenciesMeta:
+ '@typescript-eslint/parser':
+ optional: true
+ eslint:
+ optional: true
+ eslint-import-resolver-node:
+ optional: true
+ eslint-import-resolver-typescript:
+ optional: true
+ eslint-import-resolver-webpack:
+ optional: true
+ dependencies:
+ '@typescript-eslint/parser': 5.62.0(eslint@8.57.0)(typescript@5.4.5)
+ debug: 3.2.7
+ eslint: 8.57.0
+ eslint-import-resolver-node: 0.3.9
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /eslint-plugin-import@2.29.1(@typescript-eslint/parser@5.62.0)(eslint@8.57.0):
+ resolution: {integrity: sha512-BbPC0cuExzhiMo4Ff1BTVwHpjjv28C5R+btTOGaCRC7UEz801up0JadwkeSk5Ued6TG34uaczuVuH6qyy5YUxw==}
+ engines: {node: '>=4'}
+ peerDependencies:
+ '@typescript-eslint/parser': '*'
+ eslint: ^2 || ^3 || ^4 || ^5 || ^6 || ^7.2.0 || ^8
+ peerDependenciesMeta:
+ '@typescript-eslint/parser':
+ optional: true
+ dependencies:
+ '@typescript-eslint/parser': 5.62.0(eslint@8.57.0)(typescript@5.4.5)
+ array-includes: 3.1.8
+ array.prototype.findlastindex: 1.2.5
+ array.prototype.flat: 1.3.2
+ array.prototype.flatmap: 1.3.2
+ debug: 3.2.7
+ doctrine: 2.1.0
+ eslint: 8.57.0
+ eslint-import-resolver-node: 0.3.9
+ eslint-module-utils: 2.8.1(@typescript-eslint/parser@5.62.0)(eslint-import-resolver-node@0.3.9)(eslint@8.57.0)
+ hasown: 2.0.2
+ is-core-module: 2.13.1
+ is-glob: 4.0.3
+ minimatch: 3.1.2
+ object.fromentries: 2.0.8
+ object.groupby: 1.0.3
+ object.values: 1.2.0
+ semver: 6.3.1
+ tsconfig-paths: 3.15.0
+ transitivePeerDependencies:
+ - eslint-import-resolver-typescript
+ - eslint-import-resolver-webpack
+ - supports-color
+ dev: true
+
+ /eslint-plugin-jsx-a11y@6.8.0(eslint@8.57.0):
+ resolution: {integrity: sha512-Hdh937BS3KdwwbBaKd5+PLCOmYY6U4f2h9Z2ktwtNKvIdIEu137rjYbcb9ApSbVJfWxANNuiKTD/9tOKjK9qOA==}
+ engines: {node: '>=4.0'}
+ peerDependencies:
+ eslint: ^3 || ^4 || ^5 || ^6 || ^7 || ^8
+ dependencies:
+ '@babel/runtime': 7.24.4
+ aria-query: 5.3.0
+ array-includes: 3.1.8
+ array.prototype.flatmap: 1.3.2
+ ast-types-flow: 0.0.8
+ axe-core: 4.7.0
+ axobject-query: 3.2.1
+ damerau-levenshtein: 1.0.8
+ emoji-regex: 9.2.2
+ es-iterator-helpers: 1.0.19
+ eslint: 8.57.0
+ hasown: 2.0.2
+ jsx-ast-utils: 3.3.5
+ language-tags: 1.0.9
+ minimatch: 3.1.2
+ object.entries: 1.1.8
+ object.fromentries: 2.0.8
+ dev: true
+
+ /eslint-plugin-prettier@4.2.1(eslint-config-prettier@8.10.0)(eslint@8.57.0)(prettier@2.8.8):
+ resolution: {integrity: sha512-f/0rXLXUt0oFYs8ra4w49wYZBG5GKZpAYsJSm6rnYL5uVDjd+zowwMwVZHnAjf4edNrKpCDYfXDgmRE/Ak7QyQ==}
+ engines: {node: '>=12.0.0'}
+ peerDependencies:
+ eslint: '>=7.28.0'
+ eslint-config-prettier: '*'
+ prettier: '>=2.0.0'
+ peerDependenciesMeta:
+ eslint-config-prettier:
+ optional: true
+ dependencies:
+ eslint: 8.57.0
+ eslint-config-prettier: 8.10.0(eslint@8.57.0)
+ prettier: 2.8.8
+ prettier-linter-helpers: 1.0.0
+ dev: true
+
+ /eslint-plugin-react-hooks@4.6.1(eslint@8.57.0):
+ resolution: {integrity: sha512-Ck77j8hF7l9N4S/rzSLOWEKpn994YH6iwUK8fr9mXIaQvGpQYmOnQLbiue1u5kI5T1y+gdgqosnEAO9NCz0DBg==}
+ engines: {node: '>=10'}
+ peerDependencies:
+ eslint: ^3.0.0 || ^4.0.0 || ^5.0.0 || ^6.0.0 || ^7.0.0 || ^8.0.0-0
+ dependencies:
+ eslint: 8.57.0
+ dev: true
+
+ /eslint-plugin-react@7.34.1(eslint@8.57.0):
+ resolution: {integrity: sha512-N97CxlouPT1AHt8Jn0mhhN2RrADlUAsk1/atcT2KyA/l9Q/E6ll7OIGwNumFmWfZ9skV3XXccYS19h80rHtgkw==}
+ engines: {node: '>=4'}
+ peerDependencies:
+ eslint: ^3 || ^4 || ^5 || ^6 || ^7 || ^8
+ dependencies:
+ array-includes: 3.1.8
+ array.prototype.findlast: 1.2.5
+ array.prototype.flatmap: 1.3.2
+ array.prototype.toreversed: 1.1.2
+ array.prototype.tosorted: 1.1.3
+ doctrine: 2.1.0
+ es-iterator-helpers: 1.0.19
+ eslint: 8.57.0
+ estraverse: 5.3.0
+ jsx-ast-utils: 3.3.5
+ minimatch: 3.1.2
+ object.entries: 1.1.8
+ object.fromentries: 2.0.8
+ object.hasown: 1.1.4
+ object.values: 1.2.0
+ prop-types: 15.8.1
+ resolve: 2.0.0-next.5
+ semver: 6.3.1
+ string.prototype.matchall: 4.0.11
+ dev: true
+
+ /eslint-plugin-unused-imports@2.0.0(@typescript-eslint/eslint-plugin@5.62.0)(eslint@8.57.0):
+ resolution: {integrity: sha512-3APeS/tQlTrFa167ThtP0Zm0vctjr4M44HMpeg1P4bK6wItarumq0Ma82xorMKdFsWpphQBlRPzw/pxiVELX1A==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ peerDependencies:
+ '@typescript-eslint/eslint-plugin': ^5.0.0
+ eslint: ^8.0.0
+ peerDependenciesMeta:
+ '@typescript-eslint/eslint-plugin':
+ optional: true
+ dependencies:
+ '@typescript-eslint/eslint-plugin': 5.62.0(@typescript-eslint/parser@5.62.0)(eslint@8.57.0)(typescript@5.4.5)
+ eslint: 8.57.0
+ eslint-rule-composer: 0.3.0
+ dev: true
+
+ /eslint-rule-composer@0.3.0:
+ resolution: {integrity: sha512-bt+Sh8CtDmn2OajxvNO+BX7Wn4CIWMpTRm3MaiKPCQcnnlm0CS2mhui6QaoeQugs+3Kj2ESKEEGJUdVafwhiCg==}
+ engines: {node: '>=4.0.0'}
+ dev: true
+
+ /eslint-scope@5.1.1:
+ resolution: {integrity: sha512-2NxwbF/hZ0KpepYN0cNbo+FN6XoK7GaHlQhgx/hIZl6Va0bF45RQOOwhLIy8lQDbuCiadSLCBnH2CFYquit5bw==}
+ engines: {node: '>=8.0.0'}
+ dependencies:
+ esrecurse: 4.3.0
+ estraverse: 4.3.0
+ dev: true
+
+ /eslint-scope@7.2.2:
+ resolution: {integrity: sha512-dOt21O7lTMhDM+X9mB4GX+DZrZtCUJPL/wlcTqxyrx5IvO0IYtILdtrQGQp+8n5S0gwSVmOf9NQrjMOgfQZlIg==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ dependencies:
+ esrecurse: 4.3.0
+ estraverse: 5.3.0
+ dev: true
+
+ /eslint-visitor-keys@3.4.3:
+ resolution: {integrity: sha512-wpc+LXeiyiisxPlEkUzU6svyS1frIO3Mgxj1fdy7Pm8Ygzguax2N3Fa/D/ag1WqbOprdI+uY6wMUl8/a2G+iag==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ dev: true
+
+ /eslint@8.57.0:
+ resolution: {integrity: sha512-dZ6+mexnaTIbSBZWgou51U6OmzIhYM2VcNdtiTtI7qPNZm35Akpr0f6vtw3w1Kmn5PYo+tZVfh13WrhpS6oLqQ==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ hasBin: true
+ dependencies:
+ '@eslint-community/eslint-utils': 4.4.0(eslint@8.57.0)
+ '@eslint-community/regexpp': 4.10.0
+ '@eslint/eslintrc': 2.1.4
+ '@eslint/js': 8.57.0
+ '@humanwhocodes/config-array': 0.11.14
+ '@humanwhocodes/module-importer': 1.0.1
+ '@nodelib/fs.walk': 1.2.8
+ '@ungap/structured-clone': 1.2.0
+ ajv: 6.12.6
+ chalk: 4.1.2
+ cross-spawn: 7.0.3
+ debug: 4.3.4
+ doctrine: 3.0.0
+ escape-string-regexp: 4.0.0
+ eslint-scope: 7.2.2
+ eslint-visitor-keys: 3.4.3
+ espree: 9.6.1
+ esquery: 1.5.0
+ esutils: 2.0.3
+ fast-deep-equal: 3.1.3
+ file-entry-cache: 6.0.1
+ find-up: 5.0.0
+ glob-parent: 6.0.2
+ globals: 13.24.0
+ graphemer: 1.4.0
+ ignore: 5.3.1
+ imurmurhash: 0.1.4
+ is-glob: 4.0.3
+ is-path-inside: 3.0.3
+ js-yaml: 4.1.0
+ json-stable-stringify-without-jsonify: 1.0.1
+ levn: 0.4.1
+ lodash.merge: 4.6.2
+ minimatch: 3.1.2
+ natural-compare: 1.4.0
+ optionator: 0.9.3
+ strip-ansi: 6.0.1
+ text-table: 0.2.0
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /espree@9.6.1:
+ resolution: {integrity: sha512-oruZaFkjorTpF32kDSI5/75ViwGeZginGGy2NoOSg3Q9bnwlnmDm4HLnkl0RE3n+njDXR037aY1+x58Z/zFdwQ==}
+ engines: {node: ^12.22.0 || ^14.17.0 || >=16.0.0}
+ dependencies:
+ acorn: 8.11.3
+ acorn-jsx: 5.3.2(acorn@8.11.3)
+ eslint-visitor-keys: 3.4.3
+ dev: true
+
+ /esprima@4.0.1:
+ resolution: {integrity: sha512-eGuFFw7Upda+g4p+QHvnW0RyTX/SVeJBDM/gCtMARO0cLuT2HcEKnTPvhjV6aGeqrCB/sbNop0Kszm0jsaWU4A==}
+ engines: {node: '>=4'}
+ hasBin: true
+ dev: true
+
+ /esquery@1.5.0:
+ resolution: {integrity: sha512-YQLXUplAwJgCydQ78IMJywZCceoqk1oH01OERdSAJc/7U2AylwjhSCLDEtqwg811idIS/9fIU5GjG73IgjKMVg==}
+ engines: {node: '>=0.10'}
+ dependencies:
+ estraverse: 5.3.0
+ dev: true
+
+ /esrecurse@4.3.0:
+ resolution: {integrity: sha512-KmfKL3b6G+RXvP8N1vr3Tq1kL/oCFgn2NYXEtqP8/L3pKapUA4G8cFVaoF3SU323CD4XypR/ffioHmkti6/Tag==}
+ engines: {node: '>=4.0'}
+ dependencies:
+ estraverse: 5.3.0
+ dev: true
+
+ /estraverse@4.3.0:
+ resolution: {integrity: sha512-39nnKffWz8xN1BU/2c79n9nB9HDzo0niYUqx6xyqUnyoAnQyyWpOTdZEeiCch8BBu515t4wp9ZmgVfVhn9EBpw==}
+ engines: {node: '>=4.0'}
+ dev: true
+
+ /estraverse@5.3.0:
+ resolution: {integrity: sha512-MMdARuVEQziNTeJD8DgMqmhwR11BRQ/cBP+pLtYdSTnf3MIO8fFeiINEbX36ZdNlfU/7A9f3gUw49B3oQsvwBA==}
+ engines: {node: '>=4.0'}
+ dev: true
+
+ /esutils@2.0.3:
+ resolution: {integrity: sha512-kVscqXk4OCp68SZ0dkgEKVi6/8ij300KBWTJq32P/dYeWTSwK41WyTxalN1eRmA5Z9UU/LX9D7FWSmV9SAYx6g==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /etag@1.8.1:
+ resolution: {integrity: sha512-aIL5Fx7mawVa300al2BnEE4iNvo1qETxLrPI/o05L7z6go7fCw1J6EQmbK4FmJ2AS7kgVF/KEZWufBfdClMcPg==}
+ engines: {node: '>= 0.6'}
+ dev: true
+
+ /eventemitter3@2.0.3:
+ resolution: {integrity: sha512-jLN68Dx5kyFHaePoXWPsCGW5qdyZQtLYHkxkg02/Mz6g0kYpDx4FyP6XfArhQdlOC4b8Mv+EMxPo/8La7Tzghg==}
+ dev: false
+
+ /eventemitter3@5.0.1:
+ resolution: {integrity: sha512-GWkBvjiSZK87ELrYOSESUYeVIc9mvLLf/nXalMOS5dYrgZq9o5OVkbZAVM06CVxYsCwH9BDZFPlQTlPA1j4ahA==}
+ dev: true
+
+ /events@3.3.0:
+ resolution: {integrity: sha512-mQw+2fkQbALzQ7V0MY0IqdnXNOeTtP4r0lN9z7AAawCXgqea7bDii20AYrIBrFd/Hx0M2Ocz6S111CaFkUcb0Q==}
+ engines: {node: '>=0.8.x'}
+ dev: true
+
+ /execa@5.1.1:
+ resolution: {integrity: sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==}
+ engines: {node: '>=10'}
+ dependencies:
+ cross-spawn: 7.0.3
+ get-stream: 6.0.1
+ human-signals: 2.1.0
+ is-stream: 2.0.1
+ merge-stream: 2.0.0
+ npm-run-path: 4.0.1
+ onetime: 5.1.2
+ signal-exit: 3.0.7
+ strip-final-newline: 2.0.0
+ dev: true
+
+ /execa@7.2.0:
+ resolution: {integrity: sha512-UduyVP7TLB5IcAQl+OzLyLcS/l32W/GLg+AhHJ+ow40FOk2U3SAllPwR44v4vmdFwIWqpdwxxpQbF1n5ta9seA==}
+ engines: {node: ^14.18.0 || ^16.14.0 || >=18.0.0}
+ dependencies:
+ cross-spawn: 7.0.3
+ get-stream: 6.0.1
+ human-signals: 4.3.1
+ is-stream: 3.0.0
+ merge-stream: 2.0.0
+ npm-run-path: 5.3.0
+ onetime: 6.0.0
+ signal-exit: 3.0.7
+ strip-final-newline: 3.0.0
+ dev: true
+
+ /execall@1.0.0:
+ resolution: {integrity: sha512-/J0Q8CvOvlAdpvhfkD/WnTQ4H1eU0exze2nFGPj/RSC7jpQ0NkKe2r28T5eMkhEEs+fzepMZNy1kVRKNlC04nQ==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ clone-regexp: 1.0.1
+ dev: true
+
+ /expand-brackets@2.1.4:
+ resolution: {integrity: sha512-w/ozOKR9Obk3qoWeY/WDi6MFta9AoMR+zud60mdnbniMcBxRuFJyDt2LdX/14A1UABeqk+Uk+LDfUpvoGKppZA==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ debug: 2.6.9
+ define-property: 0.2.5
+ extend-shallow: 2.0.1
+ posix-character-classes: 0.1.1
+ regex-not: 1.0.2
+ snapdragon: 0.8.2
+ to-regex: 3.0.2
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /extend-shallow@2.0.1:
+ resolution: {integrity: sha512-zCnTtlxNoAiDc3gqY2aYAWFx7XWWiasuF2K8Me5WbN8otHKTUKBwjPtNpRs/rbUZm7KxWAaNj7P1a/p52GbVug==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-extendable: 0.1.1
+ dev: true
+
+ /extend-shallow@3.0.2:
+ resolution: {integrity: sha512-BwY5b5Ql4+qZoefgMj2NUmx+tehVTH/Kf4k1ZEtOHNFcm2wSxMRo992l6X3TIgni2eZVTZ85xMOjF31fwZAj6Q==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ assign-symbols: 1.0.0
+ is-extendable: 1.0.1
+ dev: true
+
+ /extend@3.0.2:
+ resolution: {integrity: sha512-fjquC59cD7CyW6urNXK0FBufkZcoiGG80wTuPujX590cB5Ttln20E2UB4S/WARVqhXffZl2LNgS+gQdPIIim/g==}
+
+ /extglob@2.0.4:
+ resolution: {integrity: sha512-Nmb6QXkELsuBr24CJSkilo6UHHgbekK5UiZgfE6UHD3Eb27YC6oD+bhcT+tJ6cl8dmsgdQxnWlcry8ksBIBLpw==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ array-unique: 0.3.2
+ define-property: 1.0.0
+ expand-brackets: 2.1.4
+ extend-shallow: 2.0.1
+ fragment-cache: 0.2.1
+ regex-not: 1.0.2
+ snapdragon: 0.8.2
+ to-regex: 3.0.2
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /fast-deep-equal@3.1.3:
+ resolution: {integrity: sha512-f3qQ9oQy9j2AhBe/H9VC91wLmKBCCU/gDOnKNAYG5hswO7BLKj09Hc5HYNz9cGI++xlpDCIgDaitVs03ATR84Q==}
+
+ /fast-diff@1.1.2:
+ resolution: {integrity: sha512-KaJUt+M9t1qaIteSvjc6P3RbMdXsNhK61GRftR6SNxqmhthcd9MGIi4T+o0jD8LUSpSnSKXE20nLtJ3fOHxQig==}
+ dev: false
+
+ /fast-diff@1.3.0:
+ resolution: {integrity: sha512-VxPP4NqbUjj6MaAOafWeUn2cXWLcCtljklUtZf0Ind4XQ+QPtmA0b18zZy0jIQx+ExRVCR/ZQpBmik5lXshNsw==}
+ dev: true
+
+ /fast-glob@2.2.7:
+ resolution: {integrity: sha512-g1KuQwHOZAmOZMuBtHdxDtju+T2RT8jgCC9aANsbpdiDDTSnjgfuVsIBNKbUeJI3oKMRExcfNDtJl4OhbffMsw==}
+ engines: {node: '>=4.0.0'}
+ dependencies:
+ '@mrmlnc/readdir-enhanced': 2.2.1
+ '@nodelib/fs.stat': 1.1.3
+ glob-parent: 3.1.0
+ is-glob: 4.0.3
+ merge2: 1.4.1
+ micromatch: 3.1.10
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /fast-glob@3.3.2:
+ resolution: {integrity: sha512-oX2ruAFQwf/Orj8m737Y5adxDQO0LAB7/S5MnxCdTNDd4p6BsyIVsv9JQsATbTSq8KHRpLwIHbVlUNatxd+1Ow==}
+ engines: {node: '>=8.6.0'}
+ dependencies:
+ '@nodelib/fs.stat': 2.0.5
+ '@nodelib/fs.walk': 1.2.8
+ glob-parent: 5.1.2
+ merge2: 1.4.1
+ micromatch: 4.0.5
+ dev: true
+
+ /fast-json-stable-stringify@2.1.0:
+ resolution: {integrity: sha512-lhd/wF+Lk98HZoTCtlVraHtfh5XYijIjalXck7saUtuanSDyLMxnHhSXEDJqHxD7msR8D0uCmqlkwjCV8xvwHw==}
+ dev: true
+
+ /fast-levenshtein@2.0.6:
+ resolution: {integrity: sha512-DCXu6Ifhqcks7TZKY3Hxp3y6qphY5SJZmrWMDrKcERSOXWQdMhU9Ig/PYrzyw/ul9jOIyh0N4M0tbC5hodg8dw==}
+ dev: true
+
+ /fast-loops@1.1.3:
+ resolution: {integrity: sha512-8EZzEP0eKkEEVX+drtd9mtuQ+/QrlfW/5MlwcwK5Nds6EkZ/tRzEexkzUY2mIssnAyVLT+TKHuRXmFNNXYUd6g==}
+ dev: false
+
+ /fast-shallow-equal@1.0.0:
+ resolution: {integrity: sha512-HPtaa38cPgWvaCFmRNhlc6NG7pv6NUHqjPgVAkWGoB9mQMwYB27/K0CvOM5Czy+qpT3e8XJ6Q4aPAnzpNpzNaw==}
+ dev: false
+
+ /fastest-levenshtein@1.0.16:
+ resolution: {integrity: sha512-eRnCtTTtGZFpQCwhJiUOuxPQWRXVKYDn0b2PeHfXL6/Zi53SLAzAHfVhVWK2AryC/WH05kGfxhFIPvTF0SXQzg==}
+ engines: {node: '>= 4.9.1'}
+ dev: true
+
+ /fastest-stable-stringify@2.0.2:
+ resolution: {integrity: sha512-bijHueCGd0LqqNK9b5oCMHc0MluJAx0cwqASgbWMvkO01lCYgIhacVRLcaDz3QnyYIRNJRDwMb41VuT6pHJ91Q==}
+ dev: false
+
+ /fastq@1.17.1:
+ resolution: {integrity: sha512-sRVD3lWVIXWg6By68ZN7vho9a1pQcN/WBFaAAsDDFzlJjvoGx0P8z7V1t72grFJfJhu3YPZBuu25f7Kaw2jN1w==}
+ dependencies:
+ reusify: 1.0.4
+ dev: true
+
+ /fault@2.0.1:
+ resolution: {integrity: sha512-WtySTkS4OKev5JtpHXnib4Gxiurzh5NCGvWrFaZ34m6JehfTUhKZvn9njTfw48t6JumVQOmrKqpmGcdwxnhqBQ==}
+ dependencies:
+ format: 0.2.2
+ dev: false
+
+ /file-entry-cache@4.0.0:
+ resolution: {integrity: sha512-AVSwsnbV8vH/UVbvgEhf3saVQXORNv0ZzSkvkhQIaia5Tia+JhGTaa/ePUSVoPHQyGayQNmYfkzFi3WZV5zcpA==}
+ engines: {node: '>=4'}
+ dependencies:
+ flat-cache: 2.0.1
+ dev: true
+
+ /file-entry-cache@6.0.1:
+ resolution: {integrity: sha512-7Gps/XWymbLk2QLYK4NzpMOrYjMhdIxXuIvy2QBsLE6ljuodKvdkWs/cpyJJ3CVIVpH0Oi1Hvg1ovbMzLdFBBg==}
+ engines: {node: ^10.12.0 || >=12.0.0}
+ dependencies:
+ flat-cache: 3.2.0
+ dev: true
+
+ /file-entry-cache@7.0.2:
+ resolution: {integrity: sha512-TfW7/1iI4Cy7Y8L6iqNdZQVvdXn0f8B4QcIXmkIbtTIe/Okm/nSlHb4IwGzRVOd3WfSieCgvf5cMzEfySAIl0g==}
+ engines: {node: '>=12.0.0'}
+ dependencies:
+ flat-cache: 3.2.0
+ dev: true
+
+ /filesize@8.0.7:
+ resolution: {integrity: sha512-pjmC+bkIF8XI7fWaH8KxHcZL3DPybs1roSKP4rKDvy20tAWwIObE4+JIseG2byfGKhud5ZnM4YSGKBz7Sh0ndQ==}
+ engines: {node: '>= 0.4.0'}
+ dev: true
+
+ /fill-range@4.0.0:
+ resolution: {integrity: sha512-VcpLTWqWDiTerugjj8e3+esbg+skS3M9e54UuR3iCeIDMXCLTsAH8hTSzDQU/X6/6t3eYkOKoZSef2PlU6U1XQ==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ extend-shallow: 2.0.1
+ is-number: 3.0.0
+ repeat-string: 1.6.1
+ to-regex-range: 2.1.1
+ dev: true
+
+ /fill-range@7.0.1:
+ resolution: {integrity: sha512-qOo9F+dMUmC2Lcb4BbVvnKJxTPjCm+RRpe4gDuGrzkL7mEVl/djYSu2OdQ2Pa302N4oqkSg9ir6jaLWJ2USVpQ==}
+ engines: {node: '>=8'}
+ dependencies:
+ to-regex-range: 5.0.1
+
+ /find-root@1.1.0:
+ resolution: {integrity: sha512-NKfW6bec6GfKc0SGx1e07QZY9PE99u0Bft/0rzSD5k3sO/vwkVUpDUKVm5Gpp5Ue3YfShPFTX2070tDs5kB9Ng==}
+ dev: false
+
+ /find-up@2.1.0:
+ resolution: {integrity: sha512-NWzkk0jSJtTt08+FBFMvXoeZnOJD+jTtsRmBYbAIzJdX6l7dLgR7CTubCM5/eDdPUBvLCeVasP1brfVR/9/EZQ==}
+ engines: {node: '>=4'}
+ dependencies:
+ locate-path: 2.0.0
+ dev: true
+
+ /find-up@3.0.0:
+ resolution: {integrity: sha512-1yD6RmLI1XBfxugvORwlck6f75tYL+iR0jqwsOrOxMZyGYqUuDhJ0l4AXdO1iX/FTs9cBAMEk1gWSEx1kSbylg==}
+ engines: {node: '>=6'}
+ dependencies:
+ locate-path: 3.0.0
+ dev: true
+
+ /find-up@4.1.0:
+ resolution: {integrity: sha512-PpOwAdQ/YlXQ2vj8a3h8IipDuYRi3wceVQQGYWxNINccq40Anw7BlsEXCMbt1Zt+OLA6Fq9suIpIWD0OsnISlw==}
+ engines: {node: '>=8'}
+ dependencies:
+ locate-path: 5.0.0
+ path-exists: 4.0.0
+ dev: true
+
+ /find-up@5.0.0:
+ resolution: {integrity: sha512-78/PXT1wlLLDgTzDs7sjq9hzz0vXD+zn+7wypEe4fXQxCmdmqfGsEPQxmiCSQI3ajFV91bVSsvNtrJRiW6nGng==}
+ engines: {node: '>=10'}
+ dependencies:
+ locate-path: 6.0.0
+ path-exists: 4.0.0
+ dev: true
+
+ /flat-cache@2.0.1:
+ resolution: {integrity: sha512-LoQe6yDuUMDzQAEH8sgmh4Md6oZnc/7PjtwjNFSzveXqSHt6ka9fPBuso7IGf9Rz4uqnSnWiFH2B/zj24a5ReA==}
+ engines: {node: '>=4'}
+ dependencies:
+ flatted: 2.0.2
+ rimraf: 2.6.3
+ write: 1.0.3
+ dev: true
+
+ /flat-cache@3.2.0:
+ resolution: {integrity: sha512-CYcENa+FtcUKLmhhqyctpclsq7QF38pKjZHsGNiSQF5r4FtoKDWabFDl3hzaEQMvT1LHEysw5twgLvpYYb4vbw==}
+ engines: {node: ^10.12.0 || >=12.0.0}
+ dependencies:
+ flatted: 3.3.1
+ keyv: 4.5.4
+ rimraf: 3.0.2
+ dev: true
+
+ /flatted@2.0.2:
+ resolution: {integrity: sha512-r5wGx7YeOwNWNlCA0wQ86zKyDLMQr+/RB8xy74M4hTphfmjlijTSSXGuH8rnvKZnfT9i+75zmd8jcKdMR4O6jA==}
+ dev: true
+
+ /flatted@3.3.1:
+ resolution: {integrity: sha512-X8cqMLLie7KsNUDSdzeN8FYK9rEt4Dt67OsG/DNGnYTSDBG4uFAJFBnUeiV+zCVAvwFy56IjM9sH51jVaEhNxw==}
+ dev: true
+
+ /follow-redirects@1.15.6:
+ resolution: {integrity: sha512-wWN62YITEaOpSK584EZXJafH1AGpO8RVgElfkuXbTOrPX4fIfOyEpW/CsiNd8JdYrAoOvafRTOEnvsO++qCqFA==}
+ engines: {node: '>=4.0'}
+ peerDependencies:
+ debug: '*'
+ peerDependenciesMeta:
+ debug:
+ optional: true
+ dev: false
+
+ /for-each@0.3.3:
+ resolution: {integrity: sha512-jqYfLp7mo9vIyQf8ykW2v7A+2N4QjeCeI5+Dz9XraiO1ign81wjiH7Fb9vSOWvQfNtmSa4H2RoQTrrXivdUZmw==}
+ dependencies:
+ is-callable: 1.2.7
+ dev: true
+
+ /for-in@1.0.2:
+ resolution: {integrity: sha512-7EwmXrOjyL+ChxMhmG5lnW9MPt1aIeZEwKhQzoBUdTV0N3zuwWDZYVJatDvZ2OyzPUvdIAZDsCetk3coyMfcnQ==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /foreground-child@3.1.1:
+ resolution: {integrity: sha512-TMKDUnIte6bfb5nWv7V/caI169OHgvwjb7V4WkeUvbQQdjr5rWKqHFiKWb/fcOwB+CzBT+qbWjvj+DVwRskpIg==}
+ engines: {node: '>=14'}
+ dependencies:
+ cross-spawn: 7.0.3
+ signal-exit: 4.1.0
+ dev: true
+
+ /fork-ts-checker-webpack-plugin@6.5.3(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0):
+ resolution: {integrity: sha512-SbH/l9ikmMWycd5puHJKTkZJKddF4iRLyW3DeZ08HTI7NGyLS38MXd/KGgeWumQO7YNQbW2u/NtPT2YowbPaGQ==}
+ engines: {node: '>=10', yarn: '>=1.0.0'}
+ peerDependencies:
+ eslint: '>= 6'
+ typescript: '>= 2.7'
+ vue-template-compiler: '*'
+ webpack: '>= 4'
+ peerDependenciesMeta:
+ eslint:
+ optional: true
+ vue-template-compiler:
+ optional: true
+ dependencies:
+ '@babel/code-frame': 7.24.2
+ '@types/json-schema': 7.0.15
+ chalk: 4.1.2
+ chokidar: 3.6.0
+ cosmiconfig: 6.0.0
+ deepmerge: 4.3.1
+ eslint: 8.57.0
+ fs-extra: 9.1.0
+ glob: 7.2.3
+ memfs: 3.5.3
+ minimatch: 3.1.2
+ schema-utils: 2.7.0
+ semver: 7.6.0
+ tapable: 1.1.3
+ typescript: 5.4.5
+ webpack: 5.91.0
+ dev: true
+
+ /form-data@4.0.0:
+ resolution: {integrity: sha512-ETEklSGi5t0QMZuiXoA/Q6vcnxcLQP5vdugSpuAyi6SVGi2clPPp+xgEhuMaHC+zGgn31Kd235W35f7Hykkaww==}
+ engines: {node: '>= 6'}
+ dependencies:
+ asynckit: 0.4.0
+ combined-stream: 1.0.8
+ mime-types: 2.1.35
+ dev: false
+
+ /format@0.2.2:
+ resolution: {integrity: sha512-wzsgA6WOq+09wrU1tsJ09udeR/YZRaeArL9e1wPbFg3GG2yDnC2ldKpxs4xunpFF9DgqCqOIra3bc1HWrJ37Ww==}
+ engines: {node: '>=0.4.x'}
+ dev: false
+
+ /fraction.js@4.3.7:
+ resolution: {integrity: sha512-ZsDfxO51wGAXREY55a7la9LScWpwv9RxIrYABrlvOFBlH/ShPnrtsXeuUIfXKKOVicNxQ+o8JTbJvjS4M89yew==}
+ dev: true
+
+ /fragment-cache@0.2.1:
+ resolution: {integrity: sha512-GMBAbW9antB8iZRHLoGw0b3HANt57diZYFO/HL1JGIC1MjKrdmhxvrJbupnVvpys0zsz7yBApXdQyfepKly2kA==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ map-cache: 0.2.2
+ dev: true
+
+ /framer-motion@10.18.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-oGlDh1Q1XqYPksuTD/usb0I70hq95OUzmL9+6Zd+Hs4XV0oaISBa/UUMSjYiq6m8EUF32132mOJ8xVZS+I0S6w==}
+ peerDependencies:
+ react: ^18.0.0
+ react-dom: ^18.0.0
+ peerDependenciesMeta:
+ react:
+ optional: true
+ react-dom:
+ optional: true
+ dependencies:
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ tslib: 2.6.2
+ optionalDependencies:
+ '@emotion/is-prop-valid': 0.8.8
+ dev: false
+
+ /fs-extra@10.1.0:
+ resolution: {integrity: sha512-oRXApq54ETRj4eMiFzGnHWGy+zo5raudjuxN0b8H7s/RU2oW0Wvsx9O0ACRN/kRq9E8Vu/ReskGB5o3ji+FzHQ==}
+ engines: {node: '>=12'}
+ dependencies:
+ graceful-fs: 4.2.11
+ jsonfile: 6.1.0
+ universalify: 2.0.1
+ dev: true
+
+ /fs-extra@11.2.0:
+ resolution: {integrity: sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==}
+ engines: {node: '>=14.14'}
+ dependencies:
+ graceful-fs: 4.2.11
+ jsonfile: 6.1.0
+ universalify: 2.0.1
+ dev: true
+
+ /fs-extra@9.1.0:
+ resolution: {integrity: sha512-hcg3ZmepS30/7BSFqRvoo3DOMQu7IjqxO5nCDt+zM9XWjb33Wg7ziNT+Qvqbuc3+gWpzO02JubVyk2G4Zvo1OQ==}
+ engines: {node: '>=10'}
+ dependencies:
+ at-least-node: 1.0.0
+ graceful-fs: 4.2.11
+ jsonfile: 6.1.0
+ universalify: 2.0.1
+ dev: true
+
+ /fs-monkey@1.0.5:
+ resolution: {integrity: sha512-8uMbBjrhzW76TYgEV27Y5E//W2f/lTFmx78P2w19FZSxarhI/798APGQyuGCwmkNxgwGRhrLfvWyLBvNtuOmew==}
+ dev: true
+
+ /fs.realpath@1.0.0:
+ resolution: {integrity: sha512-OO0pH2lK6a0hZnAdau5ItzHPI6pUlvI7jMVnxUQRtw4owF2wk8lOSabtGDCTP4Ggrg2MbGnWO9X8K1t4+fGMDw==}
+ dev: true
+
+ /fsevents@2.3.3:
+ resolution: {integrity: sha512-5xoDfX+fL7faATnagmWPpbFtwh/R77WmMMqqHGS65C3vvB0YHrgF+B1YmZ3441tMj5n63k0212XNoJwzlhffQw==}
+ engines: {node: ^8.16.0 || ^10.6.0 || >=11.0.0}
+ os: [darwin]
+ requiresBuild: true
+ optional: true
+
+ /function-bind@1.1.2:
+ resolution: {integrity: sha512-7XHNxH7qX9xG5mIwxkhumTox/MIRNcOgDrxWsMt2pAr23WHp6MrRlN7FBSFpCpr+oVO0F744iUgR82nJMfG2SA==}
+
+ /function.prototype.name@1.1.6:
+ resolution: {integrity: sha512-Z5kx79swU5P27WEayXM1tBi5Ze/lbIyiNgU3qyXUOf9b2rgXYyF9Dy9Cx+IQv/Lc8WCG6L82zwUPpSS9hGehIg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ functions-have-names: 1.2.3
+ dev: true
+
+ /functions-have-names@1.2.3:
+ resolution: {integrity: sha512-xckBUXyTIqT97tq2x2AMb+g163b5JFysYk0x4qxNFwbfQkmNZoiRHb6sPzI9/QV33WeuvVYBUIiD4NzNIyqaRQ==}
+
+ /gensync@1.0.0-beta.2:
+ resolution: {integrity: sha512-3hN7NaskYvMDLQY55gnW3NQ+mesEAepTqlg+VEbj7zzqEMBVNhzcGYYeqFo/TlYz6eQiFcp1HcsCZO+nGgS8zg==}
+ engines: {node: '>=6.9.0'}
+
+ /get-caller-file@2.0.5:
+ resolution: {integrity: sha512-DyFP3BM/3YHTQOCUL/w0OZHR0lpKeGrxotcHWcqNEdnltqFwXVfhEBQ94eIo34AfQpo0rGki4cyIiftY06h2Fg==}
+ engines: {node: 6.* || 8.* || >= 10.*}
+ dev: true
+
+ /get-intrinsic@1.2.4:
+ resolution: {integrity: sha512-5uYhsJH8VJBTv7oslg4BznJYhDoRI6waYCxMmCdnTrcCrHA/fCFKoTFz2JKKE0HdDFUF7/oQuhzumXJK7paBRQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ es-errors: 1.3.0
+ function-bind: 1.1.2
+ has-proto: 1.0.3
+ has-symbols: 1.0.3
+ hasown: 2.0.2
+
+ /get-stdin@6.0.0:
+ resolution: {integrity: sha512-jp4tHawyV7+fkkSKyvjuLZswblUtz+SQKzSWnBbii16BuZksJlU1wuBYXY75r+duh/llF1ur6oNwi+2ZzjKZ7g==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /get-stream@6.0.1:
+ resolution: {integrity: sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /get-symbol-description@1.0.2:
+ resolution: {integrity: sha512-g0QYk1dZBxGwk+Ngc+ltRH2IBp2f7zBkBMBJZCDerh6EhlhSR6+9irMCuT/09zD6qkarHUSn529sK/yL4S27mg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ es-errors: 1.3.0
+ get-intrinsic: 1.2.4
+ dev: true
+
+ /get-value@2.0.6:
+ resolution: {integrity: sha512-Ln0UQDlxH1BapMu3GPtf7CuYNwRZf2gwCuPqbyG6pB8WfmFpzqcy4xtAaAMUhnNqjMKTiCPZG2oMT3YSx8U2NA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /git-raw-commits@2.0.11:
+ resolution: {integrity: sha512-VnctFhw+xfj8Va1xtfEqCUD2XDrbAPSJx+hSrE5K7fGdjZruW7XV+QOrN7LF/RJyvspRiD2I0asWsxFp0ya26A==}
+ engines: {node: '>=10'}
+ hasBin: true
+ dependencies:
+ dargs: 7.0.0
+ lodash: 4.17.21
+ meow: 8.1.2
+ split2: 3.2.2
+ through2: 4.0.2
+ dev: true
+
+ /glob-parent@3.1.0:
+ resolution: {integrity: sha512-E8Ak/2+dZY6fnzlR7+ueWvhsH1SjHr4jjss4YS/h4py44jY9MhK/VFdaZJAWDz6BbL21KeteKxFSFpq8OS5gVA==}
+ dependencies:
+ is-glob: 3.1.0
+ path-dirname: 1.0.2
+ dev: true
+
+ /glob-parent@5.1.2:
+ resolution: {integrity: sha512-AOIgSQCepiJYwP3ARnGx+5VnTu2HBYdzbGP45eLw1vr3zB3vZLeyed1sC9hnbcOc9/SrMyM5RPQrkGz4aS9Zow==}
+ engines: {node: '>= 6'}
+ dependencies:
+ is-glob: 4.0.3
+
+ /glob-parent@6.0.2:
+ resolution: {integrity: sha512-XxwI8EOhVQgWp6iDL+3b0r86f4d6AX6zSU55HfB4ydCEuXLXc5FcYeOu+nnGftS4TEju/11rt4KJPTMgbfmv4A==}
+ engines: {node: '>=10.13.0'}
+ dependencies:
+ is-glob: 4.0.3
+ dev: true
+
+ /glob-to-regexp@0.3.0:
+ resolution: {integrity: sha512-Iozmtbqv0noj0uDDqoL0zNq0VBEfK2YFoMAZoxJe4cwphvLR+JskfF30QhXHOR4m3KrE6NLRYw+U9MRXvifyig==}
+ dev: true
+
+ /glob-to-regexp@0.4.1:
+ resolution: {integrity: sha512-lkX1HJXwyMcprw/5YUZc2s7DrpAiHB21/V+E1rHUrVNokkvB6bqMzT0VfV6/86ZNabt1k14YOIaT7nDvOX3Iiw==}
+ dev: true
+
+ /glob@10.3.12:
+ resolution: {integrity: sha512-TCNv8vJ+xz4QiqTpfOJA7HvYv+tNIRHKfUWw/q+v2jdgN4ebz+KY9tGx5J4rHP0o84mNP+ApH66HRX8us3Khqg==}
+ engines: {node: '>=16 || 14 >=14.17'}
+ hasBin: true
+ dependencies:
+ foreground-child: 3.1.1
+ jackspeak: 2.3.6
+ minimatch: 9.0.4
+ minipass: 7.0.4
+ path-scurry: 1.10.2
+ dev: true
+
+ /glob@7.2.3:
+ resolution: {integrity: sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==}
+ dependencies:
+ fs.realpath: 1.0.0
+ inflight: 1.0.6
+ inherits: 2.0.4
+ minimatch: 3.1.2
+ once: 1.4.0
+ path-is-absolute: 1.0.1
+ dev: true
+
+ /global-dirs@0.1.1:
+ resolution: {integrity: sha512-NknMLn7F2J7aflwFOlGdNIuCDpN3VGoSoB+aap3KABFWbHVn1TCgFC+np23J8W2BiZbjfEw3BFBycSMv1AFblg==}
+ engines: {node: '>=4'}
+ dependencies:
+ ini: 1.3.8
+ dev: true
+
+ /global-modules@2.0.0:
+ resolution: {integrity: sha512-NGbfmJBp9x8IxyJSd1P+otYK8vonoJactOogrVfFRIAEY1ukil8RSKDz2Yo7wh1oihl51l/r6W4epkeKJHqL8A==}
+ engines: {node: '>=6'}
+ dependencies:
+ global-prefix: 3.0.0
+ dev: true
+
+ /global-prefix@3.0.0:
+ resolution: {integrity: sha512-awConJSVCHVGND6x3tmMaKcQvwXLhjdkmomy2W+Goaui8YPgYgXJZewhg3fWC+DlfqqQuWg8AwqjGTD2nAPVWg==}
+ engines: {node: '>=6'}
+ dependencies:
+ ini: 1.3.8
+ kind-of: 6.0.3
+ which: 1.3.1
+ dev: true
+
+ /globals@11.12.0:
+ resolution: {integrity: sha512-WOBp/EEGUiIsJSp7wcv/y6MO+lV9UoncWqxuFfm8eBwzWNgyfBd6Gz+IeKQ9jCmyhoH99g15M3T+QaVHFjizVA==}
+ engines: {node: '>=4'}
+
+ /globals@13.24.0:
+ resolution: {integrity: sha512-AhO5QUcj8llrbG09iWhPU2B204J1xnPeL8kQmVorSsy+Sjj1sk8gIyh6cUocGmH4L0UuhAJy+hJMRA4mgA4mFQ==}
+ engines: {node: '>=8'}
+ dependencies:
+ type-fest: 0.20.2
+ dev: true
+
+ /globalthis@1.0.3:
+ resolution: {integrity: sha512-sFdI5LyBiNTHjRd7cGPWapiHWMOXKyuBNX/cWJ3NfzrZQVa8GI/8cofCl74AOVqq9W5kNmguTIzJ/1s2gyI9wA==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ define-properties: 1.2.1
+ dev: true
+
+ /globby@11.1.0:
+ resolution: {integrity: sha512-jhIXaOzy1sb8IyocaruWSn1TjmnBVs8Ayhcy83rmxNJ8q2uWKCAj3CnJY+KpGSXCueAPc0i05kVvVKtP1t9S3g==}
+ engines: {node: '>=10'}
+ dependencies:
+ array-union: 2.1.0
+ dir-glob: 3.0.1
+ fast-glob: 3.3.2
+ ignore: 5.3.1
+ merge2: 1.4.1
+ slash: 3.0.0
+ dev: true
+
+ /globby@9.2.0:
+ resolution: {integrity: sha512-ollPHROa5mcxDEkwg6bPt3QbEf4pDQSNtd6JPL1YvOvAo/7/0VAm9TccUeoTmarjPw4pfUthSCqcyfNB1I3ZSg==}
+ engines: {node: '>=6'}
+ dependencies:
+ '@types/glob': 7.2.0
+ array-union: 1.0.2
+ dir-glob: 2.2.2
+ fast-glob: 2.2.7
+ glob: 7.2.3
+ ignore: 4.0.6
+ pify: 4.0.1
+ slash: 2.0.0
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /globjoin@0.1.4:
+ resolution: {integrity: sha512-xYfnw62CKG8nLkZBfWbhWwDw02CHty86jfPcc2cr3ZfeuK9ysoVPPEUxf21bAD/rWAgk52SuBrLJlefNy8mvFg==}
+ dev: true
+
+ /globrex@0.1.2:
+ resolution: {integrity: sha512-uHJgbwAMwNFf5mLst7IWLNg14x1CkeqglJb/K3doi4dw6q2IvAAmM/Y81kevy83wP+Sst+nutFTYOGg3d1lsxg==}
+ dev: true
+
+ /gonzales-pe@4.3.0:
+ resolution: {integrity: sha512-otgSPpUmdWJ43VXyiNgEYE4luzHCL2pz4wQ0OnDluC6Eg4Ko3Vexy/SrSynglw/eR+OhkzmqFCZa/OFa/RgAOQ==}
+ engines: {node: '>=0.6.0'}
+ hasBin: true
+ dependencies:
+ minimist: 1.2.8
+ dev: true
+
+ /gopd@1.0.1:
+ resolution: {integrity: sha512-d65bNlIadxvpb/A2abVdlqKqV563juRnZ1Wtk6s1sIR8uNsXR70xqIzVqxVf1eTqDunwT2MkczEeaezCKTZhwA==}
+ dependencies:
+ get-intrinsic: 1.2.4
+
+ /graceful-fs@4.2.11:
+ resolution: {integrity: sha512-RbJ5/jmFcNNCcDV5o9eTnBLJ/HszWV0P73bc+Ff4nS/rJj+YaS6IGyiOL0VoBYX+l1Wrl3k63h/KrH+nhJ0XvQ==}
+ dev: true
+
+ /graphemer@1.4.0:
+ resolution: {integrity: sha512-EtKwoO6kxCL9WO5xipiHTZlSzBm7WLT627TqC/uVRd0HKmq8NXyebnNYxDoBi7wt8eTWrUrKXCOVaFq9x1kgag==}
+ dev: true
+
+ /gzip-size@6.0.0:
+ resolution: {integrity: sha512-ax7ZYomf6jqPTQ4+XCpUGyXKHk5WweS+e05MBO4/y3WJ5RkmPXNKvX+bx1behVILVwr6JSQvZAku021CHPXG3Q==}
+ engines: {node: '>=10'}
+ dependencies:
+ duplexer: 0.1.2
+ dev: true
+
+ /hard-rejection@2.1.0:
+ resolution: {integrity: sha512-VIZB+ibDhx7ObhAe7OVtoEbuP4h/MuOTHJ+J8h/eBXotJYl0fBgR72xDFCKgIh22OJZIOVNxBMWuhAr10r8HdA==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /has-ansi@2.0.0:
+ resolution: {integrity: sha512-C8vBJ8DwUCx19vhm7urhTuUsr4/IyP6l4VzNQDv+ryHQObW3TTTp9yB68WpYgRe2bbaGuZ/se74IqFeVnMnLZg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ ansi-regex: 2.1.1
+ dev: true
+
+ /has-bigints@1.0.2:
+ resolution: {integrity: sha512-tSvCKtBr9lkF0Ex0aQiP9N+OpV4zi2r/Nee5VkRDbaqv35RLYMzbwQfFSZZH0kR+Rd6302UJZ2p/bJCEoR3VoQ==}
+ dev: true
+
+ /has-flag@1.0.0:
+ resolution: {integrity: sha512-DyYHfIYwAJmjAjSSPKANxI8bFY9YtFrgkAfinBojQ8YJTOuOuav64tMUJv584SES4xl74PmuaevIyaLESHdTAA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /has-flag@3.0.0:
+ resolution: {integrity: sha512-sKJf1+ceQBr4SMkvQnBDNDtf4TXpVhVGateu0t918bl30FnbE2m4vNLX+VWe/dpjlb+HugGYzW7uQXH98HPEYw==}
+ engines: {node: '>=4'}
+
+ /has-flag@4.0.0:
+ resolution: {integrity: sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /has-property-descriptors@1.0.2:
+ resolution: {integrity: sha512-55JNKuIW+vq4Ke1BjOTjM2YctQIvCT7GFzHwmfZPGo5wnrgkid0YQtnAleFSqumZm4az3n2BS+erby5ipJdgrg==}
+ dependencies:
+ es-define-property: 1.0.0
+
+ /has-proto@1.0.3:
+ resolution: {integrity: sha512-SJ1amZAJUiZS+PhsVLf5tGydlaVB8EdFpaSO4gmiUKUOxk8qzn5AIy4ZeJUmh22znIdk/uMAUT2pl3FxzVUH+Q==}
+ engines: {node: '>= 0.4'}
+
+ /has-symbols@1.0.3:
+ resolution: {integrity: sha512-l3LCuF6MgDNwTDKkdYGEihYjt5pRPbEg46rtlmnSPlUbgmB8LOIrKJbYYFBSbnPaJexMKtiPO8hmeRjRz2Td+A==}
+ engines: {node: '>= 0.4'}
+
+ /has-tostringtag@1.0.2:
+ resolution: {integrity: sha512-NqADB8VjPFLM2V0VvHUewwwsw0ZWBaIdgo+ieHtK3hasLz4qeCRjYcqfB6AQrBggRKppKF8L52/VqdVsO47Dlw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ has-symbols: 1.0.3
+
+ /has-value@0.3.1:
+ resolution: {integrity: sha512-gpG936j8/MzaeID5Yif+577c17TxaDmhuyVgSwtnL/q8UUTySg8Mecb+8Cf1otgLoD7DDH75axp86ER7LFsf3Q==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ get-value: 2.0.6
+ has-values: 0.1.4
+ isobject: 2.1.0
+ dev: true
+
+ /has-value@1.0.0:
+ resolution: {integrity: sha512-IBXk4GTsLYdQ7Rvt+GRBrFSVEkmuOUy4re0Xjd9kJSUQpnTrWR4/y9RpfexN9vkAPMFuQoeWKwqzPozRTlasGw==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ get-value: 2.0.6
+ has-values: 1.0.0
+ isobject: 3.0.1
+ dev: true
+
+ /has-values@0.1.4:
+ resolution: {integrity: sha512-J8S0cEdWuQbqD9//tlZxiMuMNmxB8PlEwvYwuxsTmR1G5RXUePEX/SJn7aD0GMLieuZYSwNH0cQuJGwnYunXRQ==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /has-values@1.0.0:
+ resolution: {integrity: sha512-ODYZC64uqzmtfGMEAX/FvZiRyWLpAC3vYnNunURUnkGVTS+mI0smVsWaPydRBsE3g+ok7h960jChO8mFcWlHaQ==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-number: 3.0.0
+ kind-of: 4.0.0
+ dev: true
+
+ /hasown@2.0.2:
+ resolution: {integrity: sha512-0hJU9SCPvmMzIBdZFqNPXWa6dqh7WdH0cII9y+CyS8rG3nL48Bclra9HmKhVVUHyPWNH5Y7xDwAB7bfgSjkUMQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ function-bind: 1.1.2
+
+ /hast-util-from-parse5@7.1.2:
+ resolution: {integrity: sha512-Nz7FfPBuljzsN3tCQ4kCBKqdNhQE2l0Tn+X1ubgKBPRoiDIu1mL08Cfw4k7q71+Duyaw7DXDN+VTAp4Vh3oCOw==}
+ dependencies:
+ '@types/hast': 2.3.10
+ '@types/unist': 2.0.10
+ hastscript: 7.2.0
+ property-information: 6.5.0
+ vfile: 5.3.7
+ vfile-location: 4.1.0
+ web-namespaces: 2.0.1
+ dev: false
+
+ /hast-util-is-element@2.1.3:
+ resolution: {integrity: sha512-O1bKah6mhgEq2WtVMk+Ta5K7pPMqsBBlmzysLdcwKVrqzZQ0CHqUPiIVspNhAG1rvxpvJjtGee17XfauZYKqVA==}
+ dependencies:
+ '@types/hast': 2.3.10
+ '@types/unist': 2.0.10
+ dev: false
+
+ /hast-util-parse-selector@3.1.1:
+ resolution: {integrity: sha512-jdlwBjEexy1oGz0aJ2f4GKMaVKkA9jwjr4MjAAI22E5fM/TXVZHuS5OpONtdeIkRKqAaryQ2E9xNQxijoThSZA==}
+ dependencies:
+ '@types/hast': 2.3.10
+ dev: false
+
+ /hast-util-raw@7.2.3:
+ resolution: {integrity: sha512-RujVQfVsOrxzPOPSzZFiwofMArbQke6DJjnFfceiEbFh7S05CbPt0cYN+A5YeD3pso0JQk6O1aHBnx9+Pm2uqg==}
+ dependencies:
+ '@types/hast': 2.3.10
+ '@types/parse5': 6.0.3
+ hast-util-from-parse5: 7.1.2
+ hast-util-to-parse5: 7.1.0
+ html-void-elements: 2.0.1
+ parse5: 6.0.1
+ unist-util-position: 4.0.4
+ unist-util-visit: 4.1.2
+ vfile: 5.3.7
+ web-namespaces: 2.0.1
+ zwitch: 2.0.4
+ dev: false
+
+ /hast-util-to-parse5@7.1.0:
+ resolution: {integrity: sha512-YNRgAJkH2Jky5ySkIqFXTQiaqcAtJyVE+D5lkN6CdtOqrnkLfGYYrEcKuHOJZlp+MwjSwuD3fZuawI+sic/RBw==}
+ dependencies:
+ '@types/hast': 2.3.10
+ comma-separated-tokens: 2.0.3
+ property-information: 6.5.0
+ space-separated-tokens: 2.0.2
+ web-namespaces: 2.0.1
+ zwitch: 2.0.4
+ dev: false
+
+ /hast-util-to-text@3.1.2:
+ resolution: {integrity: sha512-tcllLfp23dJJ+ju5wCCZHVpzsQQ43+moJbqVX3jNWPB7z/KFC4FyZD6R7y94cHL6MQ33YtMZL8Z0aIXXI4XFTw==}
+ dependencies:
+ '@types/hast': 2.3.10
+ '@types/unist': 2.0.10
+ hast-util-is-element: 2.1.3
+ unist-util-find-after: 4.0.1
+ dev: false
+
+ /hast-util-whitespace@2.0.1:
+ resolution: {integrity: sha512-nAxA0v8+vXSBDt3AnRUNjyRIQ0rD+ntpbAp4LnPkumc5M9yUbSMa4XDU9Q6etY4f1Wp4bNgvc1yjiZtsTTrSng==}
+ dev: false
+
+ /hastscript@7.2.0:
+ resolution: {integrity: sha512-TtYPq24IldU8iKoJQqvZOuhi5CyCQRAbvDOX0x1eW6rsHSxa/1i2CCiptNTotGHJ3VoHRGmqiv6/D3q113ikkw==}
+ dependencies:
+ '@types/hast': 2.3.10
+ comma-separated-tokens: 2.0.3
+ hast-util-parse-selector: 3.1.1
+ property-information: 6.5.0
+ space-separated-tokens: 2.0.2
+ dev: false
+
+ /he@1.2.0:
+ resolution: {integrity: sha512-F/1DnUGPopORZi0ni+CvrCgHQ5FyEAHRLSApuYWMmrbSwoN2Mn/7k+Gl38gJnR7yyDZk6WLXwiGod1JOWNDKGw==}
+ hasBin: true
+ dev: true
+
+ /highlight.js@11.8.0:
+ resolution: {integrity: sha512-MedQhoqVdr0U6SSnWPzfiadUcDHfN/Wzq25AkXiQv9oiOO/sG0S7XkvpFIqWBl9Yq1UYyYOOVORs5UW2XlPyzg==}
+ engines: {node: '>=12.0.0'}
+ dev: false
+
+ /highlight.js@11.9.0:
+ resolution: {integrity: sha512-fJ7cW7fQGCYAkgv4CPfwFHrfd/cLS4Hau96JuJ+ZTOWhjnhoeN1ub1tFmALm/+lW5z4WCAuAV9bm05AP0mS6Gw==}
+ engines: {node: '>=12.0.0'}
+ dev: false
+
+ /hoist-non-react-statics@3.3.2:
+ resolution: {integrity: sha512-/gGivxi8JPKWNm/W0jSmzcMPpfpPLc3dY/6GxhX2hQ9iGj3aDfklV4ET7NjKpSinLpJ5vafa9iiGIEZg10SfBw==}
+ dependencies:
+ react-is: 16.13.1
+
+ /hosted-git-info@2.8.9:
+ resolution: {integrity: sha512-mxIDAb9Lsm6DoOJ7xH+5+X4y1LU/4Hi50L9C5sIswK3JzULS4bwk1FvjdBgvYR4bzT4tuUQiC15FE2f5HbLvYw==}
+ dev: true
+
+ /hosted-git-info@4.1.0:
+ resolution: {integrity: sha512-kyCuEOWjJqZuDbRHzL8V93NzQhwIB71oFWSyzVo+KPZI+pnQPPxucdkrOZvkLRnrf5URsQM+IJ09Dw29cRALIA==}
+ engines: {node: '>=10'}
+ dependencies:
+ lru-cache: 6.0.0
+ dev: true
+
+ /hotkeys-js@3.13.7:
+ resolution: {integrity: sha512-ygFIdTqqwG4fFP7kkiYlvayZppeIQX2aPpirsngkv1xM1lP0piDY5QEh68nQnIKvz64hfocxhBaD/uK3sSK1yQ==}
+ dev: true
+
+ /html-parse-stringify@3.0.1:
+ resolution: {integrity: sha512-KknJ50kTInJ7qIScF3jeaFRpMpE8/lfiTdzf/twXyPBLAGrLRTmkz3AdTnKeh40X8k9L2fdYwEp/42WGXIRGcg==}
+ dependencies:
+ void-elements: 3.1.0
+ dev: false
+
+ /html-tags@2.0.0:
+ resolution: {integrity: sha512-+Il6N8cCo2wB/Vd3gqy/8TZhTD3QvcVeQLCnZiGkGCH3JP28IgGAY41giccp2W4R3jfyJPAP318FQTa1yU7K7g==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /html-tags@3.3.1:
+ resolution: {integrity: sha512-ztqyC3kLto0e9WbNp0aeP+M3kTt+nbaIveGmUxAtZa+8iFgKLUOD4YKM5j+f3QD89bra7UeumolZHKuOXnTmeQ==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /html-void-elements@2.0.1:
+ resolution: {integrity: sha512-0quDb7s97CfemeJAnW9wC0hw78MtW7NU3hqtCD75g2vFlDLt36llsYD7uB7SUzojLMP24N5IatXf7ylGXiGG9A==}
+ dev: false
+
+ /htmlparser2@3.10.1:
+ resolution: {integrity: sha512-IgieNijUMbkDovyoKObU1DUhm1iwNYE/fuifEoEHfd1oZKZDaONBSkal7Y01shxsM49R4XaMdGez3WnF9UfiCQ==}
+ dependencies:
+ domelementtype: 1.3.1
+ domhandler: 2.4.2
+ domutils: 1.7.0
+ entities: 1.1.2
+ inherits: 2.0.4
+ readable-stream: 3.6.2
+ dev: true
+
+ /human-signals@2.1.0:
+ resolution: {integrity: sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==}
+ engines: {node: '>=10.17.0'}
+ dev: true
+
+ /human-signals@4.3.1:
+ resolution: {integrity: sha512-nZXjEF2nbo7lIw3mgYjItAfgQXog3OjJogSbKa2CQIIvSGWcKgeJnQlNXip6NglNzYH45nSRiEVimMvYL8DDqQ==}
+ engines: {node: '>=14.18.0'}
+ dev: true
+
+ /humps@2.0.1:
+ resolution: {integrity: sha512-E0eIbrFWUhwfXJmsbdjRQFQPrl5pTEoKlz163j1mTqqUnU9PgR4AgB8AIITzuB3vLBdxZXyZ9TDIrwB2OASz4g==}
+ dev: false
+
+ /husky@8.0.3:
+ resolution: {integrity: sha512-+dQSyqPh4x1hlO1swXBiNb2HzTDN1I2IGLQx1GrBuiqFJfoMrnZWwVmatvSiO+Iz8fBUnf+lekwNo4c2LlXItg==}
+ engines: {node: '>=14'}
+ hasBin: true
+ dev: true
+
+ /hyphenate-style-name@1.0.4:
+ resolution: {integrity: sha512-ygGZLjmXfPHj+ZWh6LwbC37l43MhfztxetbFCoYTM2VjkIUpeHgSNn7QIyVFj7YQ1Wl9Cbw5sholVJPzWvC2MQ==}
+ dev: false
+
+ /i18next-browser-languagedetector@7.2.1:
+ resolution: {integrity: sha512-h/pM34bcH6tbz8WgGXcmWauNpQupCGr25XPp9cZwZInR9XHSjIFDYp1SIok7zSPsTOMxdvuLyu86V+g2Kycnfw==}
+ dependencies:
+ '@babel/runtime': 7.24.4
+ dev: false
+
+ /i18next@23.11.2:
+ resolution: {integrity: sha512-qMBm7+qT8jdpmmDw/kQD16VpmkL9BdL+XNAK5MNbNFaf1iQQq35ZbPrSlqmnNPOSUY4m342+c0t0evinF5l7sA==}
+ dependencies:
+ '@babel/runtime': 7.24.4
+ dev: false
+
+ /ignore@4.0.6:
+ resolution: {integrity: sha512-cyFDKrqc/YdcWFniJhzI42+AzS+gNwmUzOSFcRCQYwySuBBBy/KjuxWLZ/FHEH6Moq1NizMOBWyTcv8O4OZIMg==}
+ engines: {node: '>= 4'}
+ dev: true
+
+ /ignore@5.3.1:
+ resolution: {integrity: sha512-5Fytz/IraMjqpwfd34ke28PTVMjZjJG2MPn5t7OE4eUCUNf8BAa7b5WUS9/Qvr6mwOQS7Mk6vdsMno5he+T8Xw==}
+ engines: {node: '>= 4'}
+ dev: true
+
+ /image-size@0.5.5:
+ resolution: {integrity: sha512-6TDAlDPZxUFCv+fuOkIoXT/V/f3Qbq8e37p+YOiYrUv3v9cc3/6x78VdfPgFVaB9dZYeLUfKgHRebpkm/oP2VQ==}
+ engines: {node: '>=0.10.0'}
+ hasBin: true
+ dev: true
+
+ /immer@9.0.21:
+ resolution: {integrity: sha512-bc4NBHqOqSfRW7POMkHd51LvClaeMXpm8dx0e8oE2GORbq5aRK7Bxl4FyzVLdGtLmvLKL7BTDBG5ACQm4HWjTA==}
+ dev: true
+
+ /immutable@4.3.5:
+ resolution: {integrity: sha512-8eabxkth9gZatlwl5TBuJnCsoTADlL6ftEr7A4qgdaTsPyreilDSnUk57SO+jfKcNtxPa22U5KK6DSeAYhpBJw==}
+
+ /import-fresh@2.0.0:
+ resolution: {integrity: sha512-eZ5H8rcgYazHbKC3PG4ClHNykCSxtAhxSSEM+2mb+7evD2CKF5V7c0dNum7AdpDh0ZdICwZY9sRSn8f+KH96sg==}
+ engines: {node: '>=4'}
+ dependencies:
+ caller-path: 2.0.0
+ resolve-from: 3.0.0
+ dev: true
+
+ /import-fresh@3.3.0:
+ resolution: {integrity: sha512-veYYhQa+D1QBKznvhUHxb8faxlrwUnxseDAbAp457E0wLNio2bOSKnjYDhMj+YiAq61xrMGhQk9iXVk5FzgQMw==}
+ engines: {node: '>=6'}
+ dependencies:
+ parent-module: 1.0.1
+ resolve-from: 4.0.0
+
+ /import-lazy@3.1.0:
+ resolution: {integrity: sha512-8/gvXvX2JMn0F+CDlSC4l6kOmVaLOO3XLkksI7CI3Ud95KDYJuYur2b9P/PUt/i/pDAMd/DulQsNbbbmRRsDIQ==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /import-lazy@4.0.0:
+ resolution: {integrity: sha512-rKtvo6a868b5Hu3heneU+L4yEQ4jYKLtjpnPeUdK7h0yzXGmyBTypknlkCvHFBqfX9YlorEiMM6Dnq/5atfHkw==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /imurmurhash@0.1.4:
+ resolution: {integrity: sha512-JmXMZ6wuvDmLiHEml9ykzqO6lwFbof0GG4IkcGaENdCRDDmMVnny7s5HsIgHCbaq0w2MyPhDqkhTUgS2LU2PHA==}
+ engines: {node: '>=0.8.19'}
+ dev: true
+
+ /indent-string@3.2.0:
+ resolution: {integrity: sha512-BYqTHXTGUIvg7t1r4sJNKcbDZkL92nkXA8YtRpbjFHRHGDL/NtUeiBJMeE60kIFN/Mg8ESaWQvftaYMGJzQZCQ==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /indent-string@4.0.0:
+ resolution: {integrity: sha512-EdDDZu4A2OyIK7Lr/2zG+w5jmbuk1DVBnEwREQvBzspBJkCEbRa8GxU1lghYcaGJCnRWibjDXlq779X1/y5xwg==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /indent-string@5.0.0:
+ resolution: {integrity: sha512-m6FAo/spmsW2Ab2fU35JTYwtOKa2yAwXSwgjSv1TJzh4Mh7mC3lzAOVLBprb72XsTrgkEIsl7YrFNAiDiRhIGg==}
+ engines: {node: '>=12'}
+ dev: true
+
+ /indexes-of@1.0.1:
+ resolution: {integrity: sha512-bup+4tap3Hympa+JBJUG7XuOsdNQ6fxt0MHyXMKuLBKn0OqsTfvUxkUrroEX1+B2VsSHvCjiIcZVxRtYa4nllA==}
+ dev: true
+
+ /inflight@1.0.6:
+ resolution: {integrity: sha512-k92I/b08q4wvFscXCLvqfsHCrjrF7yiXsQuIVvVE7N82W3+aqpzuUdBbfhWcy/FZR3/4IgflMgKLOsvPDrGCJA==}
+ dependencies:
+ once: 1.4.0
+ wrappy: 1.0.2
+ dev: true
+
+ /inherits@2.0.4:
+ resolution: {integrity: sha512-k/vGaX4/Yla3WzyMCvTQOXYeIHvqOKtnqBduzTHpzpQZzAskKMhZ2K+EnBiSM9zGSoIFeMpXKxa4dYeZIQqewQ==}
+ dev: true
+
+ /ini@1.3.8:
+ resolution: {integrity: sha512-JV/yugV2uzW5iMRSiZAyDtQd+nxtUnjeLt0acNdw98kKLrvuRVyB80tsREOE7yvGVgalhZ6RNXCmEHkUKBKxew==}
+ dev: true
+
+ /inline-style-parser@0.1.1:
+ resolution: {integrity: sha512-7NXolsK4CAS5+xvdj5OMMbI962hU/wvwoxk+LWR9Ek9bVtyuuYScDN6eS0rUm6TxApFpw7CX1o4uJzcd4AyD3Q==}
+ dev: false
+
+ /inline-style-prefixer@7.0.0:
+ resolution: {integrity: sha512-I7GEdScunP1dQ6IM2mQWh6v0mOYdYmH3Bp31UecKdrcUgcURTcctSe1IECdUznSHKSmsHtjrT3CwCPI1pyxfUQ==}
+ dependencies:
+ css-in-js-utils: 3.1.0
+ fast-loops: 1.1.3
+ dev: false
+
+ /internal-slot@1.0.7:
+ resolution: {integrity: sha512-NGnrKwXzSms2qUUih/ILZ5JBqNTSa1+ZmP6flaIp6KmSElgE9qdndzS3cqjrDovwFdmwsGsLdeFgB6suw+1e9g==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ es-errors: 1.3.0
+ hasown: 2.0.2
+ side-channel: 1.0.6
+ dev: true
+
+ /invariant@2.2.4:
+ resolution: {integrity: sha512-phJfQVBuaJM5raOpJjSfkiD6BpbCE4Ns//LaXl6wGYtUBY83nWS6Rf9tXm2e8VaK60JEjYldbPif/A2B1C2gNA==}
+ dependencies:
+ loose-envify: 1.4.0
+ dev: false
+
+ /is-accessor-descriptor@1.0.1:
+ resolution: {integrity: sha512-YBUanLI8Yoihw923YeFUS5fs0fF2f5TSFTNiYAAzhhDscDa3lEqYuz1pDOEP5KvX94I9ey3vsqjJcLVFVU+3QA==}
+ engines: {node: '>= 0.10'}
+ dependencies:
+ hasown: 2.0.2
+ dev: true
+
+ /is-alphabetical@1.0.4:
+ resolution: {integrity: sha512-DwzsA04LQ10FHTZuL0/grVDk4rFoVH1pjAToYwBrHSxcrBIGQuXrQMtD5U1b0U2XVgKZCTLLP8u2Qxqhy3l2Vg==}
+ dev: true
+
+ /is-alphanumeric@1.0.0:
+ resolution: {integrity: sha512-ZmRL7++ZkcMOfDuWZuMJyIVLr2keE1o/DeNWh1EmgqGhUcV+9BIVsx0BcSBOHTZqzjs4+dISzr2KAeBEWGgXeA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /is-alphanumerical@1.0.4:
+ resolution: {integrity: sha512-UzoZUr+XfVz3t3v4KyGEniVL9BDRoQtY7tOyrRybkVNjDFWyo1yhXNGrrBTQxp3ib9BLAWs7k2YKBQsFRkZG9A==}
+ dependencies:
+ is-alphabetical: 1.0.4
+ is-decimal: 1.0.4
+ dev: true
+
+ /is-arguments@1.1.1:
+ resolution: {integrity: sha512-8Q7EARjzEnKpt/PCD7e1cgUS0a6X8u5tdSiMqXhojOdoV9TsMsiO+9VLC5vAmO8N7/GmXn7yjR8qnA6bVAEzfA==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ has-tostringtag: 1.0.2
+ dev: false
+
+ /is-array-buffer@3.0.4:
+ resolution: {integrity: sha512-wcjaerHw0ydZwfhiKbXJWLDY8A7yV7KhjQOpb83hGgGfId/aQa4TOvwyzn2PuswW2gPCYEL/nEAiSVpdOj1lXw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ get-intrinsic: 1.2.4
+ dev: true
+
+ /is-arrayish@0.2.1:
+ resolution: {integrity: sha512-zz06S8t0ozoDXMG+ube26zeCTNXcKIPJZJi8hBrF4idCLms4CG9QtK7qBl1boi5ODzFpjswb5JPmHCbMpjaYzg==}
+
+ /is-arrayish@0.3.2:
+ resolution: {integrity: sha512-eVRqCvVlZbuw3GrM63ovNSNAeA1K16kaR/LRY/92w0zxQ5/1YzwblUX652i4Xs9RwAGjW9d9y6X88t8OaAJfWQ==}
+ dev: false
+
+ /is-async-function@2.0.0:
+ resolution: {integrity: sha512-Y1JXKrfykRJGdlDwdKlLpLyMIiWqWvuSd17TvZk68PLAOGOoF4Xyav1z0Xhoi+gCYjZVeC5SI+hYFOfvXmGRCA==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ has-tostringtag: 1.0.2
+ dev: true
+
+ /is-bigint@1.0.4:
+ resolution: {integrity: sha512-zB9CruMamjym81i2JZ3UMn54PKGsQzsJeo6xvN3HJJ4CAsQNB6iRutp2To77OfCNuoxspsIhzaPoO1zyCEhFOg==}
+ dependencies:
+ has-bigints: 1.0.2
+ dev: true
+
+ /is-binary-path@2.1.0:
+ resolution: {integrity: sha512-ZMERYes6pDydyuGidse7OsHxtbI7WVeUEozgR/g7rd0xUimYNlvZRE/K2MgZTjWy725IfelLeVcEM97mmtRGXw==}
+ engines: {node: '>=8'}
+ dependencies:
+ binary-extensions: 2.3.0
+
+ /is-boolean-object@1.1.2:
+ resolution: {integrity: sha512-gDYaKHJmnj4aWxyj6YHyXVpdQawtVLHU5cb+eztPGczf6cjuTdwve5ZIEfgXqH4e57An1D1AKf8CZ3kYrQRqYA==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ has-tostringtag: 1.0.2
+ dev: true
+
+ /is-buffer@1.1.6:
+ resolution: {integrity: sha512-NcdALwpXkTm5Zvvbk7owOUSvVvBKDgKP5/ewfXEznmQFfs4ZRmanOeKBTjRVjka3QFoN6XJ+9F3USqfHqTaU5w==}
+ dev: true
+
+ /is-buffer@2.0.5:
+ resolution: {integrity: sha512-i2R6zNFDwgEHJyQUtJEk0XFi1i0dPFn/oqjK3/vPCcDeJvW5NQ83V8QbicfF1SupOaB0h8ntgBC2YiE7dfyctQ==}
+ engines: {node: '>=4'}
+
+ /is-callable@1.2.7:
+ resolution: {integrity: sha512-1BC0BVFhS/p0qtw6enp8e+8OD0UrK0oFLztSjNzhcKA3WDuJxxAPXzPuPtKkjEY9UUoEWlX/8fgKeu2S8i9JTA==}
+ engines: {node: '>= 0.4'}
+ dev: true
+
+ /is-core-module@2.13.1:
+ resolution: {integrity: sha512-hHrIjvZsftOsvKSn2TRYl63zvxsgE0K+0mYMoH6gD4omR5IWB2KynivBQczo3+wF1cCkjzvptnI9Q0sPU66ilw==}
+ dependencies:
+ hasown: 2.0.2
+
+ /is-data-descriptor@1.0.1:
+ resolution: {integrity: sha512-bc4NlCDiCr28U4aEsQ3Qs2491gVq4V8G7MQyws968ImqjKuYtTJXrl7Vq7jsN7Ly/C3xj5KWFrY7sHNeDkAzXw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ hasown: 2.0.2
+ dev: true
+
+ /is-data-view@1.0.1:
+ resolution: {integrity: sha512-AHkaJrsUVW6wq6JS8y3JnM/GJF/9cf+k20+iDzlSaJrinEo5+7vRiteOSwBhHRiAyQATN1AmY4hwzxJKPmYf+w==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ is-typed-array: 1.1.13
+ dev: true
+
+ /is-date-object@1.0.5:
+ resolution: {integrity: sha512-9YQaSxsAiSwcvS33MBk3wTCVnWK+HhF8VZR2jRxehM16QcVOdHqPn4VPHmRK4lSr38n9JriurInLcP90xsYNfQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ has-tostringtag: 1.0.2
+
+ /is-decimal@1.0.4:
+ resolution: {integrity: sha512-RGdriMmQQvZ2aqaQq3awNA6dCGtKpiDFcOzrTWrDAT2MiWrKQVPmxLGHl7Y2nNu6led0kEyoX0enY0qXYsv9zw==}
+ dev: true
+
+ /is-descriptor@0.1.7:
+ resolution: {integrity: sha512-C3grZTvObeN1xud4cRWl366OMXZTj0+HGyk4hvfpx4ZHt1Pb60ANSXqCK7pdOTeUQpRzECBSTphqvD7U+l22Eg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ is-accessor-descriptor: 1.0.1
+ is-data-descriptor: 1.0.1
+ dev: true
+
+ /is-descriptor@1.0.3:
+ resolution: {integrity: sha512-JCNNGbwWZEVaSPtS45mdtrneRWJFp07LLmykxeFV5F6oBvNF8vHSfJuJgoT472pSfk+Mf8VnlrspaFBHWM8JAw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ is-accessor-descriptor: 1.0.1
+ is-data-descriptor: 1.0.1
+ dev: true
+
+ /is-directory@0.3.1:
+ resolution: {integrity: sha512-yVChGzahRFvbkscn2MlwGismPO12i9+znNruC5gVEntG3qu0xQMzsGg/JFbrsqDOHtHFPci+V5aP5T9I+yeKqw==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /is-docker@2.2.1:
+ resolution: {integrity: sha512-F+i2BKsFrH66iaUFc0woD8sLy8getkwTwtOBjvs56Cx4CgJDeKQeqfz8wAYiSb8JOprWhHH5p77PbmYCvvUuXQ==}
+ engines: {node: '>=8'}
+ hasBin: true
+ dev: true
+
+ /is-extendable@0.1.1:
+ resolution: {integrity: sha512-5BMULNob1vgFX6EjQw5izWDxrecWK9AM72rugNr0TFldMOi0fj6Jk+zeKIt0xGj4cEfQIJth4w3OKWOJ4f+AFw==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /is-extendable@1.0.1:
+ resolution: {integrity: sha512-arnXMxT1hhoKo9k1LZdmlNyJdDDfy2v0fXjFlmok4+i8ul/6WlbVge9bhM74OpNPQPMGUToDtz+KXa1PneJxOA==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-plain-object: 2.0.4
+ dev: true
+
+ /is-extglob@2.1.1:
+ resolution: {integrity: sha512-SbKbANkN603Vi4jEZv49LeVJMn4yGwsbzZworEoyEiutsN3nJYdbO36zfhGJ6QEDpOZIFkDtnq5JRxmvl3jsoQ==}
+ engines: {node: '>=0.10.0'}
+
+ /is-finalizationregistry@1.0.2:
+ resolution: {integrity: sha512-0by5vtUJs8iFQb5TYUHHPudOR+qXYIMKtiUzvLIZITZUjknFmziyBJuLhVRc+Ds0dREFlskDNJKYIdIzu/9pfw==}
+ dependencies:
+ call-bind: 1.0.7
+ dev: true
+
+ /is-fullwidth-code-point@2.0.0:
+ resolution: {integrity: sha512-VHskAKYM8RfSFXwee5t5cbN5PZeq1Wrh6qd5bkyiXIf6UQcN6w/A0eXM9r6t8d+GYOh+o6ZhiEnb88LN/Y8m2w==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /is-fullwidth-code-point@3.0.0:
+ resolution: {integrity: sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /is-fullwidth-code-point@4.0.0:
+ resolution: {integrity: sha512-O4L094N2/dZ7xqVdrXhh9r1KODPJpFms8B5sGdJLPy664AgvXsreZUyCQQNItZRDlYug4xStLjNp/sz3HvBowQ==}
+ engines: {node: '>=12'}
+ dev: true
+
+ /is-generator-function@1.0.10:
+ resolution: {integrity: sha512-jsEjy9l3yiXEQ+PsXdmBwEPcOxaXWLspKdplFUVI9vq1iZgIekeC0L167qeu86czQaxed3q/Uzuw0swL0irL8A==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ has-tostringtag: 1.0.2
+ dev: true
+
+ /is-glob@3.1.0:
+ resolution: {integrity: sha512-UFpDDrPgM6qpnFNI+rh/p3bUaq9hKLZN8bMUWzxmcnZVS3omf4IPK+BrewlnWjO1WmUsMYuSjKh4UJuV4+Lqmw==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-extglob: 2.1.1
+ dev: true
+
+ /is-glob@4.0.3:
+ resolution: {integrity: sha512-xelSayHH36ZgE7ZWhli7pW34hNbNl8Ojv5KVmkJD4hBdD3th8Tfk9vYasLM+mXWOZhFkgZfxhLSnrwRr4elSSg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-extglob: 2.1.1
+
+ /is-hexadecimal@1.0.4:
+ resolution: {integrity: sha512-gyPJuv83bHMpocVYoqof5VDiZveEoGoFL8m3BXNb2VW8Xs+rz9kqO8LOQ5DH6EsuvilT1ApazU0pyl+ytbPtlw==}
+ dev: true
+
+ /is-map@2.0.3:
+ resolution: {integrity: sha512-1Qed0/Hr2m+YqxnM09CjA2d/i6YZNfF6R2oRAOj36eUdS6qIV/huPJNSEpKbupewFs+ZsJlxsjjPbc0/afW6Lw==}
+ engines: {node: '>= 0.4'}
+ dev: true
+
+ /is-negative-zero@2.0.3:
+ resolution: {integrity: sha512-5KoIu2Ngpyek75jXodFvnafB6DJgr3u8uuK0LEZJjrU19DrMD3EVERaR8sjz8CCGgpZvxPl9SuE1GMVPFHx1mw==}
+ engines: {node: '>= 0.4'}
+ dev: true
+
+ /is-number-object@1.0.7:
+ resolution: {integrity: sha512-k1U0IRzLMo7ZlYIfzRu23Oh6MiIFasgpb9X76eqfFZAqwH44UI4KTBvBYIZ1dSL9ZzChTB9ShHfLkR4pdW5krQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ has-tostringtag: 1.0.2
+ dev: true
+
+ /is-number@3.0.0:
+ resolution: {integrity: sha512-4cboCqIpliH+mAvFNegjZQ4kgKc3ZUhQVr3HvWbSh5q3WH2v82ct+T2Y1hdU5Gdtorx/cLifQjqCbL7bpznLTg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ kind-of: 3.2.2
+ dev: true
+
+ /is-number@7.0.0:
+ resolution: {integrity: sha512-41Cifkg6e8TylSpdtTpeLVMqvSBEVzTttHvERD741+pnZ8ANv0004MRL43QKPDlK9cGvNp6NZWZUBlbGXYxxng==}
+ engines: {node: '>=0.12.0'}
+
+ /is-obj@2.0.0:
+ resolution: {integrity: sha512-drqDG3cbczxxEJRoOXcOjtdp1J/lyp1mNn0xaznRs8+muBhgQcrnbspox5X5fOw0HnMnbfDzvnEMEtqDEJEo8w==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /is-path-inside@3.0.3:
+ resolution: {integrity: sha512-Fd4gABb+ycGAmKou8eMftCupSir5lRxqf4aD/vd0cD2qc4HL07OjCeuHMr8Ro4CoMaeCKDB0/ECBOVWjTwUvPQ==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /is-plain-obj@1.1.0:
+ resolution: {integrity: sha512-yvkRyxmFKEOQ4pNXCmJG5AEQNlXJS5LaONXo5/cLdTZdWvsZ1ioJEonLGAosKlMWE8lwUy/bJzMjcw8az73+Fg==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /is-plain-obj@4.1.0:
+ resolution: {integrity: sha512-+Pgi+vMuUNkJyExiMBt5IlFoMyKnr5zhJ4Uspz58WOhBF5QoIZkFyNHIbBAtHwzVAgk5RtndVNsDRN61/mmDqg==}
+ engines: {node: '>=12'}
+ dev: false
+
+ /is-plain-object@2.0.4:
+ resolution: {integrity: sha512-h5PpgXkWitc38BBMYawTYMWJHFZJVnBquFE57xFpjB8pJFiF6gZ+bU+WyI/yqXiFR5mdLsgYNaPe8uao6Uv9Og==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ isobject: 3.0.1
+ dev: true
+
+ /is-plain-object@5.0.0:
+ resolution: {integrity: sha512-VRSzKkbMm5jMDoKLbltAkFQ5Qr7VDiTFGXxYFXXowVj387GeGNOCsOH6Msy00SGZ3Fp84b1Naa1psqgcCIEP5Q==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /is-regex@1.1.4:
+ resolution: {integrity: sha512-kvRdxDsxZjhzUX07ZnLydzS1TU/TJlTUHHY4YLL87e37oUA49DfkLqgy+VjFocowy29cKvcSiu+kIv728jTTVg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ has-tostringtag: 1.0.2
+
+ /is-regexp@1.0.0:
+ resolution: {integrity: sha512-7zjFAPO4/gwyQAAgRRmqeEeyIICSdmCqa3tsVHMdBzaXXRiqopZL4Cyghg/XulGWrtABTpbnYYzzIRffLkP4oA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /is-root@2.1.0:
+ resolution: {integrity: sha512-AGOriNp96vNBd3HtU+RzFEc75FfR5ymiYv8E553I71SCeXBiMsVDUtdio1OEFvrPyLIQ9tVR5RxXIFe5PUFjMg==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /is-set@2.0.3:
+ resolution: {integrity: sha512-iPAjerrse27/ygGLxw+EBR9agv9Y6uLeYVJMu+QNCoouJ1/1ri0mGrcWpfCqFZuzzx3WjtwxG098X+n4OuRkPg==}
+ engines: {node: '>= 0.4'}
+ dev: true
+
+ /is-shared-array-buffer@1.0.3:
+ resolution: {integrity: sha512-nA2hv5XIhLR3uVzDDfCIknerhx8XUKnstuOERPNNIinXG7v9u+ohXF67vxm4TPTEPU6lm61ZkwP3c9PCB97rhg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ dev: true
+
+ /is-stream@2.0.1:
+ resolution: {integrity: sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /is-stream@3.0.0:
+ resolution: {integrity: sha512-LnQR4bZ9IADDRSkvpqMGvt/tEJWclzklNgSw48V5EAaAeDd6qGvN8ei6k5p0tvxSR171VmGyHuTiAOfxAbr8kA==}
+ engines: {node: ^12.20.0 || ^14.13.1 || >=16.0.0}
+ dev: true
+
+ /is-string@1.0.7:
+ resolution: {integrity: sha512-tE2UXzivje6ofPW7l23cjDOMa09gb7xlAqG6jG5ej6uPV32TlWP3NKPigtaGeHNu9fohccRYvIiZMfOOnOYUtg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ has-tostringtag: 1.0.2
+ dev: true
+
+ /is-supported-regexp-flag@1.0.1:
+ resolution: {integrity: sha512-3vcJecUUrpgCqc/ca0aWeNu64UGgxcvO60K/Fkr1N6RSvfGCTU60UKN68JDmKokgba0rFFJs12EnzOQa14ubKQ==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /is-symbol@1.0.4:
+ resolution: {integrity: sha512-C/CPBqKWnvdcxqIARxyOh4v1UUEOCHpgDa0WYgpKDFMszcrPcffg5uhwSgPCLD2WWxmq6isisz87tzT01tuGhg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ has-symbols: 1.0.3
+ dev: true
+
+ /is-text-path@1.0.1:
+ resolution: {integrity: sha512-xFuJpne9oFz5qDaodwmmG08e3CawH/2ZV8Qqza1Ko7Sk8POWbkRdwIoAWVhqvq0XeUzANEhKo2n0IXUGBm7A/w==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ text-extensions: 1.9.0
+ dev: true
+
+ /is-typed-array@1.1.13:
+ resolution: {integrity: sha512-uZ25/bUAlUY5fR4OKT4rZQEBrzQWYV9ZJYGGsUmEJ6thodVJ1HX64ePQ6Z0qPWP+m+Uq6e9UugrE38jeYsDSMw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ which-typed-array: 1.1.15
+ dev: true
+
+ /is-weakmap@2.0.2:
+ resolution: {integrity: sha512-K5pXYOm9wqY1RgjpL3YTkF39tni1XajUIkawTLUo9EZEVUFga5gSQJF8nNS7ZwJQ02y+1YCNYcMh+HIf1ZqE+w==}
+ engines: {node: '>= 0.4'}
+ dev: true
+
+ /is-weakref@1.0.2:
+ resolution: {integrity: sha512-qctsuLZmIQ0+vSSMfoVvyFe2+GSEvnmZ2ezTup1SBse9+twCCeial6EEi3Nc2KFcf6+qz2FBPnjXsk8xhKSaPQ==}
+ dependencies:
+ call-bind: 1.0.7
+ dev: true
+
+ /is-weakset@2.0.3:
+ resolution: {integrity: sha512-LvIm3/KWzS9oRFHugab7d+M/GcBXuXX5xZkzPmN+NxihdQlZUQ4dWuSV1xR/sq6upL1TJEDrfBgRepHFdBtSNQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ get-intrinsic: 1.2.4
+ dev: true
+
+ /is-what@4.1.16:
+ resolution: {integrity: sha512-ZhMwEosbFJkA0YhFnNDgTM4ZxDRsS6HqTo7qsZM08fehyRYIYa0yHu5R6mgo1n/8MgaPBXiPimPD77baVFYg+A==}
+ engines: {node: '>=12.13'}
+ dev: false
+
+ /is-whitespace-character@1.0.4:
+ resolution: {integrity: sha512-SDweEzfIZM0SJV0EUga669UTKlmL0Pq8Lno0QDQsPnvECB3IM2aP0gdx5TrU0A01MAPfViaZiI2V1QMZLaKK5w==}
+ dev: true
+
+ /is-windows@1.0.2:
+ resolution: {integrity: sha512-eXK1UInq2bPmjyX6e3VHIzMLobc4J94i4AWn+Hpq3OU5KkrRC96OAcR3PRJ/pGu6m8TRnBHP9dkXQVsT/COVIA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /is-word-character@1.0.4:
+ resolution: {integrity: sha512-5SMO8RVennx3nZrqtKwCGyyetPE9VDba5ugvKLaD4KopPG5kR4mQ7tNt/r7feL5yt5h3lpuBbIUmCOG2eSzXHA==}
+ dev: true
+
+ /is-wsl@2.2.0:
+ resolution: {integrity: sha512-fKzAra0rGJUUBwGBgNkHZuToZcn+TtXHpeCgmkMJMMYx1sQDYaCSyjJBSCa2nH1DGm7s3n1oBnohoVTBaN7Lww==}
+ engines: {node: '>=8'}
+ dependencies:
+ is-docker: 2.2.1
+ dev: true
+
+ /isarray@1.0.0:
+ resolution: {integrity: sha512-VLghIWNM6ELQzo7zwmcg0NmTVyWKYjvIeM83yjp0wRDTmUnrM678fQbcKBo6n2CJEF0szoG//ytg+TKla89ALQ==}
+ dev: true
+
+ /isarray@2.0.5:
+ resolution: {integrity: sha512-xHjhDr3cNBK0BzdUJSPXZntQUx/mwMS5Rw4A7lPJ90XGAO6ISP/ePDNuo0vhqOZU+UD5JoodwCAAoZQd3FeAKw==}
+ dev: true
+
+ /isexe@2.0.0:
+ resolution: {integrity: sha512-RHxMLp9lnKHGHRng9QFhRCMbYAcVpn69smSGcq3f36xjgVVWThj4qqLbTLlq7Ssj8B+fIQ1EuCEGI2lKsyQeIw==}
+ dev: true
+
+ /isobject@2.1.0:
+ resolution: {integrity: sha512-+OUdGJlgjOBZDfxnDjYYG6zp487z0JGNQq3cYQYg5f5hKR+syHMsaztzGeml/4kGG55CSpKSpWTY+jYGgsHLgA==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ isarray: 1.0.0
+ dev: true
+
+ /isobject@3.0.1:
+ resolution: {integrity: sha512-WhB9zCku7EGTj/HQQRz5aUQEUeoQZH2bWcltRErOpymJ4boYE6wL9Tbr23krRPSZ+C5zqNSrSw+Cc7sZZ4b7vg==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /iterator.prototype@1.1.2:
+ resolution: {integrity: sha512-DR33HMMr8EzwuRL8Y9D3u2BMj8+RqSE850jfGu59kS7tbmPLzGkZmVSfyCFSDxuZiEY6Rzt3T2NA/qU+NwVj1w==}
+ dependencies:
+ define-properties: 1.2.1
+ get-intrinsic: 1.2.4
+ has-symbols: 1.0.3
+ reflect.getprototypeof: 1.0.6
+ set-function-name: 2.0.2
+ dev: true
+
+ /jackspeak@2.3.6:
+ resolution: {integrity: sha512-N3yCS/NegsOBokc8GAdM8UcmfsKiSS8cipheD/nivzr700H+nsMOxJjQnvwOcRYVuFkdH0wGUvW2WbXGmrZGbQ==}
+ engines: {node: '>=14'}
+ dependencies:
+ '@isaacs/cliui': 8.0.2
+ optionalDependencies:
+ '@pkgjs/parseargs': 0.11.0
+ dev: true
+
+ /jest-worker@27.5.1:
+ resolution: {integrity: sha512-7vuh85V5cdDofPyxn58nrPjBktZo0u9x1g8WtjQol+jZDaE+fhN+cIvTj11GndBnMnyfrUOG1sZQxCdjKh+DKg==}
+ engines: {node: '>= 10.13.0'}
+ dependencies:
+ '@types/node': 20.12.7
+ merge-stream: 2.0.0
+ supports-color: 8.1.1
+ dev: true
+
+ /jiti@1.21.0:
+ resolution: {integrity: sha512-gFqAIbuKyyso/3G2qhiO2OM6shY6EPP/R0+mkDbyspxKazh8BXDC5FiFsUjlczgdNz/vfra0da2y+aHrusLG/Q==}
+ hasBin: true
+ dev: true
+
+ /js-base64@2.6.4:
+ resolution: {integrity: sha512-pZe//GGmwJndub7ZghVHz7vjb2LgC1m8B07Au3eYqeqv9emhESByMXxaEgkUkEqJe87oBbSniGYoQNIBklc7IQ==}
+ dev: true
+
+ /js-cookie@2.2.1:
+ resolution: {integrity: sha512-HvdH2LzI/EAZcUwA8+0nKNtWHqS+ZmijLA30RwZA0bo7ToCckjK5MkGhjED9KoRcXO6BaGI3I9UIzSA1FKFPOQ==}
+ dev: false
+
+ /js-tokens@4.0.0:
+ resolution: {integrity: sha512-RdJUflcE3cUzKiMqQgsCu06FPu9UdIJO0beYbPhHN4k6apgJtifcoCtT9bcxOpYBtpD2kCM6Sbzg4CausW/PKQ==}
+
+ /js-yaml@3.14.1:
+ resolution: {integrity: sha512-okMH7OXXJ7YrN9Ok3/SXrnu4iX9yOk+25nqX4imS2npuvTYDmo/QEZoqwZkYaIDk3jVvBOTOIEgEhaLOynBS9g==}
+ hasBin: true
+ dependencies:
+ argparse: 1.0.10
+ esprima: 4.0.1
+ dev: true
+
+ /js-yaml@4.1.0:
+ resolution: {integrity: sha512-wpxZs9NoxZaJESJGIZTyDEaYpl0FKSA+FB9aJiyemKhMwkxQg63h4T1KJgUGHpTqPDNRcmmYLugrRjJlBtWvRA==}
+ hasBin: true
+ dependencies:
+ argparse: 2.0.1
+ dev: true
+
+ /jsesc@2.5.2:
+ resolution: {integrity: sha512-OYu7XEzjkCQ3C5Ps3QIZsQfNpqoJyZZA99wd9aWd05NCtC5pWOkShK2mkL6HXQR6/Cy2lbNdPlZBpuQHXE63gA==}
+ engines: {node: '>=4'}
+ hasBin: true
+
+ /json-buffer@3.0.1:
+ resolution: {integrity: sha512-4bV5BfR2mqfQTJm+V5tPPdf+ZpuhiIvTuAB5g8kcrXOZpTT/QwwVRWBywX1ozr6lEuPdbHxwaJlm9G6mI2sfSQ==}
+ dev: true
+
+ /json-parse-better-errors@1.0.2:
+ resolution: {integrity: sha512-mrqyZKfX5EhL7hvqcV6WG1yYjnjeuYDzDhhcAAUrq8Po85NBQBJP+ZDUT75qZQ98IkUoBqdkExkukOU7Ts2wrw==}
+ dev: true
+
+ /json-parse-even-better-errors@2.3.1:
+ resolution: {integrity: sha512-xyFwyhro/JEof6Ghe2iz2NcXoj2sloNsWr/XsERDK/oiPCfaNhl5ONfp+jQdAZRQQ0IJWNzH9zIZF7li91kh2w==}
+
+ /json-schema-traverse@0.4.1:
+ resolution: {integrity: sha512-xbbCH5dCYU5T8LcEhhuh7HJ88HXuW3qsI3Y0zOZFKfZEHcpWiHU/Jxzk629Brsab/mMiHQti9wMP+845RPe3Vg==}
+ dev: true
+
+ /json-schema-traverse@1.0.0:
+ resolution: {integrity: sha512-NM8/P9n3XjXhIZn1lLhkFaACTOURQXjWhV4BA/RnOv8xvgqtqpAX9IO4mRQxSx1Rlo4tqzeqb0sOlruaOy3dug==}
+ dev: true
+
+ /json-stable-stringify-without-jsonify@1.0.1:
+ resolution: {integrity: sha512-Bdboy+l7tA3OGW6FjyFHWkP5LuByj1Tk33Ljyq0axyzdk9//JSi2u3fP1QSmd1KNwq6VOKYGlAu87CisVir6Pw==}
+ dev: true
+
+ /json2mq@0.2.0:
+ resolution: {integrity: sha512-SzoRg7ux5DWTII9J2qkrZrqV1gt+rTaoufMxEzXbS26Uid0NwaJd123HcoB80TgubEppxxIGdNxCx50fEoEWQA==}
+ dependencies:
+ string-convert: 0.2.1
+ dev: false
+
+ /json5@1.0.2:
+ resolution: {integrity: sha512-g1MWMLBiz8FKi1e4w0UyVL3w+iJceWAFBAaBnnGKOpNa5f8TLktkbre1+s6oICydWAm+HRUGTmI+//xv2hvXYA==}
+ hasBin: true
+ dependencies:
+ minimist: 1.2.8
+ dev: true
+
+ /json5@2.2.3:
+ resolution: {integrity: sha512-XmOWe7eyHYH14cLdVPoyg+GOH3rYX++KpzrylJwSW98t3Nk+U8XOl8FWKOgwtzdb8lXGf6zYwDUzeHMWfxasyg==}
+ engines: {node: '>=6'}
+ hasBin: true
+
+ /jsonfile@6.1.0:
+ resolution: {integrity: sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==}
+ dependencies:
+ universalify: 2.0.1
+ optionalDependencies:
+ graceful-fs: 4.2.11
+ dev: true
+
+ /jsonparse@1.3.1:
+ resolution: {integrity: sha512-POQXvpdL69+CluYsillJ7SUhKvytYjW9vG/GKpnf+xP8UWgYEM/RaMzHHofbALDiKbbP1W8UEYmgGl39WkPZsg==}
+ engines: {'0': node >= 0.2.0}
+ dev: true
+
+ /jsx-ast-utils@3.3.5:
+ resolution: {integrity: sha512-ZZow9HBI5O6EPgSJLUb8n2NKgmVWTwCvHGwFuJlMjvLFqlGG6pjirPhtdsseaLZjSibD8eegzmYpUZwoIlj2cQ==}
+ engines: {node: '>=4.0'}
+ dependencies:
+ array-includes: 3.1.8
+ array.prototype.flat: 1.3.2
+ object.assign: 4.1.5
