-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
0 parents
commit 9f2e57f
Showing
335 changed files
with
97,873 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,111 @@ | ||
# Byte-compiled / optimized / DLL files | ||
__pycache__/ | ||
*.py[cod] | ||
*$py.class | ||
|
||
# C extensions | ||
*.so | ||
|
||
# Distribution / packaging | ||
.Python | ||
build/ | ||
develop-eggs/ | ||
dist/ | ||
downloads/ | ||
eggs/ | ||
.eggs/ | ||
lib/ | ||
lib64/ | ||
parts/ | ||
sdist/ | ||
var/ | ||
wheels/ | ||
*.egg-info/ | ||
.installed.cfg | ||
*.egg | ||
MANIFEST | ||
|
||
# PyInstaller | ||
# Usually these files are written by a python script from a template | ||
# before PyInstaller builds the exe, so as to inject date/other infos into it. | ||
*.manifest | ||
*.spec | ||
|
||
# Installer logs | ||
pip-log.txt | ||
pip-delete-this-directory.txt | ||
|
||
# Unit test / coverage reports | ||
htmlcov/ | ||
.tox/ | ||
.coverage | ||
.coverage.* | ||
.cache | ||
nosetests.xml | ||
coverage.xml | ||
*.cover | ||
.hypothesis/ | ||
.pytest_cache/ | ||
|
||
# Translations | ||
*.mo | ||
*.pot | ||
|
||
# Django stuff: | ||
*.log | ||
local_settings.py | ||
db.sqlite3 | ||
|
||
# Flask stuff: | ||
instance/ | ||
.webassets-cache | ||
|
||
# Scrapy stuff: | ||
.scrapy | ||
|
||
# Sphinx documentation | ||
docs/_build/ | ||
|
||
# PyBuilder | ||
target/ | ||
|
||
# Jupyter Notebook | ||
.ipynb_checkpoints | ||
|
||
# pyenv | ||
.python-version | ||
|
||
# celery beat schedule file | ||
celerybeat-schedule | ||
|
||
# SageMath parsed files | ||
*.sage.py | ||
|
||
# Environments | ||
.env | ||
.venv | ||
env/ | ||
venv/ | ||
ENV/ | ||
env.bak/ | ||
venv.bak/ | ||
|
||
# Spyder project settings | ||
.spyderproject | ||
.spyproject | ||
|
||
# Rope project settings | ||
.ropeproject | ||
|
||
# mkdocs documentation | ||
/site | ||
|
||
# mypy | ||
.mypy_cache/ | ||
|
||
# Additional ignore folders | ||
.idea/ | ||
__pycache__/ | ||
datasets/ | ||
#trained_model/ | ||
weights/ |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
# Parking_DeepLearning | ||
|
||
|
||
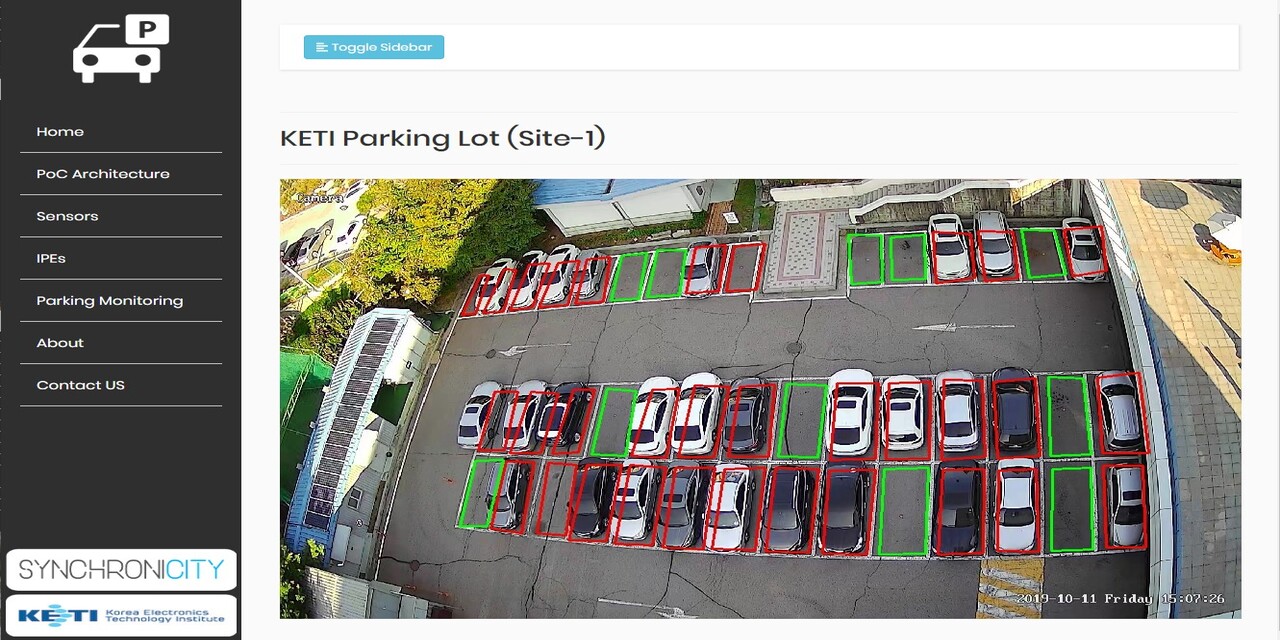 | ||
|
||
|
||
|
||
This project is implemented by referring following two references: | ||
|
||
CNN for Parking lot: | ||
https://github.com/dalmia/WannaPark/tree/master/parking_lot_detection | ||
|
||
Flask Streaming Web server: | ||
https://blog.miguelgrinberg.com/post/flask-video-streaming-revisited | ||
|
||
Parking dataset: | ||
https://web.inf.ufpr.br/vri/databases/parking-lot-database/ |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,38 @@ | ||
import keras.backend as K | ||
from keras.engine.topology import Layer, InputSpec | ||
|
||
class LocalResponseNormalization(Layer): | ||
|
||
def __init__(self, n=5, alpha=0.0005, beta=0.75, k=2, **kwargs): | ||
self.n = n | ||
self.alpha = alpha | ||
self.beta = beta | ||
self.k = k | ||
super(LocalResponseNormalization, self).__init__(**kwargs) | ||
