-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
9412b2c
commit 580ee28
Showing
48 changed files
with
1,001 additions
and
2 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,2 +1,79 @@ | ||
# vocal-mastering | ||
The Vocal Mastering Application is a Django-based web application designed to facilitate the uploading and processing of vocal audio files. It utilizes advanced audio processing techniques to enhance the quality of vocal recordings, making it suitable for musicians, producers, and audio engineers. | ||
|
||
--- | ||
|
||
# Vocal Mastering Application | ||
|
||
## Overview | ||
The Vocal Mastering Application is a Django-based web application designed to facilitate the uploading and processing of vocal audio files. It utilizes advanced audio processing techniques to enhance the quality of vocal recordings, making it suitable for musicians, producers, and audio engineers. | ||
|
||
## Features | ||
- **File Upload:** Users can upload vocal audio files in various formats (WAV, MP3, M4A). | ||
- **Asynchronous Processing:** Audio processing is handled in the background using Celery, allowing users to continue using the application while their files are being processed. | ||
- **Advanced Audio Processing:** The application employs a range of audio processing techniques, including noise reduction, dynamic compression, equalization, and loudness normalization. | ||
- **Job Status Tracking:** Users can check the status of their audio processing jobs and download the mastered audio once completed. | ||
|
||
## Technologies Used | ||
- **Django:** A high-level Python web framework for building web applications. | ||
- **Celery:** An asynchronous task queue/job queue based on distributed message passing. | ||
- **Librosa:** A Python package for music and audio analysis. | ||
- **NumPy:** A library for numerical computations in Python. | ||
- **SoundFile:** A library for reading and writing sound files. | ||
- **Pyloudnorm:** A library for loudness normalization. | ||
|
||
## Installation | ||
To set up the Vocal Mastering Application locally, follow these steps: | ||
|
||
### Clone the Repository: | ||
```bash | ||
git clone https://github.com/yourusername/vocal-mastering.git | ||
cd vocal-mastering | ||
``` | ||
|
||
### Create a Virtual Environment: | ||
```bash | ||
python -m venv venv | ||
source venv/bin/activate # On Windows use `venv\Scripts\activate` | ||
``` | ||
|
||
### Install Dependencies: | ||
```bash | ||
pip install -r requirements.txt | ||
``` | ||
|
||
### Set Up the Database: | ||
```bash | ||
python manage.py migrate | ||
``` | ||
|
||
### Run the Development Server: | ||
```bash | ||
python manage.py runserver | ||
``` | ||
|
||
### Access the Application: | ||
Open your web browser and navigate to [http://127.0.0.1:8000/](http://127.0.0.1:8000/). | ||
|
||
## Usage | ||
1. **Upload Vocal:** Navigate to the upload page and select an audio file to upload. | ||
2. **Processing:** After uploading, the application will process the audio file in the background. You will be redirected to a job status page. | ||
3. **Download Mastered Audio:** Once processing is complete, you can download the mastered audio file. | ||
|
||
## Code Structure | ||
The application is organized into several key components: | ||
- `forms.py`: Contains the form for uploading audio files. | ||
- `models.py`: Defines the data models for storing audio files and processing jobs. | ||
