-
Notifications
You must be signed in to change notification settings - Fork 48
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
update docs and add pwm i2c etc. demo
- Loading branch information
Showing
21 changed files
with
491 additions
and
29 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,47 @@ | ||
# MaixPy Using GPIO | ||
|
||
## Introduction | ||
|
||
Using GPIO allows you to control pins for input or output high and low levels, which is commonly used to read signals or output control signals. | ||
|
||
## Using GPIO in MaixPy | ||
|
||
> MaixPy Firmware should > 4.1.2(not include) | ||
First, we need to know which pins and GPIOs the device has. For MaixCAM, each pin corresponds to a GPIO controller, as shown in the figure: | ||
|
||
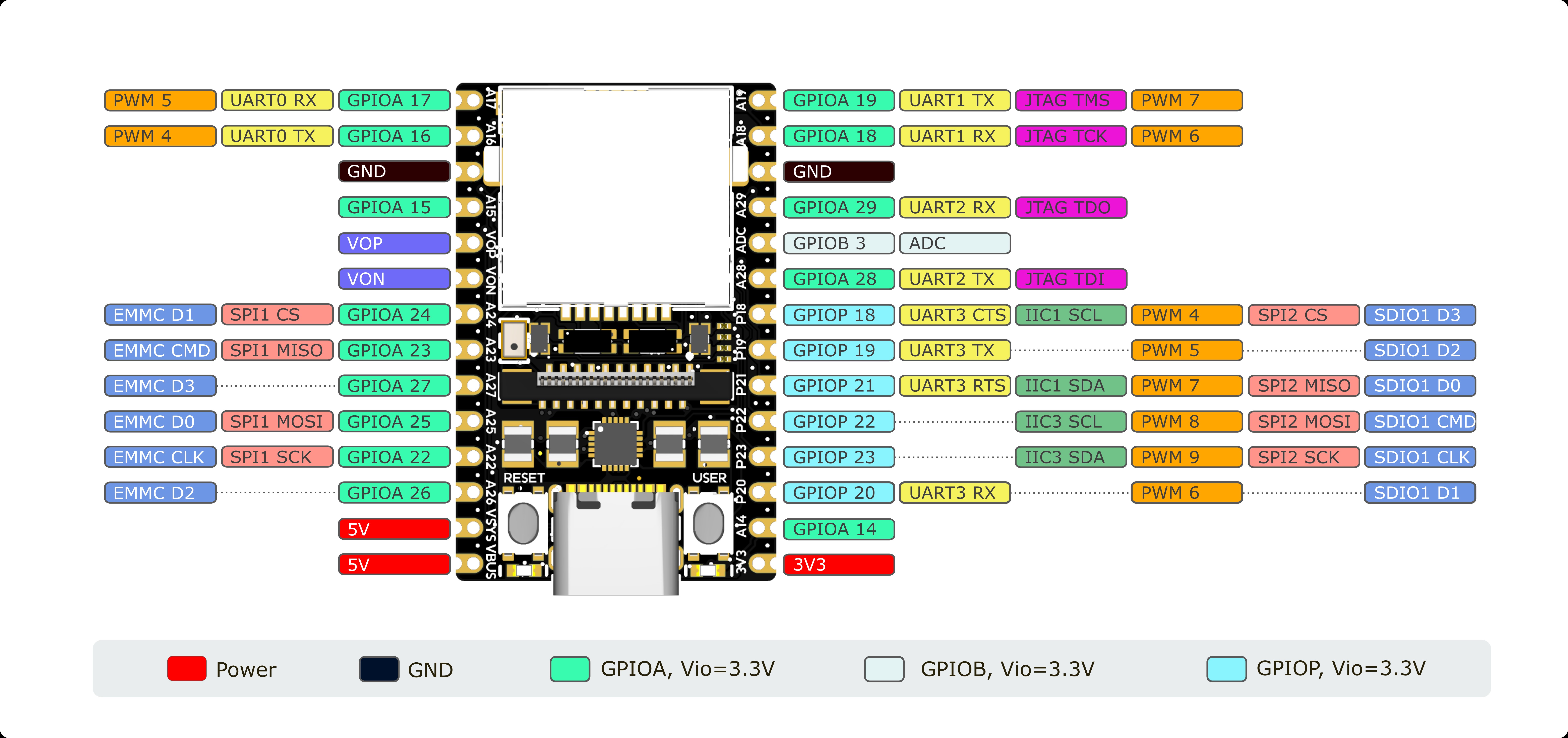 | ||
|
||
It is important to note that pins can be used not only as GPIOs but also for other functions like PWM. Before using them, we need to set the pin function to GPIO. | ||
|
||
For example, on MaixCAM, some pins are already occupied by other functions by default, such as UART0 and WiFi (SDIO1 + A26), so it is not recommended to use them. Other pins can be used, and the A14 pin is connected to the onboard