+ object.values: 1.2.0
+ dev: true
+
+ /keyv@4.5.4:
+ resolution: {integrity: sha512-oxVHkHR/EJf2CNXnWxRLW6mg7JyCCUcG0DtEGmL2ctUo1PNTin1PUil+r/+4r5MpVgC/fn1kjsx7mjSujKqIpw==}
+ dependencies:
+ json-buffer: 3.0.1
+ dev: true
+
+ /kind-of@3.2.2:
+ resolution: {integrity: sha512-NOW9QQXMoZGg/oqnVNoNTTIFEIid1627WCffUBJEdMxYApq7mNE7CpzucIPc+ZQg25Phej7IJSmX3hO+oblOtQ==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-buffer: 1.1.6
+ dev: true
+
+ /kind-of@4.0.0:
+ resolution: {integrity: sha512-24XsCxmEbRwEDbz/qz3stgin8TTzZ1ESR56OMCN0ujYg+vRutNSiOj9bHH9u85DKgXguraugV5sFuvbD4FW/hw==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-buffer: 1.1.6
+ dev: true
+
+ /kind-of@5.1.0:
+ resolution: {integrity: sha512-NGEErnH6F2vUuXDh+OlbcKW7/wOcfdRHaZ7VWtqCztfHri/++YKmP51OdWeGPuqCOba6kk2OTe5d02VmTB80Pw==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /kind-of@6.0.3:
+ resolution: {integrity: sha512-dcS1ul+9tmeD95T+x28/ehLgd9mENa3LsvDTtzm3vyBEO7RPptvAD+t44WVXaUjTBRcrpFeFlC8WCruUR456hw==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /kleur@3.0.3:
+ resolution: {integrity: sha512-eTIzlVOSUR+JxdDFepEYcBMtZ9Qqdef+rnzWdRZuMbOywu5tO2w2N7rqjoANZ5k9vywhL6Br1VRjUIgTQx4E8w==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /kleur@4.1.5:
+ resolution: {integrity: sha512-o+NO+8WrRiQEE4/7nwRJhN1HWpVmJm511pBHUxPLtp0BUISzlBplORYSmTclCnJvQq2tKu/sgl3xVpkc7ZWuQQ==}
+ engines: {node: '>=6'}
+ dev: false
+
+ /known-css-properties@0.11.0:
+ resolution: {integrity: sha512-bEZlJzXo5V/ApNNa5z375mJC6Nrz4vG43UgcSCrg2OHC+yuB6j0iDSrY7RQ/+PRofFB03wNIIt9iXIVLr4wc7w==}
+ dev: true
+
+ /known-css-properties@0.29.0:
+ resolution: {integrity: sha512-Ne7wqW7/9Cz54PDt4I3tcV+hAyat8ypyOGzYRJQfdxnnjeWsTxt1cy8pjvvKeI5kfXuyvULyeeAvwvvtAX3ayQ==}
+ dev: true
+
+ /language-subtag-registry@0.3.22:
+ resolution: {integrity: sha512-tN0MCzyWnoz/4nHS6uxdlFWoUZT7ABptwKPQ52Ea7URk6vll88bWBVhodtnlfEuCcKWNGoc+uGbw1cwa9IKh/w==}
+ dev: true
+
+ /language-tags@1.0.9:
+ resolution: {integrity: sha512-MbjN408fEndfiQXbFQ1vnd+1NoLDsnQW41410oQBXiyXDMYH5z505juWa4KUE1LqxRC7DgOgZDbKLxHIwm27hA==}
+ engines: {node: '>=0.10'}
+ dependencies:
+ language-subtag-registry: 0.3.22
+ dev: true
+
+ /leven@2.1.0:
+ resolution: {integrity: sha512-nvVPLpIHUxCUoRLrFqTgSxXJ614d8AgQoWl7zPe/2VadE8+1dpU3LBhowRuBAcuwruWtOdD8oYC9jDNJjXDPyA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /levn@0.4.1:
+ resolution: {integrity: sha512-+bT2uH4E5LGE7h/n3evcS/sQlJXCpIp6ym8OWJ5eV6+67Dsql/LaaT7qJBAt2rzfoa/5QBGBhxDix1dMt2kQKQ==}
+ engines: {node: '>= 0.8.0'}
+ dependencies:
+ prelude-ls: 1.2.1
+ type-check: 0.4.0
+ dev: true
+
+ /lilconfig@2.1.0:
+ resolution: {integrity: sha512-utWOt/GHzuUxnLKxB6dk81RoOeoNeHgbrXiuGk4yyF5qlRz+iIVWu56E2fqGHFrXz0QNUhLB/8nKqvRH66JKGQ==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /lilconfig@3.1.1:
+ resolution: {integrity: sha512-O18pf7nyvHTckunPWCV1XUNXU1piu01y2b7ATJ0ppkUkk8ocqVWBrYjJBCwHDjD/ZWcfyrA0P4gKhzWGi5EINQ==}
+ engines: {node: '>=14'}
+ dev: true
+
+ /lines-and-columns@1.2.4:
+ resolution: {integrity: sha512-7ylylesZQ/PV29jhEDl3Ufjo6ZX7gCqJr5F7PKrqc93v7fzSymt1BpwEU8nAUXs8qzzvqhbjhK5QZg6Mt/HkBg==}
+
+ /lint-staged@13.3.0:
+ resolution: {integrity: sha512-mPRtrYnipYYv1FEE134ufbWpeggNTo+O/UPzngoaKzbzHAthvR55am+8GfHTnqNRQVRRrYQLGW9ZyUoD7DsBHQ==}
+ engines: {node: ^16.14.0 || >=18.0.0}
+ hasBin: true
+ dependencies:
+ chalk: 5.3.0
+ commander: 11.0.0
+ debug: 4.3.4
+ execa: 7.2.0
+ lilconfig: 2.1.0
+ listr2: 6.6.1
+ micromatch: 4.0.5
+ pidtree: 0.6.0
+ string-argv: 0.3.2
+ yaml: 2.3.1
+ transitivePeerDependencies:
+ - enquirer
+ - supports-color
+ dev: true
+
+ /listr2@6.6.1:
+ resolution: {integrity: sha512-+rAXGHh0fkEWdXBmX+L6mmfmXmXvDGEKzkjxO+8mP3+nI/r/CWznVBvsibXdxda9Zz0OW2e2ikphN3OwCT/jSg==}
+ engines: {node: '>=16.0.0'}
+ peerDependencies:
+ enquirer: '>= 2.3.0 < 3'
+ peerDependenciesMeta:
+ enquirer:
+ optional: true
+ dependencies:
+ cli-truncate: 3.1.0
+ colorette: 2.0.20
+ eventemitter3: 5.0.1
+ log-update: 5.0.1
+ rfdc: 1.3.1
+ wrap-ansi: 8.1.0
+ dev: true
+
+ /load-json-file@4.0.0:
+ resolution: {integrity: sha512-Kx8hMakjX03tiGTLAIdJ+lL0htKnXjEZN6hk/tozf/WOuYGdZBJrZ+rCJRbVCugsjB3jMLn9746NsQIf5VjBMw==}
+ engines: {node: '>=4'}
+ dependencies:
+ graceful-fs: 4.2.11
+ parse-json: 4.0.0
+ pify: 3.0.0
+ strip-bom: 3.0.0
+ dev: true
+
+ /loader-runner@4.3.0:
+ resolution: {integrity: sha512-3R/1M+yS3j5ou80Me59j7F9IMs4PXs3VqRrm0TU3AbKPxlmpoY1TNscJV/oGJXo8qCatFGTfDbY6W6ipGOYXfg==}
+ engines: {node: '>=6.11.5'}
+ dev: true
+
+ /loader-utils@1.4.2:
+ resolution: {integrity: sha512-I5d00Pd/jwMD2QCduo657+YM/6L3KZu++pmX9VFncxaxvHcru9jx1lBaFft+r4Mt2jK0Yhp41XlRAihzPxHNCg==}
+ engines: {node: '>=4.0.0'}
+ dependencies:
+ big.js: 5.2.2
+ emojis-list: 3.0.0
+ json5: 1.0.2
+ dev: true
+
+ /loader-utils@3.2.1:
+ resolution: {integrity: sha512-ZvFw1KWS3GVyYBYb7qkmRM/WwL2TQQBxgCK62rlvm4WpVQ23Nb4tYjApUlfjrEGvOs7KHEsmyUn75OHZrJMWPw==}
+ engines: {node: '>= 12.13.0'}
+ dev: true
+
+ /locate-path@2.0.0:
+ resolution: {integrity: sha512-NCI2kiDkyR7VeEKm27Kda/iQHyKJe1Bu0FlTbYp3CqJu+9IFe9bLyAjMxf5ZDDbEg+iMPzB5zYyUTSm8wVTKmA==}
+ engines: {node: '>=4'}
+ dependencies:
+ p-locate: 2.0.0
+ path-exists: 3.0.0
+ dev: true
+
+ /locate-path@3.0.0:
+ resolution: {integrity: sha512-7AO748wWnIhNqAuaty2ZWHkQHRSNfPVIsPIfwEOWO22AmaoVrWavlOcMR5nzTLNYvp36X220/maaRsrec1G65A==}
+ engines: {node: '>=6'}
+ dependencies:
+ p-locate: 3.0.0
+ path-exists: 3.0.0
+ dev: true
+
+ /locate-path@5.0.0:
+ resolution: {integrity: sha512-t7hw9pI+WvuwNJXwk5zVHpyhIqzg2qTlklJOf0mVxGSbe3Fp2VieZcduNYjaLDoy6p9uGpQEGWG87WpMKlNq8g==}
+ engines: {node: '>=8'}
+ dependencies:
+ p-locate: 4.1.0
+ dev: true
+
+ /locate-path@6.0.0:
+ resolution: {integrity: sha512-iPZK6eYjbxRu3uB4/WZ3EsEIMJFMqAoopl3R+zuq0UjcAm/MO6KCweDgPfP3elTztoKP3KtnVHxTn2NHBSDVUw==}
+ engines: {node: '>=10'}
+ dependencies:
+ p-locate: 5.0.0
+ dev: true
+
+ /lodash-es@4.17.21:
+ resolution: {integrity: sha512-mKnC+QJ9pWVzv+C4/U3rRsHapFfHvQFoFB92e52xeyGMcX6/OlIl78je1u8vePzYZSkkogMPJ2yjxxsb89cxyw==}
+ dev: false
+
+ /lodash.camelcase@4.3.0:
+ resolution: {integrity: sha512-TwuEnCnxbc3rAvhf/LbG7tJUDzhqXyFnv3dtzLOPgCG/hODL7WFnsbwktkD7yUV0RrreP/l1PALq/YSg6VvjlA==}
+ dev: true
+
+ /lodash.isfunction@3.0.9:
+ resolution: {integrity: sha512-AirXNj15uRIMMPihnkInB4i3NHeb4iBtNg9WRWuK2o31S+ePwwNmDPaTL3o7dTJ+VXNZim7rFs4rxN4YU1oUJw==}
+ dev: true
+
+ /lodash.isplainobject@4.0.6:
+ resolution: {integrity: sha512-oSXzaWypCMHkPC3NvBEaPHf0KsA5mvPrOPgQWDsbg8n7orZ290M0BmC/jgRZ4vcJ6DTAhjrsSYgdsW/F+MFOBA==}
+ dev: true
+
+ /lodash.kebabcase@4.1.1:
+ resolution: {integrity: sha512-N8XRTIMMqqDgSy4VLKPnJ/+hpGZN+PHQiJnSenYqPaVV/NCqEogTnAdZLQiGKhxX+JCs8waWq2t1XHWKOmlY8g==}
+ dev: true
+
+ /lodash.merge@4.6.2:
+ resolution: {integrity: sha512-0KpjqXRVvrYyCsX1swR/XTK0va6VQkQM6MNo7PqW77ByjAhoARA8EfrP1N4+KlKj8YS0ZUCtRT/YUuhyYDujIQ==}
+ dev: true
+
+ /lodash.mergewith@4.6.2:
+ resolution: {integrity: sha512-GK3g5RPZWTRSeLSpgP8Xhra+pnjBC56q9FZYe1d5RN3TJ35dbkGy3YqBSMbyCrlbi+CM9Z3Jk5yTL7RCsqboyQ==}
+ dev: true
+
+ /lodash.snakecase@4.1.1:
+ resolution: {integrity: sha512-QZ1d4xoBHYUeuouhEq3lk3Uq7ldgyFXGBhg04+oRLnIz8o9T65Eh+8YdroUwn846zchkA9yDsDl5CVVaV2nqYw==}
+ dev: true
+
+ /lodash.startcase@4.4.0:
+ resolution: {integrity: sha512-+WKqsK294HMSc2jEbNgpHpd0JfIBhp7rEV4aqXWqFr6AlXov+SlcgB1Fv01y2kGe3Gc8nMW7VA0SrGuSkRfIEg==}
+ dev: true
+
+ /lodash.truncate@4.4.2:
+ resolution: {integrity: sha512-jttmRe7bRse52OsWIMDLaXxWqRAmtIUccAQ3garviCqJjafXOfNMO0yMfNpdD6zbGaTU0P5Nz7e7gAT6cKmJRw==}
+ dev: true
+
+ /lodash.uniq@4.5.0:
+ resolution: {integrity: sha512-xfBaXQd9ryd9dlSDvnvI0lvxfLJlYAZzXomUYzLKtUeOQvOP5piqAWuGtrhWeqaXK9hhoM/iyJc5AV+XfsX3HQ==}
+ dev: true
+
+ /lodash.upperfirst@4.3.1:
+ resolution: {integrity: sha512-sReKOYJIJf74dhJONhU4e0/shzi1trVbSWDOhKYE5XV2O+H7Sb2Dihwuc7xWxVl+DgFPyTqIN3zMfT9cq5iWDg==}
+ dev: true
+
+ /lodash@4.17.21:
+ resolution: {integrity: sha512-v2kDEe57lecTulaDIuNTPy3Ry4gLGJ6Z1O3vE1krgXZNrsQ+LFTGHVxVjcXPs17LhbZVGedAJv8XZ1tvj5FvSg==}
+
+ /log-symbols@2.2.0:
+ resolution: {integrity: sha512-VeIAFslyIerEJLXHziedo2basKbMKtTw3vfn5IzG0XTjhAVEJyNHnL2p7vc+wBDSdQuUpNw3M2u6xb9QsAY5Eg==}
+ engines: {node: '>=4'}
+ dependencies:
+ chalk: 2.4.2
+ dev: true
+
+ /log-update@5.0.1:
+ resolution: {integrity: sha512-5UtUDQ/6edw4ofyljDNcOVJQ4c7OjDro4h3y8e1GQL5iYElYclVHJ3zeWchylvMaKnDbDilC8irOVyexnA/Slw==}
+ engines: {node: ^12.20.0 || ^14.13.1 || >=16.0.0}
+ dependencies:
+ ansi-escapes: 5.0.0
+ cli-cursor: 4.0.0
+ slice-ansi: 5.0.0
+ strip-ansi: 7.1.0
+ wrap-ansi: 8.1.0
+ dev: true
+
+ /longest-streak@2.0.4:
+ resolution: {integrity: sha512-vM6rUVCVUJJt33bnmHiZEvr7wPT78ztX7rojL+LW51bHtLh6HTjx84LA5W4+oa6aKEJA7jJu5LR6vQRBpA5DVg==}
+ dev: true
+
+ /longest-streak@3.1.0:
+ resolution: {integrity: sha512-9Ri+o0JYgehTaVBBDoMqIl8GXtbWg711O3srftcHhZ0dqnETqLaoIK0x17fUw9rFSlK/0NlsKe0Ahhyl5pXE2g==}
+ dev: false
+
+ /loose-envify@1.4.0:
+ resolution: {integrity: sha512-lyuxPGr/Wfhrlem2CL/UcnUc1zcqKAImBDzukY7Y5F/yQiNdko6+fRLevlw1HgMySw7f611UIY408EtxRSoK3Q==}
+ hasBin: true
+ dependencies:
+ js-tokens: 4.0.0
+
+ /loud-rejection@1.6.0:
+ resolution: {integrity: sha512-RPNliZOFkqFumDhvYqOaNY4Uz9oJM2K9tC6JWsJJsNdhuONW4LQHRBpb0qf4pJApVffI5N39SwzWZJuEhfd7eQ==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ currently-unhandled: 0.4.1
+ signal-exit: 3.0.7
+ dev: true
+
+ /lowlight@2.9.0:
+ resolution: {integrity: sha512-OpcaUTCLmHuVuBcyNckKfH5B0oA4JUavb/M/8n9iAvanJYNQkrVm4pvyX0SUaqkBG4dnWHKt7p50B3ngAG2Rfw==}
+ dependencies:
+ '@types/hast': 2.3.10
+ fault: 2.0.1
+ highlight.js: 11.8.0
+ dev: false
+
+ /lru-cache@10.2.1:
+ resolution: {integrity: sha512-tS24spDe/zXhWbNPErCHs/AGOzbKGHT+ybSBqmdLm8WZ1xXLWvH8Qn71QPAlqVhd0qUTWjy+Kl9JmISgDdEjsA==}
+ engines: {node: 14 || >=16.14}
+ dev: true
+
+ /lru-cache@5.1.1:
+ resolution: {integrity: sha512-KpNARQA3Iwv+jTA0utUVVbrh+Jlrr1Fv0e56GGzAFOXN7dk/FviaDW8LHmK52DlcH4WP2n6gI8vN1aesBFgo9w==}
+ dependencies:
+ yallist: 3.1.1
+
+ /lru-cache@6.0.0:
+ resolution: {integrity: sha512-Jo6dJ04CmSjuznwJSS3pUeWmd/H0ffTlkXXgwZi+eq1UCmqQwCh+eLsYOYCwY991i2Fah4h1BEMCx4qThGbsiA==}
+ engines: {node: '>=10'}
+ dependencies:
+ yallist: 4.0.0
+ dev: true
+
+ /make-error@1.3.6:
+ resolution: {integrity: sha512-s8UhlNe7vPKomQhC1qFelMokr/Sc3AgNbso3n74mVPA5LTZwkB9NlXf4XPamLxJE8h0gh73rM94xvwRT2CVInw==}
+ dev: true
+
+ /map-cache@0.2.2:
+ resolution: {integrity: sha512-8y/eV9QQZCiyn1SprXSrCmqJN0yNRATe+PO8ztwqrvrbdRLA3eYJF0yaR0YayLWkMbsQSKWS9N2gPcGEc4UsZg==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /map-obj@1.0.1:
+ resolution: {integrity: sha512-7N/q3lyZ+LVCp7PzuxrJr4KMbBE2hW7BT7YNia330OFxIf4d3r5zVpicP2650l7CPN6RM9zOJRl3NGpqSiw3Eg==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /map-obj@2.0.0:
+ resolution: {integrity: sha512-TzQSV2DiMYgoF5RycneKVUzIa9bQsj/B3tTgsE3dOGqlzHnGIDaC7XBE7grnA+8kZPnfqSGFe95VHc2oc0VFUQ==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /map-obj@4.3.0:
+ resolution: {integrity: sha512-hdN1wVrZbb29eBGiGjJbeP8JbKjq1urkHJ/LIP/NY48MZ1QVXUsQBV1G1zvYFHn1XE06cwjBsOI2K3Ulnj1YXQ==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /map-visit@1.0.0:
+ resolution: {integrity: sha512-4y7uGv8bd2WdM9vpQsiQNo41Ln1NvhvDRuVt0k2JZQ+ezN2uaQes7lZeZ+QQUHOLQAtDaBJ+7wCbi+ab/KFs+w==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ object-visit: 1.0.1
+ dev: true
+
+ /markdown-escapes@1.0.4:
+ resolution: {integrity: sha512-8z4efJYk43E0upd0NbVXwgSTQs6cT3T06etieCMEg7dRbzCbxUCK/GHlX8mhHRDcp+OLlHkPKsvqQTCvsRl2cg==}
+ dev: true
+
+ /markdown-table@1.1.3:
+ resolution: {integrity: sha512-1RUZVgQlpJSPWYbFSpmudq5nHY1doEIv89gBtF0s4gW1GF2XorxcA/70M5vq7rLv0a6mhOUccRsqkwhwLCIQ2Q==}
+ dev: true
+
+ /markdown-table@3.0.3:
+ resolution: {integrity: sha512-Z1NL3Tb1M9wH4XESsCDEksWoKTdlUafKc4pt0GRwjUyXaCFZ+dc3g2erqB6zm3szA2IUSi7VnPI+o/9jnxh9hw==}
+ dev: false
+
+ /mathml-tag-names@2.1.3:
+ resolution: {integrity: sha512-APMBEanjybaPzUrfqU0IMU5I0AswKMH7k8OTLs0vvV4KZpExkTkY87nR/zpbuTPj+gARop7aGUbl11pnDfW6xg==}
+ dev: true
+
+ /mdast-util-compact@1.0.4:
+ resolution: {integrity: sha512-3YDMQHI5vRiS2uygEFYaqckibpJtKq5Sj2c8JioeOQBU6INpKbdWzfyLqFFnDwEcEnRFIdMsguzs5pC1Jp4Isg==}
+ dependencies:
+ unist-util-visit: 1.4.1
+ dev: true
+
+ /mdast-util-definitions@5.1.2:
+ resolution: {integrity: sha512-8SVPMuHqlPME/z3gqVwWY4zVXn8lqKv/pAhC57FuJ40ImXyBpmO5ukh98zB2v7Blql2FiHjHv9LVztSIqjY+MA==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ '@types/unist': 2.0.10
+ unist-util-visit: 4.1.2
+ dev: false
+
+ /mdast-util-find-and-replace@2.2.2:
+ resolution: {integrity: sha512-MTtdFRz/eMDHXzeK6W3dO7mXUlF82Gom4y0oOgvHhh/HXZAGvIQDUvQ0SuUx+j2tv44b8xTHOm8K/9OoRFnXKw==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ escape-string-regexp: 5.0.0
+ unist-util-is: 5.2.1
+ unist-util-visit-parents: 5.1.3
+ dev: false
+
+ /mdast-util-from-markdown@1.3.1:
+ resolution: {integrity: sha512-4xTO/M8c82qBcnQc1tgpNtubGUW/Y1tBQ1B0i5CtSoelOLKFYlElIr3bvgREYYO5iRqbMY1YuqZng0GVOI8Qww==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ '@types/unist': 2.0.10
+ decode-named-character-reference: 1.0.2
+ mdast-util-to-string: 3.2.0
+ micromark: 3.2.0
+ micromark-util-decode-numeric-character-reference: 1.1.0
+ micromark-util-decode-string: 1.1.0
+ micromark-util-normalize-identifier: 1.1.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ unist-util-stringify-position: 3.0.3
+ uvu: 0.5.6
+ transitivePeerDependencies:
+ - supports-color
+ dev: false
+
+ /mdast-util-gfm-autolink-literal@1.0.3:
+ resolution: {integrity: sha512-My8KJ57FYEy2W2LyNom4n3E7hKTuQk/0SES0u16tjA9Z3oFkF4RrC/hPAPgjlSpezsOvI8ObcXcElo92wn5IGA==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ ccount: 2.0.1
+ mdast-util-find-and-replace: 2.2.2
+ micromark-util-character: 1.2.0
+ dev: false
+
+ /mdast-util-gfm-footnote@1.0.2:
+ resolution: {integrity: sha512-56D19KOGbE00uKVj3sgIykpwKL179QsVFwx/DCW0u/0+URsryacI4MAdNJl0dh+u2PSsD9FtxPFbHCzJ78qJFQ==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ mdast-util-to-markdown: 1.5.0
+ micromark-util-normalize-identifier: 1.1.0
+ dev: false
+
+ /mdast-util-gfm-strikethrough@1.0.3:
+ resolution: {integrity: sha512-DAPhYzTYrRcXdMjUtUjKvW9z/FNAMTdU0ORyMcbmkwYNbKocDpdk+PX1L1dQgOID/+vVs1uBQ7ElrBQfZ0cuiQ==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ mdast-util-to-markdown: 1.5.0
+ dev: false
+
+ /mdast-util-gfm-table@1.0.7:
+ resolution: {integrity: sha512-jjcpmNnQvrmN5Vx7y7lEc2iIOEytYv7rTvu+MeyAsSHTASGCCRA79Igg2uKssgOs1i1po8s3plW0sTu1wkkLGg==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ markdown-table: 3.0.3
+ mdast-util-from-markdown: 1.3.1
+ mdast-util-to-markdown: 1.5.0
+ transitivePeerDependencies:
+ - supports-color
+ dev: false
+
+ /mdast-util-gfm-task-list-item@1.0.2:
+ resolution: {integrity: sha512-PFTA1gzfp1B1UaiJVyhJZA1rm0+Tzn690frc/L8vNX1Jop4STZgOE6bxUhnzdVSB+vm2GU1tIsuQcA9bxTQpMQ==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ mdast-util-to-markdown: 1.5.0
+ dev: false
+
+ /mdast-util-gfm@2.0.2:
+ resolution: {integrity: sha512-qvZ608nBppZ4icQlhQQIAdc6S3Ffj9RGmzwUKUWuEICFnd1LVkN3EktF7ZHAgfcEdvZB5owU9tQgt99e2TlLjg==}
+ dependencies:
+ mdast-util-from-markdown: 1.3.1
+ mdast-util-gfm-autolink-literal: 1.0.3
+ mdast-util-gfm-footnote: 1.0.2
+ mdast-util-gfm-strikethrough: 1.0.3
+ mdast-util-gfm-table: 1.0.7
+ mdast-util-gfm-task-list-item: 1.0.2
+ mdast-util-to-markdown: 1.5.0
+ transitivePeerDependencies:
+ - supports-color
+ dev: false
+
+ /mdast-util-phrasing@3.0.1:
+ resolution: {integrity: sha512-WmI1gTXUBJo4/ZmSk79Wcb2HcjPJBzM1nlI/OUWA8yk2X9ik3ffNbBGsU+09BFmXaL1IBb9fiuvq6/KMiNycSg==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ unist-util-is: 5.2.1
+ dev: false
+
+ /mdast-util-to-hast@12.3.0:
+ resolution: {integrity: sha512-pits93r8PhnIoU4Vy9bjW39M2jJ6/tdHyja9rrot9uujkN7UTU9SDnE6WNJz/IGyQk3XHX6yNNtrBH6cQzm8Hw==}
+ dependencies:
+ '@types/hast': 2.3.10
+ '@types/mdast': 3.0.15
+ mdast-util-definitions: 5.1.2
+ micromark-util-sanitize-uri: 1.2.0
+ trim-lines: 3.0.1
+ unist-util-generated: 2.0.1
+ unist-util-position: 4.0.4
+ unist-util-visit: 4.1.2
+ dev: false
+
+ /mdast-util-to-markdown@1.5.0:
+ resolution: {integrity: sha512-bbv7TPv/WC49thZPg3jXuqzuvI45IL2EVAr/KxF0BSdHsU0ceFHOmwQn6evxAh1GaoK/6GQ1wp4R4oW2+LFL/A==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ '@types/unist': 2.0.10
+ longest-streak: 3.1.0
+ mdast-util-phrasing: 3.0.1
+ mdast-util-to-string: 3.2.0
+ micromark-util-decode-string: 1.1.0
+ unist-util-visit: 4.1.2
+ zwitch: 2.0.4
+ dev: false
+
+ /mdast-util-to-string@3.2.0:
+ resolution: {integrity: sha512-V4Zn/ncyN1QNSqSBxTrMOLpjr+IKdHl2v3KVLoWmDPscP4r9GcCi71gjgvUV1SFSKh92AjAG4peFuBl2/YgCJg==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ dev: false
+
+ /mdn-data@2.0.14:
+ resolution: {integrity: sha512-dn6wd0uw5GsdswPFfsgMp5NSB0/aDe6fK94YJV/AJDYXL6HVLWBsxeq7js7Ad+mU2K9LAlwpk6kN2D5mwCPVow==}
+
+ /mdn-data@2.0.30:
+ resolution: {integrity: sha512-GaqWWShW4kv/G9IEucWScBx9G1/vsFZZJUO+tD26M8J8z3Kw5RDQjaoZe03YAClgeS/SWPOcb4nkFBTEi5DUEA==}
+ dev: true
+
+ /memfs@3.5.3:
+ resolution: {integrity: sha512-UERzLsxzllchadvbPs5aolHh65ISpKpM+ccLbOJ8/vvpBKmAWf+la7dXFy7Mr0ySHbdHrFv5kGFCUHHe6GFEmw==}
+ engines: {node: '>= 4.0.0'}
+ dependencies:
+ fs-monkey: 1.0.5
+ dev: true
+
+ /memoize-one@5.2.1:
+ resolution: {integrity: sha512-zYiwtZUcYyXKo/np96AGZAckk+FWWsUdJ3cHGGmld7+AhvcWmQyGCYUh1hc4Q/pkOhb65dQR/pqCyK0cOaHz4Q==}
+ dev: false
+
+ /meow@10.1.5:
+ resolution: {integrity: sha512-/d+PQ4GKmGvM9Bee/DPa8z3mXs/pkvJE2KEThngVNOqtmljC6K7NMPxtc2JeZYTmpWb9k/TmxjeL18ez3h7vCw==}
+ engines: {node: ^12.20.0 || ^14.13.1 || >=16.0.0}
+ dependencies:
+ '@types/minimist': 1.2.5
+ camelcase-keys: 7.0.2
+ decamelize: 5.0.1
+ decamelize-keys: 1.1.1
+ hard-rejection: 2.1.0
+ minimist-options: 4.1.0
+ normalize-package-data: 3.0.3
+ read-pkg-up: 8.0.0
+ redent: 4.0.0
+ trim-newlines: 4.1.1
+ type-fest: 1.4.0
+ yargs-parser: 20.2.9
+ dev: true
+
+ /meow@5.0.0:
+ resolution: {integrity: sha512-CbTqYU17ABaLefO8vCU153ZZlprKYWDljcndKKDCFcYQITzWCXZAVk4QMFZPgvzrnUQ3uItnIE/LoUOwrT15Ig==}
+ engines: {node: '>=6'}
+ dependencies:
+ camelcase-keys: 4.2.0
+ decamelize-keys: 1.1.1
+ loud-rejection: 1.6.0
+ minimist-options: 3.0.2
+ normalize-package-data: 2.5.0
+ read-pkg-up: 3.0.0
+ redent: 2.0.0
+ trim-newlines: 2.0.0
+ yargs-parser: 10.1.0
+ dev: true
+
+ /meow@8.1.2:
+ resolution: {integrity: sha512-r85E3NdZ+mpYk1C6RjPFEMSE+s1iZMuHtsHAqY0DT3jZczl0diWUZ8g6oU7h0M9cD2EL+PzaYghhCLzR0ZNn5Q==}
+ engines: {node: '>=10'}
+ dependencies:
+ '@types/minimist': 1.2.5
+ camelcase-keys: 6.2.2
+ decamelize-keys: 1.1.1
+ hard-rejection: 2.1.0
+ minimist-options: 4.1.0
+ normalize-package-data: 3.0.3
+ read-pkg-up: 7.0.1
+ redent: 3.0.0
+ trim-newlines: 3.0.1
+ type-fest: 0.18.1
+ yargs-parser: 20.2.9
+ dev: true
+
+ /merge-options@1.0.1:
+ resolution: {integrity: sha512-iuPV41VWKWBIOpBsjoxjDZw8/GbSfZ2mk7N1453bwMrfzdrIk7EzBd+8UVR6rkw67th7xnk9Dytl3J+lHPdxvg==}
+ engines: {node: '>=4'}
+ dependencies:
+ is-plain-obj: 1.1.0
+ dev: true
+
+ /merge-stream@2.0.0:
+ resolution: {integrity: sha512-abv/qOcuPfk3URPfDzmZU1LKmuw8kT+0nIHvKrKgFrwifol/doWcdA4ZqsWQ8ENrFKkd67Mfpo/LovbIUsbt3w==}
+ dev: true
+
+ /merge2@1.4.1:
+ resolution: {integrity: sha512-8q7VEgMJW4J8tcfVPy8g09NcQwZdbwFEqhe/WZkoIzjn/3TGDwtOCYtXGxA3O8tPzpczCCDgv+P2P5y00ZJOOg==}
+ engines: {node: '>= 8'}
+ dev: true
+
+ /micromark-core-commonmark@1.1.0:
+ resolution: {integrity: sha512-BgHO1aRbolh2hcrzL2d1La37V0Aoz73ymF8rAcKnohLy93titmv62E0gP8Hrx9PKcKrqCZ1BbLGbP3bEhoXYlw==}
+ dependencies:
+ decode-named-character-reference: 1.0.2
+ micromark-factory-destination: 1.1.0
+ micromark-factory-label: 1.1.0
+ micromark-factory-space: 1.1.0
+ micromark-factory-title: 1.1.0
+ micromark-factory-whitespace: 1.1.0
+ micromark-util-character: 1.2.0
+ micromark-util-chunked: 1.1.0
+ micromark-util-classify-character: 1.1.0
+ micromark-util-html-tag-name: 1.2.0
+ micromark-util-normalize-identifier: 1.1.0
+ micromark-util-resolve-all: 1.1.0
+ micromark-util-subtokenize: 1.1.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ uvu: 0.5.6
+ dev: false
+
+ /micromark-extension-gfm-autolink-literal@1.0.5:
+ resolution: {integrity: sha512-z3wJSLrDf8kRDOh2qBtoTRD53vJ+CWIyo7uyZuxf/JAbNJjiHsOpG1y5wxk8drtv3ETAHutCu6N3thkOOgueWg==}
+ dependencies:
+ micromark-util-character: 1.2.0
+ micromark-util-sanitize-uri: 1.2.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ dev: false
+
+ /micromark-extension-gfm-footnote@1.1.2:
+ resolution: {integrity: sha512-Yxn7z7SxgyGWRNa4wzf8AhYYWNrwl5q1Z8ii+CSTTIqVkmGZF1CElX2JI8g5yGoM3GAman9/PVCUFUSJ0kB/8Q==}
+ dependencies:
+ micromark-core-commonmark: 1.1.0
+ micromark-factory-space: 1.1.0
+ micromark-util-character: 1.2.0
+ micromark-util-normalize-identifier: 1.1.0
+ micromark-util-sanitize-uri: 1.2.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ uvu: 0.5.6
+ dev: false
+
+ /micromark-extension-gfm-strikethrough@1.0.7:
+ resolution: {integrity: sha512-sX0FawVE1o3abGk3vRjOH50L5TTLr3b5XMqnP9YDRb34M0v5OoZhG+OHFz1OffZ9dlwgpTBKaT4XW/AsUVnSDw==}
+ dependencies:
+ micromark-util-chunked: 1.1.0
+ micromark-util-classify-character: 1.1.0
+ micromark-util-resolve-all: 1.1.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ uvu: 0.5.6
+ dev: false
+
+ /micromark-extension-gfm-table@1.0.7:
+ resolution: {integrity: sha512-3ZORTHtcSnMQEKtAOsBQ9/oHp9096pI/UvdPtN7ehKvrmZZ2+bbWhi0ln+I9drmwXMt5boocn6OlwQzNXeVeqw==}
+ dependencies:
+ micromark-factory-space: 1.1.0
+ micromark-util-character: 1.2.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ uvu: 0.5.6
+ dev: false
+
+ /micromark-extension-gfm-tagfilter@1.0.2:
+ resolution: {integrity: sha512-5XWB9GbAUSHTn8VPU8/1DBXMuKYT5uOgEjJb8gN3mW0PNW5OPHpSdojoqf+iq1xo7vWzw/P8bAHY0n6ijpXF7g==}
+ dependencies:
+ micromark-util-types: 1.1.0
+ dev: false
+
+ /micromark-extension-gfm-task-list-item@1.0.5:
+ resolution: {integrity: sha512-RMFXl2uQ0pNQy6Lun2YBYT9g9INXtWJULgbt01D/x8/6yJ2qpKyzdZD3pi6UIkzF++Da49xAelVKUeUMqd5eIQ==}
+ dependencies:
+ micromark-factory-space: 1.1.0
+ micromark-util-character: 1.2.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ uvu: 0.5.6
+ dev: false
+
+ /micromark-extension-gfm@2.0.3:
+ resolution: {integrity: sha512-vb9OoHqrhCmbRidQv/2+Bc6pkP0FrtlhurxZofvOEy5o8RtuuvTq+RQ1Vw5ZDNrVraQZu3HixESqbG+0iKk/MQ==}
+ dependencies:
+ micromark-extension-gfm-autolink-literal: 1.0.5
+ micromark-extension-gfm-footnote: 1.1.2
+ micromark-extension-gfm-strikethrough: 1.0.7
+ micromark-extension-gfm-table: 1.0.7
+ micromark-extension-gfm-tagfilter: 1.0.2
+ micromark-extension-gfm-task-list-item: 1.0.5
+ micromark-util-combine-extensions: 1.1.0
+ micromark-util-types: 1.1.0
+ dev: false
+
+ /micromark-factory-destination@1.1.0:
+ resolution: {integrity: sha512-XaNDROBgx9SgSChd69pjiGKbV+nfHGDPVYFs5dOoDd7ZnMAE+Cuu91BCpsY8RT2NP9vo/B8pds2VQNCLiu0zhg==}
+ dependencies:
+ micromark-util-character: 1.2.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ dev: false
+
+ /micromark-factory-label@1.1.0:
+ resolution: {integrity: sha512-OLtyez4vZo/1NjxGhcpDSbHQ+m0IIGnT8BoPamh+7jVlzLJBH98zzuCoUeMxvM6WsNeh8wx8cKvqLiPHEACn0w==}
+ dependencies:
+ micromark-util-character: 1.2.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ uvu: 0.5.6
+ dev: false
+
+ /micromark-factory-space@1.1.0:
+ resolution: {integrity: sha512-cRzEj7c0OL4Mw2v6nwzttyOZe8XY/Z8G0rzmWQZTBi/jjwyw/U4uqKtUORXQrR5bAZZnbTI/feRV/R7hc4jQYQ==}
+ dependencies:
+ micromark-util-character: 1.2.0
+ micromark-util-types: 1.1.0
+ dev: false
+
+ /micromark-factory-title@1.1.0:
+ resolution: {integrity: sha512-J7n9R3vMmgjDOCY8NPw55jiyaQnH5kBdV2/UXCtZIpnHH3P6nHUKaH7XXEYuWwx/xUJcawa8plLBEjMPU24HzQ==}
+ dependencies:
+ micromark-factory-space: 1.1.0
+ micromark-util-character: 1.2.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ dev: false
+
+ /micromark-factory-whitespace@1.1.0:
+ resolution: {integrity: sha512-v2WlmiymVSp5oMg+1Q0N1Lxmt6pMhIHD457whWM7/GUlEks1hI9xj5w3zbc4uuMKXGisksZk8DzP2UyGbGqNsQ==}
+ dependencies:
+ micromark-factory-space: 1.1.0
+ micromark-util-character: 1.2.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ dev: false
+
+ /micromark-util-character@1.2.0:
+ resolution: {integrity: sha512-lXraTwcX3yH/vMDaFWCQJP1uIszLVebzUa3ZHdrgxr7KEU/9mL4mVgCpGbyhvNLNlauROiNUq7WN5u7ndbY6xg==}
+ dependencies:
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ dev: false
+
+ /micromark-util-chunked@1.1.0:
+ resolution: {integrity: sha512-Ye01HXpkZPNcV6FiyoW2fGZDUw4Yc7vT0E9Sad83+bEDiCJ1uXu0S3mr8WLpsz3HaG3x2q0HM6CTuPdcZcluFQ==}
+ dependencies:
+ micromark-util-symbol: 1.1.0
+ dev: false
+
+ /micromark-util-classify-character@1.1.0:
+ resolution: {integrity: sha512-SL0wLxtKSnklKSUplok1WQFoGhUdWYKggKUiqhX+Swala+BtptGCu5iPRc+xvzJ4PXE/hwM3FNXsfEVgoZsWbw==}
+ dependencies:
+ micromark-util-character: 1.2.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ dev: false
+
+ /micromark-util-combine-extensions@1.1.0:
+ resolution: {integrity: sha512-Q20sp4mfNf9yEqDL50WwuWZHUrCO4fEyeDCnMGmG5Pr0Cz15Uo7KBs6jq+dq0EgX4DPwwrh9m0X+zPV1ypFvUA==}
+ dependencies:
+ micromark-util-chunked: 1.1.0
+ micromark-util-types: 1.1.0
+ dev: false
+
+ /micromark-util-decode-numeric-character-reference@1.1.0:
+ resolution: {integrity: sha512-m9V0ExGv0jB1OT21mrWcuf4QhP46pH1KkfWy9ZEezqHKAxkj4mPCy3nIH1rkbdMlChLHX531eOrymlwyZIf2iw==}
+ dependencies:
+ micromark-util-symbol: 1.1.0
+ dev: false
+
+ /micromark-util-decode-string@1.1.0:
+ resolution: {integrity: sha512-YphLGCK8gM1tG1bd54azwyrQRjCFcmgj2S2GoJDNnh4vYtnL38JS8M4gpxzOPNyHdNEpheyWXCTnnTDY3N+NVQ==}
+ dependencies:
+ decode-named-character-reference: 1.0.2
+ micromark-util-character: 1.2.0
+ micromark-util-decode-numeric-character-reference: 1.1.0
+ micromark-util-symbol: 1.1.0
+ dev: false
+
+ /micromark-util-encode@1.1.0:
+ resolution: {integrity: sha512-EuEzTWSTAj9PA5GOAs992GzNh2dGQO52UvAbtSOMvXTxv3Criqb6IOzJUBCmEqrrXSblJIJBbFFv6zPxpreiJw==}
+ dev: false
+
+ /micromark-util-html-tag-name@1.2.0:
+ resolution: {integrity: sha512-VTQzcuQgFUD7yYztuQFKXT49KghjtETQ+Wv/zUjGSGBioZnkA4P1XXZPT1FHeJA6RwRXSF47yvJ1tsJdoxwO+Q==}
+ dev: false
+
+ /micromark-util-normalize-identifier@1.1.0:
+ resolution: {integrity: sha512-N+w5vhqrBihhjdpM8+5Xsxy71QWqGn7HYNUvch71iV2PM7+E3uWGox1Qp90loa1ephtCxG2ftRV/Conitc6P2Q==}
+ dependencies:
+ micromark-util-symbol: 1.1.0
+ dev: false
+
+ /micromark-util-resolve-all@1.1.0:
+ resolution: {integrity: sha512-b/G6BTMSg+bX+xVCshPTPyAu2tmA0E4X98NSR7eIbeC6ycCqCeE7wjfDIgzEbkzdEVJXRtOG4FbEm/uGbCRouA==}
+ dependencies:
+ micromark-util-types: 1.1.0
+ dev: false
+
+ /micromark-util-sanitize-uri@1.2.0:
+ resolution: {integrity: sha512-QO4GXv0XZfWey4pYFndLUKEAktKkG5kZTdUNaTAkzbuJxn2tNBOr+QtxR2XpWaMhbImT2dPzyLrPXLlPhph34A==}
+ dependencies:
+ micromark-util-character: 1.2.0
+ micromark-util-encode: 1.1.0
+ micromark-util-symbol: 1.1.0
+ dev: false
+
+ /micromark-util-subtokenize@1.1.0:
+ resolution: {integrity: sha512-kUQHyzRoxvZO2PuLzMt2P/dwVsTiivCK8icYTeR+3WgbuPqfHgPPy7nFKbeqRivBvn/3N3GBiNC+JRTMSxEC7A==}
+ dependencies:
+ micromark-util-chunked: 1.1.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ uvu: 0.5.6
+ dev: false
+
+ /micromark-util-symbol@1.1.0:
+ resolution: {integrity: sha512-uEjpEYY6KMs1g7QfJ2eX1SQEV+ZT4rUD3UcF6l57acZvLNK7PBZL+ty82Z1qhK1/yXIY4bdx04FKMgR0g4IAag==}
+ dev: false
+
+ /micromark-util-types@1.1.0:
+ resolution: {integrity: sha512-ukRBgie8TIAcacscVHSiddHjO4k/q3pnedmzMQ4iwDcK0FtFCohKOlFbaOL/mPgfnPsL3C1ZyxJa4sbWrBl3jg==}
+ dev: false
+
+ /micromark@3.2.0:
+ resolution: {integrity: sha512-uD66tJj54JLYq0De10AhWycZWGQNUvDI55xPgk2sQM5kn1JYlhbCMTtEeT27+vAhW2FBQxLlOmS3pmA7/2z4aA==}
+ dependencies:
+ '@types/debug': 4.1.12
+ debug: 4.3.4
+ decode-named-character-reference: 1.0.2
+ micromark-core-commonmark: 1.1.0
+ micromark-factory-space: 1.1.0
+ micromark-util-character: 1.2.0
+ micromark-util-chunked: 1.1.0
+ micromark-util-combine-extensions: 1.1.0
+ micromark-util-decode-numeric-character-reference: 1.1.0
+ micromark-util-encode: 1.1.0
+ micromark-util-normalize-identifier: 1.1.0
+ micromark-util-resolve-all: 1.1.0
+ micromark-util-sanitize-uri: 1.2.0
+ micromark-util-subtokenize: 1.1.0
+ micromark-util-symbol: 1.1.0
+ micromark-util-types: 1.1.0
+ uvu: 0.5.6
+ transitivePeerDependencies:
+ - supports-color
+ dev: false
+
+ /micromatch@3.1.0:
+ resolution: {integrity: sha512-3StSelAE+hnRvMs8IdVW7Uhk8CVed5tp+kLLGlBP6WiRAXS21GPGu/Nat4WNPXj2Eoc24B02SaeoyozPMfj0/g==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ arr-diff: 4.0.0
+ array-unique: 0.3.2
+ braces: 2.3.2
+ define-property: 1.0.0
+ extend-shallow: 2.0.1
+ extglob: 2.0.4
+ fragment-cache: 0.2.1
+ kind-of: 5.1.0
+ nanomatch: 1.2.13
+ object.pick: 1.3.0
+ regex-not: 1.0.2
+ snapdragon: 0.8.2
+ to-regex: 3.0.2
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /micromatch@3.1.10:
+ resolution: {integrity: sha512-MWikgl9n9M3w+bpsY3He8L+w9eF9338xRl8IAO5viDizwSzziFEyUzo2xrrloB64ADbTf8uA8vRqqttDTOmccg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ arr-diff: 4.0.0
+ array-unique: 0.3.2
+ braces: 2.3.2
+ define-property: 2.0.2
+ extend-shallow: 3.0.2
+ extglob: 2.0.4
+ fragment-cache: 0.2.1
+ kind-of: 6.0.3
+ nanomatch: 1.2.13
+ object.pick: 1.3.0
+ regex-not: 1.0.2
+ snapdragon: 0.8.2
+ to-regex: 3.0.2
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /micromatch@4.0.5:
+ resolution: {integrity: sha512-DMy+ERcEW2q8Z2Po+WNXuw3c5YaUSFjAO5GsJqfEl7UjvtIuFKO6ZrKvcItdy98dwFI2N1tg3zNIdKaQT+aNdA==}
+ engines: {node: '>=8.6'}
+ dependencies:
+ braces: 3.0.2
+ picomatch: 2.3.1
+ dev: true
+
+ /mime-db@1.52.0:
+ resolution: {integrity: sha512-sPU4uV7dYlvtWJxwwxHD0PuihVNiE7TyAbQ5SWxDCB9mUYvOgroQOwYQQOKPJ8CIbE+1ETVlOoK1UC2nU3gYvg==}
+ engines: {node: '>= 0.6'}
+
+ /mime-types@2.1.35:
+ resolution: {integrity: sha512-ZDY+bPm5zTTF+YpCrAU9nK0UgICYPT0QtT1NZWFv4s++TNkcgVaT0g6+4R2uI4MjQjzysHB1zxuWL50hzaeXiw==}
+ engines: {node: '>= 0.6'}
+ dependencies:
+ mime-db: 1.52.0
+
+ /mimic-fn@2.1.0:
+ resolution: {integrity: sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /mimic-fn@4.0.0:
+ resolution: {integrity: sha512-vqiC06CuhBTUdZH+RYl8sFrL096vA45Ok5ISO6sE/Mr1jRbGH4Csnhi8f3wKVl7x8mO4Au7Ir9D3Oyv1VYMFJw==}
+ engines: {node: '>=12'}
+ dev: true
+
+ /min-indent@1.0.1:
+ resolution: {integrity: sha512-I9jwMn07Sy/IwOj3zVkVik2JTvgpaykDZEigL6Rx6N9LbMywwUSMtxET+7lVoDLLd3O3IXwJwvuuns8UB/HeAg==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /minimatch@3.1.2:
+ resolution: {integrity: sha512-J7p63hRiAjw1NDEww1W7i37+ByIrOWO5XQQAzZ3VOcL0PNybwpfmV/N05zFAzwQ9USyEcX6t3UO+K5aqBQOIHw==}
+ dependencies:
+ brace-expansion: 1.1.11
+ dev: true
+
+ /minimatch@9.0.4:
+ resolution: {integrity: sha512-KqWh+VchfxcMNRAJjj2tnsSJdNbHsVgnkBhTNrW7AjVo6OvLtxw8zfT9oLw1JSohlFzJ8jCoTgaoXvJ+kHt6fw==}
+ engines: {node: '>=16 || 14 >=14.17'}
+ dependencies:
+ brace-expansion: 2.0.1
+ dev: true
+
+ /minimist-options@3.0.2:
+ resolution: {integrity: sha512-FyBrT/d0d4+uiZRbqznPXqw3IpZZG3gl3wKWiX784FycUKVwBt0uLBFkQrtE4tZOrgo78nZp2jnKz3L65T5LdQ==}
+ engines: {node: '>= 4'}
+ dependencies:
+ arrify: 1.0.1
+ is-plain-obj: 1.1.0
+ dev: true
+
+ /minimist-options@4.1.0:
+ resolution: {integrity: sha512-Q4r8ghd80yhO/0j1O3B2BjweX3fiHg9cdOwjJd2J76Q135c+NDxGCqdYKQ1SKBuFfgWbAUzBfvYjPUEeNgqN1A==}
+ engines: {node: '>= 6'}
+ dependencies:
+ arrify: 1.0.1
+ is-plain-obj: 1.1.0
+ kind-of: 6.0.3
+ dev: true
+
+ /minimist@1.2.8:
+ resolution: {integrity: sha512-2yyAR8qBkN3YuheJanUpWC5U3bb5osDywNB8RzDVlDwDHbocAJveqqj1u8+SVD7jkWT4yvsHCpWqqWqAxb0zCA==}
+ dev: true
+
+ /minipass@7.0.4:
+ resolution: {integrity: sha512-jYofLM5Dam9279rdkWzqHozUo4ybjdZmCsDHePy5V/PbBcVMiSZR97gmAy45aqi8CK1lG2ECd356FU86avfwUQ==}
+ engines: {node: '>=16 || 14 >=14.17'}
+ dev: true
+
+ /mixin-deep@1.3.2:
+ resolution: {integrity: sha512-WRoDn//mXBiJ1H40rqa3vH0toePwSsGb45iInWlTySa+Uu4k3tYUSxa2v1KqAiLtvlrSzaExqS1gtk96A9zvEA==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ for-in: 1.0.2
+ is-extendable: 1.0.1
+ dev: true
+
+ /mkdirp@0.5.6:
+ resolution: {integrity: sha512-FP+p8RB8OWpF3YZBCrP5gtADmtXApB5AMLn+vdyA+PyxCjrCs00mjyUozssO33cwDeT3wNGdLxJ5M//YqtHAJw==}
+ hasBin: true
+ dependencies:
+ minimist: 1.2.8
+ dev: true
+
+ /mri@1.2.0:
+ resolution: {integrity: sha512-tzzskb3bG8LvYGFF/mDTpq3jpI6Q9wc3LEmBaghu+DdCssd1FakN7Bc0hVNmEyGq1bq3RgfkCb3cmQLpNPOroA==}
+ engines: {node: '>=4'}
+ dev: false
+
+ /ms@2.0.0:
+ resolution: {integrity: sha512-Tpp60P6IUJDTuOq/5Z8cdskzJujfwqfOTkrwIwj7IRISpnkJnT6SyJ4PCPnGMoFjC9ddhal5KVIYtAt97ix05A==}
+ dev: true
+
+ /ms@2.1.2:
+ resolution: {integrity: sha512-sGkPx+VjMtmA6MX27oA4FBFELFCZZ4S4XqeGOXCv68tT+jb3vk/RyaKWP0PTKyWtmLSM0b+adUTEvbs1PEaH2w==}
+
+ /ms@2.1.3:
+ resolution: {integrity: sha512-6FlzubTLZG3J2a/NVCAleEhjzq5oxgHyaCU9yYXvcLsvoVaHJq/s5xXI6/XXP6tz7R9xAOtHnSO/tXtF3WRTlA==}
+ dev: true
+
+ /mz@2.7.0:
+ resolution: {integrity: sha512-z81GNO7nnYMEhrGh9LeymoE4+Yr0Wn5McHIZMK5cfQCl+NDX08sCZgUc9/6MHni9IWuFLm1Z3HTCXu2z9fN62Q==}
+ dependencies:
+ any-promise: 1.3.0
+ object-assign: 4.1.1
+ thenify-all: 1.6.0
+ dev: true
+
+ /nano-css@5.6.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-T2Mhc//CepkTa3X4pUhKgbEheJHYAxD0VptuqFhDbGMUWVV2m+lkNiW/Ieuj35wrfC8Zm0l7HvssQh7zcEttSw==}
+ peerDependencies:
+ react: '*'
+ react-dom: '*'
+ dependencies:
+ '@jridgewell/sourcemap-codec': 1.4.15
+ css-tree: 1.1.3
+ csstype: 3.1.3
+ fastest-stable-stringify: 2.0.2
+ inline-style-prefixer: 7.0.0
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ rtl-css-js: 1.16.1
+ stacktrace-js: 2.0.2
+ stylis: 4.3.2
+ dev: false
+
+ /nanoid@3.3.7:
+ resolution: {integrity: sha512-eSRppjcPIatRIMC1U6UngP8XFcz8MQWGQdt1MTBQ7NaAmvXDfvNxbvWV3x2y6CdEUciCSsDHDQZbhYaB8QEo2g==}
+ engines: {node: ^10 || ^12 || ^13.7 || ^14 || >=15.0.1}
+ hasBin: true
+
+ /nanomatch@1.2.13:
+ resolution: {integrity: sha512-fpoe2T0RbHwBTBUOftAfBPaDEi06ufaUai0mE6Yn1kacc3SnTErfb/h+X94VXzI64rKFHYImXSvdwGGCmwOqCA==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ arr-diff: 4.0.0
+ array-unique: 0.3.2
+ define-property: 2.0.2
+ extend-shallow: 3.0.2
+ fragment-cache: 0.2.1
+ is-windows: 1.0.2
+ kind-of: 6.0.3
+ object.pick: 1.3.0
+ regex-not: 1.0.2
+ snapdragon: 0.8.2
+ to-regex: 3.0.2
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /natural-compare-lite@1.4.0:
+ resolution: {integrity: sha512-Tj+HTDSJJKaZnfiuw+iaF9skdPpTo2GtEly5JHnWV/hfv2Qj/9RKsGISQtLh2ox3l5EAGw487hnBee0sIJ6v2g==}
+ dev: true
+
+ /natural-compare@1.4.0:
+ resolution: {integrity: sha512-OWND8ei3VtNC9h7V60qff3SVobHr996CTwgxubgyQYEpg290h9J0buyECNNJexkFm5sOajh5G116RYA1c8ZMSw==}
+ dev: true
+
+ /neo-async@2.6.2:
+ resolution: {integrity: sha512-Yd3UES5mWCSqR+qNT93S3UoYUkqAZ9lLg8a7g9rimsWmYGK8cVToA4/sF3RrshdyV3sAGMXVUmpMYOw+dLpOuw==}
+ dev: true
+
+ /node-releases@2.0.14:
+ resolution: {integrity: sha512-y10wOWt8yZpqXmOgRo77WaHEmhYQYGNA6y421PKsKYWEK8aW+cqAphborZDhqfyKrbZEN92CN1X2KbafY2s7Yw==}
+
+ /normalize-package-data@2.5.0:
+ resolution: {integrity: sha512-/5CMN3T0R4XTj4DcGaexo+roZSdSFW/0AOOTROrjxzCG1wrWXEsGbRKevjlIL+ZDE4sZlJr5ED4YW0yqmkK+eA==}
+ dependencies:
+ hosted-git-info: 2.8.9
+ resolve: 1.22.8
+ semver: 5.7.2
+ validate-npm-package-license: 3.0.4
+ dev: true
+
+ /normalize-package-data@3.0.3:
+ resolution: {integrity: sha512-p2W1sgqij3zMMyRC067Dg16bfzVH+w7hyegmpIvZ4JNjqtGOVAIvLmjBx3yP7YTe9vKJgkoNOPjwQGogDoMXFA==}
+ engines: {node: '>=10'}
+ dependencies:
+ hosted-git-info: 4.1.0
+ is-core-module: 2.13.1
+ semver: 7.6.0
+ validate-npm-package-license: 3.0.4
+ dev: true
+
+ /normalize-path@3.0.0:
+ resolution: {integrity: sha512-6eZs5Ls3WtCisHWp9S2GUy8dqkpGi4BVSz3GaqiE6ezub0512ESztXUwUB6C6IKbQkY2Pnb/mD4WYojCRwcwLA==}
+ engines: {node: '>=0.10.0'}
+
+ /normalize-range@0.1.2:
+ resolution: {integrity: sha512-bdok/XvKII3nUpklnV6P2hxtMNrCboOjAcyBuQnWEhO665FwrSNRxU+AqpsyvO6LgGYPspN+lu5CLtw4jPRKNA==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /normalize-selector@0.2.0:
+ resolution: {integrity: sha512-dxvWdI8gw6eAvk9BlPffgEoGfM7AdijoCwOEJge3e3ulT2XLgmU7KvvxprOaCu05Q1uGRHmOhHe1r6emZoKyFw==}
+ dev: true
+
+ /npm-run-path@4.0.1:
+ resolution: {integrity: sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==}
+ engines: {node: '>=8'}
+ dependencies:
+ path-key: 3.1.1
+ dev: true
+
+ /npm-run-path@5.3.0:
+ resolution: {integrity: sha512-ppwTtiJZq0O/ai0z7yfudtBpWIoxM8yE6nHi1X47eFR2EWORqfbu6CnPlNsjeN683eT0qG6H/Pyf9fCcvjnnnQ==}
+ engines: {node: ^12.20.0 || ^14.13.1 || >=16.0.0}
+ dependencies:
+ path-key: 4.0.0
+ dev: true
+
+ /nprogress@0.2.0:
+ resolution: {integrity: sha512-I19aIingLgR1fmhftnbWWO3dXc0hSxqHQHQb3H8m+K3TnEn/iSeTZZOyvKXWqQESMwuUVnatlCnZdLBZZt2VSA==}
+ dev: false
+
+ /nth-check@2.1.1:
+ resolution: {integrity: sha512-lqjrjmaOoAnWfMmBPL+XNnynZh2+swxiX3WUE0s4yEHI6m+AwrK2UZOimIRl3X/4QctVqS8AiZjFqyOGrMXb/w==}
+ dependencies:
+ boolbase: 1.0.0
+ dev: true
+
+ /num2fraction@1.2.2:
+ resolution: {integrity: sha512-Y1wZESM7VUThYY+4W+X4ySH2maqcA+p7UR+w8VWNWVAd6lwuXXWz/w/Cz43J/dI2I+PS6wD5N+bJUF+gjWvIqg==}
+ dev: true
+
+ /numeral@2.0.6:
+ resolution: {integrity: sha512-qaKRmtYPZ5qdw4jWJD6bxEf1FJEqllJrwxCLIm0sQU/A7v2/czigzOb+C2uSiFsa9lBUzeH7M1oK+Q+OLxL3kA==}
+ dev: false
+
+ /object-assign@4.1.1:
+ resolution: {integrity: sha512-rJgTQnkUnH1sFw8yT6VSU3zD3sWmu6sZhIseY8VX+GRu3P6F7Fu+JNDoXfklElbLJSnc3FUQHVe4cU5hj+BcUg==}
+ engines: {node: '>=0.10.0'}
+
+ /object-copy@0.1.0:
+ resolution: {integrity: sha512-79LYn6VAb63zgtmAteVOWo9Vdj71ZVBy3Pbse+VqxDpEP83XuujMrGqHIwAXJ5I/aM0zU7dIyIAhifVTPrNItQ==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ copy-descriptor: 0.1.1
+ define-property: 0.2.5
+ kind-of: 3.2.2
+ dev: true
+
+ /object-hash@3.0.0:
+ resolution: {integrity: sha512-RSn9F68PjH9HqtltsSnqYC1XXoWe9Bju5+213R98cNGttag9q9yAOTzdbsqvIa7aNm5WffBZFpWYr2aWrklWAw==}
+ engines: {node: '>= 6'}
+ dev: true
+
+ /object-inspect@1.13.1:
+ resolution: {integrity: sha512-5qoj1RUiKOMsCCNLV1CBiPYE10sziTsnmNxkAI/rZhiD63CF7IqdFGC/XzjWjpSgLf0LxXX3bDFIh0E18f6UhQ==}
+ dev: true
+
+ /object-is@1.1.6:
+ resolution: {integrity: sha512-F8cZ+KfGlSGi09lJT7/Nd6KJZ9ygtvYC0/UYYLI9nmQKLMnydpB9yvbv9K1uSkEu7FU9vYPmVwLg328tX+ot3Q==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ dev: false
+
+ /object-keys@1.1.1:
+ resolution: {integrity: sha512-NuAESUOUMrlIXOfHKzD6bpPu3tYt3xvjNdRIQ+FeT0lNb4K8WR70CaDxhuNguS2XG+GjkyMwOzsN5ZktImfhLA==}
+ engines: {node: '>= 0.4'}
+
+ /object-visit@1.0.1:
+ resolution: {integrity: sha512-GBaMwwAVK9qbQN3Scdo0OyvgPW7l3lnaVMj84uTOZlswkX0KpF6fyDBJhtTthf7pymztoN36/KEr1DyhF96zEA==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ isobject: 3.0.1
+ dev: true
+
+ /object.assign@4.1.5:
+ resolution: {integrity: sha512-byy+U7gp+FVwmyzKPYhW2h5l3crpmGsxl7X2s8y43IgxvG4g3QZ6CffDtsNQy1WsmZpQbO+ybo0AlW7TY6DcBQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ has-symbols: 1.0.3
+ object-keys: 1.1.1
+ dev: true
+
+ /object.entries@1.1.8:
+ resolution: {integrity: sha512-cmopxi8VwRIAw/fkijJohSfpef5PdN0pMQJN6VC/ZKvn0LIknWD8KtgY6KlQdEc4tIjcQ3HxSMmnvtzIscdaYQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-object-atoms: 1.0.0
+ dev: true
+
+ /object.fromentries@2.0.8:
+ resolution: {integrity: sha512-k6E21FzySsSK5a21KRADBd/NGneRegFO5pLHfdQLpRDETUNJueLXs3WCzyQ3tFRDYgbq3KHGXfTbi2bs8WQ6rQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-object-atoms: 1.0.0
+ dev: true
+
+ /object.groupby@1.0.3:
+ resolution: {integrity: sha512-+Lhy3TQTuzXI5hevh8sBGqbmurHbbIjAi0Z4S63nthVLmLxfbj4T54a4CfZrXIrt9iP4mVAPYMo/v99taj3wjQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ dev: true
+
+ /object.hasown@1.1.4:
+ resolution: {integrity: sha512-FZ9LZt9/RHzGySlBARE3VF+gE26TxR38SdmqOqliuTnl9wrKulaQs+4dee1V+Io8VfxqzAfHu6YuRgUy8OHoTg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-object-atoms: 1.0.0
+ dev: true
+
+ /object.pick@1.3.0:
+ resolution: {integrity: sha512-tqa/UMy/CCoYmj+H5qc07qvSL9dqcs/WZENZ1JbtWBlATP+iVOe778gE6MSijnyCnORzDuX6hU+LA4SZ09YjFQ==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ isobject: 3.0.1
+ dev: true
+
+ /object.values@1.2.0:
+ resolution: {integrity: sha512-yBYjY9QX2hnRmZHAjG/f13MzmBzxzYgQhFrke06TTyKY5zSTEqkOeukBzIdVA3j3ulu8Qa3MbVFShV7T2RmGtQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-object-atoms: 1.0.0
+ dev: true
+
+ /once@1.4.0:
+ resolution: {integrity: sha512-lNaJgI+2Q5URQBkccEKHTQOPaXdUxnZZElQTZY0MFUAuaEqe1E+Nyvgdz/aIyNi6Z9MzO5dv1H8n58/GELp3+w==}
+ dependencies:
+ wrappy: 1.0.2
+ dev: true
+
+ /onetime@5.1.2:
+ resolution: {integrity: sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==}
+ engines: {node: '>=6'}
+ dependencies:
+ mimic-fn: 2.1.0
+ dev: true
+
+ /onetime@6.0.0:
+ resolution: {integrity: sha512-1FlR+gjXK7X+AsAHso35MnyN5KqGwJRi/31ft6x0M194ht7S+rWAvd7PHss9xSKMzE0asv1pyIHaJYq+BbacAQ==}
+ engines: {node: '>=12'}
+ dependencies:
+ mimic-fn: 4.0.0
+ dev: true
+
+ /open@8.4.2:
+ resolution: {integrity: sha512-7x81NCL719oNbsq/3mh+hVrAWmFuEYUqrq/Iw3kUzH8ReypT9QQ0BLoJS7/G9k6N81XjW4qHWtjWwe/9eLy1EQ==}
+ engines: {node: '>=12'}
+ dependencies:
+ define-lazy-prop: 2.0.0
+ is-docker: 2.2.1
+ is-wsl: 2.2.0
+ dev: true
+
+ /optionator@0.9.3:
+ resolution: {integrity: sha512-JjCoypp+jKn1ttEFExxhetCKeJt9zhAgAve5FXHixTvFDW/5aEktX9bufBKLRRMdU7bNtpLfcGu94B3cdEJgjg==}
+ engines: {node: '>= 0.8.0'}
+ dependencies:
+ '@aashutoshrathi/word-wrap': 1.2.6
+ deep-is: 0.1.4
+ fast-levenshtein: 2.0.6
+ levn: 0.4.1
+ prelude-ls: 1.2.1
+ type-check: 0.4.0
+ dev: true
+
+ /p-limit@1.3.0:
+ resolution: {integrity: sha512-vvcXsLAJ9Dr5rQOPk7toZQZJApBl2K4J6dANSsEuh6QI41JYcsS/qhTGa9ErIUUgK3WNQoJYvylxvjqmiqEA9Q==}
+ engines: {node: '>=4'}
+ dependencies:
+ p-try: 1.0.0
+ dev: true
+
+ /p-limit@2.3.0:
+ resolution: {integrity: sha512-//88mFWSJx8lxCzwdAABTJL2MyWB12+eIY7MDL2SqLmAkeKU9qxRvWuSyTjm3FUmpBEMuFfckAIqEaVGUDxb6w==}
+ engines: {node: '>=6'}
+ dependencies:
+ p-try: 2.2.0
+ dev: true
+
+ /p-limit@3.1.0:
+ resolution: {integrity: sha512-TYOanM3wGwNGsZN2cVTYPArw454xnXj5qmWF1bEoAc4+cU/ol7GVh7odevjp1FNHduHc3KZMcFduxU5Xc6uJRQ==}
+ engines: {node: '>=10'}
+ dependencies:
+ yocto-queue: 0.1.0
+ dev: true
+
+ /p-locate@2.0.0:
+ resolution: {integrity: sha512-nQja7m7gSKuewoVRen45CtVfODR3crN3goVQ0DDZ9N3yHxgpkuBhZqsaiotSQRrADUrne346peY7kT3TSACykg==}
+ engines: {node: '>=4'}
+ dependencies:
+ p-limit: 1.3.0
+ dev: true
+
+ /p-locate@3.0.0:
+ resolution: {integrity: sha512-x+12w/To+4GFfgJhBEpiDcLozRJGegY+Ei7/z0tSLkMmxGZNybVMSfWj9aJn8Z5Fc7dBUNJOOVgPv2H7IwulSQ==}
+ engines: {node: '>=6'}
+ dependencies:
+ p-limit: 2.3.0
+ dev: true
+
+ /p-locate@4.1.0:
+ resolution: {integrity: sha512-R79ZZ/0wAxKGu3oYMlz8jy/kbhsNrS7SKZ7PxEHBgJ5+F2mtFW2fK2cOtBh1cHYkQsbzFV7I+EoRKe6Yt0oK7A==}
+ engines: {node: '>=8'}
+ dependencies:
+ p-limit: 2.3.0
+ dev: true
+
+ /p-locate@5.0.0:
+ resolution: {integrity: sha512-LaNjtRWUBY++zB5nE/NwcaoMylSPk+S+ZHNB1TzdbMJMny6dynpAGt7X/tl/QYq3TIeE6nxHppbo2LGymrG5Pw==}
+ engines: {node: '>=10'}
+ dependencies:
+ p-limit: 3.1.0
+ dev: true
+
+ /p-try@1.0.0:
+ resolution: {integrity: sha512-U1etNYuMJoIz3ZXSrrySFjsXQTWOx2/jdi86L+2pRvph/qMKL6sbcCYdH23fqsbm8TH2Gn0OybpT4eSFlCVHww==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /p-try@2.2.0:
+ resolution: {integrity: sha512-R4nPAVTAU0B9D35/Gk3uJf/7XYbQcyohSKdvAxIRSNghFl4e71hVoGnBNQz9cWaXxO2I10KTC+3jMdvvoKw6dQ==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /parchment@1.1.4:
+ resolution: {integrity: sha512-J5FBQt/pM2inLzg4hEWmzQx/8h8D0CiDxaG3vyp9rKrQRSDgBlhjdP5jQGgosEajXPSQouXGHOmVdgo7QmJuOg==}
+ dev: false
+
+ /parent-module@1.0.1:
+ resolution: {integrity: sha512-GQ2EWRpQV8/o+Aw8YqtfZZPfNRWZYkbidE9k5rpl/hC3vtHHBfGm2Ifi6qWV+coDGkrUKZAxE3Lot5kcsRlh+g==}
+ engines: {node: '>=6'}
+ dependencies:
+ callsites: 3.1.0
+
+ /parse-entities@1.2.2:
+ resolution: {integrity: sha512-NzfpbxW/NPrzZ/yYSoQxyqUZMZXIdCfE0OIN4ESsnptHJECoUk3FZktxNuzQf4tjt5UEopnxpYJbvYuxIFDdsg==}
+ dependencies:
+ character-entities: 1.2.4
+ character-entities-legacy: 1.1.4
+ character-reference-invalid: 1.1.4
+ is-alphanumerical: 1.0.4
+ is-decimal: 1.0.4
+ is-hexadecimal: 1.0.4
+ dev: true
+
+ /parse-json@4.0.0:
+ resolution: {integrity: sha512-aOIos8bujGN93/8Ox/jPLh7RwVnPEysynVFE+fQZyg6jKELEHwzgKdLRFHUgXJL6kylijVSBC4BvN9OmsB48Rw==}
+ engines: {node: '>=4'}
+ dependencies:
+ error-ex: 1.3.2
+ json-parse-better-errors: 1.0.2
+ dev: true
+
+ /parse-json@5.2.0:
+ resolution: {integrity: sha512-ayCKvm/phCGxOkYRSCM82iDwct8/EonSEgCSxWxD7ve6jHggsFl4fZVQBPRNgQoKiuV/odhFrGzQXZwbifC8Rg==}
+ engines: {node: '>=8'}
+ dependencies:
+ '@babel/code-frame': 7.24.2
+ error-ex: 1.3.2
+ json-parse-even-better-errors: 2.3.1
+ lines-and-columns: 1.2.4
+
+ /parse5@6.0.1:
+ resolution: {integrity: sha512-Ofn/CTFzRGTTxwpNEs9PP93gXShHcTq255nzRYSKe8AkVpZY7e1fpmTfOyoIvjP5HG7Z2ZM7VS9PPhQGW2pOpw==}
+ dev: false
+
+ /pascalcase@0.1.1:
+ resolution: {integrity: sha512-XHXfu/yOQRy9vYOtUDVMN60OEJjW013GoObG1o+xwQTpB9eYJX/BjXMsdW13ZDPruFhYYn0AG22w0xgQMwl3Nw==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /path-dirname@1.0.2:
+ resolution: {integrity: sha512-ALzNPpyNq9AqXMBjeymIjFDAkAFH06mHJH/cSBHAgU0s4vfpBn6b2nf8tiRLvagKD8RbTpq2FKTBg7cl9l3c7Q==}
+ dev: true
+
+ /path-exists@3.0.0:
+ resolution: {integrity: sha512-bpC7GYwiDYQ4wYLe+FA8lhRjhQCMcQGuSgGGqDkg/QerRWw9CmGRT0iSOVRSZJ29NMLZgIzqaljJ63oaL4NIJQ==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /path-exists@4.0.0:
+ resolution: {integrity: sha512-ak9Qy5Q7jYb2Wwcey5Fpvg2KoAc/ZIhLSLOSBmRmygPsGwkVVt0fZa0qrtMz+m6tJTAHfZQ8FnmB4MG4LWy7/w==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /path-is-absolute@1.0.1:
+ resolution: {integrity: sha512-AVbw3UJ2e9bq64vSaS9Am0fje1Pa8pbGqTTsmXfaIiMpnr5DlDhfJOuLj9Sf95ZPVDAUerDfEk88MPmPe7UCQg==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /path-key@3.1.1:
+ resolution: {integrity: sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /path-key@4.0.0:
+ resolution: {integrity: sha512-haREypq7xkM7ErfgIyA0z+Bj4AGKlMSdlQE2jvJo6huWD1EdkKYV+G/T4nq0YEF2vgTT8kqMFKo1uHn950r4SQ==}
+ engines: {node: '>=12'}
+ dev: true
+
+ /path-parse@1.0.7:
+ resolution: {integrity: sha512-LDJzPVEEEPR+y48z93A0Ed0yXb8pAByGWo/k5YYdYgpY2/2EsOsksJrq7lOHxryrVOn1ejG6oAp8ahvOIQD8sw==}
+
+ /path-scurry@1.10.2:
+ resolution: {integrity: sha512-7xTavNy5RQXnsjANvVvMkEjvloOinkAjv/Z6Ildz9v2RinZ4SBKTWFOVRbaF8p0vpHnyjV/UwNDdKuUv6M5qcA==}
+ engines: {node: '>=16 || 14 >=14.17'}
+ dependencies:
+ lru-cache: 10.2.1
+ minipass: 7.0.4
+ dev: true
+
+ /path-type@3.0.0:
+ resolution: {integrity: sha512-T2ZUsdZFHgA3u4e5PfPbjd7HDDpxPnQb5jN0SrDsjNSuVXHJqtwTnWqG0B1jZrgmJ/7lj1EmVIByWt1gxGkWvg==}
+ engines: {node: '>=4'}
+ dependencies:
+ pify: 3.0.0
+ dev: true
+
+ /path-type@4.0.0:
+ resolution: {integrity: sha512-gDKb8aZMDeD/tZWs9P6+q0J9Mwkdl6xMV8TjnGP3qJVJ06bdMgkbBlLU8IdfOsIsFz2BW1rNVT3XuNEl8zPAvw==}
+ engines: {node: '>=8'}
+
+ /pathe@0.2.0:
+ resolution: {integrity: sha512-sTitTPYnn23esFR3RlqYBWn4c45WGeLcsKzQiUpXJAyfcWkolvlYpV8FLo7JishK946oQwMFUCHXQ9AjGPKExw==}
+ dev: true
+
+ /picocolors@0.2.1:
+ resolution: {integrity: sha512-cMlDqaLEqfSaW8Z7N5Jw+lyIW869EzT73/F5lhtY9cLGoVxSXznfgfXMO0Z5K0o0Q2TkTXq+0KFsdnSe3jDViA==}
+ dev: true
+
+ /picocolors@1.0.0:
+ resolution: {integrity: sha512-1fygroTLlHu66zi26VoTDv8yRgm0Fccecssto+MhsZ0D/DGW2sm8E8AjW7NU5VVTRt5GxbeZ5qBuJr+HyLYkjQ==}
+
+ /picomatch@2.3.1:
+ resolution: {integrity: sha512-JU3teHTNjmE2VCGFzuY8EXzCDVwEqB2a8fsIvwaStHhAWJEeVd1o1QD80CU6+ZdEXXSLbSsuLwJjkCBWqRQUVA==}
+ engines: {node: '>=8.6'}
+
+ /pidtree@0.6.0:
+ resolution: {integrity: sha512-eG2dWTVw5bzqGRztnHExczNxt5VGsE6OwTeCG3fdUf9KBsZzO3R5OIIIzWR+iZA0NtZ+RDVdaoE2dK1cn6jH4g==}
+ engines: {node: '>=0.10'}
+ hasBin: true
+ dev: true
+
+ /pify@2.3.0:
+ resolution: {integrity: sha512-udgsAY+fTnvv7kI7aaxbqwWNb0AHiB0qBO89PZKPkoTmGOgdbrHDKD+0B2X4uTfJ/FT1R09r9gTsjUjNJotuog==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /pify@3.0.0:
+ resolution: {integrity: sha512-C3FsVNH1udSEX48gGX1xfvwTWfsYWj5U+8/uK15BGzIGrKoUpghX8hWZwa/OFnakBiiVNmBvemTJR5mcy7iPcg==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /pify@4.0.1:
+ resolution: {integrity: sha512-uB80kBFb/tfd68bVleG9T5GGsGPjJrLAUpR5PZIrhBnIaRTQRjqdJSsIKkOP6OAIFbj7GOrcudc5pNjZ+geV2g==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /pirates@4.0.6:
+ resolution: {integrity: sha512-saLsH7WeYYPiD25LDuLRRY/i+6HaPYr6G1OUlN39otzkSTxKnubR9RTxS3/Kk50s1g2JTgFwWQDQyplC5/SHZg==}
+ engines: {node: '>= 6'}
+ dev: true
+
+ /pkg-up@3.1.0:
+ resolution: {integrity: sha512-nDywThFk1i4BQK4twPQ6TA4RT8bDY96yeuCVBWL3ePARCiEKDRSrNGbFIgUJpLp+XeIR65v8ra7WuJOFUBtkMA==}
+ engines: {node: '>=8'}
+ dependencies:
+ find-up: 3.0.0
+ dev: true
+
+ /posix-character-classes@0.1.1:
+ resolution: {integrity: sha512-xTgYBc3fuo7Yt7JbiuFxSYGToMoz8fLoE6TC9Wx1P/u+LfeThMOAqmuyECnlBaaJb+u1m9hHiXUEtwW4OzfUJg==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /possible-typed-array-names@1.0.0:
+ resolution: {integrity: sha512-d7Uw+eZoloe0EHDIYoe+bQ5WXnGMOpmiZFTuMWCwpjzzkL2nTjcKiAk4hh8TjnGye2TwWOk3UXucZ+3rbmBa8Q==}
+ engines: {node: '>= 0.4'}
+ dev: true
+
+ /postcss-html@0.36.0(postcss-syntax@0.36.2)(postcss@7.0.39):
+ resolution: {integrity: sha512-HeiOxGcuwID0AFsNAL0ox3mW6MHH5cstWN1Z3Y+n6H+g12ih7LHdYxWwEA/QmrebctLjo79xz9ouK3MroHwOJw==}
+ peerDependencies:
+ postcss: '>=5.0.0'
+ postcss-syntax: '>=0.36.0'
+ dependencies:
+ htmlparser2: 3.10.1
+ postcss: 7.0.39
+ postcss-syntax: 0.36.2(postcss@8.4.38)
+ dev: true
+
+ /postcss-import@15.1.0(postcss@8.4.38):
+ resolution: {integrity: sha512-hpr+J05B2FVYUAXHeK1YyI267J/dDDhMU6B6civm8hSY1jYJnBXxzKDKDswzJmtLHryrjhnDjqqp/49t8FALew==}
+ engines: {node: '>=14.0.0'}
+ peerDependencies:
+ postcss: ^8.0.0
+ dependencies:
+ postcss: 8.4.38
+ postcss-value-parser: 4.2.0
+ read-cache: 1.0.0
+ resolve: 1.22.8
+ dev: true
+
+ /postcss-js@4.0.1(postcss@8.4.38):
+ resolution: {integrity: sha512-dDLF8pEO191hJMtlHFPRa8xsizHaM82MLfNkUHdUtVEV3tgTp5oj+8qbEqYM57SLfc74KSbw//4SeJma2LRVIw==}
+ engines: {node: ^12 || ^14 || >= 16}
+ peerDependencies:
+ postcss: ^8.4.21
+ dependencies:
+ camelcase-css: 2.0.1
+ postcss: 8.4.38
+ dev: true
+
+ /postcss-jsx@0.36.4(postcss-syntax@0.36.2)(postcss@7.0.39):
+ resolution: {integrity: sha512-jwO/7qWUvYuWYnpOb0+4bIIgJt7003pgU3P6nETBLaOyBXuTD55ho21xnals5nBrlpTIFodyd3/jBi6UO3dHvA==}
+ peerDependencies:
+ postcss: '>=5.0.0'
+ postcss-syntax: '>=0.36.0'
+ dependencies:
+ '@babel/core': 7.24.4
+ postcss: 7.0.39
+ postcss-syntax: 0.36.2(postcss@8.4.38)
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /postcss-less@3.1.4:
+ resolution: {integrity: sha512-7TvleQWNM2QLcHqvudt3VYjULVB49uiW6XzEUFmvwHzvsOEF5MwBrIXZDJQvJNFGjJQTzSzZnDoCJ8h/ljyGXA==}
+ engines: {node: '>=6.14.4'}
+ dependencies:
+ postcss: 7.0.39
+ dev: true
+
+ /postcss-load-config@4.0.2(postcss@8.4.38)(ts-node@10.9.2):
+ resolution: {integrity: sha512-bSVhyJGL00wMVoPUzAVAnbEoWyqRxkjv64tUl427SKnPrENtq6hJwUojroMz2VB+Q1edmi4IfrAPpami5VVgMQ==}
+ engines: {node: '>= 14'}
+ peerDependencies:
+ postcss: '>=8.0.9'
+ ts-node: '>=9.0.0'
+ peerDependenciesMeta:
+ postcss:
+ optional: true
+ ts-node:
+ optional: true
+ dependencies:
+ lilconfig: 3.1.1
+ postcss: 8.4.38
+ ts-node: 10.9.2(@types/node@20.12.7)(typescript@5.4.5)
+ yaml: 2.4.1
+ dev: true
+
+ /postcss-markdown@0.36.0(postcss-syntax@0.36.2)(postcss@7.0.39):
+ resolution: {integrity: sha512-rl7fs1r/LNSB2bWRhyZ+lM/0bwKv9fhl38/06gF6mKMo/NPnp55+K1dSTosSVjFZc0e1ppBlu+WT91ba0PMBfQ==}
+ peerDependencies:
+ postcss: '>=5.0.0'
+ postcss-syntax: '>=0.36.0'
+ dependencies:
+ postcss: 7.0.39
+ postcss-syntax: 0.36.2(postcss@8.4.38)
+ remark: 10.0.1
+ unist-util-find-all-after: 1.0.5
+ dev: true
+
+ /postcss-media-query-parser@0.2.3:
+ resolution: {integrity: sha512-3sOlxmbKcSHMjlUXQZKQ06jOswE7oVkXPxmZdoB1r5l0q6gTFTQSHxNxOrCccElbW7dxNytifNEo8qidX2Vsig==}
+ dev: true
+
+ /postcss-nested@6.0.1(postcss@8.4.38):
+ resolution: {integrity: sha512-mEp4xPMi5bSWiMbsgoPfcP74lsWLHkQbZc3sY+jWYd65CUwXrUaTp0fmNpa01ZcETKlIgUdFN/MpS2xZtqL9dQ==}
+ engines: {node: '>=12.0'}
+ peerDependencies:
+ postcss: ^8.2.14
+ dependencies:
+ postcss: 8.4.38
+ postcss-selector-parser: 6.0.16
+ dev: true
+
+ /postcss-nesting@11.3.0(postcss@8.4.38):
+ resolution: {integrity: sha512-JlS10AQm/RzyrUGgl5irVkAlZYTJ99mNueUl+Qab+TcHhVedLiylWVkKBhRale+rS9yWIJK48JVzQlq3LcSdeA==}
+ engines: {node: ^14 || ^16 || >=18}
+ peerDependencies:
+ postcss: ^8.4
+ dependencies:
+ '@csstools/selector-specificity': 2.2.0(postcss-selector-parser@6.0.16)
+ postcss: 8.4.38
+ postcss-selector-parser: 6.0.16
+ dev: true
+
+ /postcss-prefix-selector@1.16.1(postcss@5.2.18):
+ resolution: {integrity: sha512-Umxu+FvKMwlY6TyDzGFoSUnzW+NOfMBLyC1tAkIjgX+Z/qGspJeRjVC903D7mx7TuBpJlwti2ibXtWuA7fKMeQ==}
+ peerDependencies:
+ postcss: '>4 <9'
+ dependencies:
+ postcss: 5.2.18
+ dev: true
+
+ /postcss-reporter@6.0.1:
+ resolution: {integrity: sha512-LpmQjfRWyabc+fRygxZjpRxfhRf9u/fdlKf4VHG4TSPbV2XNsuISzYW1KL+1aQzx53CAppa1bKG4APIB/DOXXw==}
+ engines: {node: '>=6'}
+ dependencies:
+ chalk: 2.4.2
+ lodash: 4.17.21
+ log-symbols: 2.2.0
+ postcss: 7.0.39
+ dev: true
+
+ /postcss-resolve-nested-selector@0.1.1:
+ resolution: {integrity: sha512-HvExULSwLqHLgUy1rl3ANIqCsvMS0WHss2UOsXhXnQaZ9VCc2oBvIpXrl00IUFT5ZDITME0o6oiXeiHr2SAIfw==}
+ dev: true
+
+ /postcss-safe-parser@4.0.2:
+ resolution: {integrity: sha512-Uw6ekxSWNLCPesSv/cmqf2bY/77z11O7jZGPax3ycZMFU/oi2DMH9i89AdHc1tRwFg/arFoEwX0IS3LCUxJh1g==}
+ engines: {node: '>=6.0.0'}
+ dependencies:
+ postcss: 7.0.39
+ dev: true
+
+ /postcss-safe-parser@6.0.0(postcss@8.4.38):
+ resolution: {integrity: sha512-FARHN8pwH+WiS2OPCxJI8FuRJpTVnn6ZNFiqAM2aeW2LwTHWWmWgIyKC6cUo0L8aeKiF/14MNvnpls6R2PBeMQ==}
+ engines: {node: '>=12.0'}
+ peerDependencies:
+ postcss: ^8.3.3
+ dependencies:
+ postcss: 8.4.38
+ dev: true
+
+ /postcss-sass@0.3.5:
+ resolution: {integrity: sha512-B5z2Kob4xBxFjcufFnhQ2HqJQ2y/Zs/ic5EZbCywCkxKd756Q40cIQ/veRDwSrw1BF6+4wUgmpm0sBASqVi65A==}
+ dependencies:
+ gonzales-pe: 4.3.0
+ postcss: 7.0.39
+ dev: true
+
+ /postcss-scss@2.1.1:
+ resolution: {integrity: sha512-jQmGnj0hSGLd9RscFw9LyuSVAa5Bl1/KBPqG1NQw9w8ND55nY4ZEsdlVuYJvLPpV+y0nwTV5v/4rHPzZRihQbA==}
+ engines: {node: '>=6.0.0'}
+ dependencies:
+ postcss: 7.0.39
+ dev: true
+
+ /postcss-selector-parser@3.1.2:
+ resolution: {integrity: sha512-h7fJ/5uWuRVyOtkO45pnt1Ih40CEleeyCHzipqAZO2e5H20g25Y48uYnFUiShvY4rZWNJ/Bib/KVPmanaCtOhA==}
+ engines: {node: '>=8'}
+ dependencies:
+ dot-prop: 5.3.0
+ indexes-of: 1.0.1
+ uniq: 1.0.1
+ dev: true
+
+ /postcss-selector-parser@6.0.16:
+ resolution: {integrity: sha512-A0RVJrX+IUkVZbW3ClroRWurercFhieevHB38sr2+l9eUClMqome3LmEmnhlNy+5Mr2EYN6B2Kaw9wYdd+VHiw==}
+ engines: {node: '>=4'}
+ dependencies:
+ cssesc: 3.0.0
+ util-deprecate: 1.0.2
+ dev: true
+
+ /postcss-sorting@4.1.0:
+ resolution: {integrity: sha512-r4T2oQd1giURJdHQ/RMb72dKZCuLOdWx2B/XhXN1Y1ZdnwXsKH896Qz6vD4tFy9xSjpKNYhlZoJmWyhH/7JUQw==}
+ engines: {node: '>=6.14.3'}
+ dependencies:
+ lodash: 4.17.21
+ postcss: 7.0.39
+ dev: true
+
+ /postcss-sorting@8.0.2(postcss@8.4.38):
+ resolution: {integrity: sha512-M9dkSrmU00t/jK7rF6BZSZauA5MAaBW4i5EnJXspMwt4iqTh/L9j6fgMnbElEOfyRyfLfVbIHj/R52zHzAPe1Q==}
+ peerDependencies:
+ postcss: ^8.4.20
+ dependencies:
+ postcss: 8.4.38
+ dev: true
+
+ /postcss-syntax@0.36.2(postcss@8.4.38):
+ resolution: {integrity: sha512-nBRg/i7E3SOHWxF3PpF5WnJM/jQ1YpY9000OaVXlAQj6Zp/kIqJxEDWIZ67tAd7NLuk7zqN4yqe9nc0oNAOs1w==}
+ peerDependencies:
+ postcss: '>=5.0.0'
+ postcss-html: '*'
+ postcss-jsx: '*'
+ postcss-less: '*'
+ postcss-markdown: '*'
+ postcss-scss: '*'
+ peerDependenciesMeta:
+ postcss-html:
+ optional: true
+ postcss-jsx:
+ optional: true
+ postcss-less:
+ optional: true
+ postcss-markdown:
+ optional: true
+ postcss-scss:
+ optional: true
+ dependencies:
+ postcss: 8.4.38
+ dev: true
+
+ /postcss-value-parser@3.3.1:
+ resolution: {integrity: sha512-pISE66AbVkp4fDQ7VHBwRNXzAAKJjw4Vw7nWI/+Q3vuly7SNfgYXvm6i5IgFylHGK5sP/xHAbB7N49OS4gWNyQ==}
+ dev: true
+
+ /postcss-value-parser@4.2.0:
+ resolution: {integrity: sha512-1NNCs6uurfkVbeXG4S8JFT9t19m45ICnif8zWLd5oPSZ50QnwMfK+H3jv408d4jw/7Bttv5axS5IiHoLaVNHeQ==}
+
+ /postcss@5.2.18:
+ resolution: {integrity: sha512-zrUjRRe1bpXKsX1qAJNJjqZViErVuyEkMTRrwu4ud4sbTtIBRmtaYDrHmcGgmrbsW3MHfmtIf+vJumgQn+PrXg==}
+ engines: {node: '>=0.12'}
+ dependencies:
+ chalk: 1.1.3
+ js-base64: 2.6.4
+ source-map: 0.5.7
+ supports-color: 3.2.3
+ dev: true
+
+ /postcss@7.0.39:
+ resolution: {integrity: sha512-yioayjNbHn6z1/Bywyb2Y4s3yvDAeXGOyxqD+LnVOinq6Mdmd++SW2wUNVzavyyHxd6+DxzWGIuosg6P1Rj8uA==}
+ engines: {node: '>=6.0.0'}
+ dependencies:
+ picocolors: 0.2.1
+ source-map: 0.6.1
+ dev: true
+
+ /postcss@8.4.31:
+ resolution: {integrity: sha512-PS08Iboia9mts/2ygV3eLpY5ghnUcfLV/EXTOW1E2qYxJKGGBUtNjN76FYHnMs36RmARn41bC0AZmn+rR0OVpQ==}
+ engines: {node: ^10 || ^12 || >=14}
+ dependencies:
+ nanoid: 3.3.7
+ picocolors: 1.0.0
+ source-map-js: 1.2.0
+ dev: false
+
+ /postcss@8.4.38:
+ resolution: {integrity: sha512-Wglpdk03BSfXkHoQa3b/oulrotAkwrlLDRSOb9D0bN86FdRyE9lppSp33aHNPgBa0JKCoB+drFLZkQoRRYae5A==}
+ engines: {node: ^10 || ^12 || >=14}
+ dependencies:
+ nanoid: 3.3.7
+ picocolors: 1.0.0
+ source-map-js: 1.2.0
+
+ /posthtml-parser@0.2.1:
+ resolution: {integrity: sha512-nPC53YMqJnc/+1x4fRYFfm81KV2V+G9NZY+hTohpYg64Ay7NemWWcV4UWuy/SgMupqQ3kJ88M/iRfZmSnxT+pw==}
+ dependencies:
+ htmlparser2: 3.10.1
+ isobject: 2.1.0
+ dev: true
+
+ /posthtml-rename-id@1.0.12:
+ resolution: {integrity: sha512-UKXf9OF/no8WZo9edRzvuMenb6AD5hDLzIepJW+a4oJT+T/Lx7vfMYWT4aWlGNQh0WMhnUx1ipN9OkZ9q+ddEw==}
+ dependencies:
+ escape-string-regexp: 1.0.5
+ dev: true
+
+ /posthtml-render@1.4.0:
+ resolution: {integrity: sha512-W1779iVHGfq0Fvh2PROhCe2QhB8mEErgqzo1wpIt36tCgChafP+hbXIhLDOM8ePJrZcFs0vkNEtdibEWVqChqw==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /posthtml-svg-mode@1.0.3:
+ resolution: {integrity: sha512-hEqw9NHZ9YgJ2/0G7CECOeuLQKZi8HjWLkBaSVtOWjygQ9ZD8P7tqeowYs7WrFdKsWEKG7o+IlsPY8jrr0CJpQ==}
+ dependencies:
+ merge-options: 1.0.1
+ posthtml: 0.9.2
+ posthtml-parser: 0.2.1
+ posthtml-render: 1.4.0
+ dev: true
+
+ /posthtml@0.9.2:
+ resolution: {integrity: sha512-spBB5sgC4cv2YcW03f/IAUN1pgDJWNWD8FzkyY4mArLUMJW+KlQhlmUdKAHQuPfb00Jl5xIfImeOsf6YL8QK7Q==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ posthtml-parser: 0.2.1
+ posthtml-render: 1.4.0
+ dev: true
+
+ /preact@10.12.1:
+ resolution: {integrity: sha512-l8386ixSsBdbreOAkqtrwqHwdvR35ID8c3rKPa8lCWuO86dBi32QWHV4vfsZK1utLLFMvw+Z5Ad4XLkZzchscg==}
+ dev: false
+
+ /prelude-ls@1.2.1:
+ resolution: {integrity: sha512-vkcDPrRZo1QZLbn5RLGPpg/WmIQ65qoWWhcGKf/b5eplkkarX0m9z8ppCat4mlOqUsWpyNuYgO3VRyrYHSzX5g==}
+ engines: {node: '>= 0.8.0'}
+ dev: true
+
+ /prettier-linter-helpers@1.0.0:
+ resolution: {integrity: sha512-GbK2cP9nraSSUF9N2XwUwqfzlAFlMNYYl+ShE/V+H8a9uNl/oUqB1w2EL54Jh0OlyRSd8RfWYJ3coVS4TROP2w==}
+ engines: {node: '>=6.0.0'}
+ dependencies:
+ fast-diff: 1.3.0
+ dev: true
+
+ /prettier-plugin-tailwindcss@0.3.0(prettier@2.8.8):
+ resolution: {integrity: sha512-009/Xqdy7UmkcTBpwlq7jsViDqXAYSOMLDrHAdTMlVZOrKfM2o9Ci7EMWTMZ7SkKBFTG04UM9F9iM2+4i6boDA==}
+ engines: {node: '>=12.17.0'}
+ peerDependencies:
+ '@ianvs/prettier-plugin-sort-imports': '*'
+ '@prettier/plugin-pug': '*'
+ '@shopify/prettier-plugin-liquid': '*'
+ '@shufo/prettier-plugin-blade': '*'
+ '@trivago/prettier-plugin-sort-imports': '*'
+ prettier: '>=2.2.0'
+ prettier-plugin-astro: '*'
+ prettier-plugin-css-order: '*'
+ prettier-plugin-import-sort: '*'
+ prettier-plugin-jsdoc: '*'
+ prettier-plugin-marko: '*'
+ prettier-plugin-organize-attributes: '*'
+ prettier-plugin-organize-imports: '*'
+ prettier-plugin-style-order: '*'
+ prettier-plugin-svelte: '*'
+ prettier-plugin-twig-melody: '*'
+ peerDependenciesMeta:
+ '@ianvs/prettier-plugin-sort-imports':
+ optional: true
+ '@prettier/plugin-pug':
+ optional: true
+ '@shopify/prettier-plugin-liquid':
+ optional: true
+ '@shufo/prettier-plugin-blade':
+ optional: true
+ '@trivago/prettier-plugin-sort-imports':
+ optional: true
+ prettier-plugin-astro:
+ optional: true
+ prettier-plugin-css-order:
+ optional: true
+ prettier-plugin-import-sort:
+ optional: true
+ prettier-plugin-jsdoc:
+ optional: true
+ prettier-plugin-marko:
+ optional: true
+ prettier-plugin-organize-attributes:
+ optional: true
+ prettier-plugin-organize-imports:
+ optional: true
+ prettier-plugin-style-order:
+ optional: true
+ prettier-plugin-svelte:
+ optional: true
+ prettier-plugin-twig-melody:
+ optional: true
+ dependencies:
+ prettier: 2.8.8
+ dev: true
+
+ /prettier@2.8.8:
+ resolution: {integrity: sha512-tdN8qQGvNjw4CHbY+XXk0JgCXn9QiF21a55rBe5LJAU+kDyC4WQn4+awm2Xfk2lQMk5fKup9XgzTZtGkjBdP9Q==}
+ engines: {node: '>=10.13.0'}
+ hasBin: true
+ dev: true
+
+ /prompts@2.4.2:
+ resolution: {integrity: sha512-NxNv/kLguCA7p3jE8oL2aEBsrJWgAakBpgmgK6lpPWV+WuOmY6r2/zbAVnP+T8bQlA0nzHXSJSJW0Hq7ylaD2Q==}
+ engines: {node: '>= 6'}
+ dependencies:
+ kleur: 3.0.3
+ sisteransi: 1.0.5
+ dev: true
+
+ /prop-types@15.8.1:
+ resolution: {integrity: sha512-oj87CgZICdulUohogVAR7AjlC0327U4el4L6eAvOqCeudMDVU0NThNaV+b9Df4dXgSP1gXMTnPdhfe/2qDH5cg==}
+ dependencies:
+ loose-envify: 1.4.0
+ object-assign: 4.1.1
+ react-is: 16.13.1
+
+ /property-information@6.5.0:
+ resolution: {integrity: sha512-PgTgs/BlvHxOu8QuEN7wi5A0OmXaBcHpmCSTehcs6Uuu9IkDIEo13Hy7n898RHfrQ49vKCoGeWZSaAK01nwVig==}
+ dev: false
+
+ /proxy-from-env@1.1.0:
+ resolution: {integrity: sha512-D+zkORCbA9f1tdWRK0RaCR3GPv50cMxcrz4X8k5LTSUD1Dkw47mKJEZQNunItRTkWwgtaUSo1RVFRIG9ZXiFYg==}
+ dev: false
+
+ /punycode@2.3.1:
+ resolution: {integrity: sha512-vYt7UD1U9Wg6138shLtLOvdAu+8DsC/ilFtEVHcH+wydcSpNE20AfSOduf6MkRFahL5FY7X1oU7nKVZFtfq8Fg==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /qrcode.react@3.1.0(react@18.3.0):
+ resolution: {integrity: sha512-oyF+Urr3oAMUG/OiOuONL3HXM+53wvuH3mtIWQrYmsXoAq0DkvZp2RYUWFSMFtbdOpuS++9v+WAkzNVkMlNW6Q==}
+ peerDependencies:
+ react: ^16.8.0 || ^17.0.0 || ^18.0.0
+ dependencies:
+ react: 18.3.0
+ dev: false
+
+ /query-string@4.3.4:
+ resolution: {integrity: sha512-O2XLNDBIg1DnTOa+2XrIwSiXEV8h2KImXUnjhhn2+UsvZ+Es2uyd5CCRTNQlDGbzUQOW3aYCBx9rVA6dzsiY7Q==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ object-assign: 4.1.1
+ strict-uri-encode: 1.1.0
+ dev: true
+
+ /queue-microtask@1.2.3:
+ resolution: {integrity: sha512-NuaNSa6flKT5JaSYQzJok04JzTL1CA6aGhv5rfLW3PgqA+M2ChpZQnAC8h8i4ZFkBS8X5RqkDBHA7r4hej3K9A==}
+ dev: true
+
+ /quick-lru@1.1.0:
+ resolution: {integrity: sha512-tRS7sTgyxMXtLum8L65daJnHUhfDUgboRdcWW2bR9vBfrj2+O5HSMbQOJfJJjIVSPFqbBCF37FpwWXGitDc5tA==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /quick-lru@4.0.1:
+ resolution: {integrity: sha512-ARhCpm70fzdcvNQfPoy49IaanKkTlRWF2JMzqhcJbhSFRZv7nPTvZJdcY7301IPmvW+/p0RgIWnQDLJxifsQ7g==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /quick-lru@5.1.1:
+ resolution: {integrity: sha512-WuyALRjWPDGtt/wzJiadO5AXY+8hZ80hVpe6MyivgraREW751X3SbhRvG3eLKOYN+8VEvqLcf3wdnt44Z4S4SA==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /quill-delta@3.6.3:
+ resolution: {integrity: sha512-wdIGBlcX13tCHOXGMVnnTVFtGRLoP0imqxM696fIPwIf5ODIYUHIvHbZcyvGlZFiFhK5XzDC2lpjbxRhnM05Tg==}
+ engines: {node: '>=0.10'}
+ dependencies:
+ deep-equal: 1.1.2
+ extend: 3.0.2
+ fast-diff: 1.1.2
+ dev: false
+
+ /quill@1.3.7:
+ resolution: {integrity: sha512-hG/DVzh/TiknWtE6QmWAF/pxoZKYxfe3J/d/+ShUWkDvvkZQVTPeVmUJVu1uE6DDooC4fWTiCLh84ul89oNz5g==}
+ dependencies:
+ clone: 2.1.2
+ deep-equal: 1.1.2
+ eventemitter3: 2.0.3
+ extend: 3.0.2
+ parchment: 1.1.4
+ quill-delta: 3.6.3
+ dev: false
+
+ /raf-schd@4.0.3:
+ resolution: {integrity: sha512-tQkJl2GRWh83ui2DiPTJz9wEiMN20syf+5oKfB03yYP7ioZcJwsIK8FjrtLwH1m7C7e+Tt2yYBlrOpdT+dyeIQ==}
+ dev: false
+
+ /ramda@0.29.1:
+ resolution: {integrity: sha512-OfxIeWzd4xdUNxlWhgFazxsA/nl3mS4/jGZI5n00uWOoSSFRhC1b6gl6xvmzUamgmqELraWp0J/qqVlXYPDPyA==}
+ dev: false
+
+ /randombytes@2.1.0:
+ resolution: {integrity: sha512-vYl3iOX+4CKUWuxGi9Ukhie6fsqXqS9FE2Zaic4tNFD2N2QQaXOMFbuKK4QmDHC0JO6B1Zp41J0LpT0oR68amQ==}
+ dependencies:
+ safe-buffer: 5.2.1
+ dev: true
+
+ /rc-cascader@3.24.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-RgKuYgEGPx+6wCgguYFHjMsDZdCyydZd58YJRCfYQ8FObqLnZW0x/vUcEyPjhWIj1EhjV958IcR+NFPDbbj9kg==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ array-tree-filter: 2.1.0
+ classnames: 2.5.1
+ rc-select: 14.13.1(react-dom@18.3.0)(react@18.3.0)
+ rc-tree: 5.8.5(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-checkbox@3.2.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-8inzw4y9dAhZmv/Ydl59Qdy5tdp9CKg4oPVcRigi+ga/yKPZS5m5SyyQPtYSgbcqHRYOdUhiPSeKfktc76du1A==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-collapse@3.7.3(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-60FJcdTRn0X5sELF18TANwtVi7FtModq649H11mYF1jh83DniMoM4MqY627sEKRCTm4+WXfGDcB7hY5oW6xhyw==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-dialog@9.4.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-AScCexaLACvf8KZRqCPz12BJ8olszXOS4lKlkMyzDQHS1m0zj1KZMYgmMCh39ee0Dcv8kyrj8mTqxuLyhH+QuQ==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/portal': 1.1.2(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-drawer@7.1.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-nBE1rF5iZvpavoyqhSSz2mk/yANltA7g3aF0U45xkx381n3we/RKs9cJfNKp9mSWCedOKWt9FLEwZDaAaOGn2w==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/portal': 1.1.2(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-dropdown@4.2.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-odM8Ove+gSh0zU27DUj5cG1gNKg7mLWBYzB5E4nNLrLwBmYEgYP43vHKDGOVZcJSVElQBI0+jTQgjnq0NfLjng==}
+ peerDependencies:
+ react: '>=16.11.0'
+ react-dom: '>=16.11.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/trigger': 2.1.1(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-field-form@1.44.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-el7w87fyDUsca63Y/s8qJcq9kNkf/J5h+iTdqG5WsSHLH0e6Usl7QuYSmSVzJMgtp40mOVZIY/W/QP9zwrp1FA==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ async-validator: 4.2.5
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-image@7.6.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-tL3Rvd1sS+frZQ01i+tkeUPaOeFz2iG9/scAt/Cfs0hyCRVA/w0Pu1J/JxIX8blalvmHE0bZQRYdOmRAzWu4Hg==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/portal': 1.1.2(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ rc-dialog: 9.4.0(react-dom@18.3.0)(react@18.3.0)
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-input-number@9.0.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-RfcDBDdWFFetouWFXBA+WPEC8LzBXyngr9b+yTLVIygfFu7HiLRGn/s/v9wwno94X7KFvnb28FNynMGj9XJlDQ==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/mini-decimal': 1.1.0
+ classnames: 2.5.1
+ rc-input: 1.4.5(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-input@1.4.5(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-AjzykhwnwYTRSwwgCu70CGKBIAv6bP2nqnFptnNTprph/TF1BAs0Qxl91mie/BR6n827WIJB6ZjaRf9iiMwAfw==}
+ peerDependencies:
+ react: '>=16.0.0'
+ react-dom: '>=16.0.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-mentions@2.11.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-upb4AK1SRFql7qGnbLEvJqLMugVVIyjmwBJW9L0eLoN9po4JmJZaBzmKA4089fNtsU8k6l/tdZiVafyooeKnLw==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/trigger': 2.1.1(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ rc-input: 1.4.5(react-dom@18.3.0)(react@18.3.0)
+ rc-menu: 9.13.0(react-dom@18.3.0)(react@18.3.0)
+ rc-textarea: 1.6.3(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-menu@9.13.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-1l8ooCB3HcYJKCltC/s7OxRKRjgymdl9htrCeGZcXNaMct0RxZRK6OPV3lPhVksIvAGMgzPd54ClpZ5J4b8cZA==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/trigger': 2.1.1(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-overflow: 1.3.2(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-motion@2.9.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-XIU2+xLkdIr1/h6ohPZXyPBMvOmuyFZQ/T0xnawz+Rh+gh4FINcnZmMT5UTIj6hgI0VLDjTaPeRd+smJeSPqiQ==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-notification@5.4.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-li19y9RoYJciF3WRFvD+DvWS70jdL8Fr+Gfb/OshK+iY6iTkwzoigmSIp76/kWh5tF5i/i9im12X3nsF85GYdA==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-overflow@1.3.2(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-nsUm78jkYAoPygDAcGZeC2VwIg/IBGSodtOY3pMof4W3M9qRJgqaDYm03ZayHlde3I6ipliAxbN0RUcGf5KOzw==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-resize-observer: 1.4.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-pagination@4.0.4(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-GGrLT4NgG6wgJpT/hHIpL9nELv27A1XbSZzECIuQBQTVSf4xGKxWr6I/jhpRPauYEWEbWVw22ObG6tJQqwJqWQ==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-picker@4.4.2(dayjs@1.11.10)(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-MdbAXvwiGyhb+bHe66qPps8xPQivzEgcyCp3/MPK4T+oER0gOmVRCEDxaD4FhYG/7GLH3rDrHpu79BvEn2JFTQ==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ date-fns: '>= 2.x'
+ dayjs: '>= 1.x'
+ luxon: '>= 3.x'
+ moment: '>= 2.x'
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ peerDependenciesMeta:
+ date-fns:
+ optional: true
+ dayjs:
+ optional: true
+ luxon:
+ optional: true
+ moment:
+ optional: true
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/trigger': 2.1.1(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ dayjs: 1.11.10
+ rc-overflow: 1.3.2(react-dom@18.3.0)(react@18.3.0)
+ rc-resize-observer: 1.4.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-progress@4.0.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-oofVMMafOCokIUIBnZLNcOZFsABaUw8PPrf1/y0ZBvKZNpOiu5h4AO9vv11Sw0p4Hb3D0yGWuEattcQGtNJ/aw==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-rate@2.12.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-g092v5iZCdVzbjdn28FzvWebK2IutoVoiTeqoLTj9WM7SjA/gOJIw5/JFZMRyJYYVe1jLAU2UhAfstIpCNRozg==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-resize-observer@1.4.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-PnMVyRid9JLxFavTjeDXEXo65HCRqbmLBw9xX9gfC4BZiSzbLXKzW3jPz+J0P71pLbD5tBMTT+mkstV5gD0c9Q==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ resize-observer-polyfill: 1.5.1
+ dev: false
+
+ /rc-segmented@2.3.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-I3FtM5Smua/ESXutFfb8gJ8ZPcvFR+qUgeeGFQHBOvRiRKyAk4aBE5nfqrxXx+h8/vn60DQjOt6i4RNtrbOobg==}
+ peerDependencies:
+ react: '>=16.0.0'
+ react-dom: '>=16.0.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-select@14.13.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-A1VHqjIOemxLnUGRxLGVqXBs8jGcJemI5NXxOJwU5PQc1wigAu1T4PRLgMkTPDOz8gPhlY9dwsPzMgakMc2QjQ==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '*'
+ react-dom: '*'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/trigger': 2.1.1(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-overflow: 1.3.2(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ rc-virtual-list: 3.11.5(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-slider@10.6.2(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-FjkoFjyvUQWcBo1F3RgSglky3ar0+qHLM41PlFVYB4Bj3RD8E/Mv7kqMouLFBU+3aFglMzzctAIWRwajEuueSw==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-steps@6.0.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-lKHL+Sny0SeHkQKKDJlAjV5oZ8DwCdS2hFhAkIjuQt1/pB81M0cA0ErVFdHq9+jmPmFw1vJB2F5NBzFXLJxV+g==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-switch@4.1.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-TI8ufP2Az9oEbvyCeVE4+90PDSljGyuwix3fV58p7HV2o4wBnVToEyomJRVyTaZeqNPAp+vqeo4Wnj5u0ZZQBg==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-table@7.45.4(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-6aSbGrnkN2GLSt3s1x+wa4f3j/VEgg1uKPpaLY5qHH1/nFyreS2V7DFJ0TfUb18allf2FQl7oVYEjTixlBXEyQ==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/context': 1.4.0(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ rc-resize-observer: 1.4.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ rc-virtual-list: 3.11.5(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-tabs@14.1.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-5nOr9PVpJy2SWHTLgv1+kESDOb0tFzl0cYU9r9d8LfL0Wg9i/n1B558rmkxdQHgBwMqxmwoyPSAbQROxMQe8nw==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-dropdown: 4.2.0(react-dom@18.3.0)(react@18.3.0)
+ rc-menu: 9.13.0(react-dom@18.3.0)(react@18.3.0)
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-resize-observer: 1.4.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-textarea@1.6.3(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-8k7+8Y2GJ/cQLiClFMg8kUXOOdvcFQrnGeSchOvI2ZMIVvX5a3zQpLxoODL0HTrvU63fPkRmMuqaEcOF9dQemA==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-input: 1.4.5(react-dom@18.3.0)(react@18.3.0)
+ rc-resize-observer: 1.4.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-tooltip@6.2.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-iS/3iOAvtDh9GIx1ulY7EFUXUtktFccNLsARo3NPgLf0QW9oT0w3dA9cYWlhqAKmD+uriEwdWz1kH0Qs4zk2Aw==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@rc-component/trigger': 2.1.1(react-dom@18.3.0)(react@18.3.0)
+ classnames: 2.5.1
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-tree-select@5.19.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-f4l5EsmSGF3ggj76YTzKNPY9SnXfFaer7ZccTSGb3urUf54L+cCqyT+UsPr+S5TAr8mZSxJ7g3CgkCe+cVQ6sw==}
+ peerDependencies:
+ react: '*'
+ react-dom: '*'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-select: 14.13.1(react-dom@18.3.0)(react@18.3.0)
+ rc-tree: 5.8.5(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-tree@5.8.5(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-PRfcZtVDNkR7oh26RuNe1hpw11c1wfgzwmPFL0lnxGnYefe9lDAO6cg5wJKIAwyXFVt5zHgpjYmaz0CPy1ZtKg==}
+ engines: {node: '>=10.x'}
+ peerDependencies:
+ react: '*'
+ react-dom: '*'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-motion: 2.9.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ rc-virtual-list: 3.11.5(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-upload@4.5.2(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-QO3ne77DwnAPKFn0bA5qJM81QBjQi0e0NHdkvpFyY73Bea2NfITiotqJqVjHgeYPOJu5lLVR32TNGP084aSoXA==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /rc-util@5.39.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-OW/ERynNDgNr4y0oiFmtes3rbEamXw7GHGbkbNd9iRr7kgT03T6fT0b9WpJ3mbxKhyOcAHnGcIoh5u/cjrC2OQ==}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ react-is: 18.3.0
+ dev: false
+
+ /rc-virtual-list@3.11.5(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-iZRW99m5jAxtwKNPLwUrPryurcnKpXBdTyhuBp6ythf7kg/otKO5cCiIvL55GQwU0QGSlouQS0tnkciRMJUwRQ==}
+ engines: {node: '>=8.x'}
+ peerDependencies:
+ react: '>=16.9.0'
+ react-dom: '>=16.9.0'
+ dependencies:
+ '@babel/runtime': 7.24.4
+ classnames: 2.5.1
+ rc-resize-observer: 1.4.0(react-dom@18.3.0)(react@18.3.0)
+ rc-util: 5.39.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /react-apexcharts@1.4.1(apexcharts@3.49.0)(react@18.3.0):
+ resolution: {integrity: sha512-G14nVaD64Bnbgy8tYxkjuXEUp/7h30Q0U33xc3AwtGFijJB9nHqOt1a6eG0WBn055RgRg+NwqbKGtqPxy15d0Q==}
+ peerDependencies:
+ apexcharts: ^3.41.0
+ react: '>=0.13'
+ dependencies:
+ apexcharts: 3.49.0
+ prop-types: 15.8.1
+ react: 18.3.0
+ dev: false
+
+ /react-beautiful-dnd@13.1.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-0Lvs4tq2VcrEjEgDXHjT98r+63drkKEgqyxdA7qD3mvKwga6a5SscbdLPO2IExotU1jW8L0Ksdl0Cj2AF67nPQ==}
+ peerDependencies:
+ react: ^16.8.5 || ^17.0.0 || ^18.0.0
+ react-dom: ^16.8.5 || ^17.0.0 || ^18.0.0
+ dependencies:
+ '@babel/runtime': 7.24.4
+ css-box-model: 1.2.1
+ memoize-one: 5.2.1
+ raf-schd: 4.0.3
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ react-redux: 7.2.9(react-dom@18.3.0)(react@18.3.0)
+ redux: 4.2.1
+ use-memo-one: 1.1.3(react@18.3.0)
+ transitivePeerDependencies:
+ - react-native
+ dev: false
+
+ /react-dev-inspector@2.0.1(eslint@8.57.0)(react@18.3.0)(typescript@5.4.5)(webpack@5.91.0):
+ resolution: {integrity: sha512-b8PAmbwGFrWcxeaX8wYveqO+VTwTXGJaz/yl9RO31LK1zeLKJVlkkbeLExLnJ6IvhXY1TwL8Q4+gR2GKJ8BI6Q==}
+ engines: {node: '>=12.0.0'}
+ peerDependencies:
+ react: '>=16.8.0'
+ dependencies:
+ '@react-dev-inspector/babel-plugin': 2.0.1
+ '@react-dev-inspector/middleware': 2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0)
+ '@react-dev-inspector/umi3-plugin': 2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0)
+ '@react-dev-inspector/umi4-plugin': 2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0)
+ '@react-dev-inspector/vite-plugin': 2.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0)
+ '@types/react-reconciler': 0.28.8
+ hotkeys-js: 3.13.7
+ picocolors: 1.0.0
+ react: 18.3.0
+ react-dev-utils: 12.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0)
+ transitivePeerDependencies:
+ - eslint
+ - supports-color
+ - typescript
+ - vue-template-compiler
+ - webpack
+ dev: true
+
+ /react-dev-utils@12.0.1(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0):
+ resolution: {integrity: sha512-84Ivxmr17KjUupyqzFode6xKhjwuEJDROWKJy/BthkL7Wn6NJ8h4WE6k/exAv6ImS+0oZLRRW5j/aINMHyeGeQ==}
+ engines: {node: '>=14'}
+ peerDependencies:
+ typescript: '>=2.7'
+ webpack: '>=4'
+ peerDependenciesMeta:
+ typescript:
+ optional: true
+ dependencies:
+ '@babel/code-frame': 7.24.2
+ address: 1.2.2
+ browserslist: 4.23.0
+ chalk: 4.1.2
+ cross-spawn: 7.0.3
+ detect-port-alt: 1.1.6
+ escape-string-regexp: 4.0.0
+ filesize: 8.0.7
+ find-up: 5.0.0
+ fork-ts-checker-webpack-plugin: 6.5.3(eslint@8.57.0)(typescript@5.4.5)(webpack@5.91.0)
+ global-modules: 2.0.0
+ globby: 11.1.0
+ gzip-size: 6.0.0
+ immer: 9.0.21
+ is-root: 2.1.0
+ loader-utils: 3.2.1
+ open: 8.4.2
+ pkg-up: 3.1.0
+ prompts: 2.4.2
+ react-error-overlay: 6.0.11
+ recursive-readdir: 2.2.3
+ shell-quote: 1.8.1
+ strip-ansi: 6.0.1
+ text-table: 0.2.0
+ typescript: 5.4.5
+ webpack: 5.91.0
+ transitivePeerDependencies:
+ - eslint
+ - supports-color
+ - vue-template-compiler
+ dev: true
+
+ /react-dom@18.3.0(react@18.3.0):
+ resolution: {integrity: sha512-zaKdLBftQJnvb7FtDIpZtsAIb2MZU087RM8bRDZU8LVCCFYjPTsDZJNFUWPcVz3HFSN1n/caxi0ca4B/aaVQGQ==}
+ peerDependencies:
+ react: ^18.3.0
+ dependencies:
+ loose-envify: 1.4.0
+ react: 18.3.0
+ scheduler: 0.23.1
+ dev: false
+
+ /react-error-overlay@6.0.11:
+ resolution: {integrity: sha512-/6UZ2qgEyH2aqzYZgQPxEnz33NJ2gNsnHA2o5+o4wW9bLM/JYQitNP9xPhsXwC08hMMovfGe/8retsdDsczPRg==}
+ dev: true
+
+ /react-fast-compare@3.2.2:
+ resolution: {integrity: sha512-nsO+KSNgo1SbJqJEYRE9ERzo7YtYbou/OqjSQKxV7jcKox7+usiUVZOAC+XnDOABXggQTno0Y1CpVnuWEc1boQ==}
+ dev: false
+
+ /react-helmet-async@1.3.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-9jZ57/dAn9t3q6hneQS0wukqC2ENOBgMNVEhb/ZG9ZSxUetzVIw4iAmEU38IaVg3QGYauQPhSeUTuIUtFglWpg==}
+ peerDependencies:
+ react: ^16.6.0 || ^17.0.0 || ^18.0.0
+ react-dom: ^16.6.0 || ^17.0.0 || ^18.0.0
+ dependencies:
+ '@babel/runtime': 7.24.4
+ invariant: 2.2.4
+ prop-types: 15.8.1
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ react-fast-compare: 3.2.2
+ shallowequal: 1.1.0
+ dev: false
+
+ /react-i18next@13.5.0(i18next@23.11.2)(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-CFJ5NDGJ2MUyBohEHxljOq/39NQ972rh1ajnadG9BjTk+UXbHLq4z5DKEbEQBDoIhUmmbuS/fIMJKo6VOax1HA==}
+ peerDependencies:
+ i18next: '>= 23.2.3'
+ react: '>= 16.8.0'
+ react-dom: '*'
+ react-native: '*'
+ peerDependenciesMeta:
+ react-dom:
+ optional: true
+ react-native:
+ optional: true
+ dependencies:
+ '@babel/runtime': 7.24.4
+ html-parse-stringify: 3.0.1
+ i18next: 23.11.2
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /react-icons@4.12.0(react@18.3.0):
+ resolution: {integrity: sha512-IBaDuHiShdZqmfc/TwHu6+d6k2ltNCf3AszxNmjJc1KUfXdEeRJOKyNvLmAHaarhzGmTSVygNdyu8/opXv2gaw==}
+ peerDependencies:
+ react: '*'
+ dependencies:
+ react: 18.3.0
+ dev: false
+
+ /react-is@16.13.1:
+ resolution: {integrity: sha512-24e6ynE2H+OKt4kqsOvNd8kBpV65zoxbA4BVsEOB3ARVWQki/DHzaUoC5KuON/BiccDaCCTZBuOcfZs70kR8bQ==}
+
+ /react-is@17.0.2:
+ resolution: {integrity: sha512-w2GsyukL62IJnlaff/nRegPQR94C/XXamvMWmSHRJ4y7Ts/4ocGRmTHvOs8PSE6pB3dWOrD/nueuU5sduBsQ4w==}
+ dev: false
+
+ /react-is@18.3.0:
+ resolution: {integrity: sha512-wRiUsea88TjKDc4FBEn+sLvIDesp6brMbGWnJGjew2waAc9evdhja/2LvePc898HJbHw0L+MTWy7NhpnELAvLQ==}
+ dev: false
+
+ /react-markdown@8.0.7(@types/react@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-bvWbzG4MtOU62XqBx3Xx+zB2raaFFsq4mYiAzfjXJMEz2sixgeAfraA3tvzULF02ZdOMUOKTBFFaZJDDrq+BJQ==}
+ peerDependencies:
+ '@types/react': '>=16'
+ react: '>=16'
+ dependencies:
+ '@types/hast': 2.3.10
+ '@types/prop-types': 15.7.12
+ '@types/react': 18.3.0
+ '@types/unist': 2.0.10
+ comma-separated-tokens: 2.0.3
+ hast-util-whitespace: 2.0.1
+ prop-types: 15.8.1
+ property-information: 6.5.0
+ react: 18.3.0
+ react-is: 18.3.0
+ remark-parse: 10.0.2
+ remark-rehype: 10.1.0
+ space-separated-tokens: 2.0.2
+ style-to-object: 0.4.4
+ unified: 10.1.2
+ unist-util-visit: 4.1.2
+ vfile: 5.3.7
+ transitivePeerDependencies:
+ - supports-color
+ dev: false
+
+ /react-organizational-chart@2.2.1(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-JORmpLeYzCVtztdqCHsnKL8H3WiLRPHjohgh/PxQoszLuaQ+l3F8YefKSfpcBPZJhHwy3SlqjFjPC28a3Hh3QQ==}
+ engines: {node: '>=16', npm: '>=8'}
+ peerDependencies:
+ react: '>= 16.12.0'
+ react-dom: '>= 16.12.0'
+ dependencies:
+ '@emotion/css': 11.11.2
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /react-quill@2.0.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-4qQtv1FtCfLgoD3PXAur5RyxuUbPXQGOHgTlFie3jtxp43mXDtzCKaOgQ3mLyZfi1PUlyjycfivKelFhy13QUg==}
+ peerDependencies:
+ react: ^16 || ^17 || ^18
+ react-dom: ^16 || ^17 || ^18
+ dependencies:
+ '@types/quill': 1.3.10
+ lodash: 4.17.21
+ quill: 1.3.7
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ dev: false
+
+ /react-redux@7.2.9(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-Gx4L3uM182jEEayZfRbI/G11ZpYdNAnBs70lFVMNdHJI76XYtR+7m0MN+eAs7UHBPhWXcnFPaS+9owSCJQHNpQ==}
+ peerDependencies:
+ react: ^16.8.3 || ^17 || ^18
+ react-dom: '*'
+ react-native: '*'
+ peerDependenciesMeta:
+ react-dom:
+ optional: true
+ react-native:
+ optional: true
+ dependencies:
+ '@babel/runtime': 7.24.4
+ '@types/react-redux': 7.1.33
+ hoist-non-react-statics: 3.3.2
+ loose-envify: 1.4.0
+ prop-types: 15.8.1
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ react-is: 17.0.2
+ dev: false
+
+ /react-refresh@0.14.1:
+ resolution: {integrity: sha512-iZiRCtNGY3QYP3pYOSSBOvQmBpQTcJccr/VcK2blpJrpPTUDjeN51mxm5nsrkCzBwsbGUj+TN9q2oPz5E13FLg==}
+ engines: {node: '>=0.10.0'}
+ dev: false
+
+ /react-router-dom@6.23.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-Q9YaSYvubwgbal2c9DJKfx6hTNoBp3iJDsl+Duva/DwxoJH+OTXkxGpql4iUK2sla/8z4RpjAm6EWx1qUDuopQ==}
+ engines: {node: '>=14.0.0'}
+ peerDependencies:
+ react: '>=16.8'
+ react-dom: '>=16.8'
+ dependencies:
+ '@remix-run/router': 1.16.0
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ react-router: 6.23.0(react@18.3.0)
+ dev: false
+
+ /react-router@6.23.0(react@18.3.0):
+ resolution: {integrity: sha512-wPMZ8S2TuPadH0sF5irFGjkNLIcRvOSaEe7v+JER8508dyJumm6XZB1u5kztlX0RVq6AzRVndzqcUh6sFIauzA==}
+ engines: {node: '>=14.0.0'}
+ peerDependencies:
+ react: '>=16.8'
+ dependencies:
+ '@remix-run/router': 1.16.0
+ react: 18.3.0
+ dev: false
+
+ /react-universal-interface@0.6.2(react@18.3.0)(tslib@2.6.2):
+ resolution: {integrity: sha512-dg8yXdcQmvgR13RIlZbTRQOoUrDciFVoSBZILwjE2LFISxZZ8loVJKAkuzswl5js8BHda79bIb2b84ehU8IjXw==}
+ peerDependencies:
+ react: '*'
+ tslib: '*'
+ dependencies:
+ react: 18.3.0
+ tslib: 2.6.2
+ dev: false
+
+ /react-use@17.5.0(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-PbfwSPMwp/hoL847rLnm/qkjg3sTRCvn6YhUZiHaUa3FA6/aNoFX79ul5Xt70O1rK+9GxSVqkY0eTwMdsR/bWg==}
+ peerDependencies:
+ react: '*'
+ react-dom: '*'
+ dependencies:
+ '@types/js-cookie': 2.2.7
+ '@xobotyi/scrollbar-width': 1.9.5
+ copy-to-clipboard: 3.3.3
+ fast-deep-equal: 3.1.3
+ fast-shallow-equal: 1.0.0
+ js-cookie: 2.2.1
+ nano-css: 5.6.1(react-dom@18.3.0)(react@18.3.0)
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ react-universal-interface: 0.6.2(react@18.3.0)(tslib@2.6.2)
+ resize-observer-polyfill: 1.5.1
+ screenfull: 5.2.0
+ set-harmonic-interval: 1.0.1
+ throttle-debounce: 3.0.1
+ ts-easing: 0.2.0
+ tslib: 2.6.2
+ dev: false
+
+ /react@18.3.0:
+ resolution: {integrity: sha512-RPutkJftSAldDibyrjuku7q11d3oy6wKOyPe5K1HA/HwwrXcEqBdHsLypkC2FFYjP7bPUa6gbzSBhw4sY2JcDg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ loose-envify: 1.4.0
+
+ /read-cache@1.0.0:
+ resolution: {integrity: sha512-Owdv/Ft7IjOgm/i0xvNDZ1LrRANRfew4b2prF3OWMQLxLfu3bS8FVhCsrSCMK4lR56Y9ya+AThoTpDCTxCmpRA==}
+ dependencies:
+ pify: 2.3.0
+ dev: true
+
+ /read-pkg-up@3.0.0:
+ resolution: {integrity: sha512-YFzFrVvpC6frF1sz8psoHDBGF7fLPc+llq/8NB43oagqWkx8ar5zYtsTORtOjw9W2RHLpWP+zTWwBvf1bCmcSw==}
+ engines: {node: '>=4'}
+ dependencies:
+ find-up: 2.1.0
+ read-pkg: 3.0.0
+ dev: true
+
+ /read-pkg-up@7.0.1:
+ resolution: {integrity: sha512-zK0TB7Xd6JpCLmlLmufqykGE+/TlOePD6qKClNW7hHDKFh/J7/7gCWGR7joEQEW1bKq3a3yUZSObOoWLFQ4ohg==}
+ engines: {node: '>=8'}
+ dependencies:
+ find-up: 4.1.0
+ read-pkg: 5.2.0
+ type-fest: 0.8.1
+ dev: true
+
+ /read-pkg-up@8.0.0:
+ resolution: {integrity: sha512-snVCqPczksT0HS2EC+SxUndvSzn6LRCwpfSvLrIfR5BKDQQZMaI6jPRC9dYvYFDRAuFEAnkwww8kBBNE/3VvzQ==}
+ engines: {node: '>=12'}
+ dependencies:
+ find-up: 5.0.0
+ read-pkg: 6.0.0
+ type-fest: 1.4.0
+ dev: true
+
+ /read-pkg@3.0.0:
+ resolution: {integrity: sha512-BLq/cCO9two+lBgiTYNqD6GdtK8s4NpaWrl6/rCO9w0TUS8oJl7cmToOZfRYllKTISY6nt1U7jQ53brmKqY6BA==}
+ engines: {node: '>=4'}
+ dependencies:
+ load-json-file: 4.0.0
+ normalize-package-data: 2.5.0
+ path-type: 3.0.0
+ dev: true
+
+ /read-pkg@5.2.0:
+ resolution: {integrity: sha512-Ug69mNOpfvKDAc2Q8DRpMjjzdtrnv9HcSMX+4VsZxD1aZ6ZzrIE7rlzXBtWTyhULSMKg076AW6WR5iZpD0JiOg==}
+ engines: {node: '>=8'}
+ dependencies:
+ '@types/normalize-package-data': 2.4.4
+ normalize-package-data: 2.5.0
+ parse-json: 5.2.0
+ type-fest: 0.6.0
+ dev: true
+
+ /read-pkg@6.0.0:
+ resolution: {integrity: sha512-X1Fu3dPuk/8ZLsMhEj5f4wFAF0DWoK7qhGJvgaijocXxBmSToKfbFtqbxMO7bVjNA1dmE5huAzjXj/ey86iw9Q==}
+ engines: {node: '>=12'}
+ dependencies:
+ '@types/normalize-package-data': 2.4.4
+ normalize-package-data: 3.0.3
+ parse-json: 5.2.0
+ type-fest: 1.4.0
+ dev: true
+
+ /readable-stream@3.6.2:
+ resolution: {integrity: sha512-9u/sniCrY3D5WdsERHzHE4G2YCXqoG5FTHUiCC4SIbr6XcLZBY05ya9EKjYek9O5xOAwjGq+1JdGBAS7Q9ScoA==}
+ engines: {node: '>= 6'}
+ dependencies:
+ inherits: 2.0.4
+ string_decoder: 1.3.0
+ util-deprecate: 1.0.2
+ dev: true
+
+ /readdirp@3.6.0:
+ resolution: {integrity: sha512-hOS089on8RduqdbhvQ5Z37A0ESjsqz6qnRcffsMU3495FuTdqSm+7bhJ29JvIOsBDEEnan5DPu9t3To9VRlMzA==}
+ engines: {node: '>=8.10.0'}
+ dependencies:
+ picomatch: 2.3.1
+
+ /recursive-readdir@2.2.3:
+ resolution: {integrity: sha512-8HrF5ZsXk5FAH9dgsx3BlUer73nIhuj+9OrQwEbLTPOBzGkL1lsFCR01am+v+0m2Cmbs1nP12hLDl5FA7EszKA==}
+ engines: {node: '>=6.0.0'}
+ dependencies:
+ minimatch: 3.1.2
+ dev: true
+
+ /redent@2.0.0:
+ resolution: {integrity: sha512-XNwrTx77JQCEMXTeb8movBKuK75MgH0RZkujNuDKCezemx/voapl9i2gCSi8WWm8+ox5ycJi1gxF22fR7c0Ciw==}
+ engines: {node: '>=4'}
+ dependencies:
+ indent-string: 3.2.0
+ strip-indent: 2.0.0
+ dev: true
+
+ /redent@3.0.0:
+ resolution: {integrity: sha512-6tDA8g98We0zd0GvVeMT9arEOnTw9qM03L9cJXaCjrip1OO764RDBLBfrB4cwzNGDj5OA5ioymC9GkizgWJDUg==}
+ engines: {node: '>=8'}
+ dependencies:
+ indent-string: 4.0.0
+ strip-indent: 3.0.0
+ dev: true
+
+ /redent@4.0.0:
+ resolution: {integrity: sha512-tYkDkVVtYkSVhuQ4zBgfvciymHaeuel+zFKXShfDnFP5SyVEP7qo70Rf1jTOTCx3vGNAbnEi/xFkcfQVMIBWag==}
+ engines: {node: '>=12'}
+ dependencies:
+ indent-string: 5.0.0
+ strip-indent: 4.0.0
+ dev: true
+
+ /redux@4.2.1:
+ resolution: {integrity: sha512-LAUYz4lc+Do8/g7aeRa8JkyDErK6ekstQaqWQrNRW//MY1TvCEpMtpTWvlQ+FPbWCx+Xixu/6SHt5N0HR+SB4w==}
+ dependencies:
+ '@babel/runtime': 7.24.4
+ dev: false
+
+ /reflect.getprototypeof@1.0.6:
+ resolution: {integrity: sha512-fmfw4XgoDke3kdI6h4xcUz1dG8uaiv5q9gcEwLS4Pnth2kxT+GZ7YehS1JTMGBQmtV7Y4GFGbs2re2NqhdozUg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-errors: 1.3.0
+ get-intrinsic: 1.2.4
+ globalthis: 1.0.3
+ which-builtin-type: 1.1.3
+ dev: true
+
+ /regenerator-runtime@0.14.1:
+ resolution: {integrity: sha512-dYnhHh0nJoMfnkZs6GmmhFknAGRrLznOu5nc9ML+EJxGvrx6H7teuevqVqCuPcPK//3eDrrjQhehXVx9cnkGdw==}
+
+ /regex-not@1.0.2:
+ resolution: {integrity: sha512-J6SDjUgDxQj5NusnOtdFxDwN/+HWykR8GELwctJ7mdqhcyy1xEc4SRFHUXvxTp661YaVKAjfRLZ9cCqS6tn32A==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ extend-shallow: 3.0.2
+ safe-regex: 1.1.0
+ dev: true
+
+ /regexp.prototype.flags@1.5.2:
+ resolution: {integrity: sha512-NcDiDkTLuPR+++OCKB0nWafEmhg/Da8aUPLPMQbK+bxKKCm1/S5he+AqYa4PlMCVBalb4/yxIRub6qkEx5yJbw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-errors: 1.3.0
+ set-function-name: 2.0.2
+
+ /rehype-highlight@6.0.0:
+ resolution: {integrity: sha512-q7UtlFicLhetp7K48ZgZiJgchYscMma7XjzX7t23bqEJF8m6/s+viXQEe4oHjrATTIZpX7RG8CKD7BlNZoh9gw==}
+ dependencies:
+ '@types/hast': 2.3.10
+ hast-util-to-text: 3.1.2
+ lowlight: 2.9.0
+ unified: 10.1.2
+ unist-util-visit: 4.1.2
+ dev: false
+
+ /rehype-raw@6.1.1:
+ resolution: {integrity: sha512-d6AKtisSRtDRX4aSPsJGTfnzrX2ZkHQLE5kiUuGOeEoLpbEulFF4hj0mLPbsa+7vmguDKOVVEQdHKDSwoaIDsQ==}
+ dependencies:
+ '@types/hast': 2.3.10
+ hast-util-raw: 7.2.3
+ unified: 10.1.2
+ dev: false
+
+ /remark-gfm@3.0.1:
+ resolution: {integrity: sha512-lEFDoi2PICJyNrACFOfDD3JlLkuSbOa5Wd8EPt06HUdptv8Gn0bxYTdbU/XXQ3swAPkEaGxxPN9cbnMHvVu1Ig==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ mdast-util-gfm: 2.0.2
+ micromark-extension-gfm: 2.0.3
+ unified: 10.1.2
+ transitivePeerDependencies:
+ - supports-color
+ dev: false
+
+ /remark-parse@10.0.2:
+ resolution: {integrity: sha512-3ydxgHa/ZQzG8LvC7jTXccARYDcRld3VfcgIIFs7bI6vbRSxJJmzgLEIIoYKyrfhaY+ujuWaf/PJiMZXoiCXgw==}
+ dependencies:
+ '@types/mdast': 3.0.15
+ mdast-util-from-markdown: 1.3.1
+ unified: 10.1.2
+ transitivePeerDependencies:
+ - supports-color
+ dev: false
+
+ /remark-parse@6.0.3:
+ resolution: {integrity: sha512-QbDXWN4HfKTUC0hHa4teU463KclLAnwpn/FBn87j9cKYJWWawbiLgMfP2Q4XwhxxuuuOxHlw+pSN0OKuJwyVvg==}
+ dependencies:
+ collapse-white-space: 1.0.6
+ is-alphabetical: 1.0.4
+ is-decimal: 1.0.4
+ is-whitespace-character: 1.0.4
+ is-word-character: 1.0.4
+ markdown-escapes: 1.0.4
+ parse-entities: 1.2.2
+ repeat-string: 1.6.1
+ state-toggle: 1.0.3
+ trim: 0.0.1
+ trim-trailing-lines: 1.1.4
+ unherit: 1.1.3
+ unist-util-remove-position: 1.1.4
+ vfile-location: 2.0.6
+ xtend: 4.0.2
+ dev: true
+
+ /remark-rehype@10.1.0:
+ resolution: {integrity: sha512-EFmR5zppdBp0WQeDVZ/b66CWJipB2q2VLNFMabzDSGR66Z2fQii83G5gTBbgGEnEEA0QRussvrFHxk1HWGJskw==}
+ dependencies:
+ '@types/hast': 2.3.10
+ '@types/mdast': 3.0.15
+ mdast-util-to-hast: 12.3.0
+ unified: 10.1.2
+ dev: false
+
+ /remark-stringify@6.0.4:
+ resolution: {integrity: sha512-eRWGdEPMVudijE/psbIDNcnJLRVx3xhfuEsTDGgH4GsFF91dVhw5nhmnBppafJ7+NWINW6C7ZwWbi30ImJzqWg==}
+ dependencies:
+ ccount: 1.1.0
+ is-alphanumeric: 1.0.0
+ is-decimal: 1.0.4
+ is-whitespace-character: 1.0.4
+ longest-streak: 2.0.4
+ markdown-escapes: 1.0.4
+ markdown-table: 1.1.3
+ mdast-util-compact: 1.0.4
+ parse-entities: 1.2.2
+ repeat-string: 1.6.1
+ state-toggle: 1.0.3
+ stringify-entities: 1.3.2
+ unherit: 1.1.3
+ xtend: 4.0.2
+ dev: true
+
+ /remark@10.0.1:
+ resolution: {integrity: sha512-E6lMuoLIy2TyiokHprMjcWNJ5UxfGQjaMSMhV+f4idM625UjjK4j798+gPs5mfjzDE6vL0oFKVeZM6gZVSVrzQ==}
+ dependencies:
+ remark-parse: 6.0.3
+ remark-stringify: 6.0.4
+ unified: 7.1.0
+ dev: true
+
+ /remove-accents@0.4.4:
+ resolution: {integrity: sha512-EpFcOa/ISetVHEXqu+VwI96KZBmq+a8LJnGkaeFw45epGlxIZz5dhEEnNZMsQXgORu3qaMoLX4qJCzOik6ytAg==}
+ dev: false
+
+ /remove-accents@0.5.0:
+ resolution: {integrity: sha512-8g3/Otx1eJaVD12e31UbJj1YzdtVvzH85HV7t+9MJYk/u3XmkOUJ5Ys9wQrf9PCPK8+xn4ymzqYCiZl6QWKn+A==}
+ dev: false
+
+ /repeat-element@1.1.4:
+ resolution: {integrity: sha512-LFiNfRcSu7KK3evMyYOuCzv3L10TW7yC1G2/+StMjK8Y6Vqd2MG7r/Qjw4ghtuCOjFvlnms/iMmLqpvW/ES/WQ==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /repeat-string@1.6.1:
+ resolution: {integrity: sha512-PV0dzCYDNfRi1jCDbJzpW7jNNDRuCOG/jI5ctQcGKt/clZD+YcPS3yIlWuTJMmESC8aevCFmWJy5wjAFgNqN6w==}
+ engines: {node: '>=0.10'}
+ dev: true
+
+ /replace-ext@1.0.0:
+ resolution: {integrity: sha512-vuNYXC7gG7IeVNBC1xUllqCcZKRbJoSPOBhnTEcAIiKCsbuef6zO3F0Rve3isPMMoNoQRWjQwbAgAjHUHniyEA==}
+ engines: {node: '>= 0.10'}
+ dev: true
+
+ /require-directory@2.1.1:
+ resolution: {integrity: sha512-fGxEI7+wsG9xrvdjsrlmL22OMTTiHRwAMroiEeMgq8gzoLC/PQr7RsRDSTLUg/bZAZtF+TVIkHc6/4RIKrui+Q==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /require-from-string@2.0.2:
+ resolution: {integrity: sha512-Xf0nWe6RseziFMu+Ap9biiUbmplq6S9/p+7w7YXP/JBHhrUDDUhwa+vANyubuqfZWTveU//DYVGsDG7RKL/vEw==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /reset-css@5.0.2:
+ resolution: {integrity: sha512-YtgUGSq5z5W0NPSjsBW7ys7rtWa8P8AiE7S6Fg3d1TQCPpAodgYyLuZYlU0AOsLtprk/fC9ormHN/0pAavVIDw==}
+ dev: false
+
+ /resize-observer-polyfill@1.5.1:
+ resolution: {integrity: sha512-LwZrotdHOo12nQuZlHEmtuXdqGoOD0OhaxopaNFxWzInpEgaLWoVuAMbTzixuosCx2nEG58ngzW3vxdWoxIgdg==}
+ dev: false
+
+ /resolve-from@3.0.0:
+ resolution: {integrity: sha512-GnlH6vxLymXJNMBo7XP1fJIzBFbdYt49CuTwmB/6N53t+kMPRMFKz783LlQ4tv28XoQfMWinAJX6WCGf2IlaIw==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /resolve-from@4.0.0:
+ resolution: {integrity: sha512-pb/MYmXstAkysRFx8piNI1tGFNQIFA3vkE3Gq4EuA1dF6gHp/+vgZqsCGJapvy8N3Q+4o7FwvquPJcnZ7RYy4g==}
+ engines: {node: '>=4'}
+
+ /resolve-from@5.0.0:
+ resolution: {integrity: sha512-qYg9KP24dD5qka9J47d0aVky0N+b4fTU89LN9iDnjB5waksiC49rvMB0PrUJQGoTmH50XPiqOvAjDfaijGxYZw==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /resolve-global@1.0.0:
+ resolution: {integrity: sha512-zFa12V4OLtT5XUX/Q4VLvTfBf+Ok0SPc1FNGM/z9ctUdiU618qwKpWnd0CHs3+RqROfyEg/DhuHbMWYqcgljEw==}
+ engines: {node: '>=8'}
+ dependencies:
+ global-dirs: 0.1.1
+ dev: true
+
+ /resolve-url@0.2.1:
+ resolution: {integrity: sha512-ZuF55hVUQaaczgOIwqWzkEcEidmlD/xl44x1UZnhOXcYuFN2S6+rcxpG+C1N3So0wvNI3DmJICUFfu2SxhBmvg==}
+ deprecated: https://github.com/lydell/resolve-url#deprecated
+ dev: true
+
+ /resolve@1.22.8:
+ resolution: {integrity: sha512-oKWePCxqpd6FlLvGV1VU0x7bkPmmCNolxzjMf4NczoDnQcIWrAF+cPtZn5i6n+RfD2d9i0tzpKnG6Yk168yIyw==}
+ hasBin: true
+ dependencies:
+ is-core-module: 2.13.1
+ path-parse: 1.0.7
+ supports-preserve-symlinks-flag: 1.0.0
+
+ /resolve@2.0.0-next.5:
+ resolution: {integrity: sha512-U7WjGVG9sH8tvjW5SmGbQuui75FiyjAX72HX15DwBBwF9dNiQZRQAg9nnPhYy+TUnE0+VcrttuvNI8oSxZcocA==}
+ hasBin: true
+ dependencies:
+ is-core-module: 2.13.1
+ path-parse: 1.0.7
+ supports-preserve-symlinks-flag: 1.0.0
+ dev: true
+
+ /restore-cursor@4.0.0:
+ resolution: {integrity: sha512-I9fPXU9geO9bHOt9pHHOhOkYerIMsmVaWB0rA2AI9ERh/+x/i7MV5HKBNrg+ljO5eoPVgCcnFuRjJ9uH6I/3eg==}
+ engines: {node: ^12.20.0 || ^14.13.1 || >=16.0.0}
+ dependencies:
+ onetime: 5.1.2
+ signal-exit: 3.0.7
+ dev: true
+
+ /ret@0.1.15:
+ resolution: {integrity: sha512-TTlYpa+OL+vMMNG24xSlQGEJ3B/RzEfUlLct7b5G/ytav+wPrplCpVMFuwzXbkecJrb6IYo1iFb0S9v37754mg==}
+ engines: {node: '>=0.12'}
+ dev: true
+
+ /reusify@1.0.4:
+ resolution: {integrity: sha512-U9nH88a3fc/ekCF1l0/UP1IosiuIjyTh7hBvXVMHYgVcfGvt897Xguj2UOLDeI5BG2m7/uwyaLVT6fbtCwTyzw==}
+ engines: {iojs: '>=1.0.0', node: '>=0.10.0'}
+ dev: true
+
+ /rfdc@1.3.1:
+ resolution: {integrity: sha512-r5a3l5HzYlIC68TpmYKlxWjmOP6wiPJ1vWv2HeLhNsRZMrCkxeqxiHlQ21oXmQ4F3SiryXBHhAD7JZqvOJjFmg==}
+ dev: true
+
+ /rimraf@2.6.3:
+ resolution: {integrity: sha512-mwqeW5XsA2qAejG46gYdENaxXjx9onRNCfn7L0duuP4hCuTIi/QO7PDK07KJfp1d+izWPrzEJDcSqBa0OZQriA==}
+ hasBin: true
+ dependencies:
+ glob: 7.2.3
+ dev: true
+
+ /rimraf@3.0.2:
+ resolution: {integrity: sha512-JZkJMZkAGFFPP2YqXZXPbMlMBgsxzE8ILs4lMIX/2o0L9UBw9O/Y3o6wFw/i9YLapcUJWwqbi3kdxIPdC62TIA==}
+ hasBin: true
+ dependencies:
+ glob: 7.2.3
+ dev: true
+
+ /rollup-plugin-visualizer@5.12.0:
+ resolution: {integrity: sha512-8/NU9jXcHRs7Nnj07PF2o4gjxmm9lXIrZ8r175bT9dK8qoLlvKTwRMArRCMgpMGlq8CTLugRvEmyMeMXIU2pNQ==}
+ engines: {node: '>=14'}
+ hasBin: true
+ peerDependencies:
+ rollup: 2.x || 3.x || 4.x
+ peerDependenciesMeta:
+ rollup:
+ optional: true
+ dependencies:
+ open: 8.4.2
+ picomatch: 2.3.1
+ source-map: 0.7.4
+ yargs: 17.7.2
+ dev: true
+
+ /rollup@3.29.4:
+ resolution: {integrity: sha512-oWzmBZwvYrU0iJHtDmhsm662rC15FRXmcjCk1xD771dFDx5jJ02ufAQQTn0etB2emNk4J9EZg/yWKpsn9BWGRw==}
+ engines: {node: '>=14.18.0', npm: '>=8.0.0'}
+ hasBin: true
+ optionalDependencies:
+ fsevents: 2.3.3
+
+ /rtl-css-js@1.16.1:
+ resolution: {integrity: sha512-lRQgou1mu19e+Ya0LsTvKrVJ5TYUbqCVPAiImX3UfLTenarvPUl1QFdvu5Z3PYmHT9RCcwIfbjRQBntExyj3Zg==}
+ dependencies:
+ '@babel/runtime': 7.24.4
+ dev: false
+
+ /run-parallel@1.2.0:
+ resolution: {integrity: sha512-5l4VyZR86LZ/lDxZTR6jqL8AFE2S0IFLMP26AbjsLVADxHdhB/c0GUsH+y39UfCi3dzz8OlQuPmnaJOMoDHQBA==}
+ dependencies:
+ queue-microtask: 1.2.3
+ dev: true
+
+ /sade@1.8.1:
+ resolution: {integrity: sha512-xal3CZX1Xlo/k4ApwCFrHVACi9fBqJ7V+mwhBsuf/1IOKbBy098Fex+Wa/5QMubw09pSZ/u8EY8PWgevJsXp1A==}
+ engines: {node: '>=6'}
+ dependencies:
+ mri: 1.2.0
+ dev: false
+
+ /safe-array-concat@1.1.2:
+ resolution: {integrity: sha512-vj6RsCsWBCf19jIeHEfkRMw8DPiBb+DMXklQ/1SGDHOMlHdPUkZXFQ2YdplS23zESTijAcurb1aSgJA3AgMu1Q==}
+ engines: {node: '>=0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ get-intrinsic: 1.2.4
+ has-symbols: 1.0.3
+ isarray: 2.0.5
+ dev: true
+
+ /safe-buffer@5.2.1:
+ resolution: {integrity: sha512-rp3So07KcdmmKbGvgaNxQSJr7bGVSVk5S9Eq1F+ppbRo70+YeaDxkw5Dd8NPN+GD6bjnYm2VuPuCXmpuYvmCXQ==}
+ dev: true
+
+ /safe-regex-test@1.0.3:
+ resolution: {integrity: sha512-CdASjNJPvRa7roO6Ra/gLYBTzYzzPyyBXxIMdGW3USQLyjWEls2RgW5UBTXaQVp+OrpeCK3bLem8smtmheoRuw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ es-errors: 1.3.0
+ is-regex: 1.1.4
+ dev: true
+
+ /safe-regex@1.1.0:
+ resolution: {integrity: sha512-aJXcif4xnaNUzvUuC5gcb46oTS7zvg4jpMTnuqtrEPlR3vFr4pxtdTwaF1Qs3Enjn9HK+ZlwQui+a7z0SywIzg==}
+ dependencies:
+ ret: 0.1.15
+ dev: true
+
+ /sass@1.75.0:
+ resolution: {integrity: sha512-ShMYi3WkrDWxExyxSZPst4/okE9ts46xZmJDSawJQrnte7M1V9fScVB+uNXOVKRBt0PggHOwoZcn8mYX4trnBw==}
+ engines: {node: '>=14.0.0'}
+ hasBin: true
+ dependencies:
+ chokidar: 3.6.0
+ immutable: 4.3.5
+ source-map-js: 1.2.0
+
+ /scheduler@0.23.1:
+ resolution: {integrity: sha512-5GKS5JGfiah1O38Vfa9srZE4s3wdHbwjlCrvIookrg2FO9aIwKLOJXuJQFlEfNcVSOXuaL2hzDeY20uVXcUtrw==}
+ dependencies:
+ loose-envify: 1.4.0
+ dev: false
+
+ /schema-utils@2.7.0:
+ resolution: {integrity: sha512-0ilKFI6QQF5nxDZLFn2dMjvc4hjg/Wkg7rHd3jK6/A4a1Hl9VFdQWvgB1UMGoU94pad1P/8N7fMcEnLnSiju8A==}
+ engines: {node: '>= 8.9.0'}
+ dependencies:
+ '@types/json-schema': 7.0.15
+ ajv: 6.12.6
+ ajv-keywords: 3.5.2(ajv@6.12.6)
+ dev: true
+
+ /schema-utils@3.3.0:
+ resolution: {integrity: sha512-pN/yOAvcC+5rQ5nERGuwrjLlYvLTbCibnZ1I7B1LaiAz9BRBlE9GMgE/eqV30P7aJQUf7Ddimy/RsbYO/GrVGg==}
+ engines: {node: '>= 10.13.0'}
+ dependencies:
+ '@types/json-schema': 7.0.15
+ ajv: 6.12.6
+ ajv-keywords: 3.5.2(ajv@6.12.6)
+ dev: true
+
+ /screenfull@5.2.0:
+ resolution: {integrity: sha512-9BakfsO2aUQN2K9Fdbj87RJIEZ82Q9IGim7FqM5OsebfoFC6ZHXgDq/KvniuLTPdeM8wY2o6Dj3WQ7KeQCj3cA==}
+ engines: {node: '>=0.10.0'}
+ dev: false
+
+ /screenfull@6.0.2:
+ resolution: {integrity: sha512-AQdy8s4WhNvUZ6P8F6PB21tSPIYKniic+Ogx0AacBMjKP1GUHN2E9URxQHtCusiwxudnCKkdy4GrHXPPJSkCCw==}
+ engines: {node: ^14.13.1 || >=16.0.0}
+ dev: false
+
+ /scroll-into-view-if-needed@3.1.0:
+ resolution: {integrity: sha512-49oNpRjWRvnU8NyGVmUaYG4jtTkNonFZI86MmGRDqBphEK2EXT9gdEUoQPZhuBM8yWHxCWbobltqYO5M4XrUvQ==}
+ dependencies:
+ compute-scroll-into-view: 3.1.0
+ dev: false
+
+ /semver@5.7.2:
+ resolution: {integrity: sha512-cBznnQ9KjJqU67B52RMC65CMarK2600WFnbkcaiwWq3xy/5haFJlshgnpjovMVJ+Hff49d8GEn0b87C5pDQ10g==}
+ hasBin: true
+ dev: true
+
+ /semver@6.3.1:
+ resolution: {integrity: sha512-BR7VvDCVHO+q2xBEWskxS6DJE1qRnb7DxzUrogb71CWoSficBxYsiAGd+Kl0mmq/MprG9yArRkyrQxTO6XjMzA==}
+ hasBin: true
+
+ /semver@7.5.4:
+ resolution: {integrity: sha512-1bCSESV6Pv+i21Hvpxp3Dx+pSD8lIPt8uVjRrxAUt/nbswYc+tK6Y2btiULjd4+fnq15PX+nqQDC7Oft7WkwcA==}
+ engines: {node: '>=10'}
+ hasBin: true
+ dependencies:
+ lru-cache: 6.0.0
+ dev: true
+
+ /semver@7.6.0:
+ resolution: {integrity: sha512-EnwXhrlwXMk9gKu5/flx5sv/an57AkRplG3hTK68W7FRDN+k+OWBj65M7719OkA82XLBxrcX0KSHj+X5COhOVg==}
+ engines: {node: '>=10'}
+ hasBin: true
+ dependencies:
+ lru-cache: 6.0.0
+ dev: true
+
+ /serialize-javascript@6.0.2:
+ resolution: {integrity: sha512-Saa1xPByTTq2gdeFZYLLo+RFE35NHZkAbqZeWNd3BpzppeVisAqpDjcp8dyf6uIvEqJRd46jemmyA4iFIeVk8g==}
+ dependencies:
+ randombytes: 2.1.0
+ dev: true
+
+ /set-function-length@1.2.2:
+ resolution: {integrity: sha512-pgRc4hJ4/sNjWCSS9AmnS40x3bNMDTknHgL5UaMBTMyJnU90EgWh1Rz+MC9eFu4BuN/UwZjKQuY/1v3rM7HMfg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ define-data-property: 1.1.4
+ es-errors: 1.3.0
+ function-bind: 1.1.2
+ get-intrinsic: 1.2.4
+ gopd: 1.0.1
+ has-property-descriptors: 1.0.2
+
+ /set-function-name@2.0.2:
+ resolution: {integrity: sha512-7PGFlmtwsEADb0WYyvCMa1t+yke6daIG4Wirafur5kcf+MhUnPms1UeR0CKQdTZD81yESwMHbtn+TR+dMviakQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ define-data-property: 1.1.4
+ es-errors: 1.3.0
+ functions-have-names: 1.2.3
+ has-property-descriptors: 1.0.2
+
+ /set-harmonic-interval@1.0.1:
+ resolution: {integrity: sha512-AhICkFV84tBP1aWqPwLZqFvAwqEoVA9kxNMniGEUvzOlm4vLmOFLiTT3UZ6bziJTy4bOVpzWGTfSCbmaayGx8g==}
+ engines: {node: '>=6.9'}
+ dev: false
+
+ /set-value@2.0.1:
+ resolution: {integrity: sha512-JxHc1weCN68wRY0fhCoXpyK55m/XPHafOmK4UWD7m2CI14GMcFypt4w/0+NV5f/ZMby2F6S2wwA7fgynh9gWSw==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ extend-shallow: 2.0.1
+ is-extendable: 0.1.1
+ is-plain-object: 2.0.4
+ split-string: 3.1.0
+ dev: true
+
+ /shallowequal@1.1.0:
+ resolution: {integrity: sha512-y0m1JoUZSlPAjXVtPPW70aZWfIL/dSP7AFkRnniLCrK/8MDKog3TySTBmckD+RObVxH0v4Tox67+F14PdED2oQ==}
+ dev: false
+
+ /shebang-command@2.0.0:
+ resolution: {integrity: sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==}
+ engines: {node: '>=8'}
+ dependencies:
+ shebang-regex: 3.0.0
+ dev: true
+
+ /shebang-regex@3.0.0:
+ resolution: {integrity: sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /shell-quote@1.8.1:
+ resolution: {integrity: sha512-6j1W9l1iAs/4xYBI1SYOVZyFcCis9b4KCLQ8fgAGG07QvzaRLVVRQvAy85yNmmZSjYjg4MWh4gNvlPujU/5LpA==}
+ dev: true
+
+ /side-channel@1.0.6:
+ resolution: {integrity: sha512-fDW/EZ6Q9RiO8eFG8Hj+7u/oW+XrPTIChwCOM2+th2A6OblDtYYIpve9m+KvI9Z4C9qSEXlaGR6bTEYHReuglA==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ es-errors: 1.3.0
+ get-intrinsic: 1.2.4
+ object-inspect: 1.13.1
+ dev: true
+
+ /signal-exit@3.0.7:
+ resolution: {integrity: sha512-wnD2ZE+l+SPC/uoS0vXeE9L1+0wuaMqKlfz9AMUo38JsyLSBWSFcHR1Rri62LZc12vLr1gb3jl7iwQhgwpAbGQ==}
+ dev: true
+
+ /signal-exit@4.1.0:
+ resolution: {integrity: sha512-bzyZ1e88w9O1iNJbKnOlvYTrWPDl46O1bG0D3XInv+9tkPrxrN8jUUTiFlDkkmKWgn1M6CfIA13SuGqOa9Korw==}
+ engines: {node: '>=14'}
+ dev: true
+
+ /simple-swizzle@0.2.2:
+ resolution: {integrity: sha512-JA//kQgZtbuY83m+xT+tXJkmJncGMTFT+C+g2h2R9uxkYIrE2yy9sgmcLhCnw57/WSD+Eh3J97FPEDFnbXnDUg==}
+ dependencies:
+ is-arrayish: 0.3.2
+ dev: false
+
+ /simplebar-core@1.2.4:
+ resolution: {integrity: sha512-P+Sqshef4fq3++gQ82TgNYcgl3qZFSCP5jS2/8NMmw18oagXOijMzs1G+vm6RUY3oMvpwH3wGoqh9u6SyDjHfQ==}
+ dependencies:
+ '@types/lodash-es': 4.17.12
+ can-use-dom: 0.1.0
+ lodash: 4.17.21
+ lodash-es: 4.17.21
+ dev: false
+
+ /simplebar-react@3.2.4(react@18.3.0):
+ resolution: {integrity: sha512-ogLN79e7JUm82wJChD7NSUB+4EHCFvDkjXpiu8hT1Alk7DnCekUWds61NXcsP9jC97KOgF5To/AVjYFbX0olgg==}
+ peerDependencies:
+ react: '>=16.8.0'
+ dependencies:
+ react: 18.3.0
+ simplebar-core: 1.2.4
+ dev: false
+
+ /sisteransi@1.0.5:
+ resolution: {integrity: sha512-bLGGlR1QxBcynn2d5YmDX4MGjlZvy2MRBDRNHLJ8VI6l6+9FUiyTFNJ0IveOSP0bcXgVDPRcfGqA0pjaqUpfVg==}
+ dev: true
+
+ /slash@2.0.0:
+ resolution: {integrity: sha512-ZYKh3Wh2z1PpEXWr0MpSBZ0V6mZHAQfYevttO11c51CaWjGTaadiKZ+wVt1PbMlDV5qhMFslpZCemhwOK7C89A==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /slash@3.0.0:
+ resolution: {integrity: sha512-g9Q1haeby36OSStwb4ntCGGGaKsaVSjQ68fBxoQcutl5fS1vuY18H3wSt3jFyFtrkx+Kz0V1G85A4MyAdDMi2Q==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /slice-ansi@2.1.0:
+ resolution: {integrity: sha512-Qu+VC3EwYLldKa1fCxuuvULvSJOKEgk9pi8dZeCVK7TqBfUNTH4sFkk4joj8afVSfAYgJoSOetjx9QWOJ5mYoQ==}
+ engines: {node: '>=6'}
+ dependencies:
+ ansi-styles: 3.2.1
+ astral-regex: 1.0.0
+ is-fullwidth-code-point: 2.0.0
+ dev: true
+
+ /slice-ansi@4.0.0:
+ resolution: {integrity: sha512-qMCMfhY040cVHT43K9BFygqYbUPFZKHOg7K73mtTWJRb8pyP3fzf4Ixd5SzdEJQ6MRUg/WBnOLxghZtKKurENQ==}
+ engines: {node: '>=10'}
+ dependencies:
+ ansi-styles: 4.3.0
+ astral-regex: 2.0.0
+ is-fullwidth-code-point: 3.0.0
+ dev: true
+
+ /slice-ansi@5.0.0:
+ resolution: {integrity: sha512-FC+lgizVPfie0kkhqUScwRu1O/lF6NOgJmlCgK+/LYxDCTk8sGelYaHDhFcDN+Sn3Cv+3VSa4Byeo+IMCzpMgQ==}
+ engines: {node: '>=12'}
+ dependencies:
+ ansi-styles: 6.2.1
+ is-fullwidth-code-point: 4.0.0
+ dev: true
+
+ /snapdragon-node@2.1.1:
+ resolution: {integrity: sha512-O27l4xaMYt/RSQ5TR3vpWCAB5Kb/czIcqUFOM/C4fYcLnbZUc1PkjTAMjof2pBWaSTwOUd6qUHcFGVGj7aIwnw==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ define-property: 1.0.0
+ isobject: 3.0.1
+ snapdragon-util: 3.0.1
+ dev: true
+
+ /snapdragon-util@3.0.1:
+ resolution: {integrity: sha512-mbKkMdQKsjX4BAL4bRYTj21edOf8cN7XHdYUJEe+Zn99hVEYcMvKPct1IqNe7+AZPirn8BCDOQBHQZknqmKlZQ==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ kind-of: 3.2.2
+ dev: true
+
+ /snapdragon@0.8.2:
+ resolution: {integrity: sha512-FtyOnWN/wCHTVXOMwvSv26d+ko5vWlIDD6zoUJ7LW8vh+ZBC8QdljveRP+crNrtBwioEUWy/4dMtbBjA4ioNlg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ base: 0.11.2
+ debug: 2.6.9
+ define-property: 0.2.5
+ extend-shallow: 2.0.1
+ map-cache: 0.2.2
+ source-map: 0.5.7
+ source-map-resolve: 0.5.3
+ use: 3.1.1
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /source-map-js@1.2.0:
+ resolution: {integrity: sha512-itJW8lvSA0TXEphiRoawsCksnlf8SyvmFzIhltqAHluXd88pkCd+cXJVHTDwdCr0IzwptSm035IHQktUu1QUMg==}
+ engines: {node: '>=0.10.0'}
+
+ /source-map-resolve@0.5.3:
+ resolution: {integrity: sha512-Htz+RnsXWk5+P2slx5Jh3Q66vhQj1Cllm0zvnaY98+NFx+Dv2CF/f5O/t8x+KaNdrdIAsruNzoh/KpialbqAnw==}
+ deprecated: See https://github.com/lydell/source-map-resolve#deprecated
+ dependencies:
+ atob: 2.1.2
+ decode-uri-component: 0.2.2
+ resolve-url: 0.2.1
+ source-map-url: 0.4.1
+ urix: 0.1.0
+ dev: true
+
+ /source-map-support@0.5.21:
+ resolution: {integrity: sha512-uBHU3L3czsIyYXKX88fdrGovxdSCoTGDRZ6SYXtSRxLZUzHg5P/66Ht6uoUlHu9EZod+inXhKo3qQgwXUT/y1w==}
+ dependencies:
+ buffer-from: 1.1.2
+ source-map: 0.6.1
+
+ /source-map-url@0.4.1:
+ resolution: {integrity: sha512-cPiFOTLUKvJFIg4SKVScy4ilPPW6rFgMgfuZJPNoDuMs3nC1HbMUycBoJw77xFIp6z1UJQJOfx6C9GMH80DiTw==}
+ deprecated: See https://github.com/lydell/source-map-url#deprecated
+ dev: true
+
+ /source-map@0.5.6:
+ resolution: {integrity: sha512-MjZkVp0NHr5+TPihLcadqnlVoGIoWo4IBHptutGh9wI3ttUYvCG26HkSuDi+K6lsZ25syXJXcctwgyVCt//xqA==}
+ engines: {node: '>=0.10.0'}
+ dev: false
+
+ /source-map@0.5.7:
+ resolution: {integrity: sha512-LbrmJOMUSdEVxIKvdcJzQC+nQhe8FUZQTXQy6+I75skNgn3OoQ0DZA8YnFa7gp8tqtL3KPf1kmo0R5DoApeSGQ==}
+ engines: {node: '>=0.10.0'}
+
+ /source-map@0.6.1:
+ resolution: {integrity: sha512-UjgapumWlbMhkBgzT7Ykc5YXUT46F0iKu8SGXq0bcwP5dz/h0Plj6enJqjz1Zbq2l5WaqYnrVbwWOWMyF3F47g==}
+ engines: {node: '>=0.10.0'}
+
+ /source-map@0.7.4:
+ resolution: {integrity: sha512-l3BikUxvPOcn5E74dZiq5BGsTb5yEwhaTSzccU6t4sDOH8NWJCstKO5QT2CvtFoK6F0saL7p9xHAqHOlCPJygA==}
+ engines: {node: '>= 8'}
+ dev: true
+
+ /space-separated-tokens@2.0.2:
+ resolution: {integrity: sha512-PEGlAwrG8yXGXRjW32fGbg66JAlOAwbObuqVoJpv/mRgoWDQfgH1wDPvtzWyUSNAXBGSk8h755YDbbcEy3SH2Q==}
+ dev: false
+
+ /spdx-correct@3.2.0:
+ resolution: {integrity: sha512-kN9dJbvnySHULIluDHy32WHRUu3Og7B9sbY7tsFLctQkIqnMh3hErYgdMjTYuqmcXX+lK5T1lnUt3G7zNswmZA==}
+ dependencies:
+ spdx-expression-parse: 3.0.1
+ spdx-license-ids: 3.0.17
+ dev: true
+
+ /spdx-exceptions@2.5.0:
+ resolution: {integrity: sha512-PiU42r+xO4UbUS1buo3LPJkjlO7430Xn5SVAhdpzzsPHsjbYVflnnFdATgabnLude+Cqu25p6N+g2lw/PFsa4w==}
+ dev: true
+
+ /spdx-expression-parse@3.0.1:
+ resolution: {integrity: sha512-cbqHunsQWnJNE6KhVSMsMeH5H/L9EpymbzqTQ3uLwNCLZ1Q481oWaofqH7nO6V07xlXwY6PhQdQ2IedWx/ZK4Q==}
+ dependencies:
+ spdx-exceptions: 2.5.0
+ spdx-license-ids: 3.0.17
+ dev: true
+
+ /spdx-license-ids@3.0.17:
+ resolution: {integrity: sha512-sh8PWc/ftMqAAdFiBu6Fy6JUOYjqDJBJvIhpfDMyHrr0Rbp5liZqd4TjtQ/RgfLjKFZb+LMx5hpml5qOWy0qvg==}
+ dev: true
+
+ /specificity@0.4.1:
+ resolution: {integrity: sha512-1klA3Gi5PD1Wv9Q0wUoOQN1IWAuPu0D1U03ThXTr0cJ20+/iq2tHSDnK7Kk/0LXJ1ztUB2/1Os0wKmfyNgUQfg==}
+ hasBin: true
+ dev: true
+
+ /split-string@3.1.0:
+ resolution: {integrity: sha512-NzNVhJDYpwceVVii8/Hu6DKfD2G+NrQHlS/V/qgv763EYudVwEcMQNxd2lh+0VrUByXN/oJkl5grOhYWvQUYiw==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ extend-shallow: 3.0.2
+ dev: true
+
+ /split2@3.2.2:
+ resolution: {integrity: sha512-9NThjpgZnifTkJpzTZ7Eue85S49QwpNhZTq6GRJwObb6jnLFNGB7Qm73V5HewTROPyxD0C29xqmaI68bQtV+hg==}
+ dependencies:
+ readable-stream: 3.6.2
+ dev: true
+
+ /sprintf-js@1.0.3:
+ resolution: {integrity: sha512-D9cPgkvLlV3t3IzL0D0YLvGA9Ahk4PcvVwUbN0dSGr1aP0Nrt4AEnTUbuGvquEC0mA64Gqt1fzirlRs5ibXx8g==}
+ dev: true
+
+ /stable@0.1.8:
+ resolution: {integrity: sha512-ji9qxRnOVfcuLDySj9qzhGSEFVobyt1kIOSkj1qZzYLzq7Tos/oUUWvotUPQLlrsidqsK6tBH89Bc9kL5zHA6w==}
+ deprecated: 'Modern JS already guarantees Array#sort() is a stable sort, so this library is deprecated. See the compatibility table on MDN: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort#browser_compatibility'
+ dev: true
+
+ /stack-generator@2.0.10:
+ resolution: {integrity: sha512-mwnua/hkqM6pF4k8SnmZ2zfETsRUpWXREfA/goT8SLCV4iOFa4bzOX2nDipWAZFPTjLvQB82f5yaodMVhK0yJQ==}
+ dependencies:
+ stackframe: 1.3.4
+ dev: false
+
+ /stackframe@1.3.4:
+ resolution: {integrity: sha512-oeVtt7eWQS+Na6F//S4kJ2K2VbRlS9D43mAlMyVpVWovy9o+jfgH8O9agzANzaiLjclA0oYzUXEM4PurhSUChw==}
+ dev: false
+
+ /stacktrace-gps@3.1.2:
+ resolution: {integrity: sha512-GcUgbO4Jsqqg6RxfyTHFiPxdPqF+3LFmQhm7MgCuYQOYuWyqxo5pwRPz5d/u6/WYJdEnWfK4r+jGbyD8TSggXQ==}
+ dependencies:
+ source-map: 0.5.6
+ stackframe: 1.3.4
+ dev: false
+
+ /stacktrace-js@2.0.2:
+ resolution: {integrity: sha512-Je5vBeY4S1r/RnLydLl0TBTi3F2qdfWmYsGvtfZgEI+SCprPppaIhQf5nGcal4gI4cGpCV/duLcAzT1np6sQqg==}
+ dependencies:
+ error-stack-parser: 2.1.4
+ stack-generator: 2.0.10
+ stacktrace-gps: 3.1.2
+ dev: false
+
+ /state-toggle@1.0.3:
+ resolution: {integrity: sha512-d/5Z4/2iiCnHw6Xzghyhb+GcmF89bxwgXG60wjIiZaxnymbyOmI8Hk4VqHXiVVp6u2ysaskFfXg3ekCj4WNftQ==}
+ dev: true
+
+ /static-extend@0.1.2:
+ resolution: {integrity: sha512-72E9+uLc27Mt718pMHt9VMNiAL4LMsmDbBva8mxWUCkT07fSzEGMYUCk0XWY6lp0j6RBAG4cJ3mWuZv2OE3s0g==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ define-property: 0.2.5
+ object-copy: 0.1.0
+ dev: true
+
+ /strict-uri-encode@1.1.0:
+ resolution: {integrity: sha512-R3f198pcvnB+5IpnBlRkphuE9n46WyVl8I39W/ZUTZLz4nqSP/oLYUrcnJrw462Ds8he4YKMov2efsTIw1BDGQ==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /string-argv@0.3.2:
+ resolution: {integrity: sha512-aqD2Q0144Z+/RqG52NeHEkZauTAUWJO8c6yTftGJKO3Tja5tUgIfmIl6kExvhtxSDP7fXB6DvzkfMpCd/F3G+Q==}
+ engines: {node: '>=0.6.19'}
+ dev: true
+
+ /string-convert@0.2.1:
+ resolution: {integrity: sha512-u/1tdPl4yQnPBjnVrmdLo9gtuLvELKsAoRapekWggdiQNvvvum+jYF329d84NAa660KQw7pB2n36KrIKVoXa3A==}
+ dev: false
+
+ /string-width@3.1.0:
+ resolution: {integrity: sha512-vafcv6KjVZKSgz06oM/H6GDBrAtz8vdhQakGjFIvNrHA6y3HCF1CInLy+QLq8dTJPQ1b+KDUqDFctkdRW44e1w==}
+ engines: {node: '>=6'}
+ dependencies:
+ emoji-regex: 7.0.3
+ is-fullwidth-code-point: 2.0.0
+ strip-ansi: 5.2.0
+ dev: true
+
+ /string-width@4.2.3:
+ resolution: {integrity: sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==}
+ engines: {node: '>=8'}
+ dependencies:
+ emoji-regex: 8.0.0
+ is-fullwidth-code-point: 3.0.0
+ strip-ansi: 6.0.1
+ dev: true
+
+ /string-width@5.1.2:
+ resolution: {integrity: sha512-HnLOCR3vjcY8beoNLtcjZ5/nxn2afmME6lhrDrebokqMap+XbeW8n9TXpPDOqdGK5qcI3oT0GKTW6wC7EMiVqA==}
+ engines: {node: '>=12'}
+ dependencies:
+ eastasianwidth: 0.2.0
+ emoji-regex: 9.2.2
+ strip-ansi: 7.1.0
+ dev: true
+
+ /string.prototype.matchall@4.0.11:
+ resolution: {integrity: sha512-NUdh0aDavY2og7IbBPenWqR9exH+E26Sv8e0/eTe1tltDGZL+GtBkDAnnyBtmekfK6/Dq3MkcGtzXFEd1LQrtg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-errors: 1.3.0
+ es-object-atoms: 1.0.0
+ get-intrinsic: 1.2.4
+ gopd: 1.0.1
+ has-symbols: 1.0.3
+ internal-slot: 1.0.7
+ regexp.prototype.flags: 1.5.2
+ set-function-name: 2.0.2
+ side-channel: 1.0.6
+ dev: true
+
+ /string.prototype.trim@1.2.9:
+ resolution: {integrity: sha512-klHuCNxiMZ8MlsOihJhJEBJAiMVqU3Z2nEXWfWnIqjN0gEFS9J9+IxKozWWtQGcgoa1WUZzLjKPTr4ZHNFTFxw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-object-atoms: 1.0.0
+ dev: true
+
+ /string.prototype.trimend@1.0.8:
+ resolution: {integrity: sha512-p73uL5VCHCO2BZZ6krwwQE3kCzM7NKmis8S//xEC6fQonchbum4eP6kR4DLEjQFO3Wnj3Fuo8NM0kOSjVdHjZQ==}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-object-atoms: 1.0.0
+ dev: true
+
+ /string.prototype.trimstart@1.0.8:
+ resolution: {integrity: sha512-UXSH262CSZY1tfu3G3Secr6uGLCFVPMhIqHjlgCUtCCcgihYc/xKs9djMTMUOb2j1mVSeU8EU6NWc/iQKU6Gfg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-object-atoms: 1.0.0
+ dev: true
+
+ /string_decoder@1.3.0:
+ resolution: {integrity: sha512-hkRX8U1WjJFd8LsDJ2yQ/wWWxaopEsABU1XfkM8A+j0+85JAGppt16cr1Whg6KIbb4okU6Mql6BOj+uup/wKeA==}
+ dependencies:
+ safe-buffer: 5.2.1
+ dev: true
+
+ /stringify-entities@1.3.2:
+ resolution: {integrity: sha512-nrBAQClJAPN2p+uGCVJRPIPakKeKWZ9GtBCmormE7pWOSlHat7+x5A8gx85M7HM5Dt0BP3pP5RhVW77WdbJJ3A==}
+ dependencies:
+ character-entities-html4: 1.1.4
+ character-entities-legacy: 1.1.4
+ is-alphanumerical: 1.0.4
+ is-hexadecimal: 1.0.4
+ dev: true
+
+ /strip-ansi@3.0.1:
+ resolution: {integrity: sha512-VhumSSbBqDTP8p2ZLKj40UjBCV4+v8bUSEpUb4KjRgWk9pbqGF4REFj6KEagidb2f/M6AzC0EmFyDNGaw9OCzg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ ansi-regex: 2.1.1
+ dev: true
+
+ /strip-ansi@5.2.0:
+ resolution: {integrity: sha512-DuRs1gKbBqsMKIZlrffwlug8MHkcnpjs5VPmL1PAh+mA30U0DTotfDZ0d2UUsXpPmPmMMJ6W773MaA3J+lbiWA==}
+ engines: {node: '>=6'}
+ dependencies:
+ ansi-regex: 4.1.1
+ dev: true
+
+ /strip-ansi@6.0.1:
+ resolution: {integrity: sha512-Y38VPSHcqkFrCpFnQ9vuSXmquuv5oXOKpGeT6aGrr3o3Gc9AlVa6JBfUSOCnbxGGZF+/0ooI7KrPuUSztUdU5A==}
+ engines: {node: '>=8'}
+ dependencies:
+ ansi-regex: 5.0.1
+ dev: true
+
+ /strip-ansi@7.1.0:
+ resolution: {integrity: sha512-iq6eVVI64nQQTRYq2KtEg2d2uU7LElhTJwsH4YzIHZshxlgZms/wIc4VoDQTlG/IvVIrBKG06CrZnp0qv7hkcQ==}
+ engines: {node: '>=12'}
+ dependencies:
+ ansi-regex: 6.0.1
+ dev: true
+
+ /strip-bom@3.0.0:
+ resolution: {integrity: sha512-vavAMRXOgBVNF6nyEEmL3DBK19iRpDcoIwW+swQ+CbGiu7lju6t+JklA1MHweoWtadgt4ISVUsXLyDq34ddcwA==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /strip-final-newline@2.0.0:
+ resolution: {integrity: sha512-BrpvfNAE3dcvq7ll3xVumzjKjZQ5tI1sEUIKr3Uoks0XUl45St3FlatVqef9prk4jRDzhW6WZg+3bk93y6pLjA==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /strip-final-newline@3.0.0:
+ resolution: {integrity: sha512-dOESqjYr96iWYylGObzd39EuNTa5VJxyvVAEm5Jnh7KGo75V43Hk1odPQkNDyXNmUR6k+gEiDVXnjB8HJ3crXw==}
+ engines: {node: '>=12'}
+ dev: true
+
+ /strip-indent@2.0.0:
+ resolution: {integrity: sha512-RsSNPLpq6YUL7QYy44RnPVTn/lcVZtb48Uof3X5JLbF4zD/Gs7ZFDv2HWol+leoQN2mT86LAzSshGfkTlSOpsA==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /strip-indent@3.0.0:
+ resolution: {integrity: sha512-laJTa3Jb+VQpaC6DseHhF7dXVqHTfJPCRDaEbid/drOhgitgYku/letMUqOXFoWV0zIIUbjpdH2t+tYj4bQMRQ==}
+ engines: {node: '>=8'}
+ dependencies:
+ min-indent: 1.0.1
+ dev: true
+
+ /strip-indent@4.0.0:
+ resolution: {integrity: sha512-mnVSV2l+Zv6BLpSD/8V87CW/y9EmmbYzGCIavsnsI6/nwn26DwffM/yztm30Z/I2DY9wdS3vXVCMnHDgZaVNoA==}
+ engines: {node: '>=12'}
+ dependencies:
+ min-indent: 1.0.1
+ dev: true
+
+ /strip-json-comments@3.1.1:
+ resolution: {integrity: sha512-6fPc+R4ihwqP6N/aIv2f1gMH8lOVtWQHoqC4yK6oSDVVocumAsfCqjkXnqiYMhmMwS/mEHLp7Vehlt3ql6lEig==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /style-search@0.1.0:
+ resolution: {integrity: sha512-Dj1Okke1C3uKKwQcetra4jSuk0DqbzbYtXipzFlFMZtowbF1x7BKJwB9AayVMyFARvU8EDrZdcax4At/452cAg==}
+ dev: true
+
+ /style-to-object@0.4.4:
+ resolution: {integrity: sha512-HYNoHZa2GorYNyqiCaBgsxvcJIn7OHq6inEga+E6Ke3m5JkoqpQbnFssk4jwe+K7AhGa2fcha4wSOf1Kn01dMg==}
+ dependencies:
+ inline-style-parser: 0.1.1
+ dev: false
+
+ /styled-components@6.1.8(react-dom@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-PQ6Dn+QxlWyEGCKDS71NGsXoVLKfE1c3vApkvDYS5KAK+V8fNWGhbSUEo9Gg2iaID2tjLXegEW3bZDUGpofRWw==}
+ engines: {node: '>= 16'}
+ peerDependencies:
+ react: '>= 16.8.0'
+ react-dom: '>= 16.8.0'
+ dependencies:
+ '@emotion/is-prop-valid': 1.2.1
+ '@emotion/unitless': 0.8.0
+ '@types/stylis': 4.2.0
+ css-to-react-native: 3.2.0
+ csstype: 3.1.2
+ postcss: 8.4.31
+ react: 18.3.0
+ react-dom: 18.3.0(react@18.3.0)
+ shallowequal: 1.1.0
+ stylis: 4.3.1
+ tslib: 2.5.0
+ dev: false
+
+ /stylelint-config-rational-order@0.1.2:
+ resolution: {integrity: sha512-Qo7ZQaihCwTqijfZg4sbdQQHtugOX/B1/fYh018EiDZHW+lkqH9uHOnsDwDPGZrYJuB6CoyI7MZh2ecw2dOkew==}
+ dependencies:
+ stylelint: 9.10.1
+ stylelint-order: 2.2.1(stylelint@9.10.1)
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /stylelint-config-recommended@12.0.0(stylelint@15.11.0):
+ resolution: {integrity: sha512-x6x8QNARrGO2sG6iURkzqL+Dp+4bJorPMMRNPScdvaUK8PsynriOcMW7AFDKqkWAS5wbue/u8fUT/4ynzcmqdQ==}
+ peerDependencies:
+ stylelint: ^15.5.0
+ dependencies:
+ stylelint: 15.11.0(typescript@5.4.5)
+ dev: true
+
+ /stylelint-config-standard@33.0.0(stylelint@15.11.0):
+ resolution: {integrity: sha512-eyxnLWoXImUn77+ODIuW9qXBDNM+ALN68L3wT1lN2oNspZ7D9NVGlNHb2QCUn4xDug6VZLsh0tF8NyoYzkgTzg==}
+ peerDependencies:
+ stylelint: ^15.5.0
+ dependencies:
+ stylelint: 15.11.0(typescript@5.4.5)
+ stylelint-config-recommended: 12.0.0(stylelint@15.11.0)
+ dev: true
+
+ /stylelint-declaration-block-no-ignored-properties@2.8.0(stylelint@15.11.0):
+ resolution: {integrity: sha512-Ws8Cav7Y+SPN0JsV407LrnNXWOrqGjxShf+37GBtnU/C58Syve9c0+I/xpLcFOosST3ternykn3Lp77f3ITnFw==}
+ engines: {node: '>=6'}
+ peerDependencies:
+ stylelint: ^7.0.0 || ^8.0.0 || ^9.0.0 || ^10.0.0 || ^11.0.0 || ^12.0.0 || ^13.0.0 || ^14.0.0 || ^15.0.0 || ^16.0.0
+ dependencies:
+ stylelint: 15.11.0(typescript@5.4.5)
+ dev: true
+
+ /stylelint-order@2.2.1(stylelint@9.10.1):
+ resolution: {integrity: sha512-019KBV9j8qp1MfBjJuotse6MgaZqGVtXMc91GU9MsS9Feb+jYUvUU3Z8XiClqPdqJZQ0ryXQJGg3U3PcEjXwfg==}
+ engines: {node: '>=6'}
+ peerDependencies:
+ stylelint: ^9.10.1 || ^10.0.0
+ dependencies:
+ lodash: 4.17.21
+ postcss: 7.0.39
+ postcss-sorting: 4.1.0
+ stylelint: 9.10.1
+ dev: true
+
+ /stylelint-order@6.0.4(stylelint@15.11.0):
+ resolution: {integrity: sha512-0UuKo4+s1hgQ/uAxlYU4h0o0HS4NiQDud0NAUNI0aa8FJdmYHA5ZZTFHiV5FpmE3071e9pZx5j0QpVJW5zOCUA==}
+ peerDependencies:
+ stylelint: ^14.0.0 || ^15.0.0 || ^16.0.1
+ dependencies:
+ postcss: 8.4.38
+ postcss-sorting: 8.0.2(postcss@8.4.38)
+ stylelint: 15.11.0(typescript@5.4.5)
+ dev: true
+
+ /stylelint@15.11.0(typescript@5.4.5):
+ resolution: {integrity: sha512-78O4c6IswZ9TzpcIiQJIN49K3qNoXTM8zEJzhaTE/xRTCZswaovSEVIa/uwbOltZrk16X4jAxjaOhzz/hTm1Kw==}
+ engines: {node: ^14.13.1 || >=16.0.0}
+ hasBin: true
+ dependencies:
+ '@csstools/css-parser-algorithms': 2.6.1(@csstools/css-tokenizer@2.2.4)
+ '@csstools/css-tokenizer': 2.2.4
+ '@csstools/media-query-list-parser': 2.1.9(@csstools/css-parser-algorithms@2.6.1)(@csstools/css-tokenizer@2.2.4)
+ '@csstools/selector-specificity': 3.0.3(postcss-selector-parser@6.0.16)
+ balanced-match: 2.0.0
+ colord: 2.9.3
+ cosmiconfig: 8.3.6(typescript@5.4.5)
+ css-functions-list: 3.2.2
+ css-tree: 2.3.1
+ debug: 4.3.4
+ fast-glob: 3.3.2
+ fastest-levenshtein: 1.0.16
+ file-entry-cache: 7.0.2
+ global-modules: 2.0.0
+ globby: 11.1.0
+ globjoin: 0.1.4
+ html-tags: 3.3.1
+ ignore: 5.3.1
+ import-lazy: 4.0.0
+ imurmurhash: 0.1.4
+ is-plain-object: 5.0.0
+ known-css-properties: 0.29.0
+ mathml-tag-names: 2.1.3
+ meow: 10.1.5
+ micromatch: 4.0.5
+ normalize-path: 3.0.0
+ picocolors: 1.0.0
+ postcss: 8.4.38
+ postcss-resolve-nested-selector: 0.1.1
+ postcss-safe-parser: 6.0.0(postcss@8.4.38)
+ postcss-selector-parser: 6.0.16
+ postcss-value-parser: 4.2.0
+ resolve-from: 5.0.0
+ string-width: 4.2.3
+ strip-ansi: 6.0.1
+ style-search: 0.1.0
+ supports-hyperlinks: 3.0.0
+ svg-tags: 1.0.0
+ table: 6.8.2
+ write-file-atomic: 5.0.1
+ transitivePeerDependencies:
+ - supports-color
+ - typescript
+ dev: true
+
+ /stylelint@9.10.1:
+ resolution: {integrity: sha512-9UiHxZhOAHEgeQ7oLGwrwoDR8vclBKlSX7r4fH0iuu0SfPwFaLkb1c7Q2j1cqg9P7IDXeAV2TvQML/fRQzGBBQ==}
+ engines: {node: '>=6'}
+ hasBin: true
+ dependencies:
+ autoprefixer: 9.8.8
+ balanced-match: 1.0.2
+ chalk: 2.4.2
+ cosmiconfig: 5.2.1
+ debug: 4.3.4
+ execall: 1.0.0
+ file-entry-cache: 4.0.0
+ get-stdin: 6.0.0
+ global-modules: 2.0.0
+ globby: 9.2.0
+ globjoin: 0.1.4
+ html-tags: 2.0.0
+ ignore: 5.3.1
+ import-lazy: 3.1.0
+ imurmurhash: 0.1.4
+ known-css-properties: 0.11.0
+ leven: 2.1.0
+ lodash: 4.17.21
+ log-symbols: 2.2.0
+ mathml-tag-names: 2.1.3
+ meow: 5.0.0
+ micromatch: 3.1.10
+ normalize-selector: 0.2.0
+ pify: 4.0.1
+ postcss: 7.0.39
+ postcss-html: 0.36.0(postcss-syntax@0.36.2)(postcss@7.0.39)
+ postcss-jsx: 0.36.4(postcss-syntax@0.36.2)(postcss@7.0.39)
+ postcss-less: 3.1.4
+ postcss-markdown: 0.36.0(postcss-syntax@0.36.2)(postcss@7.0.39)
+ postcss-media-query-parser: 0.2.3
+ postcss-reporter: 6.0.1
+ postcss-resolve-nested-selector: 0.1.1
+ postcss-safe-parser: 4.0.2
+ postcss-sass: 0.3.5
+ postcss-scss: 2.1.1
+ postcss-selector-parser: 3.1.2
+ postcss-syntax: 0.36.2(postcss@8.4.38)
+ postcss-value-parser: 3.3.1
+ resolve-from: 4.0.0
+ signal-exit: 3.0.7
+ slash: 2.0.0
+ specificity: 0.4.1
+ string-width: 3.1.0
+ style-search: 0.1.0
+ sugarss: 2.0.0
+ svg-tags: 1.0.0
+ table: 5.4.6
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /stylis@4.2.0:
+ resolution: {integrity: sha512-Orov6g6BB1sDfYgzWfTHDOxamtX1bE/zo104Dh9e6fqJ3PooipYyfJ0pUmrZO2wAvO8YbEyeFrkV91XTsGMSrw==}
+ dev: false
+
+ /stylis@4.3.1:
+ resolution: {integrity: sha512-EQepAV+wMsIaGVGX1RECzgrcqRRU/0sYOHkeLsZ3fzHaHXZy4DaOOX0vOlGQdlsjkh3mFHAIlVimpwAs4dslyQ==}
+ dev: false
+
+ /stylis@4.3.2:
+ resolution: {integrity: sha512-bhtUjWd/z6ltJiQwg0dUfxEJ+W+jdqQd8TbWLWyeIJHlnsqmGLRFFd8e5mA0AZi/zx90smXRlN66YMTcaSFifg==}
+ dev: false
+
+ /sucrase@3.35.0:
+ resolution: {integrity: sha512-8EbVDiu9iN/nESwxeSxDKe0dunta1GOlHufmSSXxMD2z2/tMZpDMpvXQGsc+ajGo8y2uYUmixaSRUc/QPoQ0GA==}
+ engines: {node: '>=16 || 14 >=14.17'}
+ hasBin: true
+ dependencies:
+ '@jridgewell/gen-mapping': 0.3.5
+ commander: 4.1.1
+ glob: 10.3.12
+ lines-and-columns: 1.2.4
+ mz: 2.7.0
+ pirates: 4.0.6
+ ts-interface-checker: 0.1.13
+ dev: true
+
+ /sugarss@2.0.0:
+ resolution: {integrity: sha512-WfxjozUk0UVA4jm+U1d736AUpzSrNsQcIbyOkoE364GrtWmIrFdk5lksEupgWMD4VaT/0kVx1dobpiDumSgmJQ==}
+ dependencies:
+ postcss: 7.0.39
+ dev: true
+
+ /superjson@1.13.3:
+ resolution: {integrity: sha512-mJiVjfd2vokfDxsQPOwJ/PtanO87LhpYY88ubI5dUB1Ab58Txbyje3+jpm+/83R/fevaq/107NNhtYBLuoTrFg==}
+ engines: {node: '>=10'}
+ dependencies:
+ copy-anything: 3.0.5
+ dev: false
+
+ /supports-color@2.0.0:
+ resolution: {integrity: sha512-KKNVtd6pCYgPIKU4cp2733HWYCpplQhddZLBUryaAHou723x+FRzQ5Df824Fj+IyyuiQTRoub4SnIFfIcrp70g==}
+ engines: {node: '>=0.8.0'}
+ dev: true
+
+ /supports-color@3.2.3:
+ resolution: {integrity: sha512-Jds2VIYDrlp5ui7t8abHN2bjAu4LV/q4N2KivFPpGH0lrka0BMq/33AmECUXlKPcHigkNaqfXRENFju+rlcy+A==}
+ engines: {node: '>=0.8.0'}
+ dependencies:
+ has-flag: 1.0.0
+ dev: true
+
+ /supports-color@5.5.0:
+ resolution: {integrity: sha512-QjVjwdXIt408MIiAqCX4oUKsgU2EqAGzs2Ppkm4aQYbjm+ZEWEcW4SfFNTr4uMNZma0ey4f5lgLrkB0aX0QMow==}
+ engines: {node: '>=4'}
+ dependencies:
+ has-flag: 3.0.0
+
+ /supports-color@7.2.0:
+ resolution: {integrity: sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==}
+ engines: {node: '>=8'}
+ dependencies:
+ has-flag: 4.0.0
+ dev: true
+
+ /supports-color@8.1.1:
+ resolution: {integrity: sha512-MpUEN2OodtUzxvKQl72cUF7RQ5EiHsGvSsVG0ia9c5RbWGL2CI4C7EpPS8UTBIplnlzZiNuV56w+FuNxy3ty2Q==}
+ engines: {node: '>=10'}
+ dependencies:
+ has-flag: 4.0.0
+ dev: true
+
+ /supports-hyperlinks@3.0.0:
+ resolution: {integrity: sha512-QBDPHyPQDRTy9ku4URNGY5Lah8PAaXs6tAAwp55sL5WCsSW7GIfdf6W5ixfziW+t7wh3GVvHyHHyQ1ESsoRvaA==}
+ engines: {node: '>=14.18'}
+ dependencies:
+ has-flag: 4.0.0
+ supports-color: 7.2.0
+ dev: true
+
+ /supports-preserve-symlinks-flag@1.0.0:
+ resolution: {integrity: sha512-ot0WnXS9fgdkgIcePe6RHNk1WA8+muPa6cSjeR3V8K27q9BB1rTE3R1p7Hv0z1ZyAc8s6Vvv8DIyWf681MAt0w==}
+ engines: {node: '>= 0.4'}
+
+ /svg-baker@1.7.0:
+ resolution: {integrity: sha512-nibslMbkXOIkqKVrfcncwha45f97fGuAOn1G99YwnwTj8kF9YiM6XexPcUso97NxOm6GsP0SIvYVIosBis1xLg==}
+ dependencies:
+ bluebird: 3.7.2
+ clone: 2.1.2
+ he: 1.2.0
+ image-size: 0.5.5
+ loader-utils: 1.4.2
+ merge-options: 1.0.1
+ micromatch: 3.1.0
+ postcss: 5.2.18
+ postcss-prefix-selector: 1.16.1(postcss@5.2.18)
+ posthtml-rename-id: 1.0.12
+ posthtml-svg-mode: 1.0.3
+ query-string: 4.3.4
+ traverse: 0.6.9
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /svg-tags@1.0.0:
+ resolution: {integrity: sha512-ovssysQTa+luh7A5Weu3Rta6FJlFBBbInjOh722LIt6klpU2/HtdUbszju/G4devcvk8PGt7FCLv5wftu3THUA==}
+ dev: true
+
+ /svg.draggable.js@2.2.2:
+ resolution: {integrity: sha512-JzNHBc2fLQMzYCZ90KZHN2ohXL0BQJGQimK1kGk6AvSeibuKcIdDX9Kr0dT9+UJ5O8nYA0RB839Lhvk4CY4MZw==}
+ engines: {node: '>= 0.8.0'}
+ dependencies:
+ svg.js: 2.7.1
+ dev: false
+
+ /svg.easing.js@2.0.0:
+ resolution: {integrity: sha512-//ctPdJMGy22YoYGV+3HEfHbm6/69LJUTAqI2/5qBvaNHZ9uUFVC82B0Pl299HzgH13rKrBgi4+XyXXyVWWthA==}
+ engines: {node: '>= 0.8.0'}
+ dependencies:
+ svg.js: 2.7.1
+ dev: false
+
+ /svg.filter.js@2.0.2:
+ resolution: {integrity: sha512-xkGBwU+dKBzqg5PtilaTb0EYPqPfJ9Q6saVldX+5vCRy31P6TlRCP3U9NxH3HEufkKkpNgdTLBJnmhDHeTqAkw==}
+ engines: {node: '>= 0.8.0'}
+ dependencies:
+ svg.js: 2.7.1
+ dev: false
+
+ /svg.js@2.7.1:
+ resolution: {integrity: sha512-ycbxpizEQktk3FYvn/8BH+6/EuWXg7ZpQREJvgacqn46gIddG24tNNe4Son6omdXCnSOaApnpZw6MPCBA1dODA==}
+ dev: false
+
+ /svg.pathmorphing.js@0.1.3:
+ resolution: {integrity: sha512-49HWI9X4XQR/JG1qXkSDV8xViuTLIWm/B/7YuQELV5KMOPtXjiwH4XPJvr/ghEDibmLQ9Oc22dpWpG0vUDDNww==}
+ engines: {node: '>= 0.8.0'}
+ dependencies:
+ svg.js: 2.7.1
+ dev: false
+
+ /svg.resize.js@1.4.3:
+ resolution: {integrity: sha512-9k5sXJuPKp+mVzXNvxz7U0uC9oVMQrrf7cFsETznzUDDm0x8+77dtZkWdMfRlmbkEEYvUn9btKuZ3n41oNA+uw==}
+ engines: {node: '>= 0.8.0'}
+ dependencies:
+ svg.js: 2.7.1
+ svg.select.js: 2.1.2
+ dev: false
+
+ /svg.select.js@2.1.2:
+ resolution: {integrity: sha512-tH6ABEyJsAOVAhwcCjF8mw4crjXSI1aa7j2VQR8ZuJ37H2MBUbyeqYr5nEO7sSN3cy9AR9DUwNg0t/962HlDbQ==}
+ engines: {node: '>= 0.8.0'}
+ dependencies:
+ svg.js: 2.7.1
+ dev: false
+
+ /svg.select.js@3.0.1:
+ resolution: {integrity: sha512-h5IS/hKkuVCbKSieR9uQCj9w+zLHoPh+ce19bBYyqF53g6mnPB8sAtIbe1s9dh2S2fCmYX2xel1Ln3PJBbK4kw==}
+ engines: {node: '>= 0.8.0'}
+ dependencies:
+ svg.js: 2.7.1
+ dev: false
+
+ /svgo@2.8.0:
+ resolution: {integrity: sha512-+N/Q9kV1+F+UeWYoSiULYo4xYSDQlTgb+ayMobAXPwMnLvop7oxKMo9OzIrX5x3eS4L4f2UHhc9axXwY8DpChg==}
+ engines: {node: '>=10.13.0'}
+ hasBin: true
+ dependencies:
+ '@trysound/sax': 0.2.0
+ commander: 7.2.0
+ css-select: 4.3.0
+ css-tree: 1.1.3
+ csso: 4.2.0
+ picocolors: 1.0.0
+ stable: 0.1.8
+ dev: true
+
+ /table@5.4.6:
+ resolution: {integrity: sha512-wmEc8m4fjnob4gt5riFRtTu/6+4rSe12TpAELNSqHMfF3IqnA+CH37USM6/YR3qRZv7e56kAEAtd6nKZaxe0Ug==}
+ engines: {node: '>=6.0.0'}
+ dependencies:
+ ajv: 6.12.6
+ lodash: 4.17.21
+ slice-ansi: 2.1.0
+ string-width: 3.1.0
+ dev: true
+
+ /table@6.8.2:
+ resolution: {integrity: sha512-w2sfv80nrAh2VCbqR5AK27wswXhqcck2AhfnNW76beQXskGZ1V12GwS//yYVa3d3fcvAip2OUnbDAjW2k3v9fA==}
+ engines: {node: '>=10.0.0'}
+ dependencies:
+ ajv: 8.12.0
+ lodash.truncate: 4.4.2
+ slice-ansi: 4.0.0
+ string-width: 4.2.3
+ strip-ansi: 6.0.1
+ dev: true
+
+ /tailwindcss@3.4.3(ts-node@10.9.2):
+ resolution: {integrity: sha512-U7sxQk/n397Bmx4JHbJx/iSOOv5G+II3f1kpLpY2QeUv5DcPdcTsYLlusZfq1NthHS1c1cZoyFmmkex1rzke0A==}
+ engines: {node: '>=14.0.0'}
+ hasBin: true
+ dependencies:
+ '@alloc/quick-lru': 5.2.0
+ arg: 5.0.2
+ chokidar: 3.6.0
+ didyoumean: 1.2.2
+ dlv: 1.1.3
+ fast-glob: 3.3.2
+ glob-parent: 6.0.2
+ is-glob: 4.0.3
+ jiti: 1.21.0
+ lilconfig: 2.1.0
+ micromatch: 4.0.5
+ normalize-path: 3.0.0
+ object-hash: 3.0.0
+ picocolors: 1.0.0
+ postcss: 8.4.38
+ postcss-import: 15.1.0(postcss@8.4.38)
+ postcss-js: 4.0.1(postcss@8.4.38)
+ postcss-load-config: 4.0.2(postcss@8.4.38)(ts-node@10.9.2)
+ postcss-nested: 6.0.1(postcss@8.4.38)
+ postcss-selector-parser: 6.0.16
+ resolve: 1.22.8
+ sucrase: 3.35.0
+ transitivePeerDependencies:
+ - ts-node
+ dev: true
+
+ /tapable@1.1.3:
+ resolution: {integrity: sha512-4WK/bYZmj8xLr+HUCODHGF1ZFzsYffasLUgEiMBY4fgtltdO6B4WJtlSbPaDTLpYTcGVwM2qLnFTICEcNxs3kA==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /tapable@2.2.1:
+ resolution: {integrity: sha512-GNzQvQTOIP6RyTfE2Qxb8ZVlNmw0n88vp1szwWRimP02mnTsx3Wtn5qRdqY9w2XduFNUgvOwhNnQsjwCp+kqaQ==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /terser-webpack-plugin@5.3.10(webpack@5.91.0):
+ resolution: {integrity: sha512-BKFPWlPDndPs+NGGCr1U59t0XScL5317Y0UReNrHaw9/FwhPENlq6bfgs+4yPfyP51vqC1bQ4rp1EfXW5ZSH9w==}
+ engines: {node: '>= 10.13.0'}
+ peerDependencies:
+ '@swc/core': '*'
+ esbuild: '*'
+ uglify-js: '*'
+ webpack: ^5.1.0
+ peerDependenciesMeta:
+ '@swc/core':
+ optional: true
+ esbuild:
+ optional: true
+ uglify-js:
+ optional: true
+ dependencies:
+ '@jridgewell/trace-mapping': 0.3.25
+ jest-worker: 27.5.1
+ schema-utils: 3.3.0
+ serialize-javascript: 6.0.2
+ terser: 5.30.4
+ webpack: 5.91.0
+ dev: true
+
+ /terser@5.30.4:
+ resolution: {integrity: sha512-xRdd0v64a8mFK9bnsKVdoNP9GQIKUAaJPTaqEQDL4w/J8WaW4sWXXoMZ+6SimPkfT5bElreXf8m9HnmPc3E1BQ==}
+ engines: {node: '>=10'}
+ hasBin: true
+ dependencies:
+ '@jridgewell/source-map': 0.3.6
+ acorn: 8.11.3
+ commander: 2.20.3
+ source-map-support: 0.5.21
+
+ /text-extensions@1.9.0:
+ resolution: {integrity: sha512-wiBrwC1EhBelW12Zy26JeOUkQ5mRu+5o8rpsJk5+2t+Y5vE7e842qtZDQ2g1NpX/29HdyFeJ4nSIhI47ENSxlQ==}
+ engines: {node: '>=0.10'}
+ dev: true
+
+ /text-table@0.2.0:
+ resolution: {integrity: sha512-N+8UisAXDGk8PFXP4HAzVR9nbfmVJ3zYLAWiTIoqC5v5isinhr+r5uaO8+7r3BMfuNIufIsA7RdpVgacC2cSpw==}
+ dev: true
+
+ /thenify-all@1.6.0:
+ resolution: {integrity: sha512-RNxQH/qI8/t3thXJDwcstUO4zeqo64+Uy/+sNVRBx4Xn2OX+OZ9oP+iJnNFqplFra2ZUVeKCSa2oVWi3T4uVmA==}
+ engines: {node: '>=0.8'}
+ dependencies:
+ thenify: 3.3.1
+ dev: true
+
+ /thenify@3.3.1:
+ resolution: {integrity: sha512-RVZSIV5IG10Hk3enotrhvz0T9em6cyHBLkH/YAZuKqd8hRkKhSfCGIcP2KUY0EPxndzANBmNllzWPwak+bheSw==}
+ dependencies:
+ any-promise: 1.3.0
+ dev: true
+
+ /throttle-debounce@3.0.1:
+ resolution: {integrity: sha512-dTEWWNu6JmeVXY0ZYoPuH5cRIwc0MeGbJwah9KUNYSJwommQpCzTySTpEe8Gs1J23aeWEuAobe4Ag7EHVt/LOg==}
+ engines: {node: '>=10'}
+ dev: false
+
+ /throttle-debounce@5.0.0:
+ resolution: {integrity: sha512-2iQTSgkkc1Zyk0MeVrt/3BvuOXYPl/R8Z0U2xxo9rjwNciaHDG3R+Lm6dh4EeUci49DanvBnuqI6jshoQQRGEg==}
+ engines: {node: '>=12.22'}
+ dev: false
+
+ /through2@4.0.2:
+ resolution: {integrity: sha512-iOqSav00cVxEEICeD7TjLB1sueEL+81Wpzp2bY17uZjZN0pWZPuo4suZ/61VujxmqSGFfgOcNuTZ85QJwNZQpw==}
+ dependencies:
+ readable-stream: 3.6.2
+ dev: true
+
+ /through@2.3.8:
+ resolution: {integrity: sha512-w89qg7PI8wAdvX60bMDP+bFoD5Dvhm9oLheFp5O4a2QF0cSBGsBX4qZmadPMvVqlLJBBci+WqGGOAPvcDeNSVg==}
+ dev: true
+
+ /tiny-invariant@1.3.3:
+ resolution: {integrity: sha512-+FbBPE1o9QAYvviau/qC5SE3caw21q3xkvWKBtja5vgqOWIHHJ3ioaq1VPfn/Szqctz2bU/oYeKd9/z5BL+PVg==}
+ dev: false
+
+ /to-fast-properties@2.0.0:
+ resolution: {integrity: sha512-/OaKK0xYrs3DmxRYqL/yDc+FxFUVYhDlXMhRmv3z915w2HF1tnN1omB354j8VUGO/hbRzyD6Y3sA7v7GS/ceog==}
+ engines: {node: '>=4'}
+
+ /to-object-path@0.3.0:
+ resolution: {integrity: sha512-9mWHdnGRuh3onocaHzukyvCZhzvr6tiflAy/JRFXcJX0TjgfWA9pk9t8CMbzmBE4Jfw58pXbkngtBtqYxzNEyg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ kind-of: 3.2.2
+ dev: true
+
+ /to-regex-range@2.1.1:
+ resolution: {integrity: sha512-ZZWNfCjUokXXDGXFpZehJIkZqq91BcULFq/Pi7M5i4JnxXdhMKAK682z8bCW3o8Hj1wuuzoKcW3DfVzaP6VuNg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ is-number: 3.0.0
+ repeat-string: 1.6.1
+ dev: true
+
+ /to-regex-range@5.0.1:
+ resolution: {integrity: sha512-65P7iz6X5yEr1cwcgvQxbbIw7Uk3gOy5dIdtZ4rDveLqhrdJP+Li/Hx6tyK0NEb+2GCyneCMJiGqrADCSNk8sQ==}
+ engines: {node: '>=8.0'}
+ dependencies:
+ is-number: 7.0.0
+
+ /to-regex@3.0.2:
+ resolution: {integrity: sha512-FWtleNAtZ/Ki2qtqej2CXTOayOH9bHDQF+Q48VpWyDXjbYxA4Yz8iDB31zXOBUlOHHKidDbqGVrTUvQMPmBGBw==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ define-property: 2.0.2
+ extend-shallow: 3.0.2
+ regex-not: 1.0.2
+ safe-regex: 1.1.0
+ dev: true
+
+ /toggle-selection@1.0.6:
+ resolution: {integrity: sha512-BiZS+C1OS8g/q2RRbJmy59xpyghNBqrr6k5L/uKBGRsTfxmu3ffiRnd8mlGPUVayg8pvfi5urfnu8TU7DVOkLQ==}
+ dev: false
+
+ /traverse@0.6.9:
+ resolution: {integrity: sha512-7bBrcF+/LQzSgFmT0X5YclVqQxtv7TDJ1f8Wj7ibBu/U6BMLeOpUxuZjV7rMc44UtKxlnMFigdhFAIszSX1DMg==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ gopd: 1.0.1
+ typedarray.prototype.slice: 1.0.3
+ which-typed-array: 1.1.15
+ dev: true
+
+ /trim-lines@3.0.1:
+ resolution: {integrity: sha512-kRj8B+YHZCc9kQYdWfJB2/oUl9rA99qbowYYBtr4ui4mZyAQ2JpvVBd/6U2YloATfqBhBTSMhTpgBHtU0Mf3Rg==}
+ dev: false
+
+ /trim-newlines@2.0.0:
+ resolution: {integrity: sha512-MTBWv3jhVjTU7XR3IQHllbiJs8sc75a80OEhB6or/q7pLTWgQ0bMGQXXYQSrSuXe6WiKWDZ5txXY5P59a/coVA==}
+ engines: {node: '>=4'}
+ dev: true
+
+ /trim-newlines@3.0.1:
+ resolution: {integrity: sha512-c1PTsA3tYrIsLGkJkzHF+w9F2EyxfXGo4UyJc4pFL++FMjnq0HJS69T3M7d//gKrFKwy429bouPescbjecU+Zw==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /trim-newlines@4.1.1:
+ resolution: {integrity: sha512-jRKj0n0jXWo6kh62nA5TEh3+4igKDXLvzBJcPpiizP7oOolUrYIxmVBG9TOtHYFHoddUk6YvAkGeGoSVTXfQXQ==}
+ engines: {node: '>=12'}
+ dev: true
+
+ /trim-trailing-lines@1.1.4:
+ resolution: {integrity: sha512-rjUWSqnfTNrjbB9NQWfPMH/xRK1deHeGsHoVfpxJ++XeYXE0d6B1En37AHfw3jtfTU7dzMzZL2jjpe8Qb5gLIQ==}
+ dev: true
+
+ /trim@0.0.1:
+ resolution: {integrity: sha512-YzQV+TZg4AxpKxaTHK3c3D+kRDCGVEE7LemdlQZoQXn0iennk10RsIoY6ikzAqJTc9Xjl9C1/waHom/J86ziAQ==}
+ deprecated: Use String.prototype.trim() instead
+ dev: true
+
+ /trough@1.0.5:
+ resolution: {integrity: sha512-rvuRbTarPXmMb79SmzEp8aqXNKcK+y0XaB298IXueQ8I2PsrATcPBCSPyK/dDNa2iWOhKlfNnOjdAOTBU/nkFA==}
+ dev: true
+
+ /trough@2.2.0:
+ resolution: {integrity: sha512-tmMpK00BjZiUyVyvrBK7knerNgmgvcV/KLVyuma/SC+TQN167GrMRciANTz09+k3zW8L8t60jWO1GpfkZdjTaw==}
+ dev: false
+
+ /ts-easing@0.2.0:
+ resolution: {integrity: sha512-Z86EW+fFFh/IFB1fqQ3/+7Zpf9t2ebOAxNI/V6Wo7r5gqiqtxmgTlQ1qbqQcjLKYeSHPTsEmvlJUDg/EuL0uHQ==}
+ dev: false
+
+ /ts-interface-checker@0.1.13:
+ resolution: {integrity: sha512-Y/arvbn+rrz3JCKl9C4kVNfTfSm2/mEp5FSz5EsZSANGPSlQrpRI5M4PKF+mJnE52jOO90PnPSc3Ur3bTQw0gA==}
+ dev: true
+
+ /ts-node@10.9.2(@types/node@20.12.7)(typescript@5.4.5):
+ resolution: {integrity: sha512-f0FFpIdcHgn8zcPSbf1dRevwt047YMnaiJM3u2w2RewrB+fob/zePZcrOyQoLMMO7aBIddLcQIEK5dYjkLnGrQ==}
+ hasBin: true
+ peerDependencies:
+ '@swc/core': '>=1.2.50'
+ '@swc/wasm': '>=1.2.50'
+ '@types/node': '*'
+ typescript: '>=2.7'
+ peerDependenciesMeta:
+ '@swc/core':
+ optional: true
+ '@swc/wasm':
+ optional: true
+ dependencies:
+ '@cspotcode/source-map-support': 0.8.1
+ '@tsconfig/node10': 1.0.11
+ '@tsconfig/node12': 1.0.11
+ '@tsconfig/node14': 1.0.3
+ '@tsconfig/node16': 1.0.4
+ '@types/node': 20.12.7
+ acorn: 8.11.3
+ acorn-walk: 8.3.2
+ arg: 4.1.3
+ create-require: 1.1.1
+ diff: 4.0.2
+ make-error: 1.3.6
+ typescript: 5.4.5
+ v8-compile-cache-lib: 3.0.1
+ yn: 3.1.1
+ dev: true
+
+ /ts-node@10.9.2(@types/node@20.5.1)(typescript@5.4.5):
+ resolution: {integrity: sha512-f0FFpIdcHgn8zcPSbf1dRevwt047YMnaiJM3u2w2RewrB+fob/zePZcrOyQoLMMO7aBIddLcQIEK5dYjkLnGrQ==}
+ hasBin: true
+ peerDependencies:
+ '@swc/core': '>=1.2.50'
+ '@swc/wasm': '>=1.2.50'
+ '@types/node': '*'
+ typescript: '>=2.7'
+ peerDependenciesMeta:
+ '@swc/core':
+ optional: true
+ '@swc/wasm':
+ optional: true
+ dependencies:
+ '@cspotcode/source-map-support': 0.8.1
+ '@tsconfig/node10': 1.0.11
+ '@tsconfig/node12': 1.0.11
+ '@tsconfig/node14': 1.0.3
+ '@tsconfig/node16': 1.0.4
+ '@types/node': 20.5.1
+ acorn: 8.11.3
+ acorn-walk: 8.3.2
+ arg: 4.1.3
+ create-require: 1.1.1
+ diff: 4.0.2
+ make-error: 1.3.6
+ typescript: 5.4.5
+ v8-compile-cache-lib: 3.0.1
+ yn: 3.1.1
+ dev: true
+
+ /ts-toolbelt@9.6.0:
+ resolution: {integrity: sha512-nsZd8ZeNUzukXPlJmTBwUAuABDe/9qtVDelJeT/qW0ow3ZS3BsQJtNkan1802aM9Uf68/Y8ljw86Hu0h5IUW3w==}
+ dev: true
+
+ /tsconfck@3.0.3(typescript@5.4.5):
+ resolution: {integrity: sha512-4t0noZX9t6GcPTfBAbIbbIU4pfpCwh0ueq3S4O/5qXI1VwK1outmxhe9dOiEWqMz3MW2LKgDTpqWV+37IWuVbA==}
+ engines: {node: ^18 || >=20}
+ hasBin: true
+ peerDependencies:
+ typescript: ^5.0.0
+ peerDependenciesMeta:
+ typescript:
+ optional: true
+ dependencies:
+ typescript: 5.4.5
+ dev: true
+
+ /tsconfig-paths@3.15.0:
+ resolution: {integrity: sha512-2Ac2RgzDe/cn48GvOe3M+o82pEFewD3UPbyoUHHdKasHwJKjds4fLXWf/Ux5kATBKN20oaFGu+jbElp1pos0mg==}
+ dependencies:
+ '@types/json5': 0.0.29
+ json5: 1.0.2
+ minimist: 1.2.8
+ strip-bom: 3.0.0
+ dev: true
+
+ /tslib@1.14.1:
+ resolution: {integrity: sha512-Xni35NKzjgMrwevysHTCArtLDpPvye8zV/0E4EyYn43P7/7qvQwPh9BGkHewbMulVntbigmcT7rdX3BNo9wRJg==}
+ dev: true
+
+ /tslib@2.5.0:
+ resolution: {integrity: sha512-336iVw3rtn2BUK7ORdIAHTyxHGRIHVReokCR3XjbckJMK7ms8FysBfhLR8IXnAgy7T0PTPNBWKiH514FOW/WSg==}
+ dev: false
+
+ /tslib@2.6.2:
+ resolution: {integrity: sha512-AEYxH93jGFPn/a2iVAwW87VuUIkR1FVUKB77NwMF7nBTDkDrrT/Hpt/IrCJ0QXhW27jTBDcf5ZY7w6RiqTMw2Q==}
+ dev: false
+
+ /tsutils@3.21.0(typescript@5.4.5):
+ resolution: {integrity: sha512-mHKK3iUXL+3UF6xL5k0PEhKRUBKPBCv/+RkEOpjRWxxx27KKRBmmA60A9pgOUvMi8GKhRMPEmjBRPzs2W7O1OA==}
+ engines: {node: '>= 6'}
+ peerDependencies:
+ typescript: '>=2.8.0 || >= 3.2.0-dev || >= 3.3.0-dev || >= 3.4.0-dev || >= 3.5.0-dev || >= 3.6.0-dev || >= 3.6.0-beta || >= 3.7.0-dev || >= 3.7.0-beta'
+ dependencies:
+ tslib: 1.14.1
+ typescript: 5.4.5
+ dev: true
+
+ /type-check@0.4.0:
+ resolution: {integrity: sha512-XleUoc9uwGXqjWwXaUTZAmzMcFZ5858QA2vvx1Ur5xIcixXIP+8LnFDgRplU30us6teqdlskFfu+ae4K79Ooew==}
+ engines: {node: '>= 0.8.0'}
+ dependencies:
+ prelude-ls: 1.2.1
+ dev: true
+
+ /type-fest@0.18.1:
+ resolution: {integrity: sha512-OIAYXk8+ISY+qTOwkHtKqzAuxchoMiD9Udx+FSGQDuiRR+PJKJHc2NJAXlbhkGwTt/4/nKZxELY1w3ReWOL8mw==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /type-fest@0.20.2:
+ resolution: {integrity: sha512-Ne+eE4r0/iWnpAxD852z3A+N0Bt5RN//NjJwRd2VFHEmrywxf5vsZlh4R6lixl6B+wz/8d+maTSAkN1FIkI3LQ==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /type-fest@0.6.0:
+ resolution: {integrity: sha512-q+MB8nYR1KDLrgr4G5yemftpMC7/QLqVndBmEEdqzmNj5dcFOO4Oo8qlwZE3ULT3+Zim1F8Kq4cBnikNhlCMlg==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /type-fest@0.8.1:
+ resolution: {integrity: sha512-4dbzIzqvjtgiM5rw1k5rEHtBANKmdudhGyBEajN01fEyhaAIhsoKNy6y7+IN93IfpFtwY9iqi7kD+xwKhQsNJA==}
+ engines: {node: '>=8'}
+ dev: true
+
+ /type-fest@1.4.0:
+ resolution: {integrity: sha512-yGSza74xk0UG8k+pLh5oeoYirvIiWo5t0/o3zHHAO2tRDiZcxWP7fywNlXhqb6/r6sWvwi+RsyQMWhVLe4BVuA==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /typed-array-buffer@1.0.2:
+ resolution: {integrity: sha512-gEymJYKZtKXzzBzM4jqa9w6Q1Jjm7x2d+sh19AdsD4wqnMPDYyvwpsIc2Q/835kHuo3BEQ7CjelGhfTsoBb2MQ==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ es-errors: 1.3.0
+ is-typed-array: 1.1.13
+ dev: true
+
+ /typed-array-byte-length@1.0.1:
+ resolution: {integrity: sha512-3iMJ9q0ao7WE9tWcaYKIptkNBuOIcZCCT0d4MRvuuH88fEoEH62IuQe0OtraD3ebQEoTRk8XCBoknUNc1Y67pw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ for-each: 0.3.3
+ gopd: 1.0.1
+ has-proto: 1.0.3
+ is-typed-array: 1.1.13
+ dev: true
+
+ /typed-array-byte-offset@1.0.2:
+ resolution: {integrity: sha512-Ous0vodHa56FviZucS2E63zkgtgrACj7omjwd/8lTEMEPFFyjfixMZ1ZXenpgCFBBt4EC1J2XsyVS2gkG0eTFA==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ available-typed-arrays: 1.0.7
+ call-bind: 1.0.7
+ for-each: 0.3.3
+ gopd: 1.0.1
+ has-proto: 1.0.3
+ is-typed-array: 1.1.13
+ dev: true
+
+ /typed-array-length@1.0.6:
+ resolution: {integrity: sha512-/OxDN6OtAk5KBpGb28T+HZc2M+ADtvRxXrKKbUwtsLgdoxgX13hyy7ek6bFRl5+aBs2yZzB0c4CnQfAtVypW/g==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ for-each: 0.3.3
+ gopd: 1.0.1
+ has-proto: 1.0.3
+ is-typed-array: 1.1.13
+ possible-typed-array-names: 1.0.0
+ dev: true
+
+ /typedarray.prototype.slice@1.0.3:
+ resolution: {integrity: sha512-8WbVAQAUlENo1q3c3zZYuy5k9VzBQvp8AX9WOtbvyWlLM1v5JaSRmjubLjzHF4JFtptjH/5c/i95yaElvcjC0A==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ call-bind: 1.0.7
+ define-properties: 1.2.1
+ es-abstract: 1.23.3
+ es-errors: 1.3.0
+ typed-array-buffer: 1.0.2
+ typed-array-byte-offset: 1.0.2
+ dev: true
+
+ /types-ramda@0.29.10:
+ resolution: {integrity: sha512-5PJiW/eiTPyXXBYGZOYGezMl6qj7keBiZheRwfjJZY26QPHsNrjfJnz0mru6oeqqoTHOni893Jfd6zyUXfQRWg==}
+ dependencies:
+ ts-toolbelt: 9.6.0
+ dev: true
+
+ /typescript@5.4.5:
+ resolution: {integrity: sha512-vcI4UpRgg81oIRUFwR0WSIHKt11nJ7SAVlYNIu+QpqeyXP+gpQJy/Z4+F0aGxSE4MqwjyXvW/TzgkLAx2AGHwQ==}
+ engines: {node: '>=14.17'}
+ hasBin: true
+ dev: true
+
+ /unbox-primitive@1.0.2:
+ resolution: {integrity: sha512-61pPlCD9h51VoreyJ0BReideM3MDKMKnh6+V9L08331ipq6Q8OFXZYiqP6n/tbHx4s5I9uRhcye6BrbkizkBDw==}
+ dependencies:
+ call-bind: 1.0.7
+ has-bigints: 1.0.2
+ has-symbols: 1.0.3
+ which-boxed-primitive: 1.0.2
+ dev: true
+
+ /undici-types@5.26.5:
+ resolution: {integrity: sha512-JlCMO+ehdEIKqlFxk6IfVoAUVmgz7cU7zD/h9XZ0qzeosSHmUJVOzSQvvYSYWXkFXC+IfLKSIffhv0sVZup6pA==}
+
+ /unherit@1.1.3:
+ resolution: {integrity: sha512-Ft16BJcnapDKp0+J/rqFC3Rrk6Y/Ng4nzsC028k2jdDII/rdZ7Wd3pPT/6+vIIxRagwRc9K0IUX0Ra4fKvw+WQ==}
+ dependencies:
+ inherits: 2.0.4
+ xtend: 4.0.2
+ dev: true
+
+ /unified@10.1.2:
+ resolution: {integrity: sha512-pUSWAi/RAnVy1Pif2kAoeWNBa3JVrx0MId2LASj8G+7AiHWoKZNTomq6LG326T68U7/e263X6fTdcXIy7XnF7Q==}
+ dependencies:
+ '@types/unist': 2.0.10
+ bail: 2.0.2
+ extend: 3.0.2
+ is-buffer: 2.0.5
+ is-plain-obj: 4.1.0
+ trough: 2.2.0
+ vfile: 5.3.7
+ dev: false
+
+ /unified@7.1.0:
+ resolution: {integrity: sha512-lbk82UOIGuCEsZhPj8rNAkXSDXd6p0QLzIuSsCdxrqnqU56St4eyOB+AlXsVgVeRmetPTYydIuvFfpDIed8mqw==}
+ dependencies:
+ '@types/unist': 2.0.10
+ '@types/vfile': 3.0.2
+ bail: 1.0.5
+ extend: 3.0.2
+ is-plain-obj: 1.1.0
+ trough: 1.0.5
+ vfile: 3.0.1
+ x-is-string: 0.1.0
+ dev: true
+
+ /union-value@1.0.1:
+ resolution: {integrity: sha512-tJfXmxMeWYnczCVs7XAEvIV7ieppALdyepWMkHkwciRpZraG/xwT+s2JN8+pr1+8jCRf80FFzvr+MpQeeoF4Xg==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ arr-union: 3.1.0
+ get-value: 2.0.6
+ is-extendable: 0.1.1
+ set-value: 2.0.1
+ dev: true
+
+ /uniq@1.0.1:
+ resolution: {integrity: sha512-Gw+zz50YNKPDKXs+9d+aKAjVwpjNwqzvNpLigIruT4HA9lMZNdMqs9x07kKHB/L9WRzqp4+DlTU5s4wG2esdoA==}
+ dev: true
+
+ /unist-util-find-after@4.0.1:
+ resolution: {integrity: sha512-QO/PuPMm2ERxC6vFXEPtmAutOopy5PknD+Oq64gGwxKtk4xwo9Z97t9Av1obPmGU0IyTa6EKYUfTrK2QJS3Ozw==}
+ dependencies:
+ '@types/unist': 2.0.10
+ unist-util-is: 5.2.1
+ dev: false
+
+ /unist-util-find-all-after@1.0.5:
+ resolution: {integrity: sha512-lWgIc3rrTMTlK1Y0hEuL+k+ApzFk78h+lsaa2gHf63Gp5Ww+mt11huDniuaoq1H+XMK2lIIjjPkncxXcDp3QDw==}
+ dependencies:
+ unist-util-is: 3.0.0
+ dev: true
+
+ /unist-util-generated@2.0.1:
+ resolution: {integrity: sha512-qF72kLmPxAw0oN2fwpWIqbXAVyEqUzDHMsbtPvOudIlUzXYFIeQIuxXQCRCFh22B7cixvU0MG7m3MW8FTq/S+A==}
+ dev: false
+
+ /unist-util-is@3.0.0:
+ resolution: {integrity: sha512-sVZZX3+kspVNmLWBPAB6r+7D9ZgAFPNWm66f7YNb420RlQSbn+n8rG8dGZSkrER7ZIXGQYNm5pqC3v3HopH24A==}
+ dev: true
+
+ /unist-util-is@5.2.1:
+ resolution: {integrity: sha512-u9njyyfEh43npf1M+yGKDGVPbY/JWEemg5nH05ncKPfi+kBbKBJoTdsogMu33uhytuLlv9y0O7GH7fEdwLdLQw==}
+ dependencies:
+ '@types/unist': 2.0.10
+ dev: false
+
+ /unist-util-position@4.0.4:
+ resolution: {integrity: sha512-kUBE91efOWfIVBo8xzh/uZQ7p9ffYRtUbMRZBNFYwf0RK8koUMx6dGUfwylLOKmaT2cs4wSW96QoYUSXAyEtpg==}
+ dependencies:
+ '@types/unist': 2.0.10
+ dev: false
+
+ /unist-util-remove-position@1.1.4:
+ resolution: {integrity: sha512-tLqd653ArxJIPnKII6LMZwH+mb5q+n/GtXQZo6S6csPRs5zB0u79Yw8ouR3wTw8wxvdJFhpP6Y7jorWdCgLO0A==}
+ dependencies:
+ unist-util-visit: 1.4.1
+ dev: true
+
+ /unist-util-stringify-position@1.1.2:
+ resolution: {integrity: sha512-pNCVrk64LZv1kElr0N1wPiHEUoXNVFERp+mlTg/s9R5Lwg87f9bM/3sQB99w+N9D/qnM9ar3+AKDBwo/gm/iQQ==}
+ dev: true
+
+ /unist-util-stringify-position@3.0.3:
+ resolution: {integrity: sha512-k5GzIBZ/QatR8N5X2y+drfpWG8IDBzdnVj6OInRNWm1oXrzydiaAT2OQiA8DPRRZyAKb9b6I2a6PxYklZD0gKg==}
+ dependencies:
+ '@types/unist': 2.0.10
+ dev: false
+
+ /unist-util-stringify-position@4.0.0:
+ resolution: {integrity: sha512-0ASV06AAoKCDkS2+xw5RXJywruurpbC4JZSm7nr7MOt1ojAzvyyaO+UxZf18j8FCF6kmzCZKcAgN/yu2gm2XgQ==}
+ dependencies:
+ '@types/unist': 3.0.2
+ dev: true
+
+ /unist-util-visit-parents@2.1.2:
+ resolution: {integrity: sha512-DyN5vD4NE3aSeB+PXYNKxzGsfocxp6asDc2XXE3b0ekO2BaRUpBicbbUygfSvYfUz1IkmjFR1YF7dPklraMZ2g==}
+ dependencies:
+ unist-util-is: 3.0.0
+ dev: true
+
+ /unist-util-visit-parents@5.1.3:
+ resolution: {integrity: sha512-x6+y8g7wWMyQhL1iZfhIPhDAs7Xwbn9nRosDXl7qoPTSCy0yNxnKc+hWokFifWQIDGi154rdUqKvbCa4+1kLhg==}
+ dependencies:
+ '@types/unist': 2.0.10
+ unist-util-is: 5.2.1
+ dev: false
+
+ /unist-util-visit@1.4.1:
+ resolution: {integrity: sha512-AvGNk7Bb//EmJZyhtRUnNMEpId/AZ5Ph/KUpTI09WHQuDZHKovQ1oEv3mfmKpWKtoMzyMC4GLBm1Zy5k12fjIw==}
+ dependencies:
+ unist-util-visit-parents: 2.1.2
+ dev: true
+
+ /unist-util-visit@4.1.2:
+ resolution: {integrity: sha512-MSd8OUGISqHdVvfY9TPhyK2VdUrPgxkUtWSuMHF6XAAFuL4LokseigBnZtPnJMu+FbynTkFNnFlyjxpVKujMRg==}
+ dependencies:
+ '@types/unist': 2.0.10
+ unist-util-is: 5.2.1
+ unist-util-visit-parents: 5.1.3
+ dev: false
+
+ /universalify@2.0.1:
+ resolution: {integrity: sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==}
+ engines: {node: '>= 10.0.0'}
+ dev: true
+
+ /unset-value@1.0.0:
+ resolution: {integrity: sha512-PcA2tsuGSF9cnySLHTLSh2qrQiJ70mn+r+Glzxv2TWZblxsxCC52BDlZoPCsz7STd9pN7EZetkWZBAvk4cgZdQ==}
+ engines: {node: '>=0.10.0'}
+ dependencies:
+ has-value: 0.3.1
+ isobject: 3.0.1
+ dev: true
+
+ /update-browserslist-db@1.0.13(browserslist@4.23.0):
+ resolution: {integrity: sha512-xebP81SNcPuNpPP3uzeW1NYXxI3rxyJzF3pD6sH4jE7o/IX+WtSpwnVU+qIsDPyk0d3hmFQ7mjqc6AtV604hbg==}
+ hasBin: true
+ peerDependencies:
+ browserslist: '>= 4.21.0'
+ dependencies:
+ browserslist: 4.23.0
+ escalade: 3.1.2
+ picocolors: 1.0.0
+
+ /uri-js@4.4.1:
+ resolution: {integrity: sha512-7rKUyy33Q1yc98pQ1DAmLtwX109F7TIfWlW1Ydo8Wl1ii1SeHieeh0HHfPeL2fMXK6z0s8ecKs9frCuLJvndBg==}
+ dependencies:
+ punycode: 2.3.1
+ dev: true
+
+ /urix@0.1.0:
+ resolution: {integrity: sha512-Am1ousAhSLBeB9cG/7k7r2R0zj50uDRlZHPGbazid5s9rlF1F/QKYObEKSIunSjIOkJZqwRRLpvewjEkM7pSqg==}
+ deprecated: Please see https://github.com/lydell/urix#deprecated
+ dev: true
+
+ /use-memo-one@1.1.3(react@18.3.0):
+ resolution: {integrity: sha512-g66/K7ZQGYrI6dy8GLpVcMsBp4s17xNkYJVSMvTEevGy3nDxHOfE6z8BVE22+5G5x7t3+bhzrlTDB7ObrEE0cQ==}
+ peerDependencies:
+ react: ^16.8.0 || ^17.0.0 || ^18.0.0
+ dependencies:
+ react: 18.3.0
+ dev: false
+
+ /use-sync-external-store@1.2.0(react@18.3.0):
+ resolution: {integrity: sha512-eEgnFxGQ1Ife9bzYs6VLi8/4X6CObHMw9Qr9tPY43iKwsPw8xE8+EFsf/2cFZ5S3esXgpWgtSCtLNS41F+sKPA==}
+ peerDependencies:
+ react: ^16.8.0 || ^17.0.0 || ^18.0.0
+ dependencies:
+ react: 18.3.0
+ dev: false
+
+ /use-sync-external-store@1.2.1(react@18.3.0):
+ resolution: {integrity: sha512-6MCBDr76UJmRpbF8pzP27uIoTocf3tITaMJ52mccgAhMJycuh5A/RL6mDZCTwTisj0Qfeq69FtjMCUX27U78oA==}
+ peerDependencies:
+ react: ^16.8.0 || ^17.0.0 || ^18.0.0
+ dependencies:
+ react: 18.3.0
+ dev: false
+
+ /use@3.1.1:
+ resolution: {integrity: sha512-cwESVXlO3url9YWlFW/TA9cshCEhtu7IKJ/p5soJ/gGpj7vbvFrAY/eIioQ6Dw23KjZhYgiIo8HOs1nQ2vr/oQ==}
+ engines: {node: '>=0.10.0'}
+ dev: true
+
+ /util-deprecate@1.0.2:
+ resolution: {integrity: sha512-EPD5q1uXyFxJpCrLnCc1nHnq3gOa6DZBocAIiI2TaSCA7VCJ1UJDMagCzIkXNsUYfD1daK//LTEQ8xiIbrHtcw==}
+ dev: true
+
+ /uvu@0.5.6:
+ resolution: {integrity: sha512-+g8ENReyr8YsOc6fv/NVJs2vFdHBnBNdfE49rshrTzDWOlUx4Gq7KOS2GD8eqhy2j+Ejq29+SbKH8yjkAqXqoA==}
+ engines: {node: '>=8'}
+ hasBin: true
+ dependencies:
+ dequal: 2.0.3
+ diff: 5.2.0
+ kleur: 4.1.5
+ sade: 1.8.1
+ dev: false
+
+ /v8-compile-cache-lib@3.0.1:
+ resolution: {integrity: sha512-wa7YjyUGfNZngI/vtK0UHAN+lgDCxBPCylVXGp0zu59Fz5aiGtNXaq3DhIov063MorB+VfufLh3JlF2KdTK3xg==}
+ dev: true
+
+ /validate-npm-package-license@3.0.4:
+ resolution: {integrity: sha512-DpKm2Ui/xN7/HQKCtpZxoRWBhZ9Z0kqtygG8XCgNQ8ZlDnxuQmWhj566j8fN4Cu3/JmbhsDo7fcAJq4s9h27Ew==}
+ dependencies:
+ spdx-correct: 3.2.0
+ spdx-expression-parse: 3.0.1
+ dev: true
+
+ /vary@1.1.2:
+ resolution: {integrity: sha512-BNGbWLfd0eUPabhkXUVm0j8uuvREyTh5ovRa/dyow/BqAbZJyC+5fU+IzQOzmAKzYqYRAISoRhdQr3eIZ/PXqg==}
+ engines: {node: '>= 0.8'}
+ dev: true
+
+ /vfile-location@2.0.6:
+ resolution: {integrity: sha512-sSFdyCP3G6Ka0CEmN83A2YCMKIieHx0EDaj5IDP4g1pa5ZJ4FJDvpO0WODLxo4LUX4oe52gmSCK7Jw4SBghqxA==}
+ dev: true
+
+ /vfile-location@4.1.0:
+ resolution: {integrity: sha512-YF23YMyASIIJXpktBa4vIGLJ5Gs88UB/XePgqPmTa7cDA+JeO3yclbpheQYCHjVHBn/yePzrXuygIL+xbvRYHw==}
+ dependencies:
+ '@types/unist': 2.0.10
+ vfile: 5.3.7
+ dev: false
+
+ /vfile-message@1.1.1:
+ resolution: {integrity: sha512-1WmsopSGhWt5laNir+633LszXvZ+Z/lxveBf6yhGsqnQIhlhzooZae7zV6YVM1Sdkw68dtAW3ow0pOdPANugvA==}
+ dependencies:
+ unist-util-stringify-position: 1.1.2
+ dev: true
+
+ /vfile-message@3.1.4:
+ resolution: {integrity: sha512-fa0Z6P8HUrQN4BZaX05SIVXic+7kE3b05PWAtPuYP9QLHsLKYR7/AlLW3NtOrpXRLeawpDLMsVkmk5DG0NXgWw==}
+ dependencies:
+ '@types/unist': 2.0.10
+ unist-util-stringify-position: 3.0.3
+ dev: false
+
+ /vfile-message@4.0.2:
+ resolution: {integrity: sha512-jRDZ1IMLttGj41KcZvlrYAaI3CfqpLpfpf+Mfig13viT6NKvRzWZ+lXz0Y5D60w6uJIBAOGq9mSHf0gktF0duw==}
+ dependencies:
+ '@types/unist': 3.0.2
+ unist-util-stringify-position: 4.0.0
+ dev: true
+
+ /vfile@3.0.1:
+ resolution: {integrity: sha512-y7Y3gH9BsUSdD4KzHsuMaCzRjglXN0W2EcMf0gpvu6+SbsGhMje7xDc8AEoeXy6mIwCKMI6BkjMsRjzQbhMEjQ==}
+ dependencies:
+ is-buffer: 2.0.5
+ replace-ext: 1.0.0
+ unist-util-stringify-position: 1.1.2
+ vfile-message: 1.1.1
+ dev: true
+
+ /vfile@5.3.7:
+ resolution: {integrity: sha512-r7qlzkgErKjobAmyNIkkSpizsFPYiUPuJb5pNW1RB4JcYVZhs4lIbVqk8XPk033CV/1z8ss5pkax8SuhGpcG8g==}
+ dependencies:
+ '@types/unist': 2.0.10
+ is-buffer: 2.0.5
+ unist-util-stringify-position: 3.0.3
+ vfile-message: 3.1.4
+ dev: false
+
+ /vite-plugin-svg-icons@2.0.1(vite@4.5.3):
+ resolution: {integrity: sha512-6ktD+DhV6Rz3VtedYvBKKVA2eXF+sAQVaKkKLDSqGUfnhqXl3bj5PPkVTl3VexfTuZy66PmINi8Q6eFnVfRUmA==}
+ peerDependencies:
+ vite: '>=2.0.0'
+ dependencies:
+ '@types/svgo': 2.6.4
+ cors: 2.8.5
+ debug: 4.3.4
+ etag: 1.8.1
+ fs-extra: 10.1.0
+ pathe: 0.2.0
+ svg-baker: 1.7.0
+ svgo: 2.8.0
+ vite: 4.5.3(@types/node@20.12.7)(sass@1.75.0)(terser@5.30.4)
+ transitivePeerDependencies:
+ - supports-color
+ dev: true
+
+ /vite-tsconfig-paths@4.3.2(typescript@5.4.5)(vite@4.5.3):
+ resolution: {integrity: sha512-0Vd/a6po6Q+86rPlntHye7F31zA2URZMbH8M3saAZ/xR9QoGN/L21bxEGfXdWmFdNkqPpRdxFT7nmNe12e9/uA==}
+ peerDependencies:
+ vite: '*'
+ peerDependenciesMeta:
+ vite:
+ optional: true
+ dependencies:
+ debug: 4.3.4
+ globrex: 0.1.2
+ tsconfck: 3.0.3(typescript@5.4.5)
+ vite: 4.5.3(@types/node@20.12.7)(sass@1.75.0)(terser@5.30.4)
+ transitivePeerDependencies:
+ - supports-color
+ - typescript
+ dev: true
+
+ /vite@4.5.3(@types/node@20.12.7)(sass@1.75.0)(terser@5.30.4):
+ resolution: {integrity: sha512-kQL23kMeX92v3ph7IauVkXkikdDRsYMGTVl5KY2E9OY4ONLvkHf04MDTbnfo6NKxZiDLWzVpP5oTa8hQD8U3dg==}
+ engines: {node: ^14.18.0 || >=16.0.0}
+ hasBin: true
+ peerDependencies:
+ '@types/node': '>= 14'
+ less: '*'
+ lightningcss: ^1.21.0
+ sass: '*'
+ stylus: '*'
+ sugarss: '*'
+ terser: ^5.4.0
+ peerDependenciesMeta:
+ '@types/node':
+ optional: true
+ less:
+ optional: true
+ lightningcss:
+ optional: true
+ sass:
+ optional: true
+ stylus:
+ optional: true
+ sugarss:
+ optional: true
+ terser:
+ optional: true
+ dependencies:
+ '@types/node': 20.12.7
+ esbuild: 0.18.20
+ postcss: 8.4.38
+ rollup: 3.29.4
+ sass: 1.75.0
+ terser: 5.30.4
+ optionalDependencies:
+ fsevents: 2.3.3
+
+ /void-elements@3.1.0:
+ resolution: {integrity: sha512-Dhxzh5HZuiHQhbvTW9AMetFfBHDMYpo23Uo9btPXgdYP+3T5S+p+jgNy7spra+veYhBP2dCSgxR/i2Y02h5/6w==}
+ engines: {node: '>=0.10.0'}
+ dev: false
+
+ /watchpack@2.4.1:
+ resolution: {integrity: sha512-8wrBCMtVhqcXP2Sup1ctSkga6uc2Bx0IIvKyT7yTFier5AXHooSI+QyQQAtTb7+E0IUCCKyTFmXqdqgum2XWGg==}
+ engines: {node: '>=10.13.0'}
+ dependencies:
+ glob-to-regexp: 0.4.1
+ graceful-fs: 4.2.11
+ dev: true
+
+ /web-namespaces@2.0.1:
+ resolution: {integrity: sha512-bKr1DkiNa2krS7qxNtdrtHAmzuYGFQLiQ13TsorsdT6ULTkPLKuu5+GsFpDlg6JFjUTwX2DyhMPG2be8uPrqsQ==}
+ dev: false
+
+ /webpack-sources@3.2.3:
+ resolution: {integrity: sha512-/DyMEOrDgLKKIG0fmvtz+4dUX/3Ghozwgm6iPp8KRhvn+eQf9+Q7GWxVNMk3+uCPWfdXYC4ExGBckIXdFEfH1w==}
+ engines: {node: '>=10.13.0'}
+ dev: true
+
+ /webpack@5.91.0:
+ resolution: {integrity: sha512-rzVwlLeBWHJbmgTC/8TvAcu5vpJNII+MelQpylD4jNERPwpBJOE2lEcko1zJX3QJeLjTTAnQxn/OJ8bjDzVQaw==}
+ engines: {node: '>=10.13.0'}
+ hasBin: true
+ peerDependencies:
+ webpack-cli: '*'
+ peerDependenciesMeta:
+ webpack-cli:
+ optional: true
+ dependencies:
+ '@types/eslint-scope': 3.7.7
+ '@types/estree': 1.0.5
+ '@webassemblyjs/ast': 1.12.1
+ '@webassemblyjs/wasm-edit': 1.12.1
+ '@webassemblyjs/wasm-parser': 1.12.1
+ acorn: 8.11.3
+ acorn-import-assertions: 1.9.0(acorn@8.11.3)
+ browserslist: 4.23.0
+ chrome-trace-event: 1.0.3
+ enhanced-resolve: 5.16.0
+ es-module-lexer: 1.5.0
+ eslint-scope: 5.1.1
+ events: 3.3.0
+ glob-to-regexp: 0.4.1
+ graceful-fs: 4.2.11
+ json-parse-even-better-errors: 2.3.1
+ loader-runner: 4.3.0
+ mime-types: 2.1.35
+ neo-async: 2.6.2
+ schema-utils: 3.3.0
+ tapable: 2.2.1
+ terser-webpack-plugin: 5.3.10(webpack@5.91.0)
+ watchpack: 2.4.1
+ webpack-sources: 3.2.3
+ transitivePeerDependencies:
+ - '@swc/core'
+ - esbuild
+ - uglify-js
+ dev: true
+
+ /which-boxed-primitive@1.0.2:
+ resolution: {integrity: sha512-bwZdv0AKLpplFY2KZRX6TvyuN7ojjr7lwkg6ml0roIy9YeuSr7JS372qlNW18UQYzgYK9ziGcerWqZOmEn9VNg==}
+ dependencies:
+ is-bigint: 1.0.4
+ is-boolean-object: 1.1.2
+ is-number-object: 1.0.7
+ is-string: 1.0.7
+ is-symbol: 1.0.4
+ dev: true
+
+ /which-builtin-type@1.1.3:
+ resolution: {integrity: sha512-YmjsSMDBYsM1CaFiayOVT06+KJeXf0o5M/CAd4o1lTadFAtacTUM49zoYxr/oroopFDfhvN6iEcBxUyc3gvKmw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ function.prototype.name: 1.1.6
+ has-tostringtag: 1.0.2
+ is-async-function: 2.0.0
+ is-date-object: 1.0.5
+ is-finalizationregistry: 1.0.2
+ is-generator-function: 1.0.10
+ is-regex: 1.1.4
+ is-weakref: 1.0.2
+ isarray: 2.0.5
+ which-boxed-primitive: 1.0.2
+ which-collection: 1.0.2
+ which-typed-array: 1.1.15
+ dev: true
+
+ /which-collection@1.0.2:
+ resolution: {integrity: sha512-K4jVyjnBdgvc86Y6BkaLZEN933SwYOuBFkdmBu9ZfkcAbdVbpITnDmjvZ/aQjRXQrv5EPkTnD1s39GiiqbngCw==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ is-map: 2.0.3
+ is-set: 2.0.3
+ is-weakmap: 2.0.2
+ is-weakset: 2.0.3
+ dev: true
+
+ /which-typed-array@1.1.15:
+ resolution: {integrity: sha512-oV0jmFtUky6CXfkqehVvBP/LSWJ2sy4vWMioiENyJLePrBO/yKyV9OyJySfAKosh+RYkIl5zJCNZ8/4JncrpdA==}
+ engines: {node: '>= 0.4'}
+ dependencies:
+ available-typed-arrays: 1.0.7
+ call-bind: 1.0.7
+ for-each: 0.3.3
+ gopd: 1.0.1
+ has-tostringtag: 1.0.2
+ dev: true
+
+ /which@1.3.1:
+ resolution: {integrity: sha512-HxJdYWq1MTIQbJ3nw0cqssHoTNU267KlrDuGZ1WYlxDStUtKUhOaJmh112/TZmHxxUfuJqPXSOm7tDyas0OSIQ==}
+ hasBin: true
+ dependencies:
+ isexe: 2.0.0
+ dev: true
+
+ /which@2.0.2:
+ resolution: {integrity: sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==}
+ engines: {node: '>= 8'}
+ hasBin: true
+ dependencies:
+ isexe: 2.0.0
+ dev: true
+
+ /wrap-ansi@7.0.0:
+ resolution: {integrity: sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==}
+ engines: {node: '>=10'}
+ dependencies:
+ ansi-styles: 4.3.0
+ string-width: 4.2.3
+ strip-ansi: 6.0.1
+ dev: true
+
+ /wrap-ansi@8.1.0:
+ resolution: {integrity: sha512-si7QWI6zUMq56bESFvagtmzMdGOtoxfR+Sez11Mobfc7tm+VkUckk9bW2UeffTGVUbOksxmSw0AA2gs8g71NCQ==}
+ engines: {node: '>=12'}
+ dependencies:
+ ansi-styles: 6.2.1
+ string-width: 5.1.2
+ strip-ansi: 7.1.0
+ dev: true
+
+ /wrappy@1.0.2:
+ resolution: {integrity: sha512-l4Sp/DRseor9wL6EvV2+TuQn63dMkPjZ/sp9XkghTEbV9KlPS1xUsZ3u7/IQO4wxtcFB4bgpQPRcR3QCvezPcQ==}
+ dev: true
+
+ /write-file-atomic@5.0.1:
+ resolution: {integrity: sha512-+QU2zd6OTD8XWIJCbffaiQeH9U73qIqafo1x6V1snCWYGJf6cVE0cDR4D8xRzcEnfI21IFrUPzPGtcPf8AC+Rw==}
+ engines: {node: ^14.17.0 || ^16.13.0 || >=18.0.0}
+ dependencies:
+ imurmurhash: 0.1.4
+ signal-exit: 4.1.0
+ dev: true
+
+ /write@1.0.3:
+ resolution: {integrity: sha512-/lg70HAjtkUgWPVZhZcm+T4hkL8Zbtp1nFNOn3lRrxnlv50SRBv7cR7RqR+GMsd3hUXy9hWBo4CHTbFTcOYwig==}
+ engines: {node: '>=4'}
+ dependencies:
+ mkdirp: 0.5.6
+ dev: true
+
+ /x-is-string@0.1.0:
+ resolution: {integrity: sha512-GojqklwG8gpzOVEVki5KudKNoq7MbbjYZCbyWzEz7tyPA7eleiE0+ePwOWQQRb5fm86rD3S8Tc0tSFf3AOv50w==}
+ dev: true
+
+ /xtend@4.0.2:
+ resolution: {integrity: sha512-LKYU1iAXJXUgAXn9URjiu+MWhyUXHsvfp7mcuYm9dSUKK0/CjtrUwFAxD82/mCWbtLsGjFIad0wIsod4zrTAEQ==}
+ engines: {node: '>=0.4'}
+ dev: true
+
+ /y18n@5.0.8:
+ resolution: {integrity: sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /yallist@3.1.1:
+ resolution: {integrity: sha512-a4UGQaWPH59mOXUYnAG2ewncQS4i4F43Tv3JoAM+s2VDAmS9NsK8GpDMLrCHPksFT7h3K6TOoUNn2pb7RoXx4g==}
+
+ /yallist@4.0.0:
+ resolution: {integrity: sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==}
+ dev: true
+
+ /yaml@1.10.2:
+ resolution: {integrity: sha512-r3vXyErRCYJ7wg28yvBY5VSoAF8ZvlcW9/BwUzEtUsjvX/DKs24dIkuwjtuprwJJHsbyUbLApepYTR1BN4uHrg==}
+ engines: {node: '>= 6'}
+
+ /yaml@2.3.1:
+ resolution: {integrity: sha512-2eHWfjaoXgTBC2jNM1LRef62VQa0umtvRiDSk6HSzW7RvS5YtkabJrwYLLEKWBc8a5U2PTSCs+dJjUTJdlHsWQ==}
+ engines: {node: '>= 14'}
+ dev: true
+
+ /yaml@2.4.1:
+ resolution: {integrity: sha512-pIXzoImaqmfOrL7teGUBt/T7ZDnyeGBWyXQBvOVhLkWLN37GXv8NMLK406UY6dS51JfcQHsmcW5cJ441bHg6Lg==}
+ engines: {node: '>= 14'}
+ hasBin: true
+ dev: true
+
+ /yargs-parser@10.1.0:
+ resolution: {integrity: sha512-VCIyR1wJoEBZUqk5PA+oOBF6ypbwh5aNB3I50guxAL/quggdfs4TtNHQrSazFA3fYZ+tEqfs0zIGlv0c/rgjbQ==}
+ dependencies:
+ camelcase: 4.1.0
+ dev: true
+
+ /yargs-parser@20.2.9:
+ resolution: {integrity: sha512-y11nGElTIV+CT3Zv9t7VKl+Q3hTQoT9a1Qzezhhl6Rp21gJ/IVTW7Z3y9EWXhuUBC2Shnf+DX0antecpAwSP8w==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /yargs-parser@21.1.1:
+ resolution: {integrity: sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==}
+ engines: {node: '>=12'}
+ dev: true
+
+ /yargs@17.7.2:
+ resolution: {integrity: sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==}
+ engines: {node: '>=12'}
+ dependencies:
+ cliui: 8.0.1
+ escalade: 3.1.2
+ get-caller-file: 2.0.5
+ require-directory: 2.1.1
+ string-width: 4.2.3
+ y18n: 5.0.8
+ yargs-parser: 21.1.1
+ dev: true
+
+ /yn@3.1.1:
+ resolution: {integrity: sha512-Ux4ygGWsu2c7isFWe8Yu1YluJmqVhxqK2cLXNQA5AcC3QfbGNpM7fu0Y8b/z16pXLnFxZYvWhd3fhBY9DLmC6Q==}
+ engines: {node: '>=6'}
+ dev: true
+
+ /yocto-queue@0.1.0:
+ resolution: {integrity: sha512-rVksvsnNCdJ/ohGc6xgPwyN8eheCxsiLM8mxuE/t/mOVqJewPuO1miLpTHQiRgTKCLexL4MeAFVagts7HmNZ2Q==}
+ engines: {node: '>=10'}
+ dev: true
+
+ /zustand@4.5.2(@types/react@18.3.0)(react@18.3.0):
+ resolution: {integrity: sha512-2cN1tPkDVkwCy5ickKrI7vijSjPksFRfqS6237NzT0vqSsztTNnQdHw9mmN7uBdk3gceVXU0a+21jFzFzAc9+g==}
+ engines: {node: '>=12.7.0'}
+ peerDependencies:
+ '@types/react': '>=16.8'
+ immer: '>=9.0.6'
+ react: '>=16.8'
+ peerDependenciesMeta:
+ '@types/react':
+ optional: true
+ immer:
+ optional: true
+ react:
+ optional: true
+ dependencies:
+ '@types/react': 18.3.0
+ react: 18.3.0
+ use-sync-external-store: 1.2.0(react@18.3.0)
+ dev: false
+
+ /zwitch@2.0.4:
+ resolution: {integrity: sha512-bXE4cR/kVZhKZX/RjPEflHaKVhUVl85noU3v6b8apfQEc1x4A+zBxjZ4lN8LqGd6WZ3dl98pY4o717VFmoPp+A==}
+ dev: false
diff --git a/frontend/postcss.config.js b/frontend/postcss.config.js
new file mode 100644
index 0000000..e61a917
--- /dev/null
+++ b/frontend/postcss.config.js
@@ -0,0 +1,8 @@
+export default {
+ plugins: {
+ 'postcss-import': {},
+ 'tailwindcss/nesting': 'postcss-nesting',
+ tailwindcss: {},
+ autoprefixer: {},
+ },
+};
diff --git a/frontend/src/App.tsx b/frontend/src/App.tsx
new file mode 100644
index 0000000..3dced77
--- /dev/null
+++ b/frontend/src/App.tsx
@@ -0,0 +1,23 @@
+import { App as AntdApp } from 'antd';
+
+import Router from '@/router/index';
+import AntdConfig from '@/theme/antd';
+
+
+import { MotionLazy } from './components/animate/motion-lazy';
+import { Inspector } from 'react-dev-inspector';
+
+function App() {
+ return (
+
+
+
+
+
+
+
+
+ );
+}
+
+export default App;
diff --git a/frontend/src/api/apiClient.ts b/frontend/src/api/apiClient.ts
new file mode 100644
index 0000000..537dc2c
--- /dev/null
+++ b/frontend/src/api/apiClient.ts
@@ -0,0 +1,104 @@
+import {message as Message} from 'antd';
+import axios, {AxiosError, AxiosRequestConfig, AxiosResponse} from 'axios';
+import {isEmpty} from 'ramda';
+import {t} from '@/locales/i18n';
+
+import {Result} from '#/api';
+import {ResultEnum, StorageEnum} from '#/enum';
+import {getItem, removeItem} from "@/utils/storage.ts";
+import {UserToken} from "#/entity.ts";
+
+// 创建 axios 实例
+const axiosInstance = axios.create({
+ baseURL: import.meta.env.VITE_APP_BASE_API as string,
+ timeout: 50000,
+ headers: { 'Content-Type': 'application/json;charset=utf-8' },
+});
+
+// 请求拦截
+axiosInstance.interceptors.request.use(
+ (config) => {
+ // 在请求被发送之前做些什么
+ // if(config.data){
+ // config.data = humps.decamelizeKeys(config.data)
+ // }
+ const token = getItem(StorageEnum.Token)
+ config.headers.Authorization = `Bearer ${token?.accessToken}`;
+ return config;
+ },
+ (error) => {
+ return Promise.reject(error);
+ },
+);
+
+// 响应拦截
+axiosInstance.interceptors.response.use(
+ (res: AxiosResponse) => {
+ if (!res.data && !res.status) throw new Error(t('sys_info.api.apiRequestFailed'));
+
+ let { status, data, message } = res.data;
+
+ // 业务请求成功
+ const hasSuccess = data && Reflect.has(res.data, 'status') && status === ResultEnum.SUCCESS;
+ if (hasSuccess) {
+ return data;
+ }
+ // 业务请求错误
+ throw new Error(message || t('sys_info.api.apiRequestFailed'));
+ },
+ (error: AxiosError) => {
+ const { response, message } = error || {};
+ if(response?.status === 401){
+ // Token失效,移除Token并跳转到登录页
+ Message.error("登录失效", 5);
+ removeItem(StorageEnum.Token);
+ window.location.href = '#/login'
+ }
+ let errMsg = '';
+ try {
+ errMsg = response?.data?.message || message;
+ } catch (error) {
+ throw new Error(error as unknown as string);
+ }
+ // 对响应错误做点什么
+ if (isEmpty(errMsg)) {
+ // checkStatus
+ // errMsg = checkStatus(response.data.status);
+ errMsg = t('sys_info.api.errorMessage');
+ }
+ Message.error(errMsg, 5);
+ return Promise.reject(error);
+ },
+);
+
+class APIClient {
+ get(config: AxiosRequestConfig): Promise {
+ return this.request({ ...config, method: 'GET' });
+ }
+
+ post(config: AxiosRequestConfig): Promise {
+ return this.request({ ...config, method: 'POST' });
+ }
+
+ put(config: AxiosRequestConfig): Promise {
+ return this.request({ ...config, method: 'PUT' });
+ }
+
+ delete(config: AxiosRequestConfig): Promise {
+ return this.request({ ...config, method: 'DELETE' });
+ }
+
+ request(config: AxiosRequestConfig): Promise {
+ return new Promise((resolve, reject) => {
+ axiosInstance
+ .request>(config)
+ .then((res: AxiosResponse) => {
+ resolve(res as unknown as Promise);
+ })
+ .catch((e: Error | AxiosError) => {
+ reject(e);
+ });
+ });
+ }
+}
+export default new APIClient();
diff --git a/frontend/src/api/services/accountService.ts b/frontend/src/api/services/accountService.ts
new file mode 100644
index 0000000..15b0b9e
--- /dev/null
+++ b/frontend/src/api/services/accountService.ts
@@ -0,0 +1,55 @@
+import apiClient from '../apiClient';
+
+import { Account } from '#/entity';
+
+export enum AccountApi {
+ list = '/account/list',
+ add = '/account/add',
+ update = '/account/update',
+ delete = '/account/delete',
+ refresh = '/account/refresh',
+ search = '/account/search',
+}
+
+const getAccountList = () =>
+ apiClient.get({ url: AccountApi.list }).then((res) => {
+ // 将shareList转为json对象
+ res.forEach((item) => {
+ if (item.shareList) {
+ item.shareList = JSON.parse(item.shareList);
+ }
+ });
+ return res;
+ });
+
+const searchAccountList = (email: string) =>
+ apiClient.post({ url: AccountApi.search, data: { email } }).then((res) => {
+ // 将shareList转为json对象
+ res.forEach((item) => {
+ if (item.shareList) {
+ item.shareList = JSON.parse(item.shareList);
+ }
+ });
+ return res;
+ });
+export interface AccountAddReq {
+ id?: number;
+ email: string;
+ password?: string;
+ shared?: number;
+ refreshToken?: string;
+ accessToken?: string;
+}
+const addAccount = (data: AccountAddReq) => apiClient.post({ url: AccountApi.add, data });
+const updateAccount = (data: AccountAddReq) => apiClient.post({ url: AccountApi.update, data });
+const deleteAccount = (id: number) => apiClient.post({ url: AccountApi.delete, data: { id } });
+const refreshAccount = (id: number) => apiClient.post({ url: AccountApi.refresh, data: { id } });
+
+export default {
+ getAccountList,
+ searchAccountList,
+ addAccount,
+ updateAccount,
+ deleteAccount,
+ refreshAccount,
+};
diff --git a/frontend/src/api/services/shareService.ts b/frontend/src/api/services/shareService.ts
new file mode 100644
index 0000000..c078b35
--- /dev/null
+++ b/frontend/src/api/services/shareService.ts
@@ -0,0 +1,37 @@
+import apiClient from '../apiClient';
+
+import {Share} from '#/entity';
+
+export enum ShareApi {
+ list = '/share/list',
+ add = '/share/add',
+ search = '/share/search',
+ delete = '/share/delete',
+ update = '/share/update',
+ statistic = '/share/statistic'
+}
+
+const getShareList = () => apiClient.get({ url: ShareApi.list });
+const addShare = (data: Share) => apiClient.post({ url: ShareApi.add, data });
+const updateShare = (data: Share) => apiClient.post({ url: ShareApi.update, data });
+const deleteShare = (data: Share) => apiClient.post({ url: ShareApi.delete, data });
+const searchShare = (email?: string,uniqueName?:string ) => apiClient.post({ url: ShareApi.search, data: {
+ email,
+ uniqueName
+}});
+
+type ShareStatistic = {
+ series: ApexAxisChartSeries;
+ categories: string[]
+}
+
+const getShareStatistic = (accountId: number) => apiClient.post({ url: ShareApi.statistic, data: { accountId } });
+
+export default {
+ getShareList,
+ addShare,
+ updateShare,
+ searchShare,
+ deleteShare,
+ getShareStatistic
+};
diff --git a/frontend/src/api/services/sysService.ts b/frontend/src/api/services/sysService.ts
new file mode 100644
index 0000000..03b92f3
--- /dev/null
+++ b/frontend/src/api/services/sysService.ts
@@ -0,0 +1,20 @@
+import apiClient from '../apiClient';
+
+export enum SysApi {
+ pandoraUsage = '/sys_info/usage',
+ setting = '/sys_info/info',
+}
+
+export type PandoraUsage = {
+ total: number;
+ current: number;
+ ttl: number;
+};
+
+const getPandoraUsage = () => apiClient.get({ url: SysApi.pandoraUsage });
+const getSetting = () => apiClient.get({ url: SysApi.setting });
+
+export default {
+ getPandoraUsage,
+ getSetting,
+};
diff --git a/frontend/src/api/services/userService.ts b/frontend/src/api/services/userService.ts
new file mode 100644
index 0000000..d566560
--- /dev/null
+++ b/frontend/src/api/services/userService.ts
@@ -0,0 +1,29 @@
+import apiClient from '../apiClient';
+import {UserInfo} from "#/entity.ts";
+
+// import {UserInfo, UserToken} from '#/entity';
+
+export interface SignInReq {
+ password: string;
+ token?: string;
+}
+
+// export type SignInRes = UserToken & {user: UserInfo};
+export type SignInRes = {
+ accessToken: string;
+ user: UserInfo;
+};
+
+export enum UserApi {
+ SignIn = 'login',
+ // SignIn = '/auth/signin',
+ Logout = '/auth/logout',
+}
+
+const signin = (data: SignInReq) => apiClient.post({ url: UserApi.SignIn, data });
+const logout = () => apiClient.get({ url: UserApi.Logout });
+
+export default {
+ signin,
+ logout,
+};
diff --git a/frontend/src/assets/icons/ic-analysis.svg b/frontend/src/assets/icons/ic-analysis.svg
new file mode 100644
index 0000000..1308805
--- /dev/null
+++ b/frontend/src/assets/icons/ic-analysis.svg
@@ -0,0 +1,8 @@
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-blog.svg b/frontend/src/assets/icons/ic-blog.svg
new file mode 100644
index 0000000..eb74d96
--- /dev/null
+++ b/frontend/src/assets/icons/ic-blog.svg
@@ -0,0 +1,7 @@
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-clear.svg b/frontend/src/assets/icons/ic-clear.svg
new file mode 100644
index 0000000..bc535b3
--- /dev/null
+++ b/frontend/src/assets/icons/ic-clear.svg
@@ -0,0 +1,10 @@
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-dashboard.svg b/frontend/src/assets/icons/ic-dashboard.svg
new file mode 100644
index 0000000..6cbedde
--- /dev/null
+++ b/frontend/src/assets/icons/ic-dashboard.svg
@@ -0,0 +1 @@
+
diff --git a/frontend/src/assets/icons/ic-left-arrow.svg b/frontend/src/assets/icons/ic-left-arrow.svg
new file mode 100644
index 0000000..7301e2c
--- /dev/null
+++ b/frontend/src/assets/icons/ic-left-arrow.svg
@@ -0,0 +1 @@
+
diff --git a/frontend/src/assets/icons/ic-locale_en_US.svg b/frontend/src/assets/icons/ic-locale_en_US.svg
new file mode 100644
index 0000000..f3980e2
--- /dev/null
+++ b/frontend/src/assets/icons/ic-locale_en_US.svg
@@ -0,0 +1 @@
+
diff --git a/frontend/src/assets/icons/ic-locale_zh_CN.svg b/frontend/src/assets/icons/ic-locale_zh_CN.svg
new file mode 100644
index 0000000..b9aa7a2
--- /dev/null
+++ b/frontend/src/assets/icons/ic-locale_zh_CN.svg
@@ -0,0 +1 @@
+
diff --git a/frontend/src/assets/icons/ic-logo.svg b/frontend/src/assets/icons/ic-logo.svg
new file mode 100644
index 0000000..63f85c3
--- /dev/null
+++ b/frontend/src/assets/icons/ic-logo.svg
@@ -0,0 +1,21 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-management.svg b/frontend/src/assets/icons/ic-management.svg
new file mode 100644
index 0000000..281dec2
--- /dev/null
+++ b/frontend/src/assets/icons/ic-management.svg
@@ -0,0 +1,4 @@
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-markdown.svg b/frontend/src/assets/icons/ic-markdown.svg
new file mode 100644
index 0000000..a9f882b
--- /dev/null
+++ b/frontend/src/assets/icons/ic-markdown.svg
@@ -0,0 +1,40 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-menu.svg b/frontend/src/assets/icons/ic-menu.svg
new file mode 100644
index 0000000..c9d8d36
--- /dev/null
+++ b/frontend/src/assets/icons/ic-menu.svg
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-menulevel.svg b/frontend/src/assets/icons/ic-menulevel.svg
new file mode 100644
index 0000000..e557e94
--- /dev/null
+++ b/frontend/src/assets/icons/ic-menulevel.svg
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-reset-password.svg b/frontend/src/assets/icons/ic-reset-password.svg
new file mode 100644
index 0000000..cf9e8c3
--- /dev/null
+++ b/frontend/src/assets/icons/ic-reset-password.svg
@@ -0,0 +1 @@
+
diff --git a/frontend/src/assets/icons/ic-right-arrow.svg b/frontend/src/assets/icons/ic-right-arrow.svg
new file mode 100644
index 0000000..9400f21
--- /dev/null
+++ b/frontend/src/assets/icons/ic-right-arrow.svg
@@ -0,0 +1 @@
+
diff --git a/frontend/src/assets/icons/ic-search.svg b/frontend/src/assets/icons/ic-search.svg
new file mode 100644
index 0000000..d06a451
--- /dev/null
+++ b/frontend/src/assets/icons/ic-search.svg
@@ -0,0 +1 @@
+
diff --git a/frontend/src/assets/icons/ic-setting.svg b/frontend/src/assets/icons/ic-setting.svg
new file mode 100644
index 0000000..48468ac
--- /dev/null
+++ b/frontend/src/assets/icons/ic-setting.svg
@@ -0,0 +1 @@
+
diff --git a/frontend/src/assets/icons/ic-settings-exit-fullscreen.svg b/frontend/src/assets/icons/ic-settings-exit-fullscreen.svg
new file mode 100644
index 0000000..46453e9
--- /dev/null
+++ b/frontend/src/assets/icons/ic-settings-exit-fullscreen.svg
@@ -0,0 +1,6 @@
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-settings-fullscreen.svg b/frontend/src/assets/icons/ic-settings-fullscreen.svg
new file mode 100644
index 0000000..a13fcc8
--- /dev/null
+++ b/frontend/src/assets/icons/ic-settings-fullscreen.svg
@@ -0,0 +1,6 @@
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-settings-mode-moon.svg b/frontend/src/assets/icons/ic-settings-mode-moon.svg
new file mode 100644
index 0000000..be81693
--- /dev/null
+++ b/frontend/src/assets/icons/ic-settings-mode-moon.svg
@@ -0,0 +1,4 @@
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-settings-mode-sun.svg b/frontend/src/assets/icons/ic-settings-mode-sun.svg
new file mode 100644
index 0000000..aeaeeac
--- /dev/null
+++ b/frontend/src/assets/icons/ic-settings-mode-sun.svg
@@ -0,0 +1,13 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-user.svg b/frontend/src/assets/icons/ic-user.svg
new file mode 100644
index 0000000..141aeba
--- /dev/null
+++ b/frontend/src/assets/icons/ic-user.svg
@@ -0,0 +1,4 @@
+
+
+
+
diff --git a/frontend/src/assets/icons/ic-workbench.svg b/frontend/src/assets/icons/ic-workbench.svg
new file mode 100644
index 0000000..6ea1c6d
--- /dev/null
+++ b/frontend/src/assets/icons/ic-workbench.svg
@@ -0,0 +1,4 @@
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_blank.svg b/frontend/src/assets/icons/ic_blank.svg
new file mode 100644
index 0000000..d187ec2
--- /dev/null
+++ b/frontend/src/assets/icons/ic_blank.svg
@@ -0,0 +1,4 @@
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_chat.svg b/frontend/src/assets/icons/ic_chat.svg
new file mode 100644
index 0000000..d8b905c
--- /dev/null
+++ b/frontend/src/assets/icons/ic_chat.svg
@@ -0,0 +1,28 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_decline.svg b/frontend/src/assets/icons/ic_decline.svg
new file mode 100644
index 0000000..0931d38
--- /dev/null
+++ b/frontend/src/assets/icons/ic_decline.svg
@@ -0,0 +1 @@
+
diff --git a/frontend/src/assets/icons/ic_delivery.svg b/frontend/src/assets/icons/ic_delivery.svg
new file mode 100644
index 0000000..e0b077c
--- /dev/null
+++ b/frontend/src/assets/icons/ic_delivery.svg
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_disabled.svg b/frontend/src/assets/icons/ic_disabled.svg
new file mode 100644
index 0000000..2164101
--- /dev/null
+++ b/frontend/src/assets/icons/ic_disabled.svg
@@ -0,0 +1,4 @@
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_external.svg b/frontend/src/assets/icons/ic_external.svg
new file mode 100644
index 0000000..d38340e
--- /dev/null
+++ b/frontend/src/assets/icons/ic_external.svg
@@ -0,0 +1,4 @@
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file.svg b/frontend/src/assets/icons/ic_file.svg
new file mode 100644
index 0000000..f5295c2
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file.svg
@@ -0,0 +1,18 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file_ai.svg b/frontend/src/assets/icons/ic_file_ai.svg
new file mode 100644
index 0000000..4d8098a
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file_ai.svg
@@ -0,0 +1,25 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file_audio.svg b/frontend/src/assets/icons/ic_file_audio.svg
new file mode 100644
index 0000000..329f232
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file_audio.svg
@@ -0,0 +1,24 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file_excel.svg b/frontend/src/assets/icons/ic_file_excel.svg
new file mode 100644
index 0000000..cb80eb2
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file_excel.svg
@@ -0,0 +1,19 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file_img.svg b/frontend/src/assets/icons/ic_file_img.svg
new file mode 100644
index 0000000..a95194a
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file_img.svg
@@ -0,0 +1,26 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file_pdf.svg b/frontend/src/assets/icons/ic_file_pdf.svg
new file mode 100644
index 0000000..8ed54c9
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file_pdf.svg
@@ -0,0 +1,19 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file_ppt.svg b/frontend/src/assets/icons/ic_file_ppt.svg
new file mode 100644
index 0000000..f2d7f14
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file_ppt.svg
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file_psd.svg b/frontend/src/assets/icons/ic_file_psd.svg
new file mode 100644
index 0000000..7ecbee0
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file_psd.svg
@@ -0,0 +1,25 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file_txt.svg b/frontend/src/assets/icons/ic_file_txt.svg
new file mode 100644
index 0000000..1d34c34
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file_txt.svg
@@ -0,0 +1,26 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file_video.svg b/frontend/src/assets/icons/ic_file_video.svg
new file mode 100644
index 0000000..fb6eca6
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file_video.svg
@@ -0,0 +1,20 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file_word.svg b/frontend/src/assets/icons/ic_file_word.svg
new file mode 100644
index 0000000..b112fe5
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file_word.svg
@@ -0,0 +1,19 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_file_zip.svg b/frontend/src/assets/icons/ic_file_zip.svg
new file mode 100644
index 0000000..f34001e
--- /dev/null
+++ b/frontend/src/assets/icons/ic_file_zip.svg
@@ -0,0 +1,27 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_folder.svg b/frontend/src/assets/icons/ic_folder.svg
new file mode 100644
index 0000000..01f6671
--- /dev/null
+++ b/frontend/src/assets/icons/ic_folder.svg
@@ -0,0 +1,5 @@
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_label.svg b/frontend/src/assets/icons/ic_label.svg
new file mode 100644
index 0000000..933e635
--- /dev/null
+++ b/frontend/src/assets/icons/ic_label.svg
@@ -0,0 +1,4 @@
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_mail.svg b/frontend/src/assets/icons/ic_mail.svg
new file mode 100644
index 0000000..f9561c4
--- /dev/null
+++ b/frontend/src/assets/icons/ic_mail.svg
@@ -0,0 +1,15 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_order.svg b/frontend/src/assets/icons/ic_order.svg
new file mode 100644
index 0000000..cc8dbb7
--- /dev/null
+++ b/frontend/src/assets/icons/ic_order.svg
@@ -0,0 +1,45 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/frontend/src/assets/icons/ic_rise.svg b/frontend/src/assets/icons/ic_rise.svg
new file mode 100644
index 0000000..9368912
--- /dev/null
+++ b/frontend/src/assets/icons/ic_rise.svg
@@ -0,0 +1 @@
+
diff --git a/frontend/src/assets/images/background/cyan-blur.png b/frontend/src/assets/images/background/cyan-blur.png
new file mode 100644
index 0000000..b5dbc95
Binary files /dev/null and b/frontend/src/assets/images/background/cyan-blur.png differ
diff --git a/frontend/src/assets/images/background/dashboard.png b/frontend/src/assets/images/background/dashboard.png
new file mode 100644
index 0000000..26a2e88
Binary files /dev/null and b/frontend/src/assets/images/background/dashboard.png differ
diff --git a/frontend/src/assets/images/background/dashboard2.png b/frontend/src/assets/images/background/dashboard2.png
new file mode 100644
index 0000000..5f008e5
Binary files /dev/null and b/frontend/src/assets/images/background/dashboard2.png differ
diff --git a/frontend/src/assets/images/background/overlay_2.jpg b/frontend/src/assets/images/background/overlay_2.jpg
new file mode 100644
index 0000000..119d428
Binary files /dev/null and b/frontend/src/assets/images/background/overlay_2.jpg differ
diff --git a/frontend/src/assets/images/background/red-blur.png b/frontend/src/assets/images/background/red-blur.png
new file mode 100644
index 0000000..a0df012
Binary files /dev/null and b/frontend/src/assets/images/background/red-blur.png differ
diff --git a/frontend/src/assets/images/characters/character_3.png b/frontend/src/assets/images/characters/character_3.png
new file mode 100644
index 0000000..2d639cc
Binary files /dev/null and b/frontend/src/assets/images/characters/character_3.png differ
diff --git a/frontend/src/assets/images/characters/character_4.png b/frontend/src/assets/images/characters/character_4.png