- `processors.py`: Implements the audio processing logic. | ||
- `tasks.py`: Contains the Celery tasks for asynchronous processing. | ||
- `views.py`: Handles the web requests and responses. | ||
- `urls.py`: Defines the URL routing for the application. | ||
|
||
## Contributing | ||
Contributions are welcome! If you have suggestions for improvements or new features, please open an issue or submit a pull request. | ||
|
||
## License | ||
This project is licensed under the MIT License. See the LICENSE file for details. | ||
|
||
## Sample Screenshot | ||
[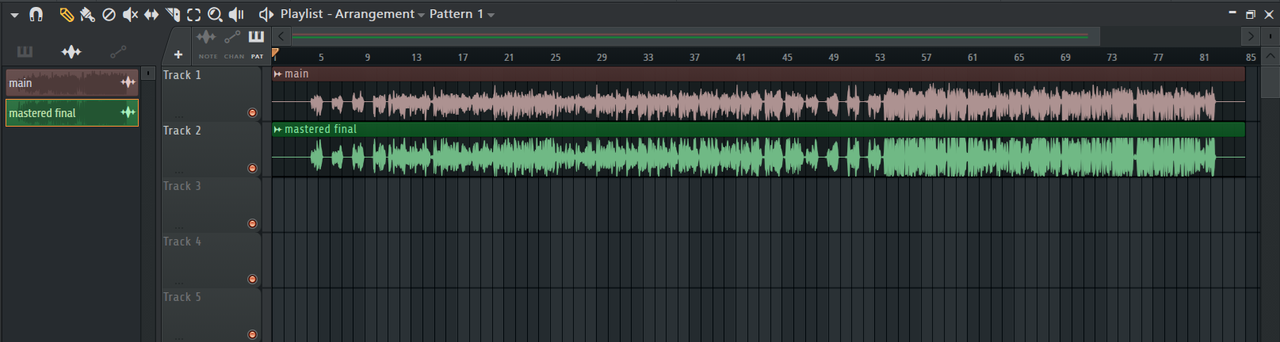](https://postimg.cc/sGsrW7ym) | ||
|
||
--- |
Binary file not shown.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,22 @@ | ||
#!/usr/bin/env python | ||
"""Django's command-line utility for administrative tasks.""" | ||
import os | ||
import sys | ||
|
||
|
||
def main(): | ||
"""Run administrative tasks.""" | ||
os.environ.setdefault("DJANGO_SETTINGS_MODULE", "vocal_mastering.settings") | ||
try: | ||
from django.core.management import execute_from_command_line | ||
except ImportError as exc: | ||
raise ImportError( | ||
"Couldn't import Django. Are you sure it's installed and " | ||
"available on your PYTHONPATH environment variable? Did you " | ||
"forget to activate a virtual environment?" | ||
) from exc | ||
execute_from_command_line(sys.argv) | ||
|
||
|
||
if __name__ == "__main__": | ||
main() |
Empty file.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,3 @@ | ||
from django.contrib import admin | ||
|
||
# Register your models here. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,6 @@ | ||
from django.apps import AppConfig | ||
|
||
|
||
class MasteringConfig(AppConfig): | ||
default_auto_field = "django.db.models.BigAutoField" | ||
name = "mastering" |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,10 @@ | ||
from django import forms | ||
from .models import VocalMastering | ||
|
||
class VocalUploadForm(forms.ModelForm): | ||
class Meta: | ||
model = VocalMastering | ||
fields = ['original_audio'] | ||
widgets = { | ||
'original_audio': forms.FileInput(attrs={'accept': 'audio/*'}) | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,61 @@ | ||
# Generated by Django 5.1.4 on 2024-12-10 09:10 | ||
|
||
import django.db.models.deletion | ||
from django.conf import settings | ||
from django.db import migrations, models | ||
|
||
|
||
class Migration(migrations.Migration): | ||
|
||
initial = True | ||
|
||
dependencies = [ | ||
migrations.swappable_dependency(settings.AUTH_USER_MODEL), | ||
] | ||
|
||
operations = [ | ||
migrations.CreateModel( | ||
name="VocalProcessingJob", | ||
fields=[ | ||
( | ||
"id", | ||
models.BigAutoField( | ||
auto_created=True, | ||
primary_key=True, | ||
serialize=False, | ||
verbose_name="ID", | ||
), | ||
), | ||
("original_file", models.FileField(upload_to="original_vocals/")), | ||
( | ||
"processed_file", | ||
models.FileField( | ||
blank=True, null=True, upload_to="processed_vocals/" | ||
), | ||
), | ||
( | ||
"status", | ||
models.CharField( | ||
choices=[ | ||
("pending", "Pending"), | ||
("processing", "Processing"), | ||
("completed", "Completed"), | ||
("failed", "Failed"), | ||
], | ||
default="pending", | ||
max_length=20, | ||
), | ||
), | ||
("created_at", models.DateTimeField(auto_now_add=True)), | ||
("completed_at", models.DateTimeField(blank=True, null=True)), | ||