LED, which is used as a system load indicator by default. If initialized, it will automatically disable the system indicator function and can be used as a regular GPIO (note that `A14` can only be used as an output). This way, you can control the LED's on and off state. | ||
|
||
```python | ||
from maix import gpio, pinmap, time | ||
|
||
pinmap.set_pin_function("A14", "GPIOA14") | ||
led = gpio.GPIO("A14", gpio.Mode.OUT) | ||
led.value(0) | ||
|
||
while 1: | ||
led.toggle() | ||
time.sleep_ms(500) | ||
``` | ||
|
||
Here, we first use `pinmap` to set the function of the `A14` pin to `GPIO`. Of course, for `A14`, since it only has the `GPIO` function, it can be omitted. For the sake of generality, other pins may need to be set, so it is set in this example. | ||
|
||
For more APIs, please refer to the [GPIO API Documentation](https://wiki.sipeed.com/maixpy/api/maix/peripheral/gpio.html) | ||
|
||
## GPIO in Input Mode | ||
|
||
```python | ||
from maix import gpio, pinmap, time | ||
|
||
pinmap.set_pin_function("A19", "GPIOA19") | ||
led = gpio.GPIO("A19", gpio.Mode.IN) | ||
|
||
while 1: | ||
print(led.value()) | ||
time.sleep_ms(1) # sleep to make cpu free | ||
``` | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,46 @@ | ||
--- | ||
title: MaixPy Using I2C | ||
--- | ||
|
||
For MaixCAM, due to the tight pin resource constraints, the I2C pins overlap with the WiFi module (SDIO1), so you can only use either WiFi or hardware I2C, but not both. | ||
|
||
Therefore, it is recommended to use IO emulation for I2C. | ||
|
||
> Note: Requires MaixPy image and firmware > 4.1.2 (not included) | ||
## IO Emulation I2C | ||
|
||
To emulate `I2C`, you only need to use the `GPIO` functionality for the pins. On `MaixCAM`, the emulated `I2C` is fixed to pins `A15` (`SCL`) and `A27` (`SDA`), with the `i2c` number being `5`. To use it, simply: | ||
|
||
```python | ||
from maix import i2c, pinmap | ||
|
||
# pinmap.set_pin_function("A15", "GPIOA23") | ||
# pinmap.set_pin_function("A27", "GPIOA24") | ||
|
||
bus1 = i2c.I2C(5, i2c.Mode.MASTER) | ||
slaves = bus1.scan() | ||
print("find slaves:", slaves) | ||
``` | ||
|
||
More API visit [i2c API doc](https://wiki.sipeed.com/maixpy/api/maix/peripheral/i2c.html) | ||
|
||
## Hardware I2C | ||
|
||
As mentioned above, for `MaixCAM`, hardware `I2C` and `WiFi` are mutually exclusive. If you must use hardware `I2C`, you need to disable `WiFi` and use the `pinmap` module to set the pin functions to `I2C`, then use the `maix.i2c` module to operate. | ||