|
||
def build(self, input_shape): | ||
self.shape = input_shape | ||
super(LocalResponseNormalization, self).build(input_shape) | ||
|
||
def call(self, x, mask=None): | ||
if K.image_dim_ordering == "th": | ||
_, f, r, c = self.shape | ||
else: | ||
_, r, c, f = self.shape | ||
squared = K.square(x) | ||
pooled = K.pool2d(squared, (self.n, self.n), strides=(1, 1), | ||
padding="same", pool_mode="avg") | ||
if K.image_dim_ordering == "th": | ||
summed = K.sum(pooled, axis=1, keepdims=True) | ||
averaged = self.alpha * K.repeat_elements(summed, f, axis=1) | ||
else: | ||
summed = K.sum(pooled, axis=3, keepdims=True) | ||
averaged = self.alpha * K.repeat_elements(summed, f, axis=3) | ||
denom = K.pow(self.k + averaged, self.beta) | ||
return x / denom | ||
|
||
def get_output_shape_for(self, input_shape): | ||
return input_shape | ||
|
||
def compute_output_shape(self, input_shape): | ||
return input_shape |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,104 @@ | ||
import time | ||
import threading | ||
try: | ||
from greenlet import getcurrent as get_ident | ||
except ImportError: | ||
try: | ||
from thread import get_ident | ||
except ImportError: | ||
from _thread import get_ident | ||
|
||
|
||
class CameraEvent(object): | ||
"""An Event-like class that signals all active clients when a new frame is | ||
available. | ||
""" | ||
def __init__(self): | ||
self.events = {} | ||
|
||
def wait(self): | ||
"""Invoked from each client's thread to wait for the next frame.""" | ||
ident = get_ident() | ||
if ident not in self.events: | ||
# this is a new client | ||
# add an entry for it in the self.events dict | ||
# each entry has two elements, a threading.Event() and a timestamp | ||
self.events[ident] = [threading.Event(), time.time()] | ||
return self.events[ident][0].wait() | ||
|
||
def set(self): | ||
"""Invoked by the camera thread when a new frame is available.""" | ||
now = time.time() | ||
remove = None | ||
for ident, event in self.events.items(): | ||
if not event[0].isSet(): | ||
# if this client's event is not set, then set it | ||
# also update the last set timestamp to now | ||
event[0].set() | ||
event[1] = now | ||
else: | ||
# if the client's event is already set, it means the client | ||
# did not process a previous frame | ||
# if the event stays set for more than 5 seconds, then assume | ||
# the client is gone and remove it | ||
if now - event[1] > 5: | ||
remove = ident | ||
if remove: | ||
del self.events[remove] | ||
|
||
def clear(self): | ||
"""Invoked from each client's thread after a frame was processed.""" | ||
self.events[get_ident()][0].clear() | ||
|
||
|
||
class BaseCamera(object): | ||
thread = None # background thread that reads frames from camera | ||
frame = None # current frame is stored here by background thread | ||
last_access = 0 # time of last client access to the camera | ||
event = CameraEvent() | ||
|
||