("error_message", models.TextField(blank=True, null=True)), | ||
( | ||
"user", | ||
models.ForeignKey( | ||
on_delete=django.db.models.deletion.CASCADE, | ||
to=settings.AUTH_USER_MODEL, | ||
), | ||
), | ||
], | ||
), | ||
] |
37 changes: 37 additions & 0 deletions
37
mastering/migrations/0002_vocalmastering_delete_vocalprocessingjob.py
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,37 @@ | ||
# Generated by Django 5.1.4 on 2024-12-10 09:46 | ||
|
||
import uuid | ||
from django.db import migrations, models | ||
|
||
|
||
class Migration(migrations.Migration): | ||
|
||
dependencies = [ | ||
("mastering", "0001_initial"), | ||
] | ||
|
||
operations = [ | ||
migrations.CreateModel( | ||
name="VocalMastering", | ||
fields=[ | ||
( | ||
"id", | ||
models.UUIDField( | ||
default=uuid.uuid4, | ||
editable=False, | ||
primary_key=True, | ||
serialize=False, | ||
), | ||
), | ||
("original_audio", models.FileField(upload_to="vocals/")), | ||
( | ||
"mastered_audio", | ||
models.FileField(blank=True, null=True, upload_to="mastered/"), | ||
), | ||
("created_at", models.DateTimeField(auto_now_add=True)), | ||
], | ||
), | ||
migrations.DeleteModel( | ||
name="VocalProcessingJob", | ||
), | ||
] |
18 changes: 18 additions & 0 deletions
18
mastering/migrations/0003_vocalmastering_reference_audio.py
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,18 @@ | ||
# Generated by Django 5.1.4 on 2024-12-12 11:01 | ||
|
||
from django.db import migrations, models | ||
|
||
|
||
class Migration(migrations.Migration): | ||
|
||
dependencies = [ | ||
("mastering", "0002_vocalmastering_delete_vocalprocessingjob"), | ||
] | ||
|
||
operations = [ | ||
migrations.AddField( | ||
model_name="vocalmastering", | ||
name="reference_audio", | ||
field=models.FileField(blank=True, null=True, upload_to="references/"), | ||
), | ||
] |
17 changes: 17 additions & 0 deletions
17
mastering/migrations/0004_remove_vocalmastering_reference_audio.py
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
# Generated by Django 5.1.4 on 2024-12-12 11:45 | ||
|
||
from django.db import migrations | ||
|
||
|
||
class Migration(migrations.Migration): | ||
|
||
dependencies = [ | ||
("mastering", "0003_vocalmastering_reference_audio"), | ||
] | ||
|
||
operations = [ | ||
migrations.RemoveField( | ||
model_name="vocalmastering", | ||
name="reference_audio", | ||
), | ||
] |
Empty file.
Binary file not shown.
Binary file added
BIN
+1.3 KB
...ring/migrations/__pycache__/0002_vocalmastering_delete_vocalprocessingjob.cpython-312.pyc
Binary file not shown.
Binary file added
BIN
+855 Bytes
mastering/migrations/__pycache__/0003_vocalmastering_reference_audio.cpython-312.pyc
Binary file not shown.
Binary file added
BIN
+714 Bytes
mastering/migrations/__pycache__/0004_remove_vocalmastering_reference_audio.cpython-312.pyc
Binary file not shown.
Binary file not shown.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
import uuid | ||
from django.db import models | ||
|
||
class VocalMastering(models.Model): | ||
id = models.UUIDField(primary_key=True, default=uuid.uuid4, editable=False) | ||
original_audio = models.FileField(upload_to='vocals/') | ||
mastered_audio = models.FileField(upload_to='mastered/', null=True, blank=True) | ||
created_at = models.DateTimeField(auto_now_add=True) | ||
|
||
def __str__(self): | ||
return f"Vocal Mastering - {self.id}" |
Oops, something went wrong.