> TODO: Provide a method to disable WiFi (requires disabling the WiFi driver in the system, which is relatively complex) | ||
Hardware `I2C` and its correspond pin see: | ||
|
||
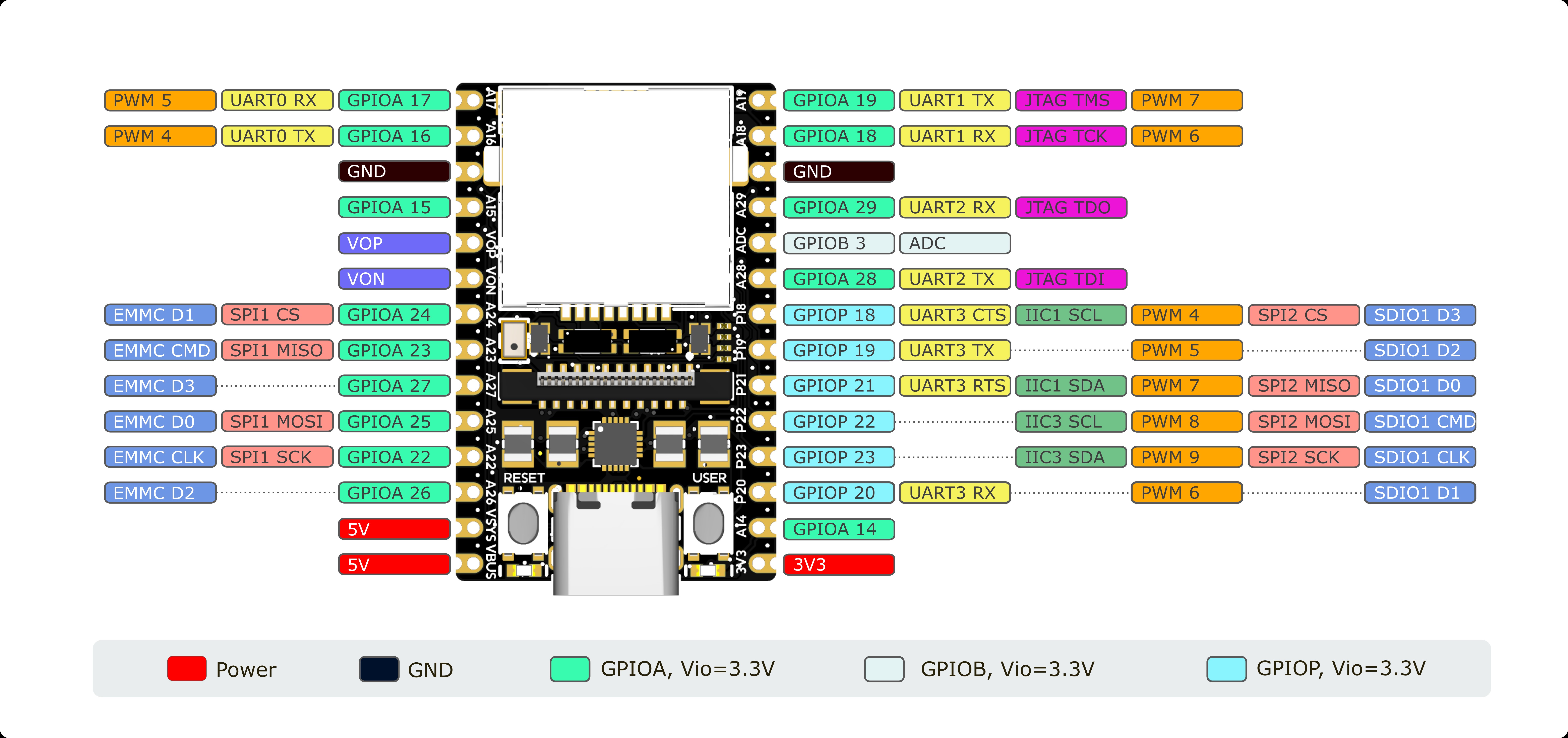 | ||
|
||
```python | ||
from maix import i2c, pinmap | ||
|
||
pinmap.set_pin_function("P18", "I2C1_SCL") | ||
pinmap.set_pin_function("P21", "I2C1_SDA") | ||
|
||
bus1 = i2c.I2C(1, i2c.Mode.MASTER) | ||
slaves = bus1.scan() | ||
print("find slaves:", slaves) | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,48 @@ | ||
# Using PWM in MaixPy | ||
|
||
## Introduction | ||
|
||
To use `PWM` in MaixPy (v4), first set the pin function to `PWM` using `pinmap`. | ||
|
||
Each `PWM` corresponds to a specific pin, as shown in the pin diagram of MaixCAM: | ||
|
||
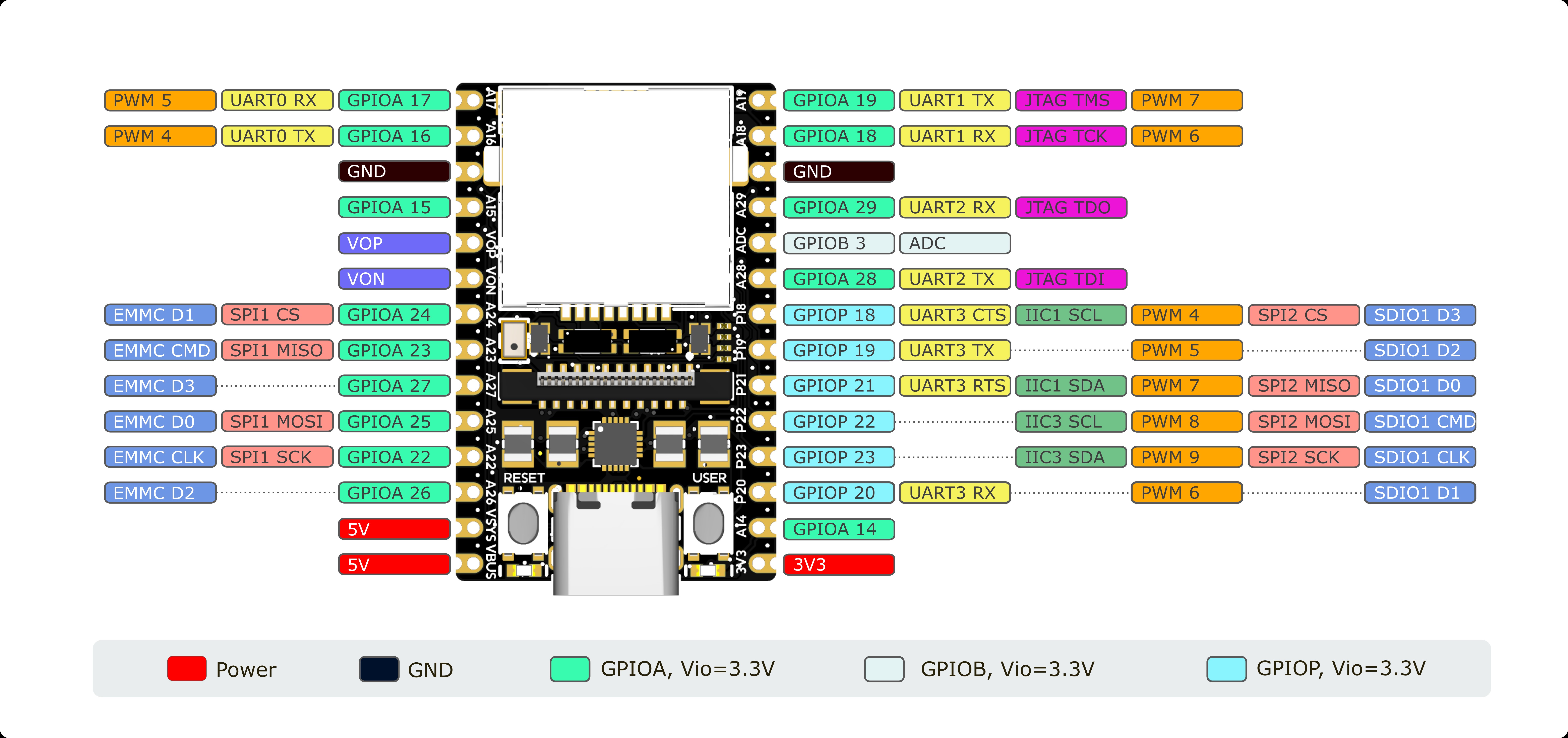 | ||
|
||
We recommend using `PWM6` and `PWM7`. | ||
|
||
For `MaixCAM`, since `WiFi` uses all pins of `SDIO1`, `PWM4~9` can only be used alternatively with `WiFi`. | ||
> TODO: Provide a method to disable WiFi (requires disabling the WiFi driver in the system, which is quite complex) | ||