new file mode 100644
index 0000000..0ce49ff
Binary files /dev/null and b/frontend/src/assets/images/characters/character_4.png differ
diff --git a/frontend/src/assets/images/characters/character_6.png b/frontend/src/assets/images/characters/character_6.png
new file mode 100644
index 0000000..f59a844
Binary files /dev/null and b/frontend/src/assets/images/characters/character_6.png differ
diff --git a/frontend/src/assets/images/characters/character_8.png b/frontend/src/assets/images/characters/character_8.png
new file mode 100644
index 0000000..44f1708
Binary files /dev/null and b/frontend/src/assets/images/characters/character_8.png differ
diff --git a/frontend/src/assets/images/cover/cover_3.jpg b/frontend/src/assets/images/cover/cover_3.jpg
new file mode 100644
index 0000000..d54c5f1
Binary files /dev/null and b/frontend/src/assets/images/cover/cover_3.jpg differ
diff --git a/frontend/src/assets/images/cover/cover_4.jpg b/frontend/src/assets/images/cover/cover_4.jpg
new file mode 100644
index 0000000..4f500f2
Binary files /dev/null and b/frontend/src/assets/images/cover/cover_4.jpg differ
diff --git a/frontend/src/assets/images/cover/profile-banner.png b/frontend/src/assets/images/cover/profile-banner.png
new file mode 100644
index 0000000..16cbfe9
Binary files /dev/null and b/frontend/src/assets/images/cover/profile-banner.png differ
diff --git a/frontend/src/assets/images/glass/ic_glass_bag.png b/frontend/src/assets/images/glass/ic_glass_bag.png
new file mode 100644
index 0000000..41cb3c5
Binary files /dev/null and b/frontend/src/assets/images/glass/ic_glass_bag.png differ
diff --git a/frontend/src/assets/images/glass/ic_glass_buy.png b/frontend/src/assets/images/glass/ic_glass_buy.png
new file mode 100644
index 0000000..065ffcd
Binary files /dev/null and b/frontend/src/assets/images/glass/ic_glass_buy.png differ
diff --git a/frontend/src/assets/images/glass/ic_glass_message.png b/frontend/src/assets/images/glass/ic_glass_message.png
new file mode 100644
index 0000000..10b6c95
Binary files /dev/null and b/frontend/src/assets/images/glass/ic_glass_message.png differ
diff --git a/frontend/src/assets/images/glass/ic_glass_users.png b/frontend/src/assets/images/glass/ic_glass_users.png
new file mode 100644
index 0000000..415f0c9
Binary files /dev/null and b/frontend/src/assets/images/glass/ic_glass_users.png differ
diff --git a/frontend/src/assets/images/logo.png b/frontend/src/assets/images/logo.png
new file mode 100644
index 0000000..596c94f
Binary files /dev/null and b/frontend/src/assets/images/logo.png differ
diff --git a/frontend/src/assets/images/qinshihuang.jpg b/frontend/src/assets/images/qinshihuang.jpg
new file mode 100644
index 0000000..a4a0e43
Binary files /dev/null and b/frontend/src/assets/images/qinshihuang.jpg differ
diff --git a/frontend/src/assets/react.svg b/frontend/src/assets/react.svg
new file mode 100644
index 0000000..6c87de9
--- /dev/null
+++ b/frontend/src/assets/react.svg
@@ -0,0 +1 @@
+
\ No newline at end of file
diff --git a/frontend/src/assets/vite.svg b/frontend/src/assets/vite.svg
new file mode 100644
index 0000000..e7b8dfb
--- /dev/null
+++ b/frontend/src/assets/vite.svg
@@ -0,0 +1 @@
+
\ No newline at end of file
diff --git a/frontend/src/components/animate/motion-container.tsx b/frontend/src/components/animate/motion-container.tsx
new file mode 100644
index 0000000..e602413
--- /dev/null
+++ b/frontend/src/components/animate/motion-container.tsx
@@ -0,0 +1,40 @@
+import { m, MotionProps } from 'framer-motion';
+
+import { varContainer } from './variants/container';
+
+interface Props extends MotionProps {
+ className?: string;
+}
+
+/**
+ * Motion 通用容器
+ *
+ * variants: [变体可以用于使用单个动画道具为组件的整个子树设置动画](https://www.framer.com/motion/animation/#variants)
+ *
+ * Variants 是一组预定义的对象
+ * const variants = {
+ * visible: { opacity: 1 },
+ * hidden: { opacity: 0 },
+ * }
+ *
+ * 需要指定 inital 和 animate 属性名
+ *
+ */
+export default function MotionContainer({ children, className }: Props) {
+ return (
+
+ {children}
+
+ );
+}
diff --git a/frontend/src/components/animate/motion-lazy.tsx b/frontend/src/components/animate/motion-lazy.tsx
new file mode 100644
index 0000000..e28642f
--- /dev/null
+++ b/frontend/src/components/animate/motion-lazy.tsx
@@ -0,0 +1,15 @@
+import { LazyMotion, m, domMax } from 'framer-motion';
+
+type Props = {
+ children: React.ReactNode;
+};
+/**
+ * [Reduce bundle size by lazy-loading a subset of Motion's features](https://www.framer.com/motion/lazy-motion/)
+ */
+export function MotionLazy({ children }: Props) {
+ return (
+
+ {children}
+
+ );
+}
diff --git a/frontend/src/components/animate/motion-viewport.tsx b/frontend/src/components/animate/motion-viewport.tsx
new file mode 100644
index 0000000..51c146b
--- /dev/null
+++ b/frontend/src/components/animate/motion-viewport.tsx
@@ -0,0 +1,28 @@
+import { MotionProps, m } from 'framer-motion';
+
+import { varContainer } from './variants';
+
+interface Props extends MotionProps {
+ className?: string;
+}
+/**
+ * [whileInView: 元素可以在进出视口时设置动画](https://www.framer.com/motion/scroll-animations/#scroll-triggered-animations)
+ *
+ * + viewport: [视口](https://www.framer.com/motion/scroll-animations/###viewport)
+ *
+ * + once: 仅触发一次
+ */
+export default function MotionViewport({ children, className, ...other }: Props) {
+ return (
+
+ {children}
+
+ );
+}
diff --git a/frontend/src/components/animate/types.ts b/frontend/src/components/animate/types.ts
new file mode 100644
index 0000000..2b236c6
--- /dev/null
+++ b/frontend/src/components/animate/types.ts
@@ -0,0 +1,26 @@
+export type VariantsType = {
+ durationIn?: number;
+ durationOut?: number;
+ easeIn?: [];
+ easeOut?: [];
+ distance?: number;
+};
+
+export type TranHoverType = {
+ duration?: number;
+ ease?: [];
+};
+export type TranEnterType = {
+ durationIn?: number;
+ easeIn?: [];
+};
+export type TranExitType = {
+ durationOut?: number;
+ easeOut?: [];
+};
+
+export type BackgroundType = {
+ duration?: number;
+ ease?: [];
+ colors?: string[];
+};
diff --git a/frontend/src/components/animate/variants/action.ts b/frontend/src/components/animate/variants/action.ts
new file mode 100644
index 0000000..caee72a
--- /dev/null
+++ b/frontend/src/components/animate/variants/action.ts
@@ -0,0 +1,9 @@
+/**
+ * https://www.framer.com/motion/gestures/
+ * @param hover
+ * @param tap
+ */
+export const varHover = (hover = 1.09, tap = 0.97) => ({
+ hover: { scale: hover },
+ tap: { scale: tap },
+});
diff --git a/frontend/src/components/animate/variants/background.ts b/frontend/src/components/animate/variants/background.ts
new file mode 100644
index 0000000..9f2123c
--- /dev/null
+++ b/frontend/src/components/animate/variants/background.ts
@@ -0,0 +1,103 @@
+import { BackgroundType } from '../types';
+
+export const varBgColor = (props?: BackgroundType) => {
+ const colors = props?.colors || ['#19dcea', '#b22cff'];
+ const duration = props?.duration || 5;
+ const ease = props?.ease || 'linear';
+
+ return {
+ animate: {
+ background: colors,
+ transition: { duration, ease },
+ },
+ };
+};
+
+// ----------------------------------------------------------------------
+
+export const varBgKenburns = (props?: BackgroundType) => {
+ const duration = props?.duration || 5;
+ const ease = props?.ease || 'easeOut';
+
+ return {
+ top: {
+ animate: {
+ scale: [1, 1.25],
+ y: [0, -15],
+ transformOrigin: ['50% 16%', '50% top'],
+ transition: { duration, ease },
+ },
+ },
+ bottom: {
+ animate: {
+ scale: [1, 1.25],
+ y: [0, 15],
+ transformOrigin: ['50% 84%', '50% bottom'],
+ transition: { duration, ease },
+ },
+ },
+ left: {
+ animate: {
+ scale: [1, 1.25],
+ x: [0, 20],
+ y: [0, 15],
+ transformOrigin: ['16% 50%', '0% left'],
+ transition: { duration, ease },
+ },
+ },
+ right: {
+ animate: {
+ scale: [1, 1.25],
+ x: [0, -20],
+ y: [0, -15],
+ transformOrigin: ['84% 50%', '0% right'],
+ transition: { duration, ease },
+ },
+ },
+ };
+};
+
+// ----------------------------------------------------------------------
+
+export const varBgPan = (props?: BackgroundType) => {
+ const colors = props?.colors || ['#ee7752', '#e73c7e', '#23a6d5', '#23d5ab'];
+ const duration = props?.duration || 5;
+ const ease = props?.ease || 'linear';
+
+ const gradient = (deg: number) => `linear-gradient(${deg}deg, ${colors})`;
+
+ return {
+ top: {
+ animate: {
+ backgroundImage: [gradient(0), gradient(0)],
+ backgroundPosition: ['center 99%', 'center 1%'],
+ backgroundSize: ['100% 600%', '100% 600%'],
+ transition: { duration, ease },
+ },
+ },
+ right: {
+ animate: {
+ backgroundPosition: ['1% center', '99% center'],
+ backgroundImage: [gradient(270), gradient(270)],
+ backgroundSize: ['600% 100%', '600% 100%'],
+ transition: { duration, ease },
+ },
+ },
+ bottom: {
+ animate: {
+ backgroundImage: [gradient(0), gradient(0)],
+ backgroundPosition: ['center 1%', 'center 99%'],
+ backgroundSize: ['100% 600%', '100% 600%'],
+ transition: { duration, ease },
+ },
+ },
+ left: {
+ animate: {
+ backgroundPosition: ['99% center', '1% center'],
+ backgroundImage: [gradient(270), gradient(270)],
+ backgroundSize: ['600% 100%', '600% 100%'],
+ transition: { duration, ease },
+ },
+ },
+ };
+};
diff --git a/frontend/src/components/animate/variants/bounce.ts b/frontend/src/components/animate/variants/bounce.ts
new file mode 100644
index 0000000..880bad4
--- /dev/null
+++ b/frontend/src/components/animate/variants/bounce.ts
@@ -0,0 +1,111 @@
+import { VariantsType } from '../types';
+
+import { varTranEnter, varTranExit } from './transition';
+
+export const varBounce = (props?: VariantsType) => {
+ const durationIn = props?.durationIn;
+ const durationOut = props?.durationOut;
+ const easeIn = props?.easeIn;
+ const easeOut = props?.easeOut;
+
+ return {
+ // IN
+ in: {
+ initial: {},
+ animate: {
+ scale: [0.3, 1.1, 0.9, 1.03, 0.97, 1],
+ opacity: [0, 1, 1, 1, 1, 1],
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ scale: [0.9, 1.1, 0.3],
+ opacity: [1, 1, 0],
+ },
+ },
+ inUp: {
+ initial: {},
+ animate: {
+ y: [720, -24, 12, -4, 0],
+ scaleY: [4, 0.9, 0.95, 0.985, 1],
+ opacity: [0, 1, 1, 1, 1],
+ transition: { ...varTranEnter({ durationIn, easeIn }) },
+ },
+ exit: {
+ y: [12, -24, 720],
+ scaleY: [0.985, 0.9, 3],
+ opacity: [1, 1, 0],
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inDown: {
+ initial: {},
+ animate: {
+ y: [-720, 24, -12, 4, 0],
+ scaleY: [4, 0.9, 0.95, 0.985, 1],
+ opacity: [0, 1, 1, 1, 1],
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ y: [-12, 24, -720],
+ scaleY: [0.985, 0.9, 3],
+ opacity: [1, 1, 0],
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inLeft: {
+ initial: {},
+ animate: {
+ x: [-720, 24, -12, 4, 0],
+ scaleX: [3, 1, 0.98, 0.995, 1],
+ opacity: [0, 1, 1, 1, 1],
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ x: [0, 24, -720],
+ scaleX: [1, 0.9, 2],
+ opacity: [1, 1, 0],
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inRight: {
+ initial: {},
+ animate: {
+ x: [720, -24, 12, -4, 0],
+ scaleX: [3, 1, 0.98, 0.995, 1],
+ opacity: [0, 1, 1, 1, 1],
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ x: [0, -24, 720],
+ scaleX: [1, 0.9, 2],
+ opacity: [1, 1, 0],
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+
+ // OUT
+ out: {
+ animate: { scale: [0.9, 1.1, 0.3], opacity: [1, 1, 0] },
+ },
+ outUp: {
+ animate: {
+ y: [-12, 24, -720],
+ scaleY: [0.985, 0.9, 3],
+ opacity: [1, 1, 0],
+ },
+ },
+ outDown: {
+ animate: {
+ y: [12, -24, 720],
+ scaleY: [0.985, 0.9, 3],
+ opacity: [1, 1, 0],
+ },
+ },
+ outLeft: {
+ animate: { x: [0, 24, -720], scaleX: [1, 0.9, 2], opacity: [1, 1, 0] },
+ },
+ outRight: {
+ animate: { x: [0, -24, 720], scaleX: [1, 0.9, 2], opacity: [1, 1, 0] },
+ },
+ };
+};
diff --git a/frontend/src/components/animate/variants/container.ts b/frontend/src/components/animate/variants/container.ts
new file mode 100644
index 0000000..1ff849c
--- /dev/null
+++ b/frontend/src/components/animate/variants/container.ts
@@ -0,0 +1,26 @@
+export type Props = {
+ staggerIn?: number;
+ delayIn?: number;
+ staggerOut?: number;
+};
+
+export const varContainer = (props?: Props) => {
+ const staggerIn = props?.staggerIn || 0.05;
+ const delayIn = props?.staggerIn || 0.05;
+ const staggerOut = props?.staggerIn || 0.05;
+
+ return {
+ animate: {
+ transition: {
+ staggerChildren: staggerIn,
+ delayChildren: delayIn,
+ },
+ },
+ exit: {
+ transition: {
+ staggerChildren: staggerOut,
+ staggerDirection: -1,
+ },
+ },
+ };
+};
diff --git a/frontend/src/components/animate/variants/fade.ts b/frontend/src/components/animate/variants/fade.ts
new file mode 100644
index 0000000..decd7f2
--- /dev/null
+++ b/frontend/src/components/animate/variants/fade.ts
@@ -0,0 +1,134 @@
+import { VariantsType } from '../types';
+
+//
+import { varTranEnter, varTranExit } from './transition';
+
+// ----------------------------------------------------------------------
+
+export const varFade = (props?: VariantsType) => {
+ const distance = props?.distance || 120;
+ const durationIn = props?.durationIn;
+ const durationOut = props?.durationOut;
+ const easeIn = props?.easeIn;
+ const easeOut = props?.easeOut;
+
+ return {
+ // IN
+ in: {
+ initial: { opacity: 0 },
+ animate: { opacity: 1, transition: varTranEnter },
+ exit: { opacity: 0, transition: varTranExit },
+ },
+ inUp: {
+ initial: { y: distance, opacity: 0 },
+ animate: {
+ y: 0,
+ opacity: 1,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ y: distance,
+ opacity: 0,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inDown: {
+ initial: { y: -distance, opacity: 0 },
+ animate: {
+ y: 0,
+ opacity: 1,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ y: -distance,
+ opacity: 0,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inLeft: {
+ initial: { x: -distance, opacity: 0 },
+ animate: {
+ x: 0,
+ opacity: 1,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ x: -distance,
+ opacity: 0,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inRight: {
+ initial: { x: distance, opacity: 0 },
+ animate: {
+ x: 0,
+ opacity: 1,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ x: distance,
+ opacity: 0,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+
+ // OUT
+ out: {
+ initial: { opacity: 1 },
+ animate: { opacity: 0, transition: varTranEnter({ durationIn, easeIn }) },
+ exit: { opacity: 1, transition: varTranExit({ durationOut, easeOut }) },
+ },
+ outUp: {
+ initial: { y: 0, opacity: 1 },
+ animate: {
+ y: -distance,
+ opacity: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ y: 0,
+ opacity: 1,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ outDown: {
+ initial: { y: 0, opacity: 1 },
+ animate: {
+ y: distance,
+ opacity: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ y: 0,
+ opacity: 1,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ outLeft: {
+ initial: { x: 0, opacity: 1 },
+ animate: {
+ x: -distance,
+ opacity: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ x: 0,
+ opacity: 1,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ outRight: {
+ initial: { x: 0, opacity: 1 },
+ animate: {
+ x: distance,
+ opacity: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ x: 0,
+ opacity: 1,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ };
+};
diff --git a/frontend/src/components/animate/variants/flip.ts b/frontend/src/components/animate/variants/flip.ts
new file mode 100644
index 0000000..98fc35d
--- /dev/null
+++ b/frontend/src/components/animate/variants/flip.ts
@@ -0,0 +1,61 @@
+import { VariantsType } from '../types';
+
+//
+import { varTranEnter, varTranExit } from './transition';
+
+// ----------------------------------------------------------------------
+
+export const varFlip = (props?: VariantsType) => {
+ const durationIn = props?.durationIn;
+ const durationOut = props?.durationOut;
+ const easeIn = props?.easeIn;
+ const easeOut = props?.easeOut;
+
+ return {
+ // IN
+ inX: {
+ initial: { rotateX: -180, opacity: 0 },
+ animate: {
+ rotateX: 0,
+ opacity: 1,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ rotateX: -180,
+ opacity: 0,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inY: {
+ initial: { rotateY: -180, opacity: 0 },
+ animate: {
+ rotateY: 0,
+ opacity: 1,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ rotateY: -180,
+ opacity: 0,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+
+ // OUT
+ outX: {
+ initial: { rotateX: 0, opacity: 1 },
+ animate: {
+ rotateX: 70,
+ opacity: 0,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ outY: {
+ initial: { rotateY: 0, opacity: 1 },
+ animate: {
+ rotateY: 70,
+ opacity: 0,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ };
+};
diff --git a/frontend/src/components/animate/variants/index.ts b/frontend/src/components/animate/variants/index.ts
new file mode 100644
index 0000000..b3994f8
--- /dev/null
+++ b/frontend/src/components/animate/variants/index.ts
@@ -0,0 +1,98 @@
+import { varBgKenburns, varBgPan, varBgColor } from './background';
+import { varBounce } from './bounce';
+import { varFade } from './fade';
+import { varFlip } from './flip';
+import { varRotate } from './rotate';
+import { varScale } from './scale';
+import { varSlide } from './slide';
+import { varZoom } from './zoom';
+
+export * from './action';
+export * from './background';
+export * from './bounce';
+export * from './container';
+export * from './fade';
+export * from './flip';
+export * from './path';
+export * from './rotate';
+export * from './scale';
+export * from './slide';
+export * from './transition';
+export * from './zoom';
+
+export function getVariant(variant = 'slideInUp') {
+ return {
+ // Slide
+ slideInUp: varSlide().inUp,
+ slideInDown: varSlide().inDown,
+ slideInLeft: varSlide().inLeft,
+ slideInRight: varSlide().inRight,
+ slideOutUp: varSlide().outUp,
+ slideOutDown: varSlide().outDown,
+ slideOutLeft: varSlide().outLeft,
+ slideOutRight: varSlide().outRight,
+ // Fade
+ fadeIn: varFade().in,
+ fadeInUp: varFade().inUp,
+ fadeInDown: varFade().inDown,
+ fadeInLeft: varFade().inLeft,
+ fadeInRight: varFade().inRight,
+ fadeOut: varFade().out,
+ fadeOutUp: varFade().outUp,
+ fadeOutDown: varFade().outDown,
+ fadeOutLeft: varFade().outLeft,
+ fadeOutRight: varFade().outRight,
+ // Zoom
+ zoomIn: varZoom({ distance: 80 }).in,
+ zoomInUp: varZoom({ distance: 80 }).inUp,
+ zoomInDown: varZoom({ distance: 80 }).inDown,
+ zoomInLeft: varZoom({ distance: 240 }).inLeft,
+ zoomInRight: varZoom({ distance: 240 }).inRight,
+ zoomOut: varZoom().out,
+ zoomOutLeft: varZoom().outLeft,
+ zoomOutRight: varZoom().outRight,
+ zoomOutUp: varZoom().outUp,
+ zoomOutDown: varZoom().outDown,
+ // Bounce
+ bounceIn: varBounce().in,
+ bounceInUp: varBounce().inUp,
+ bounceInDown: varBounce().inDown,
+ bounceInLeft: varBounce().inLeft,
+ bounceInRight: varBounce().inRight,
+ bounceOut: varBounce().out,
+ bounceOutUp: varBounce().outUp,
+ bounceOutDown: varBounce().outDown,
+ bounceOutLeft: varBounce().outLeft,
+ bounceOutRight: varBounce().outRight,
+ // Flip
+ flipInX: varFlip().inX,
+ flipInY: varFlip().inY,
+ flipOutX: varFlip().outX,
+ flipOutY: varFlip().outY,
+ // Scale
+ scaleInX: varScale().inX,
+ scaleInY: varScale().inY,
+ scaleOutX: varScale().outX,
+ scaleOutY: varScale().outY,
+ // Rotate
+ rotateIn: varRotate().in,
+ rotateOut: varRotate().out,
+ // Background
+ kenburnsTop: varBgKenburns().top,
+ kenburnsBottom: varBgKenburns().bottom,
+ kenburnsLeft: varBgKenburns().left,
+ kenburnsRight: varBgKenburns().right,
+ panTop: varBgPan().top,
+ panBottom: varBgPan().bottom,
+ panLeft: varBgPan().left,
+ panRight: varBgPan().right,
+ color2x: varBgColor(),
+ color3x: varBgColor({ colors: ['#19dcea', '#b22cff', '#ea2222'] }),
+ color4x: varBgColor({
+ colors: ['#19dcea', '#b22cff', '#ea2222', '#f5be10'],
+ }),
+ color5x: varBgColor({
+ colors: ['#19dcea', '#b22cff', '#ea2222', '#f5be10', '#3bd80d'],
+ }),
+ }[variant];
+}
diff --git a/frontend/src/components/animate/variants/path.ts b/frontend/src/components/animate/variants/path.ts
new file mode 100644
index 0000000..a4bee2c
--- /dev/null
+++ b/frontend/src/components/animate/variants/path.ts
@@ -0,0 +1,14 @@
+// ----------------------------------------------------------------------
+
+export const TRANSITION = {
+ duration: 2,
+ ease: [0.43, 0.13, 0.23, 0.96],
+};
+
+export const varPath = {
+ animate: {
+ fillOpacity: [0, 0, 1],
+ pathLength: [1, 0.4, 0],
+ transition: TRANSITION,
+ },
+};
diff --git a/frontend/src/components/animate/variants/rotate.ts b/frontend/src/components/animate/variants/rotate.ts
new file mode 100644
index 0000000..0b3fdfb
--- /dev/null
+++ b/frontend/src/components/animate/variants/rotate.ts
@@ -0,0 +1,40 @@
+import { VariantsType } from '../types';
+
+//
+import { varTranEnter, varTranExit } from './transition';
+
+// ----------------------------------------------------------------------
+
+export const varRotate = (props?: VariantsType) => {
+ const durationIn = props?.durationIn;
+ const durationOut = props?.durationOut;
+ const easeIn = props?.easeIn;
+ const easeOut = props?.easeOut;
+
+ return {
+ // IN
+ in: {
+ initial: { opacity: 0, rotate: -360 },
+ animate: {
+ opacity: 1,
+ rotate: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ opacity: 0,
+ rotate: -360,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+
+ // OUT
+ out: {
+ initial: { opacity: 1, rotate: 0 },
+ animate: {
+ opacity: 0,
+ rotate: -360,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ };
+};
diff --git a/frontend/src/components/animate/variants/scale.ts b/frontend/src/components/animate/variants/scale.ts
new file mode 100644
index 0000000..abee403
--- /dev/null
+++ b/frontend/src/components/animate/variants/scale.ts
@@ -0,0 +1,60 @@
+import { VariantsType } from '../types';
+
+import { varTranEnter, varTranExit } from './transition';
+
+// ----------------------------------------------------------------------
+
+export const varScale = (props?: VariantsType) => {
+ const durationIn = props?.durationIn;
+ const durationOut = props?.durationOut;
+ const easeIn = props?.easeIn;
+ const easeOut = props?.easeOut;
+
+ return {
+ // IN
+ inX: {
+ initial: { scaleX: 0, opacity: 0 },
+ animate: {
+ scaleX: 1,
+ opacity: 1,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ scaleX: 0,
+ opacity: 0,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inY: {
+ initial: { scaleY: 0, opacity: 0 },
+ animate: {
+ scaleY: 1,
+ opacity: 1,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ scaleY: 0,
+ opacity: 0,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+
+ // OUT
+ outX: {
+ initial: { scaleX: 1, opacity: 1 },
+ animate: {
+ scaleX: 0,
+ opacity: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ },
+ outY: {
+ initial: { scaleY: 1, opacity: 1 },
+ animate: {
+ scaleY: 0,
+ opacity: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ },
+ };
+};
diff --git a/frontend/src/components/animate/variants/slide.ts b/frontend/src/components/animate/variants/slide.ts
new file mode 100644
index 0000000..d9adacb
--- /dev/null
+++ b/frontend/src/components/animate/variants/slide.ts
@@ -0,0 +1,72 @@
+import { VariantsType } from '../types';
+
+//
+import { varTranEnter, varTranExit } from './transition';
+
+// ----------------------------------------------------------------------
+
+export const varSlide = (props?: VariantsType) => {
+ const distance = props?.distance || 160;
+ const durationIn = props?.durationIn;
+ const durationOut = props?.durationOut;
+ const easeIn = props?.easeIn;
+ const easeOut = props?.easeOut;
+
+ return {
+ // IN
+ inUp: {
+ initial: { y: distance },
+ animate: { y: 0, transition: varTranEnter({ durationIn, easeIn }) },
+ exit: { y: distance, transition: varTranExit({ durationOut, easeOut }) },
+ },
+ inDown: {
+ initial: { y: -distance },
+ animate: { y: 0, transition: varTranEnter({ durationIn, easeIn }) },
+ exit: { y: -distance, transition: varTranExit({ durationOut, easeOut }) },
+ },
+ inLeft: {
+ initial: { x: -distance },
+ animate: { x: 0, transition: varTranEnter({ durationIn, easeIn }) },
+ exit: { x: -distance, transition: varTranExit({ durationOut, easeOut }) },
+ },
+ inRight: {
+ initial: { x: distance },
+ animate: { x: 0, transition: varTranEnter({ durationIn, easeIn }) },
+ exit: { x: distance, transition: varTranExit({ durationOut, easeOut }) },
+ },
+
+ // OUT
+ outUp: {
+ initial: { y: 0 },
+ animate: {
+ y: -distance,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: { y: 0, transition: varTranExit({ durationOut, easeOut }) },
+ },
+ outDown: {
+ initial: { y: 0 },
+ animate: {
+ y: distance,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: { y: 0, transition: varTranExit({ durationOut, easeOut }) },
+ },
+ outLeft: {
+ initial: { x: 0 },
+ animate: {
+ x: -distance,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: { x: 0, transition: varTranExit({ durationOut, easeOut }) },
+ },
+ outRight: {
+ initial: { x: 0 },
+ animate: {
+ x: distance,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: { x: 0, transition: varTranExit({ durationOut, easeOut }) },
+ },
+ };
+};
diff --git a/frontend/src/components/animate/variants/transition.ts b/frontend/src/components/animate/variants/transition.ts
new file mode 100644
index 0000000..7ed7081
--- /dev/null
+++ b/frontend/src/components/animate/variants/transition.ts
@@ -0,0 +1,25 @@
+import { TranHoverType, TranEnterType, TranExitType } from '../types';
+
+// https://www.framer.com/motion/transition/
+// A transition defines how values animate from one state to another.
+
+export const varTranHover = (props?: TranHoverType) => {
+ const duration = props?.duration || 0.32;
+ const ease = props?.ease || [0.43, 0.13, 0.23, 0.96];
+
+ return { duration, ease };
+};
+
+export const varTranEnter = (props?: TranEnterType) => {
+ const duration = props?.durationIn || 0.64;
+ const ease = props?.easeIn || [0.43, 0.13, 0.23, 0.96];
+
+ return { duration, ease };
+};
+
+export const varTranExit = (props?: TranExitType) => {
+ const duration = props?.durationOut || 0.48;
+ const ease = props?.easeOut || [0.43, 0.13, 0.23, 0.96];
+
+ return { duration, ease };
+};
diff --git a/frontend/src/components/animate/variants/zoom.ts b/frontend/src/components/animate/variants/zoom.ts
new file mode 100644
index 0000000..86e078b
--- /dev/null
+++ b/frontend/src/components/animate/variants/zoom.ts
@@ -0,0 +1,137 @@
+import { VariantsType } from '../types';
+
+//
+import { varTranEnter, varTranExit } from './transition';
+
+// ----------------------------------------------------------------------
+
+export const varZoom = (props?: VariantsType) => {
+ const distance = props?.distance || 720;
+ const durationIn = props?.durationIn;
+ const durationOut = props?.durationOut;
+ const easeIn = props?.easeIn;
+ const easeOut = props?.easeOut;
+
+ return {
+ // IN
+ in: {
+ initial: { scale: 0, opacity: 0 },
+ animate: {
+ scale: 1,
+ opacity: 1,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ scale: 0,
+ opacity: 0,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inUp: {
+ initial: { scale: 0, opacity: 0, translateY: distance },
+ animate: {
+ scale: 1,
+ opacity: 1,
+ translateY: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ scale: 0,
+ opacity: 0,
+ translateY: distance,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inDown: {
+ initial: { scale: 0, opacity: 0, translateY: -distance },
+ animate: {
+ scale: 1,
+ opacity: 1,
+ translateY: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ scale: 0,
+ opacity: 0,
+ translateY: -distance,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inLeft: {
+ initial: { scale: 0, opacity: 0, translateX: -distance },
+ animate: {
+ scale: 1,
+ opacity: 1,
+ translateX: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ scale: 0,
+ opacity: 0,
+ translateX: -distance,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+ inRight: {
+ initial: { scale: 0, opacity: 0, translateX: distance },
+ animate: {
+ scale: 1,
+ opacity: 1,
+ translateX: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ exit: {
+ scale: 0,
+ opacity: 0,
+ translateX: distance,
+ transition: varTranExit({ durationOut, easeOut }),
+ },
+ },
+
+ // OUT
+ out: {
+ initial: { scale: 1, opacity: 1 },
+ animate: {
+ scale: 0,
+ opacity: 0,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ },
+ outUp: {
+ initial: { scale: 1, opacity: 1 },
+ animate: {
+ scale: 0,
+ opacity: 0,
+ translateY: -distance,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ },
+ outDown: {
+ initial: { scale: 1, opacity: 1 },
+ animate: {
+ scale: 0,
+ opacity: 0,
+ translateY: distance,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ },
+ outLeft: {
+ initial: { scale: 1, opacity: 1 },
+ animate: {
+ scale: 0,
+ opacity: 0,
+ translateX: -distance,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ },
+ outRight: {
+ initial: { scale: 1, opacity: 1 },
+ animate: {
+ scale: 0,
+ opacity: 0,
+ translateX: distance,
+ transition: varTranEnter({ durationIn, easeIn }),
+ },
+ },
+ };
+};
diff --git a/frontend/src/components/card/index.tsx b/frontend/src/components/card/index.tsx
new file mode 100644
index 0000000..130267e
--- /dev/null
+++ b/frontend/src/components/card/index.tsx
@@ -0,0 +1,40 @@
+import { CSSProperties, ReactNode } from 'react';
+
+import { useSettings } from '@/store/settingStore';
+import { useThemeToken } from '@/theme/hooks';
+
+import { ThemeMode } from '#/enum';
+
+type Props = {
+ children?: ReactNode;
+ className?: string;
+ style?: CSSProperties;
+};
+export default function Card({ children, ...other }: Props) {
+ const { colorBgContainer } = useThemeToken();
+ const { themeMode } = useSettings();
+
+ const boxShadow: { [key in ThemeMode]: string } = {
+ light: 'rgba(145, 158, 171, 0.2) 0px 0px 2px 0px, rgba(145, 158, 171, 0.12) 0px 12px 24px -4px',
+ dark: 'rgba(0, 0, 0, 0.2) 0px 0px 2px 0px, rgba(0, 0, 0, 0.12) 0px 12px 24px -4px',
+ };
+ return (
+
+ {children}
+
+ );
+}
diff --git a/frontend/src/components/chart/chart.tsx b/frontend/src/components/chart/chart.tsx
new file mode 100644
index 0000000..c5fd5c6
--- /dev/null
+++ b/frontend/src/components/chart/chart.tsx
@@ -0,0 +1,21 @@
+import { memo } from 'react';
+import ApexChart from 'react-apexcharts';
+
+import { useSettings } from '@/store/settingStore';
+import { useThemeToken } from '@/theme/hooks';
+
+import { StyledApexChart } from './styles';
+
+import type { Props as ApexChartProps } from 'react-apexcharts';
+
+function Chart(props: ApexChartProps) {
+ const { themeMode } = useSettings();
+ const theme = useThemeToken();
+ return (
+
+
+
+ );
+}
+
+export default memo(Chart);
diff --git a/frontend/src/components/chart/styles.ts b/frontend/src/components/chart/styles.ts
new file mode 100644
index 0000000..b59cba1
--- /dev/null
+++ b/frontend/src/components/chart/styles.ts
@@ -0,0 +1,58 @@
+import { GlobalToken } from 'antd';
+import Color from 'color';
+import styled from 'styled-components';
+
+import { ThemeMode } from '#/enum';
+
+export const StyledApexChart = styled.div<{ $thememode: ThemeMode; $theme: GlobalToken }>`
+ .apexcharts-canvas {
+ /* TOOLTIP */
+ .apexcharts-tooltip {
+ color: ${(props) => props.$theme.colorText};
+ border-radius: 10px;
+ backdrop-filter: blur(6px);
+ background-color: ${(props) => Color(props.$theme.colorBgElevated).alpha(0.8).toString()};
+ box-shadow: ${(props) =>
+ props.$thememode === ThemeMode.Light
+ ? `rgba(145, 158, 171, 0.24) 0px 0px 2px 0px, rgba(145, 158, 171, 0.24) -20px 20px 40px -4px`
+ : `rgba(0, 0, 0, 0.24) 0px 0px 2px 0px, rgba(0, 0, 0, 0.24) -20px 20px 40px -4px;`};
+ .apexcharts-tooltip-title {
+ text-align: center;
+ font-weight: bold;
+ background-color: rgba(145, 158, 171, 0.08);
+ }
+ }
+
+ /* TOOLTIP X */
+ .apexcharts-xaxistooltip {
+ color: ${(props) => props.$theme.colorText};
+ border-radius: 10px;
+ backdrop-filter: blur(6px);
+ border-color: transparent;
+ box-shadow: ${(props) =>
+ props.$thememode === ThemeMode.Light
+ ? `rgba(145, 158, 171, 0.24) 0px 0px 2px 0px, rgba(145, 158, 171, 0.24) -20px 20px 40px -4px`
+ : `rgba(0, 0, 0, 0.24) 0px 0px 2px 0px, rgba(0, 0, 0, 0.24) -20px 20px 40px -4px;`};
+ background-color: ${(props) => Color(props.$theme.colorBgElevated).alpha(0.8).toString()};
+ &::before {
+ border-bottom-color: rgba(145, 158, 171, 0.24);
+ }
+ &::after {
+ border-bottom-color: rgba(255, 255, 255, 0.8);
+ }
+ }
+
+ /* LEGEND */
+ .apexcharts-legend {
+ padding: 0;
+ .apexcharts-legend-series {
+ display: inline-flex !important;
+ align-items: ecnter;
+ }
+ .apexcharts-legend-text {
+ line-height: 18px;
+ text-transform: capitalize;
+ }
+ }
+ }
+`;
diff --git a/frontend/src/components/chart/useChart.ts b/frontend/src/components/chart/useChart.ts
new file mode 100644
index 0000000..07d6367
--- /dev/null
+++ b/frontend/src/components/chart/useChart.ts
@@ -0,0 +1,206 @@
+import { ApexOptions } from 'apexcharts';
+import { mergeDeepRight } from 'ramda';
+
+import { useThemeToken } from '@/theme/hooks';
+
+export default function useChart(options: ApexOptions) {
+ const theme = useThemeToken();
+
+ const LABEL_TOTAL = {
+ show: true,
+ label: 'Total',
+ color: theme.colorTextSecondary,
+ fontSize: theme.fontSizeHeading2,
+ lineHeight: theme.lineHeightHeading2,
+ };
+
+ const LABEL_VALUE = {
+ offsetY: 8,
+ color: theme.colorText,
+ fontSize: theme.fontSizeHeading3,
+ lineHeight: theme.lineHeightHeading3,
+ };
+
+ const baseOptions = {
+ // Colors
+ colors: [
+ theme.colorPrimary,
+ theme.colorWarning,
+ theme.colorInfo,
+ theme.colorError,
+ theme.colorSuccess,
+ theme.colorWarningActive,
+ theme.colorSuccessActive,
+ theme.colorInfoActive,
+ theme.colorInfoText,
+ ],
+
+ // Chart
+ chart: {
+ toolbar: { show: false },
+ zoom: { enabled: false },
+ foreColor: theme.colorTextDisabled,
+ fontFamily: theme.fontFamily,
+ },
+
+ // States
+ states: {
+ hover: {
+ filter: {
+ type: 'lighten',
+ value: 0.04,
+ },
+ },
+ active: {
+ filter: {
+ type: 'darken',
+ value: 0.88,
+ },
+ },
+ },
+
+ // Fill
+ fill: {
+ opacity: 1,
+ gradient: {
+ type: 'vertical',
+ shadeIntensity: 0,
+ opacityFrom: 0.4,
+ opacityTo: 0,
+ stops: [0, 100],
+ },
+ },
+
+ // Datalabels
+ dataLabels: {
+ enabled: false,
+ },
+
+ // Stroke
+ stroke: {
+ width: 3,
+ curve: 'smooth',
+ lineCap: 'round',
+ },
+
+ // Grid
+ grid: {
+ strokeDashArray: 3,
+ borderColor: theme.colorSplit,
+ xaxis: {
+ lines: {
+ show: false,
+ },
+ },
+ },
+
+ // Xaxis
+ xaxis: {
+ axisBorder: { show: false },
+ axisTicks: { show: false },
+ },
+
+ // Markers
+ markers: {
+ size: 0,
+ },
+
+ // Tooltip
+ tooltip: {
+ theme: false,
+ x: {
+ show: true,
+ },
+ },
+
+ // Legend
+ legend: {
+ show: true,
+ fontSize: 13,
+ position: 'top',
+ horizontalAlign: 'right',
+ markers: {
+ radius: 12,
+ },
+ fontWeight: 500,
+ itemMargin: {
+ horizontal: 8,
+ },
+ labels: {
+ colors: theme.colorText,
+ },
+ },
+
+ // plotOptions
+ plotOptions: {
+ // Bar
+ bar: {
+ borderRadius: 4,
+ columnWidth: '28%',
+ borderRadiusApplication: 'end',
+ borderRadiusWhenStacked: 'last',
+ },
+
+ // Pie + Donut
+ pie: {
+ donut: {
+ labels: {
+ show: true,
+ value: LABEL_VALUE,
+ total: LABEL_TOTAL,
+ },
+ },
+ },
+
+ // Radialbar
+ radialBar: {
+ track: {
+ strokeWidth: '100%',
+ },
+ dataLabels: {
+ value: LABEL_VALUE,
+ total: LABEL_TOTAL,
+ },
+ },
+
+ // Radar
+ radar: {
+ polygons: {
+ fill: { colors: ['transparent'] },
+ strokeColors: theme.colorSplit,
+ connectorColors: theme.colorSplit,
+ },
+ },
+
+ // polarArea
+ polarArea: {
+ rings: {
+ strokeColor: theme.colorSplit,
+ },
+ spokes: {
+ connectorColors: theme.colorSplit,
+ },
+ },
+ },
+
+ // Responsive
+ responsive: [
+ {
+ // sm
+ breakpoint: theme.screenSM,
+ options: {
+ plotOptions: { bar: { columnWidth: '40%' } },
+ },
+ },
+ {
+ // md
+ breakpoint: theme.screenMD,
+ options: {
+ plotOptions: { bar: { columnWidth: '32%' } },
+ },
+ },
+ ],
+ };
+
+ return mergeDeepRight(baseOptions, options) as ApexOptions;
+}
diff --git a/frontend/src/components/editor/index.tsx b/frontend/src/components/editor/index.tsx
new file mode 100644
index 0000000..3e8f661
--- /dev/null
+++ b/frontend/src/components/editor/index.tsx
@@ -0,0 +1,40 @@
+/* eslint-disable import/order */
+import '@/utils/highlight';
+import ReactQuill, { ReactQuillProps } from 'react-quill';
+import Toolbar, { formats } from './toolbar';
+import { useSettings } from '@/store/settingStore';
+import { useThemeToken } from '@/theme/hooks';
+import { StyledEditor } from './styles';
+
+interface Props extends ReactQuillProps {
+ sample?: boolean;
+}
+export default function Editor({ id = 'slash-quill', sample = false, ...other }: Props) {
+ const token = useThemeToken();
+ const { themeMode } = useSettings();
+ const modules = {
+ toolbar: {
+ container: `#${id}`,
+ },
+ history: {
+ delay: 500,
+ maxStack: 100,
+ userOnly: true,
+ },
+ syntax: true,
+ clipboard: {
+ matchVisual: false,
+ },
+ };
+ return (
+
+
+
+
+ );
+}
diff --git a/frontend/src/components/editor/styles.ts b/frontend/src/components/editor/styles.ts
new file mode 100644
index 0000000..241a764
--- /dev/null
+++ b/frontend/src/components/editor/styles.ts
@@ -0,0 +1,187 @@
+import { GlobalToken } from 'antd';
+import styled from 'styled-components';
+
+import { ThemeMode } from '#/enum';
+
+type KeyofToken = keyof GlobalToken;
+const getHeadingStyle = (level: 1 | 2 | 3 | 4 | 5, token: GlobalToken) => {
+ const fontSizeHeading: KeyofToken = `fontSizeHeading${level}`;
+ const lineHeightHeading: KeyofToken = `lineHeightHeading${level}`;
+
+ return {
+ margin: 0,
+ color: token.colorTextHeading,
+ fontWeight: 800,
+ fontSize: token[fontSizeHeading],
+ lineHeight: token[lineHeightHeading],
+ };
+};
+
+const StyledEditor = styled.div<{ $token: GlobalToken; $thememode: ThemeMode }>`
+ h1 {
+ ${(props) => getHeadingStyle(1, props.$token)};
+ }
+ h2 {
+ ${(props) => getHeadingStyle(2, props.$token)};
+ }
+ h3 {
+ ${(props) => getHeadingStyle(3, props.$token)};
+ }
+ h4 {
+ ${(props) => getHeadingStyle(4, props.$token)};
+ }
+ h5 {
+ ${(props) => getHeadingStyle(5, props.$token)};
+ }
+ overflow: hidden;
+ position: relative;
+ border-radius: 8px;
+ border: 1px solid rgba(119, 145, 170, 0.2);
+ & .ql-container.ql-snow {
+ border: none;
+ line-height: 1.6;
+ font-weight: 400;
+ font-size: 0.875rem;
+ }
+ & .ql-editor {
+ min-height: 160px;
+ max-height: 640px;
+ background-color: rgba(145, 158, 171, 0.08);
+ &.ql-blank::before {
+ font-style: normal;
+ color: rgb(145, 158, 171);
+ }
+ & pre.ql-syntax {
+ border-radius: 8px;
+ line-height: 1.57143;
+ font-size: 0.875rem;
+ font-family: 'Public Sans', sans-serif;
+ font-weight: 400;
+ padding: 16px;
+ border-radius: 8px;
+ background-color: rgb(22, 28, 36);
+ }
+ }
+`;
+
+const StyledToolbar = styled.div<{ $token: GlobalToken; $thememode: ThemeMode }>`
+ & .ql-snow.ql-toolbar button:hover .ql-fill,
+ .ql-snow .ql-toolbar button:hover .ql-fill,
+ .ql-snow.ql-toolbar button:focus .ql-fill,
+ .ql-snow .ql-toolbar button:focus .ql-fill,
+ .ql-snow.ql-toolbar button.ql-active .ql-fill,
+ .ql-snow .ql-toolbar button.ql-active .ql-fill,
+ .ql-snow.ql-toolbar .ql-picker-label:hover .ql-fill,
+ .ql-snow .ql-toolbar .ql-picker-label:hover .ql-fill,
+ .ql-snow.ql-toolbar .ql-picker-label.ql-active .ql-fill,
+ .ql-snow .ql-toolbar .ql-picker-label.ql-active .ql-fill,
+ .ql-snow.ql-toolbar .ql-picker-item:hover .ql-fill,
+ .ql-snow .ql-toolbar .ql-picker-item:hover .ql-fill,
+ .ql-snow.ql-toolbar .ql-picker-item.ql-selected .ql-fill,
+ .ql-snow .ql-toolbar .ql-picker-item.ql-selected .ql-fill,
+ .ql-snow.ql-toolbar button:hover .ql-stroke.ql-fill,
+ .ql-snow .ql-toolbar button:hover .ql-stroke.ql-fill,
+ .ql-snow.ql-toolbar button:focus .ql-stroke.ql-fill,
+ .ql-snow .ql-toolbar button:focus .ql-stroke.ql-fill,
+ .ql-snow.ql-toolbar button.ql-active .ql-stroke.ql-fill,
+ .ql-snow .ql-toolbar button.ql-active .ql-stroke.ql-fill,
+ .ql-snow.ql-toolbar .ql-picker-label:hover .ql-stroke.ql-fill,
+ .ql-snow .ql-toolbar .ql-picker-label:hover .ql-stroke.ql-fill,
+ .ql-snow.ql-toolbar .ql-picker-label.ql-active .ql-stroke.ql-fill,
+ .ql-snow .ql-toolbar .ql-picker-label.ql-active .ql-stroke.ql-fill,
+ .ql-snow.ql-toolbar .ql-picker-item:hover .ql-stroke.ql-fill,
+ .ql-snow .ql-toolbar .ql-picker-item:hover .ql-stroke.ql-fill,
+ .ql-snow.ql-toolbar .ql-picker-item.ql-selected .ql-stroke.ql-fill,
+ .ql-snow .ql-toolbar .ql-picker-item.ql-selected .ql-stroke.ql-fill {
+ fill: ${(props) => props.$token.colorPrimary};
+ }
+ & .ql-snow.ql-toolbar button:hover,
+ .ql-snow .ql-toolbar button:hover,
+ .ql-snow.ql-toolbar button:focus,
+ .ql-snow .ql-toolbar button:focus,
+ .ql-snow.ql-toolbar button.ql-active,
+ .ql-snow .ql-toolbar button.ql-active,
+ .ql-snow.ql-toolbar .ql-picker-label:hover,
+ .ql-snow .ql-toolbar .ql-picker-label:hover,
+ .ql-snow.ql-toolbar .ql-picker-label.ql-active,
+ .ql-snow .ql-toolbar .ql-picker-label.ql-active,
+ .ql-snow.ql-toolbar .ql-picker-item:hover,
+ .ql-snow .ql-toolbar .ql-picker-item:hover,
+ .ql-snow.ql-toolbar .ql-picker-item.ql-selected,
+ .ql-snow .ql-toolbar .ql-picker-item.ql-selected {
+ color: ${(props) => props.$token.colorPrimary};
+ }
+
+ & .ql-snow.ql-toolbar button:hover .ql-stroke,
+ .ql-snow .ql-toolbar button:hover .ql-stroke,
+ .ql-snow.ql-toolbar button:focus .ql-stroke,
+ .ql-snow .ql-toolbar button:focus .ql-stroke,
+ .ql-snow.ql-toolbar button.ql-active .ql-stroke,
+ .ql-snow .ql-toolbar button.ql-active .ql-stroke,
+ .ql-snow.ql-toolbar .ql-picker-label:hover .ql-stroke,
+ .ql-snow .ql-toolbar .ql-picker-label:hover .ql-stroke,
+ .ql-snow.ql-toolbar .ql-picker-label.ql-active .ql-stroke,
+ .ql-snow .ql-toolbar .ql-picker-label.ql-active .ql-stroke,
+ .ql-snow.ql-toolbar .ql-picker-item:hover .ql-stroke,
+ .ql-snow .ql-toolbar .ql-picker-item:hover .ql-stroke,
+ .ql-snow.ql-toolbar .ql-picker-item.ql-selected .ql-stroke,
+ .ql-snow .ql-toolbar .ql-picker-item.ql-selected .ql-stroke,
+ .ql-snow.ql-toolbar button:hover .ql-stroke-miter,
+ .ql-snow .ql-toolbar button:hover .ql-stroke-miter,
+ .ql-snow.ql-toolbar button:focus .ql-stroke-miter,
+ .ql-snow .ql-toolbar button:focus .ql-stroke-miter,
+ .ql-snow.ql-toolbar button.ql-active .ql-stroke-miter,
+ .ql-snow .ql-toolbar button.ql-active .ql-stroke-miter,
+ .ql-snow.ql-toolbar .ql-picker-label:hover .ql-stroke-miter,
+ .ql-snow .ql-toolbar .ql-picker-label:hover .ql-stroke-miter,
+ .ql-snow.ql-toolbar .ql-picker-label.ql-active .ql-stroke-miter,
+ .ql-snow .ql-toolbar .ql-picker-label.ql-active .ql-stroke-miter,
+ .ql-snow.ql-toolbar .ql-picker-item:hover .ql-stroke-miter,
+ .ql-snow .ql-toolbar .ql-picker-item:hover .ql-stroke-miter,
+ .ql-snow.ql-toolbar .ql-picker-item.ql-selected .ql-stroke-miter,
+ .ql-snow .ql-toolbar .ql-picker-item.ql-selected .ql-stroke-miter {
+ stroke: ${(props) => props.$token.colorPrimary};
+ }
+
+ & .ql-stroke {
+ stroke: ${(props) => props.$token.colorTextBase};
+ }
+ & .ql-fill,
+ .ql-stroke.ql-fill {
+ fill: ${(props) => props.$token.colorTextBase};
+ }
+
+ & .ql-toolbar.ql-snow {
+ border: none;
+ border-bottom: 1px solid rgba(119, 145, 170, 0.2);
+ // Button
+ & button {
+ padding: 0;
+ display: flex;
+ align-items: center;
+ justify-content: center;
+ border-radius: 4px;
+ }
+ & button svg,
+ span svg {
+ width: 20px;
+ height: 20px;
+ }
+ & .ql-picker-label {
+ border-radius: 4px;
+ border-color: transparent !important;
+ background-color: ${(props) => props.$token.colorBgContainerDisabled};
+ color: ${(props) => props.$token.colorTextBase};
+ }
+ & .ql-picker-options {
+ margin-top: 4px;
+ border: none;
+ max-height: 200px;
+ overflow: auto;
+ border-radius: 8px;
+ color: ${(props) => props.$token.colorTextBase};
+ background-color: ${(props) => props.$token.colorBgContainer};
+ }
+ }
+`;
+export { StyledEditor, StyledToolbar };
diff --git a/frontend/src/components/editor/toolbar.tsx b/frontend/src/components/editor/toolbar.tsx
new file mode 100644
index 0000000..64a7140
--- /dev/null
+++ b/frontend/src/components/editor/toolbar.tsx
@@ -0,0 +1,109 @@
+import { useSettings } from '@/store/settingStore';
+import { useThemeToken } from '@/theme/hooks';
+
+import { StyledToolbar } from './styles';
+
+const HEADINGS = ['Heading 1', 'Heading 2', 'Heading 3', 'Heading 4', 'Heading 5', 'Heading 6'];
+
+export const formats = [
+ 'align',
+ 'background',
+ 'blockquote',
+ 'bold',
+ 'bullet',
+ 'code',
+ 'code-block',
+ 'color',
+ 'direction',
+ 'font',
+ 'formula',
+ 'header',
+ 'image',
+ 'indent',
+ 'italic',
+ 'link',
+ 'list',
+ 'script',
+ 'size',
+ 'strike',
+ 'table',
+ 'underline',
+ 'video',
+];
+
+type EditorToolbarProps = {
+ id: string;
+ isSimple?: boolean;
+};
+
+export default function Toolbar({ id, isSimple, ...other }: EditorToolbarProps) {
+ const token = useThemeToken();
+ const { themeMode } = useSettings();
+ return (
+
+
+
+
+ {HEADINGS.map((heading, index) => (
+
+ {heading}
+
+ ))}
+ Normal
+
+
+
+
+
+
+
+
+
+
+ {!isSimple && (
+
+
+
+
+ )}
+
+
+
+
+ {!isSimple && }
+ {!isSimple && }
+
+
+ {!isSimple && (
+
+
+
+
+ )}
+
+ {!isSimple && (
+
+
+
+
+ )}
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {!isSimple && }
+
+
+
+
+ );
+}
diff --git a/frontend/src/components/icon/icon-button.tsx b/frontend/src/components/icon/icon-button.tsx
new file mode 100644
index 0000000..8cb7a24
--- /dev/null
+++ b/frontend/src/components/icon/icon-button.tsx
@@ -0,0 +1,19 @@
+import { ButtonProps } from 'antd';
+import { CSSProperties, ReactNode } from 'react';
+
+type Props = {
+ children: ReactNode;
+ className?: string;
+ style?: CSSProperties;
+} & ButtonProps;
+export default function IconButton({ children, className, style, onClick }: Props) {
+ return (
+
+ {children}
+
+ );
+}
diff --git a/frontend/src/components/icon/iconify-icon.tsx b/frontend/src/components/icon/iconify-icon.tsx
new file mode 100644
index 0000000..e6bc075
--- /dev/null
+++ b/frontend/src/components/icon/iconify-icon.tsx
@@ -0,0 +1,23 @@
+import { Icon } from '@iconify/react';
+import styled from 'styled-components';
+
+import type { IconProps } from '@iconify/react';
+
+interface Props extends IconProps {
+ size?: IconProps['width'];
+}
+export default function Iconify({ icon, size = '1em', className = '', ...other }: Props) {
+ return (
+
+
+
+ );
+}
+
+const StyledIconify = styled.div`
+ display: inline-flex;
+ vertical-align: middle;
+ svg {
+ display: inline-block;
+ }
+`;
diff --git a/frontend/src/components/icon/index.ts b/frontend/src/components/icon/index.ts
new file mode 100644
index 0000000..7738a5c
--- /dev/null
+++ b/frontend/src/components/icon/index.ts
@@ -0,0 +1,5 @@
+import IconButton from './icon-button';
+import Iconify from './iconify-icon';
+import SvgIcon from './svg-icon';
+
+export { Iconify, SvgIcon, IconButton };
diff --git a/frontend/src/components/icon/svg-icon.tsx b/frontend/src/components/icon/svg-icon.tsx
new file mode 100644
index 0000000..2b5cfbc
--- /dev/null
+++ b/frontend/src/components/icon/svg-icon.tsx
@@ -0,0 +1,38 @@
+import { CSSProperties } from 'react';
+
+interface SvgIconProps {
+ prefix?: string;
+ icon: string;
+ color?: string;
+ size?: string | number;
+ className?: string;
+ style?: CSSProperties;
+}
+
+export default function SvgIcon({
+ icon,
+ prefix = 'icon',
+ color = 'currentColor',
+ size = '1em',
+ className = '',
+ style = {},
+}: SvgIconProps) {
+ const symbolId = `#${prefix}-${icon}`;
+ const svgStyle: CSSProperties = {
+ verticalAlign: 'middle',
+ width: size,
+ height: size,
+ color,
+ ...style,
+ };
+ return (
+
+
+
+ );
+}
diff --git a/frontend/src/components/loading/circle-loading.tsx b/frontend/src/components/loading/circle-loading.tsx
new file mode 100644
index 0000000..a1dd839
--- /dev/null
+++ b/frontend/src/components/loading/circle-loading.tsx
@@ -0,0 +1,9 @@
+import { Spin } from 'antd';
+
+export function CircleLoading() {
+ return (
+
+
+
+ );
+}
diff --git a/frontend/src/components/loading/index.tsx b/frontend/src/components/loading/index.tsx
new file mode 100644
index 0000000..e1d4954
--- /dev/null
+++ b/frontend/src/components/loading/index.tsx
@@ -0,0 +1,2 @@
+export * from './circle-loading';
+export * from './line-loading';
diff --git a/frontend/src/components/loading/line-loading.tsx b/frontend/src/components/loading/line-loading.tsx
new file mode 100644
index 0000000..9fb2310
--- /dev/null
+++ b/frontend/src/components/loading/line-loading.tsx
@@ -0,0 +1,41 @@
+import { Progress } from 'antd';
+import Color from 'color';
+import { useEffect, useState } from 'react';
+
+import { useThemeToken } from '@/theme/hooks';
+
+export function LineLoading() {
+ const { colorTextBase, colorBgContainerDisabled } = useThemeToken();
+ const [percent, setPercent] = useState(10);
+ const [increasing, setIncreasing] = useState(true);
+
+ useEffect(() => {
+ const interval = setInterval(() => {
+ if (increasing) {
+ setPercent((prevPercent) => prevPercent + 20);
+ if (percent === 100) {
+ setIncreasing(false);
+ }
+ } else {
+ setPercent(0);
+ setIncreasing(true);
+ }
+ }, 50);
+
+ return () => {
+ clearInterval(interval);
+ };
+ }, [percent, increasing]);
+
+ return (
+
+ );
+}
diff --git a/frontend/src/components/locale-picker/index.tsx b/frontend/src/components/locale-picker/index.tsx
new file mode 100644
index 0000000..f126692
--- /dev/null
+++ b/frontend/src/components/locale-picker/index.tsx
@@ -0,0 +1,38 @@
+import { Dropdown } from 'antd';
+
+import useLocale, { LANGUAGE_MAP } from '@/locales/useLocale';
+
+import { IconButton, SvgIcon } from '../icon';
+
+import { LocalEnum } from '#/enum';
+import type { MenuProps } from 'antd';
+
+type Locale = keyof typeof LocalEnum;
+
+/**
+ * Locale Picker
+ */
+export default function LocalePicker() {
+ const { setLocale, locale } = useLocale();
+
+ const localeList: MenuProps['items'] = Object.values(LANGUAGE_MAP).map((item) => {
+ return {
+ key: item.locale,
+ label: item.label,
+ icon: ,
+ };
+ });
+
+ return (
+ setLocale(e.key as Locale) }}
+ >
+
+
+
+
+ );
+}
diff --git a/frontend/src/components/logo/index.tsx b/frontend/src/components/logo/index.tsx
new file mode 100644
index 0000000..2a77381
--- /dev/null
+++ b/frontend/src/components/logo/index.tsx
@@ -0,0 +1,17 @@
+import { NavLink } from 'react-router-dom';
+
+import { useThemeToken } from '@/theme/hooks';
+
+function Logo({ className = '' }: { className?: string }) {
+ const { colorPrimary } = useThemeToken();
+
+ return (
+
+
+ Helper
+
+
+ );
+}
+
+export default Logo;
diff --git a/frontend/src/components/markdown/index.tsx b/frontend/src/components/markdown/index.tsx
new file mode 100644
index 0000000..4065458
--- /dev/null
+++ b/frontend/src/components/markdown/index.tsx
@@ -0,0 +1,28 @@
+import ReactMarkdown from 'react-markdown';
+import { ReactMarkdownOptions } from 'react-markdown/lib/react-markdown';
+// markdown plugins
+import rehypeHighlight from 'rehype-highlight';
+import rehypeRaw from 'rehype-raw';
+import remarkGfm from 'remark-gfm'; // add support for strikethrough, tables, tasklists and URLs directly
+
+import { useSettings } from '@/store/settingStore';
+import { useThemeToken } from '@/theme/hooks';
+import '@/utils/highlight';
+
+import StyledMarkdown from './styles';
+
+type Props = ReactMarkdownOptions;
+export default function Markdown({ children }: Props) {
+ const token = useThemeToken();
+ const { themeMode } = useSettings();
+ return (
+
+
+ {children}
+
+
+ );
+}
diff --git a/frontend/src/components/markdown/styles.ts b/frontend/src/components/markdown/styles.ts
new file mode 100644
index 0000000..32e2505
--- /dev/null
+++ b/frontend/src/components/markdown/styles.ts
@@ -0,0 +1,166 @@
+import { GlobalToken } from 'antd';
+/**
+ * https://styled-components.com/
+ * vscode plugin: https://github.com/styled-components/vscode-styled-components
+ */
+import styled from 'styled-components';
+
+import { ThemeMode } from '#/enum';
+
+type KeyofToken = keyof GlobalToken;
+const getHeadingStyle = (level: 1 | 2 | 3 | 4 | 5, token: GlobalToken) => {
+ const fontSizeHeading: KeyofToken = `fontSizeHeading${level}`;
+ const lineHeightHeading: KeyofToken = `lineHeightHeading${level}`;
+
+ return {
+ margin: 0,
+ color: token.colorTextHeading,
+ fontWeight: 800,
+ fontSize: token[fontSizeHeading],
+ lineHeight: token[lineHeightHeading],
+ };
+};
+const StyledMarkdown = styled.div<{ $token: GlobalToken; $thememode: ThemeMode }>`
+ display: grid;
+ // Text
+ h1 {
+ ${(props) => getHeadingStyle(1, props.$token)};
+ }
+ h2 {
+ ${(props) => getHeadingStyle(2, props.$token)};
+ }
+ h3 {
+ ${(props) => getHeadingStyle(3, props.$token)};
+ }
+ h4 {
+ ${(props) => getHeadingStyle(4, props.$token)};
+ }
+ h5 {
+ ${(props) => getHeadingStyle(5, props.$token)};
+ }
+ a {
+ color: ${(props) => props.$token.colorPrimary};
+ }
+ img {
+ border-radius: 4px;
+ }
+ br {
+ display: grid;
+ content: '';
+ margin-top: 0.75em;
+ }
+
+ // Divider
+ hr {
+ margin: 0;
+ border-width: 1;
+ border-style: solid;
+ }
+
+ // List
+ ul,
+ ol {
+ margin: 0;
+ li {
+ line-height: 2;
+ display: flex;
+ align-items: center;
+ }
+ }
+
+ // Blockquote
+ blockquote {
+ line-height: 1.5;
+ font-size: 1.5em;
+ margin: 40px auto;
+ position: relative;
+ padding: 24px 24px 24px 64px;
+ border-radius: 16px;
+ background-color: #f4f6f8;
+ color: #637381;
+ p,
+ span {
+ margin-bottom: 0;
+ font-size: inherit;
+ font-family: inherit;
+ }
+ &::before {
+ left: 16px;
+ top: -8px;
+ display: block;
+ font-size: 3em;
+ position: absolute;
+ content: '“';
+ }
+ }
+
+ // Code Block
+ pre,
+ pre > code {
+ font-size: 16px;
+ overflow-x: auto;
+ white-space: pre;
+ border-radius: 8px;
+ }
+ code {
+ font-size: 14px;
+ border-radius: 4px;
+ white-space: pre;
+ padding: 0px;
+ background-color: ${(props) =>
+ props.$thememode === ThemeMode.Light ? '#161c24' : '#919eab29'};
+ }
+
+ // Table
+ table {
+ width: 100%;
+ border-collapse: collapse;
+ border: 1px solid #919eab33;
+ th,
+ td {
+ padding: 8px;
+ border: 1px solid #919eab33;
+ }
+ tbody tr:nth-of-type(odd) {
+ background-color: ${(props) =>
+ props.$thememode === ThemeMode.Light ? '#f4f6f8' : '#919eab1f '};
+ }
+ }
+
+ // Checkbox
+ input {
+ margin-right: 10px;
+ &[type='checkbox'] {
+ position: relative;
+ cursor: pointer;
+ &::before {
+ content: '';
+ top: -2px;
+ left: -2px;
+ width: 17px;
+ height: 17px;
+ border-radius: 3px;
+ position: absolute;
+ background-color: #f4f6f8;
+ }
+ &:checked {
+ &::before {
+ background-color: ${(props) => props.$token.colorPrimary};
+ }
+ &::after {
+ content: '';
+ top: 1px;
+ left: 5px;
+ width: 4px;
+ height: 9px;
+ position: absolute;
+ transform: rotate(45deg);
+ border: solid white;
+ border-width: 0 2px 2px 0;
+ }
+ }
+ }
+ }
+`;
+
+export default StyledMarkdown;
diff --git a/frontend/src/components/progress-bar/index.tsx b/frontend/src/components/progress-bar/index.tsx
new file mode 100644
index 0000000..bb52e0c
--- /dev/null
+++ b/frontend/src/components/progress-bar/index.tsx
@@ -0,0 +1,58 @@
+import NProgress from 'nprogress';
+import 'nprogress/nprogress.css';
+import { useEffect, useState } from 'react';
+
+import { usePathname } from '@/router/hooks';
+import { useThemeToken } from '@/theme/hooks';
+
+export default function ProgressBar() {
+ const pathname = usePathname();
+ const { colorPrimary } = useThemeToken();
+
+ const [mounted, setMounted] = useState(false);
+ const [visible, setVisible] = useState(false);
+
+ useEffect(() => {
+ setMounted(true);
+ }, []);
+
+ useEffect(() => {
+ if (!visible) {
+ NProgress.configure({
+ showSpinner: false,
+ });
+ NProgress.start();
+ changeNprogressBar();
+ setVisible(true);
+ }
+
+ if (visible) {
+ NProgress.done();
+ setVisible(false);
+ }
+
+ if (!visible && mounted) {
+ setVisible(false);
+ NProgress.done();
+ }
+
+ return () => {
+ NProgress.done();
+ };
+ // eslint-disable-next-line react-hooks/exhaustive-deps
+ }, [pathname, mounted]);
+
+ const changeNprogressBar = () => {
+ const nprogress = document.getElementById('nprogress');
+ if (nprogress) {
+ const bar: HTMLElement = nprogress.querySelector('.bar')!;
+ const peg: HTMLElement = nprogress.querySelector('.peg')!;
+
+ bar.style.background = colorPrimary;
+ bar.style.boxShadow = `0 0 2px ${colorPrimary}`;
+
+ peg.style.boxShadow = `0 0 10px ${colorPrimary}, 0 0 5px ${colorPrimary}`;
+ }
+ };
+ return null;
+}
diff --git a/frontend/src/components/scroll-progress/index.tsx b/frontend/src/components/scroll-progress/index.tsx
new file mode 100644
index 0000000..05f635d
--- /dev/null
+++ b/frontend/src/components/scroll-progress/index.tsx
@@ -0,0 +1,31 @@
+import { HTMLMotionProps, MotionValue, m, useSpring } from 'framer-motion';
+import { CSSProperties } from 'react';
+
+import { useThemeToken } from '@/theme/hooks';
+
+interface Props extends HTMLMotionProps<'div'> {
+ color?: string;
+ scrollYProgress: MotionValue;
+ height?: number;
+}
+/**
+ * https://www.framer.com/motion/scroll-animations/##spring-smoothing
+ */
+export default function ScrollProgress({ scrollYProgress, height = 4, color, ...other }: Props) {
+ const scaleX = useSpring(scrollYProgress, {
+ stiffness: 100,
+ damping: 30,
+ restDelta: 0.001,
+ });
+
+ const { colorPrimary } = useThemeToken();
+ const backgroundColor = color || colorPrimary;
+
+ const style: CSSProperties = {
+ transformOrigin: '0%',
+ height,
+ backgroundColor,
+ };
+
+ return ;
+}
diff --git a/frontend/src/components/scrollbar/index.tsx b/frontend/src/components/scrollbar/index.tsx
new file mode 100644
index 0000000..985f070
--- /dev/null
+++ b/frontend/src/components/scrollbar/index.tsx
@@ -0,0 +1,14 @@
+import { forwardRef, memo } from 'react';
+import SimpleBar, { type Props as SimplebarProps } from 'simplebar-react';
+/**
+ * https://www.npmjs.com/package/simplebar-react?activeTab=readme
+ */
+const Scrollbar = forwardRef(({ children, ...other }, ref) => {
+ return (
+
+ {children}
+
+ );
+});
+
+export default memo(Scrollbar);
diff --git a/frontend/src/components/upload/index.ts b/frontend/src/components/upload/index.ts
new file mode 100644
index 0000000..4956048
--- /dev/null
+++ b/frontend/src/components/upload/index.ts
@@ -0,0 +1,3 @@
+export * from './upload';
+export * from './upload-avatar';
+export * from './upload-box';
diff --git a/frontend/src/components/upload/styles.ts b/frontend/src/components/upload/styles.ts
new file mode 100644
index 0000000..3e17fe9
--- /dev/null
+++ b/frontend/src/components/upload/styles.ts
@@ -0,0 +1,28 @@
+import styled from 'styled-components';
+
+export const StyledUpload = styled.div<{ $thumbnail?: boolean }>`
+ .ant-upload {
+ border: none !important;
+ }
+ .ant-upload-list {
+ display: ${(props) => (props.$thumbnail ? 'flex' : 'block')};
+ flex-wrap: wrap;
+ }
+`;
+
+export const StyledUploadAvatar = styled.div`
+ transition: opacity 200ms cubic-bezier(0.4, 0, 0.2, 1) 0ms;
+ .ant-upload,
+ .ant-upload-select {
+ border: none !important;
+ }
+`;
+
+export const StyledUploadBox = styled.div`
+ .ant-upload {
+ border: none !important;
+ }
+ .ant-upload-list {
+ display: none;
+ }
+`;
diff --git a/frontend/src/components/upload/upload-avatar.tsx b/frontend/src/components/upload/upload-avatar.tsx
new file mode 100644
index 0000000..af2acdc
--- /dev/null
+++ b/frontend/src/components/upload/upload-avatar.tsx
@@ -0,0 +1,84 @@
+import { Typography, Upload } from 'antd';
+import { UploadChangeParam, UploadFile, UploadProps } from 'antd/es/upload';
+import { useState } from 'react';
+
+import { fBytes } from '@/utils/format-number';
+
+import { Iconify } from '../icon';
+
+import { StyledUploadAvatar } from './styles';
+import { beforeAvatarUpload, getBlobUrl } from './utils';
+
+interface Props extends UploadProps {
+ defaultAvatar?: string;
+ helperText?: React.ReactElement | string;
+}
+export function UploadAvatar({ helperText, defaultAvatar = '', ...other }: Props) {
+ const [imageUrl, setImageUrl] = useState(defaultAvatar);
+
+ const [isHover, setIsHover] = useState(false);
+ const handelHover = (hover: boolean) => {
+ setIsHover(hover);
+ };
+
+ const handleChange: UploadProps['onChange'] = (info: UploadChangeParam) => {
+ if (info.file.status === 'uploading') {
+ return;
+ }
+ if (['done', 'error'].includes(info.file.status!)) {
+ // TODO: Get this url from response in real world.