def __init__(self): | ||
"""Start the background camera thread if it isn't running yet.""" | ||
if BaseCamera.thread is None: | ||
BaseCamera.last_access = time.time() | ||
|
||
# start background frame thread | ||
BaseCamera.thread = threading.Thread(target=self._thread) | ||
BaseCamera.thread.start() | ||
|
||
# wait until frames are available | ||
while self.get_frame() is None: | ||
time.sleep(0) | ||
|
||
def get_frame(self): | ||
"""Return the current camera frame.""" | ||
BaseCamera.last_access = time.time() | ||
|
||
# wait for a signal from the camera thread | ||
BaseCamera.event.wait() | ||
BaseCamera.event.clear() | ||
|
||
return BaseCamera.frame | ||
|
||
@staticmethod | ||
def frames(): | ||
""""Generator that returns frames from the camera.""" | ||
raise RuntimeError('Must be implemented by subclasses.') | ||
|
||
@classmethod | ||
def _thread(cls): | ||
"""Camera background thread.""" | ||
print('Starting camera thread.') | ||
frames_iterator = cls.frames() | ||
for frame in frames_iterator: | ||
BaseCamera.frame = frame | ||
BaseCamera.event.set() # send signal to clients | ||
time.sleep(0) | ||
|
||
# if there hasn't been any clients asking for frames in | ||
# the last 10 seconds then stop the thread | ||
if time.time() - BaseCamera.last_access > 120: | ||
frames_iterator.close() | ||
print('Stopping camera thread due to inactivity.') | ||
break | ||
BaseCamera.thread = None |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,5 @@ | ||
COLOR_BLACK = (0, 0, 0) | ||
COLOR_BLUE = (255, 0, 0) | ||
COLOR_GREEN = (0, 255, 0) | ||
COLOR_RED = (0, 0, 255) | ||
COLOR_WHITE = (255, 255, 255) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,37 @@ | ||
#!/usr/bin/env python | ||
import os | ||
from importlib import import_module | ||
from flask import Flask, render_template, Response | ||
|
||
# import camera driver | ||
if os.environ.get('CAMERA'): | ||
Camera = import_module('camera_' + os.environ['CAMERA']).Camera | ||
else: | ||
from parking_prediction import Camera | ||
|
||
# Raspberry Pi camera module (requires picamera package) | ||
# from camera_pi import Camera | ||
|
||
app = Flask(__name__) | ||
|
||
@app.route('/') | ||
def index(): | ||
"""Video streaming home page.""" | ||
print("index page") | ||
return render_template('parking_cnn_page.html') | ||
|
||
def gen(camera): | ||
"""Video streaming generator function.""" | ||
print("Gen camera obj") | ||
while True: | ||
frame = camera.get_frame() | ||
yield (b'--frame\r\n'b'Content-Type: image/jpeg\r\n\r\n' + frame + b'\r\n') | ||
|
||
@app.route('/video_feed') | ||
def video_feed(): | ||
print("video feed page") | ||
"""Video streaming route. Put this in the src attribute of an img tag.""" | ||
return Response(gen(Camera()), mimetype='multipart/x-mixed-replace; boundary=frame') | ||
|
||
if __name__ == '__main__': | ||
app.run(host='127.0.0.1', port=62597, threaded=True) |
Oops, something went wrong.