|
||
## Using PWM to Control a Servo in MaixPy | ||
|
||
Here we take controlling a servo as an example, using `PWM7` and the `A19` pin of `MaixCAM`: | ||
|
||
```python | ||
from maix import pwm, time, pinmap | ||
|
||
SERVO_PERIOD = 50 # 50Hz 20ms | ||
SERVO_MIN_DUTY = 2.5 # 2.5% -> 0.5ms | ||
SERVO_MAX_DUTY = 12.5 # 12.5% -> 2.5ms | ||
|
||
# Use PWM7 | ||
pwm_id = 7 | ||
# !! set pinmap to use PWM7 | ||
pinmap.set_pin_function("A19", "PWM7") | ||
|
||
def angle_to_duty(percent): | ||
return (SERVO_MAX_DUTY - SERVO_MIN_DUTY) * percent / 100.0 + SERVO_MIN_DUTY | ||
|
||
out = pwm.PWM(pwm_id, freq=SERVO_PERIOD, duty=angle_to_duty(0), enable=True) | ||
|
||
for i in range(100): | ||
out.duty(angle_to_duty(i)) | ||
time.sleep_ms(100) | ||
|
||
for i in range(100): | ||
out.duty(angle_to_duty(100 - i)) | ||
time.sleep_ms(100) | ||
``` | ||
|
||
This code controls the servo to rotate from the minimum angle to the maximum angle and then back to the minimum angle. | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,6 @@ | ||
--- | ||
title: MaixPy ues SPI | ||
--- | ||
|
||
TODO: | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,24 @@ | ||
# Using Watchdog Timer in MaixPy | ||
|
||