+ setImageUrl(getBlobUrl(info.file.originFileObj!));
+ }
+ };
+
+ const renderPreview = ;
+
+ const renderPlaceholder = (
+
+ );
+
+ const renderContent = (
+ handelHover(true)}
+ onMouseLeave={() => handelHover(false)}
+ >
+ {imageUrl ? renderPreview : null}
+ {!imageUrl || isHover ? renderPlaceholder : null}
+
+ );
+
+ const defaultHelperText = (
+
+ Allowed *.jpeg, *.jpg, *.png, *.gif
+ max size of {fBytes(3145728)}
+
+ );
+ const renderHelpText = {helperText || defaultHelperText}
;
+
+ return (
+
+
+ {renderContent}
+
+ {renderHelpText}
+
+ );
+}
diff --git a/frontend/src/components/upload/upload-box.tsx b/frontend/src/components/upload/upload-box.tsx
new file mode 100644
index 0000000..10d54ca
--- /dev/null
+++ b/frontend/src/components/upload/upload-box.tsx
@@ -0,0 +1,26 @@
+import { UploadProps } from 'antd';
+import Dragger from 'antd/es/upload/Dragger';
+import { ReactElement } from 'react';
+
+import { Iconify } from '../icon';
+
+import { StyledUploadBox } from './styles';
+
+interface Props extends UploadProps {
+ placeholder?: ReactElement;
+}
+export function UploadBox({ placeholder, ...other }: Props) {
+ return (
+
+
+
+ {placeholder || (
+
+
+
+ )}
+
+
+
+ );
+}
diff --git a/frontend/src/components/upload/upload-illustration.tsx b/frontend/src/components/upload/upload-illustration.tsx
new file mode 100644
index 0000000..7204773
--- /dev/null
+++ b/frontend/src/components/upload/upload-illustration.tsx
@@ -0,0 +1,529 @@
+import { useThemeToken } from '@/theme/hooks';
+
+export default function UploadIllustration() {
+ const {
+ colorPrimary: PRIMARY_MAIN,
+ colorPrimaryActive: PRIMARY_DARK,
+ colorPrimaryTextActive: PRIMARY_DARKER,
+ } = useThemeToken();
+ return (
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ );
+}
diff --git a/frontend/src/components/upload/upload-list-item.tsx b/frontend/src/components/upload/upload-list-item.tsx
new file mode 100644
index 0000000..7c59322
--- /dev/null
+++ b/frontend/src/components/upload/upload-list-item.tsx
@@ -0,0 +1,79 @@
+import { Card, Tooltip, Typography, Image } from 'antd';
+import { ItemRender } from 'antd/es/upload/interface';
+import { m } from 'framer-motion';
+import { useEffect, useState } from 'react';
+
+import { varFade } from '@/components/animate/variants';
+import { Iconify, SvgIcon } from '@/components/icon';
+import { fBytes } from '@/utils/format-number';
+
+import { getBlobUrl, getFileFormat, getFileThumb } from './utils';
+
+type Props = {
+ file: Parameters['1'];
+ actions: Parameters['3'];
+ thumbnail?: boolean;
+};
+
+export default function UploadListItem({ file, actions, thumbnail = false }: Props) {
+ const { name, size } = file;
+ const thumb = getFileThumb(name);
+ const format = getFileFormat(name);
+ const [imgThumbUrl, setImgThumbUrl] = useState('');
+
+ useEffect(() => {
+ // TODO: mock upload sucess, you should delete 'error' in the production environment
+ if (['done', 'error'].includes(file.status!) && format === 'img') {
+ setImgThumbUrl(getBlobUrl(file.originFileObj!));
+ }
+ }, [file, format]);
+
+ const closeButton = (
+
+
+
+ );
+
+ const thumbList = (
+
+
+ {format === 'img' ? (
+
+ ) : (
+
+ )}
+
+ {closeButton}
+
+ );
+ const cardList = (
+
+ {format === 'img' ? (
+
+ ) : (
+
+ )}
+
+ {name}
+
+ {fBytes(size)}
+
+
+ {closeButton}
+
+ );
+ return (
+
+ {thumbnail ? thumbList : cardList}
+
+ );
+}
diff --git a/frontend/src/components/upload/upload.tsx b/frontend/src/components/upload/upload.tsx
new file mode 100644
index 0000000..86a1ec3
--- /dev/null
+++ b/frontend/src/components/upload/upload.tsx
@@ -0,0 +1,51 @@
+import { Typography, Upload as AntdUpload } from 'antd';
+import { ItemRender } from 'antd/es/upload/interface';
+
+import { useThemeToken } from '@/theme/hooks';
+
+import { StyledUpload } from './styles';
+import UploadIllustration from './upload-illustration';
+import UploadListItem from './upload-list-item';
+
+import type { UploadProps } from 'antd';
+
+const { Dragger } = AntdUpload;
+const { Text, Title } = Typography;
+
+interface Props extends UploadProps {
+ thumbnail?: boolean;
+}
+
+const itemRender: (thumbnail: boolean) => ItemRender = (thumbnail) => {
+ return function temp() {
+ // eslint-disable-next-line prefer-rest-params
+ const [, file, , actions] = arguments;
+ return ;
+ };
+};
+export function Upload({ thumbnail = false, ...other }: Props) {
+ const { colorPrimary } = useThemeToken();
+ return (
+
+
+
+
+
+
+
+
+ Drop or Select file
+
+
+ Drop files here or click
+
+ browse
+
+ thorough your machine
+
+
+
+
+
+ );
+}
diff --git a/frontend/src/components/upload/utils.ts b/frontend/src/components/upload/utils.ts
new file mode 100644
index 0000000..b917cd2
--- /dev/null
+++ b/frontend/src/components/upload/utils.ts
@@ -0,0 +1,139 @@
+import { message } from 'antd';
+import { RcFile } from 'antd/es/upload';
+
+// Define more types here
+const FORMAT_PDF = ['pdf'];
+const FORMAT_TEXT = ['txt'];
+const FORMAT_PHOTOSHOP = ['psd'];
+const FORMAT_WORD = ['doc', 'docx'];
+const FORMAT_EXCEL = ['xls', 'xlsx'];
+const FORMAT_ZIP = ['zip', 'rar', 'iso'];
+const FORMAT_ILLUSTRATOR = ['ai', 'esp'];
+const FORMAT_POWERPOINT = ['ppt', 'pptx'];
+const FORMAT_AUDIO = ['wav', 'aif', 'mp3', 'aac'];
+const FORMAT_IMG = ['jpg', 'jpeg', 'gif', 'bmp', 'png', 'svg'];
+const FORMAT_VIDEO = ['m4v', 'avi', 'mpg', 'mp4', 'webm'];
+
+/**
+ * 获取文件格式
+ * @param fileName
+ */
+export function getFileFormat(fileName: string | undefined) {
+ let format;
+ switch (true) {
+ case FORMAT_PDF.includes(fileTypeByName(fileName)):
+ format = 'pdf';
+ break;
+ case FORMAT_TEXT.includes(fileTypeByName(fileName)):
+ format = 'txt';
+ break;
+ case FORMAT_PHOTOSHOP.includes(fileTypeByName(fileName)):
+ format = 'psd';
+ break;
+ case FORMAT_WORD.includes(fileTypeByName(fileName)):
+ format = 'word';
+ break;
+ case FORMAT_EXCEL.includes(fileTypeByName(fileName)):
+ format = 'excel';
+ break;
+ case FORMAT_ZIP.includes(fileTypeByName(fileName)):
+ format = 'zip';
+ break;
+ case FORMAT_ILLUSTRATOR.includes(fileTypeByName(fileName)):
+ format = 'ai';
+ break;
+ case FORMAT_POWERPOINT.includes(fileTypeByName(fileName)):
+ format = 'ppt';
+ break;
+ case FORMAT_AUDIO.includes(fileTypeByName(fileName)):
+ format = 'audio';
+ break;
+ case FORMAT_IMG.includes(fileTypeByName(fileName)):
+ format = 'img';
+ break;
+ case FORMAT_VIDEO.includes(fileTypeByName(fileName)):
+ format = 'video';
+ break;
+ default:
+ format = fileTypeByName(fileName);
+ }
+ return format;
+}
+
+/**
+ * 获取文件缩略图
+ * @param fileName
+ */
+export function getFileThumb(fileName: string | undefined) {
+ let thumb;
+ const format = getFileFormat(fileName);
+ switch (format) {
+ case 'txt':
+ thumb = 'ic_file_txt';
+ break;
+ case 'zip':
+ thumb = 'ic_file_zip';
+ break;
+ case 'audio':
+ thumb = 'ic_file_audio';
+ break;
+ case 'video':
+ thumb = 'ic_file_video';
+ break;
+ case 'word':
+ thumb = 'ic_file_word';
+ break;
+ case 'excel':
+ thumb = 'ic_file_excel';
+ break;
+ case 'ppt':
+ thumb = 'ic_file_ppt';
+ break;
+ case 'pdf':
+ thumb = 'ic_file_pdf';
+ break;
+ case 'psd':
+ thumb = 'ic_file_psd';
+ break;
+ case 'ai':
+ thumb = 'ic_file_ai';
+ break;
+ case 'img':
+ thumb = 'ic_file_img';
+ break;
+ case 'folder':
+ thumb = 'ic_folder';
+ break;
+ default:
+ thumb = 'ic_file';
+ }
+ return thumb;
+}
+
+export function fileTypeByName(fileName = '') {
+ return (fileName && fileName.split('.').pop()) || 'folder';
+}
+
+export function beforeAvatarUpload(file: RcFile) {
+ const isJpgOrPng = file.type === 'image/jpeg' || file.type === 'image/png';
+ if (!isJpgOrPng) {
+ message.error('You can only upload JPG/PNG file!');
+ }
+ const isLt2M = file.size / 1024 / 1024 < 2;
+ if (!isLt2M) {
+ message.error('Image must smaller than 2MB!');
+ }
+ return isJpgOrPng && isLt2M;
+}
+
+export function getBase64(img: RcFile, callback: (url: string) => void) {
+ const reader = new FileReader();
+ reader.addEventListener('load', () => callback(reader.result as string));
+ reader.readAsDataURL(img);
+}
+
+export function getBlobUrl(imgFile: RcFile) {
+ const fileBlob = new Blob([imgFile]);
+ const thumbnailUrl = URL.createObjectURL(fileBlob);
+ return thumbnailUrl;
+}
diff --git a/frontend/src/hooks/event/use-copy-to-clipboard.ts b/frontend/src/hooks/event/use-copy-to-clipboard.ts
new file mode 100644
index 0000000..648052c
--- /dev/null
+++ b/frontend/src/hooks/event/use-copy-to-clipboard.ts
@@ -0,0 +1,41 @@
+import { App } from 'antd';
+import { useState } from 'react';
+
+// ----------------------------------------------------------------------
+
+type CopiedValue = string | null;
+
+type CopyFn = (text: string) => Promise;
+
+type ReturnType = {
+ copyFn: CopyFn;
+ copiedText: CopiedValue;
+};
+
+export function useCopyToClipboard(): ReturnType {
+ const [copiedText, setCopiedText] = useState(null);
+ const { notification } = App.useApp();
+
+ const copyFn: CopyFn = async (text) => {
+ if (!navigator?.clipboard) {
+ console.warn('Clipboard not supported');
+ return false;
+ }
+
+ // Try to save to clipboard then save it in the state if worked
+ try {
+ await navigator.clipboard.writeText(text);
+ setCopiedText(text);
+ notification.success({
+ message: 'Copied!',
+ });
+ return true;
+ } catch (error) {
+ console.warn('Copy failed', error);
+ setCopiedText(null);
+ return false;
+ }
+ };
+
+ return { copiedText, copyFn };
+}
diff --git a/frontend/src/hooks/web/use-keepalive.ts b/frontend/src/hooks/web/use-keepalive.ts
new file mode 100644
index 0000000..481a811
--- /dev/null
+++ b/frontend/src/hooks/web/use-keepalive.ts
@@ -0,0 +1,135 @@
+import { useCallback, useEffect, useState } from 'react';
+
+import { useMatchRouteMeta, useRouter } from '@/router/hooks';
+
+import { RouteMeta } from '#/router';
+
+export type KeepAliveTab = RouteMeta & {
+ children: any;
+};
+export default function useKeepAlive() {
+ const { VITE_APP_HOMEPAGE: HOMEPAGE } = import.meta.env;
+ const { push } = useRouter();
+ // tabs
+ const [tabs, setTabs] = useState([]);
+
+ // active tab
+ const [activeTabRoutePath, setActiveTabRoutePath] = useState();
+
+ // current route meta
+ const currentRouteMeta = useMatchRouteMeta();
+
+ /**
+ * Close specified tab
+ */
+ const closeTab = useCallback(
+ (path = activeTabRoutePath) => {
+ if (tabs.length === 1) return;
+ const deleteTabIndex = tabs.findIndex((item) => item.key === path);
+ if (deleteTabIndex > 0) {
+ push(tabs[deleteTabIndex - 1].key);
+ } else {
+ push(tabs[deleteTabIndex + 1].key);
+ }
+
+ tabs.splice(deleteTabIndex, 1);
+ setTabs([...tabs]);
+ },
+ // eslint-disable-next-line react-hooks/exhaustive-deps
+ [activeTabRoutePath],
+ );
+
+ /**
+ * Close other tabs besides the specified tab
+ */
+ const closeOthersTab = useCallback(
+ (path = activeTabRoutePath) => {
+ setTabs((prev) => prev.filter((item) => item.key === path));
+ },
+ [activeTabRoutePath],
+ );
+
+ /**
+ * Close all tabs then navigate to the home page
+ */
+ const closeAll = useCallback(() => {
+ // setTabs([tabHomePage]);
+ setTabs([]);
+ push(HOMEPAGE);
+ // eslint-disable-next-line react-hooks/exhaustive-deps
+ }, [push]);
+
+ /**
+ * Close all tabs in the left of specified tab
+ */
+ const closeLeft = useCallback(
+ (path: string) => {
+ const currentTabIndex = tabs.findIndex((item) => item.key === path);
+ const newTabs = tabs.slice(currentTabIndex);
+ setTabs(newTabs);
+ push(path);
+ },
+ [push, tabs],
+ );
+
+ /**
+ * Close all tabs in the right of specified tab
+ */
+ const closeRight = useCallback(
+ (path: string) => {
+ const currentTabIndex = tabs.findIndex((item) => item.key === path);
+ const newTabs = tabs.slice(0, currentTabIndex + 1);
+ setTabs(newTabs);
+ push(path);
+ },
+ [push, tabs],
+ );
+
+ /**
+ * Refresh specified tab
+ */
+ const refreshTab = useCallback(
+ (path = activeTabRoutePath) => {
+ setTabs((prev) => {
+ const index = prev.findIndex((item) => item.key === path);
+
+ if (index >= 0) {
+ prev[index].timeStamp = getKey();
+ }
+
+ return [...prev];
+ });
+ },
+ [activeTabRoutePath],
+ );
+
+ useEffect(() => {
+ if (!currentRouteMeta) return;
+ const existed = tabs.find((item) => item.key === currentRouteMeta.key);
+ if (!existed) {
+ setTabs((prev) => [
+ ...prev,
+ { ...currentRouteMeta, children: currentRouteMeta.outlet, timeStamp: getKey() },
+ ]);
+ }
+
+ setActiveTabRoutePath(currentRouteMeta.key);
+ // eslint-disable-next-line react-hooks/exhaustive-deps
+ }, [currentRouteMeta]);
+
+ return {
+ tabs,
+ activeTabRoutePath,
+ setTabs,
+ closeTab,
+ closeOthersTab,
+ refreshTab,
+ closeAll,
+ closeLeft,
+ closeRight,
+ };
+}
+
+function getKey() {
+ return new Date().getTime().toString();
+}
diff --git a/frontend/src/layouts/_common/account-dropdown.tsx b/frontend/src/layouts/_common/account-dropdown.tsx
new file mode 100644
index 0000000..0b45721
--- /dev/null
+++ b/frontend/src/layouts/_common/account-dropdown.tsx
@@ -0,0 +1,85 @@
+import { Divider, MenuProps } from 'antd';
+import Dropdown, { DropdownProps } from 'antd/es/dropdown/dropdown';
+import React from 'react';
+import { useTranslation } from 'react-i18next';
+import { NavLink } from 'react-router-dom';
+
+import { IconButton } from '@/components/icon';
+import { useLoginStateContext } from '@/pages/sys/login/providers/LoginStateProvider';
+import { useRouter } from '@/router/hooks';
+import { useUserInfo, useUserActions } from '@/store/userStore';
+import { useThemeToken } from '@/theme/hooks';
+import avatar from '@/assets/images/qinshihuang.jpg';
+
+const { VITE_APP_HOMEPAGE: HOMEPAGE } = import.meta.env;
+
+/**
+ * Account Dropdown
+ */
+export default function AccountDropdown() {
+ const { replace } = useRouter();
+ const { username, email } = useUserInfo();
+ const { clearUserInfoAndToken } = useUserActions();
+ const { backToLogin } = useLoginStateContext();
+ const { t } = useTranslation();
+ const logout = () => {
+ try {
+ // todo const logoutMutation = useMutation(userService.logout);
+ // todo logoutMutation.mutateAsync();
+ clearUserInfoAndToken();
+ backToLogin();
+ } catch (error) {
+ console.log(error);
+ } finally {
+ replace('/login');
+ }
+ };
+ const { colorBgElevated, borderRadiusLG, boxShadowSecondary } = useThemeToken();
+
+ const contentStyle: React.CSSProperties = {
+ backgroundColor: colorBgElevated,
+ borderRadius: borderRadiusLG,
+ boxShadow: boxShadowSecondary,
+ };
+
+ const menuStyle: React.CSSProperties = {
+ boxShadow: 'none',
+ };
+
+ const dropdownRender: DropdownProps['dropdownRender'] = (menu) => (
+
+
+
+ {React.cloneElement(menu as React.ReactElement, { style: menuStyle })}
+
+ );
+
+ const items: MenuProps['items'] = [
+ { label: {t('sys.menu.dashboard')} , key: '0' },
+ {
+ label: {t('sys.menu.user.profile')} ,
+ key: '1',
+ },
+ {
+ label: {t('sys.menu.user.account')} ,
+ key: '2',
+ },
+ { type: 'divider' },
+ {
+ label: {t('sys.login.logout')} ,
+ key: '3',
+ onClick: logout,
+ },
+ ];
+
+ return (
+
+
+
+
+
+ );
+}
diff --git a/frontend/src/layouts/_common/bread-crumb.tsx b/frontend/src/layouts/_common/bread-crumb.tsx
new file mode 100644
index 0000000..c0d8ab5
--- /dev/null
+++ b/frontend/src/layouts/_common/bread-crumb.tsx
@@ -0,0 +1,53 @@
+import { Breadcrumb } from 'antd';
+import { ItemType } from 'antd/es/breadcrumb/Breadcrumb';
+import { useEffect, useState } from 'react';
+import { useTranslation } from 'react-i18next';
+import { useMatches, Link } from 'react-router-dom';
+
+import { useFlattenedRoutes, usePermissionRoutes } from '@/router/hooks';
+import { menuFilter } from '@/router/utils';
+
+import { AppRouteObject } from '#/router';
+
+/**
+ * 动态面包屑解决方案:https://github.com/MinjieChang/myblog/issues/29
+ */
+export default function BreadCrumb() {
+ const { t } = useTranslation();
+ const matches = useMatches();
+ const [breadCrumbs, setBreadCrumbs] = useState([]);
+
+ const flattenedRoutes = useFlattenedRoutes();
+ const permissionRoutes = usePermissionRoutes();
+
+ useEffect(() => {
+ const menuRoutes = menuFilter(permissionRoutes);
+ const paths = matches.filter((item) => item.pathname !== '/').map((item) => item.pathname);
+
+ const pathRouteMetas = flattenedRoutes.filter((item) => paths.indexOf(item.key) !== -1);
+
+ let items: AppRouteObject[] | undefined = [...menuRoutes];
+ const breadCrumbs = pathRouteMetas.map((routeMeta) => {
+ const { key, label } = routeMeta;
+ items = items!
+ .find((item) => item.meta?.key === key)
+ ?.children?.filter((item) => !item.meta?.hideMenu);
+ const result: ItemType = {
+ key,
+ title: t(label),
+ };
+ if (items) {
+ result.menu = {
+ items: items.map((item) => ({
+ key: item.meta?.key,
+ label: {t(item.meta!.label)},
+ })),
+ };
+ }
+ return result;
+ });
+ setBreadCrumbs(breadCrumbs);
+ }, [matches, flattenedRoutes, t, permissionRoutes]);
+
+ return ;
+}
diff --git a/frontend/src/layouts/_common/header-simple.tsx b/frontend/src/layouts/_common/header-simple.tsx
new file mode 100644
index 0000000..512cbfd
--- /dev/null
+++ b/frontend/src/layouts/_common/header-simple.tsx
@@ -0,0 +1,12 @@
+import Logo from '@/components/logo';
+
+import SettingButton from './setting-button';
+
+export default function HeaderSimple() {
+ return (
+
+ );
+}
diff --git a/frontend/src/layouts/_common/pandora-usage.tsx b/frontend/src/layouts/_common/pandora-usage.tsx
new file mode 100644
index 0000000..20efe63
--- /dev/null
+++ b/frontend/src/layouts/_common/pandora-usage.tsx
@@ -0,0 +1,25 @@
+import {Progress, Tooltip} from "antd";
+import {useQuery} from "@tanstack/react-query";
+import sysService from "@/api/services/sysService.ts";
+import dayjs from "dayjs";
+
+const PandoraUsage = () => {
+
+ const {data} = useQuery({
+ queryKey: ['pandora-usage'],
+ queryFn: sysService.getPandoraUsage,
+ staleTime: 1000 * 30, // 30s
+ })
+
+ return (
+
+ );
+};
+
+export default PandoraUsage;
diff --git a/frontend/src/layouts/_common/search-bar.tsx b/frontend/src/layouts/_common/search-bar.tsx
new file mode 100644
index 0000000..53eaf86
--- /dev/null
+++ b/frontend/src/layouts/_common/search-bar.tsx
@@ -0,0 +1,248 @@
+import { Empty, GlobalToken, Input, InputRef, Modal } from 'antd';
+import match from 'autosuggest-highlight/match';
+import parse from 'autosuggest-highlight/parse';
+import Color from 'color';
+import { CSSProperties, useEffect, useRef, useState } from 'react';
+import { useTranslation } from 'react-i18next';
+import { useBoolean, useEvent, useKeyPressEvent } from 'react-use';
+import styled from 'styled-components';
+
+import { IconButton, SvgIcon } from '@/components/icon';
+import Scrollbar from '@/components/scrollbar';
+import { useFlattenedRoutes, useRouter } from '@/router/hooks';
+import ProTag from '@/theme/antd/components/tag';
+import { useThemeToken } from '@/theme/hooks';
+
+export default function SearchBar() {
+ const { t } = useTranslation();
+ const { replace } = useRouter();
+ const inputRef = useRef(null);
+ const listRef = useRef(null);
+
+ const [search, toggle] = useBoolean(false);
+ const themeToken = useThemeToken();
+
+ const flattenedRoutes = useFlattenedRoutes();
+
+ const activeStyle: CSSProperties = {
+ border: `1px dashed ${themeToken.colorPrimary}`,
+ backgroundColor: `${Color(themeToken.colorPrimary).alpha(0.2).toString()}`,
+ };
+
+ const [searchQuery, setSearchQuery] = useState('');
+ const [searchResult, setSearchResult] = useState(flattenedRoutes);
+ const [selectedItemIndex, setSelectedItemIndex] = useState(0);
+
+ useEffect(() => {
+ const result = flattenedRoutes.filter(
+ (item) =>
+ t(item.label).toLowerCase().indexOf(searchQuery.toLowerCase()) !== -1 ||
+ item.key.toLowerCase().indexOf(searchQuery.toLowerCase()) !== -1,
+ );
+ setSearchResult(result);
+ setSelectedItemIndex(0);
+ }, [searchQuery, t, flattenedRoutes]);
+
+ const handleMetaK = (event: KeyboardEvent) => {
+ if (event.metaKey && event.key === 'k') {
+ // https://developer.mozilla.org/zh-CN/docs/Web/API/KeyboardEvent/metaKey
+ handleOpen();
+ }
+ };
+ useEvent('keydown', handleMetaK);
+
+ useKeyPressEvent('ArrowUp', (event) => {
+ if (!search) return;
+ event.preventDefault();
+ let nextIndex = selectedItemIndex - 1;
+ if (nextIndex < 0) {
+ nextIndex = searchResult.length - 1;
+ }
+ setSelectedItemIndex(nextIndex);
+ scrollSelectedItemIntoView(nextIndex);
+ });
+
+ useKeyPressEvent('ArrowDown', (event) => {
+ if (!search) return;
+ event.preventDefault();
+ let nextIndex = selectedItemIndex + 1;
+ if (nextIndex > searchResult.length - 1) {
+ nextIndex = 0;
+ }
+ setSelectedItemIndex(nextIndex);
+ scrollSelectedItemIntoView(nextIndex);
+ });
+
+ useKeyPressEvent('Enter', (event) => {
+ if (!search || searchResult.length === 0) return;
+ event.preventDefault();
+ const selectItem = searchResult[selectedItemIndex].key;
+ if (selectItem) {
+ handleSelect(selectItem);
+ toggle(false);
+ }
+ });
+
+ useKeyPressEvent('Escape', () => {
+ handleCancel();
+ });
+
+ const handleOpen = () => {
+ toggle(true);
+ setSearchQuery('');
+ setSelectedItemIndex(0);
+ };
+ const handleCancel = () => {
+ toggle(false);
+ };
+ const handleAfterOpenChange = (open: boolean) => {
+ if (open) {
+ // auto focus
+ inputRef.current?.focus();
+ }
+ };
+
+ const scrollSelectedItemIntoView = (index: number) => {
+ if (listRef.current) {
+ const selectedItem = listRef.current.children[index];
+ selectedItem.scrollIntoView({
+ behavior: 'smooth',
+ block: 'center',
+ });
+ }
+ };
+
+ const handleHover = (index: number) => {
+ if (index === selectedItemIndex) return;
+ setSelectedItemIndex(index);
+ };
+
+ const handleSelect = (key: string) => {
+ replace(key);
+ handleCancel();
+ };
+
+ return (
+ <>
+
+
+
+
+ ⌘K
+
+ setSearchQuery(e.target.value)}
+ placeholder="Search..."
+ bordered={false}
+ autoFocus
+ prefix={ }
+ suffix={
+
+ Esc
+
+ }
+ />
+ }
+ footer={
+
+ }
+ >
+ {searchResult.length === 0 ? (
+
+ ) : (
+
+
+ {searchResult.map(({ key, label }, index) => {
+ const partsTitle = parse(t(label), match(t(label), searchQuery));
+ const partsKey = parse(key, match(key, searchQuery));
+ return (
+
handleSelect(key)}
+ onMouseMove={() => handleHover(index)}
+ >
+
+
+
+ {partsTitle.map((item) => (
+
+ {item.text}
+
+ ))}
+
+
+ {partsKey.map((item) => (
+
+ {item.text}
+
+ ))}
+
+
+
+
+ );
+ })}
+
+
+ )}
+
+ >
+ );
+}
+
+const StyledListItemButton = styled.div<{ $themetoken: GlobalToken }>`
+ display: flex;
+ flex-direction: column;
+ cursor: pointer;
+ width: 100%;
+ padding: 8px 16px;
+ border-radius: 8px;
+ border-bottom: ${(props) => `1px dashed ${props.$themetoken.colorBorder}`};
+ color: ${(props) => `${props.$themetoken.colorTextSecondary}`};
+`;
diff --git a/frontend/src/layouts/_common/setting-button.tsx b/frontend/src/layouts/_common/setting-button.tsx
new file mode 100644
index 0000000..b439948
--- /dev/null
+++ b/frontend/src/layouts/_common/setting-button.tsx
@@ -0,0 +1,404 @@
+import {
+ CloseOutlined,
+ LeftOutlined,
+ QuestionCircleOutlined,
+ RightOutlined,
+} from '@ant-design/icons';
+import { Button, Card, Drawer, Switch, Tooltip } from 'antd';
+import Color from 'color';
+import { m } from 'framer-motion';
+import { CSSProperties, useState } from 'react';
+import { MdCircle } from 'react-icons/md';
+import screenfull from 'screenfull';
+
+import CyanBlur from '@/assets/images/background/cyan-blur.png';
+import RedBlur from '@/assets/images/background/red-blur.png';
+import { varHover } from '@/components/animate/variants/action';
+import { IconButton, SvgIcon } from '@/components/icon';
+import { useSettingActions, useSettings } from '@/store/settingStore';
+import { colorPrimarys } from '@/theme/antd/theme';
+import { useThemeToken } from '@/theme/hooks';
+
+import { ThemeColorPresets, ThemeLayout, ThemeMode } from '#/enum';
+
+/**
+ * App Setting
+ */
+export default function SettingButton() {
+ const [drawerOpen, setDrawerOpen] = useState(false);
+ const { colorPrimary, colorBgBase, colorTextSecondary, colorTextTertiary, colorBgContainer } =
+ useThemeToken();
+
+ const settings = useSettings();
+ const { themeMode, themeColorPresets, themeLayout, themeStretch, breadCrumb, multiTab } =
+ settings;
+ const { setSettings } = useSettingActions();
+
+ const setThemeMode = (themeMode: ThemeMode) => {
+ setSettings({
+ ...settings,
+ themeMode,
+ });
+ };
+
+ const setThemeColorPresets = (themeColorPresets: ThemeColorPresets) => {
+ setSettings({
+ ...settings,
+ themeColorPresets,
+ });
+ };
+
+ const setThemeLayout = (themeLayout: ThemeLayout) => {
+ setSettings({
+ ...settings,
+ themeLayout,
+ });
+ };
+
+ const setThemeStretch = (themeStretch: boolean) => {
+ setSettings({
+ ...settings,
+ themeStretch,
+ });
+ };
+
+ const setBreadCrumn = (checked: boolean) => {
+ setSettings({
+ ...settings,
+ breadCrumb: checked,
+ });
+ };
+
+ const setMultiTab = (checked: boolean) => {
+ setSettings({
+ ...settings,
+ multiTab: checked,
+ });
+ };
+
+ const style: CSSProperties = {
+ backdropFilter: 'blur(20px)',
+ backgroundImage: `url("${CyanBlur}"), url("${RedBlur}")`,
+ backgroundRepeat: 'no-repeat, no-repeat',
+ backgroundColor: Color(colorBgContainer).alpha(0.9).toString(),
+ backgroundPosition: 'right top, left bottom',
+ backgroundSize: '50, 50%',
+ };
+ const bodyStyle: CSSProperties = {
+ padding: 0,
+ };
+
+ const [isFullscreen, setIsFullscreen] = useState(screenfull.isFullscreen);
+ const toggleFullScreen = () => {
+ if (screenfull.isEnabled) {
+ screenfull.toggle();
+ setIsFullscreen(!isFullscreen);
+ }
+ };
+
+ const layoutBackground = (layout: ThemeLayout) =>
+ themeLayout === layout
+ ? `linear-gradient(135deg, ${colorBgBase} 0%, ${colorPrimary} 100%)`
+ : '#919eab';
+
+ return (
+ <>
+
+ setDrawerOpen(true)}
+ >
+
+
+
+
+
+ setDrawerOpen(false)}
+ open={drawerOpen}
+ closable={false}
+ width={280}
+ bodyStyle={bodyStyle}
+ maskStyle={{ backgroundColor: 'transparent' }}
+ style={style}
+ extra={
+ setDrawerOpen(false)} className="h-9 w-9 hover:scale-105">
+
+
+ }
+ footer={
+
+
+ {isFullscreen ? (
+ <>
+
+ Exit FullScreen
+ >
+ ) : (
+ <>
+
+ FullScreen
+ >
+ )}
+
+
+ }
+ >
+
+ {/* theme mode */}
+
+
+ Mode
+
+
+ setThemeMode(ThemeMode.Light)}
+ className="flex h-20 w-full cursor-pointer items-center justify-center"
+ >
+
+
+ setThemeMode(ThemeMode.Dark)}
+ className="flex h-20 w-full cursor-pointer items-center justify-center"
+ >
+
+
+
+
+
+ {/* theme layout */}
+
+
+ Layout
+
+
+
setThemeLayout(ThemeLayout.Vertical)}
+ className="h-14 cursor-pointer"
+ style={{ flexGrow: 1, flexShrink: 0 }}
+ bodyStyle={{
+ padding: 0,
+ display: 'flex',
+ justifyContent: 'center',
+ alignItems: 'center',
+ height: '100%',
+ }}
+ >
+
+
+
+
setThemeLayout(ThemeLayout.Horizontal)}
+ className="h-14 cursor-pointer"
+ style={{ flexGrow: 1, flexShrink: 0 }}
+ bodyStyle={{
+ padding: 0,
+ display: 'flex',
+ flexDirection: 'column',
+ justifyContent: 'center',
+ alignItems: 'center',
+ height: '100%',
+ }}
+ >
+
+
+
+
setThemeLayout(ThemeLayout.Mini)}
+ className="h-14 cursor-pointer"
+ style={{ flexGrow: 1, flexShrink: 0 }}
+ bodyStyle={{
+ padding: 0,
+ display: 'flex',
+ justifyContent: 'center',
+ alignItems: 'center',
+ height: '100%',
+ }}
+ >
+
+
+
+
+
+
+ {/* theme stretch */}
+
+
+ Stretch
+
+
+
+
+
+
setThemeStretch(!themeStretch)}
+ className="flex h-20 w-full cursor-pointer items-center justify-center"
+ bodyStyle={{
+ width: '50%',
+ padding: 0,
+ display: 'flex',
+ justifyContent: 'center',
+ alignItems: 'center',
+ }}
+ >
+ {themeStretch ? (
+
+ ) : (
+
+ )}
+
+
+
+ {/* theme presets */}
+
+
+ Presets
+
+
+ {Object.entries(colorPrimarys).map(([preset, color]) => (
+
setThemeColorPresets(preset as ThemeColorPresets)}
+ >
+
+
+
+
+ ))}
+
+
+
+ {/* Page config */}
+
+
+ Page
+
+
+
+
BreadCrumb
+
setBreadCrumn(checked)}
+ />
+
+
+
Multi Tab
+
setMultiTab(checked)}
+ />
+
+
+
+
+
+ >
+ );
+}
diff --git a/frontend/src/layouts/dashboard/config.ts b/frontend/src/layouts/dashboard/config.ts
new file mode 100644
index 0000000..8001413
--- /dev/null
+++ b/frontend/src/layouts/dashboard/config.ts
@@ -0,0 +1,8 @@
+export const NAV_WIDTH = 260;
+export const NAV_COLLAPSED_WIDTH = 90;
+export const NAV_HORIZONTAL_HEIGHT = 48;
+
+export const HEADER_HEIGHT = 80;
+export const OFFSET_HEADER_HEIGHT = 64;
+
+export const MULTI_TABS_HEIGHT = 32;
diff --git a/frontend/src/layouts/dashboard/header.tsx b/frontend/src/layouts/dashboard/header.tsx
new file mode 100644
index 0000000..77b2e82
--- /dev/null
+++ b/frontend/src/layouts/dashboard/header.tsx
@@ -0,0 +1,99 @@
+import { Drawer } from 'antd';
+import Color from 'color';
+import { CSSProperties, useState } from 'react';
+
+import { IconButton, Iconify, SvgIcon } from '@/components/icon';
+import LocalePicker from '@/components/locale-picker';
+import Logo from '@/components/logo';
+import { useSettings } from '@/store/settingStore';
+import { useResponsive, useThemeToken } from '@/theme/hooks';
+
+import AccountDropdown from '../_common/account-dropdown';
+import BreadCrumb from '../_common/bread-crumb';
+import SearchBar from '../_common/search-bar';
+import SettingButton from '../_common/setting-button';
+
+import { NAV_COLLAPSED_WIDTH, NAV_WIDTH, HEADER_HEIGHT, OFFSET_HEADER_HEIGHT } from './config';
+import Nav from './nav';
+
+import { ThemeLayout } from '#/enum';
+// import PandoraUsage from "@/layouts/_common/pandora-usage.tsx";
+
+type Props = {
+ className?: string;
+ offsetTop?: boolean;
+};
+export default function Header({ className = '', offsetTop = false }: Props) {
+ const [drawerOpen, setDrawerOpen] = useState(false);
+ const { themeLayout, breadCrumb } = useSettings();
+ const { colorBgElevated, colorBorder } = useThemeToken();
+ const { screenMap } = useResponsive();
+
+ const headerStyle: CSSProperties = {
+ position: themeLayout === ThemeLayout.Horizontal ? 'relative' : 'fixed',
+ borderBottom:
+ themeLayout === ThemeLayout.Horizontal
+ ? `1px dashed ${Color(colorBorder).alpha(0.6).toString()}`
+ : '',
+ backgroundColor: Color(colorBgElevated).alpha(1).toString(),
+ };
+
+ if (themeLayout === ThemeLayout.Horizontal) {
+ headerStyle.width = '100vw';
+ } else if (screenMap.md) {
+ headerStyle.right = '0px';
+ headerStyle.left = 'auto';
+ headerStyle.width = `calc(100% - ${
+ themeLayout === ThemeLayout.Vertical ? NAV_WIDTH : NAV_COLLAPSED_WIDTH
+ }px)`;
+ } else {
+ headerStyle.width = '100vw';
+ }
+
+ return (
+ <>
+
+
+
+ {themeLayout !== ThemeLayout.Horizontal ? (
+
setDrawerOpen(true)} className="h-10 w-10 md:hidden">
+
+
+ ) : (
+
+ )}
+
{breadCrumb ? : null}
+
+
+
+ {/*
*/}
+
+
+
window.open('https://github.com/nianhua99/PandoraNext-Helper')}>
+
+
+
+
+
+
+
+ setDrawerOpen(false)}
+ open={drawerOpen}
+ closeIcon={false}
+ headerStyle={{ display: 'none' }}
+ bodyStyle={{ padding: 0, overflow: 'hidden' }}
+ width="auto"
+ >
+ setDrawerOpen(false)} />
+
+ >
+ );
+}
diff --git a/frontend/src/layouts/dashboard/index.tsx b/frontend/src/layouts/dashboard/index.tsx
new file mode 100644
index 0000000..55d530a
--- /dev/null
+++ b/frontend/src/layouts/dashboard/index.tsx
@@ -0,0 +1,103 @@
+import { useScroll } from 'framer-motion';
+import { Suspense, useCallback, useEffect, useRef, useState } from 'react';
+import styled from 'styled-components';
+
+import { CircleLoading } from '@/components/loading';
+import ProgressBar from '@/components/progress-bar';
+import { useSettings } from '@/store/settingStore';
+import { useThemeToken } from '@/theme/hooks';
+
+import Header from './header';
+import Main from './main';
+import Nav from './nav';
+import NavHorizontal from './nav-horizontal';
+
+import { ThemeLayout, ThemeMode } from '#/enum';
+
+function DashboardLayout() {
+ const { colorBgElevated, colorTextBase } = useThemeToken();
+ const { themeLayout, themeMode } = useSettings();
+
+ const mainEl = useRef(null);
+ const { scrollY } = useScroll({ container: mainEl });
+ /**
+ * y轴是否滚动
+ */
+ const [offsetTop, setOffsetTop] = useState(false);
+ const onOffSetTop = useCallback(() => {
+ scrollY.on('change', (scrollHeight) => {
+ if (scrollHeight > 0) {
+ setOffsetTop(true);
+ } else {
+ setOffsetTop(false);
+ }
+ });
+ }, [scrollY]);
+
+ useEffect(() => {
+ onOffSetTop();
+ }, [onOffSetTop]);
+
+ const verticalLayout = (
+ <>
+
+
+
+
+
+ >
+ );
+
+ const horizontalLayout = (
+
+
+
+
+
+ );
+
+ const layout = themeLayout !== ThemeLayout.Horizontal ? verticalLayout : horizontalLayout;
+
+ return (
+
+
+
+
+ }>{layout}
+
+
+ );
+}
+export default DashboardLayout;
+
+const StyleWrapper = styled.div<{ $themeMode?: ThemeMode }>`
+ /* 设置滚动条的整体样式 */
+ ::-webkit-scrollbar {
+ width: 8px; /* 设置滚动条宽度 */
+ }
+
+ /* 设置滚动条轨道的样式 */
+ ::-webkit-scrollbar-track {
+ border-radius: 8px;
+ background: ${(props) => (props.$themeMode === ThemeMode.Dark ? '#2c2c2c' : '#FAFAFA')};
+ }
+
+ /* 设置滚动条滑块的样式 */
+ ::-webkit-scrollbar-thumb {
+ border-radius: 10px;
+ background: ${(props) => (props.$themeMode === ThemeMode.Dark ? '#6b6b6b' : '#C1C1C1')};
+ }
+
+ /* 设置鼠标悬停在滚动条上的样式 */
+ ::-webkit-scrollbar-thumb:hover {
+ background: ${(props) => (props.$themeMode === ThemeMode.Dark ? '#939393' : '##7D7D7D')};
+ }
+`;
diff --git a/frontend/src/layouts/dashboard/main.tsx b/frontend/src/layouts/dashboard/main.tsx
new file mode 100644
index 0000000..f1b52c3
--- /dev/null
+++ b/frontend/src/layouts/dashboard/main.tsx
@@ -0,0 +1,49 @@
+import { Content } from 'antd/es/layout/layout';
+import { CSSProperties, forwardRef } from 'react';
+import { Outlet } from 'react-router-dom';
+
+import { useSettings } from '@/store/settingStore';
+import { useResponsive } from '@/theme/hooks';
+
+import { NAV_WIDTH, NAV_COLLAPSED_WIDTH, HEADER_HEIGHT, MULTI_TABS_HEIGHT } from './config';
+import MultiTabs from './multi-tabs';
+
+import { ThemeLayout } from '#/enum';
+
+type Props = {
+ offsetTop?: boolean;
+};
+const Main = forwardRef(({ offsetTop = false }, ref) => {
+ const { themeStretch, themeLayout, multiTab } = useSettings();
+ const { screenMap } = useResponsive();
+
+ const mainStyle: CSSProperties = {
+ paddingTop: HEADER_HEIGHT + (multiTab ? MULTI_TABS_HEIGHT : 0),
+ transition: 'padding 200ms cubic-bezier(0.4, 0, 0.2, 1) 0ms',
+ width: '100%',
+ };
+ if (themeLayout === ThemeLayout.Horizontal) {
+ mainStyle.width = '100vw';
+ mainStyle.paddingTop = multiTab ? MULTI_TABS_HEIGHT : 0;
+ } else if (screenMap.md) {
+ mainStyle.width = `calc(100% - ${
+ themeLayout === ThemeLayout.Vertical ? NAV_WIDTH : NAV_COLLAPSED_WIDTH
+ })`;
+ } else {
+ mainStyle.width = '100vw';
+ }
+
+ return (
+
+
+ {multiTab ? : }
+
+
+ );
+});
+
+export default Main;
diff --git a/frontend/src/layouts/dashboard/multi-tabs.tsx b/frontend/src/layouts/dashboard/multi-tabs.tsx
new file mode 100644
index 0000000..a2d32ae
--- /dev/null
+++ b/frontend/src/layouts/dashboard/multi-tabs.tsx
@@ -0,0 +1,419 @@
+import { Dropdown, MenuProps, Tabs, TabsProps } from 'antd';
+import Color from 'color';
+import { CSSProperties, useCallback, useEffect, useMemo, useRef, useState } from 'react';
+import { DragDropContext, Draggable, Droppable, OnDragEndResponder } from 'react-beautiful-dnd';
+import { useTranslation } from 'react-i18next';
+import { useToggle, useFullscreen } from 'react-use';
+import styled from 'styled-components';
+
+import { Iconify } from '@/components/icon';
+import useKeepAlive, { KeepAliveTab } from '@/hooks/web/use-keepalive';
+import { useRouter } from '@/router/hooks';
+import { useSettings } from '@/store/settingStore';
+import { useResponsive, useThemeToken } from '@/theme/hooks';
+
+import {
+ NAV_WIDTH,
+ NAV_COLLAPSED_WIDTH,
+ HEADER_HEIGHT,
+ OFFSET_HEADER_HEIGHT,
+ MULTI_TABS_HEIGHT,
+ NAV_HORIZONTAL_HEIGHT,
+} from './config';
+
+import { MultiTabOperation, ThemeLayout } from '#/enum';
+
+type Props = {
+ offsetTop?: boolean;
+};
+export default function MultiTabs({ offsetTop = false }: Props) {
+ const { t } = useTranslation();
+ const { push } = useRouter();
+ const scrollContainer = useRef(null);
+ const [hoveringTabKey, setHoveringTabKey] = useState('');
+ const [openDropdownTabKey, setopenDropdownTabKey] = useState('');
+ const themeToken = useThemeToken();
+
+ const tabContentRef = useRef(null);
+ const [fullScreen, toggleFullScreen] = useToggle(false);
+ useFullscreen(tabContentRef, fullScreen, { onClose: () => toggleFullScreen(false) });
+
+ const {
+ tabs,
+ activeTabRoutePath,
+ setTabs,
+ closeTab,
+ refreshTab,
+ closeOthersTab,
+ closeAll,
+ closeLeft,
+ closeRight,
+ } = useKeepAlive();
+
+ /**
+ * tab dropdown下拉选
+ */
+ const menuItems = useMemo(
+ () => [
+ {
+ label: t(`sys.tab.${MultiTabOperation.FULLSCREEN}`),
+ key: MultiTabOperation.FULLSCREEN,
+ icon: ,
+ },
+ {
+ label: t(`sys.tab.${MultiTabOperation.REFRESH}`),
+ key: MultiTabOperation.REFRESH,
+ icon: ,
+ },
+ {
+ label: t(`sys.tab.${MultiTabOperation.CLOSE}`),
+ key: MultiTabOperation.CLOSE,
+ icon: ,
+ disabled: tabs.length === 1,
+ },
+ {
+ type: 'divider',
+ },
+ {
+ label: t(`sys.tab.${MultiTabOperation.CLOSELEFT}`),
+ key: MultiTabOperation.CLOSELEFT,
+ icon: (
+
+ ),
+ disabled: tabs.findIndex((tab) => tab.key === openDropdownTabKey) === 0,
+ },
+ {
+ label: t(`sys.tab.${MultiTabOperation.CLOSERIGHT}`),
+ key: MultiTabOperation.CLOSERIGHT,
+ icon: ,
+ disabled: tabs.findIndex((tab) => tab.key === openDropdownTabKey) === tabs.length - 1,
+ },
+ {
+ type: 'divider',
+ },
+ {
+ label: t(`sys.tab.${MultiTabOperation.CLOSEOTHERS}`),
+ key: MultiTabOperation.CLOSEOTHERS,
+ icon: ,
+ disabled: tabs.length === 1,
+ },
+ {
+ label: t(`sys.tab.${MultiTabOperation.CLOSEALL}`),
+ key: MultiTabOperation.CLOSEALL,
+ icon: ,
+ },
+ ],
+ [openDropdownTabKey, t, tabs],
+ );
+
+ /**
+ * tab dropdown click
+ */
+ const menuClick = useCallback(
+ (menuInfo: any, tab: KeepAliveTab) => {
+ const { key, domEvent } = menuInfo;
+ domEvent.stopPropagation();
+ switch (key) {
+ case MultiTabOperation.REFRESH:
+ refreshTab(tab.key);
+ break;
+ case MultiTabOperation.CLOSE:
+ closeTab(tab.key);
+ break;
+ case MultiTabOperation.CLOSEOTHERS:
+ closeOthersTab(tab.key);
+ break;
+ case MultiTabOperation.CLOSELEFT:
+ closeLeft(tab.key);
+ break;
+ case MultiTabOperation.CLOSERIGHT:
+ closeRight(tab.key);
+ break;
+ case MultiTabOperation.CLOSEALL:
+ closeAll();
+ break;
+ case MultiTabOperation.FULLSCREEN:
+ toggleFullScreen();
+ break;
+ default:
+ break;
+ }
+ },
+ [refreshTab, closeTab, closeOthersTab, closeLeft, closeRight, closeAll, toggleFullScreen],
+ );
+
+ /**
+ * 当前显示dorpdown的tab
+ */
+ const onOpenChange = (open: boolean, tab: KeepAliveTab) => {
+ if (open) {
+ setopenDropdownTabKey(tab.key);
+ } else {
+ setopenDropdownTabKey('');
+ }
+ };
+
+ /**
+ * tab样式
+ */
+ const calcTabStyle: (tab: KeepAliveTab) => CSSProperties = useCallback(
+ (tab) => {
+ const isActive = tab.key === activeTabRoutePath || tab.key === hoveringTabKey;
+ const result: CSSProperties = {
+ borderRadius: '8px 8px 0 0',
+ borderWidth: '1px',
+ borderStyle: 'solid',
+ borderColor: themeToken.colorBorderSecondary,
+ backgroundColor: themeToken.colorBgLayout,
+ transition:
+ 'color 200ms cubic-bezier(0.4, 0, 0.2, 1) 0ms, background 200ms cubic-bezier(0.4, 0, 0.2, 1) 0ms',
+ };
+
+ if (isActive) {
+ result.backgroundColor = themeToken.colorBgContainer;
+ result.color = themeToken.colorPrimaryText;
+ }
+ return result;
+ },
+ [activeTabRoutePath, hoveringTabKey, themeToken],
+ );
+
+ /**
+ * 渲染单个tab
+ */
+ const renderTabLabel = useCallback(
+ (tab: KeepAliveTab) => {
+ return (
+ menuClick(menuInfo, tab) }}
+ onOpenChange={(open) => onOpenChange(open, tab)}
+ >
+ {
+ if (tab.key === activeTabRoutePath) return;
+ setHoveringTabKey(tab.key);
+ }}
+ onMouseLeave={() => setHoveringTabKey('')}
+ >
+
{t(tab.label)}
+
{
+ e.stopPropagation();
+ closeTab(tab.key);
+ }}
+ style={{
+ visibility:
+ (tab.key !== activeTabRoutePath && tab.key !== hoveringTabKey) ||
+ tabs.length === 1
+ ? 'hidden'
+ : 'visible',
+ }}
+ />
+
+
+ );
+ },
+ [
+ t,
+ menuItems,
+ activeTabRoutePath,
+ hoveringTabKey,
+ tabs.length,
+ menuClick,
+ closeTab,
+ calcTabStyle,
+ ],
+ );
+
+ /**
+ * 所有tab
+ */
+
+ const tabItems = useMemo(() => {
+ return tabs?.map((tab) => ({
+ label: renderTabLabel(tab),
+ key: tab.key,
+ closable: tabs.length > 1, // 保留一个
+ children: (
+
+ {tab.children}
+
+ ),
+ }));
+ }, [tabs, renderTabLabel]);
+
+ /**
+ * 拖拽结束事件
+ */
+ const onDragEnd: OnDragEndResponder = ({ destination, source }) => {
+ // 拖拽到非法非 droppable区域
+ if (!destination) {
+ return;
+ }
+ // 原地放下
+ if (destination.droppableId === source.droppableId && destination.index === source.index) {
+ return;
+ }
+
+ const newTabs = Array.from(tabs);
+ const [movedTab] = newTabs.splice(source.index, 1);
+ newTabs.splice(destination.index, 0, movedTab);
+ setTabs(newTabs);
+ };
+
+ /**
+ * 渲染 tabbar
+ */
+ const { themeLayout } = useSettings();
+ const { colorBorder, colorBgElevated } = useThemeToken();
+ const { screenMap } = useResponsive();
+
+ const multiTabsStyle: CSSProperties = {
+ position: 'fixed',
+ top: offsetTop ? OFFSET_HEADER_HEIGHT - 2 : HEADER_HEIGHT,
+ left: 0,
+ height: MULTI_TABS_HEIGHT,
+ backgroundColor: Color(colorBgElevated).alpha(1).toString(),
+ borderBottom: `1px dashed ${Color(colorBorder).alpha(0.6).toString()}`,
+ transition: 'top 200ms cubic-bezier(0.4, 0, 0.2, 1) 0ms',
+ };
+
+ if (themeLayout === ThemeLayout.Horizontal) {
+ multiTabsStyle.top = HEADER_HEIGHT + NAV_HORIZONTAL_HEIGHT - 2;
+ } else if (screenMap.md) {
+ multiTabsStyle.right = '0px';
+ multiTabsStyle.left = 'auto';
+ multiTabsStyle.width = `calc(100% - ${
+ themeLayout === ThemeLayout.Vertical ? NAV_WIDTH : NAV_COLLAPSED_WIDTH
+ }px`;
+ } else {
+ multiTabsStyle.width = '100vw';
+ }
+ const renderTabBar: TabsProps['renderTabBar'] = () => {
+ return (
+
+
+
+ {(provided) => (
+
+
+ {tabs.map((tab, index) => (
+
push(tab.key)}
+ >
+
+ {(provided) => (
+
+ {renderTabLabel(tab)}
+
+ )}
+
+
+ ))}
+
+ {provided.placeholder}
+
+ )}
+
+
+
+ );
+ };
+
+ /**
+ * 路由变化时,滚动到指定tab
+ */
+ useEffect(() => {
+ if (!scrollContainer || !scrollContainer.current) {
+ return;
+ }
+ const index = tabs.findIndex((tab) => tab.key === activeTabRoutePath);
+ const currentTabElement = scrollContainer.current.querySelector(`#tab-${index}`);
+ if (currentTabElement) {
+ currentTabElement.scrollIntoView({
+ block: 'nearest',
+ behavior: 'smooth',
+ });
+ }
+ }, [activeTabRoutePath, tabs]);
+
+ /**
+ * scrollContainer 监听wheel事件
+ */
+ useEffect(() => {
+ function handleMouseWheel(event: WheelEvent) {
+ event.preventDefault();
+ scrollContainer.current!.scrollLeft += event.deltaY;
+ }
+
+ scrollContainer.current!.addEventListener('mouseenter', () => {
+ scrollContainer.current!.addEventListener('wheel', handleMouseWheel);
+ });
+ scrollContainer.current!.addEventListener('mouseleave', () => {
+ scrollContainer.current!.removeEventListener('wheel', handleMouseWheel);
+ });
+ }, []);
+
+ return (
+
+
+
+ );
+}
+
+const StyledMultiTabs = styled.div`
+ height: 100%;
+ margin-top: 2px;
+ .anticon {
+ margin: 0px !important;
+ }
+ .ant-tabs {
+ height: 100%;
+ .ant-tabs-content {
+ height: 100%;
+ }
+ .ant-tabs-tabpane {
+ height: 100%;
+ & > div {
+ height: 100%;
+ }
+ }
+ }
+
+ /* 隐藏滚动条 */
+ .hide-scrollbar {
+ overflow: scroll;
+ flex-shrink: 0;
+ scrollbar-width: none; /* 隐藏滚动条 Firefox */
+ -ms-overflow-style: none; /* 隐藏滚动条 IE/Edge */
+ }
+
+ .hide-scrollbar::-webkit-scrollbar {
+ display: none; /* 隐藏滚动条 Chrome/Safari/Opera */
+ }
+`;
diff --git a/frontend/src/layouts/dashboard/nav-horizontal.tsx b/frontend/src/layouts/dashboard/nav-horizontal.tsx
new file mode 100644
index 0000000..b07a67a
--- /dev/null
+++ b/frontend/src/layouts/dashboard/nav-horizontal.tsx
@@ -0,0 +1,73 @@
+import { Menu, MenuProps } from 'antd';
+import { ItemType } from 'antd/es/menu/hooks/useItems';
+import { useState, useEffect, CSSProperties } from 'react';
+import { useNavigate, useMatches, useLocation } from 'react-router-dom';
+
+import { useRouteToMenuFn, usePermissionRoutes } from '@/router/hooks';
+import { menuFilter } from '@/router/utils';
+import { useThemeToken } from '@/theme/hooks';
+
+import { NAV_HORIZONTAL_HEIGHT } from './config';
+
+export default function NavHorizontal() {
+ const navigate = useNavigate();
+ const matches = useMatches();
+ const { pathname } = useLocation();
+
+ const { colorBgElevated } = useThemeToken();
+
+ const routeToMenuFn = useRouteToMenuFn();
+ const permissionRoutes = usePermissionRoutes();
+
+ /**
+ * state
+ */
+ const [openKeys, setOpenKeys] = useState([]);
+ const [selectedKeys, setSelectedKeys] = useState(['']);
+ const [menuList, setMenuList] = useState([]);
+
+ useEffect(() => {
+ setSelectedKeys([pathname]);
+ }, [pathname, matches]);
+
+ useEffect(() => {
+ const menuRoutes = menuFilter(permissionRoutes);
+ const menus = routeToMenuFn(menuRoutes);
+ setMenuList(menus);
+ }, [permissionRoutes, routeToMenuFn]);
+
+ /**
+ * events
+ */
+ const onOpenChange: MenuProps['onOpenChange'] = (keys) => {
+ const latestOpenKey = keys.find((key) => openKeys.indexOf(key) === -1);
+ if (latestOpenKey) {
+ setOpenKeys(keys);
+ } else {
+ setOpenKeys([]);
+ }
+ };
+ const onClick: MenuProps['onClick'] = ({ key }) => {
+ navigate(key);
+ };
+
+ const menuStyle: CSSProperties = {
+ background: colorBgElevated,
+ };
+ return (
+
+
+
+ );
+}
diff --git a/frontend/src/layouts/dashboard/nav.tsx b/frontend/src/layouts/dashboard/nav.tsx
new file mode 100644
index 0000000..933bdce
--- /dev/null
+++ b/frontend/src/layouts/dashboard/nav.tsx
@@ -0,0 +1,153 @@
+import { MenuUnfoldOutlined, MenuFoldOutlined } from '@ant-design/icons';
+import { Menu, MenuProps } from 'antd';
+import { ItemType } from 'antd/es/menu/hooks/useItems';
+import Color from 'color';
+import { CSSProperties, useEffect, useState } from 'react';
+import { useLocation, useMatches, useNavigate } from 'react-router-dom';
+
+import Logo from '@/components/logo';
+import Scrollbar from '@/components/scrollbar';
+import { useRouteToMenuFn, usePermissionRoutes } from '@/router/hooks';
+import { menuFilter } from '@/router/utils';
+import { useSettingActions, useSettings } from '@/store/settingStore';
+import { useThemeToken } from '@/theme/hooks';
+
+import { NAV_COLLAPSED_WIDTH, NAV_WIDTH } from './config';
+
+import { ThemeLayout } from '#/enum';
+
+type Props = {
+ closeSideBarDrawer?: () => void;
+};
+export default function Nav(props: Props) {
+ const navigate = useNavigate();
+ const matches = useMatches();
+ const { pathname } = useLocation();
+
+ const { colorTextBase, colorBgElevated, colorBorder } = useThemeToken();
+
+ const settings = useSettings();
+ const { themeLayout } = settings;
+ const { setSettings } = useSettingActions();
+
+ const menuStyle: CSSProperties = {
+ background: colorBgElevated,
+ };
+
+ const routeToMenuFn = useRouteToMenuFn();
+ const permissionRoutes = usePermissionRoutes();
+
+ /**
+ * state
+ */
+ const [collapsed, setCollapsed] = useState(false);
+ const [openKeys, setOpenKeys] = useState([]);
+ const [selectedKeys, setSelectedKeys] = useState(['']);
+ const [menuList, setMenuList] = useState([]);
+ const [menuMode, setMenuMode] = useState('inline');
+
+ useEffect(() => {
+ if (themeLayout === ThemeLayout.Vertical) {
+ const openKeys = matches
+ .filter((match) => match.pathname !== '/')
+ .map((match) => match.pathname);
+ setOpenKeys(openKeys);
+ }
+ setSelectedKeys([pathname]);
+ }, [pathname, matches, collapsed, themeLayout]);
+
+ useEffect(() => {
+ const menuRoutes = menuFilter(permissionRoutes);
+ const menus = routeToMenuFn(menuRoutes);
+ setMenuList(menus);
+ }, [permissionRoutes, routeToMenuFn]);
+
+ useEffect(() => {
+ if (themeLayout === ThemeLayout.Vertical) {
+ setCollapsed(false);
+ setMenuMode('inline');
+ }
+ if (themeLayout === ThemeLayout.Mini) {
+ setCollapsed(true);
+ setMenuMode('inline');
+ }
+ }, [themeLayout]);
+
+ /**
+ * events
+ */
+ const onOpenChange: MenuProps['onOpenChange'] = (keys) => {
+ const latestOpenKey = keys.find((key) => openKeys.indexOf(key) === -1);
+ if (latestOpenKey) {
+ setOpenKeys(keys);
+ } else {
+ setOpenKeys([]);
+ }
+ };
+ const onClick: MenuProps['onClick'] = ({ key }) => {
+ navigate(key);
+ props?.closeSideBarDrawer?.();
+ };
+
+ const setThemeLayout = (themeLayout: ThemeLayout) => {
+ setSettings({
+ ...settings,
+ themeLayout,
+ });
+ };
+
+ const toggleCollapsed = () => {
+ if (!collapsed) {
+ setThemeLayout(ThemeLayout.Mini);
+ } else {
+ setThemeLayout(ThemeLayout.Vertical);
+ }
+ setCollapsed(!collapsed);
+ };
+
+ return (
+
+
+ {themeLayout === ThemeLayout.Mini ? (
+
+ ) : (
+
+ )}
+
+ {collapsed ? : }
+
+
+
+
+ {/* */}
+
+
+
+ );
+}
diff --git a/frontend/src/layouts/simple/index.tsx b/frontend/src/layouts/simple/index.tsx
new file mode 100644
index 0000000..8742b7f
--- /dev/null
+++ b/frontend/src/layouts/simple/index.tsx
@@ -0,0 +1,24 @@
+import React from 'react';
+
+import { useThemeToken } from '@/theme/hooks';
+
+import HeaderSimple from '../_common/header-simple';
+
+type Props = {
+ children: React.ReactNode;
+};
+export default function SimpleLayout({ children }: Props) {
+ const { colorBgElevated, colorTextBase } = useThemeToken();
+ return (
+
+
+ {children}
+
+ );
+}
diff --git a/frontend/src/locales/i18n.ts b/frontend/src/locales/i18n.ts
new file mode 100644
index 0000000..3ea8b06
--- /dev/null
+++ b/frontend/src/locales/i18n.ts
@@ -0,0 +1,35 @@
+import i18n from 'i18next';
+import LanguageDetector from 'i18next-browser-languagedetector';
+import { initReactI18next } from 'react-i18next';
+
+import { getStringItem } from '@/utils/storage';
+
+import en_US from './lang/en_US';
+import zh_CN from './lang/zh_CN';
+
+import { LocalEnum, StorageEnum } from '#/enum';
+
+const defaultLng = getStringItem(StorageEnum.I18N) || (LocalEnum.en_US as string);
+i18n
+ // detect user language
+ // learn more: https://github.com/i18next/i18next-browser-languageDetector
+ .use(LanguageDetector)
+ // pass the i18n instance to react-i18next.
+ .use(initReactI18next)
+ // init i18next
+ // for all options read: https://www.i18next.com/overview/configuration-options
+ .init({
+ debug: true,
+ lng: defaultLng, // localstorage -> i18nextLng: en_US
+ fallbackLng: LocalEnum.en_US,
+ interpolation: {
+ escapeValue: false, // not needed for react as it escapes by default
+ },
+ resources: {
+ en_US: { translation: en_US },
+ zh_CN: { translation: zh_CN },
+ },
+ });
+
+export default i18n;
+export const { t } = i18n;
diff --git a/frontend/src/locales/lang/en_US/common.json b/frontend/src/locales/lang/en_US/common.json
new file mode 100644
index 0000000..3010920
--- /dev/null
+++ b/frontend/src/locales/lang/en_US/common.json
@@ -0,0 +1,32 @@
+{
+ "common": {
+ "okText": "OK",
+ "closeText": "Close",
+ "cancelText": "Cancel",
+ "loadingText": "Loading...",
+ "saveText": "Save",
+ "delText": "Delete",
+ "resetText": "Reset",
+ "searchText": "Search",
+ "queryText": "Search",
+
+ "inputText": "Please enter",
+ "chooseText": "Please choose",
+
+ "redo": "Refresh",
+ "back": "Back",
+
+ "light": "Light",
+ "dark": "Dark",
+ "refresh": "Refresh",
+ "refreshConfirm": "Refresh the Account",
+ "deleteConfirm": "Delete the Account",
+ "welcomeBack": "Welcome back \uD83D\uDC4B",
+ "desc": "Manage your PandoraNext account and token in 1 place.",
+ "goNow": "Go Now",
+
+ "optional": "Optional"
+ },
+ "%namespace%:password": "",
+ "%namespace%:shareStatus": ""
+}
diff --git a/frontend/src/locales/lang/en_US/index.ts b/frontend/src/locales/lang/en_US/index.ts
new file mode 100644
index 0000000..caa565e
--- /dev/null
+++ b/frontend/src/locales/lang/en_US/index.ts
@@ -0,0 +1,9 @@
+import common from './common.json';
+import sys from './sys.json';
+import token from './token.json'
+
+export default {
+ ...common,
+ ...sys,
+ ...token
+};
diff --git a/frontend/src/locales/lang/en_US/sys.json b/frontend/src/locales/lang/en_US/sys.json
new file mode 100644
index 0000000..5c93309
--- /dev/null
+++ b/frontend/src/locales/lang/en_US/sys.json
@@ -0,0 +1,146 @@
+{
+ "sys": {
+ "api": {
+ "operationSuccess": "Operation Success",
+ "operationFailed": "Operation failed",
+ "errorTip": "Error Tip",
+ "successTip": "Success Tip",
+ "errorMessage": "The operation failed, the system is abnormal!",
+ "timeoutMessage": "Login timed out, please log in again!",
+ "apiTimeoutMessage": "The interface request timed out, please refresh the page and try again!",
+ "apiRequestFailed": "The interface request failed, please try again later!",
+ "networkException": "network anomaly",
+ "networkExceptionMsg": "Please check if your network connection is normal! The network is abnormal",
+
+ "errMsg401": "The user does not have permission (token, user name, password error)!",
+ "errMsg403": "The user is authorized, but access is forbidden!",
+ "errMsg404": "Network request error, the resource was not found!",
+ "errMsg405": "Network request error, request method not allowed!",
+ "errMsg408": "Network request timed out!",
+ "errMsg500": "Server error, please contact the administrator!",
+ "errMsg501": "The network is not implemented!",
+ "errMsg502": "Network Error!",
+ "errMsg503": "The service is unavailable, the server is temporarily overloaded or maintained!",
+ "errMsg504": "Network timeout!",
+ "errMsg505": "The http version does not support the request!"
+ },
+ "login": {
+ "backSignIn": "Back sign in",
+ "mobileSignInFormTitle": "Mobile sign in",
+ "qrSignInFormTitle": "Qr code sign in",
+ "signInFormTitle": "Sign in",
+ "signUpFormTitle": "Sign up",
+ "forgetFormTitle": "Reset password",
+
+ "signInPrimaryTitle": "Hi, Welcome Back",
+ "signInSecondTitle": "Backstage management system",
+ "signInTitle": "",
+ "signInDesc": "Enter your personal details and get started!",
+ "policy": "I agree to the xxx Privacy Policy",
+ "scanSign": "scanning the code to complete the login",
+ "forgetFormSecondTitle": "Please enter the email address associated with your account and We will email you a link to reset your password.",
+
+ "loginButton": "Sign in",
+ "registerButton": "Sign up",
+ "rememberMe": "Remember me",
+ "forgetPassword": "Forget Password?",
+ "otherSignIn": "Sign in with",
+ "sendSmsButton": "Send SMS code",
+ "sendSmsText": "Reacquire in {{second}}s",
+ "sendEmailButton": "Send Email",
+
+ "loginSuccessTitle": "Login successful",
+ "loginSuccessDesc": "Welcome back",
+
+ "accountPlaceholder": "Please input username",
+ "passwordPlaceholder": "Please input password",
+ "confirmPasswordPlaceholder": "Please input confirm password",
+ "emaildPlaceholder": "Please input email",
+ "smsPlaceholder": "Please input sms code",
+ "mobilePlaceholder": "Please input mobile",
+ "policyPlaceholder": "Register after checking",
+ "diffPwd": "The two passwords are inconsistent",
+
+ "userName": "Username",
+ "password": "Password",
+ "confirmPassword": "Confirm Password",
+ "email": "Email",
+ "smsCode": "SMS code",
+ "mobile": "Mobile",
+
+ "registerAndAgree": "By signing up, I agree to",
+ "termsOfService": " Terms of service ",
+ "privacyPolicy": " Privacy policy ",
+ "logout": "Logout"
+ },
+ "tab": {
+ "fullscreen": "FullScreen",
+ "refresh": "Refresh",
+ "close": "Close",
+ "closeOthers": "Close Others",
+ "closeAll": "Close All",
+ "closeLeft": "Close Left",
+ "closeRight": "Close Right"
+ },
+ "menu": {
+ "token": "Token",
+ "account": "Account",
+ "share": "Share",
+ "dashboard": "Dashboard",
+ "workbench": "Workbench",
+ "analysis": "Analysis",
+ "management": "Management",
+ "user": {
+ "index": "User",
+ "profile": "Profile",
+ "account": "Account"
+ },
+ "system": {
+ "index": "System",
+ "organization": "Organization",
+ "permission": "Permission",
+ "role": "Role",
+ "user": "User"
+ },
+ "blog": "Blog",
+ "components": "Components",
+ "icon": "Icon",
+ "animate": "Animate",
+ "scroll": "Scroll",
+ "markdown": "Markdown",
+ "editor": "Editor",
+ "i18n": "Multi Language",
+ "upload": "Upload",
+ "chart": "Chart",
+ "functions": "Functions",
+ "clipboard": "Clipboard",
+ "menulevel": {
+ "index": "Menu Level",
+ "1a": "Menu Level 1a",
+ "1b": {
+ "index": "Menu Level 1b",
+ "2a": "Menu Level 2a",
+ "2b": {
+ "index": "Menu Level 2b",
+ "3a": "Menu Level 3a",
+ "3b": "Menu Level 3b"
+ }
+ }
+ },
+ "disabled": "Item Disabled",
+ "label": "Item Label",
+ "frame": "Item External",
+ "external_link": "External Link",
+ "iframe": "Iframe",
+ "blank": "Blank",
+ "calendar": "Calendar",
+ "kanban": "Kanban",
+ "error": {
+ "index": "Error Page",
+ "403": "403",
+ "404": "404",
+ "500": "500"
+ }
+ }
+ }
+}
diff --git a/frontend/src/locales/lang/en_US/token.json b/frontend/src/locales/lang/en_US/token.json
new file mode 100644
index 0000000..fd8badd
--- /dev/null
+++ b/frontend/src/locales/lang/en_US/token.json
@@ -0,0 +1,27 @@
+{
+ "token": {
+ "email": "Email",
+ "password": "Password",
+ "loginStatus": "Login status",
+ "shareStatus": "Share Status",
+ "updateTime": "Update Time",
+ "action": "Action",
+ "share": "Share",
+ "shareList": "Share List",
+ "createNew": "Create New",
+ "edit": "Edit",
+ "accountList": "Account List",
+ "comment": "Comment",
+ "tokenType": "Token Type",
+ "customToken": "Custom Token",
+ "statistic": "Statistic",
+ "queryingInfo": "Querying, this will take a long time...",
+ "search": "Search",
+ "reset": "Reset",
+ "deleteConfirm": "Are you sure?",
+ "stop": "Stop Auto Refresh",
+ "start": "Start Auto Refresh",
+ "copySuccess": "Copy Success",
+ "refreshCron": "Refresh Limit Everyday"
+ }
+}
diff --git a/frontend/src/locales/lang/zh_CN/common.json b/frontend/src/locales/lang/zh_CN/common.json
new file mode 100644
index 0000000..f93c265
--- /dev/null
+++ b/frontend/src/locales/lang/zh_CN/common.json
@@ -0,0 +1,24 @@
+{
+ "common": {
+ "okText": "确认",
+ "closeText": "关闭",
+ "cancelText": "取消",
+ "loadingText": "加载中...",
+ "saveText": "保存",
+ "delText": "删除",
+ "resetText": "重置",
+ "searchText": "搜索",
+ "queryText": "查询",
+
+ "inputText": "请输入",
+ "chooseText": "请选择",
+
+ "redo": "刷新",
+ "back": "返回",
+
+ "light": "亮色主题",
+ "dark": "黑暗主题",
+
+ "optional": "可选"
+ }
+}
diff --git a/frontend/src/locales/lang/zh_CN/index.ts b/frontend/src/locales/lang/zh_CN/index.ts
new file mode 100644
index 0000000..e5c9a34
--- /dev/null
+++ b/frontend/src/locales/lang/zh_CN/index.ts
@@ -0,0 +1,9 @@
+import common from './common.json';
+import sys from './sys.json';
+import token from "./token.json"
+
+export default {
+ ...common,
+ ...sys,
+ ...token
+};
diff --git a/frontend/src/locales/lang/zh_CN/sys.json b/frontend/src/locales/lang/zh_CN/sys.json
new file mode 100644
index 0000000..6c5180f
--- /dev/null
+++ b/frontend/src/locales/lang/zh_CN/sys.json
@@ -0,0 +1,145 @@
+{
+ "sys": {
+ "api": {
+ "operationSuccess": "操作成功",
+ "operationFailed": "操作失败",
+ "errorTip": "错误提示",
+ "successTip": "成功提示",
+ "errorMessage": "操作失败,系统异常!",
+ "timeoutMessage": "登录超时,请重新登录!",
+ "apiTimeoutMessage": "接口请求超时,请刷新页面重试!",
+ "apiRequestFailed": "请求出错,请稍候重试",
+ "networkException": "网络异常",
+ "networkExceptionMsg": "网络异常,请检查您的网络连接是否正常!",
+
+ "errMsg401": "用户没有权限(令牌、用户名、密码错误)!",
+ "errMsg403": "用户得到授权,但是访问是被禁止的。!",
+ "errMsg404": "网络请求错误,未找到该资源!",
+ "errMsg405": "网络请求错误,请求方法未允许!",
+ "errMsg408": "网络请求超时!",
+ "errMsg500": "服务器错误,请联系管理员!",
+ "errMsg501": "网络未实现!",
+ "errMsg502": "网络错误!",
+ "errMsg503": "服务不可用,服务器暂时过载或维护!",
+ "errMsg504": "网络超时!",
+ "errMsg505": "http版本不支持该请求!"