## Introduction | ||
|
||
To prevent program issues, a watchdog timer (WDT) is often used to automatically restart the system when the program encounters a problem. | ||
|
||
The principle is that there is a countdown timer that we need to periodically reset within the program logic (also called "feeding the dog"). If our program gets stuck and fails to reset the countdown timer, the hardware will trigger a system reboot when the timer reaches 0. | ||
|
||
## Using WDT in MaixPy | ||
|
||
```python | ||
from maix import wdt, app, time | ||
|
||
w = wdt.WDT(0, 1000) | ||
|
||
while not app.need_exit(): | ||
w.feed() | ||
# Here, sleep operation is our task | ||
# 200 ms is normal; if it exceeds 1000 ms, it will cause a system reset | ||
time.sleep_ms(200) | ||
``` | ||
|
||
This code sets up a watchdog timer that requires feeding every 1000 ms. If the program fails to feed the watchdog within this period, the system will reset. | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,53 @@ | ||
--- | ||
title: MaixPy 使用 GPIO | ||
--- | ||
|
||
## 简介 | ||
|
||
使用 GPIO 可以控制引脚输入或者输出高低电平,用来读取信号或者输出控制信号,十分常用。 | ||
|
||
|
||
## MaixPy 中使用 GPIO | ||
|
||
> MaixPy 固件版本应该 > 4.1.2(不包含) | ||
首先我们需要知道设备有哪些引脚和 GPIO,对于 MaixCAM 每个引脚都对应了一个 GPIO 控制器,如图: | ||
|
||
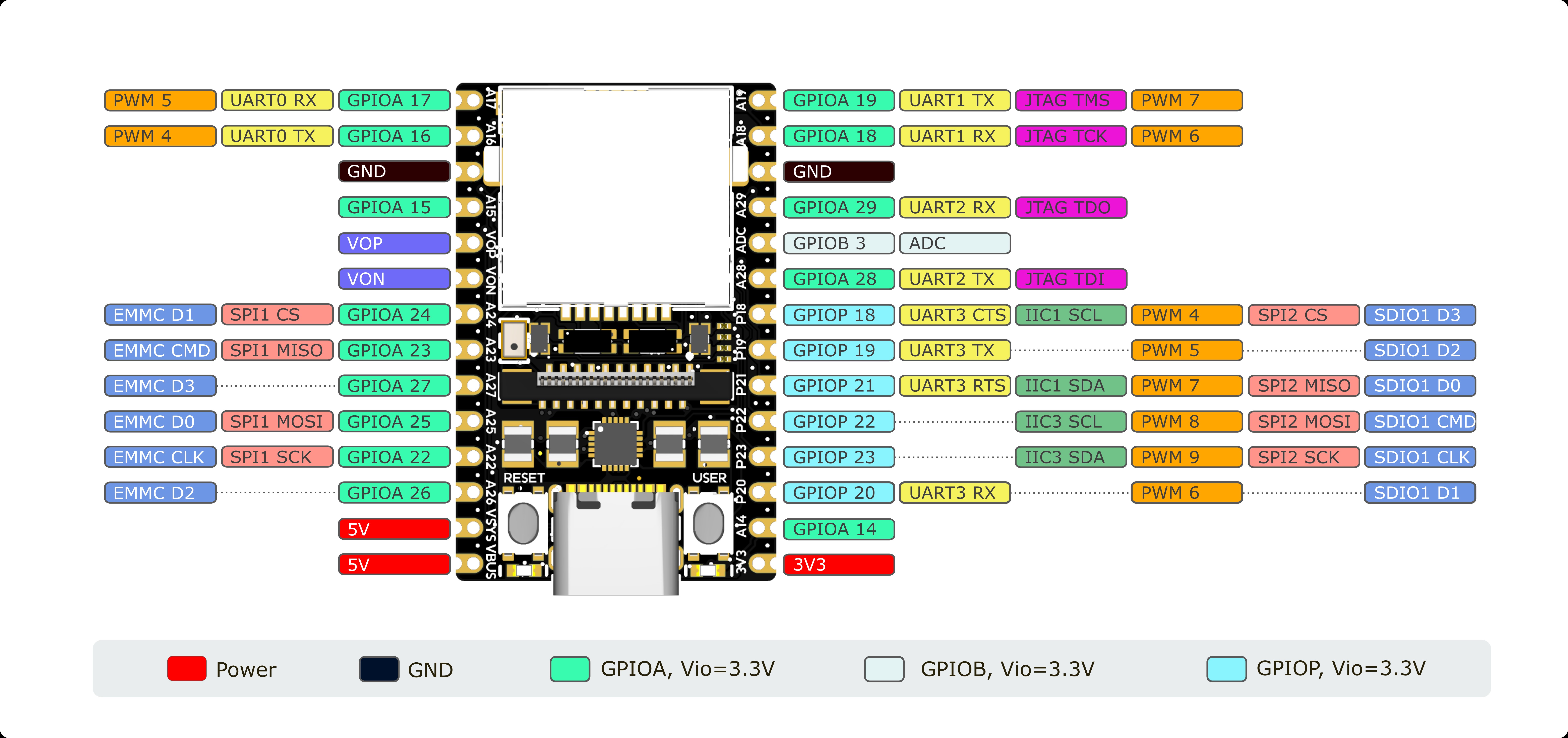 | ||
|
||
需要注意的是,引脚除了作为 GPIO 使用,还能用作其它功能比如 PWM 使用,使用前我们需要设置一下引脚的功能为 GPIO。 | ||
|
||
比如在 MaixCAM 上有些引脚默认已经被其它功能占用,比如 UART0, WiFi(SDIO1 + A26), 不建议使用它们,其它的可以使用,另外 A14 引脚连接到了板载的 LED,默认是作为系统的负载提示灯,如果初始化它会自动取消系统提示灯功能作为普通 GPIO 被使用(注意`A14`只能作为输出),这样你就能控制这颗 LED 的亮灭了。 | ||
|
||
```python | ||
from maix import gpio, pinmap, time | ||
|
||
pinmap.set_pin_function("A14", "GPIOA14") | ||
led = gpio.GPIO("A14", gpio.Mode.OUT) | ||
led.value(0) | ||
|
||
while 1: | ||
led.toggle() | ||
time.sleep_ms(500) | ||
``` | ||
|
||
这里先使用`pinmap`设置了`A14`引脚的功能为`GPIO`,当然,对于`A14`因为只有`GPIO`功能,可以不设置,为了程序通用起见,其它引脚可能需要设置,所以这里例程设置了。 | ||
|
||
|
||
更多 API 请看 [GPIO API 文档](https://wiki.sipeed.com/maixpy/api/maix/peripheral/gpio.html) | ||
|
||
|
||
## GPIO 作为输入模式 | ||
|
||
```python | ||
from maix import gpio, pinmap, time | ||
|
||
pinmap.set_pin_function("A19", "GPIOA19") | ||
led = gpio.GPIO("A19", gpio.Mode.IN) | ||
|
||
while 1: | ||
print(led.value()) | ||
time.sleep_ms(1) # sleep to make cpu free | ||
``` | ||
|
||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,52 @@ | ||
--- | ||
title: MaixPy 使用 I2C | ||
--- | ||
|
||