+ },
+ "login": {
+ "backSignIn": "返回",
+ "signInFormTitle": "登录",
+ "mobileSignInFormTitle": "手机登录",
+ "qrSignInFormTitle": "二维码登录",
+ "signUpFormTitle": "注册",
+ "forgetFormTitle": "重置密码",
+
+ "signInPrimaryTitle": "欢迎回来",
+ "signInSecondTitle": "开箱即用的中后台管理系统",
+ "signInDesc": "输入您的个人详细信息开始使用!",
+ "policy": "我同意xxx隐私政策",
+ "scanSign": "扫码后点击'确认',即可完成登录",
+ "forgetFormSecondTitle": "请输入与您的帐户关联的电子邮件地址,我们将通过电子邮件向您发送重置密码的链接。",
+
+ "loginButton": "登录",
+ "registerButton": "注册",
+ "rememberMe": "记住我",
+ "forgetPassword": "忘记密码?",
+ "otherSignIn": "其他登录方式",
+ "sendSmsButton": "发送验证码",
+ "sendSmsText": "{{second}}秒后重新获取",
+ "sendEmailButton": "发送邮件",
+
+ "loginSuccessTitle": "登录成功",
+ "loginSuccessDesc": "欢迎回来",
+
+ "accountPlaceholder": "请输入账号",
+ "passwordPlaceholder": "请输入密码",
+ "confirmPasswordPlaceholder": "请输入确认密码",
+ "emaildPlaceholder": "请输入邮箱",
+ "smsPlaceholder": "请输入验证码",
+ "mobilePlaceholder": "请输入手机号码",
+ "policyPlaceholder": "勾选后才能注册",
+ "diffPwd": "两次输入密码不一致",
+
+ "userName": "账号",
+ "password": "密码",
+ "confirmPassword": "确认密码",
+ "email": "邮箱",
+ "smsCode": "短信验证码",
+ "mobile": "手机号码",
+
+ "registerAndAgree": "注册即我同意",
+ "termsOfService": " 服务条款 ",
+ "privacyPolicy": " 隐私政策 ",
+ "logout": "退出"
+ },
+ "tab": {
+ "fullscreen": "内容全屏",
+ "refresh": "刷新",
+ "close": "关闭标签页",
+ "closeOthers": "关闭其它标签页",
+ "closeAll": "关闭所有标签页",
+ "closeLeft": "关闭左侧标签页",
+ "closeRight": "关闭右侧标签页"
+ },
+ "menu": {
+ "token": "令牌",
+ "account": "账号管理",
+ "share": "分享管理",
+ "dashboard": "仪表",
+ "workbench": "工作台",
+ "analysis": "分析",
+ "management": "管理",
+ "user": {
+ "index": "用户",
+ "profile": "个人资料",
+ "account": "账户"
+ },
+ "system": {
+ "index": "系统",
+ "organization": "组织",
+ "permission": "权限",
+ "role": "角色",
+ "user": "用户"
+ },
+ "blog": "博客",
+ "components": "组件",
+ "icon": "图标",
+ "animate": "动画",
+ "scroll": "滚动",
+ "markdown": "Markdown",
+ "editor": "富文本",
+ "i18n": "多语言",
+ "upload": "上传",
+ "chart": "图表",
+ "functions": "功能",
+ "clipboard": "剪贴板",
+ "menulevel": {
+ "index": "多级菜单",
+ "1a": "多级菜单 1a",
+ "1b": {
+ "index": "多级菜单 1b",
+ "2a": "多级菜单 2a",
+ "2b": {
+ "index": "多级菜单 2b",
+ "3a": "多级菜单 3a",
+ "3b": "多级菜单 3b"
+ }
+ }
+ },
+ "disabled": "项目禁用",
+ "label": "项目标签",
+ "frame": "项目外部链接",
+ "external_link": "外链",
+ "iframe": "内嵌",
+ "blank": "空白",
+ "calendar": "日历",
+ "kanban": "看板",
+ "error": {
+ "index": "异常页",
+ "403": "403",
+ "404": "404",
+ "500": "500"
+ }
+ }
+ }
+}
diff --git a/frontend/src/locales/lang/zh_CN/token.json b/frontend/src/locales/lang/zh_CN/token.json
new file mode 100644
index 0000000..a84d161
--- /dev/null
+++ b/frontend/src/locales/lang/zh_CN/token.json
@@ -0,0 +1,33 @@
+{
+ "token": {
+ "email": "电子邮件",
+ "password": "密码",
+ "loginStatus": "登录状态",
+ "shareStatus": "共享状态",
+ "updateTime": "更新时间",
+ "action": "操作",
+ "share": "共享",
+ "shareList": "共享列表",
+ "createNew": "新建",
+ "edit": "编辑",
+ "accountList": "账号列表",
+ "comment": "备注",
+ "tokenType": "令牌类型",
+ "customToken": "自定义令牌",
+ "statistic": "统计",
+ "queryingInfo": "查询中,这将需要很长时间......",
+ "search": "搜索",
+ "reset": "重置",
+ "deleteConfirm": "确定删除吗",
+ "stop": "停止定时刷新",
+ "start": "启动定时刷新",
+ "copySuccess": "复制成功",
+ "expiresIn": "有效期(秒)",
+ "siteLimit": "站点限制",
+ "gpt35Limit": "GPT-3.5次数",
+ "gpt4Limit": "GPT-4次数",
+ "showUserinfo": "显示用户信息",
+ "showConversations": "会话隔离",
+ "refreshEveryday": "每天重置限额"
+ }
+}
diff --git a/frontend/src/locales/useLocale.ts b/frontend/src/locales/useLocale.ts
new file mode 100644
index 0000000..8abbbf2
--- /dev/null
+++ b/frontend/src/locales/useLocale.ts
@@ -0,0 +1,50 @@
+import en_US from 'antd/locale/en_US';
+import zh_CN from 'antd/locale/zh_CN';
+import { useTranslation } from 'react-i18next';
+
+import { LocalEnum } from '#/enum';
+import type { Locale as AntdLocal } from 'antd/es/locale';
+
+type Locale = keyof typeof LocalEnum;
+type Language = {
+ locale: keyof typeof LocalEnum;
+ icon: string;
+ label: string;
+ antdLocal: AntdLocal;
+};
+
+export const LANGUAGE_MAP: Record = {
+ [LocalEnum.zh_CN]: {
+ locale: LocalEnum.zh_CN,
+ label: 'Chinese',
+ icon: 'ic-locale_zh_CN',
+ antdLocal: zh_CN,
+ },
+ [LocalEnum.en_US]: {
+ locale: LocalEnum.en_US,
+ label: 'English',
+ icon: 'ic-locale_en_US',
+ antdLocal: en_US,
+ },
+};
+
+export default function useLocale() {
+ const { i18n } = useTranslation();
+
+ /**
+ * localstorage -> i18nextLng change
+ */
+ const setLocale = (locale: Locale) => {
+ i18n.changeLanguage(locale);
+ };
+
+ const locale = (i18n.resolvedLanguage || LocalEnum.en_US) as Locale;
+
+ const language = LANGUAGE_MAP[locale];
+
+ return {
+ locale,
+ language,
+ setLocale,
+ };
+}
diff --git a/frontend/src/main.tsx b/frontend/src/main.tsx
new file mode 100644
index 0000000..bf0bd07
--- /dev/null
+++ b/frontend/src/main.tsx
@@ -0,0 +1,53 @@
+// react-query
+import { QueryClient, QueryClientProvider } from '@tanstack/react-query';
+import { ReactQueryDevtools } from '@tanstack/react-query-devtools';
+// react
+import { Suspense } from 'react';
+import ReactDOM from 'react-dom/client';
+// react helmet
+import { HelmetProvider } from 'react-helmet-async';
+// eslint-disable-next-line import/no-unresolved
+import 'virtual:svg-icons-register';
+
+import App from '@/App';
+// i18n
+import './locales/i18n';
+// tailwind css
+import './theme/index.css';
+
+const charAt = `
+ ███████╗██╗ █████╗ ███████╗██╗ ██╗
+ ██╔════╝██║ ██╔══██╗██╔════╝██║ ██║
+ ███████╗██║ ███████║███████╗███████║
+ ╚════██║██║ ██╔══██║╚════██║██╔══██║
+ ███████║███████╗██║ ██║███████║██║ ██║
+ ╚══════╝╚══════╝╚═╝ ╚═╝╚══════╝╚═╝ ╚═╝
+ `;
+console.info(`%c${charAt}`, 'color: #5BE49B');
+
+// 创建一个 client
+const queryClient = new QueryClient({
+ defaultOptions: {
+ queries: {
+ retry: 1, // 失败重试次数
+ cacheTime: 300_000, // 缓存有效期 5m
+ staleTime: 10_1000, // 数据变得 "陈旧"(stale)的时间 10s
+ refetchOnWindowFocus: false, // 禁止窗口聚焦时重新获取数据
+ refetchOnReconnect: false, // 禁止重新连接时重新获取数据
+ refetchOnMount: false, // 禁止组件挂载时重新获取数据
+ },
+ },
+});
+
+const root = ReactDOM.createRoot(document.getElementById('root') as HTMLElement);
+
+root.render(
+
+
+
+
+
+
+
+ ,
+);
diff --git a/frontend/src/pages/components/animate/control-panel.tsx b/frontend/src/pages/components/animate/control-panel.tsx
new file mode 100644
index 0000000..ea34d34
--- /dev/null
+++ b/frontend/src/pages/components/animate/control-panel.tsx
@@ -0,0 +1,45 @@
+import { Card } from 'antd';
+
+import { useThemeToken } from '@/theme/hooks';
+
+type Props = {
+ variantKey: {
+ type: string;
+ values: string[];
+ }[];
+ selectedVariant: string;
+ onChangeVarient: (varient: string) => void;
+};
+export default function ControlPanel({ variantKey, selectedVariant, onChangeVarient }: Props) {
+ const { colorPrimary, colorTextBase } = useThemeToken();
+
+ const selectedStyle = (variantKey: string) => {
+ return variantKey === selectedVariant
+ ? {
+ backgroundColor: colorPrimary,
+ color: colorTextBase,
+ }
+ : {};
+ };
+ return (
+
+ {variantKey.map((item) => (
+
+
{item.type.toUpperCase()}
+
+ {item.values.map((item) => (
+ onChangeVarient(item)}
+ style={selectedStyle(item)}
+ >
+ {item}
+
+ ))}
+
+
+ ))}
+
+ );
+}
diff --git a/frontend/src/pages/components/animate/index.tsx b/frontend/src/pages/components/animate/index.tsx
new file mode 100644
index 0000000..1bdb6e1
--- /dev/null
+++ b/frontend/src/pages/components/animate/index.tsx
@@ -0,0 +1,29 @@
+import { Tabs, TabsProps, Typography } from 'antd';
+
+import { useThemeToken } from '@/theme/hooks';
+
+import BackgroundView from './views/background';
+import Inview from './views/inview';
+import ScrollView from './views/scroll';
+
+export default function AnimatePage() {
+ const { colorPrimary } = useThemeToken();
+ const TABS: TabsProps['items'] = [
+ { key: 'inview', label: 'In View', children: },
+ { key: 'scroll', label: 'Scroll', children: },
+ { key: 'background', label: 'Background', children: },
+ ];
+
+ return (
+ <>
+
+ https://www.framer.com/motion/
+
+
+ >
+ );
+}
diff --git a/frontend/src/pages/components/animate/views/background/container.tsx b/frontend/src/pages/components/animate/views/background/container.tsx
new file mode 100644
index 0000000..1c6ce52
--- /dev/null
+++ b/frontend/src/pages/components/animate/views/background/container.tsx
@@ -0,0 +1,35 @@
+import { m } from 'framer-motion';
+import { useEffect, useMemo } from 'react';
+
+import Cover3 from '@/assets/images/cover/cover_3.jpg';
+import MotionContainer from '@/components/animate/motion-container';
+import { getVariant } from '@/components/animate/variants';
+import { useThemeToken } from '@/theme/hooks';
+
+type Props = {
+ variant: string;
+};
+export default function ContainerView({ variant }: Props) {
+ const { colorBgLayout } = useThemeToken();
+ const varients = useMemo(() => getVariant(variant), [variant]);
+ const isKenburns = variant.includes('kenburns');
+
+ useEffect(() => {
+ console.log(varients);
+ });
+ return (
+
+
+ {isKenburns ? (
+
+ ) : (
+
+ )}
+
+
+ );
+}
diff --git a/frontend/src/pages/components/animate/views/background/index.tsx b/frontend/src/pages/components/animate/views/background/index.tsx
new file mode 100644
index 0000000..63b0e06
--- /dev/null
+++ b/frontend/src/pages/components/animate/views/background/index.tsx
@@ -0,0 +1,55 @@
+import { Card, Row, Col } from 'antd';
+import { useMemo, useState } from 'react';
+
+import ControlPanel from '../../control-panel';
+
+import ContainerView from './container';
+import Toolbar from './toolbar';
+
+export default function BackgroundView() {
+ const defaultValue = useMemo(() => {
+ return {
+ selectedVariant: 'kenburnsTop',
+ };
+ }, []);
+ const [selectedVariant, setSelectedVariant] = useState(defaultValue.selectedVariant);
+
+ const onRefresh = () => {
+ setSelectedVariant(defaultValue.selectedVariant);
+ };
+ return (
+
+
+
+
+
+
+
+
+
+
+
+ setSelectedVariant(varient)}
+ />
+
+
+
+ );
+}
+const variantKey = [
+ {
+ type: 'kenburns',
+ values: ['kenburnsTop', 'kenburnsBottom', 'kenburnsLeft', 'kenburnsRight'],
+ },
+ {
+ type: 'pan',
+ values: ['panTop', 'panBottom', 'panLeft', 'panRight'],
+ },
+ {
+ type: 'color change',
+ values: ['color2x', 'color3x', 'color4x', 'color5x'],
+ },
+];
diff --git a/frontend/src/pages/components/animate/views/background/toolbar.tsx b/frontend/src/pages/components/animate/views/background/toolbar.tsx
new file mode 100644
index 0000000..30e8b2d
--- /dev/null
+++ b/frontend/src/pages/components/animate/views/background/toolbar.tsx
@@ -0,0 +1,12 @@
+import { ReloadOutlined } from '@ant-design/icons';
+
+type Props = {
+ onRefresh: VoidFunction;
+};
+export default function Toolbar({ onRefresh }: Props) {
+ return (
+
+
+
+ );
+}
diff --git a/frontend/src/pages/components/animate/views/inview/container.tsx b/frontend/src/pages/components/animate/views/inview/container.tsx
new file mode 100644
index 0000000..ecf805b
--- /dev/null
+++ b/frontend/src/pages/components/animate/views/inview/container.tsx
@@ -0,0 +1,55 @@
+import { m } from 'framer-motion';
+import { repeat } from 'ramda';
+import { useMemo } from 'react';
+
+import Cover3 from '@/assets/images/cover/cover_3.jpg';
+import MotionContainer from '@/components/animate/motion-container';
+import { getVariant } from '@/components/animate/variants';
+import { useThemeToken } from '@/theme/hooks';
+
+const TEXT = 'SlashAdmin';
+type Props = {
+ isText: boolean;
+ isMulti: boolean;
+ variant: string;
+};
+export default function ContainerView({ isText, variant, isMulti }: Props) {
+ const { colorBgLayout } = useThemeToken();
+ const varients = useMemo(() => getVariant(variant), [variant]);
+ const imgs = useMemo(() => (isMulti ? repeat(Cover3, 5) : [Cover3]), [isMulti]);
+
+ return (
+
+ {isText ? (
+
+ {TEXT.split('').map((letter, index) => (
+
+ {letter}
+
+ ))}
+
+ ) : (
+
+ {imgs.map((img, index) => (
+
+ ))}
+
+ )}
+
+ );
+}
diff --git a/frontend/src/pages/components/animate/views/inview/index.tsx b/frontend/src/pages/components/animate/views/inview/index.tsx
new file mode 100644
index 0000000..89c120c
--- /dev/null
+++ b/frontend/src/pages/components/animate/views/inview/index.tsx
@@ -0,0 +1,115 @@
+import { Card, Col, Row } from 'antd';
+import { useMemo, useState } from 'react';
+
+import ControlPanel from '../../control-panel';
+
+import ContainerView from './container';
+import Toolbar from './toolbar';
+
+const variantKey = [
+ {
+ type: 'slide in',
+ values: ['slideInUp', 'slideInDown', 'slideInLeft', 'slideInRight'],
+ },
+ {
+ type: 'slide out',
+ values: ['slideOutUp', 'slideOutDown', 'slideOutLeft', 'slideOutRight'],
+ },
+ {
+ type: 'fade in',
+ values: ['fadeIn', 'fadeInUp', 'fadeInDown', 'fadeInLeft', 'fadeInRight'],
+ },
+ {
+ type: 'fade out',
+ values: ['fadeOut', 'fadeOutUp', 'fadeOutDown', 'fadeOutLeft', 'fadeOutRight'],
+ },
+ {
+ type: 'zoom in',
+ values: ['zoomIn', 'zoomInUp', 'zoomInDown', 'zoomInLeft', 'zoomInRight'],
+ },
+ {
+ type: 'zoom out',
+ values: ['zoomOut', 'zoomOutUp', 'zoomOutDown', 'zoomOutLeft', 'zoomOutRight'],
+ },
+ {
+ type: 'bounce in',
+ values: ['bounceIn', 'bounceInUp', 'bounceInDown', 'bounceInLeft', 'bounceInRight'],
+ },
+ {
+ type: 'bounce out',
+ values: ['bounceOut', 'bounceOutUp', 'bounceOutDown', 'bounceOutLeft', 'bounceOutRight'],
+ },
+ {
+ type: 'flip in',
+ values: ['flipInX', 'flipInY'],
+ },
+ {
+ type: 'flip out',
+ values: ['flipOutX', 'flipOutY'],
+ },
+ {
+ type: 'scale in',
+ values: ['scaleInX', 'scaleInY'],
+ },
+ {
+ type: 'scale out',
+ values: ['scaleOutX', 'scaleOutY'],
+ },
+ {
+ type: 'rotate in',
+ values: ['rotateIn'],
+ },
+ {
+ type: 'rotate out',
+ values: ['rotateOut'],
+ },
+];
+
+export default function Inview() {
+ const defaultValue = useMemo(() => {
+ return {
+ isText: false,
+ isMulti: false,
+ selectedVariant: 'slideInUp',
+ };
+ }, []);
+
+ const [isText, setIsText] = useState(defaultValue.isText);
+ const [isMulti, setIsMulti] = useState(defaultValue.isMulti);
+
+ const [selectedVariant, setSelectedVariant] = useState(defaultValue.selectedVariant);
+
+ const onRefresh = () => {
+ setIsText(defaultValue.isText);
+ setIsMulti(defaultValue.isMulti);
+ setSelectedVariant(defaultValue.selectedVariant);
+ };
+
+ return (
+
+
+
+ setIsText(!isText)}
+ isMulti={isMulti}
+ onChangeMulti={() => setIsMulti(!isMulti)}
+ onRefresh={onRefresh}
+ />
+
+
+
+
+
+
+
+ setSelectedVariant(varient)}
+ />
+
+
+
+ );
+}
diff --git a/frontend/src/pages/components/animate/views/inview/toolbar.tsx b/frontend/src/pages/components/animate/views/inview/toolbar.tsx
new file mode 100644
index 0000000..eaaa592
--- /dev/null
+++ b/frontend/src/pages/components/animate/views/inview/toolbar.tsx
@@ -0,0 +1,35 @@
+import { ReloadOutlined } from '@ant-design/icons';
+import { Switch } from 'antd';
+
+type Props = {
+ isText: boolean;
+ isMulti: boolean;
+ onChnageText: (isText: boolean) => void;
+ onChangeMulti: (isMulti: boolean) => void;
+ onRefresh: VoidFunction;
+};
+export default function Toolbar({
+ isText,
+ isMulti,
+ onChnageText,
+ onChangeMulti,
+ onRefresh,
+}: Props) {
+ return (
+
+
+
+ Text Object
+
+
+ {isText ? null : (
+
+
+ Multi Item
+
+ )}
+
+
+
+ );
+}
diff --git a/frontend/src/pages/components/animate/views/scroll/container.tsx b/frontend/src/pages/components/animate/views/scroll/container.tsx
new file mode 100644
index 0000000..56c2626
--- /dev/null
+++ b/frontend/src/pages/components/animate/views/scroll/container.tsx
@@ -0,0 +1,30 @@
+import { Card, Typography } from 'antd';
+import { useMemo } from 'react';
+
+import MotionViewport from '@/components/animate/motion-viewport';
+import { getVariant } from '@/components/animate/variants';
+import { useThemeToken } from '@/theme/hooks';
+
+type Props = {
+ variant: string;
+};
+export default function ContainerView({ variant }: Props) {
+ const { colorBgLayout } = useThemeToken();
+ const varients = useMemo(() => getVariant(variant), [variant]);
+
+ return (
+
+ {[...Array(40)].map((_, index) => (
+
+
+ Item {index + 1}
+
+
+ ))}
+
+ );
+}
diff --git a/frontend/src/pages/components/animate/views/scroll/index.tsx b/frontend/src/pages/components/animate/views/scroll/index.tsx
new file mode 100644
index 0000000..a99de34
--- /dev/null
+++ b/frontend/src/pages/components/animate/views/scroll/index.tsx
@@ -0,0 +1,72 @@
+import { Card, Row, Col } from 'antd';
+import { useMemo, useState } from 'react';
+
+import ControlPanel from '../../control-panel';
+
+import ContainerView from './container';
+import Toolbar from './toolbar';
+
+export default function ScrollView() {
+ const defaultValue = useMemo(() => {
+ return {
+ selectedVariant: 'slideInUp',
+ };
+ }, []);
+ const [selectedVariant, setSelectedVariant] = useState(defaultValue.selectedVariant);
+
+ const onRefresh = () => {
+ setSelectedVariant(defaultValue.selectedVariant);
+ };
+ return (
+
+
+
+
+
+
+
+
+
+
+
+ setSelectedVariant(varient)}
+ />
+
+
+
+ );
+}
+
+const variantKey = [
+ {
+ type: 'slide',
+ values: ['slideInUp', 'slideInDown', 'slideInLeft', 'slideInRight'],
+ },
+ {
+ type: 'fade',
+ values: ['fadeIn', 'fadeInUp', 'fadeInDown', 'fadeInLeft', 'fadeInRight'],
+ },
+ {
+ type: 'zoom',
+ values: ['zoomIn', 'zoomInUp', 'zoomInDown', 'zoomInLeft', 'zoomInRight'],
+ },
+ {
+ type: 'bounce',
+ values: ['bounceIn', 'bounceInUp', 'bounceInDown', 'bounceInLeft', 'bounceInRight'],
+ },
+ {
+ type: 'flip',
+ values: ['flipInX', 'flipInY'],
+ },
+ {
+ type: 'scale',
+ values: ['scaleInX', 'scaleInY'],
+ },
+ {
+ type: 'rotate',
+ values: ['rotateIn'],
+ },
+];
diff --git a/frontend/src/pages/components/animate/views/scroll/toolbar.tsx b/frontend/src/pages/components/animate/views/scroll/toolbar.tsx
new file mode 100644
index 0000000..30e8b2d
--- /dev/null
+++ b/frontend/src/pages/components/animate/views/scroll/toolbar.tsx
@@ -0,0 +1,12 @@
+import { ReloadOutlined } from '@ant-design/icons';
+
+type Props = {
+ onRefresh: VoidFunction;
+};
+export default function Toolbar({ onRefresh }: Props) {
+ return (
+
+
+
+ );
+}
diff --git a/frontend/src/pages/components/chart/index.tsx b/frontend/src/pages/components/chart/index.tsx
new file mode 100644
index 0000000..29f6633
--- /dev/null
+++ b/frontend/src/pages/components/chart/index.tsx
@@ -0,0 +1,99 @@
+import { Card, Col, Row, Typography } from 'antd';
+
+import { useThemeToken } from '@/theme/hooks';
+
+import ChartArea from './view/chart-area';
+import ChartBar from './view/chart-bar';
+import ChartColumnMultiple from './view/chart-column-multiple';
+import ChartColumnNegative from './view/chart-column-negative';
+import ChartColumnSingle from './view/chart-column-single';
+import ChartColumnStacked from './view/chart-column-Stacked';
+import ChartDonut from './view/chart-donut';
+import ChartLine from './view/chart-line';
+import ChartMixed from './view/chart-mixed';
+import ChartPie from './view/chart-pie';
+import ChartRadar from './view/chart-radar';
+import ChartRadial from './view/chart-radial';
+
+export default function ChartPage() {
+ const { colorPrimary } = useThemeToken();
+ return (
+ <>
+
+ https://apexcharts.com
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ >
+ );
+}
diff --git a/frontend/src/pages/components/chart/view/chart-area.tsx b/frontend/src/pages/components/chart/view/chart-area.tsx
new file mode 100644
index 0000000..c759eaf
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-area.tsx
@@ -0,0 +1,30 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+
+const series = [
+ { name: 'series1', data: [31, 40, 28, 51, 42, 109, 100] },
+ { name: 'series2', data: [11, 32, 45, 32, 34, 52, 41] },
+];
+export default function ChartArea() {
+ const chartOptions = useChart({
+ xaxis: {
+ type: 'datetime',
+ categories: [
+ '2018-09-19T00:00:00.000Z',
+ '2018-09-19T01:30:00.000Z',
+ '2018-09-19T02:30:00.000Z',
+ '2018-09-19T03:30:00.000Z',
+ '2018-09-19T04:30:00.000Z',
+ '2018-09-19T05:30:00.000Z',
+ '2018-09-19T06:30:00.000Z',
+ ],
+ },
+ tooltip: {
+ x: {
+ format: 'dd/MM/yy HH:mm',
+ },
+ },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/chart/view/chart-bar.tsx b/frontend/src/pages/components/chart/view/chart-bar.tsx
new file mode 100644
index 0000000..1984107
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-bar.tsx
@@ -0,0 +1,29 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+
+const series = [400, 430, 448, 470, 540, 580, 690, 1100, 1200, 1380];
+
+export default function ChartBar() {
+ const chartOptions = useChart({
+ stroke: { show: false },
+ plotOptions: {
+ bar: { horizontal: true, barHeight: '30%' },
+ },
+ xaxis: {
+ categories: [
+ 'Italy',
+ 'Japan',
+ 'China',
+ 'Canada',
+ 'France',
+ 'Germany',
+ 'South Korea',
+ 'Netherlands',
+ 'United States',
+ 'United Kingdom',
+ ],
+ },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/chart/view/chart-column-Stacked.tsx b/frontend/src/pages/components/chart/view/chart-column-Stacked.tsx
new file mode 100644
index 0000000..c3ab8ad
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-column-Stacked.tsx
@@ -0,0 +1,47 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+
+const series = [
+ { name: 'Product A', data: [44, 55, 41, 67, 22, 43] },
+ { name: 'Product B', data: [13, 23, 20, 8, 13, 27] },
+ { name: 'Product C', data: [11, 17, 15, 15, 21, 14] },
+ { name: 'Product D', data: [21, 7, 25, 13, 22, 8] },
+];
+export default function ChartColumnStacked() {
+ const chartOptions = useChart({
+ chart: {
+ stacked: true,
+ zoom: {
+ enabled: true,
+ },
+ },
+ legend: {
+ itemMargin: {
+ vertical: 8,
+ },
+ position: 'right',
+ offsetY: 20,
+ },
+ plotOptions: {
+ bar: {
+ columnWidth: '16%',
+ },
+ },
+ stroke: {
+ show: false,
+ },
+ xaxis: {
+ type: 'datetime',
+ categories: [
+ '01/01/2011 GMT',
+ '01/02/2011 GMT',
+ '01/03/2011 GMT',
+ '01/04/2011 GMT',
+ '01/05/2011 GMT',
+ '01/06/2011 GMT',
+ ],
+ },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/chart/view/chart-column-multiple.tsx b/frontend/src/pages/components/chart/view/chart-column-multiple.tsx
new file mode 100644
index 0000000..01fe89a
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-column-multiple.tsx
@@ -0,0 +1,33 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+
+const series = [
+ {
+ name: 'Net Profit',
+ data: [44, 55, 57, 56, 61, 58, 63, 60, 66],
+ },
+ {
+ name: 'Revenue',
+ data: [76, 85, 101, 98, 87, 105, 91, 114, 94],
+ },
+];
+export default function ChartColumnMultiple() {
+ const chartOptions = useChart({
+ stroke: {
+ show: true,
+ width: 2,
+ colors: ['transparent'],
+ },
+ xaxis: {
+ categories: ['Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct'],
+ },
+ tooltip: {
+ y: {
+ formatter: (value: number) => `$ ${value} thousands`,
+ },
+ },
+ plotOptions: { bar: { columnWidth: '36%' } },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/chart/view/chart-column-negative.tsx b/frontend/src/pages/components/chart/view/chart-column-negative.tsx
new file mode 100644
index 0000000..637e372
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-column-negative.tsx
@@ -0,0 +1,76 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+import { useThemeToken } from '@/theme/hooks';
+
+const series = [
+ {
+ name: 'Cash Flow',
+ data: [
+ 1.45, 5.42, 5.9, -0.42, -12.6, -18.1, -18.2, -14.16, -11.1, -6.09, 0.34, 3.88, 13.07, 5.8, 2,
+ 7.37, 8.1, 13.57, 15.75, 17.1, 19.8, -27.03, -54.4, -47.2, -43.3, -18.6, -48.6, -41.1, -39.6,
+ -37.6, -29.4, -21.4, -2.4,
+ ],
+ },
+];
+export default function ChartColumnNegative() {
+ const theme = useThemeToken();
+ const chartOptions = useChart({
+ stroke: { show: false },
+ yaxis: {
+ labels: {
+ formatter: (value: number) => `${value.toFixed(0)}%`,
+ },
+ },
+ xaxis: {
+ type: 'datetime',
+ categories: [
+ '2011-01-01',
+ '2011-02-01',
+ '2011-03-01',
+ '2011-04-01',
+ '2011-05-01',
+ '2011-06-01',
+ '2011-07-01',
+ '2011-08-01',
+ '2011-09-01',
+ '2011-10-01',
+ '2011-11-01',
+ '2011-12-01',
+ '2012-01-01',
+ '2012-02-01',
+ '2012-03-01',
+ '2012-04-01',
+ '2012-05-01',
+ '2012-06-01',
+ '2012-07-01',
+ '2012-08-01',
+ '2012-09-01',
+ '2012-10-01',
+ '2012-11-01',
+ '2012-12-01',
+ '2013-01-01',
+ '2013-02-01',
+ '2013-03-01',
+ '2013-04-01',
+ '2013-05-01',
+ '2013-06-01',
+ '2013-07-01',
+ '2013-08-01',
+ '2013-09-01',
+ ],
+ },
+ plotOptions: {
+ bar: {
+ columnWidth: '58%',
+ colors: {
+ ranges: [
+ { from: -100, to: -46, color: theme.colorWarning },
+ { from: -45, to: 0, color: theme.colorInfo },
+ ],
+ },
+ },
+ },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/chart/view/chart-column-single.tsx b/frontend/src/pages/components/chart/view/chart-column-single.tsx
new file mode 100644
index 0000000..bd71d4e
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-column-single.tsx
@@ -0,0 +1,26 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+
+const series = [{ name: 'Net Profit', data: [44, 55, 57, 56, 61, 58, 63, 60, 66] }];
+export default function ChartColumnSingle() {
+ const chartOptions = useChart({
+ plotOptions: {
+ bar: {
+ columnWidth: '16%',
+ },
+ },
+ stroke: {
+ show: false,
+ },
+ xaxis: {
+ categories: ['Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct'],
+ },
+ tooltip: {
+ y: {
+ formatter: (value: number) => `$ ${value} thousands`,
+ },
+ },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/chart/view/chart-donut.tsx b/frontend/src/pages/components/chart/view/chart-donut.tsx
new file mode 100644
index 0000000..aad488a
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-donut.tsx
@@ -0,0 +1,27 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+
+const series = [44, 55, 13, 43];
+export default function ChartDonut() {
+ const chartOptions = useChart({
+ labels: ['Apple', 'Mango', 'Orange', 'Watermelon'],
+ stroke: {
+ show: false,
+ },
+ legend: {
+ horizontalAlign: 'center',
+ },
+ tooltip: {
+ fillSeriesColor: false,
+ },
+ plotOptions: {
+ pie: {
+ donut: {
+ size: '90%',
+ },
+ },
+ },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/chart/view/chart-line.tsx b/frontend/src/pages/components/chart/view/chart-line.tsx
new file mode 100644
index 0000000..1e1994a
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-line.tsx
@@ -0,0 +1,24 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+
+const series = [
+ {
+ name: 'Desktops',
+ data: [10, 41, 35, 51, 49, 62, 69, 91, 148],
+ },
+];
+export default function ChartLine() {
+ const chartOptions = useChart({
+ xaxis: {
+ categories: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep'],
+ },
+ tooltip: {
+ x: {
+ show: false,
+ },
+ marker: { show: false },
+ },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/chart/view/chart-mixed.tsx b/frontend/src/pages/components/chart/view/chart-mixed.tsx
new file mode 100644
index 0000000..6d925ae
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-mixed.tsx
@@ -0,0 +1,68 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+
+const series = [
+ {
+ name: 'Team A',
+ type: 'column',
+ data: [23, 11, 22, 27, 13, 22, 37, 21, 44, 22, 30],
+ },
+ {
+ name: 'Team B',
+ type: 'area',
+ data: [44, 55, 41, 67, 22, 43, 21, 41, 56, 27, 43],
+ },
+ {
+ name: 'Team C',
+ type: 'line',
+ data: [30, 25, 36, 30, 45, 35, 64, 52, 59, 36, 39],
+ },
+];
+
+export default function ChartMixed() {
+ const chartOptions = useChart({
+ stroke: {
+ width: [0, 2, 3],
+ },
+ plotOptions: {
+ bar: { columnWidth: '20%' },
+ },
+ fill: {
+ type: ['solid', 'gradient', 'solid'],
+ },
+ labels: [
+ '01/01/2003',
+ '02/01/2003',
+ '03/01/2003',
+ '04/01/2003',
+ '05/01/2003',
+ '06/01/2003',
+ '07/01/2003',
+ '08/01/2003',
+ '09/01/2003',
+ '10/01/2003',
+ '11/01/2003',
+ ],
+ xaxis: {
+ type: 'datetime',
+ },
+ yaxis: {
+ title: { text: 'Points' },
+ min: 0,
+ },
+ tooltip: {
+ shared: true,
+ intersect: false,
+ y: {
+ formatter: (value: number) => {
+ if (typeof value !== 'undefined') {
+ return `${value.toFixed(0)} points`;
+ }
+ return value;
+ },
+ },
+ },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/chart/view/chart-pie.tsx b/frontend/src/pages/components/chart/view/chart-pie.tsx
new file mode 100644
index 0000000..ab8a7ac
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-pie.tsx
@@ -0,0 +1,35 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+
+const series = [44, 55, 13, 43];
+export default function ChartPie() {
+ const chartOptions = useChart({
+ labels: ['America', 'Asia', 'Europe', 'Africa'],
+ legend: {
+ horizontalAlign: 'center',
+ },
+ stroke: {
+ show: false,
+ },
+ dataLabels: {
+ enabled: true,
+ dropShadow: {
+ enabled: false,
+ },
+ },
+ tooltip: {
+ fillSeriesColor: false,
+ },
+ plotOptions: {
+ pie: {
+ donut: {
+ labels: {
+ show: false,
+ },
+ },
+ },
+ },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/chart/view/chart-radar.tsx b/frontend/src/pages/components/chart/view/chart-radar.tsx
new file mode 100644
index 0000000..78f84be
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-radar.tsx
@@ -0,0 +1,44 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+import { useThemeToken } from '@/theme/hooks';
+
+const series = [
+ {
+ name: 'Series 1',
+ data: [80, 50, 30, 40, 100, 20],
+ },
+ {
+ name: 'Series 2',
+ data: [20, 30, 40, 80, 20, 80],
+ },
+ {
+ name: 'Series 3',
+ data: [44, 76, 78, 13, 43, 10],
+ },
+];
+export default function ChartRadar() {
+ const { colorText } = useThemeToken();
+ const chartOptions = useChart({
+ stroke: {
+ width: 2,
+ },
+ fill: {
+ opacity: 0.48,
+ },
+ legend: {
+ floating: true,
+ position: 'bottom',
+ horizontalAlign: 'center',
+ },
+ xaxis: {
+ categories: ['2011', '2012', '2013', '2014', '2015', '2016'],
+ labels: {
+ style: {
+ colors: [colorText, colorText, colorText, colorText, colorText, colorText],
+ },
+ },
+ },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/chart/view/chart-radial.tsx b/frontend/src/pages/components/chart/view/chart-radial.tsx
new file mode 100644
index 0000000..47aebfe
--- /dev/null
+++ b/frontend/src/pages/components/chart/view/chart-radial.tsx
@@ -0,0 +1,37 @@
+import Chart from '@/components/chart/chart';
+import useChart from '@/components/chart/useChart';
+import { fNumber } from '@/utils/format-number';
+
+const series = [44, 55];
+export default function ChartRadial() {
+ const chartOptions = useChart({
+ chart: {
+ sparkline: {
+ enabled: true,
+ },
+ },
+ labels: ['Apples', 'Oranges'],
+ legend: {
+ floating: true,
+ position: 'bottom',
+ horizontalAlign: 'center',
+ },
+ plotOptions: {
+ radialBar: {
+ hollow: {
+ size: '68%',
+ },
+ dataLabels: {
+ value: {
+ offsetY: 16,
+ },
+ total: {
+ formatter: () => fNumber(2324),
+ },
+ },
+ },
+ },
+ });
+
+ return ;
+}
diff --git a/frontend/src/pages/components/editor/index.tsx b/frontend/src/pages/components/editor/index.tsx
new file mode 100644
index 0000000..da8e08a
--- /dev/null
+++ b/frontend/src/pages/components/editor/index.tsx
@@ -0,0 +1,30 @@
+import { Card, Typography } from 'antd';
+import { useState } from 'react';
+
+import Editor from '@/components/editor';
+import { useThemeToken } from '@/theme/hooks';
+
+export default function EditorPage() {
+ const [quillSimple, setQuillSimple] = useState('');
+ const [quillFull, setQuillFull] = useState('');
+ const { colorPrimary } = useThemeToken();
+
+ return (
+ <>
+
+ https://github.com/zenoamaro/react-quill
+
+
+
+
+
+
+
+
+ >
+ );
+}
diff --git a/frontend/src/pages/components/form/label/index.tsx b/frontend/src/pages/components/form/label/index.tsx
new file mode 100644
index 0000000..5286b0c
--- /dev/null
+++ b/frontend/src/pages/components/form/label/index.tsx
@@ -0,0 +1,19 @@
+import {Space, Tooltip} from "antd";
+import {InfoCircleOutlined} from "@ant-design/icons";
+
+export default function LabelWithInfo(
+ props: {
+ label: string;
+ info: string;
+ }
+) {
+
+ return (
+
+ {props.label}
+
+
+
+
+ );
+}
diff --git a/frontend/src/pages/components/icon/index.tsx b/frontend/src/pages/components/icon/index.tsx
new file mode 100644
index 0000000..71b4d66
--- /dev/null
+++ b/frontend/src/pages/components/icon/index.tsx
@@ -0,0 +1,70 @@
+import { StepBackwardOutlined } from '@ant-design/icons';
+import { Card, Space, Typography } from 'antd';
+
+import { Iconify, SvgIcon } from '@/components/icon';
+import { useThemeToken } from '@/theme/hooks';
+
+export default function IconPage() {
+ const { colorPrimary, colorInfo, colorSuccess, colorWarning, colorError } = useThemeToken();
+ return (
+
+
+ For more info
+
+ click here
+
+
+
+ {`import { StepBackwardOutlined } from '@ant-design/icons';`}
+ {` `}
+
+
+
+
+
+
+
+ Simply beautiful open source icons. For more info
+
+ click here
+
+
+
+ {` `}
+
+
+
+
+
+
+
+
+
+
+
+ For more info
+
+ click here
+
+
+
+ {` `}
+
+
+
+
+
+
+
+
+
+
+
+ );
+}
diff --git a/frontend/src/pages/components/markdown/index.tsx b/frontend/src/pages/components/markdown/index.tsx
new file mode 100644
index 0000000..9256e61
--- /dev/null
+++ b/frontend/src/pages/components/markdown/index.tsx
@@ -0,0 +1,86 @@
+import { Card, Typography } from 'antd';
+import { useState } from 'react';
+
+import Markdown from '@/components/markdown';
+import { useThemeToken } from '@/theme/hooks';
+
+const TEXT = `
+# h1
+
+
+
+## h2
+
+
+
+**Paragraph** Lorem ipsum is placeholder text commonly used in the graphic, print, and publishing industries for previewing layouts and visual mockups.
+
+
+
+[Link (https://www.google.com/)](https://www.google.com/)
+
+
+
+###### Lists
+
+
+
+- [x] Write the press release
+- [ ] Update the website
+- [ ] Contact the media
+
+
+
+---
+
+
+
+###### A table:
+
+
+
+| Syntax | Description | Test Text |
+| :--- | :----: | ---: |
+| Header | Title | Here's this |
+| Paragraph | Text | And more |
+
+
+
+\`\`\`tsx
+import React from 'react';
+import ReactDOM from 'react-dom';
+import ReactMarkdown from 'react-markdown';
+import rehypeHighlight from 'rehype-highlight';
+
+ReactDOM.render(
+ {'# Your markdown here'} ,
+ document.querySelector('#content')
+);
+\`\`\`
+
+
+
+
+
+> A block quote with ~~strikethrough~~ and a URL: [https://reactjs.org](https://reactjs.org).
+
+`;
+
+export default function MarkdownPage() {
+ const [content] = useState(TEXT);
+ const { colorPrimary } = useThemeToken();
+ return (
+ <>
+
+ https://github.com/remarkjs/react-markdown
+
+
+ {content}
+
+ >
+ );
+}
diff --git a/frontend/src/pages/components/multi-language/index.tsx b/frontend/src/pages/components/multi-language/index.tsx
new file mode 100644
index 0000000..634da2a
--- /dev/null
+++ b/frontend/src/pages/components/multi-language/index.tsx
@@ -0,0 +1,43 @@
+import { Card, Pagination, Radio, Space, Typography } from 'antd';
+
+import { SvgIcon } from '@/components/icon';
+import useLocale from '@/locales/useLocale';
+import { useThemeToken } from '@/theme/hooks';
+
+import { LocalEnum } from '#/enum';
+
+export default function MultiLanguagePage() {
+ const {
+ setLocale,
+ locale,
+ language: { icon, label },
+ } = useLocale();
+
+ const { colorPrimary } = useThemeToken();
+
+ return (
+
+
+ https://www.i18next.com
+
+
+ https://ant.design/docs/react/i18n-cn
+
+
+ setLocale(e.target.value)} value={locale}>
+ English
+ Chinese
+
+
+
+
+ {label}
+
+
+
+
+
+
+
+ );
+}
diff --git a/frontend/src/pages/components/upload/index.tsx b/frontend/src/pages/components/upload/index.tsx
new file mode 100644
index 0000000..9791bfe
--- /dev/null
+++ b/frontend/src/pages/components/upload/index.tsx
@@ -0,0 +1,65 @@
+import { Card, Col, Row, Space, Switch, Tabs, TabsProps, Typography } from 'antd';
+import { useState } from 'react';
+
+import { Iconify } from '@/components/icon';
+import { Upload, UploadAvatar, UploadBox } from '@/components/upload';
+
+export default function UploadPage() {
+ const [thumbnail, setThumbnail] = useState(false);
+
+ const onChange = (checked: boolean) => {
+ setThumbnail(checked);
+ };
+
+ const ThumbnailSwitch = ;
+
+ const boxPlaceHolder = (
+
+
+
+ Upload File
+
+
+ );
+ const UploadFileTab = (
+
+
+
+
+
+
+
+
+ );
+ const UploadAvatarTab = (
+
+
+
+ );
+ const UploadBoxTab = (
+
+
+
+
+
+
+
+
+ );
+
+ const TABS: TabsProps['items'] = [
+ { key: 'upload--file', label: 'Upload Single File', children: UploadFileTab },
+ { key: 'upload-avatar', label: 'Upload Avatar', children: UploadAvatarTab },
+ { key: 'upload-box', label: 'Upload Box', children: UploadBoxTab },
+ ];
+
+ return ;
+}
diff --git a/frontend/src/pages/dashboard/analysis/banner.tsx b/frontend/src/pages/dashboard/analysis/banner.tsx
new file mode 100644
index 0000000..79fb334
--- /dev/null
+++ b/frontend/src/pages/dashboard/analysis/banner.tsx
@@ -0,0 +1,258 @@
+import { Col, Row } from 'antd';
+import Color from 'color';
+
+import Character3 from '@/assets/images/characters/character_3.png';
+import { useThemeToken } from '@/theme/hooks';
+
+import {useNavigate} from "react-router-dom";
+import {useTranslation} from "react-i18next";
+
+export default function BannerCard() {
+ const { t } = useTranslation();
+ const themeToken = useThemeToken();
+ const navigate = useNavigate();
+
+ const bg = `linear-gradient(135deg, ${Color(themeToken.colorPrimaryHover).alpha(0.2)}, ${Color(
+ themeToken.colorPrimary,
+ ).alpha(0.2)}) rgb(255, 255, 255)`;
+
+ return (
+
+
+
+
{t('common.welcomeBack')}
+ PandoraNext Helper
+
+
+ {t('common.desc')}
+
+ navigate('/token/account')}
+ >
+ {t('common.goNow')}
+
+
+
+
+
+
+
+ );
+}
+
+function BannerSvg() {
+ const { colorPrimary, colorPrimaryActive, colorPrimaryHover } = useThemeToken();
+ return (
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ );
+}
diff --git a/frontend/src/pages/dashboard/analysis/index.tsx b/frontend/src/pages/dashboard/analysis/index.tsx
new file mode 100644
index 0000000..9258885
--- /dev/null
+++ b/frontend/src/pages/dashboard/analysis/index.tsx
@@ -0,0 +1,12 @@
+import {Space} from 'antd';
+import BannerCard from "@/pages/dashboard/analysis/banner.tsx";
+
+function Analysis() {
+ return (
+
+
+
+ );
+}
+
+export default Analysis;
diff --git a/frontend/src/pages/sys/error/Page403.tsx b/frontend/src/pages/sys/error/Page403.tsx
new file mode 100644
index 0000000..2e13f3b
--- /dev/null
+++ b/frontend/src/pages/sys/error/Page403.tsx
@@ -0,0 +1,129 @@
+import { Typography } from 'antd';
+import { m } from 'framer-motion';
+import { Helmet } from 'react-helmet-async';
+import { NavLink } from 'react-router-dom';
+
+import Character4 from '@/assets/images/characters/character_4.png';
+import MotionContainer from '@/components/animate/motion-container';
+import { varBounce } from '@/components/animate/variants/bounce';
+import { useThemeToken } from '@/theme/hooks';
+
+const { VITE_APP_HOMEPAGE: HOMEPAGE } = import.meta.env;
+
+export default function Page403() {
+ const {
+ colorBgBase,
+ colorTextBase,
+ colorPrimary,
+ colorPrimaryActive,
+ colorPrimaryTextActive,
+ colorPrimaryHover,
+ } = useThemeToken();
+ return (
+ <>
+
+ 403 No Permission!
+
+
+
+
+
+
+ No permission
+
+
+
+
+
+ The page you are trying access has restricted access. Please refer to your system
+ administrator
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ {/* hand */}
+
+
+
+ {/* 0 */}
+
+ {/* 4_3 */}
+
+
+
+
+
+
+
+
+
+
+
+ Go to Home
+
+
+
+ >
+ );
+}
diff --git a/frontend/src/pages/sys/error/Page404.tsx b/frontend/src/pages/sys/error/Page404.tsx
new file mode 100644
index 0000000..273ef63
--- /dev/null
+++ b/frontend/src/pages/sys/error/Page404.tsx
@@ -0,0 +1,120 @@
+import { Typography } from 'antd';
+import { m } from 'framer-motion';
+import { Helmet } from 'react-helmet-async';
+import { NavLink } from 'react-router-dom';
+
+import Character6 from '@/assets/images/characters/character_6.png';
+import MotionContainer from '@/components/animate/motion-container';
+import { varBounce } from '@/components/animate/variants/bounce';
+import { useThemeToken } from '@/theme/hooks';
+
+const { VITE_APP_HOMEPAGE: HOMEPAGE } = import.meta.env;
+
+export default function Page404() {
+ const {
+ colorBgBase,
+ colorTextBase,
+ colorPrimary,
+ colorPrimaryActive,
+ colorPrimaryTextActive,
+ colorPrimaryHover,
+ } = useThemeToken();
+ return (
+ <>
+
+ 404 Page Not Found!
+
+
+
+
+
+
+ Sorry, Page Not Found!
+
+
+
+
+
+ Sorry, we couldn’t find the page you’re looking for. Perhaps you’ve mistyped the URL?
+ Be sure to check your spelling.
+
+
+
+
+
+
+
+
+
+
+
+ {/* background */}
+
+ {/* character */}
+
+ {/* sun */}
+
+ {/* sun */}
+
+ {/* 0 */}
+
+ {/* 4_4 */}
+
+ {/* cloud */}
+
+
+
+
+
+
+
+
+
+
+
+ Go to Home
+
+
+
+ >
+ );
+}
diff --git a/frontend/src/pages/sys/error/Page500.tsx b/frontend/src/pages/sys/error/Page500.tsx
new file mode 100644
index 0000000..7e58ebe
--- /dev/null
+++ b/frontend/src/pages/sys/error/Page500.tsx
@@ -0,0 +1,161 @@
+import { Typography } from 'antd';
+import { m } from 'framer-motion';
+import { Helmet } from 'react-helmet-async';
+import { NavLink } from 'react-router-dom';
+
+import Character8 from '@/assets/images/characters/character_8.png';
+import MotionContainer from '@/components/animate/motion-container';
+import { varBounce } from '@/components/animate/variants/bounce';
+import { useThemeToken } from '@/theme/hooks';
+
+const { VITE_APP_HOMEPAGE: HOMEPAGE } = import.meta.env;
+
+export default function Page() {
+ const { colorBgBase, colorTextBase, colorPrimary } = useThemeToken();
+ return (
+ <>
+
+ 500 Internal Server Error!
+
+
+
+
+
+
+ 500 Internal Server Error
+
+
+
+
+
+ There was an error, please try again later.
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ Go to Home
+
+
+
+ >
+ );
+}
diff --git a/frontend/src/pages/sys/login/Login.tsx b/frontend/src/pages/sys/login/Login.tsx
new file mode 100644
index 0000000..204199f
--- /dev/null
+++ b/frontend/src/pages/sys/login/Login.tsx
@@ -0,0 +1,60 @@
+import {Layout, Typography} from 'antd';
+import Color from 'color';
+import {useTranslation} from 'react-i18next';
+import {Navigate} from 'react-router-dom';
+
+import DashboardImg from '@/assets/images/background/dashboard.png';
+import Overlay2 from '@/assets/images/background/overlay_2.jpg';
+import LocalePicker from '@/components/locale-picker';
+import {useUserToken} from '@/store/userStore';
+import {useThemeToken} from '@/theme/hooks';
+
+import LoginForm from './LoginForm';
+import {LoginStateProvider} from './providers/LoginStateProvider';
+import {getItem} from "@/utils/storage.ts";
+import {StorageEnum} from "#/enum.ts";
+
+const { VITE_APP_HOMEPAGE: HOMEPAGE } = import.meta.env;
+
+function Login() {
+ const { t } = useTranslation();
+ const token = useUserToken();
+ const { colorBgElevated } = useThemeToken();
+
+ // 判断用户是否有权限
+ if (token.accessToken && getItem(StorageEnum.Token)) {
+ // 如果有授权,则跳转到首页
+ return ;
+ }
+
+ const gradientBg = Color(colorBgElevated).alpha(0.9).toString();
+ const bg = `linear-gradient(${gradientBg}, ${gradientBg}) center center / cover no-repeat,url(${Overlay2})`;
+
+ return (
+
+
+
PandoraNext Helper
+
+
+ {t('sys.login.signInSecondTitle')}
+
+
+
+
+
+
+
+
+
+
+
+
+
+ );
+}
+export default Login;
diff --git a/frontend/src/pages/sys/login/LoginForm.tsx b/frontend/src/pages/sys/login/LoginForm.tsx
new file mode 100644
index 0000000..f9475de
--- /dev/null
+++ b/frontend/src/pages/sys/login/LoginForm.tsx
@@ -0,0 +1,64 @@
+import { Button, Form, Input } from 'antd';
+import { useState } from 'react';
+import { useTranslation } from 'react-i18next';
+import { SignInReq } from '@/api/services/userService';
+import { useSignIn } from '@/store/userStore';
+import {useCaptchaSiteKey} from "@/store/settingStore.ts";
+import HCaptcha from "@hcaptcha/react-hcaptcha";
+
+
+function LoginForm() {
+ const { t } = useTranslation();
+ const [form] = Form.useForm();
+ const [loading, setLoading] = useState(false);
+ const [captchaToken, setCaptchaToken] = useState(undefined);
+
+ const captchaSiteKey = useCaptchaSiteKey();
+
+ const signIn = useSignIn();
+
+ const handleFinish = async ({ password }: SignInReq) => {
+ console.log(captchaToken);
+ setLoading(true);
+ try {
+ await signIn({ password, token: captchaToken });
+ } finally {
+ setLoading(false);
+ }
+ };
+ // @ts-ignore
+ return (
+ <>
+ {t('sys.login.signInFormTitle')}
+
+
+
+ {captchaSiteKey &&
+
+
+
+
+
+ }
+
+
+ {t('sys.login.loginButton')}
+
+
+
+ >
+ );
+}
+
+export default LoginForm;
diff --git a/frontend/src/pages/sys/login/components/ReturnButton.tsx b/frontend/src/pages/sys/login/components/ReturnButton.tsx
new file mode 100644
index 0000000..0c5a09b
--- /dev/null
+++ b/frontend/src/pages/sys/login/components/ReturnButton.tsx
@@ -0,0 +1,18 @@
+import { Button } from 'antd';
+import { useTranslation } from 'react-i18next';
+import { MdArrowBackIosNew } from 'react-icons/md';
+
+interface ReturnButtonProps {
+ onClick?: () => void;
+}
+export function ReturnButton({ onClick }: ReturnButtonProps) {
+ const { t } = useTranslation();
+ return (
+
+
+
+ {t('sys.login.backSignIn')}
+
+
+ );
+}
diff --git a/frontend/src/pages/sys/login/providers/LoginStateProvider.tsx b/frontend/src/pages/sys/login/providers/LoginStateProvider.tsx
new file mode 100644
index 0000000..a8f14e8
--- /dev/null
+++ b/frontend/src/pages/sys/login/providers/LoginStateProvider.tsx
@@ -0,0 +1,39 @@
+import { PropsWithChildren, createContext, useContext, useMemo, useState } from 'react';
+
+export enum LoginStateEnum {
+ LOGIN,
+ REGISTER,
+ RESET_PASSWORD,
+ MOBILE,
+ QR_CODE,
+}
+
+interface LoginStateContextType {
+ loginState: LoginStateEnum;
+ setLoginState: (loginState: LoginStateEnum) => void;
+ backToLogin: () => void;
+}
+const LoginStateContext = createContext({
+ loginState: LoginStateEnum.LOGIN,
+ setLoginState: () => {},
+ backToLogin: () => {},
+});
+
+export function useLoginStateContext() {
+ const context = useContext(LoginStateContext);
+ return context;
+}
+
+export function LoginStateProvider({ children }: PropsWithChildren) {
+ const [loginState, setLoginState] = useState(LoginStateEnum.LOGIN);
+
+ function backToLogin() {
+ setLoginState(LoginStateEnum.LOGIN);
+ }
+
+ const value: LoginStateContextType = useMemo(
+ () => ({ loginState, setLoginState, backToLogin }),
+ [loginState],
+ );
+ return {children} ;
+}
diff --git a/frontend/src/pages/token/account/index.tsx b/frontend/src/pages/token/account/index.tsx
new file mode 100644
index 0000000..d836a16
--- /dev/null
+++ b/frontend/src/pages/token/account/index.tsx
@@ -0,0 +1,704 @@
+import {
+ CheckCircleTwoTone,
+ DeleteOutlined,
+ EditOutlined,
+ ExclamationCircleTwoTone,
+ FundOutlined,
+ PlusOutlined,
+ ReloadOutlined,
+ ShareAltOutlined,
+} from '@ant-design/icons';
+import { useQuery } from '@tanstack/react-query';
+import {
+ Badge,
+ Button,
+ Card,
+ Checkbox,
+ Col,
+ Form,
+ Input,
+ InputNumber,
+ Modal,
+ Popconfirm,
+ Row,
+ Space,
+ Spin,
+ Switch,
+ Typography,
+} from 'antd';
+import Password from 'antd/es/input/Password';
+import Table, { ColumnsType } from 'antd/es/table';
+import { useEffect, useState } from 'react';
+import { useTranslation } from 'react-i18next';
+import { useNavigate } from 'react-router-dom';
+
+import accountService, { AccountAddReq } from '@/api/services/accountService.ts';
+import shareService from '@/api/services/shareService.ts';
+import Chart from '@/components/chart/chart.tsx';
+import useChart from '@/components/chart/useChart.ts';
+import LabelWithInfo from '@/pages/components/form/label';
+import {
+ useAddAccountMutation,
+ useDeleteAccountMutation,
+ useRefreshAccountMutation,
+ useUpdateAccountMutation,
+} from '@/store/accountStore.ts';
+import ProTag from '@/theme/antd/components/tag';
+import { onCopy } from '@/utils/copy.ts';
+
+import { useAddShareMutation } from '@/store/shareStore.ts';
+
+import { Account, defaultShare, Share } from '#/entity';
+
+type SearchFormFieldType = Pick;
+
+export default function AccountPage() {
+ const [searchForm] = Form.useForm();
+ const { t } = useTranslation();
+
+ const addAccountMutation = useAddAccountMutation();
+ const updateAccountMutation = useUpdateAccountMutation();
+ const deleteAccountMutation = useDeleteAccountMutation();
+ const refreshAccountMutation = useRefreshAccountMutation();
+ const addShareMutation = useAddShareMutation();
+
+ const navigate = useNavigate();
+
+ const [deleteAccountId, setDeleteAccountId] = useState(-1);
+ const [refreshAccountId, setRefreshAccountId] = useState(-1);
+
+ const searchEmail = Form.useWatch('email', searchForm);
+
+ const [AccountModalPros, setAccountModalProps] = useState({
+ formValue: {
+ email: '',
+ password: '',
+ refreshToken: '',
+ },
+ title: 'New',
+ show: false,
+ onOk: (values: AccountAddReq, callback) => {
+ if (values.id) {
+ updateAccountMutation.mutate(values, {
+ onSuccess: () => {
+ setAccountModalProps((prev) => ({ ...prev, show: false }));
+ },
+ onSettled: () => callback(false),
+ });
+ } else {
+ addAccountMutation.mutate(values, {
+ onSuccess: () => {
+ setAccountModalProps((prev) => ({ ...prev, show: false }));
+ },
+ onSettled: () => callback(false),
+ });
+ }
+ },
+ onCancel: () => {
+ setAccountModalProps((prev) => ({ ...prev, show: false }));
+ },
+ });
+
+ const [shareModalProps, setShareModalProps] = useState({
+ formValue: { ...defaultShare },
+ title: 'New',
+ show: false,
+ onOk: (values: Share, callback) => {
+ callback(true);
+ addShareMutation.mutate(values, {
+ onSuccess: () => {
+ setShareModalProps((prev) => ({ ...prev, show: false }));
+ },
+ onSettled: () => {
+ callback(false);
+ },
+ });
+ },
+ onCancel: () => {
+ setShareModalProps((prev) => ({ ...prev, show: false }));
+ },
+ });
+
+ const [shareInfoModalProps, setShareInfoModalProps] = useState({
+ accountId: -1,
+ show: false,
+ onOk: () => {
+ setShareInfoModalProps((prev) => ({ ...prev, show: false }));
+ },
+ });
+
+ const columns: ColumnsType = [
+ {
+ title: t('token.email'),
+ dataIndex: 'email',
+ ellipsis: true,
+ align: 'center',
+ render: (text) => (
+
+ {text}
+
+ ),
+ },
+ {
+ title: t('token.password'),
+ dataIndex: 'password',
+ align: 'center',
+ ellipsis: true,
+ render: (text) => (
+
+ {text}
+
+ ),
+ },
+ {
+ title: 'Refresh Token',
+ dataIndex: 'refresh_token',
+ align: 'center',
+ width: 150,
+ render: (_, record) =>
+ // 当refreshToken存在时,显示refreshToken,并点击复制,否则显示Emtpy Tag
+ record.refreshToken ? (
+ onCopy(record.refreshToken!, t, e)}
+ readOnly
+ />
+ ) : (
+ Empty
+ ),
+ },
+ {
+ title: 'Access Token',
+ dataIndex: 'accessToken',
+ align: 'center',
+ width: 100,
+ render: (_, record) =>
+ // 当accessToken存在时,显示accessToken,否则显示Error Tag
+ record.accessToken ? (
+ onCopy(record.accessToken!, t, e)}
+ readOnly
+ />
+ ) : (
+ Empty
+ ),
+ },
+ {
+ title: t('token.shareStatus'),
+ dataIndex: 'shared',
+ align: 'center',
+ width: 100,
+ render: (_, record) =>
+ // 当accessToken存在时,显示accessToken,否则显示Error Tag
+ record.shared == 1 ? (
+
+ ) : (
+
+ ),
+ },
+ {
+ title: t('token.updateTime'),
+ dataIndex: 'updateTime',
+ align: 'center',
+ width: 200,
+ },
+ {
+ title: t('token.share'),
+ key: 'share',
+ align: 'center',
+ render: (_, record) => (
+
+
+ }
+ onClick={() =>
+ navigate({
+ pathname: '/token/share',
+ search: `?email=${record.email}`,
+ })
+ }
+ >
+ {t('token.shareList')}
+
+
+ } onClick={() => onShareAdd(record)} />
+ } onClick={() => onShareInfo(record)} />
+
+ ),
+ },
+ {
+ title: t('token.action'),
+ key: 'operation',
+ align: 'center',
+ render: (_, record) => (
+
+ {
+ setRefreshAccountId(record.id);
+ refreshAccountMutation.mutate(record.id, {
+ onSettled: () => setRefreshAccountId(undefined),
+ });
+ }}
+ >
+ }
+ type="primary"
+ loading={refreshAccountId === record.id}
+ >
+ {t('common.refresh')}
+
+
+ onEdit(record)} icon={ } type="primary" />
+ {
+ setDeleteAccountId(record.id);
+ deleteAccountMutation.mutate(record.id, {
+ onSuccess: () => setDeleteAccountId(undefined),
+ });
+ }}
+ >
+ }
+ type="primary"
+ loading={deleteAccountId === record.id}
+ danger
+ />
+
+
+ ),
+ },
+ ];
+
+ const { data } = useQuery({
+ queryKey: ['accounts', searchEmail],
+ queryFn: () => accountService.searchAccountList(searchEmail),
+ });
+
+ const onSearchFormReset = () => {
+ searchForm.resetFields();
+ };
+
+ const onCreate = () => {
+ setAccountModalProps((prev) => ({
+ ...prev,
+ show: true,
+ title: t('token.createNew'),
+ formValue: {
+ id: undefined,
+ email: '',
+ password: '',
+ shared: 0,
+ custom_type: 'refresh_token',
+ custom_token: '',
+ },
+ }));
+ };
+
+ const onShareAdd = (record: Account) => {
+ setShareModalProps((prev) => ({
+ ...prev,
+ show: true,
+ title: t('token.share'),
+ formValue: {
+ ...defaultShare,
+ accountId: record.id,
+ },
+ }));
+ };
+
+ const onShareInfo = (record: Account) => {
+ setShareInfoModalProps((prev) => ({
+ ...prev,
+ show: true,
+ accountId: record.id,
+ }));
+ };
+
+ const onEdit = (record: Account) => {
+ setAccountModalProps((prev) => ({
+ ...prev,
+ show: true,
+ title: t('token.edit'),
+ formValue: {
+ ...prev.formValue,
+ id: record.id,
+ email: record.email,
+ password: record.password,
+ shared: record.shared,
+ refreshToken: record.refreshToken,
+ accessToken: record.accessToken,
+ },
+ }));
+ };
+
+ return (
+
+
+
+
+
+
+
+ {t('token.createNew')}
+
+
+ }
+ >
+
+
+
+
+
+
+ );
+}
+
+export type ShareModalProps = {
+ formValue: Share;
+ title: string;
+ show: boolean;
+ onOk: (values: Share, callback: any) => void;
+ onCancel: VoidFunction;
+};
+
+export function ShareModal({ title, show, formValue, onOk, onCancel }: ShareModalProps) {
+ const [form] = Form.useForm();
+ const [loading, setLoading] = useState(false);
+ const { t } = useTranslation();
+
+ const mode = title === 'Edit' || title === '编辑' ? 'edit' : 'create';
+
+ useEffect(() => {
+ form.setFieldsValue({ ...formValue });
+ }, [formValue, form]);
+
+ const onModalOk = () => {
+ form.validateFields().then((values) => {
+ onOk(values, setLoading);
+ });
+ };
+
+ return (
+ {
+ form.resetFields();
+ onCancel();
+ }}
+ okButtonProps={{
+ loading,
+ }}
+ destroyOnClose={false}
+ >
+ name="id" hidden>
+
+
+ name="shareToken" hidden>
+
+
+ name="accountId" hidden>
+
+
+ label="Unique Name" name="uniqueName" required>
+
+
+ label={t('token.password')} name="password" required>
+
+
+
+
+ label={t('token.expiresIn')} name="expiresIn">
+ (value == 0 ? '默认' : `${value} 秒`)} />
+
+
+
+ label={t('token.siteLimit')} name="siteLimit">
+
+
+
+
+
+
+ label={t('token.gpt35Limit')} name="gpt35Limit">
+
+ style={{ width: '100%' }}
+ formatter={(value) => {
+ switch (value?.toString()) {
+ case '-1':
+ return '无限制';
+ case '0':
+ return '禁用';
+ default:
+ return `${value}`;
+ }
+ }}
+ parser={(value) => {
+ switch (value) {
+ case '无限制':
+ return -1;
+ case '禁用':
+ return 0;
+ default:
+ return parseInt(value!);
+ }
+ }}
+ />
+
+
+
+
+ label={
+
+ }
+ name="gpt4Limit"
+ >
+
+ style={{ width: '100%' }}
+ formatter={(value) => {
+ switch (value?.toString()) {
+ case '-1':
+ return '无限制';
+ case '0':
+ return '禁用';
+ default:
+ return `${value}`;
+ }
+ }}
+ parser={(value) => {
+ switch (value) {
+ case '无限制':
+ return -1;
+ case '禁用':
+ return 0;
+ default:
+ return parseInt(value!);
+ }
+ }}
+ />
+
+
+
+
+
+
+
+ label={
+
+ }
+ name="refreshEveryday"
+ valuePropName="checked"
+ >
+
+
+
+
+
+ label={t('token.showUserinfo')}
+ name="showUserinfo"
+ labelCol={{ span: 18 }}
+ wrapperCol={{ span: 6 }}
+ valuePropName="checked"
+ >
+
+
+
+
+
+ label={t('token.showConversations')}
+ name="showConversations"
+ valuePropName="checked"
+ >
+
+
+
+
+ label={t('token.comment')} name="comment">
+
+
+
+
+ );
+}
+
+type AccountModalProps = {
+ formValue: AccountAddReq;
+ title: string;
+ show: boolean;
+ onOk: (values: AccountAddReq, setLoading: any) => void;
+ onCancel: VoidFunction;
+};
+
+function AccountModal({ title, show, formValue, onOk, onCancel }: AccountModalProps) {
+ const [form] = Form.useForm();
+ const [loading, setLoading] = useState(false);
+ const { t } = useTranslation();
+
+ // useEffect(() => {
+ // form.setFieldsValue({...formValue});
+ // }, [formValue, form]);
+
+ const onModalOk = () => {
+ form.validateFields().then((values) => {
+ setLoading(true);
+ onOk(values, setLoading);
+ });
+ };
+
+ return (
+ {
+ form.resetFields();
+ onCancel();
+ }}
+ okButtonProps={{
+ loading,
+ }}
+ destroyOnClose={false}
+ >
+ name="id" hidden>
+
+
+ label="Email" name="email" required>
+
+
+ label={t('token.password')} name="password">
+
+
+
+ label={t('token.share')}
+ name="shared"
+ labelAlign="left"
+ valuePropName="checked"
+ getValueFromEvent={(v) => {
+ return v ? 1 : 0;
+ }}
+ required
+ >
+
+
+
+ label={`Refresh Token(${t('common.optional')})`}
+ name="refreshToken"
+ >
+
+
+
+ label={`Access Token(${t('common.optional')})`}
+ name="accessToken"
+ >
+
+
+
+
+ );
+}
+
+type ShareInfoModalProps = {
+ accountId: number;
+ onOk: VoidFunction;
+ show: boolean;
+};
+
+function ShareInfoModal({ accountId, onOk, show }: ShareInfoModalProps) {
+ const { data: statistic, isLoading } = useQuery({
+ queryKey: ['shareInfo', accountId],
+ queryFn: () => shareService.getShareStatistic(accountId),
+ enabled: show,
+ });
+
+ const { t } = useTranslation();
+
+ const chartOptions = useChart({
+ legend: {
+ horizontalAlign: 'center',
+ },
+ stroke: {
+ show: true,
+ },
+ dataLabels: {
+ enabled: true,
+ dropShadow: {
+ enabled: false,
+ },
+ },
+ xaxis: {
+ categories: statistic?.categories || [],
+ },
+ tooltip: {
+ fillSeriesColor: false,
+ },
+ plotOptions: {
+ pie: {
+ donut: {
+ labels: {
+ show: false,
+ },
+ },
+ },
+ },
+ });
+
+ return (
+
+
+
+
+
+ );
+}
diff --git a/frontend/src/pages/token/share/index.tsx b/frontend/src/pages/token/share/index.tsx
new file mode 100644
index 0000000..64d4f92
--- /dev/null
+++ b/frontend/src/pages/token/share/index.tsx
@@ -0,0 +1,196 @@
+// import { useQuery } from '@tanstack/react-query';
+import {
+ Button,
+ Card,
+ Col,
+ Form,
+ Input,
+ Popconfirm,
+ Row,
+ Space, Typography,
+} from 'antd';
+import Table, { ColumnsType } from 'antd/es/table';
+import {useEffect, useState} from 'react';
+
+import {defaultShare, Share} from '#/entity';
+import {
+ DeleteOutlined, EditOutlined,
+} from "@ant-design/icons";
+import {useQuery} from "@tanstack/react-query";
+import {useSearchParams} from "@/router/hooks";
+import shareService from "@/api/services/shareService.ts";
+import {useDeleteShareMutation, useUpdateShareMutation} from "@/store/shareStore.ts";
+import {ShareModal, ShareModalProps} from "@/pages/token/account";
+import {useTranslation} from "react-i18next";
+import {onCopy} from "@/utils/copy.ts";
+type SearchFormFieldType = {
+ email?: string;
+ uniqueName?: string;
+};
+
+export default function SharePage() {
+
+ const deleteShareMutation = useDeleteShareMutation()
+ const updateShareMutation = useUpdateShareMutation()
+
+ const {t} = useTranslation()
+
+ const params = useSearchParams();
+ const [searchForm] = Form.useForm();
+ const email = Form.useWatch('email', searchForm);
+ const uniqueName = Form.useWatch('uniqueName', searchForm);
+ const [deleteRowKey, setDeleteRowKey] = useState(undefined);
+ const [shareModalProps, setShareModalProps] = useState({
+ formValue: {...defaultShare,},
+ title: t('token.edit'),
+ show: false,
+ onOk: (values: Share) => {
+ console.log(values)
+ setShareModalProps((prev) => ({...prev, show: false}));
+ },
+ onCancel: () => {
+ setShareModalProps((prev) => ({...prev, show: false}));
+ },
+ });
+
+
+ useEffect(() => {
+ searchForm.setFieldValue('email', params.get('email'))
+ }, [params]);
+
+ const columns: ColumnsType = [
+ {title: t('token.email'), dataIndex: ['account','email'],
+ render: (text) => (
+
+ {text}
+
+ )
+ },
+ { title: 'Unique Name', dataIndex: 'uniqueName', align: 'center', width: 120 },
+ { title: t('token.password'), dataIndex: 'password', align: 'center', width: 120 },
+ { title: t('token.gpt35Limit'), dataIndex: 'gpt35Limit', align: 'center', width: 120,
+ render: text => text == -1 ? '无限制' : text
+ },
+ { title: t('token.gpt4Limit'), dataIndex: 'gpt4Limit', align: 'center', width: 120,
+ render: text => text == -1 ? '无限制' : text
+ },
+ { title: t('token.refreshEveryday'), dataIndex: 'refreshEveryday', align: 'center', width: 120,
+ render: text => text ? '是' : '否'
+ },
+ { title: t('token.expiresIn'), dataIndex: 'expiresIn', align: 'center', width: 120,
+ render: text => text == 0 ? '最大' : text
+ },
+ { title: 'Share Token', dataIndex: 'shareToken', align: 'center',
+ render: (text) => (
+ onCopy(text, t, e)} readOnly/>
+ ),
+ },
+ { title: t('token.comment'), dataIndex: 'comment', align: 'center',
+ render: (text) => (
+
+ {text}
+
+ )
+ },
+ {
+ title: t('token.action'),
+ key: 'operation',
+ align: 'center',
+ render: (_,record) => (
+
+ } type={"primary"} onClick={() => onEdit(record)}/>
+ handleDelete(record)}>
+ } type={"primary"} loading={deleteRowKey == record.accountId + record.uniqueName} danger/>
+
+
+ ),
+ },
+ ];
+
+ const onEdit = (record: Share) => {
+ setShareModalProps({
+ formValue: record,
+ title: t('token.edit'),
+ show: true,
+ onOk: (values: Share, callback) => {
+ updateShareMutation.mutate(values, {
+ onSuccess: () => {
+ setShareModalProps((prev) => ({...prev, show: false}));
+ },
+ onSettled: () => callback(false)
+ })
+ },
+ onCancel: () => {
+ setShareModalProps((prev) => ({...prev, show: false}));
+ },
+ })
+ }
+
+ const handleDelete = (record: Share) => {
+ setDeleteRowKey(record.accountId + record.uniqueName)
+ deleteShareMutation.mutate(record, {
+ onSettled: () => {
+ setDeleteRowKey(undefined)
+ }
+ })
+ }
+
+ const { data } = useQuery({
+ queryKey: ['shareList', email, uniqueName],
+ queryFn: () => {
+ return shareService.searchShare(email, uniqueName)
+ }
+ })
+
+ const onSearchFormReset = () => {
+ searchForm.resetFields();
+ };
+
+ return (
+
+
+
+
+
+
+ record.accountId + record.uniqueName}
+ size="small"
+ scroll={{ x: 'max-content' }}
+ pagination={{ pageSize: 10 }}
+ columns={columns}
+ dataSource={data}
+ />
+
+
+
+ );
+}
diff --git a/frontend/src/router/components/auth-guard.tsx b/frontend/src/router/components/auth-guard.tsx
new file mode 100644
index 0000000..c10341b
--- /dev/null
+++ b/frontend/src/router/components/auth-guard.tsx
@@ -0,0 +1,27 @@
+import { useCallback, useEffect } from 'react';
+
+import { useUserToken } from '@/store/userStore';
+
+import { useRouter } from '../hooks';
+import {useLocation} from "react-router-dom";
+type Props = {
+ children: React.ReactNode;
+};
+export default function AuthGuard({ children }: Props) {
+ const router = useRouter();
+ const location = useLocation()
+ const { accessToken } = useUserToken();
+
+ const check = useCallback(() => {
+ console.log(location.pathname)
+ if (!accessToken && location.pathname !== '/login') {
+ router.replace('/login');
+ }
+ }, [router, accessToken]);
+
+ useEffect(() => {
+ check();
+ }, [check]);
+
+ return children;
+}
diff --git a/frontend/src/router/hooks/index.ts b/frontend/src/router/hooks/index.ts
new file mode 100644
index 0000000..94a4ff5
--- /dev/null
+++ b/frontend/src/router/hooks/index.ts
@@ -0,0 +1,8 @@
+export { useParams } from './use-params';
+export { usePathname } from './use-pathname';
+export { useSearchParams } from './use-search-params';
+export { useRouter } from './use-router';
+export { useRouteToMenuFn } from './use-route-to-menu';
+export { usePermissionRoutes } from './use-permission-routes';
+export { useMatchRouteMeta } from './use-match-route-meta';
+export { useFlattenedRoutes } from './use-flattened-routes';
diff --git a/frontend/src/router/hooks/use-flattened-routes.ts b/frontend/src/router/hooks/use-flattened-routes.ts
new file mode 100644
index 0000000..6c9a95b
--- /dev/null
+++ b/frontend/src/router/hooks/use-flattened-routes.ts
@@ -0,0 +1,17 @@
+import { useCallback, useMemo } from 'react';
+
+import { flattenMenuRoutes, menuFilter } from '../utils';
+
+import { usePermissionRoutes } from './use-permission-routes';
+
+/**
+ * 返回拍平后的菜单路由
+ */
+export function useFlattenedRoutes() {
+ const flattenRoutes = useCallback(flattenMenuRoutes, []);
+ const permissionRoutes = usePermissionRoutes();
+ return useMemo(() => {
+ const menuRoutes = menuFilter(permissionRoutes);
+ return flattenRoutes(menuRoutes);
+ }, [flattenRoutes, permissionRoutes]);
+}
diff --git a/frontend/src/router/hooks/use-match-route-meta.tsx b/frontend/src/router/hooks/use-match-route-meta.tsx
new file mode 100644
index 0000000..1f1c255
--- /dev/null
+++ b/frontend/src/router/hooks/use-match-route-meta.tsx
@@ -0,0 +1,48 @@
+import { useEffect, useState } from 'react';
+import { useMatches, useOutlet } from 'react-router-dom';
+
+import { useFlattenedRoutes } from './use-flattened-routes';
+import { useRouter } from './use-router';
+
+import { RouteMeta } from '#/router';
+
+/**
+ * 返回当前路由Meta信息
+ */
+export function useMatchRouteMeta() {
+ const { VITE_APP_HOMEPAGE: HOMEPAGE } = import.meta.env;
+ const [matchRouteMeta, setMatchRouteMeta] = useState();
+
+ // 获取路由组件实例
+ const children = useOutlet();
+
+ // 获取所有匹配的路由
+ const matchs = useMatches();
+
+ // 获取拍平后的路由菜单
+ const flattenedRoutes = useFlattenedRoutes();
+
+ // const pathname = usePathname();
+ const { push } = useRouter();
+
+ useEffect(() => {
+ // 获取当前匹配的路由
+ console.log('matchs1', matchs, flattenedRoutes);
+ const lastRoute = matchs.at(-1);
+
+ const currentRouteMeta = flattenedRoutes.find(
+ (item) => item.key === lastRoute?.pathname || `${item.key}/` === lastRoute?.pathname,
+ );
+ if (currentRouteMeta) {
+ if (!currentRouteMeta.hideTab) {
+ currentRouteMeta.outlet = children;
+ setMatchRouteMeta(currentRouteMeta);
+ }
+ } else {
+ push(HOMEPAGE);
+ }
+ // eslint-disable-next-line react-hooks/exhaustive-deps
+ }, [matchs]);
+
+ return matchRouteMeta;
+}
diff --git a/frontend/src/router/hooks/use-params.ts b/frontend/src/router/hooks/use-params.ts
new file mode 100644
index 0000000..cb09b62
--- /dev/null
+++ b/frontend/src/router/hooks/use-params.ts
@@ -0,0 +1,8 @@
+import { useMemo } from 'react';
+import { useParams as _useParams } from 'react-router-dom';
+
+export function useParams() {
+ const params = _useParams();
+
+ return useMemo(() => params, [params]);
+}
diff --git a/frontend/src/router/hooks/use-pathname.ts b/frontend/src/router/hooks/use-pathname.ts
new file mode 100644
index 0000000..2f2df8e
--- /dev/null
+++ b/frontend/src/router/hooks/use-pathname.ts
@@ -0,0 +1,8 @@
+import { useMemo } from 'react';
+import { useLocation } from 'react-router-dom';
+
+export function usePathname() {
+ const { pathname } = useLocation();
+
+ return useMemo(() => pathname, [pathname]);
+}
diff --git a/frontend/src/router/hooks/use-permission-routes.tsx b/frontend/src/router/hooks/use-permission-routes.tsx
new file mode 100644
index 0000000..ef21340
--- /dev/null
+++ b/frontend/src/router/hooks/use-permission-routes.tsx
@@ -0,0 +1,129 @@
+import { isEmpty } from 'ramda';
+import { Suspense, lazy, useMemo } from 'react';
+import { Navigate, Outlet } from 'react-router-dom';
+
+import { Iconify } from '@/components/icon';
+import { CircleLoading } from '@/components/loading';
+import { useUserPermission } from '@/store/userStore';
+import ProTag from '@/theme/antd/components/tag';
+import { flattenTrees } from '@/utils/tree';
+
+import { Permission } from '#/entity';
+import { BasicStatus, PermissionType } from '#/enum';
+import { AppRouteObject } from '#/router';
+
+// 使用 import.meta.glob 获取所有路由组件
+const pages = import.meta.glob('/src/pages/**/*.tsx');
+
+// 构建绝对路径的函数
+function resolveComponent(path: string) {
+ return pages[`/src/pages${path}`];
+}
+
+/**
+ * return routes about permission
+ */
+export function usePermissionRoutes() {
+ const permissions = useUserPermission();
+
+ return useMemo(() => {
+ const flattenedPermissions = flattenTrees(permissions!);
+ const permissionRoutes = transformPermissionToMenuRoutes(
+ permissions || [],
+ flattenedPermissions,
+ );
+ return [...permissionRoutes];
+ }, [permissions]);
+}
+
+/**
+ * transform Permission[] to AppRouteObject[]
+ * @param permissions
+ * @param parent
+ */
+function transformPermissionToMenuRoutes(
+ permissions: Permission[],
+ flattenedPermissions: Permission[],
+) {
+ return permissions.map((permission) => {
+ const {
+ route,
+ type,
+ label,
+ icon,
+ order,
+ hide,
+ status,
+ frameSrc,
+ newFeature,
+ component,
+ parentId,
+ children = [],
+ } = permission;
+
+ const appRoute: AppRouteObject = {
+ path: route,
+ meta: {
+ label,
+ key: getCompleteRoute(permission, flattenedPermissions),
+ hideMenu: !!hide,
+ disabled: status === BasicStatus.DISABLE,
+ },
+ };
+
+ if (order) appRoute.order = order;
+ if (icon) appRoute.meta!.icon = icon;
+ if (frameSrc) appRoute.meta!.frameSrc = frameSrc;
+ if (newFeature)
+ appRoute.meta!.suffix = (
+ }>
+ NEW
+
+ );
+
+ if (type === PermissionType.CATALOGUE) {
+ appRoute.meta!.hideTab = true;
+ if (!parentId) {
+ appRoute.element = (
+ }>
+
+
+ );
+ }
+ appRoute.children = transformPermissionToMenuRoutes(children, flattenedPermissions);
+ if (!isEmpty(children)) {
+ appRoute.children.unshift({
+ index: true,
+ element: ,
+ });
+ }
+ } else if (type === PermissionType.MENU) {
+ const Element = lazy(resolveComponent(component!) as any);
+ if (frameSrc) {
+ appRoute.element = ;
+ } else {
+ appRoute.element = ;
+ }
+ }
+
+ return appRoute;
+ });
+}
+
+/**
+ * Splicing from the root permission route to the current permission route
+ * @param {Permission} permission - current permission
+ * @param {Permission[]} flattenedPermissions - flattened permission array
+ * @param {string} route - parent permission route
+ * @returns {string} - The complete route after splicing
+ */
+function getCompleteRoute(permission: Permission, flattenedPermissions: Permission[], route = '') {
+ const currentRoute = route ? `/${permission.route}${route}` : `/${permission.route}`;
+
+ if (permission.parentId) {
+ const parentPermission = flattenedPermissions.find((p) => p.id === permission.parentId)!;
+ return getCompleteRoute(parentPermission, flattenedPermissions, currentRoute);
+ }
+
+ return currentRoute;
+}
diff --git a/frontend/src/router/hooks/use-route-to-menu.tsx b/frontend/src/router/hooks/use-route-to-menu.tsx
new file mode 100644
index 0000000..840d032
--- /dev/null
+++ b/frontend/src/router/hooks/use-route-to-menu.tsx
@@ -0,0 +1,59 @@
+import { ItemType } from 'antd/es/menu/hooks/useItems';
+import { useCallback } from 'react';
+import { useTranslation } from 'react-i18next';
+
+import { Iconify, SvgIcon } from '@/components/icon';
+import { useSettings } from '@/store/settingStore';
+
+import { ThemeLayout } from '#/enum';
+import { AppRouteObject } from '#/router';
+
+/**
+ * routes -> menus
+ */
+export function useRouteToMenuFn() {
+ const { t } = useTranslation();
+ const { themeLayout } = useSettings();
+ const routeToMenuFn = useCallback(
+ (items: AppRouteObject[]) => {
+ return items
+ .filter((item) => !item.meta?.hideMenu)
+ .map((item) => {
+ const menuItem: any = [];
+ const { meta, children } = item;
+ if (meta) {
+ const { key, label, icon, disabled, suffix } = meta;
+ menuItem.key = key;
+ menuItem.disabled = disabled;
+ menuItem.label = (
+
+
{t(label)}
+ {suffix}
+
+ );
+ if (icon) {
+ if (typeof icon === 'string') {
+ if (icon.startsWith('ic')) {
+ menuItem.icon = ;
+ } else {
+ menuItem.icon = ;
+ }
+ } else {
+ menuItem.icon = icon;
+ }
+ }
+ }
+ if (children) {
+ menuItem.children = routeToMenuFn(children);
+ }
+ return menuItem as ItemType;
+ });
+ },
+ [t, themeLayout],
+ );
+ return routeToMenuFn;
+}
diff --git a/frontend/src/router/hooks/use-router.ts b/frontend/src/router/hooks/use-router.ts
new file mode 100644
index 0000000..bc99bf2
--- /dev/null
+++ b/frontend/src/router/hooks/use-router.ts
@@ -0,0 +1,19 @@
+import { useMemo } from 'react';
+import { useNavigate } from 'react-router-dom';
+
+export function useRouter() {
+ const navigate = useNavigate();
+
+ const router = useMemo(
+ () => ({
+ back: () => navigate(-1),
+ forward: () => navigate(1),
+ reload: () => window.location.reload(),
+ push: (href: string) => navigate(href),
+ replace: (href: string) => navigate(href, { replace: true }),
+ }),
+ [navigate],
+ );
+
+ return router;
+}
diff --git a/frontend/src/router/hooks/use-search-params.ts b/frontend/src/router/hooks/use-search-params.ts
new file mode 100644
index 0000000..478e94c
--- /dev/null
+++ b/frontend/src/router/hooks/use-search-params.ts
@@ -0,0 +1,8 @@
+import { useMemo } from 'react';
+import { useSearchParams as _useSearchParams } from 'react-router-dom';
+
+export function useSearchParams() {
+ const [searchParams] = _useSearchParams();
+
+ return useMemo(() => searchParams, [searchParams]);
+}
diff --git a/frontend/src/router/index.tsx b/frontend/src/router/index.tsx
new file mode 100644
index 0000000..1f33ee3
--- /dev/null
+++ b/frontend/src/router/index.tsx
@@ -0,0 +1,38 @@
+import { lazy } from 'react';
+import { Navigate, RouteObject, RouterProvider, createHashRouter } from 'react-router-dom';
+
+import DashboardLayout from '@/layouts/dashboard';
+import AuthGuard from '@/router/components/auth-guard';
+import { usePermissionRoutes } from '@/router/hooks';
+import { ErrorRoutes } from '@/router/routes/error-routes';
+
+import { AppRouteObject } from '#/router';
+
+const { VITE_APP_HOMEPAGE: HOMEPAGE } = import.meta.env;
+const LoginRoute: AppRouteObject = {
+ path: '/login',
+ Component: lazy(() => import('@/pages/sys/login/Login')),
+};
+const PAGE_NOT_FOUND_ROUTE: AppRouteObject = {
+ path: '*',
+ element: ,
+};
+
+export default function Router() {
+ const permissionRoutes = usePermissionRoutes();
+ const asyncRoutes: AppRouteObject = {
+ path: '/',
+ element: (
+
+
+
+ ),
+ children: [{ index: true, element: }, ...permissionRoutes],
+ };
+
+ const routes = [LoginRoute, asyncRoutes, ErrorRoutes, PAGE_NOT_FOUND_ROUTE];
+
+ const router = createHashRouter(routes as unknown as RouteObject[]);
+
+ return ;
+}
diff --git a/frontend/src/router/routes/error-routes.tsx b/frontend/src/router/routes/error-routes.tsx
new file mode 100644
index 0000000..3a0cdfd
--- /dev/null
+++ b/frontend/src/router/routes/error-routes.tsx
@@ -0,0 +1,34 @@
+import { Suspense, lazy } from 'react';
+import { Outlet } from 'react-router-dom';
+
+import { CircleLoading } from '@/components/loading';
+import SimpleLayout from '@/layouts/simple';
+
+import AuthGuard from '../components/auth-guard';
+
+import { AppRouteObject } from '#/router';
+
+const Page403 = lazy(() => import('@/pages/sys/error/Page403'));
+const Page404 = lazy(() => import('@/pages/sys/error/Page404'));
+const Page500 = lazy(() => import('@/pages/sys/error/Page500'));
+
+/**
+ * error routes
+ * 403, 404, 500
+ */
+export const ErrorRoutes: AppRouteObject = {
+ element: (
+
+
+ }>
+
+
+
+
+ ),
+ children: [
+ { path: '403', element: },
+ { path: '404', element: },
+ { path: '500', element: },
+ ],
+};
diff --git a/frontend/src/router/routes/menu-routes.tsx b/frontend/src/router/routes/menu-routes.tsx
new file mode 100644
index 0000000..fa9126e
--- /dev/null
+++ b/frontend/src/router/routes/menu-routes.tsx
@@ -0,0 +1,25 @@
+import { Navigate } from 'react-router-dom';
+
+import DashboardLayout from '@/layouts/dashboard';
+
+import AuthGuard from '../components/auth-guard';
+import { getMenuModules } from '../utils';
+
+import { AppRouteObject } from '#/router';
+
+const menuModuleRoutes = getMenuModules();
+
+const { VITE_APP_HOMEPAGE: HOMEPAGE } = import.meta.env;
+
+/**
+ * dynamic routes
+ */
+export const menuRoutes: AppRouteObject = {
+ path: '/',
+ element: (
+
+
+
+ ),
+ children: [{ index: true, element: }, ...menuModuleRoutes],
+};
diff --git a/frontend/src/router/routes/modules/dashboard.tsx b/frontend/src/router/routes/modules/dashboard.tsx
new file mode 100644
index 0000000..e94b0c9
--- /dev/null
+++ b/frontend/src/router/routes/modules/dashboard.tsx
@@ -0,0 +1,37 @@
+import { Suspense, lazy } from 'react';
+import { Navigate, Outlet } from 'react-router-dom';
+
+import { SvgIcon } from '@/components/icon';
+import { CircleLoading } from '@/components/loading';
+
+import { AppRouteObject } from '#/router';
+
+const Analysis = lazy(() => import('@/pages/dashboard/analysis'));
+
+const dashboard: AppRouteObject = {
+ order: 1,
+ path: 'dashboard',
+ element: (
+ }>
+
+
+ ),
+ meta: {
+ label: 'sys.menu.dashboard',
+ icon: ,
+ key: '/dashboard',
+ },
+ children: [
+ {
+ index: true,
+ element: ,
+ },
+ {
+ path: 'home',
+ element: ,
+ meta: { label: 'sys.menu.analysis', key: '/dashboard/analysis' },
+ },
+ ],
+};
+
+export default dashboard;
diff --git a/frontend/src/router/routes/modules/errors.tsx b/frontend/src/router/routes/modules/errors.tsx
new file mode 100644
index 0000000..094c1cd
--- /dev/null
+++ b/frontend/src/router/routes/modules/errors.tsx
@@ -0,0 +1,56 @@
+import { Suspense, lazy } from 'react';
+import { Outlet } from 'react-router-dom';
+
+import { Iconify } from '@/components/icon';
+import { CircleLoading } from '@/components/loading';
+
+import { AppRouteObject } from '#/router';
+
+const Page403 = lazy(() => import('@/pages/sys/error/Page403'));
+const Page404 = lazy(() => import('@/pages/sys/error/Page404'));
+const Page500 = lazy(() => import('@/pages/sys/error/Page500'));
+
+const errors: AppRouteObject[] = [
+ {
+ path: 'error',
+ order: 6,
+ element: (
+ }>
+
+
+ ),
+ meta: {
+ label: 'sys_info.menu.error.index',
+ icon: ,
+ key: '/error',
+ },
+ children: [
+ {
+ path: '403',
+ element: ,
+ meta: {
+ label: 'sys_info.menu.error.403',
+ key: '/error/403',
+ },
+ },
+ {
+ path: '404',
+ element: ,
+ meta: {
+ label: 'sys_info.menu.error.404',
+ key: '/error/404',
+ },
+ },
+ {
+ path: '500',
+ element: ,
+ meta: {
+ label: 'sys_info.menu.error.500',
+ key: '/error/500',
+ },
+ },
+ ],
+ },
+];
+
+export default errors;
diff --git a/frontend/src/router/routes/modules/token.tsx b/frontend/src/router/routes/modules/token.tsx
new file mode 100644
index 0000000..541dfc5
--- /dev/null
+++ b/frontend/src/router/routes/modules/token.tsx
@@ -0,0 +1,37 @@
+import { Suspense, lazy } from 'react';
+import { Navigate, Outlet } from 'react-router-dom';
+
+import { SvgIcon } from '@/components/icon';
+import { CircleLoading } from '@/components/loading';
+
+import { AppRouteObject } from '#/router';
+
+const AccountPage = lazy(() => import(`@/pages/token/account`));
+
+const token: AppRouteObject = {
+ order: 10,
+ path: 'token',
+ element: (
+ }>
+
+
+ ),
+ meta: {
+ label: 'sys_info.menu.dashboard',
+ icon: ,
+ key: '/token',
+ },
+ children: [
+ {
+ index: true,
+ element: ,
+ },
+ {
+ path: 'account',
+ element: ,
+ meta: { label: 'sys_info.menu.account', key: '/token/account' },
+ },
+ ],
+};
+
+export default token;
diff --git a/frontend/src/router/utils.ts b/frontend/src/router/utils.ts
new file mode 100644
index 0000000..4e96f4e
--- /dev/null
+++ b/frontend/src/router/utils.ts
@@ -0,0 +1,53 @@
+import { ascend } from 'ramda';
+
+import { AppRouteObject, RouteMeta } from '#/router';
+
+/**
+ * return menu routes
+ */
+export const menuFilter = (items: AppRouteObject[]) => {
+ return items
+ .filter((item) => {
+ const show = item.meta?.key;
+ if (show && item.children) {
+ item.children = menuFilter(item.children);
+ }
+ return show;
+ })
+ .sort(ascend((item) => item.order || Infinity));
+};
+
+/**
+ * 基于 src/router/routes/modules 文件结构动态生成路由
+ */
+export function getMenuModules() {
+ const menuModules: AppRouteObject[] = [];
+
+ const modules = import.meta.glob('./routes/modules/**/*.tsx', { eager: true });
+ Object.keys(modules).forEach((key) => {
+ const mod = (modules as any)[key].default || {};
+ const modList = Array.isArray(mod) ? [...mod] : [mod];
+ menuModules.push(...modList);
+ });
+ return menuModules;
+}
+
+/**
+ * return the routes will be used in sidebar menu
+ */
+export function getMenuRoutes(appRouteObjects: AppRouteObject[]) {
+ // return menuFilter(getMenuModules());
+ return menuFilter(appRouteObjects);
+}
+
+/**
+ * return flatten routes
+ */
+export function flattenMenuRoutes(routes: AppRouteObject[]) {
+ return routes.reduce((prev, item) => {
+ const { meta, children } = item;
+ if (meta) prev.push(meta);
+ if (children) prev.push(...flattenMenuRoutes(children));
+ return prev;
+ }, []);
+}
diff --git a/frontend/src/store/accountStore.ts b/frontend/src/store/accountStore.ts
new file mode 100644
index 0000000..0bd50eb
--- /dev/null
+++ b/frontend/src/store/accountStore.ts
@@ -0,0 +1,47 @@
+import {useMutation, useQueryClient} from "@tanstack/react-query";
+import accountService from "@/api/services/accountService.ts";
+import {message} from "antd";
+
+export const useAddAccountMutation = () => {
+ const client = useQueryClient();
+ return useMutation(accountService.addAccount, {
+ onSuccess: () => {
+ /* onSuccess */
+ message.success('Add Account Success')
+ client.invalidateQueries(['accounts']);
+ },
+ });
+}
+
+export const useUpdateAccountMutation = () => {
+ const client = useQueryClient();
+ return useMutation(accountService.updateAccount, {
+ onSuccess: () => {
+ /* onSuccess */
+ message.success('Update Account Success')
+ client.invalidateQueries(['accounts']);
+ },
+ });
+}
+
+export const useDeleteAccountMutation = () => {
+ const client = useQueryClient();
+ return useMutation(accountService.deleteAccount, {
+ onSuccess: () => {
+ /* onSuccess */
+ message.success('Delete Account Success')
+ client.invalidateQueries(['accounts']);
+ }
+ });
+}
+
+export const useRefreshAccountMutation = () => {
+ const client = useQueryClient();
+ return useMutation(accountService.refreshAccount, {
+ onSuccess: () => {
+ /* onSuccess */
+ message.success('Refresh Account Success')
+ client.invalidateQueries(['accounts']);
+ }
+ });
+}
diff --git a/frontend/src/store/index.ts b/frontend/src/store/index.ts
new file mode 100644
index 0000000..881e467
--- /dev/null
+++ b/frontend/src/store/index.ts
@@ -0,0 +1,21 @@
+import { create } from 'zustand';
+
+type Store = {
+ count: number;
+ // 使用 actions 命名空间来存放所有的 action
+ actions: {
+ increment: () => void;
+ decrement: () => void;
+ };
+};
+
+const useStore = create((set) => ({
+ count: 0,
+ actions: {
+ increment: () => set((state) => ({ count: state.count + 1 })),
+ decrement: () => set((state) => ({ count: state.count - 1 })),
+ },
+}));
+
+export const useCount = () => useStore((state) => state.count);
+export const useCountActions = () => useStore((state) => state.actions);
diff --git a/frontend/src/store/settingStore.ts b/frontend/src/store/settingStore.ts
new file mode 100644
index 0000000..a699eb1
--- /dev/null
+++ b/frontend/src/store/settingStore.ts
@@ -0,0 +1,67 @@
+import { create } from 'zustand';
+
+import { getItem, removeItem, setItem } from '@/utils/storage';
+
+import { StorageEnum, ThemeColorPresets, ThemeLayout, ThemeMode } from '#/enum';
+
+type SettingsType = {
+ themeColorPresets: ThemeColorPresets;
+ themeMode: ThemeMode;
+ themeLayout: ThemeLayout;
+ themeStretch: boolean;
+ breadCrumb: boolean;
+ multiTab: boolean;
+ captchaSiteKey?: string;
+ taskStatus?: boolean;
+};
+type SettingStore = {
+ settings: SettingsType;
+ // 使用 actions 命名空间来存放所有的 action
+ actions: {
+ setTaskStatus: (taskStatus: boolean) => void;
+ setCaptchaSiteKey: (captchaSiteKey: string) => void;
+ setSettings: (settings: SettingsType) => void;
+ clearSettings: () => void;
+ };
+};
+
+const useSettingStore = create((set) => ({
+ settings: getItem(StorageEnum.Settings) || {
+ themeColorPresets: ThemeColorPresets.Default,
+ themeMode: ThemeMode.Light,
+ themeLayout: ThemeLayout.Vertical,
+ themeStretch: false,
+ breadCrumb: true,
+ multiTab: true,
+ taskStatus: false,
+ },
+ actions: {
+ setTaskStatus: (taskStatus) => {
+ set((state) => ({
+ settings: {
+ ...state.settings,
+ taskStatus,
+ },
+ }));
+ },
+ setCaptchaSiteKey: (captchaSiteKey) => {
+ set((state) => ({
+ settings: {
+ ...state.settings,
+ captchaSiteKey,
+ },
+ }));
+ },
+ setSettings: (settings) => {
+ set({ settings });
+ setItem(StorageEnum.Settings, settings);
+ },
+ clearSettings() {
+ removeItem(StorageEnum.Settings);
+ },
+ },
+}));
+
+export const useCaptchaSiteKey = () => useSettingStore((state) => state.settings.captchaSiteKey);
+export const useSettings = () => useSettingStore((state) => state.settings);
+export const useSettingActions = () => useSettingStore((state) => state.actions);
diff --git a/frontend/src/store/shareStore.ts b/frontend/src/store/shareStore.ts
new file mode 100644
index 0000000..31fb141
--- /dev/null
+++ b/frontend/src/store/shareStore.ts
@@ -0,0 +1,44 @@
+import {useMutation, useQueryClient} from "@tanstack/react-query";
+import shareService from "@/api/services/shareService.ts";
+import {message} from "antd";
+
+
+export const useAddShareMutation = () => {
+ const client = useQueryClient();
+ return useMutation(shareService.addShare, {
+ onSuccess: () => {
+ /* onSuccess */
+ client.invalidateQueries(['accounts']);
+ client.invalidateQueries(['shareList']);
+ message.success('Success')
+ },
+ });
+}
+
+export const useUpdateShareMutation = () => {
+ const client = useQueryClient();
+ return useMutation(shareService.updateShare, {
+ onSuccess: () => {
+ /* onSuccess */
+ client.invalidateQueries(['shareList']);
+ message.success('Success')
+ },
+ });
+}
+
+export const useDeleteShareMutation = () => {
+ const client = useQueryClient();
+ return useMutation(shareService.deleteShare, {
+ onSuccess: () => {
+ /* onSuccess */
+ client.invalidateQueries(['shareList']);
+ message.success('Success')
+ },
+ })
+}
+
+export default {
+ useAddShareMutation,
+ useDeleteShareMutation,
+}
+
diff --git a/frontend/src/store/userStore.ts b/frontend/src/store/userStore.ts
new file mode 100644
index 0000000..5ed9918
--- /dev/null
+++ b/frontend/src/store/userStore.ts
@@ -0,0 +1,85 @@
+import { useMutation } from '@tanstack/react-query';
+import { App } from 'antd';
+import { useCallback } from 'react';
+import { useTranslation } from 'react-i18next';
+import { useNavigate } from 'react-router-dom';
+import { create } from 'zustand';
+
+import userService, { SignInReq } from '@/api/services/userService';
+import { getItem, removeItem, setItem } from '@/utils/storage';
+
+import { UserInfo, UserToken } from '#/entity';
+import { StorageEnum } from '#/enum';
+
+const { VITE_APP_HOMEPAGE: HOMEPAGE } = import.meta.env;
+
+type UserStore = {
+ userInfo: Partial;
+ userToken: UserToken;
+ // 使用 actions 命名空间来存放所有的 action
+ actions: {
+ setUserInfo: (userInfo: UserInfo) => void;
+ setUserToken: (token: UserToken) => void;
+ clearUserInfoAndToken: () => void;
+ };
+};
+
+export const useUserStore = create((set) => ({
+ userInfo: getItem(StorageEnum.User) || {},
+ userToken: getItem(StorageEnum.Token) || {},
+ actions: {
+ setUserInfo: (userInfo) => {
+ set({ userInfo });
+ setItem(StorageEnum.User, userInfo);
+ },
+ setUserToken: (userToken) => {
+ set({ userToken });
+ setItem(StorageEnum.Token, userToken);
+ },
+ clearUserInfoAndToken() {
+ set({ userInfo: {}, userToken: {} });
+ removeItem(StorageEnum.User);
+ removeItem(StorageEnum.Token);
+ },
+ },
+}));
+
+export const useUserInfo = () => useUserStore((state) => state.userInfo);
+export const useUserToken = () => useUserStore((state) => state.userToken);
+export const useUserPermission = () => useUserStore((state) => state.userInfo.permissions);
+export const useUserActions = () => useUserStore((state) => state.actions);
+
+export const useSignIn = () => {
+ const { t } = useTranslation();
+ const navigatge = useNavigate();
+ const { notification, message } = App.useApp();
+ const { setUserToken, setUserInfo } = useUserActions();
+
+ const signInMutation = useMutation(userService.signin);
+
+ const signIn = async (data: SignInReq) => {
+ try {
+ const res = await signInMutation.mutateAsync(data);
+ console.log(res);
+ const { user, accessToken } = res;
+ setUserToken({ accessToken });
+ // 固定一个用户信息 Admin
+ setUserInfo(user);
+ navigatge(HOMEPAGE, { replace: true });
+
+ notification.success({
+ message: t('sys.login.loginSuccessTitle'),
+ description: `${t('sys.login.loginSuccessDesc')}`,
+ duration: 3,
+ });
+ } catch (err) {
+ message.warning({
+ content: err.message,
+ duration: 3,
+ });
+ }
+ };
+
+ // eslint-disable-next-line react-hooks/exhaustive-deps
+ return useCallback(signIn, []);
+};
diff --git a/frontend/src/theme/antd/components/rage.tsx b/frontend/src/theme/antd/components/rage.tsx
new file mode 100644
index 0000000..4317aca
--- /dev/null
+++ b/frontend/src/theme/antd/components/rage.tsx
@@ -0,0 +1,21 @@
+import { Rate, RateProps } from 'antd';
+import styled from 'styled-components';
+
+import { Iconify } from '@/components/icon';
+
+export default function ProRate(props: RateProps) {
+ return (
+
+ } {...props} />
+
+ );
+}
+
+const StyledRate = styled.div`
+ .ant-rate {
+ color: rgb(250, 175, 0);
+ .ant-rate-star:not(:last-child) {
+ margin-inline-end: 0;
+ }
+ }
+`;
diff --git a/frontend/src/theme/antd/components/tag.tsx b/frontend/src/theme/antd/components/tag.tsx
new file mode 100644
index 0000000..434efaf
--- /dev/null
+++ b/frontend/src/theme/antd/components/tag.tsx
@@ -0,0 +1,31 @@
+import { Tag, TagProps } from 'antd';
+import styled from 'styled-components';
+
+export default function ProTag(props: TagProps) {
+ return (
+
+
+
+ );
+}
+
+const StyledTag = styled.div`
+ display: inline-flex;
+ .ant-tag {
+ border-radius: 6px;
+ cursor: default;
+ height: 24px;
+ min-width: 24px;
+ padding: 0 6px;
+ margin: 0 6px;
+ font-size: 0.75rem;
+ font-weight: 700;
+ border-width: 0;
+ display: inline-flex;
+ justify-content: center;
+ align-items: center;
+ white-space: nowrap;
+ text-transform: capitalize;
+ transition: all 200ms cubic-bezier(0.4, 0, 0.2, 1) 0ms;
+ }
+`;
diff --git a/frontend/src/theme/antd/index.tsx b/frontend/src/theme/antd/index.tsx
new file mode 100644
index 0000000..9cf5ae5
--- /dev/null
+++ b/frontend/src/theme/antd/index.tsx
@@ -0,0 +1,41 @@
+import { StyleProvider } from '@ant-design/cssinjs';
+import { ConfigProvider, theme } from 'antd';
+import 'antd/dist/reset.css';
+
+import useLocale from '@/locales/useLocale';
+import { useSettings } from '@/store/settingStore';
+
+import {
+ customThemeTokenConfig,
+ themeModeToken,
+ colorPrimarys,
+ customComponentConfig,
+} from './theme';
+
+import { ThemeMode } from '#/enum';
+
+type Props = {
+ children: React.ReactNode;
+};
+export default function AntdConfig({ children }: Props) {
+ const { themeMode, themeColorPresets } = useSettings();
+
+ const { language } = useLocale();
+
+ const algorithm = themeMode === ThemeMode.Light ? theme.defaultAlgorithm : theme.darkAlgorithm;
+ const colorPrimary = colorPrimarys[themeColorPresets];
+
+ return (
+
+ {/* https://ant.design/docs/react/compatible-style-cn#styleprovider */}
+ {children}
+
+ );
+}
diff --git a/frontend/src/theme/antd/theme.ts b/frontend/src/theme/antd/theme.ts
new file mode 100644
index 0000000..0a8dbc2
--- /dev/null
+++ b/frontend/src/theme/antd/theme.ts
@@ -0,0 +1,63 @@
+import { ThemeConfig } from 'antd';
+
+import { ThemeColorPresets } from '#/enum';
+/**
+ * Antd theme editor: https://ant.design/theme-editor-cn
+ */
+const customThemeTokenConfig: ThemeConfig['token'] = {
+ colorSuccess: '#22c55e',
+ colorWarning: '#ff7849',
+ colorError: '#ff5630',
+ colorInfo: '#00b8d9',
+
+ // 线性化
+ wireframe: false,
+
+ borderRadiusSM: 2,
+ borderRadius: 4,
+ borderRadiusLG: 8,
+};
+
+const customComponentConfig: ThemeConfig['components'] = {
+ Breadcrumb: {
+ fontSize: 12,
+ separatorMargin: 4,
+ },
+ Menu: {
+ fontSize: 14,
+ colorFillAlter: 'transparent',
+ itemColor: 'rgb(145, 158, 171)',
+ },
+};
+
+const colorPrimarys: {
+ [k in ThemeColorPresets]: string;
+} = {
+ default: '#00a76f',
+ cyan: '#078DEE',
+ purple: '#7635DC',
+ blue: '#2065D1',
+ orange: '#FDA92D',
+ red: '#FF3030',
+};
+
+const themeModeToken: Record<'dark' | 'light', ThemeConfig> = {
+ dark: {
+ token: {
+ colorBgLayout: '#161c24',
+ colorBgContainer: '#212b36',
+ colorBgElevated: '#161c24',
+ },
+ components: {
+ Modal: {
+ headerBg: '#212b36',
+ contentBg: '#212b36',
+ footerBg: '#212b36',
+ },
+ Notification: {},
+ },
+ },
+ light: {},
+};
+
+export { customThemeTokenConfig, customComponentConfig, colorPrimarys, themeModeToken };
diff --git a/frontend/src/theme/base.css b/frontend/src/theme/base.css
new file mode 100644
index 0000000..15f398c
--- /dev/null
+++ b/frontend/src/theme/base.css
@@ -0,0 +1,365 @@
+/*
+1. Prevent padding and border from affecting element width. (https://github.com/mozdevs/cssremedy/issues/4)
+2. Allow adding a border to an element by just adding a border-width. (https://github.com/tailwindcss/tailwindcss/pull/116)
+*/
+
+*,
+::before,
+::after {
+ box-sizing: border-box; /* 1 */
+ border-style: solid; /* 2 */
+ border-width: 0; /* 2 */
+}
+
+::before,
+::after {
+ --tw-content: '';
+}
+
+/*
+1. Use a consistent sensible line-height in all browsers.