对于 MaixCAM,由于引脚资源比较紧张,引出的 I2C 引脚和 WiFi 模块(SDIO1)重合了,所以 WiFi 和硬件 I2C 只能二选一使用。 | ||
|
||
所以推荐使用 IO 模拟 I2C。 | ||
|
||
> 注意需要 MaixPy 镜像和固件 > 4.1.2(不包含) | ||
## IO 模拟 I2C | ||
|
||
模拟 `I2C` 只需要引脚使用 `GPIO` 功能即可,在 `MaixCAM` 上,模拟 `I2C` 被固定在引脚`A15`(`SCL`),`A27`(`SDA`),并且`i2c`编号为`5`, 要使用,只需要: | ||
|
||
```python | ||
from maix import i2c, pinmap | ||
|
||
# pinmap.set_pin_function("A15", "GPIOA23") | ||
# pinmap.set_pin_function("A27", "GPIOA24") | ||
|
||
bus1 = i2c.I2C(5, i2c.Mode.MASTER) | ||
slaves = bus1.scan() | ||
print("find slaves:", slaves) | ||
|
||
``` | ||
|
||
更多 API 看 [i2c API 文档](https://wiki.sipeed.com/maixpy/api/maix/peripheral/i2c.html) | ||
|
||
|
||
## 硬件 I2C | ||
|
||
如上面所说, 对于 `MaixCAM` 硬件 `I2C` 和 `WiFi` 只能二选一,如果一定要用,需要禁用`WiFi`,使用`pinmap`模块设置引脚功能为 `I2C`,在使用`maix.i2c`模块操作。 | ||
> TODO: 提供禁用 WiFi 的方法(需要系统里面禁用掉 WiFi 驱动,比较复杂) | ||
硬件`I2C`及对应的引脚看图: | ||
|
||
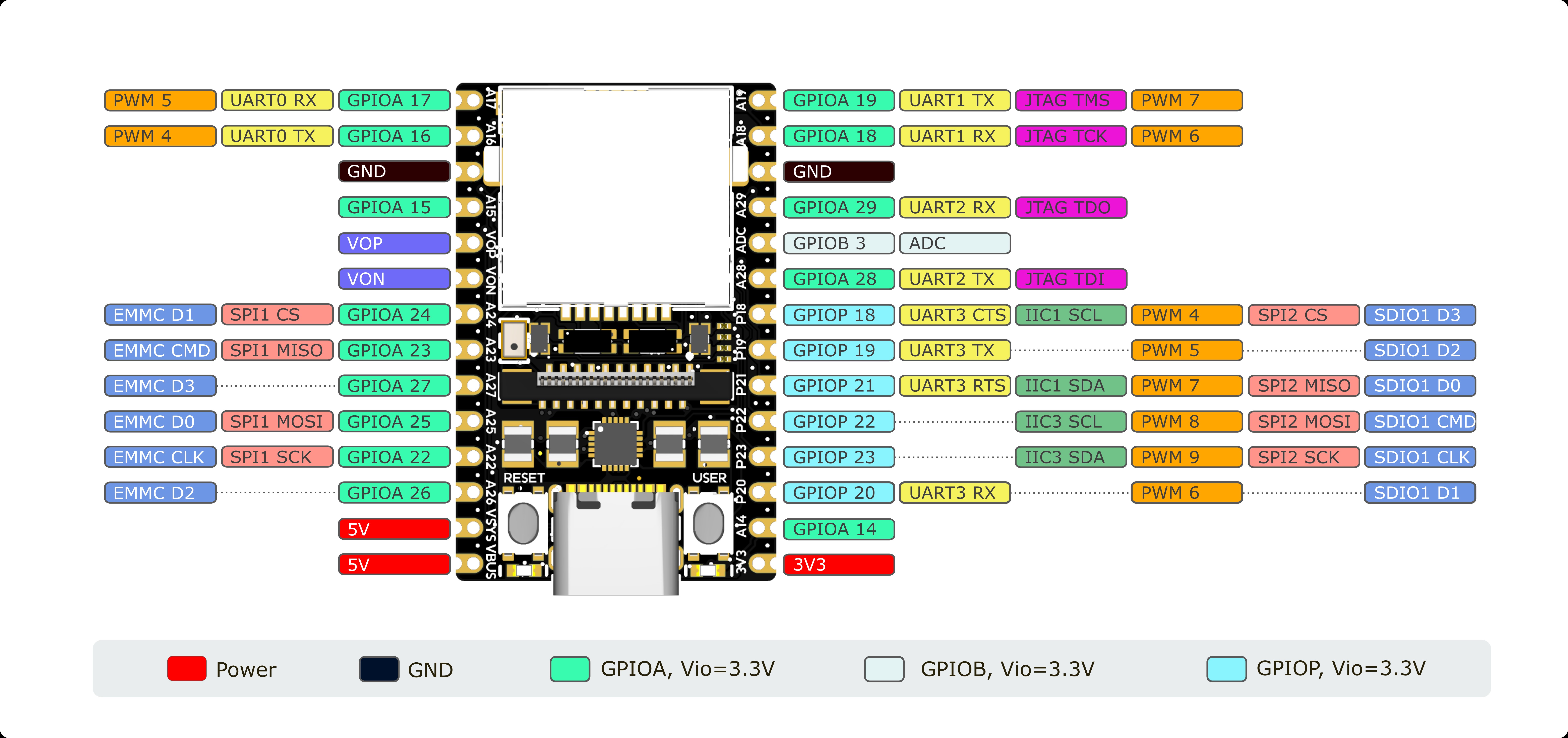 | ||
|
||
```python | ||
from maix import i2c, pinmap | ||
|
||
pinmap.set_pin_function("P18", "I2C1_SCL") | ||
pinmap.set_pin_function("P21", "I2C1_SDA") | ||
|
||
bus1 = i2c.I2C(1, i2c.Mode.MASTER) | ||
slaves = bus1.scan() | ||
print("find slaves:", slaves) | ||
|
||
``` | ||
|
||
|
||
|
Oops, something went wrong.