+2. Prevent adjustments of font size after orientation changes in iOS.
+3. Use a more readable tab size.
+4. Use the user's configured `sans` font-family by default.
+5. Use the user's configured `sans` font-feature-settings by default.
+6. Use the user's configured `sans` font-variation-settings by default.
+*/
+
+html {
+ line-height: 1.5; /* 1 */ /* 3 */
+ tab-size: 4; /* 3 */
+ text-size-adjust: 100%; /* 2 */
+}
+
+/*
+1. Remove the margin in all browsers.
+2. Inherit line-height from `html` so users can set them as a class directly on the `html` element.
+*/
+
+body {
+ margin: 0; /* 1 */
+ line-height: inherit; /* 2 */
+}
+
+/*
+1. Add the correct height in Firefox.
+2. Correct the inheritance of border color in Firefox. (https://bugzilla.mozilla.org/show_bug.cgi?id=190655)
+3. Ensure horizontal rules are visible by default.
+*/
+
+hr {
+ height: 0; /* 1 */
+ color: inherit; /* 2 */
+ border-top-width: 1px; /* 3 */
+}
+
+/*
+Add the correct text decoration in Chrome, Edge, and Safari.
+*/
+
+abbr:where([title]) {
+ text-decoration: underline dotted;
+}
+
+/*
+Remove the default font size and weight for headings.
+*/
+
+h1,
+h2,
+h3,
+h4,
+h5,
+h6 {
+ font-weight: inherit;
+ font-size: inherit;
+}
+
+/*
+Reset links to optimize for opt-in styling instead of opt-out.
+*/
+
+a {
+ color: inherit;
+ text-decoration: inherit;
+}
+
+/*
+Add the correct font weight in Edge and Safari.
+*/
+
+b,
+strong {
+ font-weight: bolder;
+}
+
+/*
+1. Use the user's configured `mono` font family by default.
+2. Correct the odd `em` font sizing in all browsers.
+*/
+
+code,
+kbd,
+samp,
+pre {
+ font-size: 1em; /* 2 */
+}
+
+/*
+Add the correct font size in all browsers.
+*/
+
+small {
+ font-size: 80%;
+}
+
+/*
+Prevent `sub` and `sup` elements from affecting the line height in all browsers.
+*/
+
+sub,
+sup {
+ position: relative;
+ font-size: 75%;
+ line-height: 0;
+ vertical-align: baseline;
+}
+
+sub {
+ bottom: -0.25em;
+}
+
+sup {
+ top: -0.5em;
+}
+
+/*
+1. Remove text indentation from table contents in Chrome and Safari. (https://bugs.chromium.org/p/chromium/issues/detail?id=999088, https://bugs.webkit.org/show_bug.cgi?id=201297)
+2. Correct table border color inheritance in all Chrome and Safari. (https://bugs.chromium.org/p/chromium/issues/detail?id=935729, https://bugs.webkit.org/show_bug.cgi?id=195016)
+3. Remove gaps between table borders by default.
+*/
+
+table {
+ text-indent: 0; /* 1 */
+ border-color: inherit; /* 2 */
+ border-collapse: collapse; /* 3 */
+}
+
+/*
+1. Change the font styles in all browsers.
+2. Remove the margin in Firefox and Safari.
+3. Remove default padding in all browsers.
+*/
+
+button,
+input,
+optgroup,
+select,
+textarea {
+ margin: 0; /* 2 */
+ padding: 0; /* 3 */
+ color: inherit; /* 1 */
+ font-weight: inherit; /* 1 */
+ font-size: 100%; /* 1 */
+ font-family: inherit; /* 1 */
+ line-height: inherit; /* 1 */
+ font-feature-settings: inherit; /* 1 */
+ font-variation-settings: inherit; /* 1 */
+}
+
+/*
+Remove the inheritance of text transform in Edge and Firefox.
+*/
+
+button,
+select {
+ text-transform: none;
+}
+
+/*
+1. Correct the inability to style clickable types in iOS and Safari.
+2. Remove default button styles.
+*/
+
+button {
+ background-color: inherit;
+}
+
+/*
+Use the modern Firefox focus style for all focusable elements.
+*/
+
+:-moz-focusring {
+ outline: auto;
+}
+
+/*
+Remove the additional `:invalid` styles in Firefox. (https://github.com/mozilla/gecko-dev/blob/2f9eacd9d3d995c937b4251a5557d95d494c9be1/layout/style/res/forms.css#L728-L737)
+*/
+
+:-moz-ui-invalid {
+ box-shadow: none;
+}
+
+/*
+Add the correct vertical alignment in Chrome and Firefox.
+*/
+
+progress {
+ vertical-align: baseline;
+}
+
+/*
+Correct the cursor style of increment and decrement buttons in Safari.
+*/
+
+::-webkit-inner-spin-button,
+::-webkit-outer-spin-button {
+ height: auto;
+}
+
+/*
+1. Correct the odd appearance in Chrome and Safari.
+2. Correct the outline style in Safari.
+*/
+
+[type='search'] {
+ outline-offset: -2px; /* 2 */
+ appearance: textfield; /* 1 */
+}
+
+/*
+Remove the inner padding in Chrome and Safari on macOS.
+*/
+
+::-webkit-search-decoration {
+ appearance: none;
+}
+
+/*
+1. Correct the inability to style clickable types in iOS and Safari.
+2. Change font properties to `inherit` in Safari.
+*/
+
+::-webkit-file-upload-button {
+ font: inherit; /* 2 */
+ appearance: button; /* 1 */
+}
+
+/*
+Add the correct display in Chrome and Safari.
+*/
+
+summary {
+ display: list-item;
+}
+
+/*
+Removes the default spacing and border for appropriate elements.
+*/
+
+blockquote,
+dl,
+dd,
+h1,
+h2,
+h3,
+h4,
+h5,
+h6,
+hr,
+figure,
+p,
+pre {
+ margin: 0;
+}
+
+fieldset {
+ margin: 0;
+ padding: 0;
+}
+
+legend {
+ padding: 0;
+}
+
+ol,
+ul,
+menu {
+ margin: 0;
+ padding: 0;
+ list-style: none;
+}
+
+/*
+Reset default styling for dialogs.
+*/
+dialog {
+ padding: 0;
+}
+
+/*
+Prevent resizing textareas horizontally by default.
+*/
+
+textarea {
+ resize: vertical;
+}
+
+/*
+1. Reset the default placeholder opacity in Firefox. (https://github.com/tailwindlabs/tailwindcss/issues/3300)
+2. Set the default placeholder color to the user's configured gray 400 color.
+*/
+
+input::placeholder,
+textarea::placeholder {
+ opacity: 1; /* 1 */
+}
+
+/*
+Set the default cursor for buttons.
+*/
+
+button,
+[role="button"] {
+ cursor: pointer;
+}
+
+/*
+Make sure disabled buttons don't get the pointer cursor.
+*/
+:disabled {
+ cursor: default;
+}
+
+/*
+1. Make replaced elements `display: block` by default. (https://github.com/mozdevs/cssremedy/issues/14)
+2. Add `vertical-align: middle` to align replaced elements more sensibly by default. (https://github.com/jensimmons/cssremedy/issues/14#issuecomment-634934210)
+ This can trigger a poorly considered lint error in some tools but is included by design.
+*/
+
+img,
+svg,
+video,
+canvas,
+audio,
+iframe,
+embed,
+object {
+ display: block; /* 1 */
+}
+
+/*
+Constrain images and videos to the parent width and preserve their intrinsic aspect ratio. (https://github.com/mozdevs/cssremedy/issues/14)
+*/
+
+img,
+video {
+ max-width: 100%;
+ height: auto;
+}
+
+/* Make elements with the HTML hidden attribute stay hidden by default */
+[hidden] {
+ display: none;
+}
diff --git a/frontend/src/theme/global.css b/frontend/src/theme/global.css
new file mode 100644
index 0000000..9be3745
--- /dev/null
+++ b/frontend/src/theme/global.css
@@ -0,0 +1,15 @@
+/* editor */
+@import 'react-quill/dist/quill.snow.css';
+
+/* simplebar */
+@import 'simplebar-react/dist/simplebar.min.css';
+
+/* 将 Tailwind CSS 的基础样式(也称为 "base" 样式)导入到当前文件中,包括一些基本的 HTML 元素样式、重置元素默认样式等。 */
+@import 'tailwindcss/base';
+
+/* 导入 Tailwind CSS 的组件样式,包括预定义的按钮、表格、表单、卡片等组件样式。 */
+@import 'tailwindcss/components';
+
+/* 导入 Tailwind CSS 的实用类,这些类通常用于添加与布局、间距、响应式设计等相关的样式,使得可以快速构建出复杂的页面 */
+@import 'tailwindcss/utilities';
+
diff --git a/frontend/src/theme/hooks/index.ts b/frontend/src/theme/hooks/index.ts
new file mode 100644
index 0000000..107354b
--- /dev/null
+++ b/frontend/src/theme/hooks/index.ts
@@ -0,0 +1,2 @@
+export { useThemeToken } from './use-theme-token';
+export { useResponsive } from './use-reponsive';
diff --git a/frontend/src/theme/hooks/use-reponsive.ts b/frontend/src/theme/hooks/use-reponsive.ts
new file mode 100644
index 0000000..9532dc6
--- /dev/null
+++ b/frontend/src/theme/hooks/use-reponsive.ts
@@ -0,0 +1,32 @@
+import { Grid, theme } from 'antd';
+import { Breakpoint, ScreenMap, ScreenSizeMap } from 'antd/es/_util/responsiveObserver';
+
+const { useBreakpoint } = Grid;
+
+export function useResponsive() {
+ const {
+ token: { screenXS, screenSM, screenMD, screenLG, screenXL, screenXXL },
+ } = theme.useToken();
+ const screenArray: Breakpoint[] = ['xs', 'sm', 'md', 'lg', 'xl', 'xxl'];
+
+ const screenEnum: ScreenSizeMap = {
+ xs: screenXS,
+ sm: screenSM,
+ md: screenMD,
+ lg: screenLG,
+ xl: screenXL,
+ xxl: screenXXL,
+ };
+ const screenMap: ScreenMap = useBreakpoint();
+
+ const currentScrren = screenArray.findLast((item) => {
+ const result = screenMap[item];
+ return result === true;
+ });
+
+ return {
+ screenEnum,
+ screenMap,
+ currentScrren,
+ };
+}
diff --git a/frontend/src/theme/hooks/use-theme-token.ts b/frontend/src/theme/hooks/use-theme-token.ts
new file mode 100644
index 0000000..52ac742
--- /dev/null
+++ b/frontend/src/theme/hooks/use-theme-token.ts
@@ -0,0 +1,7 @@
+import { theme } from 'antd';
+import { useMemo } from 'react';
+
+export function useThemeToken() {
+ const { token } = theme.useToken();
+ return useMemo(() => token, [token]);
+}
diff --git a/frontend/src/theme/index.css b/frontend/src/theme/index.css
new file mode 100644
index 0000000..a5fdd39
--- /dev/null
+++ b/frontend/src/theme/index.css
@@ -0,0 +1,5 @@
+/* 自定义重置样式 */
+@import './base.css';
+
+/* 第三方库样式 */
+@import './global.css';
diff --git a/frontend/src/utils/copy.ts b/frontend/src/utils/copy.ts
new file mode 100644
index 0000000..79a798d
--- /dev/null
+++ b/frontend/src/utils/copy.ts
@@ -0,0 +1,18 @@
+import {message} from "antd";
+
+export const onCopy = (text?: string,t?: any, e?: any) => {
+ if (!text) {
+ return;
+ }
+ e?.preventDefault();
+ e?.stopPropagation();
+ try {
+ navigator.clipboard.writeText(text);
+ // 提示复制成功
+ message.success(t('token.copySuccess'));
+ } catch (e) {
+ console.error('copy failed', e);
+ // 选定文本
+ e.target.select();
+ }
+}
diff --git a/frontend/src/utils/format-number.ts b/frontend/src/utils/format-number.ts
new file mode 100644
index 0000000..0212fbe
--- /dev/null
+++ b/frontend/src/utils/format-number.ts
@@ -0,0 +1,38 @@
+// https://numeraljs.com/
+import numeral from 'numeral';
+
+type InputValue = string | number | null | undefined;
+
+export function fNumber(number: InputValue) {
+ return numeral(number).format();
+}
+
+export function fCurrency(number: InputValue) {
+ const format = number ? numeral(number).format('$0,0.00') : '';
+
+ return result(format, '.00');
+}
+
+export function fPercent(number: InputValue) {
+ const format = number ? numeral(Number(number) / 100).format('0.0%') : '';
+
+ return result(format, '.0');
+}
+
+export function fShortenNumber(number: InputValue) {
+ const format = number ? numeral(number).format('0.00a') : '';
+
+ return result(format, '.00');
+}
+
+export function fBytes(number: InputValue) {
+ const format = number ? numeral(number).format('0.0 b') : '';
+
+ return result(format, '.0');
+}
+
+function result(format: string, key = '.00') {
+ const isInteger = format.includes(key);
+
+ return isInteger ? format.replace(key, '') : format;
+}
diff --git a/frontend/src/utils/hcaptcha.d.ts b/frontend/src/utils/hcaptcha.d.ts
new file mode 100644
index 0000000..cac8b91
--- /dev/null
+++ b/frontend/src/utils/hcaptcha.d.ts
@@ -0,0 +1,12 @@
+declare module '@hcaptcha/react-hcaptcha' {
+ import React from 'react';
+
+ interface HCaptchaProps {
+ sitekey: string;
+ onVerify: (token: string) => void;
+ style?: React.CSSProperties;
+ // 根据需要添加更多属性
+ }
+
+ export default class HCaptcha extends React.Component {}
+}
diff --git a/frontend/src/utils/highlight.ts b/frontend/src/utils/highlight.ts
new file mode 100644
index 0000000..59f9324
--- /dev/null
+++ b/frontend/src/utils/highlight.ts
@@ -0,0 +1,19 @@
+import 'highlight.js/styles/base16/tomorrow-night.css';
+
+import hljs from 'highlight.js';
+
+// ----------------------------------------------------------------------
+
+declare global {
+ interface Window {
+ hljs: any;
+ }
+}
+
+hljs.configure({
+ languages: ['javascript', 'sh', 'bash', 'html', 'scss', 'css', 'json'],
+});
+
+if (typeof window !== 'undefined') {
+ window.hljs = hljs;
+}
diff --git a/frontend/src/utils/storage.ts b/frontend/src/utils/storage.ts
new file mode 100644
index 0000000..507ec96
--- /dev/null
+++ b/frontend/src/utils/storage.ts
@@ -0,0 +1,28 @@
+import { StorageEnum } from '#/enum';
+
+export const getItem = (key: StorageEnum): T | null => {
+ let value = null;
+ try {
+ const result = window.localStorage.getItem(key);
+ if (result) {
+ value = JSON.parse(result);
+ }
+ } catch (error) {
+ console.error(error);
+ }
+ return value;
+};
+
+export const getStringItem = (key: StorageEnum): string | null => {
+ return localStorage.getItem(key);
+};
+
+export const setItem = (key: StorageEnum, value: T): void => {
+ localStorage.setItem(key, JSON.stringify(value));
+};
+export const removeItem = (key: StorageEnum): void => {
+ localStorage.removeItem(key);
+};
+export const clearItems = () => {
+ localStorage.clear();
+};
diff --git a/frontend/src/utils/tree.ts b/frontend/src/utils/tree.ts
new file mode 100644
index 0000000..a6b4ed4
--- /dev/null
+++ b/frontend/src/utils/tree.ts
@@ -0,0 +1,13 @@
+import { chain } from 'ramda';
+
+/**
+ * Flatten an array containing a tree structure
+ * @param {T[]} trees - An array containing a tree structure
+ * @returns {T[]} - Flattened array
+ */
+export function flattenTrees(trees: T[] = []): T[] {
+ return chain((node) => {
+ const children = node.children || [];
+ return [node, ...flattenTrees(children)];
+ }, trees);
+}
diff --git a/frontend/src/vite-env.d.ts b/frontend/src/vite-env.d.ts
new file mode 100644
index 0000000..11f02fe
--- /dev/null
+++ b/frontend/src/vite-env.d.ts
@@ -0,0 +1 @@
+///
diff --git a/frontend/tailwind.config.js b/frontend/tailwind.config.js
new file mode 100644
index 0000000..5994dd3
--- /dev/null
+++ b/frontend/tailwind.config.js
@@ -0,0 +1,58 @@
+/** @type {import('tailwindcss').Config} */
+export default {
+ // 使用 "class" 模式时,Tailwind 会将 "dark" 类添加到根元素(通常是 元素)上,以指示页面当前处于深色模式
+ darkMode: 'class',
+ // 通过配置 content,Tailwind CSS 将会检索和构建包含需要的 CSS 样式的文件,并生成最终的 CSS 输出文件
+ content: ['./index.html', './src/**/*.{js,ts,jsx,tsx}'],
+ theme: {
+ screens: {
+ xs: '480px',
+ sm: '576px',
+ md: '768px',
+ lg: '992px',
+ xl: '1400px',
+ '2xl': '1600px',
+ },
+ colors: {
+ black: '#000000',
+ green: '#00A76F',
+ blue: '#1fb6ff',
+ purple: '#7e5bef',
+ pink: '#ff49db',
+ orange: '#ff7849',
+ yellow: '#ffc82c',
+ gray: '#637381',
+ hover: '#63738114',
+
+ success: '#22c55e',
+ warning: '#ff7849',
+ error: '#ff5630',
+ info: '#00b8d9',
+
+ code: '#d63384',
+
+ 'gray-100': '#F9FAFB',
+ 'gray-200': '#F4F6F8',
+ 'gray-300': '#DFE3E8',
+ 'gray-400': '#C4CDD5',
+ 'gray-500': '#F9FAFB',
+ 'gray-600': '#637381',
+ 'gray-700': '#454F5B',
+ 'gray-800': '#212B36',
+ 'gray-900': '#161C24',
+ },
+ extend: {
+ transitionProperty: {
+ height: 'height',
+ },
+ animation: {
+ 'spin-slow': 'spin 8s linear infinite',
+ },
+ },
+ },
+ corePlugins: {
+ // Remove the Tailwind CSS preflight styles so it can use custom base style (src/theme/base.css)
+ preflight: false, // https://tailwindcss.com/docs/preflight#disabling-preflight
+ },
+ plugins: [],
+};
diff --git a/frontend/tsconfig.json b/frontend/tsconfig.json
new file mode 100644
index 0000000..463b412
--- /dev/null
+++ b/frontend/tsconfig.json
@@ -0,0 +1,46 @@
+{
+ "compilerOptions": {
+ "target": "ESNext", // 编译后代码的目标版本(ECMAScript)。
+ "module": "ESNext", // 使用的模块系统类型。ESNext 支持更多特性,比如动态导入等。
+ "lib": ["ESNext", "DOM", "DOM.Iterable"], // 要包含的库文件。DOM、DOM.Iterable 和 ESNext 库文件是 TypeScript 开发中比较基础的依赖。
+ "useDefineForClassFields": true,
+ "skipLibCheck": true, // 跳过编译器对引入库文件的检查,以加快构建速度
+ "allowJs": true, // 允许编译JavaScript文件
+
+ /* Bundler mode */
+ "moduleResolution": "bundler",
+ "sourceMap": true, // 输出 *.map 文件提供源码映射
+ "declaration": true, // 是否生成声明文件(.d.ts)。
+ "preserveWatchOutput": true, // 该选项允许在监视模式下保留先前编译的文件,从而避免增量编译时删除更改的文件导致的重新编译。
+ "removeComments": true, // 是否移除注释。可以提高编译速度并减小生成文件的大小
+ "allowImportingTsExtensions": true, // 允许在 import 导入语句中导入 .ts 或 .tsx 扩展名的模块,而不需要显式地指定扩展名
+ "resolveJsonModule": true, // 允许导入json模块
+ "isolatedModules": true, // 让typescript处理每个文件时都是独立的单元(可加快编译速度)
+ "noEmit": true, // 编译器不生成任何JS文件
+ "jsx": "react-jsx",
+ // 使 TypeScript 可以更好地与 CommonJS 模块兼容。在使用 CommonJS 模块系统时,导出的模块将会被包装在一个对象中,这是因为 CommonJS 使用的是类似于 module.exports 的语法来导出模块。
+ // 而某些工具和库可能需要以 ES6 模块的方式导入这些 CommonJS 模块,这时候就可以开启 esModuleInterop 选项。
+ // 开启后,在导入 CommonJS 模块时不需要再使用默认导出才能正确引入,也不需要手动处理 require() 和 module.exports。
+ // 这使得导入和使用 CommonJS 模块的过程变得更加简洁方便。
+ "esModuleInterop": true,
+
+ /* Linting */
+ "strict": true, // 启用所有严格类型检查选项
+ "strictNullChecks": true, // 对空值进行严格检查
+ "noImplicitAny": true, // 禁止隐式any类型
+ "noUnusedLocals": true, // 消除未使用变量产生的警告
+ "noUnusedParameters": true, // 消除未使用参数产生的警告
+ "noFallthroughCasesInSwitch": true, // 避免 switch 语句掉入陷阱
+ "useUnknownInCatchVariables": false, // 为 true 时,在 catch 块中声明的变量类型会被视为 unknown 而非默认的 any。
+
+ "baseUrl": ".",
+ /* alias */
+ "paths": {
+ "@/*": ["src/*"],
+ "#/*": ["types/*"]
+ }
+ },
+ "include": ["src", "test", "types/**/*.ts", "**.ts", "*.json", "**.*js"],
+ "exclude": ["node_modules", "dist"],
+ "references": [{ "path": "./tsconfig.node.json" }]
+}
diff --git a/frontend/tsconfig.node.json b/frontend/tsconfig.node.json
new file mode 100644
index 0000000..42872c5
--- /dev/null
+++ b/frontend/tsconfig.node.json
@@ -0,0 +1,10 @@
+{
+ "compilerOptions": {
+ "composite": true,
+ "skipLibCheck": true,
+ "module": "ESNext",
+ "moduleResolution": "bundler",
+ "allowSyntheticDefaultImports": true
+ },
+ "include": ["vite.config.ts"]
+}
diff --git a/frontend/types/api.ts b/frontend/types/api.ts
new file mode 100644
index 0000000..7b13cc3
--- /dev/null
+++ b/frontend/types/api.ts
@@ -0,0 +1,5 @@
+export interface Result {
+ status: number;
+ message: string;
+ data?: T;
+}
diff --git a/frontend/types/entity.ts b/frontend/types/entity.ts
new file mode 100644
index 0000000..687eba0
--- /dev/null
+++ b/frontend/types/entity.ts
@@ -0,0 +1,100 @@
+import { BasicStatus, PermissionType } from './enum';
+
+export interface UserToken {
+ accessToken?: string;
+}
+
+export interface UserInfo {
+ id: string;
+ email: string;
+ username: string;
+ password?: string;
+ avatar?: string;
+ role?: Role;
+ status?: BasicStatus;
+ permissions?: Permission[];
+}
+
+export interface Organization {
+ id: string;
+ name: string;
+ status: 'enable' | 'disable';
+ desc?: string;
+ order?: number;
+ children?: Organization[];
+}
+
+export interface Permission {
+ id: string;
+ parentId: string;
+ name: string;
+ label: string;
+ type: PermissionType;
+ route: string;
+ status?: BasicStatus;
+ order?: number;
+ icon?: string;
+ component?: string;
+ hide?: boolean;
+ frameSrc?: string;
+ newFeature?: boolean;
+ children?: Permission[];
+}
+
+export interface Role {
+ id: string;
+ name: string;
+ label: string;
+ status: BasicStatus;
+ order?: number;
+ desc?: string;
+ permission?: Permission[];
+}
+
+export interface Account {
+ id: number;
+ email: string;
+ password: string;
+ sessionToken?: string;
+ accessToken?: string;
+ createTime?: string;
+ updateTime?: string;
+ shared?: number;
+ shareList?: string;
+ refreshToken?: string;
+}
+
+export interface Share {
+ id?: number;
+ accountId: number;
+ email?: string;
+ uniqueName: string;
+ password: string;
+ shareToken?: string;
+ comment?: string;
+ refreshEveryday?: boolean;
+ expiresIn?: number;
+ siteLimit?: string;
+ gpt35Limit?: number;
+ gpt4Limit?: number;
+ showUserinfo?: boolean;
+ showConversations?: boolean;
+}
+
+// share默认值
+export const defaultShare: Share = {
+ id: undefined,
+ accountId: -1,
+ email: '',
+ uniqueName: '',
+ password: '',
+ shareToken: '',
+ refreshEveryday: false,
+ comment: '',
+ expiresIn: 0,
+ siteLimit: '',
+ gpt35Limit: -1,
+ gpt4Limit: -1,
+ showUserinfo: false,
+ showConversations: false
+};
diff --git a/frontend/types/enum.ts b/frontend/types/enum.ts
new file mode 100644
index 0000000..cf23187
--- /dev/null
+++ b/frontend/types/enum.ts
@@ -0,0 +1,58 @@
+export enum BasicStatus {
+ DISABLE,
+ ENABLE,
+}
+
+export enum ResultEnum {
+ SUCCESS = 0,
+ ERROR = -1,
+ TIMEOUT = 401,
+}
+
+export enum StorageEnum {
+ User = 'user',
+ Token = 'token',
+ Settings = 'settings',
+ I18N = 'i18nextLng',
+}
+
+export enum ThemeMode {
+ Light = 'light',
+ Dark = 'dark',
+}
+
+export enum ThemeLayout {
+ Vertical = 'vertical',
+ Horizontal = 'horizontal',
+ Mini = 'mini',
+}
+
+export enum ThemeColorPresets {
+ Default = 'default',
+ Cyan = 'cyan',
+ Purple = 'purple',
+ Blue = 'blue',
+ Orange = 'orange',
+ Red = 'red',
+}
+
+export enum LocalEnum {
+ en_US = 'en_US',
+ zh_CN = 'zh_CN',
+}
+
+export enum MultiTabOperation {
+ FULLSCREEN = 'fullscreen',
+ REFRESH = 'refresh',
+ CLOSE = 'close',
+ CLOSEOTHERS = 'closeOthers',
+ CLOSEALL = 'closeAll',
+ CLOSELEFT = 'closeLeft',
+ CLOSERIGHT = 'closeRight',
+}
+
+export enum PermissionType {
+ CATALOGUE,
+ MENU,
+ BUTTON,
+}
diff --git a/frontend/types/router.ts b/frontend/types/router.ts
new file mode 100644
index 0000000..3b561ba
--- /dev/null
+++ b/frontend/types/router.ts
@@ -0,0 +1,50 @@
+import { ReactNode } from 'react';
+import { RouteObject } from 'react-router-dom';
+
+export interface RouteMeta {
+ /**
+ * antd menu selectedKeys
+ */
+ key: string;
+ /**
+ * menu label, i18n
+ */
+ label: string;
+ /**
+ * menu prefix icon
+ */
+ icon?: ReactNode;
+ /**
+ * menu suffix icon
+ */
+ suffix?: ReactNode;
+ /**
+ * hide in menu
+ */
+ hideMenu?: boolean;
+ /**
+ * hide in multi tab
+ */
+ hideTab?: boolean;
+ /**
+ * disable in menu
+ */
+ disabled?: boolean;
+ /**
+ * react router outlet
+ */
+ outlet?: any;
+ /**
+ * use to refresh tab
+ */
+ timeStamp?: string;
+ /**
+ * external link and iframe need
+ */
+ frameSrc?: string;
+}
+export type AppRouteObject = {
+ order?: number;
+ meta?: RouteMeta;
+ children?: AppRouteObject[];
+} & Omit;
diff --git a/frontend/vite.config.ts b/frontend/vite.config.ts
new file mode 100644
index 0000000..2ea4752
--- /dev/null
+++ b/frontend/vite.config.ts
@@ -0,0 +1,70 @@
+import path from 'path';
+
+import react from '@vitejs/plugin-react';
+import { visualizer } from 'rollup-plugin-visualizer';
+import { defineConfig } from 'vite';
+import { createSvgIconsPlugin } from 'vite-plugin-svg-icons';
+import tsconfigPaths from 'vite-tsconfig-paths';
+import { inspectorServer } from '@react-dev-inspector/vite-plugin'
+
+// https://vitejs.dev/config/
+export default defineConfig({
+ base: './',
+ esbuild: {
+ // drop: ['console', 'debugger'],
+ },
+ css: {
+ // 开css sourcemap方便找css
+ devSourcemap: true,
+ },
+ plugins: [
+ react(),
+ // 同步tsconfig.json的path设置alias
+ tsconfigPaths(),
+ createSvgIconsPlugin({
+ // 指定需要缓存的图标文件夹
+ iconDirs: [path.resolve(process.cwd(), 'src/assets/icons')],
+ // 指定symbolId格式
+ symbolId: 'icon-[dir]-[name]',
+ }),
+ visualizer({
+ open: true,
+ }),
+ inspectorServer(),
+ ],
+ server: {
+ // 自动打开浏览器
+ open: true,
+ host: true,
+ port: 3001,
+ proxy: {
+ '/api': {
+ target: 'http://localhost:8080',
+ changeOrigin: true,
+ // rewrite: (path) => path.replace(/^\/api/, ''),
+ },
+ },
+ },
+ build: {
+ target: 'esnext',
+ minify: 'terser',
+ // rollupOptions: {
+ // output: {
+ // manualChunks(id) {
+ // if (id.includes('node_modules')) {
+ // // 让每个插件都打包成独立的文件
+ // return id.toString().split('node_modules/')[1].split('/')[0].toString();
+ // }
+ // return null;
+ // },
+ // },
+ // },
+ terserOptions: {
+ compress: {
+ // 生产环境移除console
+ drop_console: true,
+ drop_debugger: true,
+ },
+ },
+ },
+});
diff --git a/fs.go b/fs.go
new file mode 100644
index 0000000..7de8a76
--- /dev/null
+++ b/fs.go
@@ -0,0 +1,6 @@
+package PandoraHelper
+
+import "embed"
+
+//go:embed frontend/dist
+var EmbedFrontendFS embed.FS
diff --git a/go.mod b/go.mod
index 9d93f74..816aa1c 100644
--- a/go.mod
+++ b/go.mod
@@ -5,16 +5,17 @@ go 1.19
require (
github.com/DATA-DOG/go-sqlmock v1.5.0
github.com/duke-git/lancet/v2 v2.3.0
+ github.com/gin-contrib/static v1.1.1
github.com/gin-gonic/gin v1.9.1
github.com/glebarez/sqlite v1.11.0
github.com/go-co-op/gocron v1.28.2
+ github.com/go-resty/resty/v2 v2.12.0
github.com/golang-jwt/jwt/v5 v5.0.0
github.com/golang/mock v1.6.0
github.com/google/wire v0.5.0
- github.com/redis/go-redis/v9 v9.0.5
github.com/sony/sonyflake v1.1.0
github.com/spf13/viper v1.16.0
- github.com/stretchr/testify v1.8.4
+ github.com/stretchr/testify v1.9.0
github.com/swaggo/files v1.0.1
github.com/swaggo/gin-swagger v1.6.0
github.com/swaggo/swag v1.16.2
@@ -29,14 +30,13 @@ require (
require (
github.com/KyleBanks/depth v1.2.1 // indirect
- github.com/bytedance/sonic v1.9.1 // indirect
- github.com/cespare/xxhash/v2 v2.2.0 // indirect
- github.com/chenzhuoyu/base64x v0.0.0-20221115062448-fe3a3abad311 // indirect
+ github.com/bytedance/sonic v1.11.3 // indirect
+ github.com/chenzhuoyu/base64x v0.0.0-20230717121745-296ad89f973d // indirect
+ github.com/chenzhuoyu/iasm v0.9.1 // indirect
github.com/davecgh/go-spew v1.1.1 // indirect
- github.com/dgryski/go-rendezvous v0.0.0-20200823014737-9f7001d12a5f // indirect
github.com/dustin/go-humanize v1.0.1 // indirect
github.com/fsnotify/fsnotify v1.6.0 // indirect
- github.com/gabriel-vasile/mimetype v1.4.2 // indirect
+ github.com/gabriel-vasile/mimetype v1.4.3 // indirect
github.com/gin-contrib/sse v0.1.0 // indirect
github.com/glebarez/go-sqlite v1.21.2 // indirect
github.com/go-openapi/jsonpointer v0.20.0 // indirect
@@ -45,8 +45,7 @@ require (
github.com/go-openapi/swag v0.22.4 // indirect
github.com/go-playground/locales v0.14.1 // indirect
github.com/go-playground/universal-translator v0.18.1 // indirect
- github.com/go-playground/validator/v10 v10.14.0 // indirect
- github.com/go-resty/resty/v2 v2.12.0 // indirect
+ github.com/go-playground/validator/v10 v10.19.0 // indirect
github.com/go-sql-driver/mysql v1.7.0 // indirect
github.com/goccy/go-json v0.10.2 // indirect
github.com/golang/protobuf v1.5.3 // indirect
@@ -59,15 +58,15 @@ require (
github.com/jinzhu/now v1.1.5 // indirect
github.com/josharian/intern v1.0.0 // indirect
github.com/json-iterator/go v1.1.12 // indirect
- github.com/klauspost/cpuid/v2 v2.2.4 // indirect
- github.com/leodido/go-urn v1.2.4 // indirect
+ github.com/klauspost/cpuid/v2 v2.2.7 // indirect
+ github.com/leodido/go-urn v1.4.0 // indirect
github.com/magiconair/properties v1.8.7 // indirect
github.com/mailru/easyjson v0.7.7 // indirect
- github.com/mattn/go-isatty v0.0.19 // indirect
+ github.com/mattn/go-isatty v0.0.20 // indirect
github.com/mitchellh/mapstructure v1.5.0 // indirect
github.com/modern-go/concurrent v0.0.0-20180306012644-bacd9c7ef1dd // indirect
github.com/modern-go/reflect2 v1.0.2 // indirect
- github.com/pelletier/go-toml/v2 v2.0.8 // indirect
+ github.com/pelletier/go-toml/v2 v2.2.0 // indirect
github.com/pmezard/go-difflib v1.0.0 // indirect
github.com/remyoudompheng/bigfft v0.0.0-20230129092748-24d4a6f8daec // indirect
github.com/robfig/cron/v3 v3.0.1 // indirect
@@ -77,17 +76,17 @@ require (
github.com/spf13/pflag v1.0.5 // indirect
github.com/subosito/gotenv v1.4.2 // indirect
github.com/twitchyliquid64/golang-asm v0.15.1 // indirect
- github.com/ugorji/go/codec v1.2.11 // indirect
+ github.com/ugorji/go/codec v1.2.12 // indirect
go.uber.org/atomic v1.11.0 // indirect
go.uber.org/multierr v1.11.0 // indirect
- golang.org/x/arch v0.3.0 // indirect
+ golang.org/x/arch v0.7.0 // indirect
golang.org/x/exp v0.0.0-20221208152030-732eee02a75a // indirect
golang.org/x/net v0.22.0 // indirect
golang.org/x/sys v0.18.0 // indirect
golang.org/x/text v0.14.0 // indirect
golang.org/x/tools v0.12.0 // indirect
google.golang.org/genproto v0.0.0-20230410155749-daa745c078e1 // indirect
- google.golang.org/protobuf v1.30.0 // indirect
+ google.golang.org/protobuf v1.33.0 // indirect
gopkg.in/ini.v1 v1.67.0 // indirect
gopkg.in/yaml.v3 v3.0.1 // indirect
modernc.org/libc v1.22.5 // indirect
diff --git a/go.sum b/go.sum
index 7a7032d..8974cd9 100644
--- a/go.sum
+++ b/go.sum
@@ -42,19 +42,18 @@ github.com/DATA-DOG/go-sqlmock v1.5.0 h1:Shsta01QNfFxHCfpW6YH2STWB0MudeXXEWMr20O
github.com/DATA-DOG/go-sqlmock v1.5.0/go.mod h1:f/Ixk793poVmq4qj/V1dPUg2JEAKC73Q5eFN3EC/SaM=
github.com/KyleBanks/depth v1.2.1 h1:5h8fQADFrWtarTdtDudMmGsC7GPbOAu6RVB3ffsVFHc=
github.com/KyleBanks/depth v1.2.1/go.mod h1:jzSb9d0L43HxTQfT+oSA1EEp2q+ne2uh6XgeJcm8brE=
-github.com/bsm/ginkgo/v2 v2.7.0 h1:ItPMPH90RbmZJt5GtkcNvIRuGEdwlBItdNVoyzaNQao=
-github.com/bsm/gomega v1.26.0 h1:LhQm+AFcgV2M0WyKroMASzAzCAJVpAxQXv4SaI9a69Y=
github.com/bytedance/sonic v1.5.0/go.mod h1:ED5hyg4y6t3/9Ku1R6dU/4KyJ48DZ4jPhfY1O2AihPM=
-github.com/bytedance/sonic v1.9.1 h1:6iJ6NqdoxCDr6mbY8h18oSO+cShGSMRGCEo7F2h0x8s=
-github.com/bytedance/sonic v1.9.1/go.mod h1:i736AoUSYt75HyZLoJW9ERYxcy6eaN6h4BZXU064P/U=
-github.com/carlmjohnson/requests v0.23.5 h1:NPANcAofwwSuC6SIMwlgmHry2V3pLrSqRiSBKYbNHHA=
-github.com/carlmjohnson/requests v0.23.5/go.mod h1:zG9P28thdRnN61aD7iECFhH5iGGKX2jIjKQD9kqYH+o=
+github.com/bytedance/sonic v1.10.0-rc/go.mod h1:ElCzW+ufi8qKqNW0FY314xriJhyJhuoJ3gFZdAHF7NM=
+github.com/bytedance/sonic v1.11.3 h1:jRN+yEjakWh8aK5FzrciUHG8OFXK+4/KrAX/ysEtHAA=
+github.com/bytedance/sonic v1.11.3/go.mod h1:iZcSUejdk5aukTND/Eu/ivjQuEL0Cu9/rf50Hi0u/g4=
github.com/census-instrumentation/opencensus-proto v0.2.1/go.mod h1:f6KPmirojxKA12rnyqOA5BBL4O983OfeGPqjHWSTneU=
-github.com/cespare/xxhash/v2 v2.2.0 h1:DC2CZ1Ep5Y4k3ZQ899DldepgrayRUGE6BBZ/cd9Cj44=
-github.com/cespare/xxhash/v2 v2.2.0/go.mod h1:VGX0DQ3Q6kWi7AoAeZDth3/j3BFtOZR5XLFGgcrjCOs=
github.com/chenzhuoyu/base64x v0.0.0-20211019084208-fb5309c8db06/go.mod h1:DH46F32mSOjUmXrMHnKwZdA8wcEefY7UVqBKYGjpdQY=
-github.com/chenzhuoyu/base64x v0.0.0-20221115062448-fe3a3abad311 h1:qSGYFH7+jGhDF8vLC+iwCD4WpbV1EBDSzWkJODFLams=
github.com/chenzhuoyu/base64x v0.0.0-20221115062448-fe3a3abad311/go.mod h1:b583jCggY9gE99b6G5LEC39OIiVsWj+R97kbl5odCEk=
+github.com/chenzhuoyu/base64x v0.0.0-20230717121745-296ad89f973d h1:77cEq6EriyTZ0g/qfRdp61a3Uu/AWrgIq2s0ClJV1g0=
+github.com/chenzhuoyu/base64x v0.0.0-20230717121745-296ad89f973d/go.mod h1:8EPpVsBuRksnlj1mLy4AWzRNQYxauNi62uWcE3to6eA=
+github.com/chenzhuoyu/iasm v0.9.0/go.mod h1:Xjy2NpN3h7aUqeqM+woSuuvxmIe6+DDsiNLIrkAmYog=
+github.com/chenzhuoyu/iasm v0.9.1 h1:tUHQJXo3NhBqw6s33wkGn9SP3bvrWLdlVIJ3hQBL7P0=
+github.com/chenzhuoyu/iasm v0.9.1/go.mod h1:Xjy2NpN3h7aUqeqM+woSuuvxmIe6+DDsiNLIrkAmYog=
github.com/chzyer/logex v1.1.10/go.mod h1:+Ywpsq7O8HXn0nuIou7OrIPyXbp3wmkHB+jjWRnGsAI=
github.com/chzyer/readline v0.0.0-20180603132655-2972be24d48e/go.mod h1:nSuG5e5PlCu98SY8svDHJxuZscDgtXS6KTTbou5AhLI=
github.com/chzyer/test v0.0.0-20180213035817-a1ea475d72b1/go.mod h1:Q3SI9o4m/ZMnBNeIyt5eFwwo7qiLfzFZmjNmxjkiQlU=
@@ -66,8 +65,6 @@ github.com/creack/pty v1.1.9/go.mod h1:oKZEueFk5CKHvIhNR5MUki03XCEU+Q6VDXinZuGJ3
github.com/davecgh/go-spew v1.1.0/go.mod h1:J7Y8YcW2NihsgmVo/mv3lAwl/skON4iLHjSsI+c5H38=
github.com/davecgh/go-spew v1.1.1 h1:vj9j/u1bqnvCEfJOwUhtlOARqs3+rkHYY13jYWTU97c=
github.com/davecgh/go-spew v1.1.1/go.mod h1:J7Y8YcW2NihsgmVo/mv3lAwl/skON4iLHjSsI+c5H38=
-github.com/dgryski/go-rendezvous v0.0.0-20200823014737-9f7001d12a5f h1:lO4WD4F/rVNCu3HqELle0jiPLLBs70cWOduZpkS1E78=
-github.com/dgryski/go-rendezvous v0.0.0-20200823014737-9f7001d12a5f/go.mod h1:cuUVRXasLTGF7a8hSLbxyZXjz+1KgoB3wDUb6vlszIc=
github.com/duke-git/lancet/v2 v2.3.0 h1:Ztie0qOnC4QgGYYqmpmQxbxkPcm54kqFXj1bwhiV8zg=
github.com/duke-git/lancet/v2 v2.3.0/go.mod h1:zGa2R4xswg6EG9I6WnyubDbFO/+A/RROxIbXcwryTsc=
github.com/dustin/go-humanize v1.0.1 h1:GzkhY7T5VNhEkwH0PVJgjz+fX1rhBrR7pRT3mDkpeCY=
@@ -81,11 +78,13 @@ github.com/envoyproxy/protoc-gen-validate v0.1.0/go.mod h1:iSmxcyjqTsJpI2R4NaDN7
github.com/frankban/quicktest v1.14.4 h1:g2rn0vABPOOXmZUj+vbmUp0lPoXEMuhTpIluN0XL9UY=
github.com/fsnotify/fsnotify v1.6.0 h1:n+5WquG0fcWoWp6xPWfHdbskMCQaFnG6PfBrh1Ky4HY=
github.com/fsnotify/fsnotify v1.6.0/go.mod h1:sl3t1tCWJFWoRz9R8WJCbQihKKwmorjAbSClcnxKAGw=
-github.com/gabriel-vasile/mimetype v1.4.2 h1:w5qFW6JKBz9Y393Y4q372O9A7cUSequkh1Q7OhCmWKU=
-github.com/gabriel-vasile/mimetype v1.4.2/go.mod h1:zApsH/mKG4w07erKIaJPFiX0Tsq9BFQgN3qGY5GnNgA=
+github.com/gabriel-vasile/mimetype v1.4.3 h1:in2uUcidCuFcDKtdcBxlR0rJ1+fsokWf+uqxgUFjbI0=
+github.com/gabriel-vasile/mimetype v1.4.3/go.mod h1:d8uq/6HKRL6CGdk+aubisF/M5GcPfT7nKyLpA0lbSSk=
github.com/gin-contrib/gzip v0.0.6 h1:NjcunTcGAj5CO1gn4N8jHOSIeRFHIbn51z6K+xaN4d4=
github.com/gin-contrib/sse v0.1.0 h1:Y/yl/+YNO8GZSjAhjMsSuLt29uWRFHdHYUb5lYOV9qE=
github.com/gin-contrib/sse v0.1.0/go.mod h1:RHrZQHXnP2xjPF+u1gW/2HnVO7nvIa9PG3Gm+fLHvGI=
+github.com/gin-contrib/static v1.1.1 h1:XEvBd4DDLG1HBlyPBQU1XO8NlTpw6mgdqcPteetYA5k=
+github.com/gin-contrib/static v1.1.1/go.mod h1:yRGmar7+JYvbMLRPIi4H5TVVSBwULfT9vetnVD0IO74=
github.com/gin-gonic/gin v1.9.1 h1:4idEAncQnU5cB7BeOkPtxjfCSye0AAm1R0RVIqJ+Jmg=
github.com/gin-gonic/gin v1.9.1/go.mod h1:hPrL7YrpYKXt5YId3A/Tnip5kqbEAP+KLuI3SUcPTeU=
github.com/glebarez/go-sqlite v1.21.2 h1:3a6LFC4sKahUunAmynQKLZceZCOzUthkRkEAl9gAXWo=
@@ -117,9 +116,8 @@ github.com/go-playground/locales v0.14.1 h1:EWaQ/wswjilfKLTECiXz7Rh+3BjFhfDFKv/o
github.com/go-playground/locales v0.14.1/go.mod h1:hxrqLVvrK65+Rwrd5Fc6F2O76J/NuW9t0sjnWqG1slY=
github.com/go-playground/universal-translator v0.18.1 h1:Bcnm0ZwsGyWbCzImXv+pAJnYK9S473LQFuzCbDbfSFY=
github.com/go-playground/universal-translator v0.18.1/go.mod h1:xekY+UJKNuX9WP91TpwSH2VMlDf28Uj24BCp08ZFTUY=
-github.com/go-playground/validator/v10 v10.14.0 h1:vgvQWe3XCz3gIeFDm/HnTIbj6UGmg/+t63MyGU2n5js=
-github.com/go-playground/validator/v10 v10.14.0/go.mod h1:9iXMNT7sEkjXb0I+enO7QXmzG6QCsPWY4zveKFVRSyU=
-github.com/go-resty/resty/v2 v2.11.0/go.mod h1:iiP/OpA0CkcL3IGt1O0+/SIItFUbkkyw5BGXiVdTu+A=
+github.com/go-playground/validator/v10 v10.19.0 h1:ol+5Fu+cSq9JD7SoSqe04GMI92cbn0+wvQ3bZ8b/AU4=
+github.com/go-playground/validator/v10 v10.19.0/go.mod h1:dbuPbCMFw/DrkbEynArYaCwl3amGuJotoKCe95atGMM=
github.com/go-resty/resty/v2 v2.12.0 h1:rsVL8P90LFvkUYq/V5BTVe203WfRIU4gvcf+yfzJzGA=
github.com/go-resty/resty/v2 v2.12.0/go.mod h1:o0yGPrkS3lOe1+eFajk6kBW8ScXzwU3hD69/gt2yB/0=
github.com/go-sql-driver/mysql v1.7.0 h1:ueSltNNllEqE3qcWBTD0iQd3IpL/6U+mJxLkazJ7YPc=
@@ -220,8 +218,9 @@ github.com/jstemmer/go-junit-report v0.0.0-20190106144839-af01ea7f8024/go.mod h1
github.com/jstemmer/go-junit-report v0.9.1/go.mod h1:Brl9GWCQeLvo8nXZwPNNblvFj/XSXhF0NWZEnDohbsk=
github.com/kisielk/gotool v1.0.0/go.mod h1:XhKaO+MFFWcvkIS/tQcRk01m1F5IRFswLeQ+oQHNcck=
github.com/klauspost/cpuid/v2 v2.0.9/go.mod h1:FInQzS24/EEf25PyTYn52gqo7WaD8xa0213Md/qVLRg=
-github.com/klauspost/cpuid/v2 v2.2.4 h1:acbojRNwl3o09bUq+yDCtZFc1aiwaAAxtcn8YkZXnvk=
-github.com/klauspost/cpuid/v2 v2.2.4/go.mod h1:RVVoqg1df56z8g3pUjL/3lE5UfnlrJX8tyFgg4nqhuY=
+github.com/klauspost/cpuid/v2 v2.2.7 h1:ZWSB3igEs+d0qvnxR/ZBzXVmxkgt8DdzP6m9pfuVLDM=
+github.com/klauspost/cpuid/v2 v2.2.7/go.mod h1:Lcz8mBdAVJIBVzewtcLocK12l3Y+JytZYpaMropDUws=
+github.com/knz/go-libedit v1.10.1/go.mod h1:MZTVkCWyz0oBc7JOWP3wNAzd002ZbM/5hgShxwh4x8M=
github.com/kr/fs v0.1.0/go.mod h1:FFnZGqtBN9Gxj7eW1uZ42v5BccTP0vu6NEaFoC2HwRg=
github.com/kr/pretty v0.1.0/go.mod h1:dAy3ld7l9f0ibDNOQOHHMYYIIbhfbHSm3C4ZsoJORNo=
github.com/kr/pretty v0.2.1/go.mod h1:ipq/a2n7PKx3OHsz4KJII5eveXtPO4qwEXGdVfWzfnI=
@@ -231,8 +230,8 @@ github.com/kr/pty v1.1.1/go.mod h1:pFQYn66WHrOpPYNljwOMqo10TkYh1fy3cYio2l3bCsQ=
github.com/kr/text v0.1.0/go.mod h1:4Jbv+DJW3UT/LiOwJeYQe1efqtUx/iVham/4vfdArNI=
github.com/kr/text v0.2.0 h1:5Nx0Ya0ZqY2ygV366QzturHI13Jq95ApcVaJBhpS+AY=
github.com/kr/text v0.2.0/go.mod h1:eLer722TekiGuMkidMxC/pM04lWEeraHUUmBw8l2grE=
-github.com/leodido/go-urn v1.2.4 h1:XlAE/cm/ms7TE/VMVoduSpNBoyc2dOxHs5MZSwAN63Q=
-github.com/leodido/go-urn v1.2.4/go.mod h1:7ZrI8mTSeBSHl/UaRyKQW1qZeMgak41ANeCNaVckg+4=
+github.com/leodido/go-urn v1.4.0 h1:WT9HwE9SGECu3lg4d/dIA+jxlljEa1/ffXKmRjqdmIQ=
+github.com/leodido/go-urn v1.4.0/go.mod h1:bvxc+MVxLKB4z00jd1z+Dvzr47oO32F/QSNjSBOlFxI=
github.com/magiconair/properties v1.8.7 h1:IeQXZAiQcpL9mgcAe1Nu6cX9LLw6ExEHKjN0VQdvPDY=
github.com/magiconair/properties v1.8.7/go.mod h1:Dhd985XPs7jluiymwWYZ0G4Z61jb3vdS329zhj2hYo0=
github.com/mailru/easyjson v0.0.0-20190614124828-94de47d64c63/go.mod h1:C1wdFJiN94OJF2b5HbByQZoLdCWB1Yqtg26g4irojpc=
@@ -240,8 +239,8 @@ github.com/mailru/easyjson v0.0.0-20190626092158-b2ccc519800e/go.mod h1:C1wdFJiN
github.com/mailru/easyjson v0.7.6/go.mod h1:xzfreul335JAWq5oZzymOObrkdz5UnU4kGfJJLY9Nlc=
github.com/mailru/easyjson v0.7.7 h1:UGYAvKxe3sBsEDzO8ZeWOSlIQfWFlxbzLZe7hwFURr0=
github.com/mailru/easyjson v0.7.7/go.mod h1:xzfreul335JAWq5oZzymOObrkdz5UnU4kGfJJLY9Nlc=
-github.com/mattn/go-isatty v0.0.19 h1:JITubQf0MOLdlGRuRq+jtsDlekdYPia9ZFsB8h/APPA=
-github.com/mattn/go-isatty v0.0.19/go.mod h1:W+V8PltTTMOvKvAeJH7IuucS94S2C6jfK/D7dTCTo3Y=
+github.com/mattn/go-isatty v0.0.20 h1:xfD0iDuEKnDkl03q4limB+vH+GxLEtL/jb4xVJSWWEY=
+github.com/mattn/go-isatty v0.0.20/go.mod h1:W+V8PltTTMOvKvAeJH7IuucS94S2C6jfK/D7dTCTo3Y=
github.com/mitchellh/mapstructure v1.5.0 h1:jeMsZIYE/09sWLaz43PL7Gy6RuMjD2eJVyuac5Z2hdY=
github.com/mitchellh/mapstructure v1.5.0/go.mod h1:bFUtVrKA4DC2yAKiSyO/QUcy7e+RRV2QTWOzhPopBRo=
github.com/modern-go/concurrent v0.0.0-20180228061459-e0a39a4cb421/go.mod h1:6dJC0mAP4ikYIbvyc7fijjWJddQyLn8Ig3JB5CqoB9Q=
@@ -250,16 +249,14 @@ github.com/modern-go/concurrent v0.0.0-20180306012644-bacd9c7ef1dd/go.mod h1:6dJ
github.com/modern-go/reflect2 v1.0.2 h1:xBagoLtFs94CBntxluKeaWgTMpvLxC4ur3nMaC9Gz0M=
github.com/modern-go/reflect2 v1.0.2/go.mod h1:yWuevngMOJpCy52FWWMvUC8ws7m/LJsjYzDa0/r8luk=
github.com/niemeyer/pretty v0.0.0-20200227124842-a10e7caefd8e/go.mod h1:zD1mROLANZcx1PVRCS0qkT7pwLkGfwJo4zjcN/Tysno=
-github.com/pelletier/go-toml/v2 v2.0.8 h1:0ctb6s9mE31h0/lhu+J6OPmVeDxJn+kYnJc2jZR9tGQ=
-github.com/pelletier/go-toml/v2 v2.0.8/go.mod h1:vuYfssBdrU2XDZ9bYydBu6t+6a6PYNcZljzZR9VXg+4=
+github.com/pelletier/go-toml/v2 v2.2.0 h1:QLgLl2yMN7N+ruc31VynXs1vhMZa7CeHHejIeBAsoHo=
+github.com/pelletier/go-toml/v2 v2.2.0/go.mod h1:1t835xjRzz80PqgE6HHgN2JOsmgYu/h4qDAS4n929Rs=
github.com/pkg/diff v0.0.0-20210226163009-20ebb0f2a09e/go.mod h1:pJLUxLENpZxwdsKMEsNbx1VGcRFpLqf3715MtcvvzbA=
github.com/pkg/errors v0.9.1/go.mod h1:bwawxfHBFNV+L2hUp1rHADufV3IMtnDRdf1r5NINEl0=
github.com/pkg/sftp v1.13.1/go.mod h1:3HaPG6Dq1ILlpPZRO0HVMrsydcdLt6HRDccSgb87qRg=
github.com/pmezard/go-difflib v1.0.0 h1:4DBwDE0NGyQoBHbLQYPwSUPoCMWR5BEzIk/f1lZbAQM=
github.com/pmezard/go-difflib v1.0.0/go.mod h1:iKH77koFhYxTK1pcRnkKkqfTogsbg7gZNVY4sRDYZ/4=
github.com/prometheus/client_model v0.0.0-20190812154241-14fe0d1b01d4/go.mod h1:xMI15A0UPsDsEKsMN9yxemIoYk6Tm2C1GtYGdfGttqA=
-github.com/redis/go-redis/v9 v9.0.5 h1:CuQcn5HIEeK7BgElubPP8CGtE0KakrnbBSTLjathl5o=
-github.com/redis/go-redis/v9 v9.0.5/go.mod h1:WqMKv5vnQbRuZstUwxQI195wHy+t4PuXDOjzMvcuQHk=
github.com/remyoudompheng/bigfft v0.0.0-20200410134404-eec4a21b6bb0/go.mod h1:qqbHyh8v60DhA7CoWK5oRCqLrMHRGoxYCSS9EjAz6Eo=
github.com/remyoudompheng/bigfft v0.0.0-20230129092748-24d4a6f8daec h1:W09IVJc94icq4NjY3clb7Lk8O1qJ8BdBEF8z0ibU0rE=
github.com/remyoudompheng/bigfft v0.0.0-20230129092748-24d4a6f8daec/go.mod h1:qqbHyh8v60DhA7CoWK5oRCqLrMHRGoxYCSS9EjAz6Eo=
@@ -284,6 +281,7 @@ github.com/spf13/viper v1.16.0/go.mod h1:yg78JgCJcbrQOvV9YLXgkLaZqUidkY9K+Dd1Fof
github.com/stretchr/objx v0.1.0/go.mod h1:HFkY916IF+rwdDfMAkV7OtwuqBVzrE8GR6GFx+wExME=
github.com/stretchr/objx v0.4.0/go.mod h1:YvHI0jy2hoMjB+UWwv71VJQ9isScKT/TqJzVSSt89Yw=
github.com/stretchr/objx v0.5.0/go.mod h1:Yh+to48EsGEfYuaHDzXPcE3xhTkx73EhmCGUpEOglKo=
+github.com/stretchr/objx v0.5.2/go.mod h1:FRsXN1f5AsAjCGJKqEizvkpNtU+EGNCLh3NxZ/8L+MA=
github.com/stretchr/testify v1.2.2/go.mod h1:a8OnRcib4nhh0OaRAV+Yts87kKdq0PP7pXfy6kDkUVs=
github.com/stretchr/testify v1.3.0/go.mod h1:M5WIy9Dh21IEIfnGCwXGc5bZfKNJtfHm1UVUgZn+9EI=
github.com/stretchr/testify v1.4.0/go.mod h1:j7eGeouHqKxXV5pUuKE4zz7dFj8WfuZ+81PSLYec5m4=
@@ -294,9 +292,9 @@ github.com/stretchr/testify v1.7.1/go.mod h1:6Fq8oRcR53rry900zMqJjRRixrwX3KX962/
github.com/stretchr/testify v1.8.0/go.mod h1:yNjHg4UonilssWZ8iaSj1OCr/vHnekPRkoO+kdMU+MU=
github.com/stretchr/testify v1.8.1/go.mod h1:w2LPCIKwWwSfY2zedu0+kehJoqGctiVI29o6fzry7u4=
github.com/stretchr/testify v1.8.2/go.mod h1:w2LPCIKwWwSfY2zedu0+kehJoqGctiVI29o6fzry7u4=
-github.com/stretchr/testify v1.8.3/go.mod h1:sz/lmYIOXD/1dqDmKjjqLyZ2RngseejIcXlSw2iwfAo=
-github.com/stretchr/testify v1.8.4 h1:CcVxjf3Q8PM0mHUKJCdn+eZZtm5yQwehR5yeSVQQcUk=
github.com/stretchr/testify v1.8.4/go.mod h1:sz/lmYIOXD/1dqDmKjjqLyZ2RngseejIcXlSw2iwfAo=
+github.com/stretchr/testify v1.9.0 h1:HtqpIVDClZ4nwg75+f6Lvsy/wHu+3BoSGCbBAcpTsTg=
+github.com/stretchr/testify v1.9.0/go.mod h1:r2ic/lqez/lEtzL7wO/rwa5dbSLXVDPFyf8C91i36aY=
github.com/subosito/gotenv v1.4.2 h1:X1TuBLAMDFbaTAChgCBLu3DU3UPyELpnF2jjJ2cz/S8=
github.com/subosito/gotenv v1.4.2/go.mod h1:ayKnFf/c6rvx/2iiLrJUk1e6plDbT3edrFNGqEflhK0=
github.com/swaggo/files v1.0.1 h1:J1bVJ4XHZNq0I46UU90611i9/YzdrF7x92oX1ig5IdE=
@@ -307,8 +305,8 @@ github.com/swaggo/swag v1.16.2 h1:28Pp+8DkQoV+HLzLx8RGJZXNGKbFqnuvSbAAtoxiY04=
github.com/swaggo/swag v1.16.2/go.mod h1:6YzXnDcpr0767iOejs318CwYkCQqyGer6BizOg03f+E=
github.com/twitchyliquid64/golang-asm v0.15.1 h1:SU5vSMR7hnwNxj24w34ZyCi/FmDZTkS4MhqMhdFk5YI=
github.com/twitchyliquid64/golang-asm v0.15.1/go.mod h1:a1lVb/DtPvCB8fslRZhAngC2+aY1QWCk3Cedj/Gdt08=
-github.com/ugorji/go/codec v1.2.11 h1:BMaWp1Bb6fHwEtbplGBGJ498wD+LKlNSl25MjdZY4dU=
-github.com/ugorji/go/codec v1.2.11/go.mod h1:UNopzCgEMSXjBc6AOMqYvWC1ktqTAfzJZUZgYf6w6lg=
+github.com/ugorji/go/codec v1.2.12 h1:9LC83zGrHhuUA9l16C9AHXAqEV/2wBQ4nkvumAE65EE=
+github.com/ugorji/go/codec v1.2.12/go.mod h1:UNopzCgEMSXjBc6AOMqYvWC1ktqTAfzJZUZgYf6w6lg=
github.com/yuin/goldmark v1.1.25/go.mod h1:3hX8gzYuyVAZsxl0MRgGTJEmQBFcNTphYh9decYSb74=
github.com/yuin/goldmark v1.1.27/go.mod h1:3hX8gzYuyVAZsxl0MRgGTJEmQBFcNTphYh9decYSb74=
github.com/yuin/goldmark v1.1.32/go.mod h1:3hX8gzYuyVAZsxl0MRgGTJEmQBFcNTphYh9decYSb74=
@@ -330,8 +328,8 @@ go.uber.org/multierr v1.11.0/go.mod h1:20+QtiLqy0Nd6FdQB9TLXag12DsQkrbs3htMFfDN8
go.uber.org/zap v1.26.0 h1:sI7k6L95XOKS281NhVKOFCUNIvv9e0w4BF8N3u+tCRo=
go.uber.org/zap v1.26.0/go.mod h1:dtElttAiwGvoJ/vj4IwHBS/gXsEu/pZ50mUIRWuG0so=
golang.org/x/arch v0.0.0-20210923205945-b76863e36670/go.mod h1:5om86z9Hs0C8fWVUuoMHwpExlXzs5Tkyp9hOrfG7pp8=
-golang.org/x/arch v0.3.0 h1:02VY4/ZcO/gBOH6PUaoiptASxtXU10jazRCP865E97k=
-golang.org/x/arch v0.3.0/go.mod h1:5om86z9Hs0C8fWVUuoMHwpExlXzs5Tkyp9hOrfG7pp8=
+golang.org/x/arch v0.7.0 h1:pskyeJh/3AmoQ8CPE95vxHLqp1G1GfGNXTmcl9NEKTc=
+golang.org/x/arch v0.7.0/go.mod h1:FEVrYAQjsQXMVJ1nsMoVVXPZg6p2JE2mx8psSWTDQys=
golang.org/x/crypto v0.0.0-20190308221718-c2843e01d9a2/go.mod h1:djNgcEr1/C05ACkg1iLfiJU5Ep61QUkGW8qpdssI0+w=
golang.org/x/crypto v0.0.0-20190510104115-cbcb75029529/go.mod h1:yigFU9vqHzYiE8UmvKecakEJjdnWj3jj499lnFckfCI=
golang.org/x/crypto v0.0.0-20190605123033-f99c8df09eb5/go.mod h1:yigFU9vqHzYiE8UmvKecakEJjdnWj3jj499lnFckfCI=
@@ -340,8 +338,6 @@ golang.org/x/crypto v0.0.0-20200622213623-75b288015ac9/go.mod h1:LzIPMQfyMNhhGPh
golang.org/x/crypto v0.0.0-20210421170649-83a5a9bb288b/go.mod h1:T9bdIzuCu7OtxOm1hfPfRQxPLYneinmdGuTeoZ9dtd4=
golang.org/x/crypto v0.0.0-20210921155107-089bfa567519/go.mod h1:GvvjBRRGRdwPK5ydBHafDWAxML/pGHZbMvKqRZ5+Abc=
golang.org/x/crypto v0.0.0-20220722155217-630584e8d5aa/go.mod h1:IxCIyHEi3zRg3s0A5j5BB6A9Jmi73HwBIUl50j+osU4=
-golang.org/x/crypto v0.14.0 h1:wBqGXzWJW6m1XrIKlAH0Hs1JJ7+9KBwnIO8v66Q9cHc=
-golang.org/x/crypto v0.14.0/go.mod h1:MVFd36DqK4CsrnJYDkBA3VC4m2GkXAM0PvzMCn4JQf4=
golang.org/x/crypto v0.19.0/go.mod h1:Iy9bg/ha4yyC70EfRS8jz+B6ybOBKMaSxLj6P6oBDfU=
golang.org/x/crypto v0.21.0 h1:X31++rzVUdKhX5sWmSOFZxx8UW/ldWx55cbf08iNAMA=
golang.org/x/crypto v0.21.0/go.mod h1:0BP7YvVV9gBbVKyeTG0Gyn+gZm94bibOW5BjDEYAOMs=
@@ -421,9 +417,6 @@ golang.org/x/net v0.0.0-20220722155237-a158d28d115b/go.mod h1:XRhObCWvk6IyKnWLug
golang.org/x/net v0.6.0/go.mod h1:2Tu9+aMcznHK/AK1HMvgo6xiTLG5rD5rZLDS+rp2Bjs=
golang.org/x/net v0.7.0/go.mod h1:2Tu9+aMcznHK/AK1HMvgo6xiTLG5rD5rZLDS+rp2Bjs=
golang.org/x/net v0.10.0/go.mod h1:0qNGK6F8kojg2nk9dLZ2mShWaEBan6FAoqfSigmmuDg=
-golang.org/x/net v0.15.0/go.mod h1:idbUs1IY1+zTqbi8yxTbhexhEEk5ur9LInksu6HrEpk=
-golang.org/x/net v0.17.0 h1:pVaXccu2ozPjCXewfr1S7xza/zcXTity9cCdXQYSjIM=
-golang.org/x/net v0.17.0/go.mod h1:NxSsAGuq816PNPmqtQdLE42eU2Fs7NoRIZrHJAlaCOE=
golang.org/x/net v0.21.0/go.mod h1:bIjVDfnllIU7BJ2DNgfnXvpSvtn8VRwhlsaeUTyUS44=
golang.org/x/net v0.22.0 h1:9sGLhx7iRIHEiX0oAJ3MRZMUCElJgy7Br1nO+AMN3Tc=
golang.org/x/net v0.22.0/go.mod h1:JKghWKKOSdJwpW2GEx0Ja7fmaKnMsbu+MWVZTokSYmg=
@@ -487,14 +480,11 @@ golang.org/x/sys v0.0.0-20210423185535-09eb48e85fd7/go.mod h1:h1NjWce9XRLGQEsW7w
golang.org/x/sys v0.0.0-20210510120138-977fb7262007/go.mod h1:oPkhp1MJrh7nUepCBck5+mAzfO9JrbApNNgaTdGDITg=
golang.org/x/sys v0.0.0-20210615035016-665e8c7367d1/go.mod h1:oPkhp1MJrh7nUepCBck5+mAzfO9JrbApNNgaTdGDITg=
golang.org/x/sys v0.0.0-20220520151302-bc2c85ada10a/go.mod h1:oPkhp1MJrh7nUepCBck5+mAzfO9JrbApNNgaTdGDITg=
-golang.org/x/sys v0.0.0-20220704084225-05e143d24a9e/go.mod h1:oPkhp1MJrh7nUepCBck5+mAzfO9JrbApNNgaTdGDITg=
golang.org/x/sys v0.0.0-20220722155257-8c9f86f7a55f/go.mod h1:oPkhp1MJrh7nUepCBck5+mAzfO9JrbApNNgaTdGDITg=
golang.org/x/sys v0.0.0-20220908164124-27713097b956/go.mod h1:oPkhp1MJrh7nUepCBck5+mAzfO9JrbApNNgaTdGDITg=
golang.org/x/sys v0.5.0/go.mod h1:oPkhp1MJrh7nUepCBck5+mAzfO9JrbApNNgaTdGDITg=
golang.org/x/sys v0.6.0/go.mod h1:oPkhp1MJrh7nUepCBck5+mAzfO9JrbApNNgaTdGDITg=
golang.org/x/sys v0.8.0/go.mod h1:oPkhp1MJrh7nUepCBck5+mAzfO9JrbApNNgaTdGDITg=
-golang.org/x/sys v0.13.0 h1:Af8nKPmuFypiUBjVoU9V20FiaFXOcuZI21p0ycVYYGE=
-golang.org/x/sys v0.13.0/go.mod h1:oPkhp1MJrh7nUepCBck5+mAzfO9JrbApNNgaTdGDITg=
golang.org/x/sys v0.17.0/go.mod h1:/VUhepiaJMQUp4+oa/7Zr1D23ma6VTLIYjOOTFZPUcA=
golang.org/x/sys v0.18.0 h1:DBdB3niSjOA/O0blCZBqDefyWNYveAYMNF1Wum0DYQ4=
golang.org/x/sys v0.18.0/go.mod h1:/VUhepiaJMQUp4+oa/7Zr1D23ma6VTLIYjOOTFZPUcA=
@@ -502,7 +492,6 @@ golang.org/x/term v0.0.0-20201126162022-7de9c90e9dd1/go.mod h1:bj7SfCRtBDWHUb9sn
golang.org/x/term v0.0.0-20210927222741-03fcf44c2211/go.mod h1:jbD1KX2456YbFQfuXm/mYQcufACuNUgVhRMnK/tPxf8=
golang.org/x/term v0.5.0/go.mod h1:jMB1sMXY+tzblOD4FWmEbocvup2/aLOaQEp7JmGp78k=
golang.org/x/term v0.8.0/go.mod h1:xPskH00ivmX89bAKVGSKKtLOWNx2+17Eiy94tnKShWo=
-golang.org/x/term v0.13.0/go.mod h1:LTmsnFJwVN6bCy1rVCoS+qHT1HhALEFxKncY3WNNh4U=
golang.org/x/term v0.17.0/go.mod h1:lLRBjIVuehSbZlaOtGMbcMncT+aqLLLmKrsjNrUguwk=
golang.org/x/term v0.18.0/go.mod h1:ILwASektA3OnRv7amZ1xhE/KTR+u50pbXfZ03+6Nx58=
golang.org/x/text v0.0.0-20170915032832-14c0d48ead0c/go.mod h1:NqM8EUOU14njkJ3fqMW+pc6Ldnwhi/IjpwHt7yyuwOQ=
@@ -515,14 +504,12 @@ golang.org/x/text v0.3.6/go.mod h1:5Zoc/QRtKVWzQhOtBMvqHzDpF6irO9z98xDceosuGiQ=
golang.org/x/text v0.3.7/go.mod h1:u+2+/6zg+i71rQMx5EYifcz6MCKuco9NR6JIITiCfzQ=
golang.org/x/text v0.7.0/go.mod h1:mrYo+phRRbMaCq/xk9113O4dZlRixOauAjOtrjsXDZ8=
golang.org/x/text v0.9.0/go.mod h1:e1OnstbJyHTd6l/uOt8jFFHp6TRDWZR/bV3emEE/zU8=
-golang.org/x/text v0.13.0 h1:ablQoSUd0tRdKxZewP80B+BaqeKJuVhuRxj/dkrun3k=
-golang.org/x/text v0.13.0/go.mod h1:TvPlkZtksWOMsz7fbANvkp4WM8x/WCo/om8BMLbz+aE=
golang.org/x/text v0.14.0 h1:ScX5w1eTa3QqT8oi6+ziP7dTV1S2+ALU0bI+0zXKWiQ=
golang.org/x/text v0.14.0/go.mod h1:18ZOQIKpY8NJVqYksKHtTdi31H5itFRjB5/qKTNYzSU=
golang.org/x/time v0.0.0-20181108054448-85acf8d2951c/go.mod h1:tRJNPiyCQ0inRvYxbN9jk5I+vvW/OXSQhTDSoE431IQ=
golang.org/x/time v0.0.0-20190308202827-9d24e82272b4/go.mod h1:tRJNPiyCQ0inRvYxbN9jk5I+vvW/OXSQhTDSoE431IQ=
golang.org/x/time v0.0.0-20191024005414-555d28b269f0/go.mod h1:tRJNPiyCQ0inRvYxbN9jk5I+vvW/OXSQhTDSoE431IQ=
-golang.org/x/time v0.3.0/go.mod h1:tRJNPiyCQ0inRvYxbN9jk5I+vvW/OXSQhTDSoE431IQ=
+golang.org/x/time v0.5.0 h1:o7cqy6amK/52YcAKIPlM3a+Fpj35zvRj2TP+e1xFSfk=
golang.org/x/time v0.5.0/go.mod h1:3BpzKBy/shNhVucY/MWOyx10tF3SFh9QdLuxbVysPQM=
golang.org/x/tools v0.0.0-20180917221912-90fa682c2a6e/go.mod h1:n7NCudcB/nEzxVGmLbDWY5pfWTLqBcC2KZ6jyYvM4mQ=
golang.org/x/tools v0.0.0-20190114222345-bf090417da8b/go.mod h1:n7NCudcB/nEzxVGmLbDWY5pfWTLqBcC2KZ6jyYvM4mQ=
@@ -675,8 +662,8 @@ google.golang.org/protobuf v1.24.0/go.mod h1:r/3tXBNzIEhYS9I1OUVjXDlt8tc493IdKGj
google.golang.org/protobuf v1.25.0/go.mod h1:9JNX74DMeImyA3h4bdi1ymwjUzf21/xIlbajtzgsN7c=
google.golang.org/protobuf v1.26.0-rc.1/go.mod h1:jlhhOSvTdKEhbULTjvd4ARK9grFBp09yW+WbY/TyQbw=
google.golang.org/protobuf v1.26.0/go.mod h1:9q0QmTI4eRPtz6boOQmLYwt+qCgq0jsYwAQnmE0givc=
-google.golang.org/protobuf v1.30.0 h1:kPPoIgf3TsEvrm0PFe15JQ+570QVxYzEvvHqChK+cng=
-google.golang.org/protobuf v1.30.0/go.mod h1:HV8QOd/L58Z+nl8r43ehVNZIU/HEI6OcFqwMG9pJV4I=
+google.golang.org/protobuf v1.33.0 h1:uNO2rsAINq/JlFpSdYEKIZ0uKD/R9cpdv0T+yoGwGmI=
+google.golang.org/protobuf v1.33.0/go.mod h1:c6P6GXX6sHbq/GpV6MGZEdwhWPcYBgnhAHhKbcUYpos=
gopkg.in/check.v1 v0.0.0-20161208181325-20d25e280405/go.mod h1:Co6ibVJAznAaIkqp8huTwlJQCZ016jof/cbN4VW5Yz0=
gopkg.in/check.v1 v1.0.0-20180628173108-788fd7840127/go.mod h1:Co6ibVJAznAaIkqp8huTwlJQCZ016jof/cbN4VW5Yz0=
gopkg.in/check.v1 v1.0.0-20200227125254-8fa46927fb4f/go.mod h1:Co6ibVJAznAaIkqp8huTwlJQCZ016jof/cbN4VW5Yz0=
@@ -716,6 +703,7 @@ modernc.org/memory v1.5.0 h1:N+/8c5rE6EqugZwHii4IFsaJ7MUhoWX07J5tC/iI5Ds=
modernc.org/memory v1.5.0/go.mod h1:PkUhL0Mugw21sHPeskwZW4D6VscE/GQJOnIpCnW6pSU=
modernc.org/sqlite v1.23.1 h1:nrSBg4aRQQwq59JpvGEQ15tNxoO5pX/kUjcRNwSAGQM=
modernc.org/sqlite v1.23.1/go.mod h1:OrDj17Mggn6MhE+iPbBNf7RGKODDE9NFT0f3EwDzJqk=
+nullprogram.com/x/optparse v1.0.0/go.mod h1:KdyPE+Igbe0jQUrVfMqDMeJQIJZEuyV7pjYmp6pbG50=
rsc.io/binaryregexp v0.2.0/go.mod h1:qTv7/COck+e2FymRvadv62gMdZztPaShugOCi3I+8D8=
rsc.io/pdf v0.1.1/go.mod h1:n8OzWcQ6Sp37PL01nO98y4iUCRdTGarVfzxY20ICaU4=
rsc.io/quote/v3 v3.1.0/go.mod h1:yEA65RcK8LyAZtP9Kv3t0HmxON59tX3rD+tICJqUlj0=
diff --git a/internal/handler/user.go b/internal/handler/user.go
index 0cf2c23..247680c 100644
--- a/internal/handler/user.go
+++ b/internal/handler/user.go
@@ -38,7 +38,7 @@ func (h *UserHandler) Login(ctx *gin.Context) {
token, rules, err := h.userService.Login(ctx, &req)
if err != nil {
- v1.HandleError(ctx, http.StatusUnauthorized, v1.ErrUnauthorized, nil)
+ v1.HandleError(ctx, http.StatusUnauthorized, v1.ErrBadRequest, nil)
return
}
v1.HandleSuccess(ctx, v1.LoginResponseData{
diff --git a/internal/middleware/log.go b/internal/middleware/log.go
index f96c4aa..da184e4 100644
--- a/internal/middleware/log.go
+++ b/internal/middleware/log.go
@@ -21,7 +21,7 @@ func RequestLogMiddleware(logger *log.Logger) gin.HandlerFunc {
trace := cryptor.Md5String(uuid)
logger.WithValue(ctx, zap.String("trace", trace))
logger.WithValue(ctx, zap.String("request_method", ctx.Request.Method))
- logger.WithValue(ctx, zap.Any("request_headers", ctx.Request.Header))
+ //logger.WithValue(ctx, zap.Any("request_headers", ctx.Request.Header))
logger.WithValue(ctx, zap.String("request_url", ctx.Request.URL.String()))
if ctx.Request.Body != nil {
bodyBytes, _ := ctx.GetRawData()
@@ -39,7 +39,7 @@ func ResponseLogMiddleware(logger *log.Logger) gin.HandlerFunc {
startTime := time.Now()
ctx.Next()
duration := time.Since(startTime).String()
- logger.WithContext(ctx).Info("Response", zap.Any("response_body", blw.body.String()), zap.Any("time", duration))
+ logger.WithContext(ctx).Info("Response", zap.Any("time", duration))
}
}
diff --git a/internal/repository/repository.go b/internal/repository/repository.go
index 860de47..2515bb3 100644
--- a/internal/repository/repository.go
+++ b/internal/repository/repository.go
@@ -4,13 +4,12 @@ import (
"PandoraHelper/pkg/log"
"PandoraHelper/pkg/zapgorm2"
"context"
- "fmt"
"github.com/glebarez/sqlite"
- "github.com/redis/go-redis/v9"
"github.com/spf13/viper"
"gorm.io/driver/mysql"
"gorm.io/driver/postgres"
"gorm.io/gorm"
+ "os"
"time"
)
@@ -68,8 +67,8 @@ func NewDB(conf *viper.Viper, l *log.Logger) *gorm.DB {
)
logger := zapgorm2.New(l.Logger)
- driver := conf.GetString("data.db.user.driver")
- dsn := conf.GetString("data.db.user.dsn")
+ driver := conf.GetString("database.driver")
+ dsn := conf.GetString("database.dsn")
// GORM doc: https://gorm.io/docs/connecting_to_the_database.html
switch driver {
@@ -83,6 +82,17 @@ func NewDB(conf *viper.Viper, l *log.Logger) *gorm.DB {
PreferSimpleProtocol: true, // disables implicit prepared statement usage
}), &gorm.Config{})
case "sqlite":
+ _, err := os.Stat(dsn)
+ if err != nil {
+ if os.IsNotExist(err) {
+ _, err := os.Create(dsn)
+ if err != nil {
+ return nil
+ }
+ } else {
+ panic(err)
+ }
+ }
db, err = gorm.Open(sqlite.Open(dsn), &gorm.Config{})
default:
panic("unknown db driver")
@@ -102,20 +112,3 @@ func NewDB(conf *viper.Viper, l *log.Logger) *gorm.DB {
sqlDB.SetConnMaxLifetime(time.Hour)
return db
}
-func NewRedis(conf *viper.Viper) *redis.Client {
- rdb := redis.NewClient(&redis.Options{
- Addr: conf.GetString("data.redis.addr"),
- Password: conf.GetString("data.redis.password"),
- DB: conf.GetInt("data.redis.db"),
- })
-
- ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
- defer cancel()
-
- _, err := rdb.Ping(ctx).Result()
- if err != nil {
- panic(fmt.Sprintf("redis error: %s", err.Error()))
- }
-
- return rdb
-}
diff --git a/internal/server/http.go b/internal/server/http.go
index cbb644a..f6088af 100644
--- a/internal/server/http.go
+++ b/internal/server/http.go
@@ -1,15 +1,17 @@
package server
import (
- apiV1 "PandoraHelper/api/v1"
+ "PandoraHelper"
"PandoraHelper/docs"
"PandoraHelper/internal/handler"
"PandoraHelper/internal/middleware"
"PandoraHelper/pkg/jwt"
"PandoraHelper/pkg/log"
"PandoraHelper/pkg/server/http"
+ "github.com/gin-contrib/static"
"github.com/gin-gonic/gin"
"github.com/spf13/viper"
+
swaggerfiles "github.com/swaggo/files"
ginSwagger "github.com/swaggo/gin-swagger"
)
@@ -22,7 +24,7 @@ func NewHTTPServer(
shareHandler *handler.ShareHandler,
accountHandler *handler.AccountHandler,
) *http.Server {
- gin.SetMode(gin.DebugMode)
+ gin.SetMode(gin.ReleaseMode)
s := http.NewServer(
gin.Default(),
logger,
@@ -44,12 +46,8 @@ func NewHTTPServer(
middleware.RequestLogMiddleware(logger),
//middleware.SignMiddleware(log),
)
- s.GET("/", func(ctx *gin.Context) {
- logger.WithContext(ctx).Info("hello")
- apiV1.HandleSuccess(ctx, map[string]interface{}{
- ":)": "Thank you for using nunu!",
- })
- })
+
+ s.Use(static.Serve("/", static.EmbedFolder(PandoraHelper.EmbedFrontendFS, "frontend/dist")))
v1 := s.Group("/api")
{
diff --git a/internal/server/migration.go b/internal/server/migration.go
index 47a440a..10f707a 100644
--- a/internal/server/migration.go
+++ b/internal/server/migration.go
@@ -6,7 +6,6 @@ import (
"context"
"go.uber.org/zap"
"gorm.io/gorm"
- "os"
)
type Migrate struct {
@@ -29,7 +28,6 @@ func (m *Migrate) Start(ctx context.Context) error {
return err
}
m.log.Info("AutoMigrate success")
- os.Exit(0)
return nil
}
func (m *Migrate) Stop(ctx context.Context) error {
diff --git a/internal/server/task.go b/internal/server/task.go
index 543512c..b26dc48 100644
--- a/internal/server/task.go
+++ b/internal/server/task.go
@@ -68,12 +68,12 @@ func (t *Task) Start(ctx context.Context) error {
t.scheduler = gocron.NewScheduler(time.UTC)
- _, err := t.scheduler.Every("1d").Do(t.RefreshAllAccountEveryday, ctx)
+ _, err := t.scheduler.Every(1).Day().At("00:00").Do(t.RefreshAllAccountEveryday, ctx)
if err != nil {
return err
}
- _, err = t.scheduler.Every("1d").Do(t.RefreshShareLimitEveryday, ctx)
+ _, err = t.scheduler.Every(1).Day().At("00:05").Do(t.RefreshShareLimitEveryday, ctx)
if err != nil {
return err
}
diff --git a/internal/service/user.go b/internal/service/user.go
index cc41c9c..cc389ca 100644
--- a/internal/service/user.go
+++ b/internal/service/user.go
@@ -46,5 +46,5 @@ func (s *userService) Login(ctx context.Context, req *v1.LoginRequest) (string,
return token, user, nil
}
- return "", nil, v1.ErrUnauthorized
+ return "", nil, v1.ErrBadRequest
}
diff --git a/pkg/config/config.go b/pkg/config/config.go
index 9969ffa..dbe430a 100644
--- a/pkg/config/config.go
+++ b/pkg/config/config.go
@@ -6,18 +6,44 @@ import (
"os"
)
+func doesPathExist(path string) bool {
+ // 使用os.Stat来获取文件/目录状态
+ _, err := os.Stat(path)
+
+ // 如果没有错误,说明路径存在
+ if err == nil {
+ return true
+ }
+
+ // 如果错误是因为路径不存在,返回false
+ if os.IsNotExist(err) {
+ return false
+ }
+
+ // 对于其他错误,打印错误消息并返回false
+ fmt.Println("检查路径时发生错误:", err)
+ return false
+}
+
func NewConfig(p string) *viper.Viper {
envConf := os.Getenv("APP_CONF")
if envConf == "" {
envConf = p
}
fmt.Println("load conf file:", envConf)
- return getConfig(envConf)
+ return getConfig(envConf, ".")
}
-func getConfig(path string) *viper.Viper {
+func getConfig(path ...string) *viper.Viper {
conf := viper.New()
- conf.SetConfigFile(path)
+ conf.SetConfigName("config")
+ for _, p := range path {
+ // 路径不存在则跳过
+ if !doesPathExist(p) {
+ continue
+ }
+ conf.AddConfigPath(p)
+ }
err := conf.ReadInConfig()
if err != nil {
panic(err)
diff --git a/pkg/jwt/jwt.go b/pkg/jwt/jwt.go
index aa93e13..a650ccd 100644
--- a/pkg/jwt/jwt.go
+++ b/pkg/jwt/jwt.go
@@ -1,7 +1,10 @@
package jwt
import (
+ "crypto/rand"
+ "encoding/hex"
"errors"
+ "fmt"
"regexp"
"time"
@@ -18,8 +21,30 @@ type MyCustomClaims struct {
jwt.RegisteredClaims
}
+func generateRandomJwtSecret(length int) (string, error) {
+ // 创建一个用于存储随机字节的切片
+ randomBytes := make([]byte, length)
+ // 使用crypto/rand库生成随机字节
+ _, err := rand.Read(randomBytes)
+ if err != nil {
+ return "", fmt.Errorf("生成随机JWT密钥时出错: %v", err)
+ }
+
+ // 将随机字节转换为十六进制字符串
+ randomHex := hex.EncodeToString(randomBytes)
+
+ return randomHex, nil
+}
+
func NewJwt(conf *viper.Viper) *JWT {
- return &JWT{key: []byte(conf.GetString("security.jwt.key"))}
+ //随机生成一个Jwt密钥
+ jwtSecret, err := generateRandomJwtSecret(32)
+ if err != nil {
+ panic(err)
+ }
+ return &JWT{
+ key: []byte(jwtSecret),
+ }
}
func (j *JWT) GenToken(userId string, expiresAt time.Time) (string, error) {